The `git pull` command is used to fetch changes from a remote branch and merge them into your current local branch, allowing you to stay updated with the latest code changes from collaborators.
Here’s the syntax for pulling from a specific branch:
git pull origin branch-name
What is Git Pull?
Understanding Git Pull
The `git pull` command is an essential part of collaboration in Git. It is used to fetch changes from a remote repository and automatically merge those changes into your current branch. This command acts as a combination of two other commands: `git fetch` (which retrieves the changes) and `git merge` (which integrates those changes into your working directory).
Why Use Git Pull?
Using `git pull` allows you to keep your local repository up-to-date with the latest changes made by your teammates. This practice helps prevent merge conflicts and ensures that you are working with the most recent version of the codebase. By regularly pulling changes, you maintain a clean codebase that is less susceptible to issues during integration.
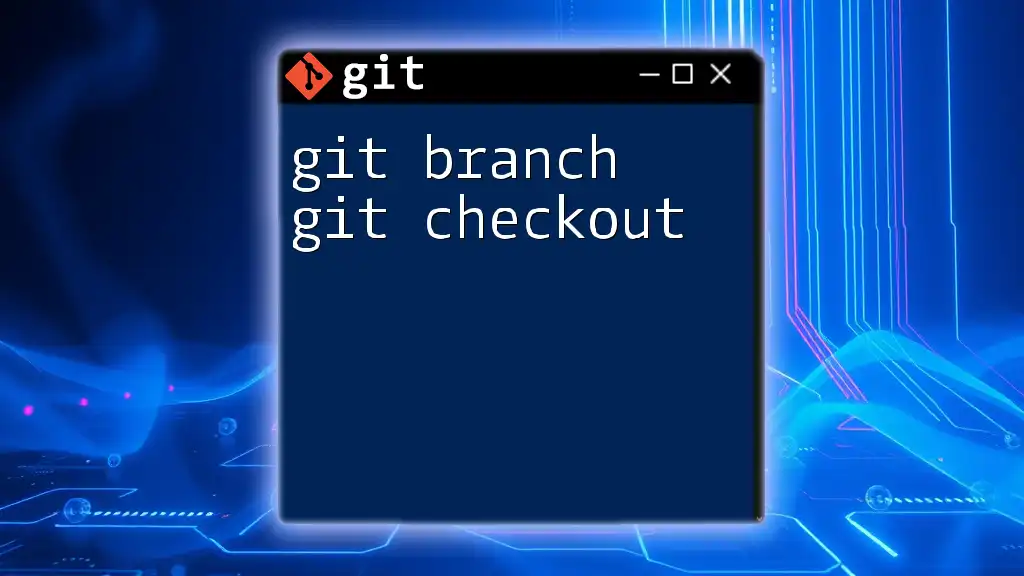
Branching in Git
What is a Git Branch?
A Git branch is essentially a pointer to a specific commit. Branches allow you to develop features, fix bugs, or experiment in isolated environments without affecting the main codebase. This feature is invaluable in collaborative workflows, enabling multiple developers to work concurrently on different aspects of a project.
Creating and Managing Branches
To effectively use branches, you need to know how to create and manage them:
-
To create a new branch, use the command:
git branch <branch-name>
This creates a new branch without switching to it.
-
To switch to a newly created branch (or any existing branch), use:
git checkout <branch-name>
-
To display all branches, simply enter:
git branch
This command will show you a list of branches and highlight the branch you are currently on.
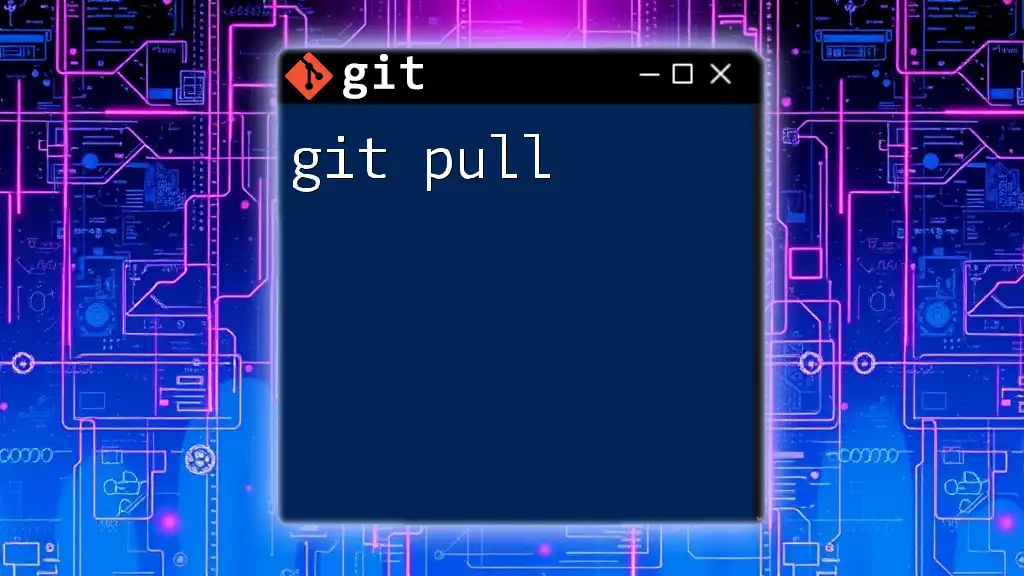
Understanding Remote Repositories
What is a Remote Repository?
A remote repository is a version of your project that is hosted on a server, enabling collaboration among multiple developers. These repositories can be hosted on platforms such as GitHub, GitLab, and Bitbucket.
Checking Remote Configuration
To view the remote repositories associated with your local repository, use:
git remote -v
This command will display the names and URLs of the remote repositories, helping you understand where you are fetching or pushing updates.
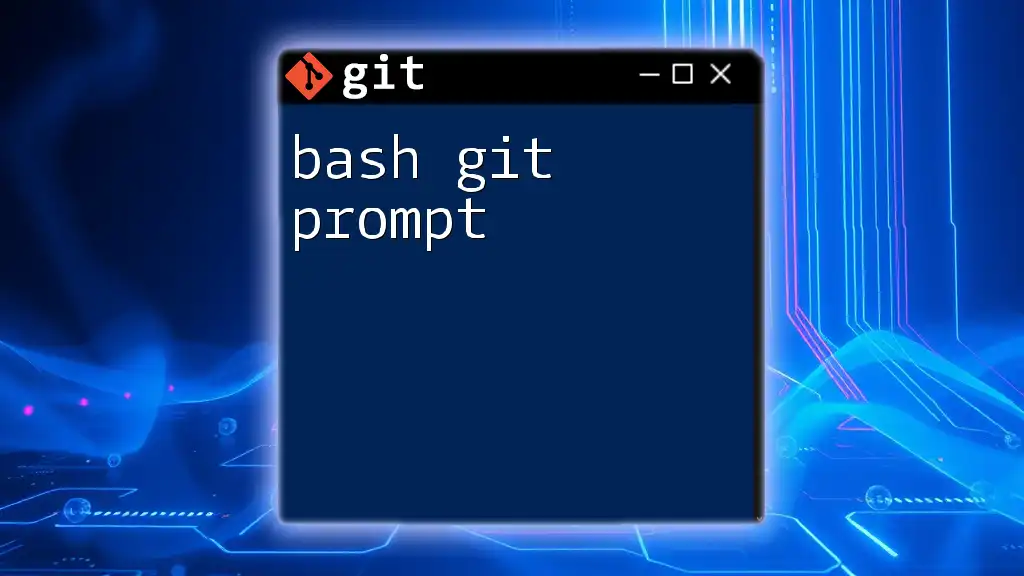
The `git pull` Command with Branches
General Syntax of `git pull`
The general syntax for `git pull` is as follows:
git pull <remote> <branch>
By default, if no remote or branch is specified, Git will pull from the current branch’s upstream.
Pulling Updates from a Remote Branch
When you want to pull updates from a remote branch, you would typically do so by running the command:
git pull origin main
In this example, `origin` is the name of the remote repository, and `main` is the branch you want to pull updates from. Upon execution, Git will first fetch the latest commits from the specified remote branch and then merge those commits into your current branch.
Handling Merge Conflicts
Despite the best preparations, conflicts may still arise during a pull operation. A merge conflict occurs when the changes from the remote branch conflict with your local changes. If you encounter a conflict, Git will let you know which files are in conflict.
To resolve such conflicts:
- Use `git status` to view which files are conflicted.
- Open the conflicted files, manually resolve the differences, and save.
- Add the resolved files using:
git add <filename>
- Finally, commit the changes:
git commit
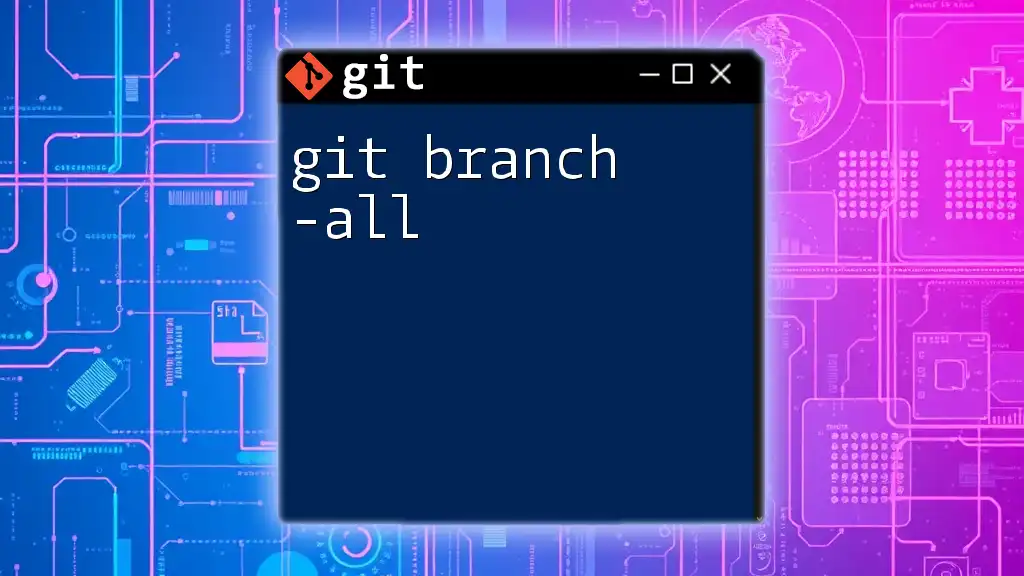
Best Practices for Using `git pull` with Branches
Pull Regularly
To ensure you are on the same page as your teammates, make it a habit to pull regularly. This frequency reduces the chance of significant conflicts, making integration smoother and less stressful.
Use Pull Requests
When collaborating on larger features, consider using a pull request workflow. Instead of pulling directly into the main branch, submit your changes as a pull request. This allows for code review and discussion, improving the overall quality of the code.
Clean Your Branches
Keeping your branches organized is essential for maintaining a tidy repository. After merging branches, consider using:
git branch -d <branch-name>
This command deletes the specified branch if it has been merged, helping you avoid confusion and clutter in your branch management.
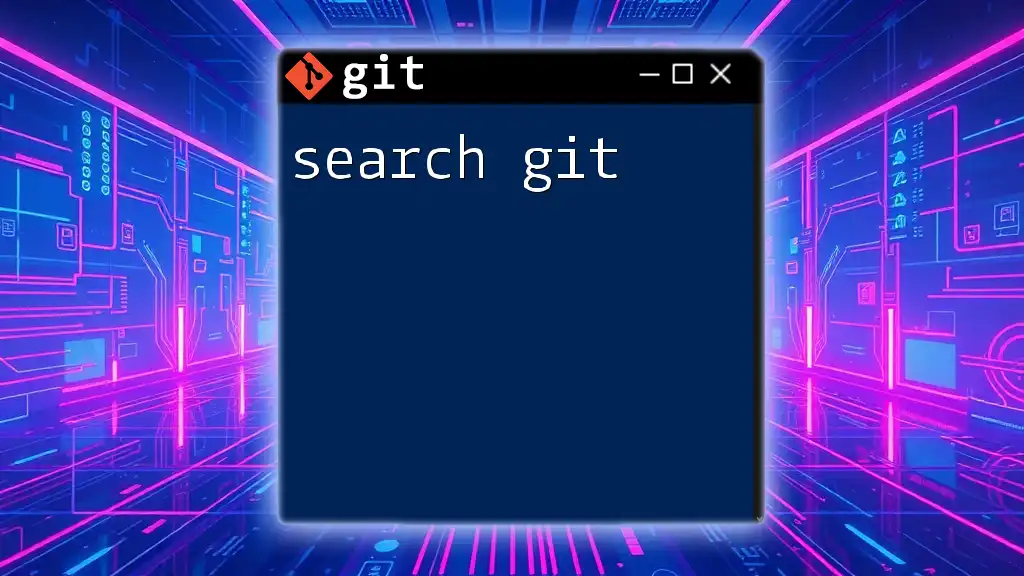
Advanced Usage of `git pull`
Pulling Changes Without Merging
Sometimes, you may want to pull updates but prefer to rebase them onto your current branch instead of performing a merge. You can accomplish this using the `--rebase` option:
git pull --rebase origin main
This command will apply the changes from the `main` branch on top of your current branch, resulting in a cleaner project history.
Pulling from Different Branches
If you want to pull changes from a branch that is different from your current branch, simply specify it in the command:
git pull origin feature-branch
This command pulls updates from `feature-branch` into your current branch, allowing you to incorporate changes effectively.
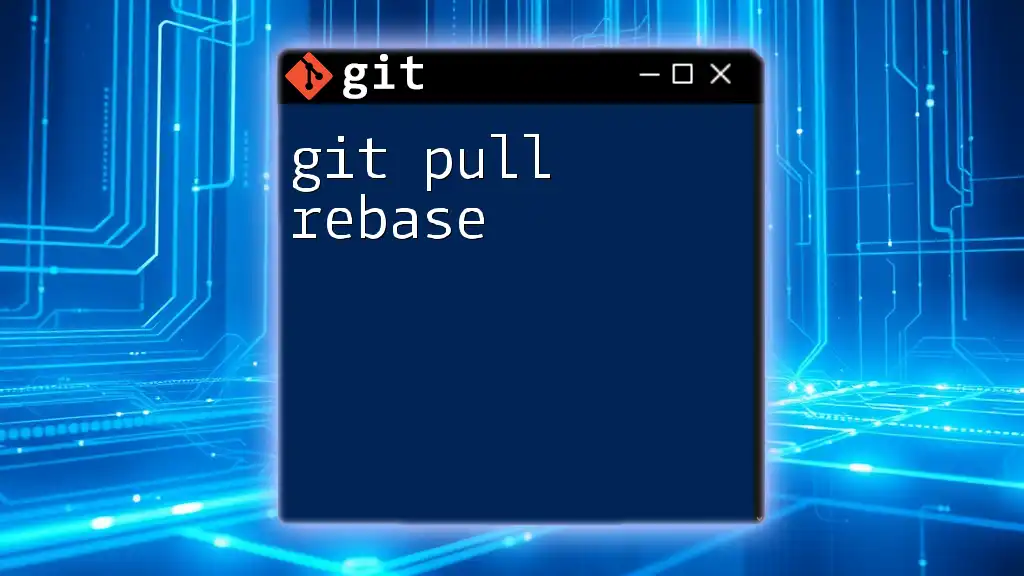
Conclusion
In summary, understanding how to effectively utilize `branch git pull` is vital for collaborative development. By regularly pulling changes, managing branches properly, and adhering to best practices, you can ensure a smoother workflow and a more organized codebase.
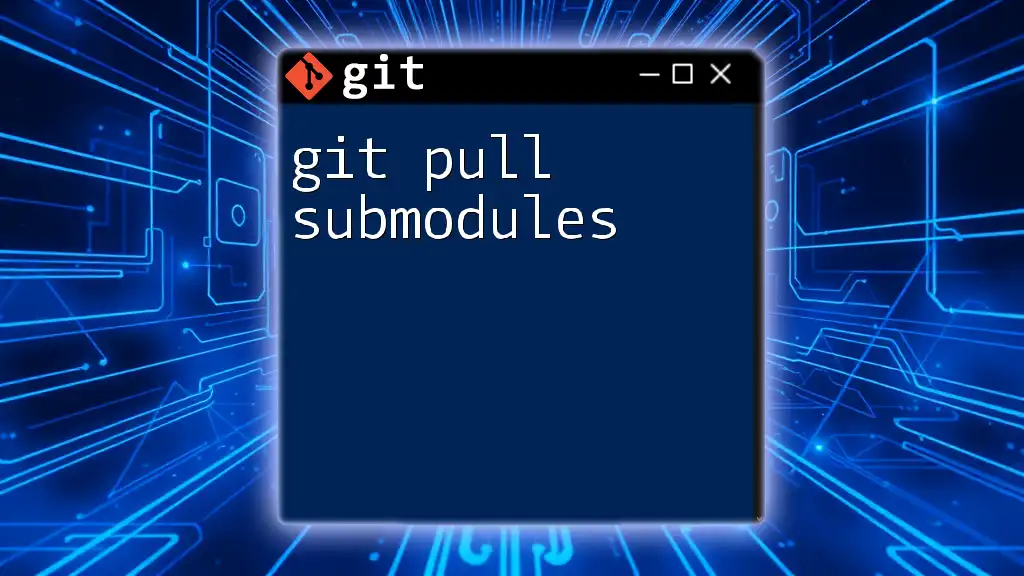
Additional Resources
- For further learning, consider diving into the official Git documentation.
- Explore online tutorials and courses to deepen your understanding of Git and branching strategies.
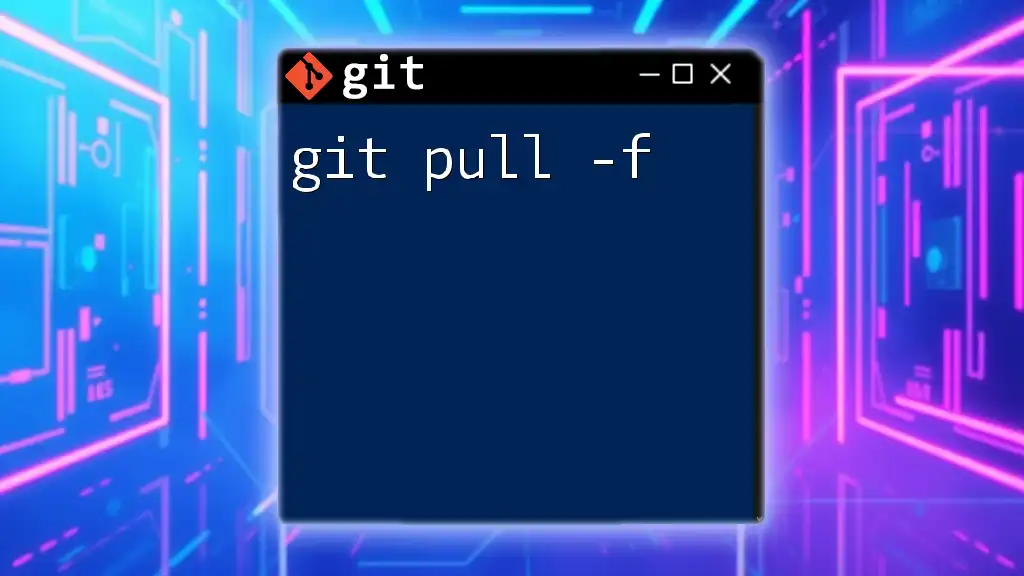
Code Snippets Repository
For your reference, consider maintaining a repository of all essential commands and examples shared throughout this guide to make your Git experience even smoother.