The `git fetch` command retrieves updates from a remote repository without merging them into your local branch, allowing you to see changes before deciding to integrate them.
git fetch origin
Understanding the Git Fetch Command
What is Git?
Git is a powerful distributed version control system used by developers to manage changes in their projects efficiently. It facilitates collaboration among multiple team members, allowing them to work on the same codebase without overwriting each other's changes. Git helps in tracking modifications, reverting to previous stages, and maintaining the integrity of code.
What Does `git fetch` Do?
The `git fetch` command is a key operation in Git that allows you to retrieve updates from a remote repository without merging those changes into your current branch. This command downloads new data from a remote repository, such as commits, branches, and tags, and saves them to your local repository, making it possible to review them without affecting your local work.
It is crucial to distinguish between `git fetch` and `git pull`. While `git pull` fetches changes and merges them in one step, `git fetch` is purely about retrieving updates. This separation allows developers to examine changes before integrating them into their own branches.
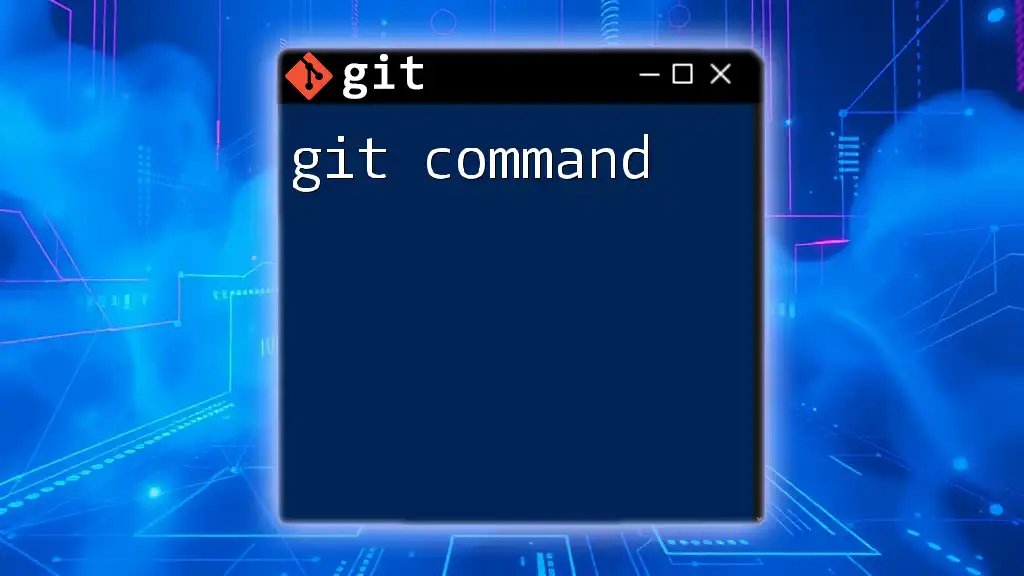
The Mechanics of Fetching Data with Git
How `git fetch` Works
When you run `git fetch`, Git connects to the remote repository and retrieves any changes that have occurred since your last fetch or clone. These changes go to remote-tracking branches in your local repository; they do not affect your working directory or currently checked-out branch. This makes it an excellent way to update yourself on a project while keeping your local changes intact.
Fetching Specific Branches
To fetch a specific branch from a remote repository, you can use:
git fetch origin branch-name
In this instance, `origin` refers to the default name for your remote repository, and `branch-name` is the name of the branch you want to fetch. This will update the corresponding remote-tracking branch in your local environment with changes from the remote branch.
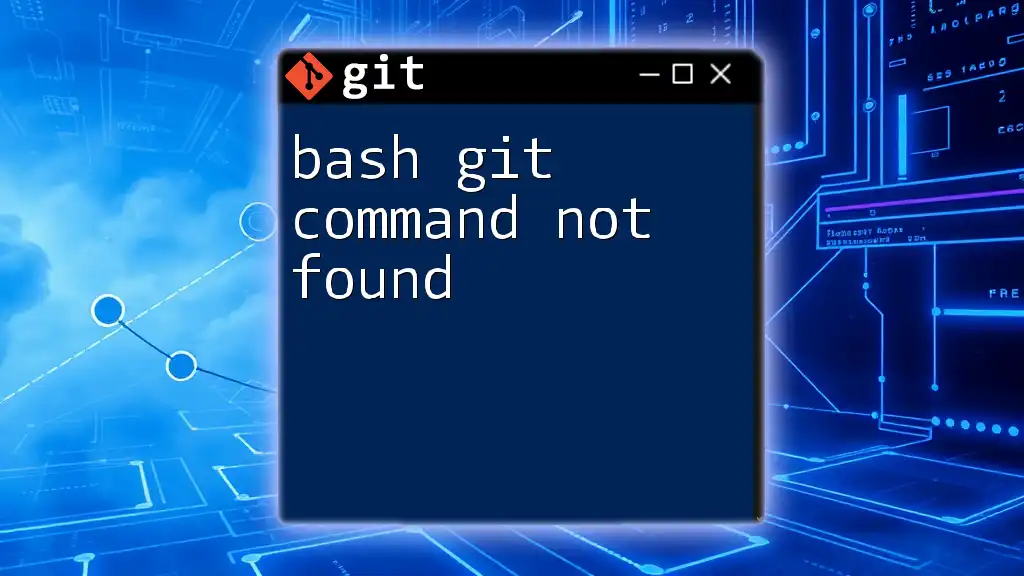
When to Use `git fetch`
Scenarios for Fetching
There are several scenarios where utilizing the `fetch git command` becomes particularly valuable. For instance, when collaborating with others, fetching allows you to check for any updates made by teammates before merging those changes into your own branch. It provides an opportunity to review changes, ensuring you are fully informed before taking actions that impact your code.
Fetching Before Merging
Before performing a merge or rebase, fetching is best practice. This helps you ensure that you're working with the most recent changes. For example, you would first run:
git fetch origin
After fetching, you can then merge it into your local branch with:
git merge origin/branch-name
This workflow updates your local repository with current changes before you introduce your modifications, helping to minimize conflicts.
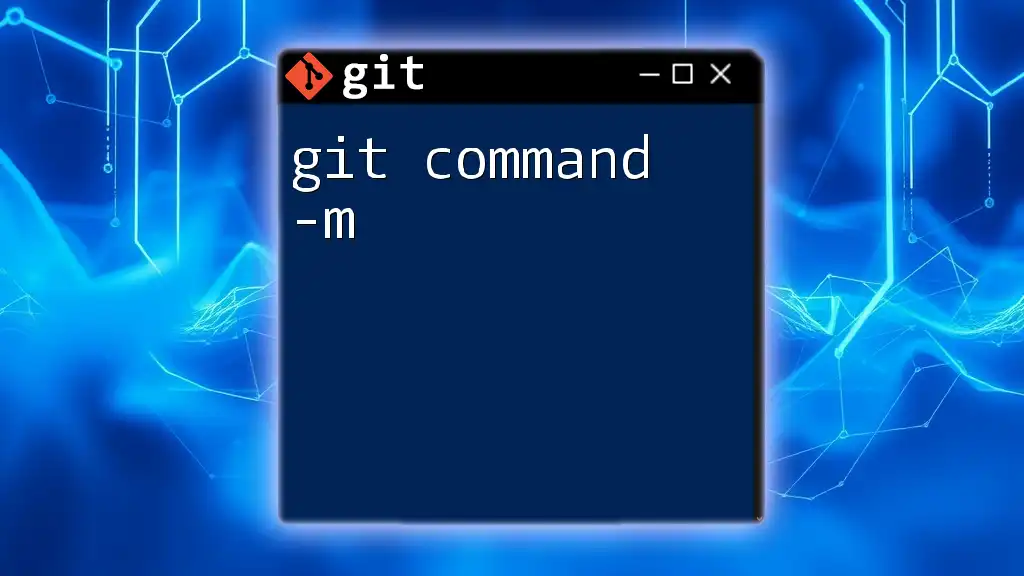
Using `git fetch` Effectively
Fetching All Branches
To fetch all branches at once from the remote, you can execute:
git fetch --all
This command is advantageous in larger projects where multiple branches may be actively developed. By fetching all branches, you ensure you have a complete view of the project's progression without needing to fetch each branch individually.
Viewing Fetched Changes
After fetching changes, you can view what has been updated in the remote repository. Use the following commands:
git log origin/branch-name
or
git diff origin/branch-name
These commands allow you to inspect the commit history and see the differences introduced in the fetched branch, providing clarity before merging it into your own work.
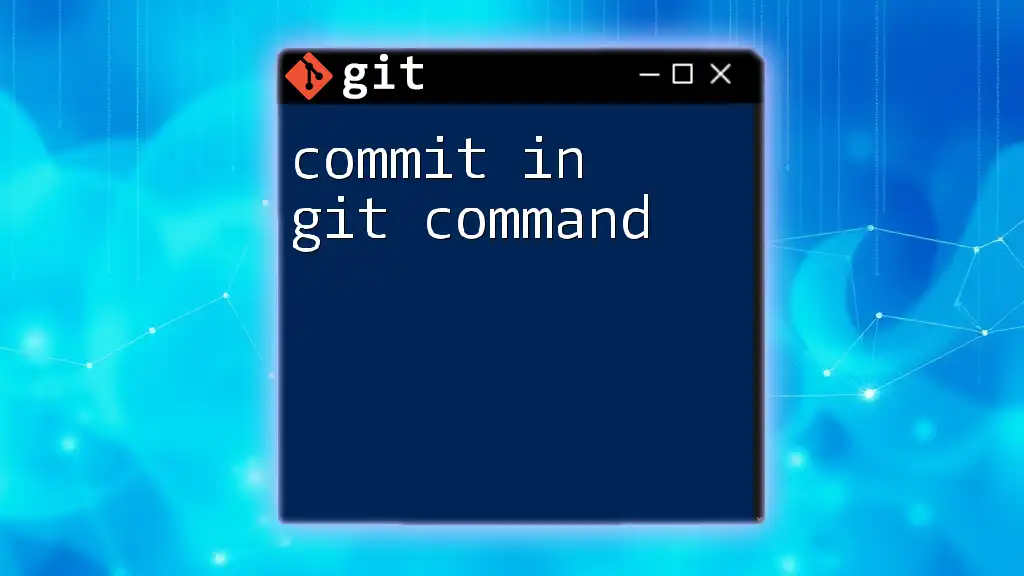
Advanced `git fetch` Techniques
Using Tags with Fetch
You can also fetch tags, which may be crucial for versioning with:
git fetch --tags
Tags are often used in release management to mark specific points in history as important, like version releases. Fetching these tags helps keep your local tracking in sync, ensuring you can reference them as needed.
Fetching with Prune
The `--prune` option can be combined with fetch to clean up your local repository:
git fetch --prune
This command removes references to branches that no longer exist on the remote, helping to keep your workspace tidy and focused on active branches.
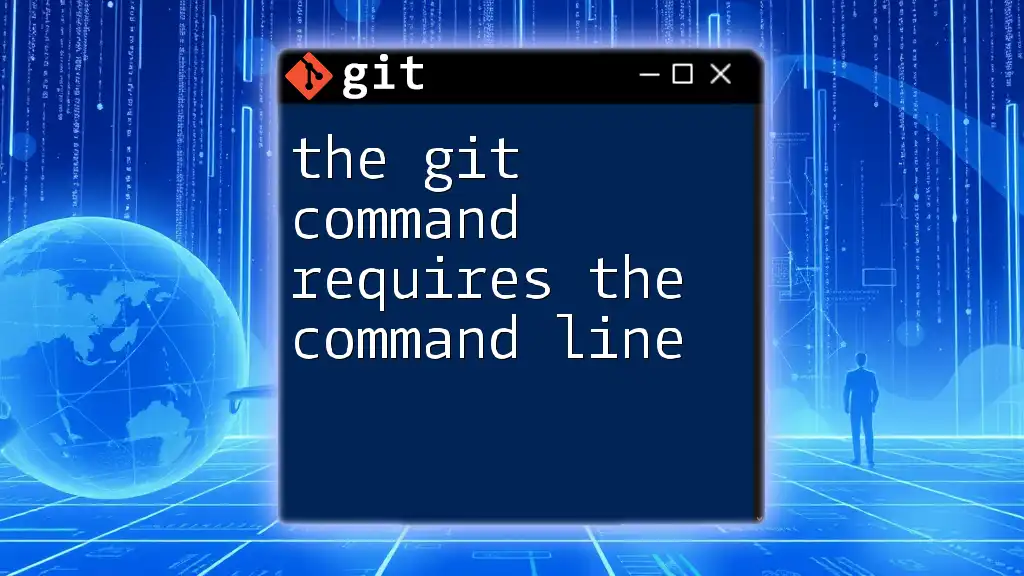
Common Use Cases and Best Practices
Best Practices for Using `git fetch`
To maintain an efficient workflow, regularly employing the `fetch git command` can help keep your local repository in sync with the remote. Make a habit of fetching before significant operations like merging or rebasing. This ensures that you’re always working on the most recent version of the project and helps avoid complicated merge conflicts.
Troubleshooting Fetch Issues
Occasionally, you may encounter problems when fetching. Common issues include connectivity problems or incorrect remote repository URLs. To check your configured remote repositories, use:
git remote -v
This command lists all remote repositories and their respective URLs, allowing you to verify that they are set correctly.
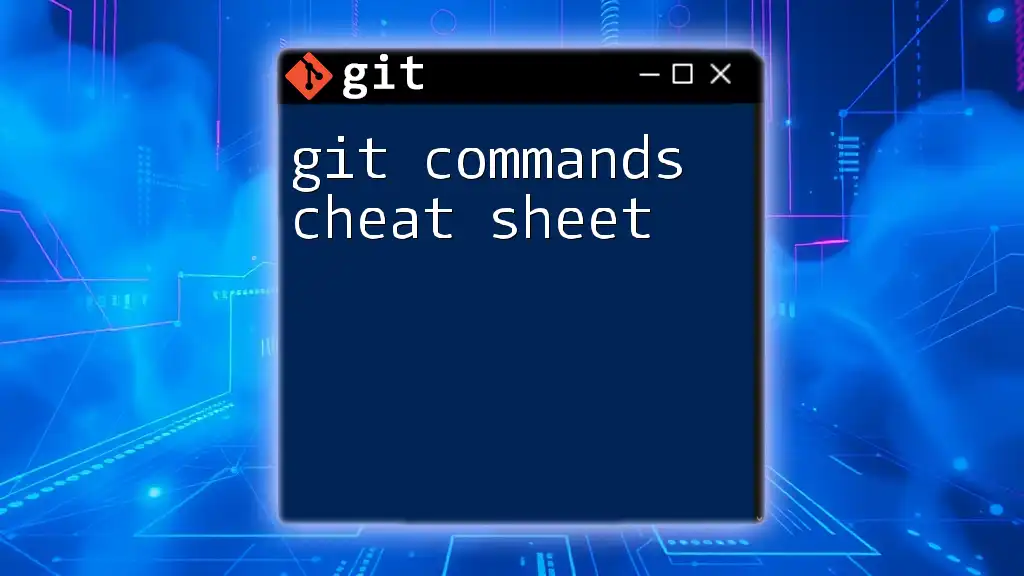
Summary
Key Takeaways
In summary, the `fetch git command` serves as a fundamental tool for keeping your local repository updated with changes from a remote source. It allows for an organized workflow, where changes can be evaluated and merged deliberately, reducing the risk of conflicts and errors.
Encouragement for Further Learning
As you gain comfort with `git fetch`, consider diving deeper into other crucial commands like `git pull`, `git merge`, and more intricacies of branch management. Understanding how these commands interrelate will vastly improve your proficiency in Git and enhance your collaborative development experience.