The "git rebase invalid upstream" error occurs when you attempt to rebase onto a branch or commit that does not exist or is not reachable from the current branch, often indicating a typo or incorrect reference.
Here's how you might encounter the error:
git rebase invalid-branch-name
Understanding Git Rebase
What is `git rebase`?
`git rebase` is a command in Git that allows you to integrate changes from one branch into another by moving or combining a sequence of commits to a new base commit. It is often used in scenarios where you want to maintain a clean and linear project history, especially when collaborating in teams.
Example: Consider you have two branches: `main` and `feature`. If you make several commits in `feature` and then decide to rebase it onto `main`, Git will reapply your `feature` changes on top of the most recent `main` commits. This results in a clearer project history compared to merging.
Why Use Rebase?
Rebasing offers several advantages:
- Cleaner History: It helps maintain a linear commit history, making it easier to navigate and understand.
- Reduced Merge Conflicts: Frequent rebasing onto the main branch can help resolve conflicts early, reducing the risk of larger conflicts later on.
When to Rebase: It's best used before merging a feature branch into the main branch to ensure that your changes are up to date with the latest commits. However, be cautious when rebasing public branches to avoid disrupting collaborations.
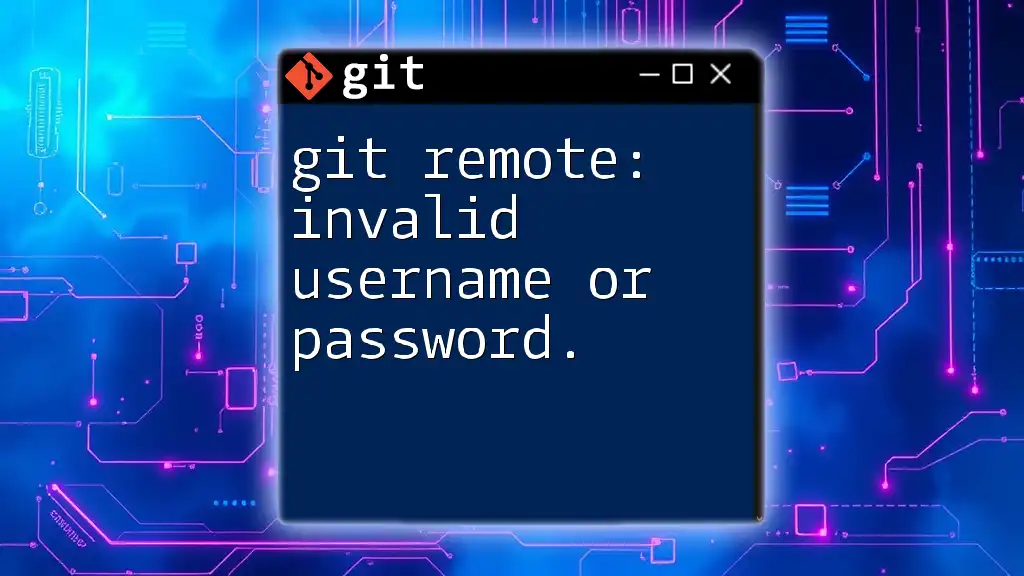
Common Errors in Git Rebase
Overview of Possible Errors
While using `git rebase`, many users encounter various errors. One of the most common and troubling ones is the "git rebase invalid upstream" error.
What Does "Invalid Upstream" Mean?
Invalid upstream refers to situations where the branch upon which you are trying to rebase does not exist or is incorrectly referenced. This could happen for several reasons, such as:
- You're trying to rebase onto a branch that hasn't been fetched yet.
- The upstream branch specified is mistyped or does not exist.
- Your current branch isn't tracking the correct upstream branch.
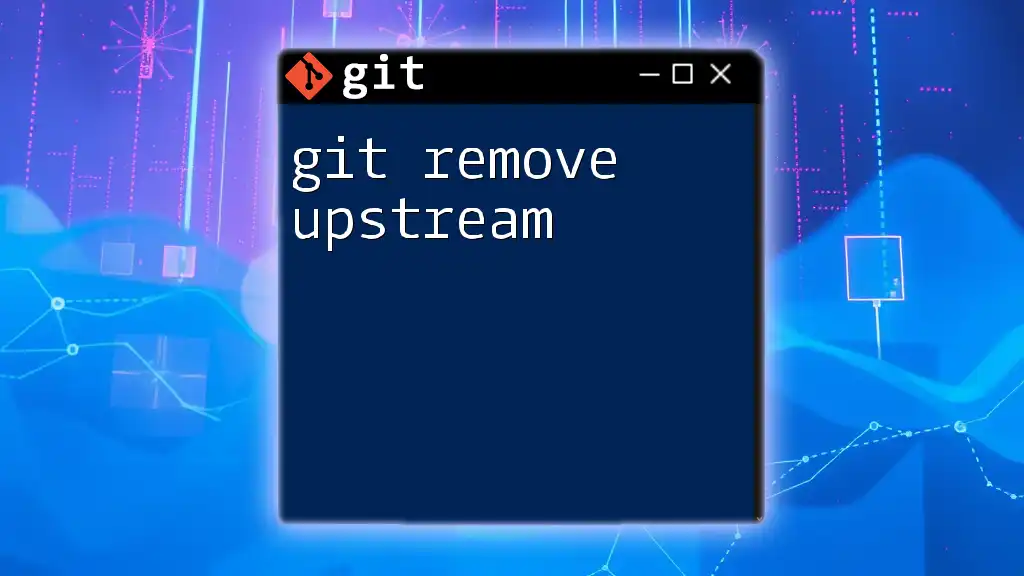
How to Troubleshoot "git rebase invalid upstream" Error
Checking Your Current Branch
Before proceeding to fix the error, it’s essential to verify the branch you’re currently on. You can use the following command:
git branch
This command lists all the local branches, highlighting the one you’re currently on. If your working branch is not what you expected, it might lead to the "invalid upstream" error.
Verifying the Upstream Branch
Next, you need to ensure that the upstream branch exists and is correctly set up. You can accomplish this with:
git remote -v
This command lists all remote repositories and their associated branches. If your upstream branch is missing from this list, it could be the reason behind the "invalid upstream" error.
Example: After running the command, you might see something like:
origin https://github.com/username/repo.git (fetch)
origin https://github.com/username/repo.git (push)
If your intended upstream branch isn’t displayed, you need to rectify this.
Correcting Your Upstream Reference
If you’ve determined that your upstream branch is inaccurate or missing, you can use the following command to set or update your upstream reference:
git branch --set-upstream-to <branch>
Replace `<branch>` with the correct upstream branch name.
Example: If you're trying to rebase your feature branch onto the main branch, the command would look like this:
git branch --set-upstream-to origin/main
By executing this command, you ensure your local branch tracks the correct upstream branch.
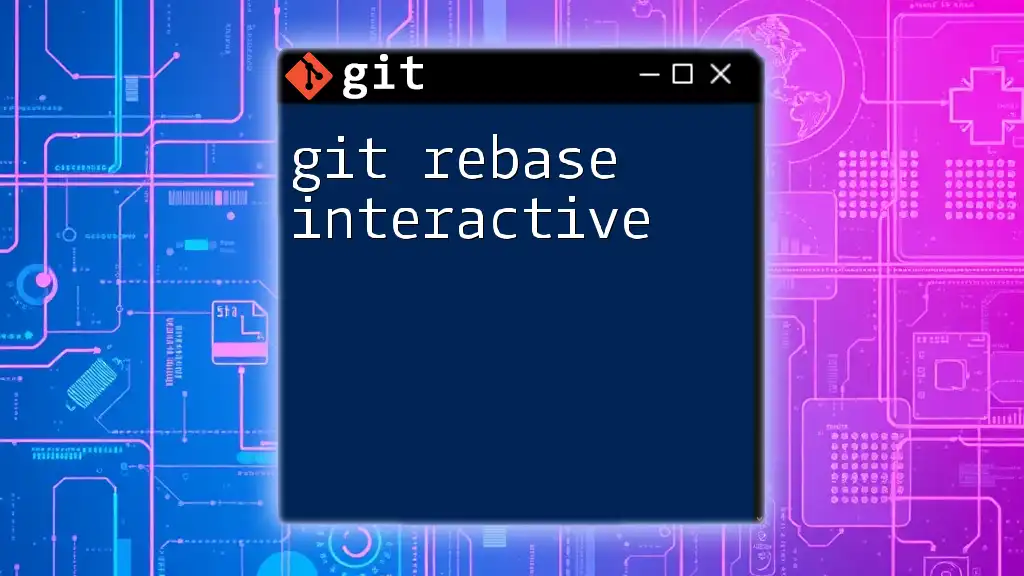
Practical Steps to Fix the "Invalid Upstream" Error
Resetting to the Correct Branch
In cases where your current branch isn’t tracking the right upstream, switching to the correct branch is vital. The commands below will help restore the correct working branch:
git checkout <correct-branch>
git rebase <upstream-branch>
For instance, if your feature branch should base onto `main`, you would execute:
git checkout feature
git rebase main
This sequence effectively resolves the upstream reference.
Creating and Using a Temporary Branch
If you are uncertain about making changes directly, consider creating a temporary branch. This allows you to keep your workflow intact while resolving potential issues:
git checkout -b temp-branch
git rebase <valid-upstream-branch>
For example:
git checkout -b temp-feature
git rebase main
Creating this temporary branch ensures that your changes are isolated while you debug and resolve issues.
Fetching and Updating Remote Branches
Often, the issue arises from a lack of up-to-date information about remote branches. Using the following command updates all local references to remote branches:
git fetch --all
This command retrieves all branches from the remote repository and updates your local reference point. Keeping your local repository synchronized with remote repositories can significantly decrease the likelihood of encountering the "invalid upstream" error in the future.
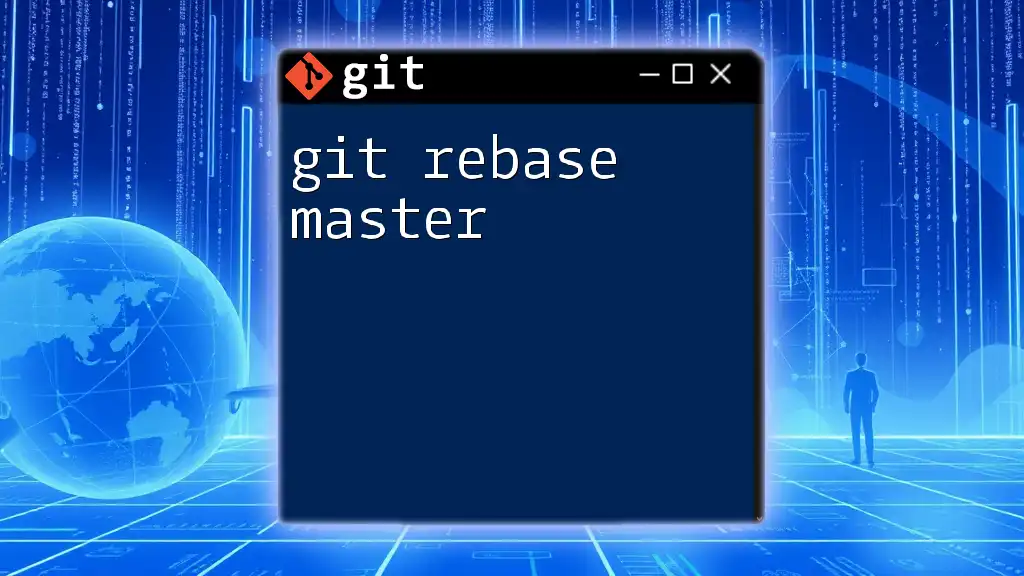
Best Practices to Avoid Future Errors
Establishing a Consistent Branching Strategy
A well-defined branching strategy is crucial for successful collaboration and reducing errors. Adopting approaches like feature branching or semantic versioning can help maintain clarity and organization in your project's commit history.
Example: Using branches named intuitively (like `feature/add-login`, `bugfix/fix-header`) can dramatically lower confusion in tracking upstream references.
Regularly Syncing with Remote Repositories
Frequent synchronization with remote repositories helps keep your local branches updated. Using the `git pull` command is one way, but be sure to understand when to use it versus `git fetch`.
While `git pull` includes fetching and merging, `git fetch` simply updates your remote branches without integrating changes. This practice can be more conservative and helps avoid conflicts during rebasing.
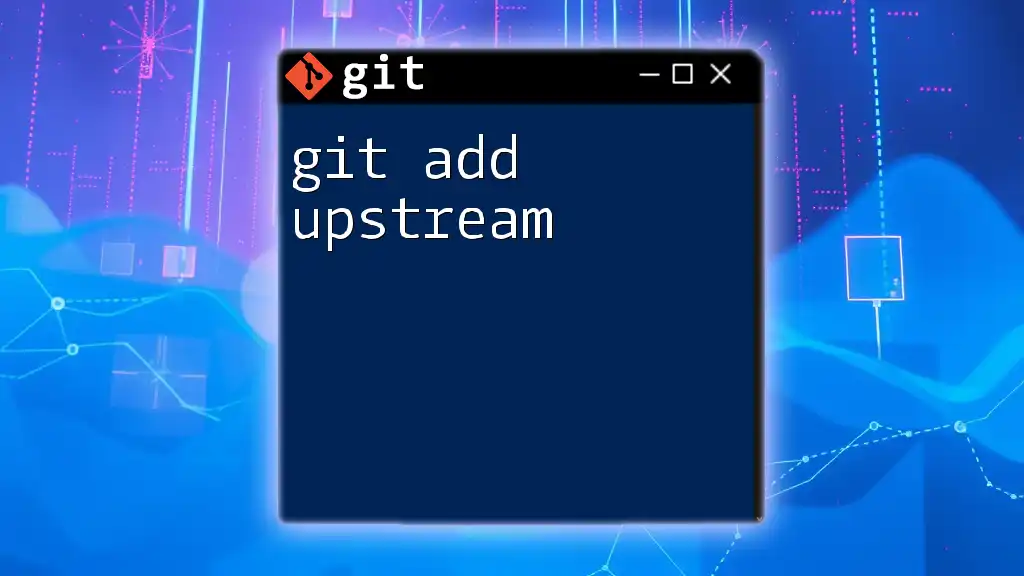
Conclusion
In summary, dealing with the "git rebase invalid upstream" error can be daunting, but understanding Git's architecture and working through troubleshooting steps can alleviate confusion. By maintaining a consistent branching strategy and regularly syncing with remote repositories, you can minimize future errors and enhance your workflow.
Remember, practice makes perfect; don't hesitate to explore the commands and procedures discussed in this guide. Mastering Git will ultimately aid you in managing your projects more effectively.
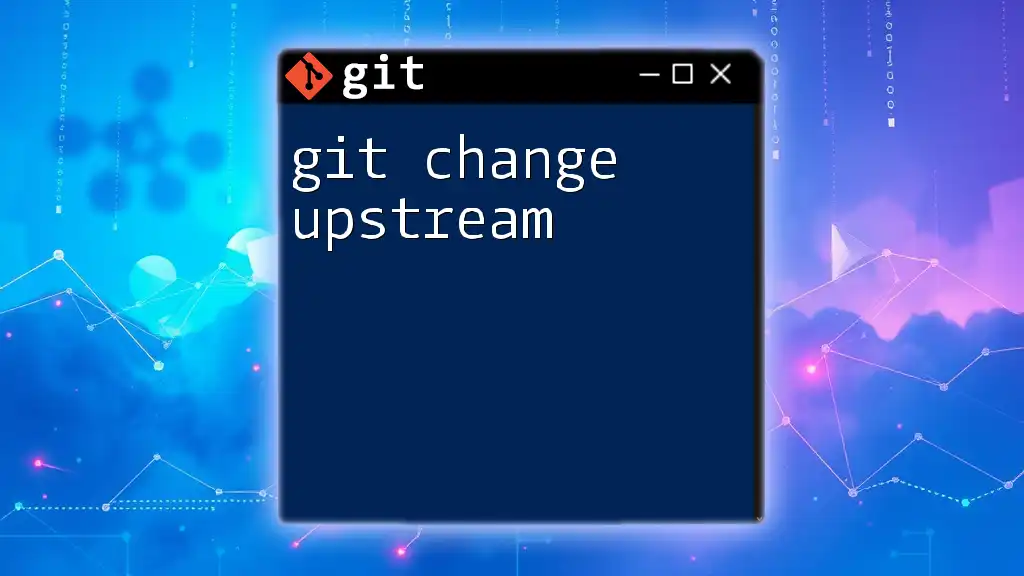
Resources and Further Reading
For deeper insights, consider looking into:
- The official Git documentation.
- Advanced Git tutorials and books that cover these aspects extensively.
- Online courses to solidify your command of Git.
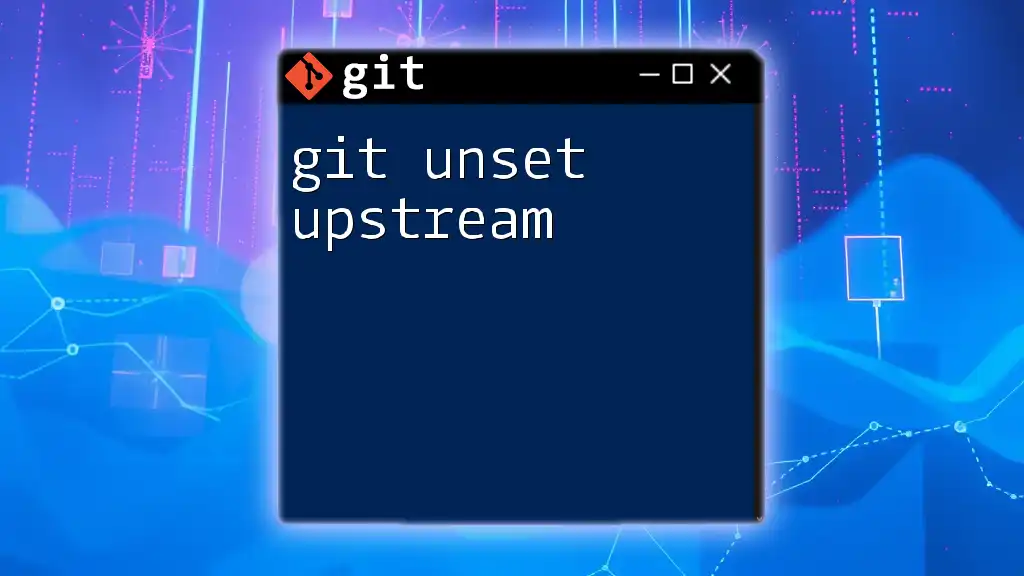
Call to Action
If you found this guide helpful, consider subscribing for more Git tips and tricks! Feel free to leave your feedback or questions about Git rebase issues and troubleshooting strategies. Your input will help us create better resources for the community.