The `git` command-line tool allows you to search through your repository's history and files using various commands such as `git grep` and `git log`.
Here’s a useful code snippet to search for a specific string in your project:
git grep "search_string"
Understanding Git Search
What is Git Search?
Git search refers to the functionalities available within Git that enable users to efficiently locate information within their repositories. Whether you need to find a specific commit, track changes in a file, or identify branches and tags, mastering the art of searching in Git is crucial for effective version control. Efficient searching not only saves time but also enhances productivity, allowing developers to focus more on coding rather than hunting for historical data.
Different Types of Searches in Git
When utilizing Git, there are primarily three types of searches you can perform:
- Searching for commits – Identifying specific changes made to the codebase over time.
- Searching for files – Locating files within the repository, including their contents.
- Searching through branches and tags – Navigating through various project versions and releases.
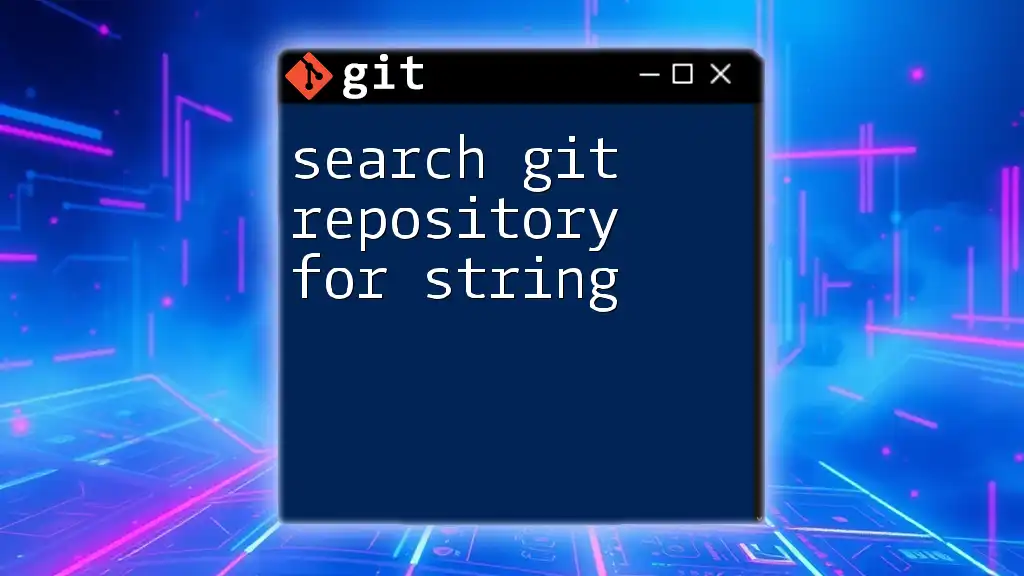
Searching for Commits
Using `git log` to Search Commits
One of the most powerful commands in Git for searching through commit history is `git log`. This command provides a chronological list of commits along with associated details such as authorship and timestamps.
To get started with the basic command, simply enter:
git log
You can refine your search further with various options:
- Filtering by author allows you to see all commits made by a specific author. For instance, to find commits by "Alice", use:
git log --author="Alice"
- If you need to search by date, you can specify a range using the `--since` and `--until` flags. For example:
git log --since="2023-01-01" --until="2023-01-31"
This command will filter commits made in January 2023.
- Another useful option is searching for commit messages containing specific terms. For instance, if you're looking for commits related to a "bug fix":
git log --grep="fix"
Using `git show`
Once you have identified a specific commit of interest, you can view its details using the command `git show`:
git show <commit-hash>
Replace `<commit-hash>` with the actual hash of the commit. This command reveals all the changes made in that particular commit, including diffs and commit messages, providing crucial context.
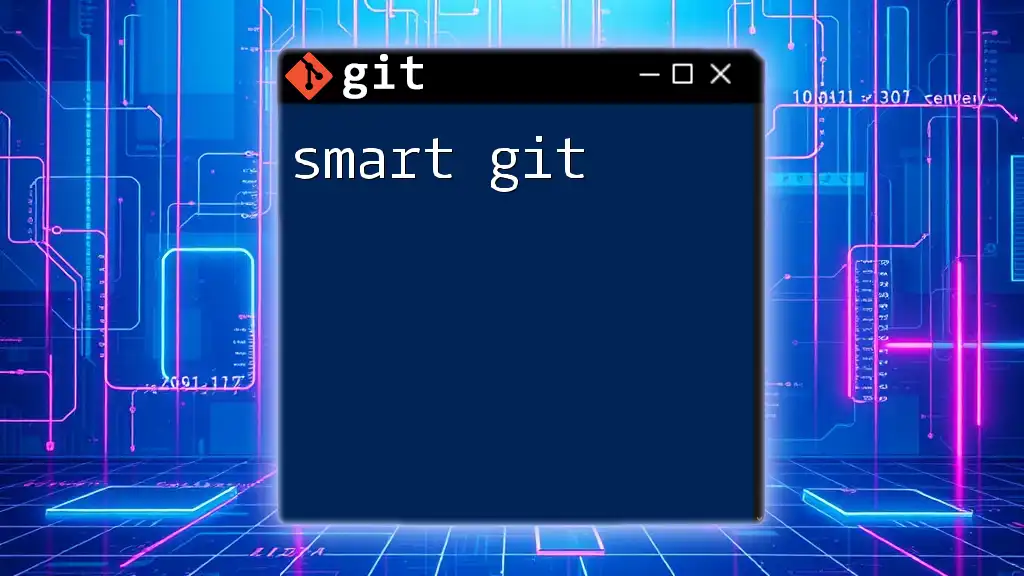
Searching for Files
Using `git ls-files`
To view all files tracked by Git in your repository, you can utilize the `git ls-files` command. This command lists all files in the current directory or the entire project.
Here's how to execute it:
git ls-files
If you need to filter files by pattern, such as finding only `.txt` files, you can do this:
git ls-files '*.txt'
Using `git grep` for Code Search
When searching for specific content within files—such as a function name or a keyword—`git grep` is the command you need. This command searches for specified patterns within the tracked files of your repository.
For example, to find all occurrences of the keyword "functionName":
git grep "functionName"
This command will quickly display all instances of "functionName" along with the respective file names and line numbers, facilitating easy navigation to the relevant code sections.
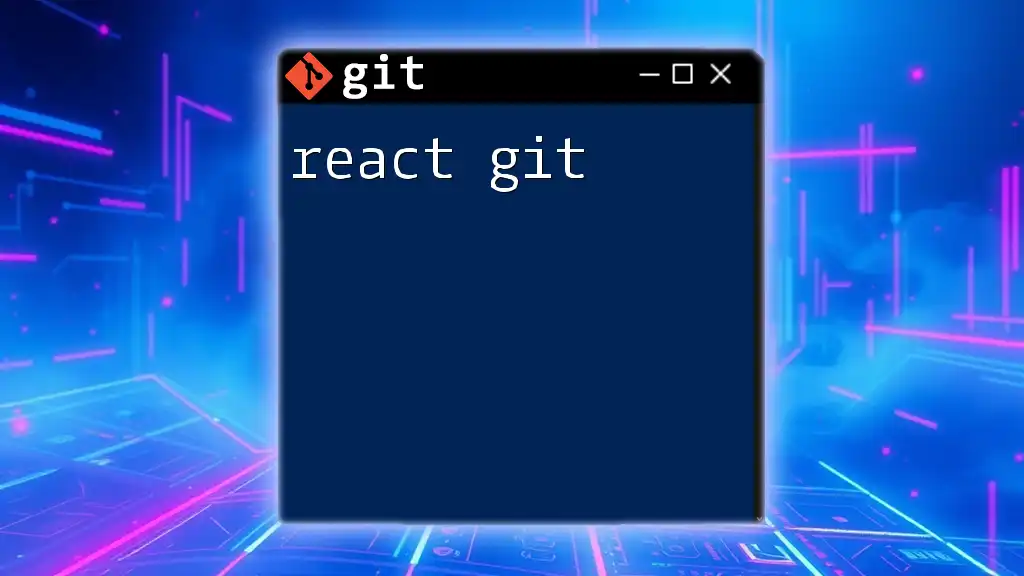
Finding Branches and Tags
Searching for Branches
To view and search through branches in your Git repository, you can use the `git branch` command. The command by itself will list all local branches:
git branch
If you're searching for branches that contain a specific term or pattern, you can filter the list with:
git branch --list '*feature*'
This command would show all branches containing the term "feature" in their names, streamlining the process of finding relevant branches for your work.
Searching for Tags
Tags are essential for marking specific points in your project's history, often used for releases. To view all existing tags, you can simply run:
git tag
To filter and search through tags for specific patterns (like version numbers), you can also use:
git tag -l '*v1.*'
This command will help you locate all tags that follow the specified versioning scheme.
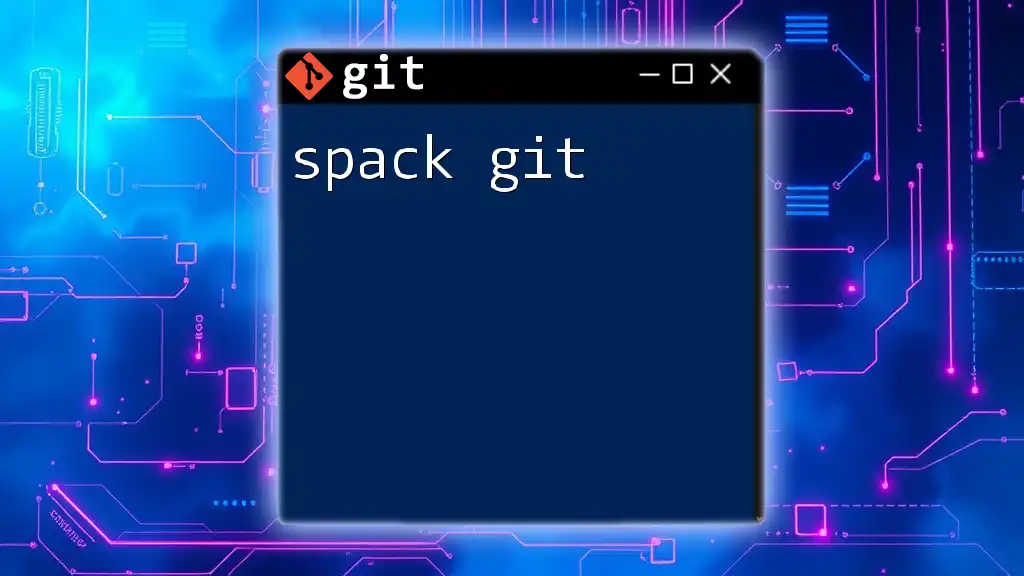
Navigating the Search Results
Understanding Output and Options
When you execute any of the search commands in Git, understanding the output is vital. The information provided usually contains a chronological listing with detailed commit messages, author information, and more. To format and enhance the readability of your logs, you can use the `--pretty` option. For example:
git log --pretty=format:"%h - %an, %ar : %s"
This command simplifies the log format, making it more user-friendly.
Paging Through Results
If your search results are extensive, viewing them all on one screen might be overwhelming. To handle larger outputs, you can pipe results through a pager like `less`:
git log | less
This will allow you to scroll through your log results easily with keyboard navigation, making it especially handy when dealing with a large commit history.
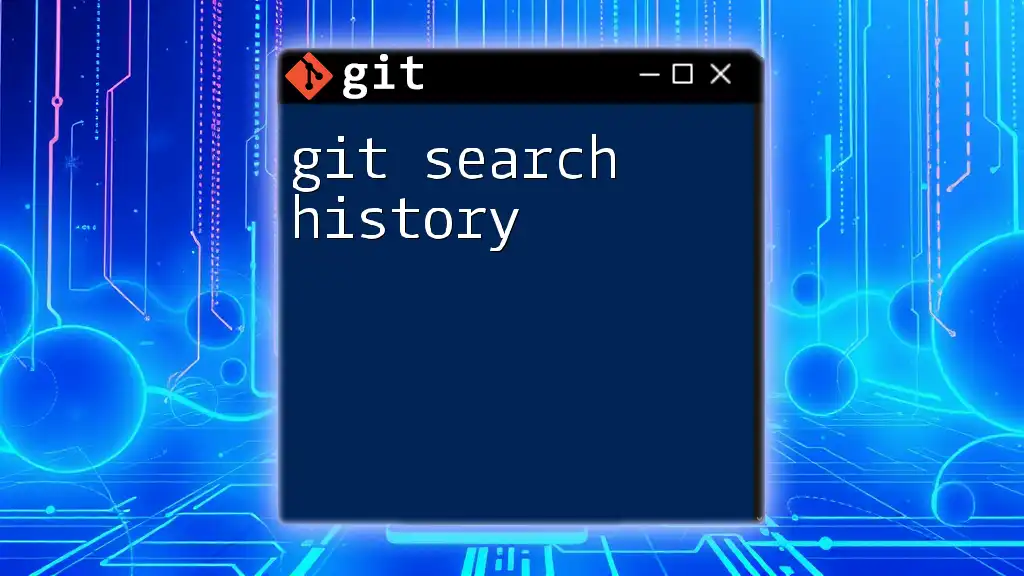
Advanced Search Techniques
Searching in Specific File Types
If you want to focus your search on particular file types, `git grep` allows you to specify file type filters. For example, you can search for occurrences of "searchTerm" only within Python files:
git grep -- '*.py' -e "searchTerm"
This is particularly useful for large projects where different file types are present.
Combining Multiple Search Options
For more refinement in your search results, you can chain multiple filters together. This is particularly helpful when dealing with complex queries. For instance, if you're searching for bug fixes authored by "John," you can combine options like this:
git log --grep="bugfix" --author="John"
This command will give you a concise set of results that fit your specific criteria.
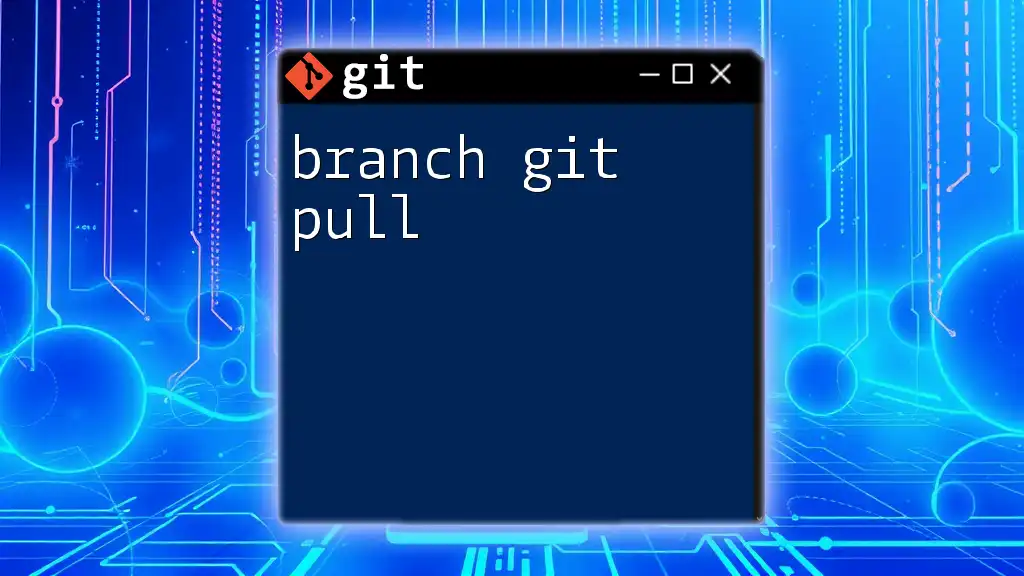
Practical Use Cases
Real-world Examples of Searching in Git
Understanding how to utilize Git search commands can significantly streamline your development workflow. For instance, if you need to track down a bug, you can quickly grep commit messages for "bug" to find relevant changes or reviews. When onboarding new team members, searching for files or functions will help them acclimate to the codebase swiftly.
Additionally, during a review process, you can combine different search techniques to gather the necessary information to make informed judgments on code quality and changes.
By incorporating these search practices into your daily Git usage, not only do you save time, but you also develop a more proactive approach to version control.
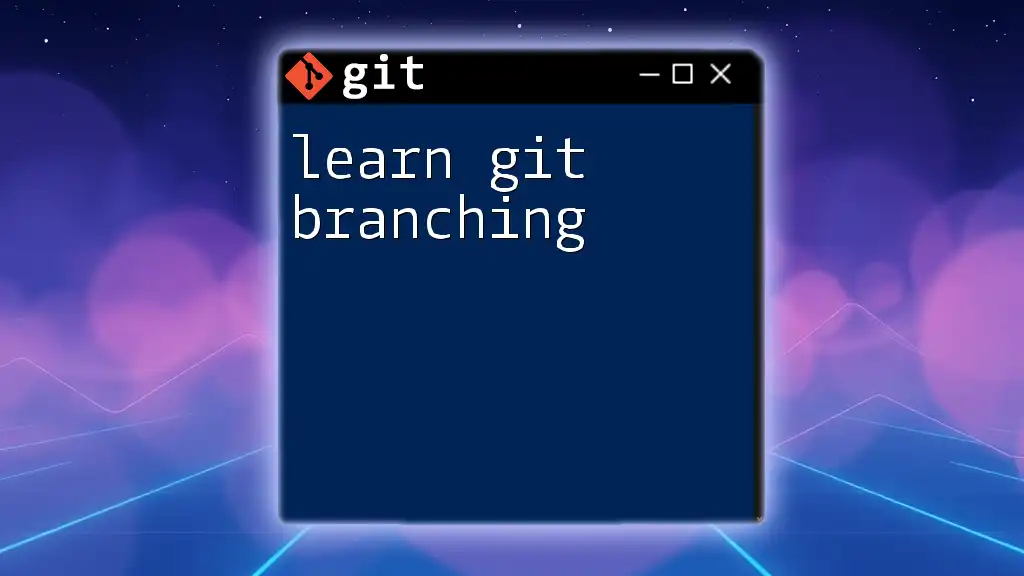
Conclusion
Learning how to search git effectively can transform your experience in using Git from one of occasional confusion to seamless productivity. As you integrate these searching strategies into your routine, remember that efficiency and accuracy in locating code changes are key components of successful software development.
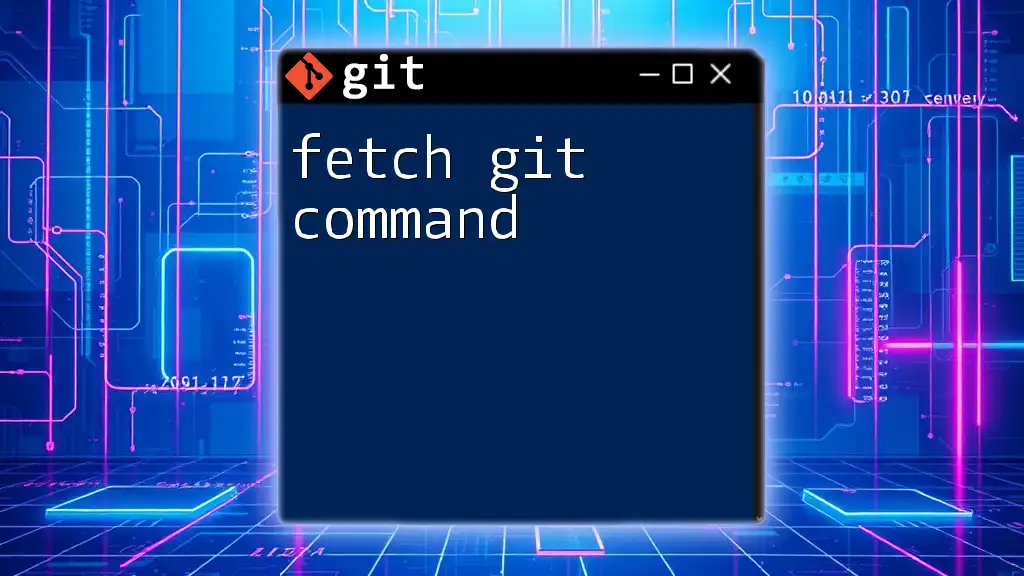
Call to Action
Ready to take your Git skills to the next level? Practice these commands in your own projects and explore additional resources. Stay curious and keep enhancing your understanding of Git; your future self will be grateful!