The key difference between `git fetch` and `git pull` is that `git fetch` retrieves updates from a remote repository without merging them into your current branch, while `git pull` fetches the updates and automatically merges them.
Here’s a code snippet demonstrating both commands:
# Fetch updates from the remote repository
git fetch origin
# Pull updates and merge them into the current branch
git pull origin main
Understanding Git Basics
What is Git?
Git is a distributed version control system that allows multiple developers to work on a project concurrently. It keeps track of code changes, enables collaboration through branches, and maintains a full history of project modifications. With its powerful features, Git is the backbone of modern software development, ensuring code integrity and facilitating teamwork.
The Importance of Remote Repositories
Remote repositories are hosted on platforms like GitHub, GitLab, and Bitbucket. They allow teams to collaborate on projects from different locations. Understanding how to interact with these remote repositories is crucial for effective teamwork. The commands `git fetch` and `git pull` are essential for syncing local work with changes made by others in these repositories.
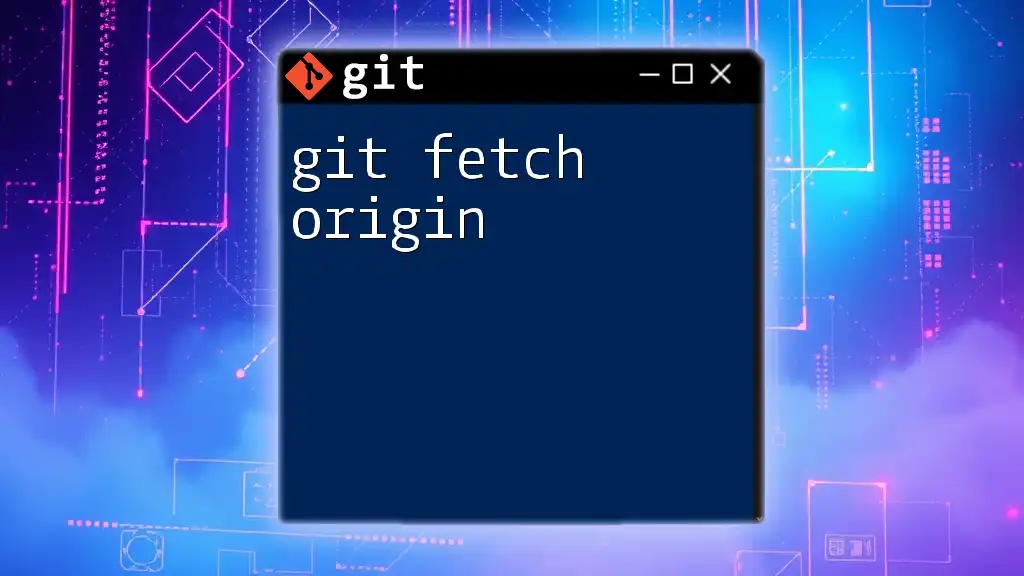
Git Fetch Explained
What is `git fetch`?
The `git fetch` command updates your local copy of a repository with the latest changes from the remote repository. Importantly, it does not modify your working directory or your current branch. Instead, it retrieves new commits and updates your remote-tracking branches, which allows you to review changes before integrating them.
When to Use `git fetch`
Use `git fetch` when you want to see what others have been doing without automatically merging their changes into your work. This is particularly useful in scenarios like:
- Reviewing the latest updates before merging.
- Comparing your local work with colleagues' changes.
- Preparing for integration, ensuring no conflicts arise unexpectedly.
How to Use `git fetch`
The basic syntax for `git fetch` is as follows:
git fetch [remote-name]
For example, if you're working with the remote repository named origin, the command would be:
git fetch origin
After executing this command, Git fetches updates from origin and records them in your local environment. You can then check the changes by viewing remote-tracking branches without impacting your current branch. For example, you might run:
git log origin/main
This lets you see the latest commits on the `main` branch of the remote repository.
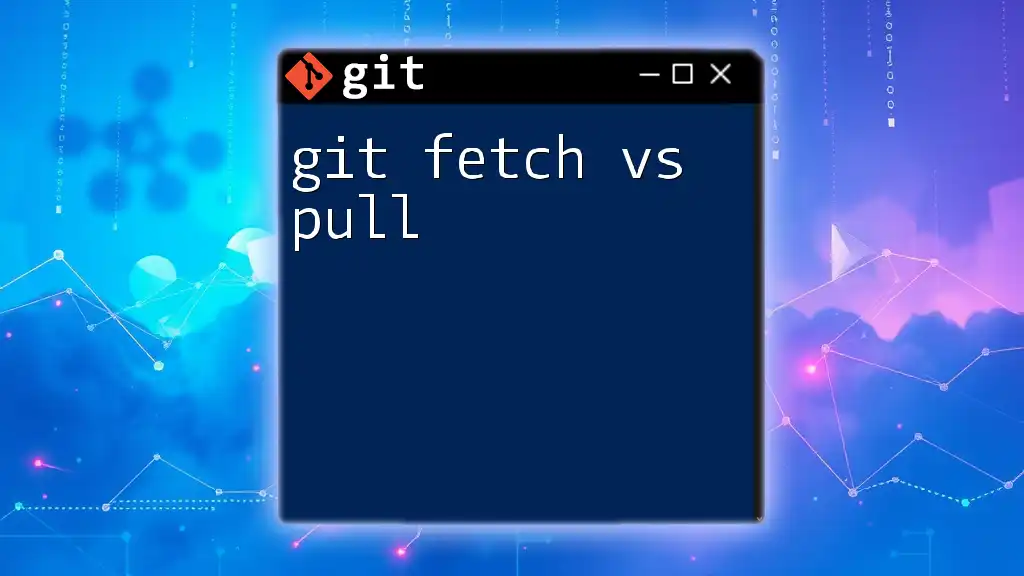
Git Pull Explained
What is `git pull`?
The command `git pull` is a higher-level command that combines `git fetch` and `git merge`. It retrieves changes from the remote repository and automatically merges them into your current working branch. This command is a time-saver when you want to quickly synchronize your local repository with the remote.
When to Use `git pull`
Use `git pull` when you're ready to incorporate changes from the remote repository into your current branch. Scenarios in which `git pull` shines include:
- You are working on a feature branch and want to quickly incorporate updates from the main branch.
- You are collaborating in an agile environment where frequent updates are expected.
How to Use `git pull`
The basic syntax for `git pull` is:
git pull [remote-name] [branch-name]
As an illustrative example, if you want to pull the latest changes from the `main` branch of the origin remote, you would execute:
git pull origin main
This command fetches the changes and merges them into your current branch in one step. It’s crucial to note that if there are any conflicts between your local changes and the fetched changes, Git will alert you, and you’ll need to resolve them before completing the merge.
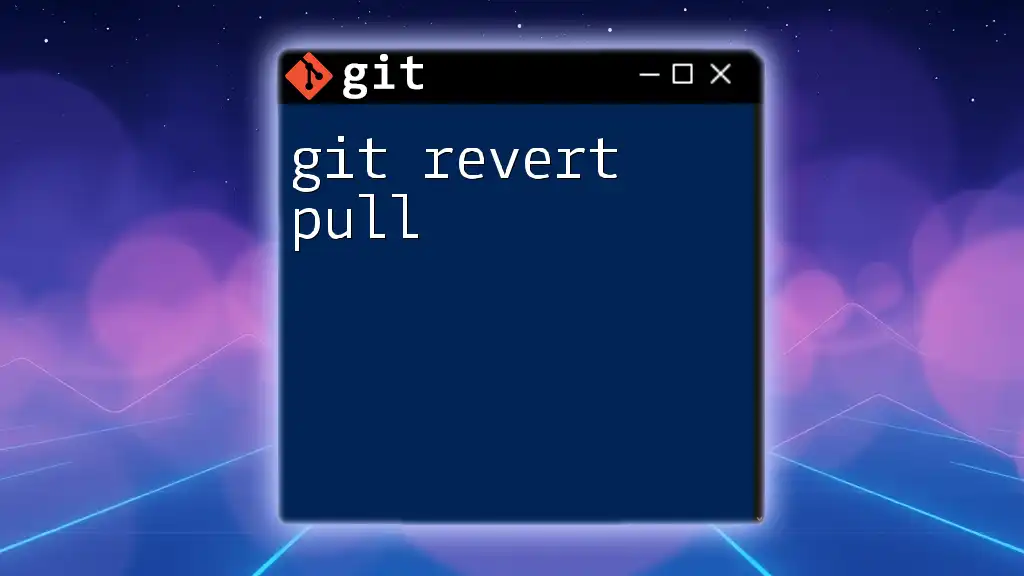
Key Differences Between `git fetch` and `git pull`
Fetch vs. Pull: The Merge Factor
The fundamental difference between `git fetch` and `git pull` is that fetch does not merge changes, while pull automatically merges them. With `git fetch`, you have the luxury of reviewing remote changes before deciding how to integrate them into your local work.
Effects on Local Repository
- `git fetch`: Updates remote-tracking branches, allowing you to inspect new changes without altering your current branch. You can run additional commands like `git log` or `git diff`.
- `git pull`: Merges changes directly into your current branch, potentially leading to merge conflicts if there are local modifications.
Safety and Control
For those who prefer a cautious approach or wish to maintain control over the integration process, `git fetch` is the better option. With this command, you have the opportunity to review incoming changes and prepare your local work accordingly.
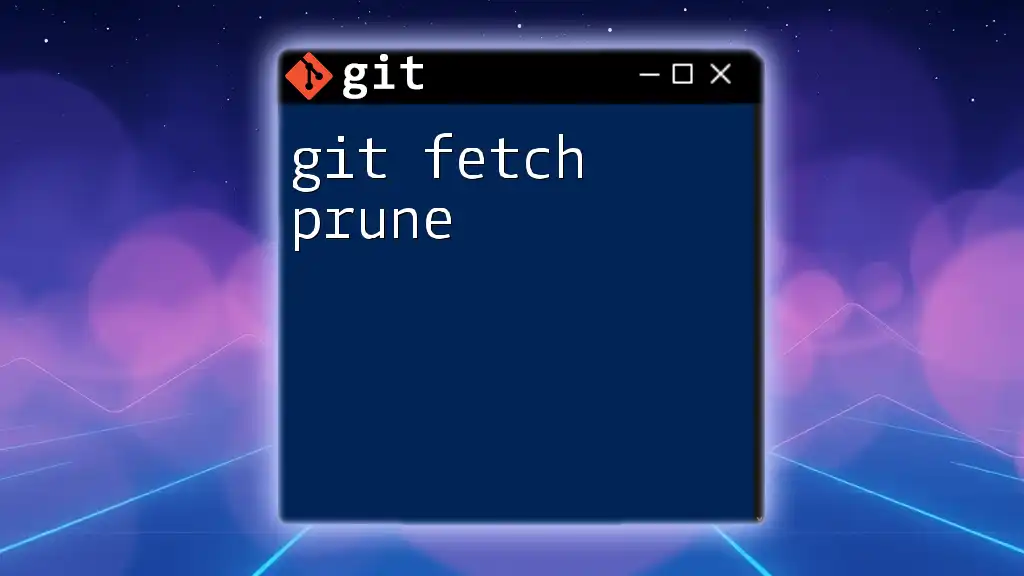
Best Practices
When to Use Each Command
- Opt for `git fetch` when you want to stay informed about ongoing changes in team projects without altering your working state. This way, you can review updates before deciding on your next steps.
- Use `git pull` for quick updates when you're ready to merge remote changes into your work without prior review. This is especially suitable for fast-paced environments where staying up-to-date is crucial.
Combining Commands for Better Workflow
A recommended workflow involves using `git fetch` before `git merge`. This pattern can look like this:
-
Fetch the latest changes:
git fetch origin
-
Review changes:
git log origin/main
-
Merge if appropriate:
git merge origin/main
This method allows you to control the integration process, ensuring your local repository stays organized and conflict-free.
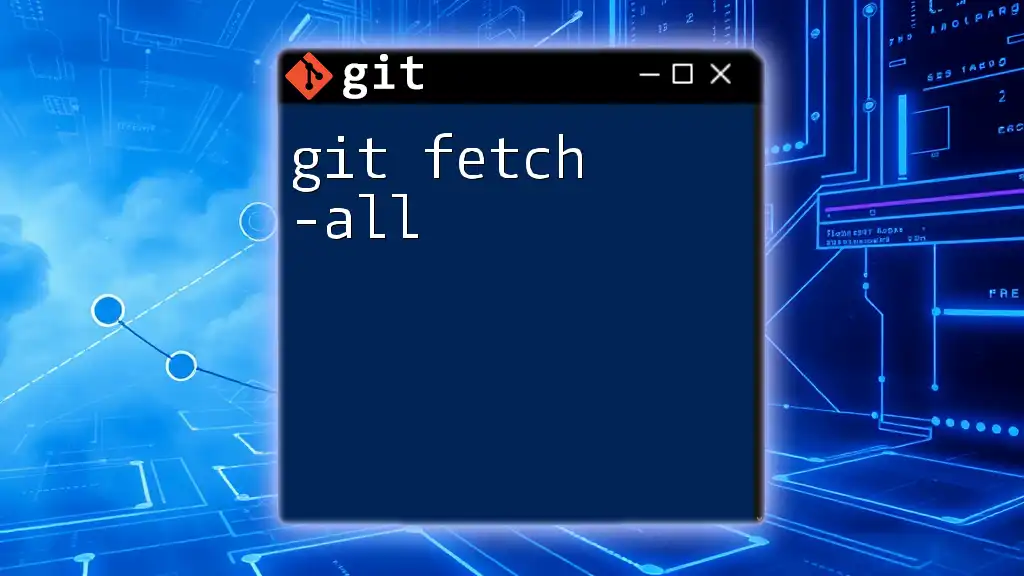
Conclusion
Choosing between `git fetch` and `git pull` can significantly impact your development workflow. `git fetch` provides safety and control, while `git pull` offers speed and efficiency. Understanding how to leverage these commands effectively is key to mastering Git and improving collaborative efforts in software development. As you navigate your projects, try incorporating these commands into your workflow for better version control and teamwork.
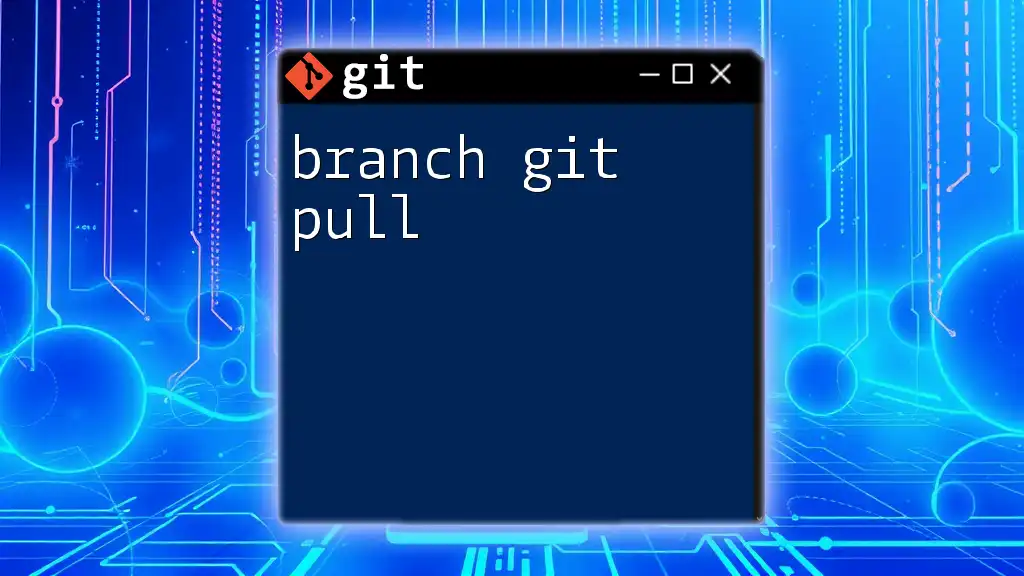
Additional Resources
Learning More About Git
For those looking to dive deeper into Git, numerous books, online courses, and tutorials are available. Seek out reputable resources that align with your learning style.
Common Git Commands Cheat Sheet
Familiarizing yourself with various Git commands beyond `fetch` and `pull` can strengthen your command over version control. Consider keeping a cheat sheet handy to continue building your Git expertise.