`git rebase interactive` is a powerful Git command that allows you to edit, rearrange, or squash commits in your branch's history to create a cleaner and more organized commit log.
Here’s a code snippet to start an interactive rebase for the last 5 commits:
git rebase -i HEAD~5
What is Git Rebase?
Git rebase is a powerful command in the version control system that allows developers to move or combine a sequence of commits to a new base commit. Unlike merging, which creates an additional merge commit and maintains the history of branches, rebasing rewrites the commit history to create a linear progression. This can make the project history cleaner and easier to read.
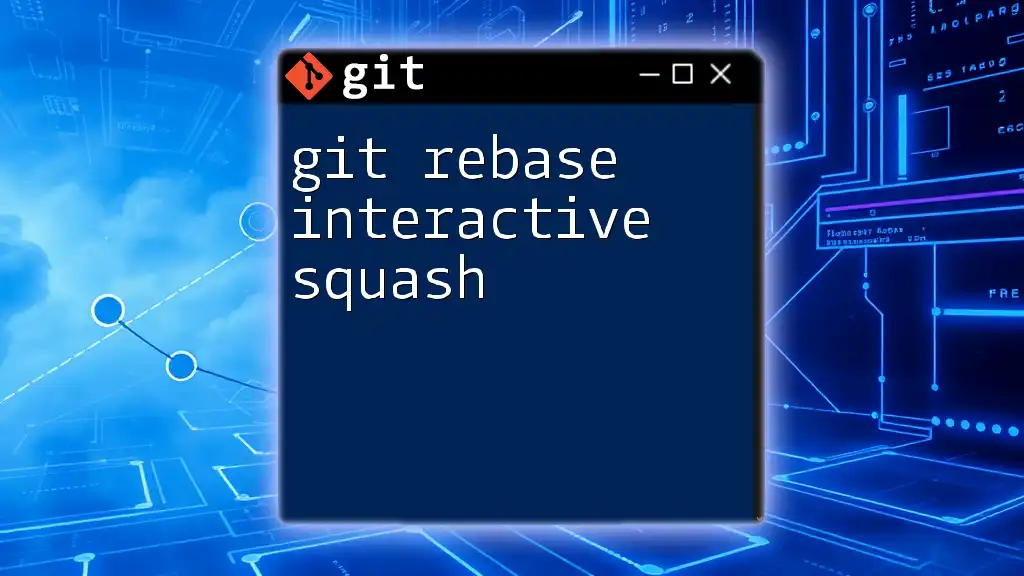
Why Use Interactive Rebase?
Interactive rebasing is particularly beneficial for cleaning up commit history before merges, especially when multiple commits have been made that are logically related. By using interactive rebase, developers can:
- Organize and simplify their commit history.
- Modify commit messages for clarity.
- Squash multiple commits together to reflect a single logical change.
- Remove unnecessary commits that do not bear significance.
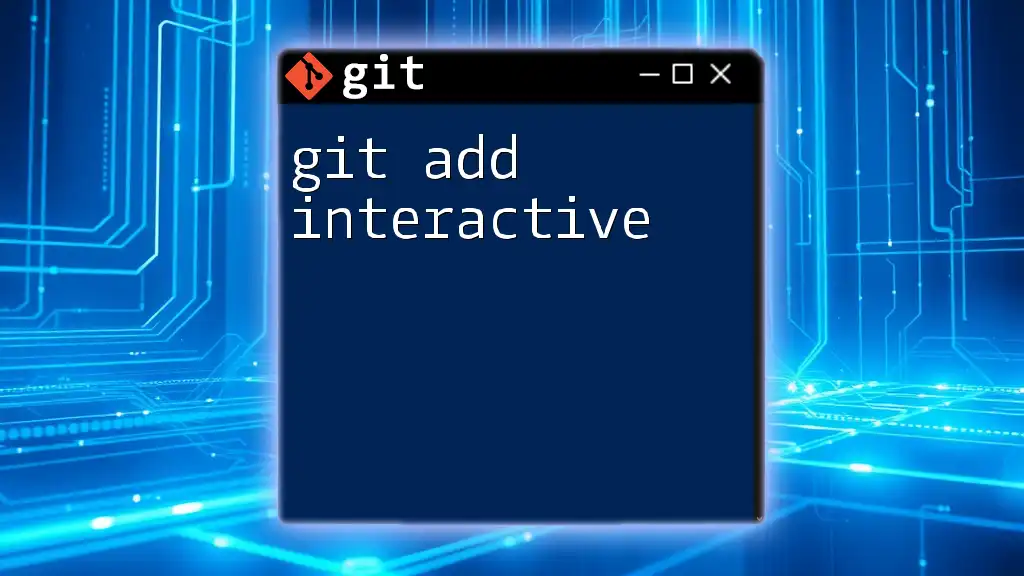
Getting Started with Interactive Rebase
Prerequisites
Before diving into interactive rebasing, it's important to have a basic understanding of Git commands and a configured Git environment. You should be familiar with commands such as `git commit`, `git push`, and `git checkout`.
How to Initiate an Interactive Rebase
To begin an interactive rebase session, you can use the command `git rebase -i <commit>`, where `<commit>` is the reference to a commit before the series you want to interact with. This reference can be a commit hash, branch name, or relative pointer (like `HEAD~3` for the last three commits).
For example, if you want to rebase the last three commits on your current branch, you would run:
git rebase -i HEAD~3
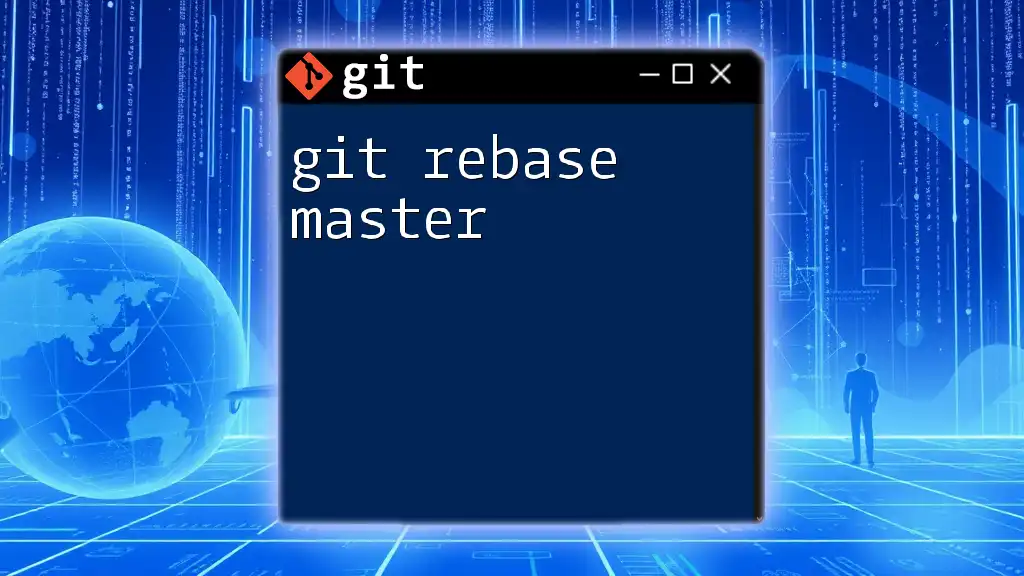
The Interactive Rebase Interface
Understanding the Editor
Upon executing the `git rebase -i` command, your designated text editor will open up with a list of the commits to be rebased. Each line will start with the word `pick`, followed by the commit hash and message.
Editing Commit Instructions
In the interactive rebase interface, you can modify the commands to dictate what should happen with each commit:
- pick: This tells Git to keep the commit as is.
- reword: Use this to change the commit message while keeping the commit itself unchanged.
- edit: This will stop at the commit and allow you to modify its content (files) if desired.
- squash: This combines the commit with the previous one, merging their changes and commit message.
- fixup: Similar to squash, but it will discard the commit message of the commit being combined.
- drop: This removes the commit entirely.
For an example, consider the following commit list:
pick 1234567 Fix bug in the feature
pick 2345678 Update documentation
pick 3456789 Add tests for new feature
If you want to squash the last two commits into the first one, you would change it to:
pick 1234567 Fix bug in the feature
squash 2345678 Update documentation
squash 3456789 Add tests for new feature
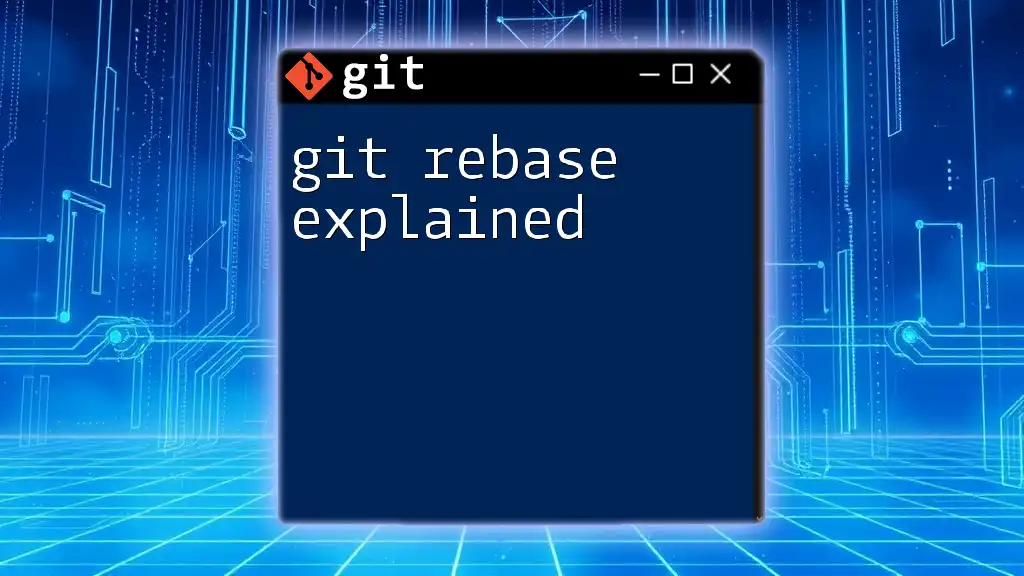
Common Use Cases for Interactive Rebase
Cleaning Up Commit History
One of the main reasons to use git rebase interactive is to consolidate multiple related commits into one. This is particularly useful when numerous minor changes have been made that are part of a larger update. By squashing commits, the result is a single, cohesive commit that reflects the entire change.
Example Scenario
Consider a scenario where you have accidentally pushed several commits that were part of implementing a feature. Instead of pushing cluttered history, you can simplify it. After initiating the interactive rebase as demonstrated above, you can then squash the commits related to that feature.
Editing Commit Messages
Sometimes commit messages aren't as clear as they should be. You may discover that your commit message lacks detail or context after reflecting on it later. Using the `reword` command allows you to refine commit messages for clarity.
An instance could look like this in your editor:
pick 1234567 Add feature implementation
reword 2345678 Update documentation for the feature
When you save the changes, Git will prompt you to edit the message for the second commit, enabling you to add clarity.
Removing Unwanted Commits
There may be times when a commit is deemed unnecessary, whether on the basis of experimentation or accidental inclusion. The `drop` command is used to eliminate a commit from the history. Simply replace the initial keyword with `drop` to remove that commit.
drop 2345678 Experimental changes to be discarded
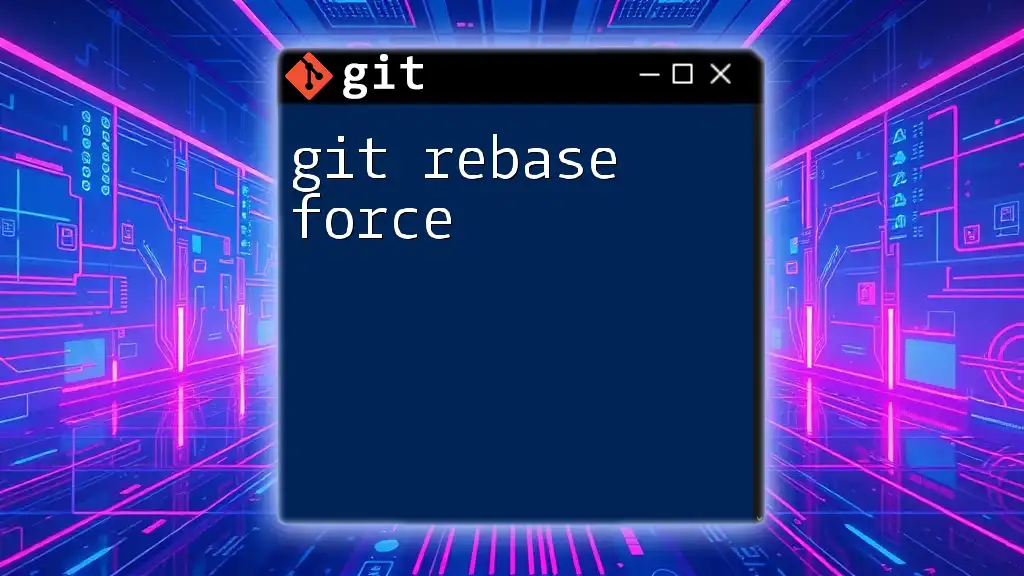
Potential Risks and How to Manage Them
Understanding the Risks of Rebasing
Rebasing can be a double-edged sword; while it helps tidy up commit history, it may lead to lost commits if not handled carefully. Each time you rebase, you are rewriting commit history, which can have implications if others are also working on the same branch.
Conflicts During Rebase and Their Resolutions
Conflicts may arise during a rebase when the changes being applied do not align with the current state of the branch. Git will pause at the conflicting commit, allowing you to resolve the changes manually. After fixing the conflicts, you will run:
git add <file>
git rebase --continue
Best Practices for Safe Rebasing
- Work on a Separate Branch: Always perform rebasing on a branch that is not shared or collaborating with others.
- Ensure Changes are Backed Up: Use `git reflog` to reference the previous state of your commits if rebasing goes awry.
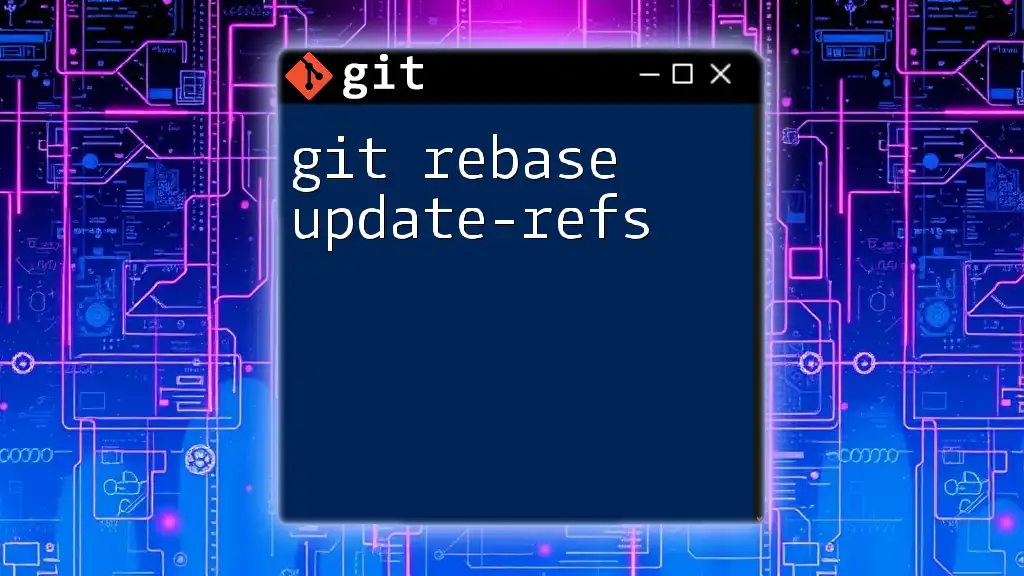
Conclusion
Incorporating git rebase interactive into your Git workflow can significantly enhance your commit history, making it cleaner and more intuitive. As you continue to develop your skills, don’t forget to practice these techniques regularly to reinforce your understanding and application of interactive rebasing.
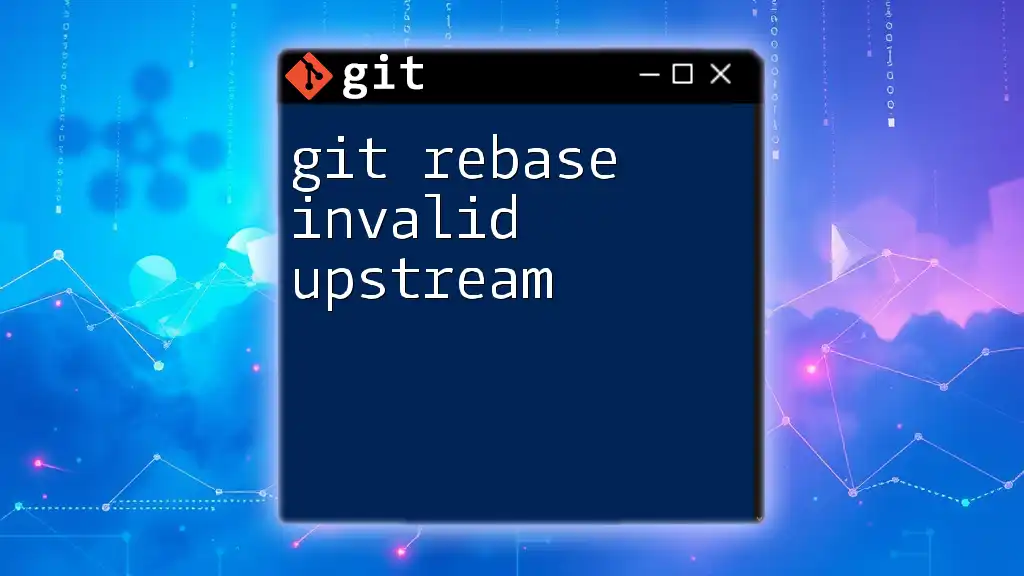
Further Learning Resources
To further enhance your understanding, consider exploring the official Git documentation, which provides in-depth information about all Git commands. Additionally, various online platforms provide tutorials and projects for hands-on practice, reinforcing your skills in using Git effectively.
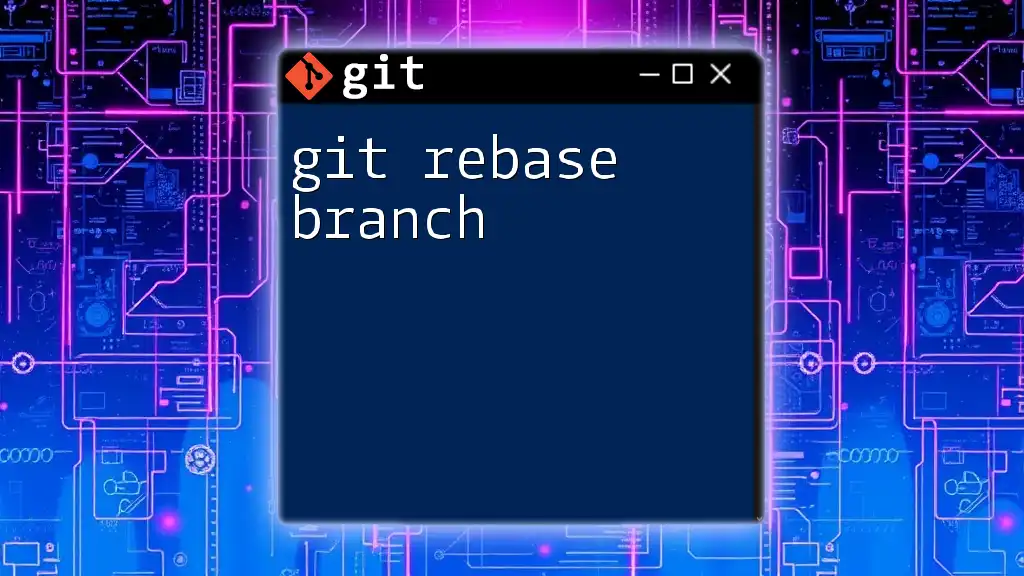
FAQs about Git Interactive Rebase
What happens if my rebase conflicts with upstream changes?
If your rebase encounters upstream changes, you will need to resolve conflicts before completing the rebase process. Git will provide information on conflicting files, allowing you to make necessary corrections.
Is interactive rebase suitable for public/shared branches?
It’s generally not recommended to use rebase on public/shared branches since it rewrites commit history. Instead, preserve the commit history of shared branches with merges.
Can I cancel an ongoing interactive rebase?
Yes, if you find that you need to abort the ongoing interactive rebase, simply run:
git rebase --abort
This will revert your branch to the state it was in before the rebase began.