The command `git fetch origin` updates your local repository with the changes from the remote repository (named "origin") without merging them into your current branch.
git fetch origin
Understanding Git Basics
What is Git?
Git is a powerful distributed version control system that allows multiple developers to work on projects simultaneously without overwriting each other’s changes. It empowers teams to manage versions of their code, track changes over time, and collaborate effectively. Understanding Git guides developers in maintaining a clear history of their work and facilitates easy coordination within projects.
The Concept of Remote Repositories
Remote repositories are copies of your project hosted on the internet or a local server. They enable developers to collaborate seamlessly, allowing multiple individuals to contribute to the same project from different locations.
Key Differences:
- Local Repository: The version of the project you have on your machine.
- Remote Repository: The centralized version hosted on a platform (e.g., GitHub, GitLab).
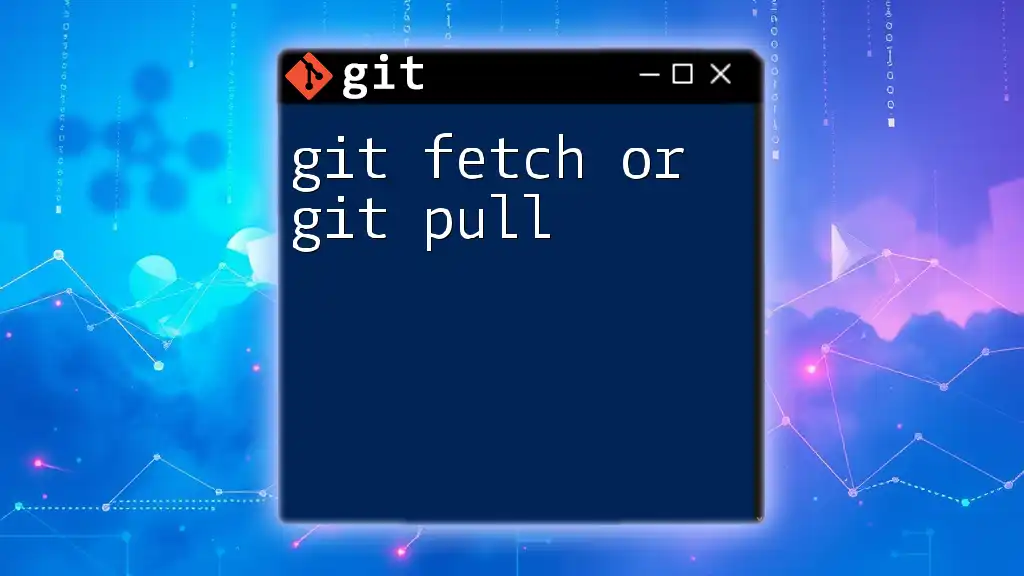
What is `git fetch origin`?
Definition
The command `git fetch origin` is a vital command used to update your local repository with any changes made in the remote repository, specifically the one known as "origin." It downloads the commits, files, and references from the remote without merging them into your current branch.
The Role of 'Origin'
In Git terminology, "origin" refers to the default name given to the remote repository from which you initially cloned or tracked. By default, Git associates remote repositories with this alias unless you specify otherwise. Understanding "origin" helps in managing multiple remotes when collaborating on larger projects.
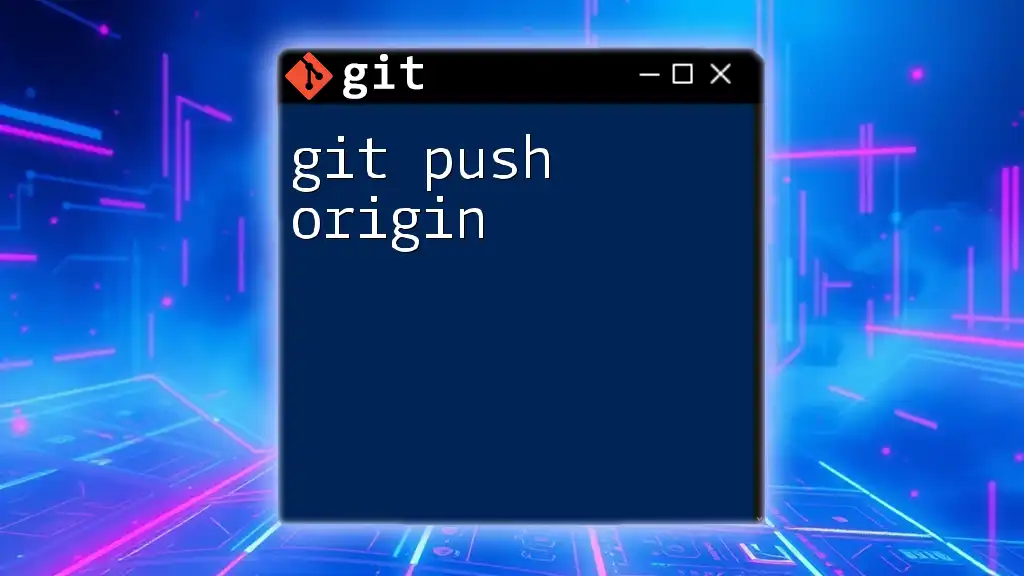
How to Use `git fetch origin`
Basic Syntax
To perform a fetch operation, you simply use:
git fetch origin
Step-by-Step Guide
Step 1: Open Your Terminal
Start by accessing your terminal or command line interface (CLI), which is where you will execute your Git commands.
Step 2: Navigate to Your Repository
Before you can fetch updates, you need to navigate to your local repository. Use the following command to change directories:
cd path/to/your/repository
Step 3: Run the Command
Once you are in your repository, execute the command:
git fetch origin
Upon running this command, your terminal will display output indicating that updates have been fetched, which may look like this:
From https://github.com/username/repo
* [new branch] feature-branch -> origin/feature-branch
Example Scenario
Consider a scenario where you are a developer collaborating with your team on a project. Your teammates have made changes and pushed them to the remote repository. By executing `git fetch origin`, you can bring your local repository up to date with their changes, but it does not integrate those changes into your working branch just yet. This separation allows you to review the new changes before potentially merging them.
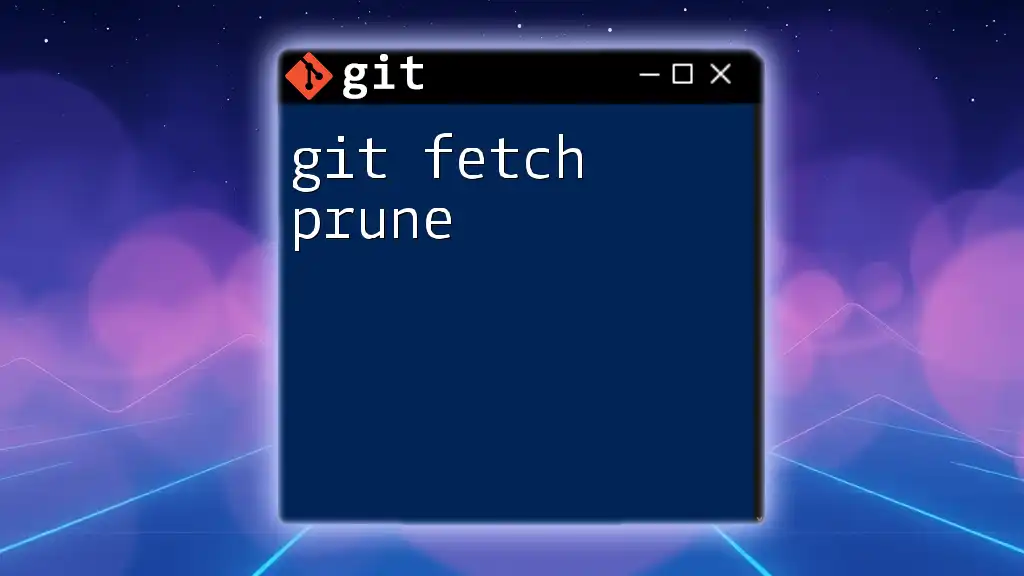
What Happens When You Execute `git fetch origin`?
Behind the Scenes
When you run `git fetch origin`, Git reaches out to the remote repository. It retrieves and updates:
- New commits from remote branches.
- Changes that have occurred in the remote repository since your last fetch.
Outcomes of the Fetch Operation
After performing the fetch, your local repository holds the latest changes from the origin. However, these changes exist in the form of remote-tracking branches (like `origin/main` or `origin/feature-branch`) and have not yet been integrated into your active branches.
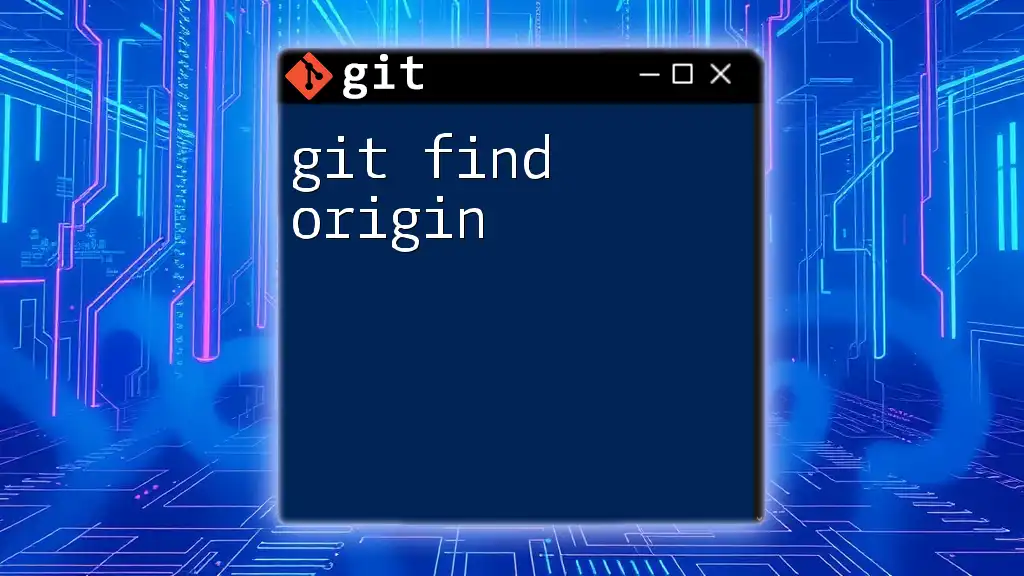
Understanding the Differences between `git fetch`, `git pull`, and `git clone`
Detailed Comparison
To clarify the distinctions among these Git commands, let’s define each:
-
`git fetch`: This command downloads the changes from the remote repository but does not merge them. It provides an opportunity to review changes before integrating them into your branch.
-
`git pull`: This command combines `git fetch` and `git merge`. It fetches changes from the remote repository and immediately merges them into your current branch, which can lead to conflicts that require resolution.
-
`git clone`: This command creates a new local copy of a remote repository. It includes all branches, tags, and commits, essentially duplicating the remote repository on your local system.
Command | Description |
---|---|
`git fetch` | Downloads changes from the remote without merging. |
`git pull` | Downloads and merges changes from the remote. |
`git clone` | Initializes a local copy of a remote repository. |
Use Cases
- Use `git fetch origin` when you want to stay informed about updates in the remote repository without altering your current workspace.
- Choose `git pull` for instances where you are ready to integrate changes directly into your working branch.
- Opt for `git clone` when you are starting a new project and need a local copy of an existing remote repository.
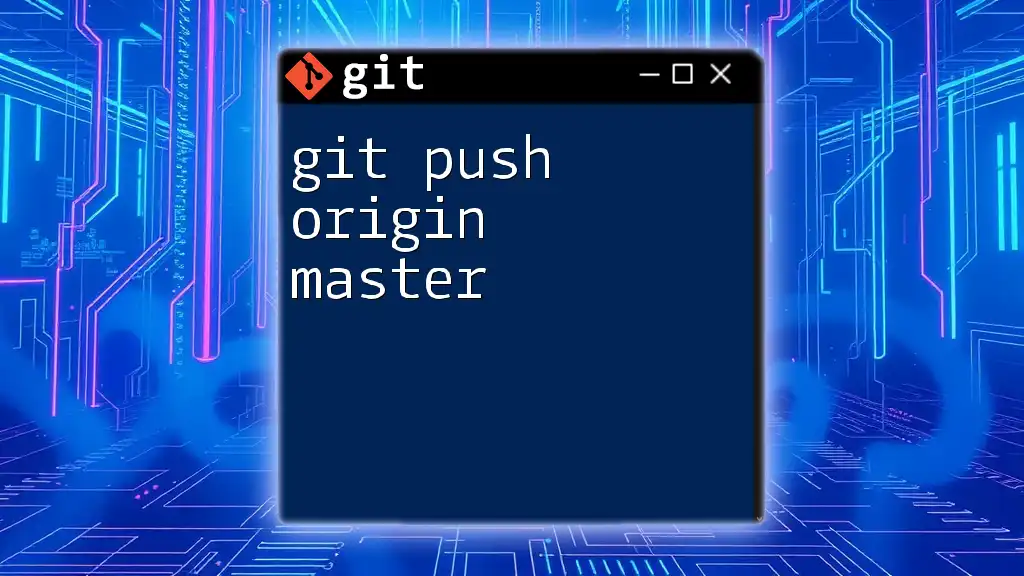
After Fetching: Next Steps
Reviewing Fetched Changes
To check what changes have been fetched and view the differences, you can use:
git log origin/main
This command displays the commit history from the `main` branch of the origin. This review is essential to understand what updates have been made by your team members.
Merging Changes
If after reviewing you want to incorporate fetched changes into your branch, you can do so with:
git merge origin/main
This command merges the changes from the fetched branch into your current branch. Be prepared to resolve any merge conflicts that may arise.
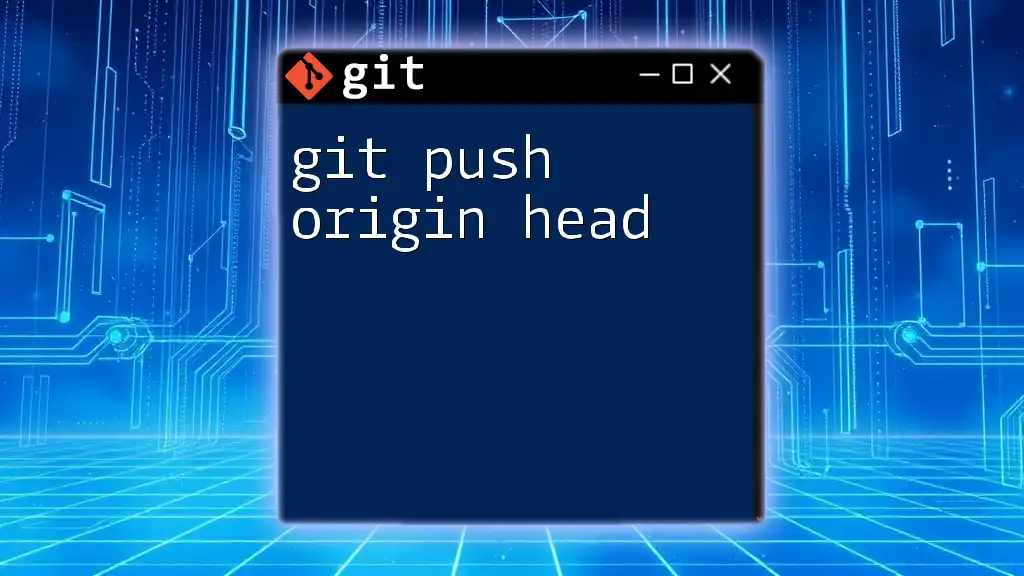
Best Practices for Using `git fetch origin`
Regular Fetching
Making it a habit to regularly run `git fetch origin` keeps your local repository updated with the latest changes from your collaborators. This practice ensures that you're always aware of modifications and can make informed decisions on when to merge updates.
Avoiding Merge Conflicts
Frequent fetching helps minimize the chances of running into major merge conflicts. Staying updated with your teammates’ changes allows you to plan your modifications effectively.
Keeping Your Work Organized
Regularly review your local branches and fetched updates. This practice helps maintain clarity about your work and ensures that your repository remains organized.
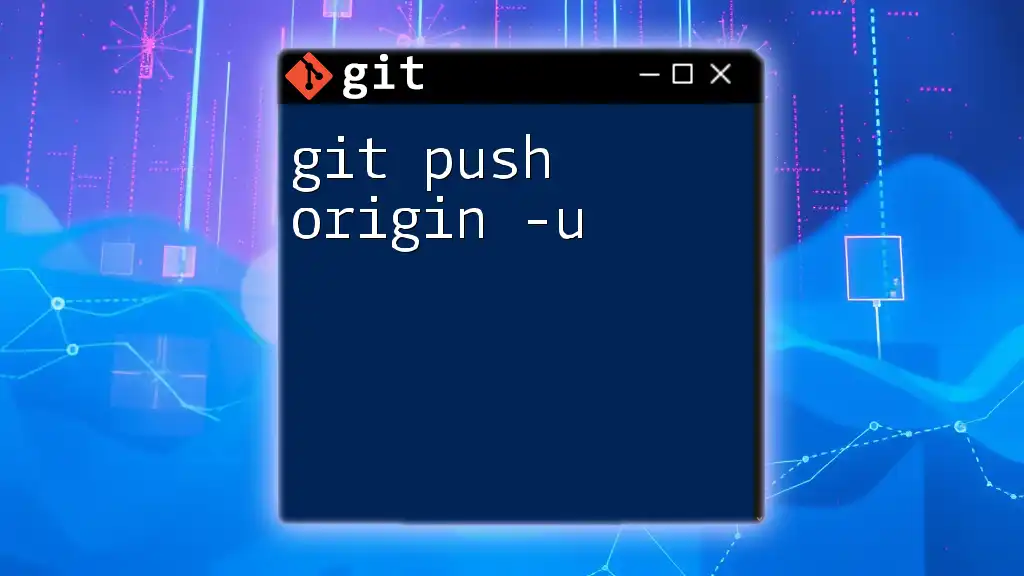
Troubleshooting Common Issues
Connectivity Problems
Sometimes, running `git fetch origin` may fail due to connectivity issues. Common messages may involve network timeouts or authentication errors. Ensure your internet connection is stable and check your remote repository configuration.
Fetching Failed
In the case of errors stating that fetching has failed, check whether the remote repository URL is accurate. You can verify your remote settings with:
git remote -v
If the URL is incorrect or requires modification, you can update it using:
git remote set-url origin new-url
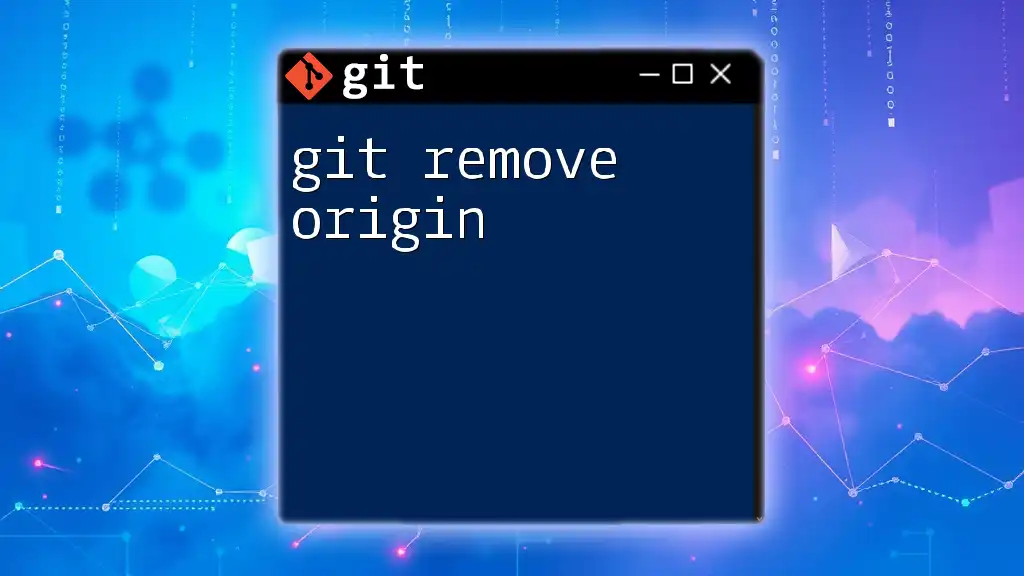
Conclusion
Using the command `git fetch origin` is essential for effective collaboration in version control with Git. By regularly fetching updates from your remote repository, you maintain awareness of changes and can manage your work more efficiently. Take the time to practice this command and explore more advanced Git functionality to enhance your development workflow.