The command `git rebase -i master` allows you to interactively rebase your current branch on top of the latest commits in the `master` branch, enabling you to modify, reorder, or squash commits as needed.
git rebase -i master
What is Git Rebase?
Git rebase is a powerful tool in version control that fundamentally exists to make your commit history cleaner and more linear. Unlike merging, which preserves the branching history between commits, rebasing re-applies commits from the current branch onto another branch, such as `master`. This approach simplifies your project history, making it easier to understand and manage.
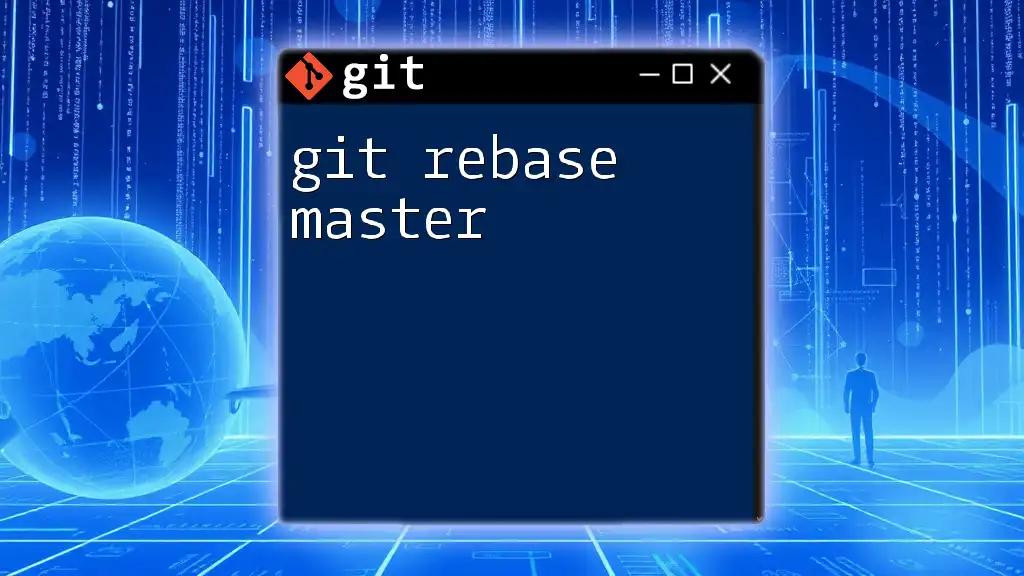
Importance of Keeping Commit History Clean
Maintaining a clean commit history is crucial, especially in a collaborative environment. A well-structured history allows team members and future contributors to follow project changes without confusion.
Rebasing is particularly useful when you want to:
- Simplify History: Remove unnecessary merge commits.
- Replay Changes: Apply changes from one branch onto another without losing context.
- Facilitate Code Reviews: A cleaner history enhances the review process by providing a logical flow of development.
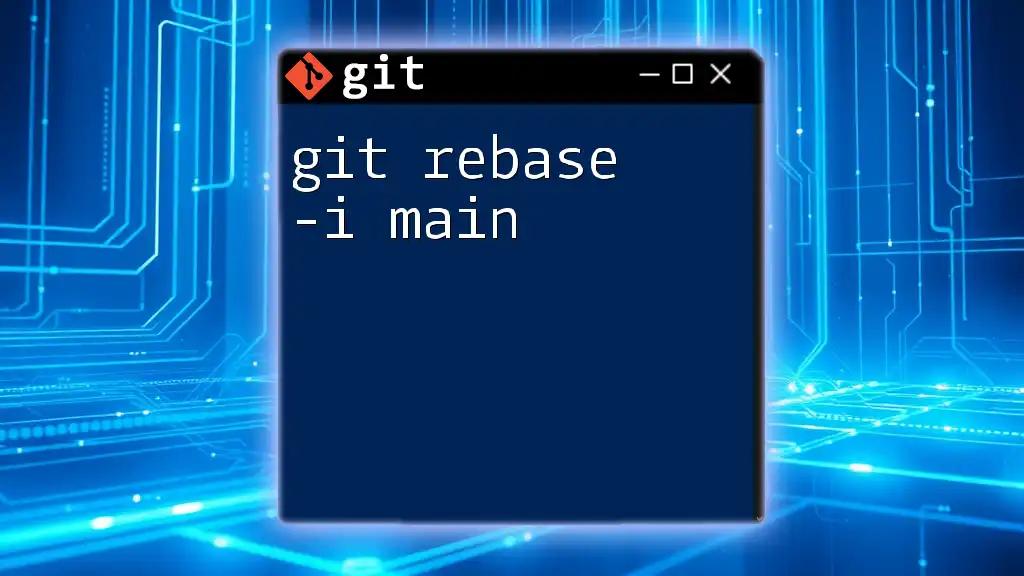
Understanding Interactive Rebase with `-i`
What Does `-i` Stand For?
The `-i` in `git rebase -i master` stands for interactive. This mode allows you to actively choose what to do with each commit, presenting you with a list of commits and several options for manipulation.
How to Trigger Interactive Rebase
To start the interactive rebase process from your current branch onto `master`, use this command:
git rebase -i master
After executing this command, Git will launch your default text editor, listing your commits that are about to be rebased.
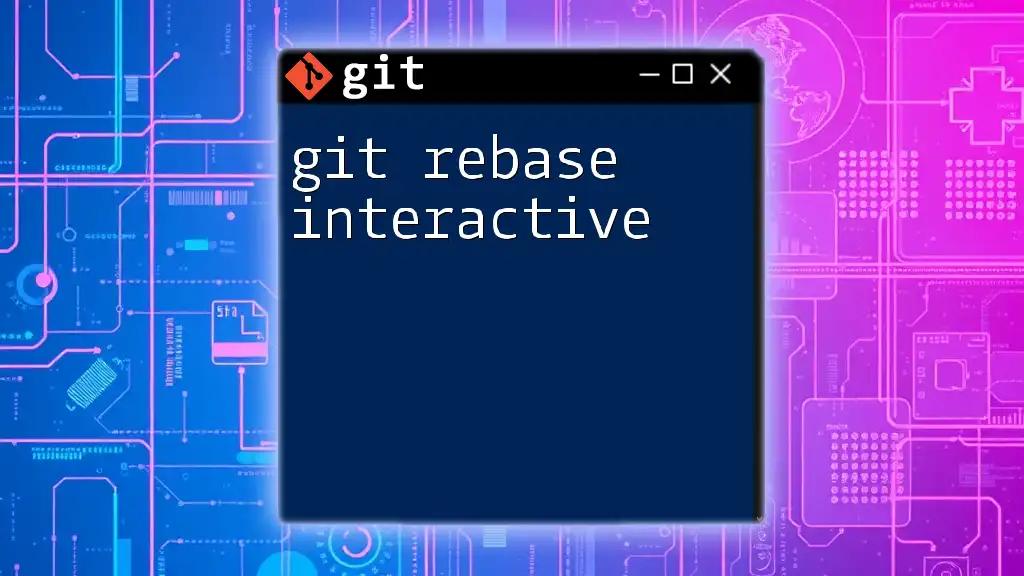
Setting Up for Interactive Rebase
Preparation Steps
Before diving into your rebase, make sure your working directory is clean:
git status
This command will show you any outstanding changes in your working directory. A clean state ensures that you can execute the rebase without complications.
Fetching the Latest Changes
Before beginning your rebase, it's a good practice to update your local repository to include the latest changes from the remote:
git fetch origin
This ensures that your rebase is based on the most current state of the `master` branch.
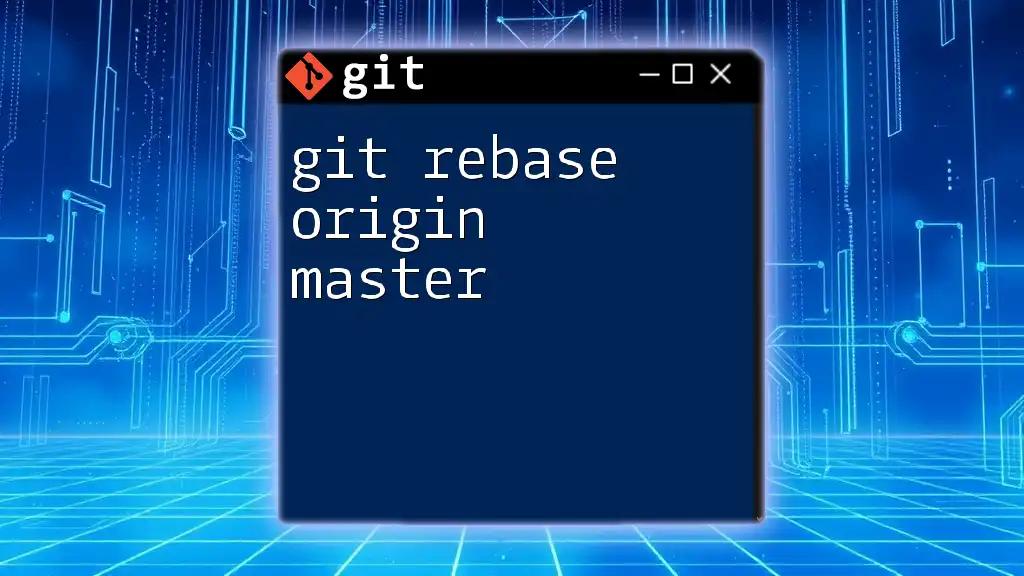
The Interactive Rebase Process
Launching the Rebase
Once you’ve run the `git rebase -i master` command, your text editor will open, revealing a list of commits you have made that are not yet in `master`. You will see lines that begin with the word `pick`, followed by the commit hash and message. This is where the magic happens!
Working with the Commit List
Changing Commit Order
You can easily change the order of commits by rearranging the lines in the editor. The order of the commits affects the final history and can be useful for logical grouping of changes.
Editing Commits
If you want to tweak a specific commit, you can change `pick` to `edit`. This will allow you to modify the commit when you reach this point in the rebase process.
Example:
pick abcdef1 First commit
edit abcdef2 Second commit
After saving and closing the editor, Git will stop at the specified commit. You can then make changes, stage them, and continue with:
git commit --amend
git rebase --continue
Squashing Commits
Squashing commits is a technique to combine multiple commits into one. This is particularly useful for cleaning up your history by merging a series of small commits into one coherent commit.
You can squash commits by changing `pick` to `squash` (or `s`). For example:
pick abcdef1 First commit
squash abcdef2 Second commit
Once you save the changes, Git will prompt you to combine the commit messages, allowing you to create a single, meaningful message.
Other Options in the Rebase Menu
Rewording Commit Messages
Sometimes, you may need to clarify or improve commit messages. You can change `pick` to `reword` (or `r`) to allow editing the commit message without modifying the content.
Dropping Commits
If you encounter commits that are no longer relevant, you can simply delete the corresponding line from the rebase list. This is known as dropping a commit, which effectively removes it from the history.
Fixing Conflicts During Rebase
Occasionally, conflicts may arise during the rebase process. If this occurs, Git will alert you, and you can address conflicts using:
git status
This command shows you the files with conflicts. After resolving the conflicts in the respective files, stage your changes:
git add <fixed-file>
Then, continue the rebase:
git rebase --continue
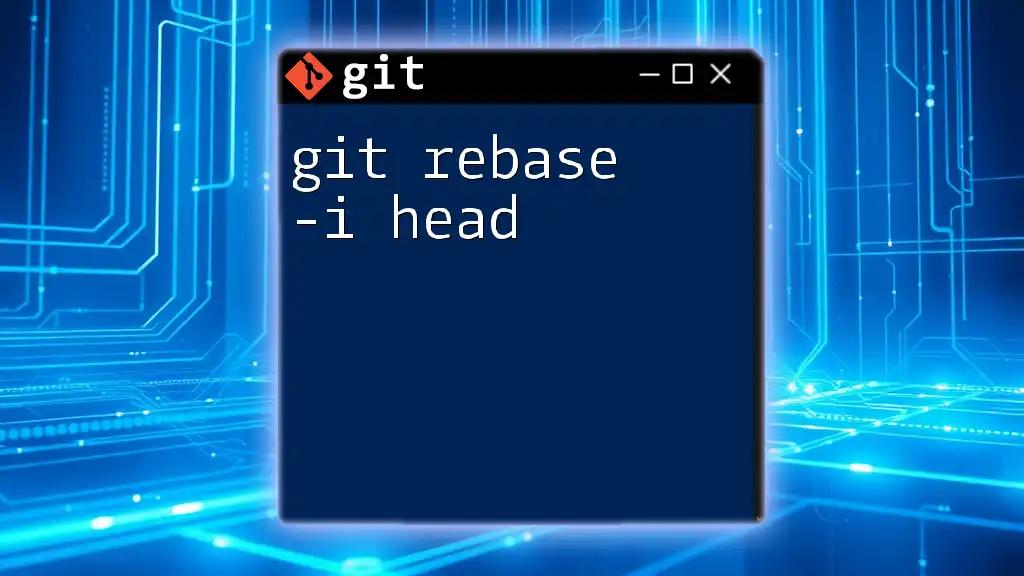
Best Practices for Using `git rebase -i master`
When to Use Interactive Rebase
Interactive rebasing is best used in situations where you have local commits that you want to polish before pushing to a shared branch. It’s especially helpful when working on feature branches. Avoid using rebase on shared branches to prevent disrupting your teammates' work.
Avoiding Common Pitfalls
Be cautious when rebasing, especially with commits that have been pushed to a shared repository. These changes may disrupt the collaborative workflow. Always communicate with your team when considering a rebase on shared branches.
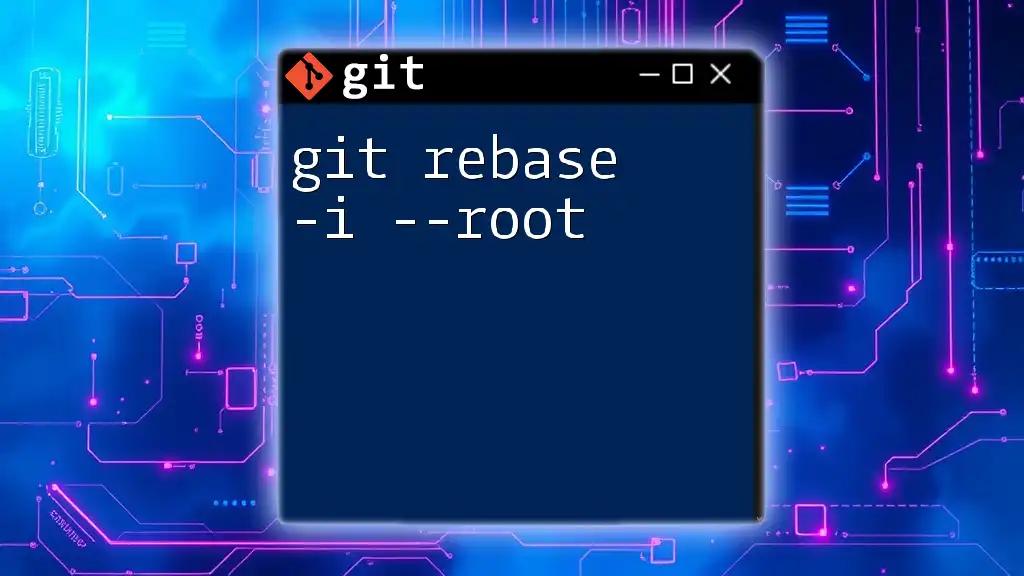
Finalizing the Rebase
Completing the Rebase Process
After you have made the necessary changes during the interactive rebase and resolved any conflicts, you will need to finalize the rebase. Ensure that all changes are committed and reviewed properly.
Pushing Changes to Remote
Once your rebase is complete, you can push your changes to the remote repository. Keep in mind, however, that you might need to force push if you have rewritten commit history:
git push origin <branch-name> --force
This action overwrites the remote branch with your newly rebased commits.
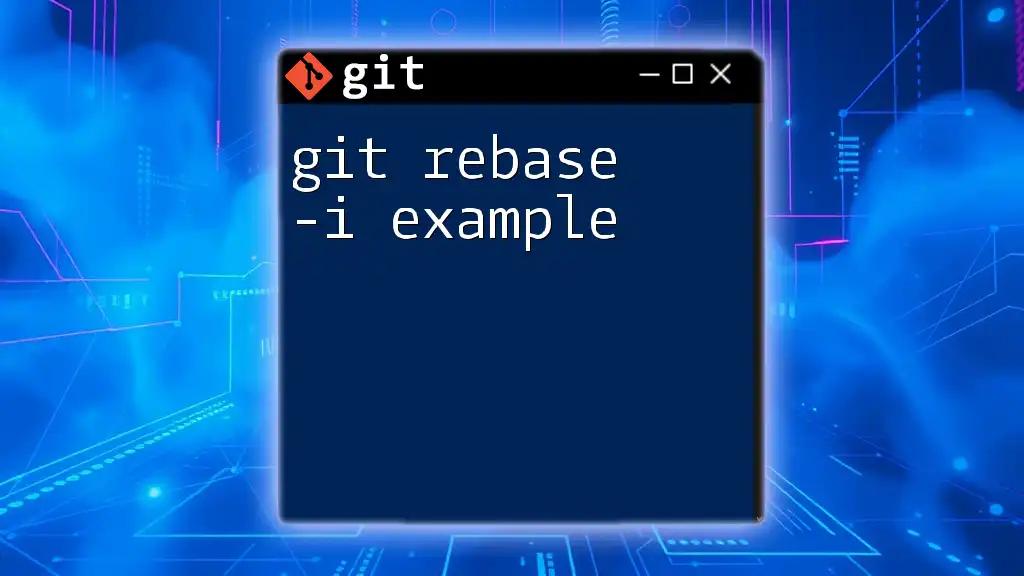
Conclusion
Using `git rebase -i master` efficiently allows for a streamlined and polished commit history, enhancing collaboration and development processes. By practicing regularly and following best practices, you can master interactive rebasing and contribute to a cleaner, more efficient project history.
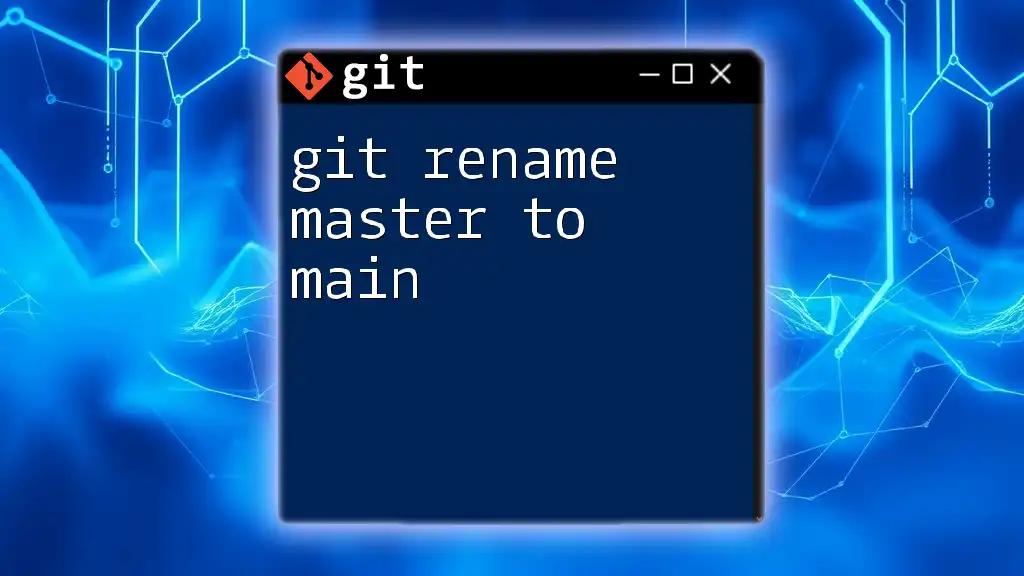
Additional Resources
For those looking to deepen their understanding of Git and its features, numerous reputable online resources and courses are available. Consider exploring Git's official documentation, advanced tutorials, or GitHub's Learning Lab for more hands-on experience.