To reset your local `master` branch to match the `origin/master` branch in Git, use the following command:
git reset --hard origin/master
Understanding Git Branches
What is a Branch?
In Git, a branch is essentially a pointer to a particular commit in your repository. Branching enables you to develop features or fixes in isolation without affecting the primary codebase. When you create a new branch, you're essentially creating a unique context for your changes, which makes collaboration and version control much simpler and more organized.
The Master Branch
The `master` branch is traditionally the default branch in Git repositories. It acts as the main development branch where the most stable, production-ready code resides. Ensuring that your `master` branch remains clean and up to date is crucial for maintaining a streamlined workflow. Striving to keep your `master` branch free of experimental code helps avoid conflicts and bugs in your project's core code.
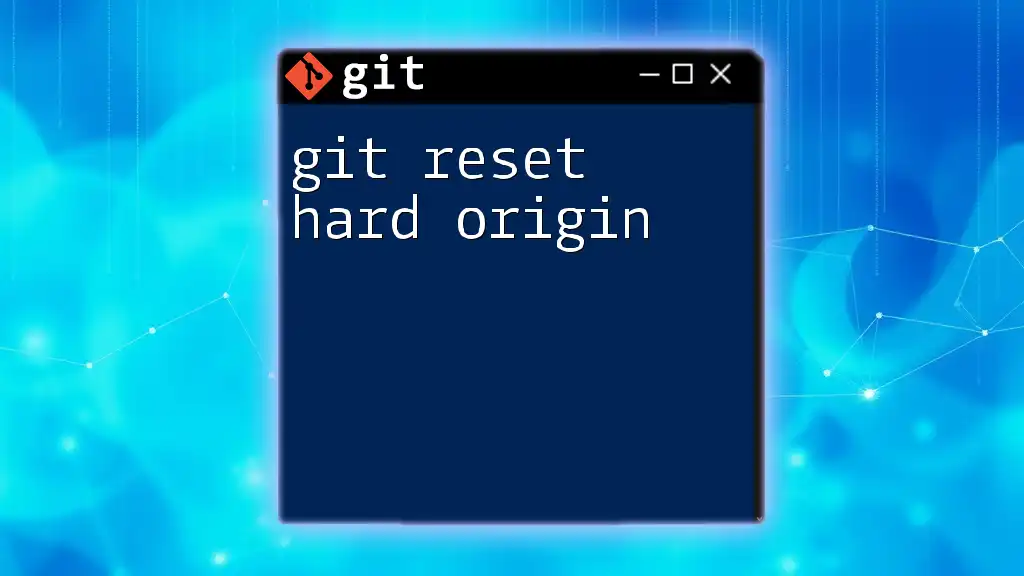
The Origin Remote
What is Origin?
In Git, origin refers to the default remote repository from which your local repository was cloned. When you clone a repository, Git automatically assigns the name origin to the URL of the source repository. This is significant because it allows you to easily fetch, pull, and push changes between your local repository and the remote repository without needing to specify the URL repeatedly.
You can verify the configured remotes in your Git repository using the command:
git remote -v
Executing this command will display the URLs associated with the names of your remotes, helping you confirm that origin points to the correct source.
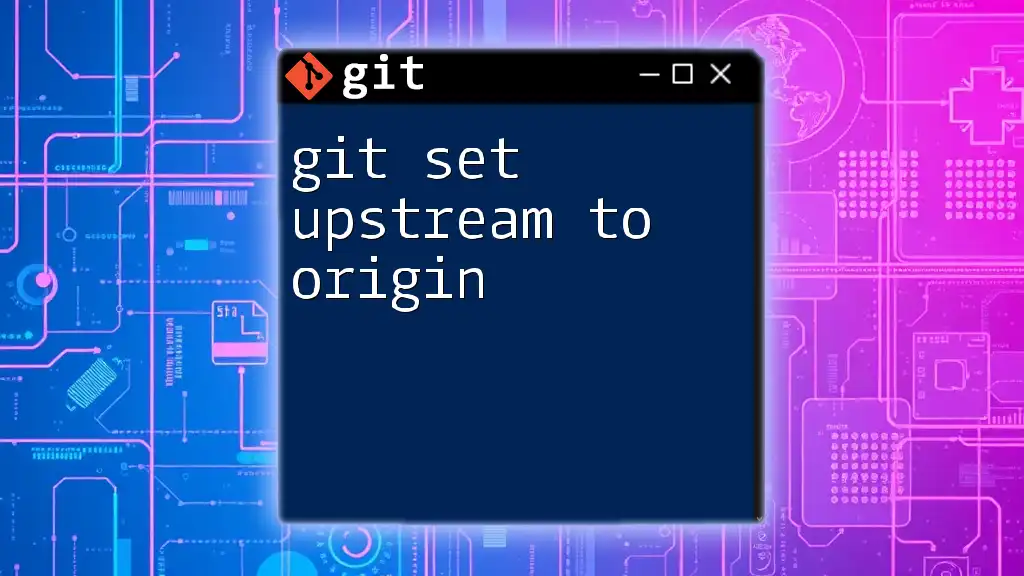
The Git Reset Command
What is Git Reset?
The `git reset` command is used to reset your current HEAD to a specified state. This powerful command can modify the staging area and the working directory based on the options used. Understanding the differences between the types of resets—soft, mixed, and hard—is essential to utilize this command effectively in your workflow.
Soft Reset: Moves the HEAD pointer but does not affect the staging area or working directory. Mixed Reset: Moves the HEAD pointer and resets the staging area, but leaves the working directory unchanged. Hard Reset: Moves the HEAD pointer, resets the staging area, and also updates the working directory to match the repository state.
Common Usage Scenarios
Knowing when to use `git reset` is critical. Situations may arise when you want to undo a recent commit, remove unwanted changes from staging, or revert to a stable state following a series of problematic commits. However, applying this command incorrectly can lead to data loss, especially when using options that alter the working directory.
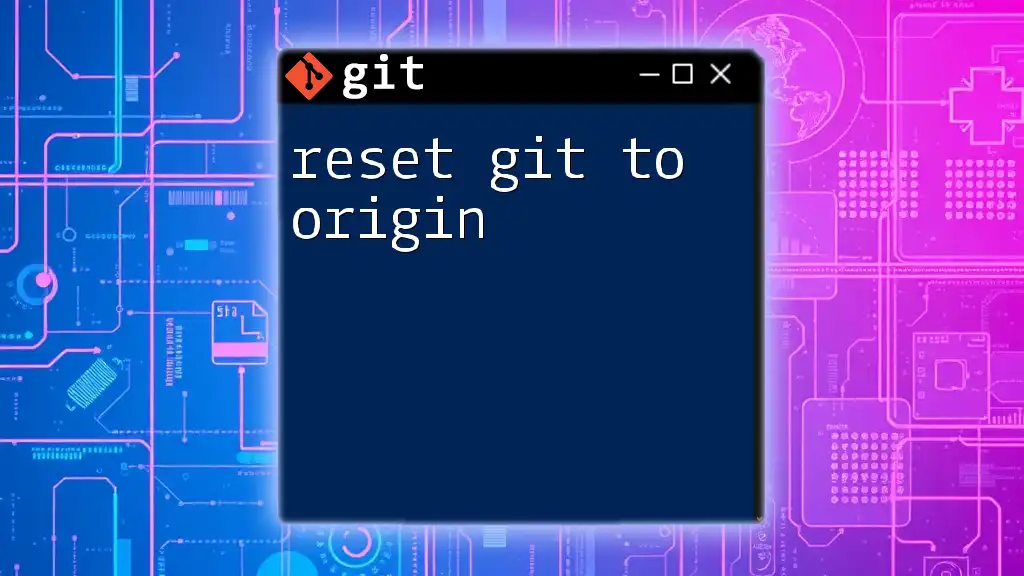
Resetting Master to Origin
Introduction to Resetting
Resetting your local `master` branch to match `origin/master` is a common practice when you want to discard local changes or align with the latest committed code from your team. This operation ensures that your local version reflects the current state of the remote repository.
The Command Syntax
To reset your local `master` branch to match `origin`, you can use the following command:
git reset --hard origin/master
Here's a breakdown of this command:
- `git reset`: The command being executed.
- `--hard`: This option tells Git to update the working directory and staging area to match the specified commit (in this case, `origin/master`), discarding any local changes.
- `origin/master`: The target state to which you want to reset your local branch.
Example Code Snippet
When you're ready to perform the reset, you should first ensure you have the latest updates from the remote repository:
git fetch origin
git reset --hard origin/master
Step-by-Step Breakdown
Step 1: Fetching Updates from the Remote
Before executing the reset command, it's vital to obtain the latest changes from the remote repository. By executing `git fetch origin`, you download all the recent commits, branches, and tags from the remote without altering your local files:
git fetch origin
This command ensures that your local repository is aware of any updates that have occurred in the remote repository since your last interaction.
Step 2: Resetting the Local Master Branch
After fetching updates, you can proceed with resetting the local `master` branch to `origin/master` using the `--hard` option. Caution is advised, as this command will permanently discard any local changes:
Using `git reset --hard origin/master` overwrites your local changes, returning your `master` branch to the state it was in at the last commit from the upstream branch. It’s important to remember that any uncommitted changes will be lost, making backups or stashes prudent practices.
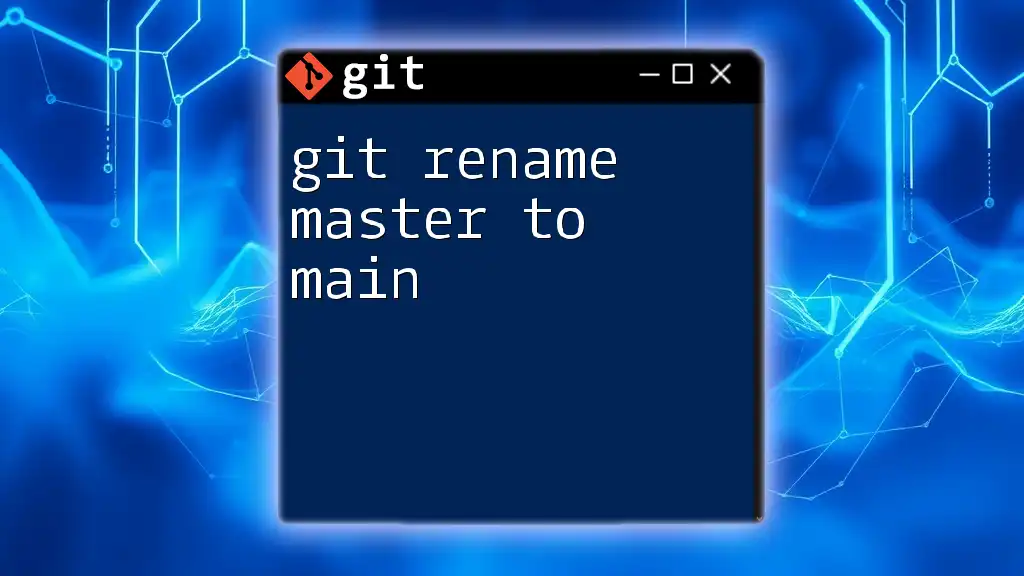
Use Cases and Considerations
Scenarios to Consider When Resetting
You might consider resetting your `master` branch to `origin` in several situations, such as:
- Reverting After a Bad Commit: If you've made a commit that introduced bugs or issues, resetting allows you to revert to a stable state.
- Synchronizing with Team Changes: Teams often push updates to `origin/master`, and resetting can help you align your local changes with those made by collaborators.
Risks and Consequences
One of the main risks of performing a hard reset is the potential loss of uncommitted changes. If there are unsaved changes in your working directory, they will be permanently discarded. Always ensure you have either stashed your changes or committed them to another branch before executing a hard reset.
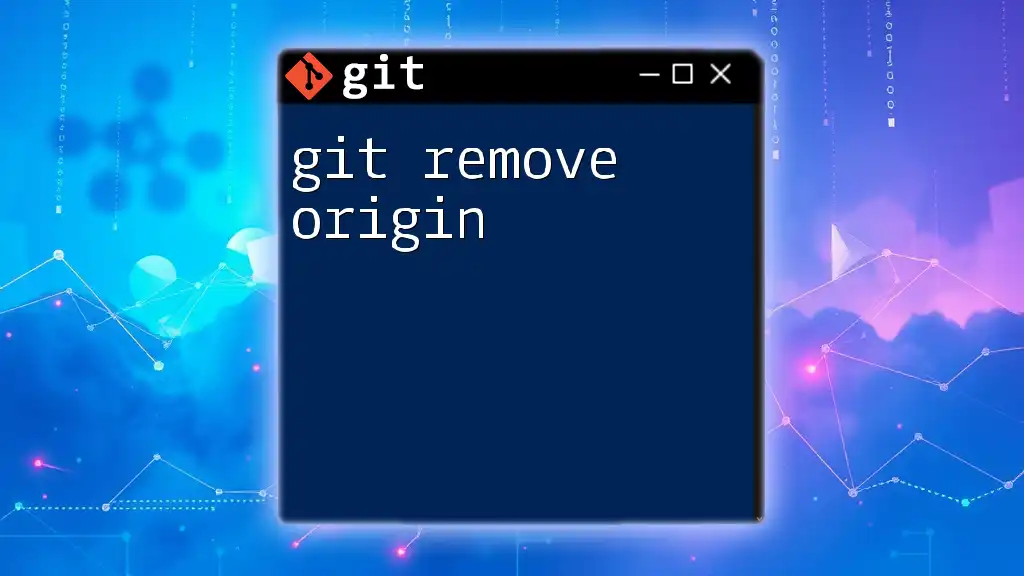
Alternative Methods
Using Git Checkout
If you wish to switch to the state of `origin/master` without affecting your current changes, you can use `git checkout` instead. This command allows you to view the remote state without permanently altering your local `master`:
git checkout origin/master
This alternative approach can be advantageous in scenarios where you want to explore or create a new branch without losing your existing work.
Using Git Revert
Another safer option is to use `git revert`. While `reset` discards changes, `revert` creates a new commit that undoes the effects of a previous commit:
git revert <commit-hash>
This command is particularly useful in collaborative environments, as it retains project history while correcting mistakes.
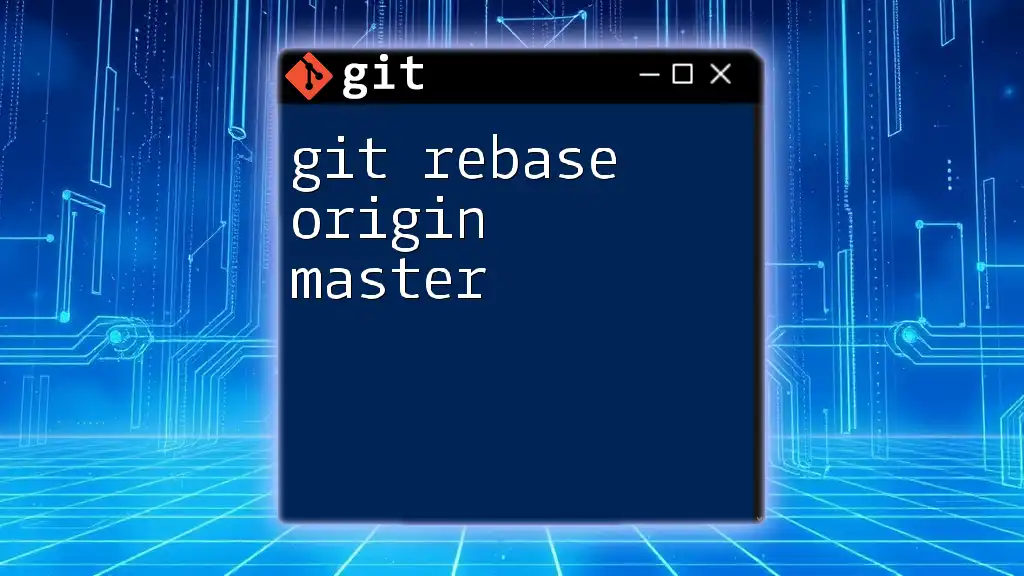
Conclusion
Grasping how to effectively reset your local master branch to origin is an essential skill for developers using Git. This command helps in maintaining a clean and up-to-date working environment, significantly streamlining your development workflow.
Adopting best practices, like ensuring you have backups and testing commands in a safe environment, will bolster your confidence and proficiency with Git. By understanding the nuances of `git reset master to origin`, you keep your project organized and improve your collaborative efforts.
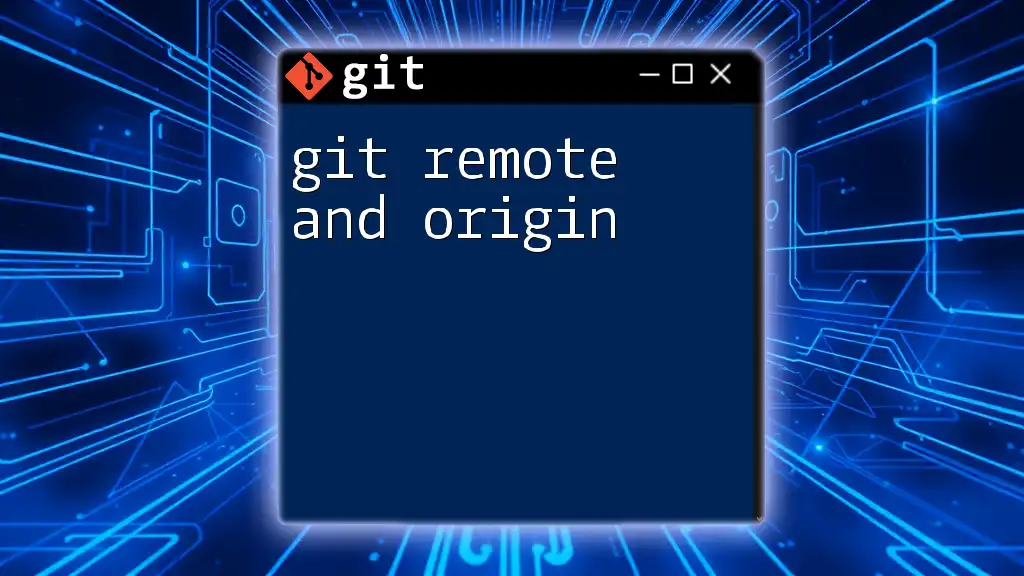
Additional Resources
For more in-depth learning, consider referring to the official Git documentation, which provides comprehensive information about the commands and workflows associated with Git. Signing up for a course on Git can also enhance your skills and understanding of advanced features.
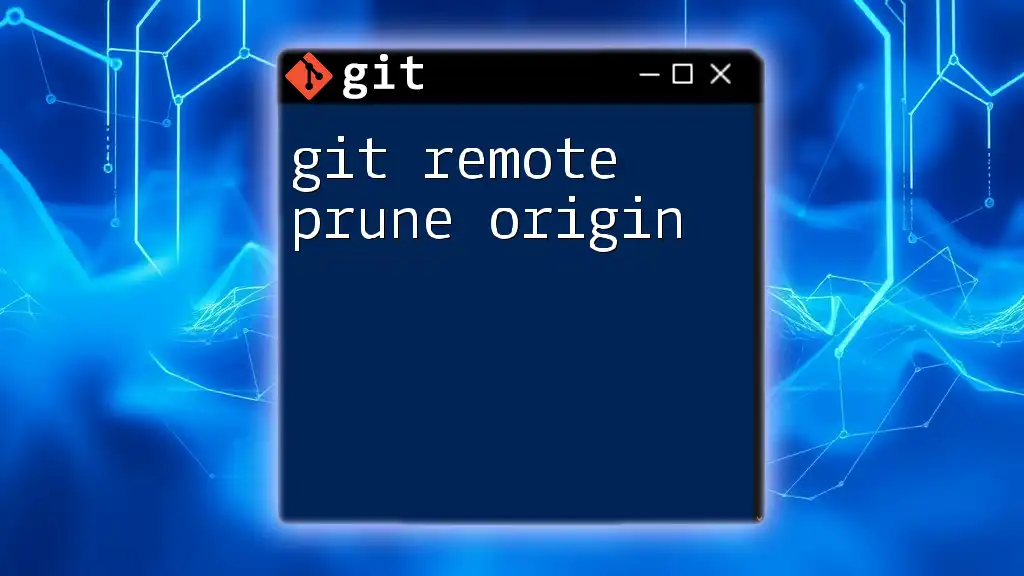
Call to Action
If you found this guide helpful, consider signing up for our newsletter for more concise tips on Git commands. We welcome your feedback and questions about your own experiences with Git!