The `git branch -m` command is used to rename a branch in Git, allowing you to specify the current branch name and the new name you want to assign to it.
git branch -m old-branch-name new-branch-name
Understanding Git Branches
What is a Git Branch?
In Git, a branch represents an independent line of development within a repository. Using branches allows developers to work on features, fixes, or experiments without affecting the main codebase. The default branch created when you initialize a new Git repository is called `main` or `master`, depending on your Git version.
Why Use Branches?
Branches are crucial for a variety of reasons. They allow for experimentation without risking the main code. If a new feature fails or needs to be discarded, it's straightforward to delete that branch without impacting other development efforts. Furthermore, branches enable parallel development, where multiple team members can work on different features simultaneously without stepping on each other’s toes.
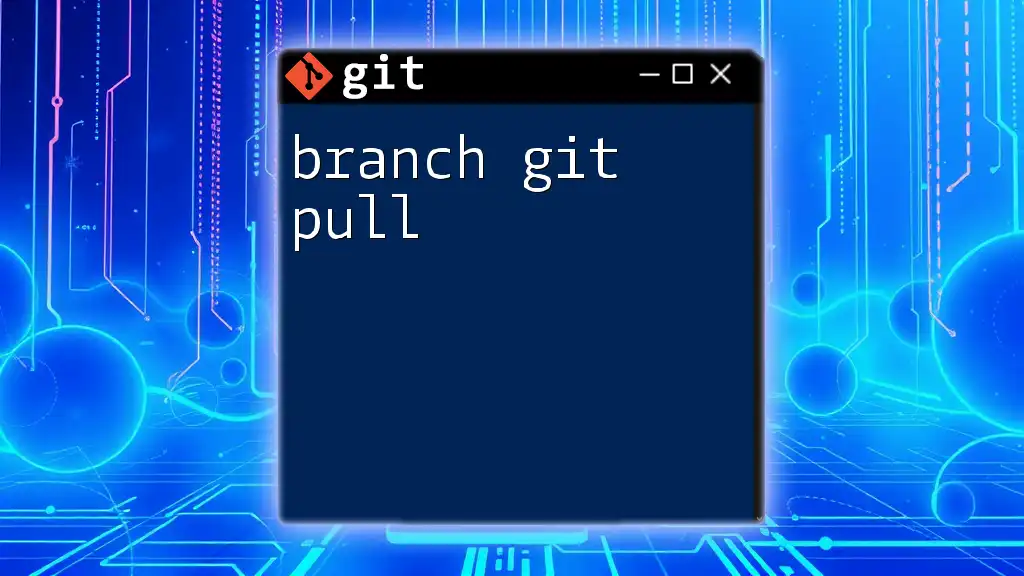
The `git branch` Command
Overview of the `git branch` Command
The `git branch` command is what you use to list, create, or delete branches in your repository. It's fundamental in navigating and managing your project’s development.
Important Note: The `git branch` command is different from `git checkout`. The former deals with branch management, while the latter allows you to switch between branches.
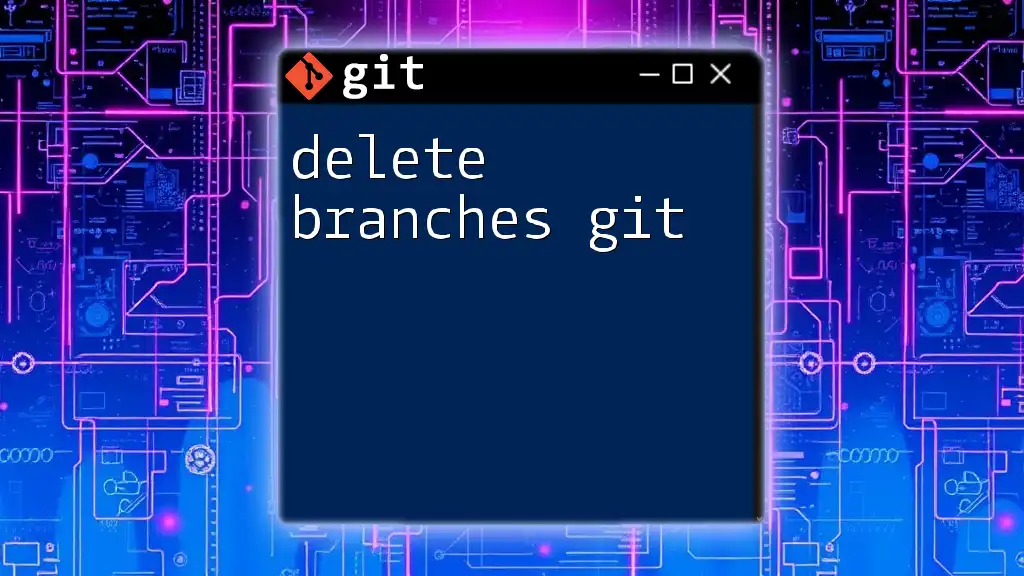
Renaming a Branch with `git branch -m`
What Does `git branch -m` Do?
The `-m` option stands for "move," and it's used to rename a branch. With this command, you can easily change the name of the current branch or any other branch without losing any work.
Syntax of `git branch -m`
The command syntax is straightforward:
git branch -m <old-branch-name> <new-branch-name>
You can use this command in two primary contexts: renaming the current branch or renaming a specified branch.
Usage when Renaming Current Branch
To rename the branch you are currently on, simply use the command without specifying an old branch name. For example, if you are on a branch called `feature/old-name` and want to rename it to `new-name`, you would do the following:
git checkout feature/old-name
git branch -m new-name
In the above example, the first command switches to the branch you intend to rename. The second command performs the actual renaming.
Usage when Renaming a Specific Branch
To rename a branch that you are not currently on, you will need to use the syntax specifying both the old and new names. For instance, if you have a branch called `old-branch-name` and want to rename it to `new-branch-name`, use:
git branch -m old-branch-name new-branch-name
This is particularly useful in scenarios where you might realize a need for a more descriptive branch name while working from another branch.
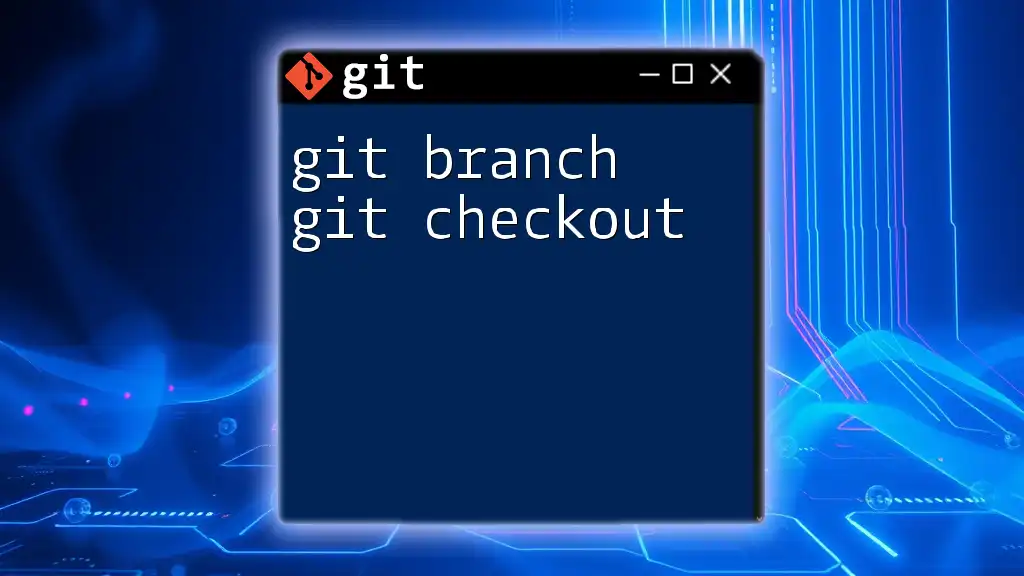
Common Use Cases
When to Rename a Branch
There are several scenarios where renaming a branch makes sense. Perhaps you misspelled a branch name initially or need to align it with updated naming conventions or feature titles. Renaming allows for better clarity and understanding when navigating through branches in a repository.
Best Practices for Naming Branches
When choosing your branch names, consider these simple guidelines:
- Be Descriptive: Use meaningful names that describe the content of the branch, such as `feature/login-system` or `bugfix/typo-fix`.
- Use Consistent Formatting: Stick to a naming convention that your team agrees on (e.g., using hyphens, underscores, or slashes).
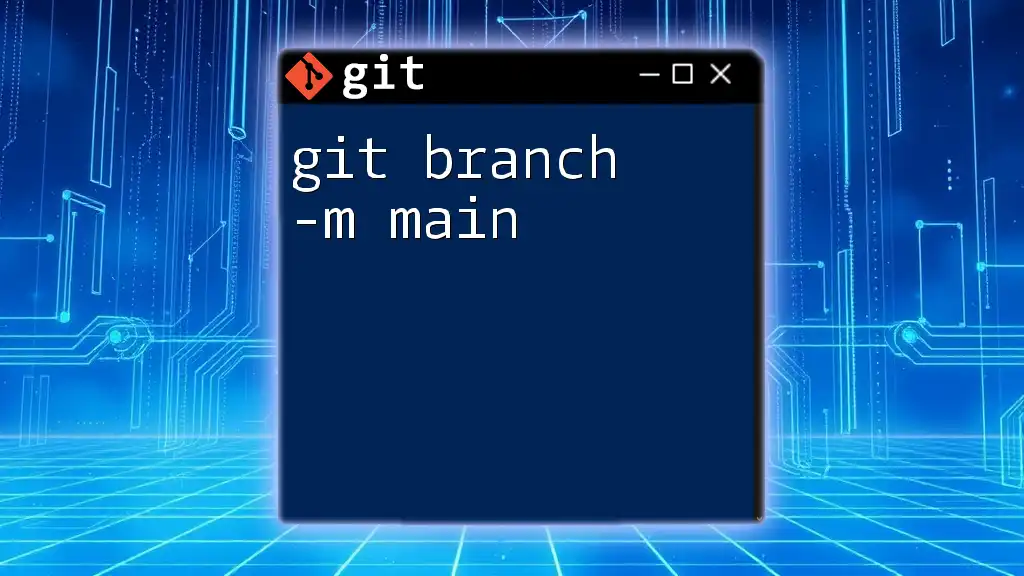
Handling Errors and Conflicts
Common Errors While Using `git branch -m`
While using the `git branch -m`, you might encounter some common errors. One such error occurs if you attempt to rename a branch to a name that already exists:
fatal: A branch named 'new-name' already exists.
How to Resolve Conflicts
If you encounter errors, the first step is to check your existing branches using the command:
git branch
This command will show you all current branches, allowing you to determine if the name you're trying to use is already taken. If it is, you'll need to choose a different name or delete the existing branch if it's no longer needed.
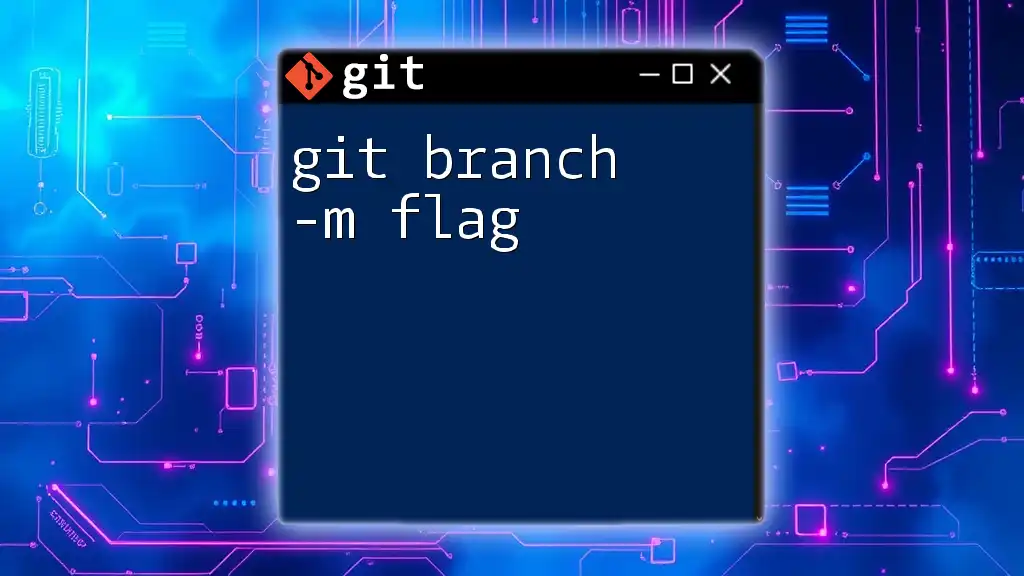
After Renaming a Branch
Updating Remote Branches
Renaming branches locally does not automatically update the repository on a remote server. After renaming a branch locally, it's crucial to ensure the remote branches are in sync. To do so, you can follow these steps:
-
Delete the old branch from the remote server:
git push origin --delete old-branch-name
-
Push the new branch to the remote server:
git push origin new-branch-name
-
Set the upstream for the newly renamed branch:
git push --set-upstream origin new-branch-name
By following these steps, you'll ensure that your team’s remote repository reflects the changes made locally.
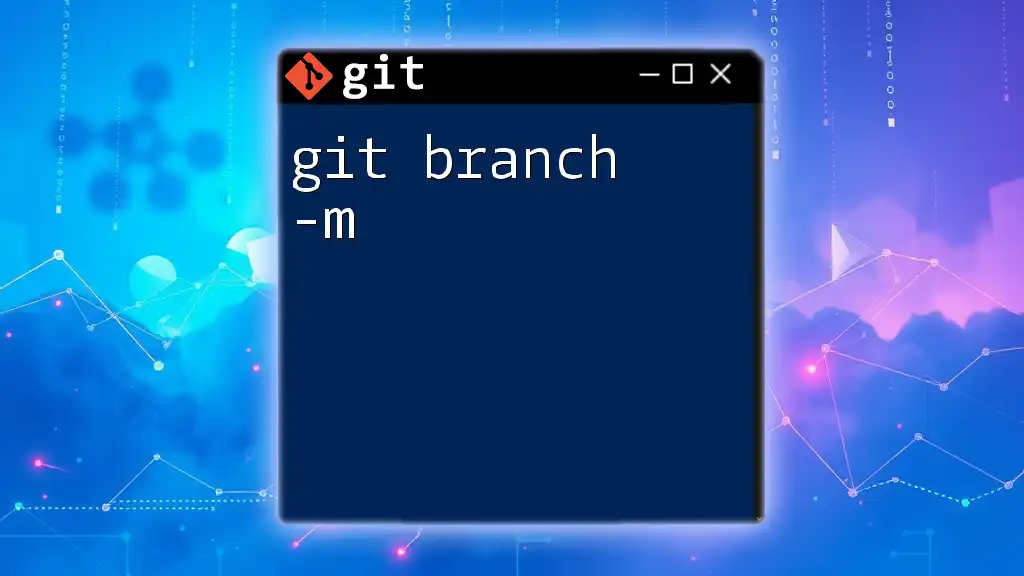
Conclusion
In this article, we’ve explored the importance of branches in Git and provided a deep dive into the `git branch -m` command. Remember, renaming branches can streamline your workflow and enhance clarity within your team's version control practices. Emphasizing clear naming conventions and resolving any conflicts will set a solid foundation for efficient collaboration. Continue exploring additional Git topics to further strengthen your version control skills!
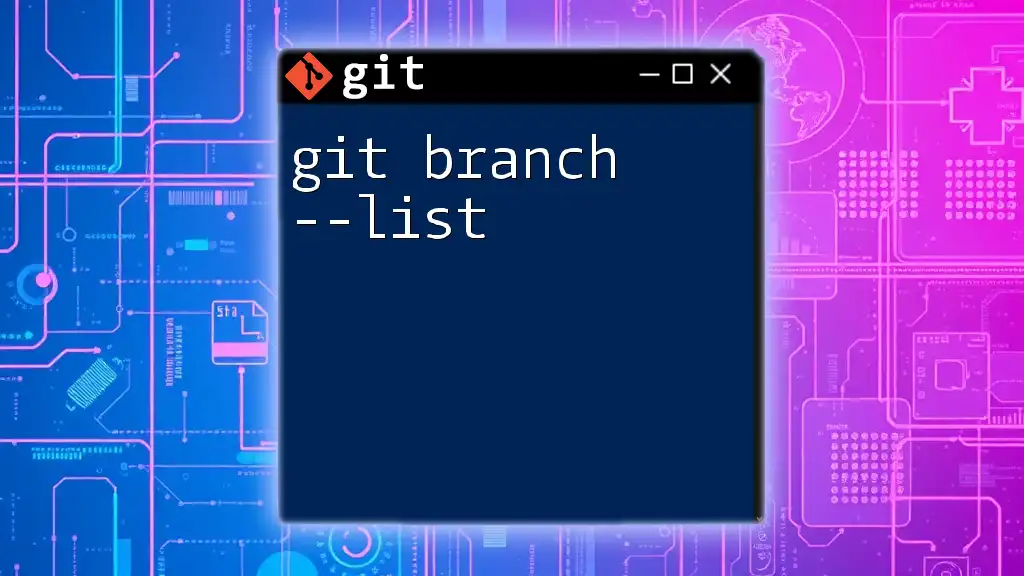
References and Further Reading
For those who want to delve deeper into the world of Git, consider checking out official documentation, tutorials, and books on Git best practices and workflows. Understanding Git thoroughly will greatly aid in managing your projects effectively.