The Git command-line interface (CLI) requires users to input commands directly into the terminal to perform version control tasks efficiently.
Here’s an example of a basic Git command to initialize a new repository:
git init
Understanding Git and Its Command Line Interface
What is Git?
Git is a powerful and widely used version control system that helps developers manage and track changes to their code over time. It allows multiple collaborators to work on a project simultaneously, maintaining the integrity of the files while preserving a complete history of all changes. Key features of Git include branching, merging, and committing – all of which can be performed efficiently from the command line.
Importance of the Command Line in Git
The command line interface (CLI) is crucial for effectively using Git, as it provides direct and immediate access to Git’s features and functions. Unlike graphical user interfaces (GUIs), which may abstract away underlying commands, the command line enables users to manage their repositories at a deeper level. The speed and precision afforded by the command line can significantly enhance productivity, especially for both novice and expert users alike.
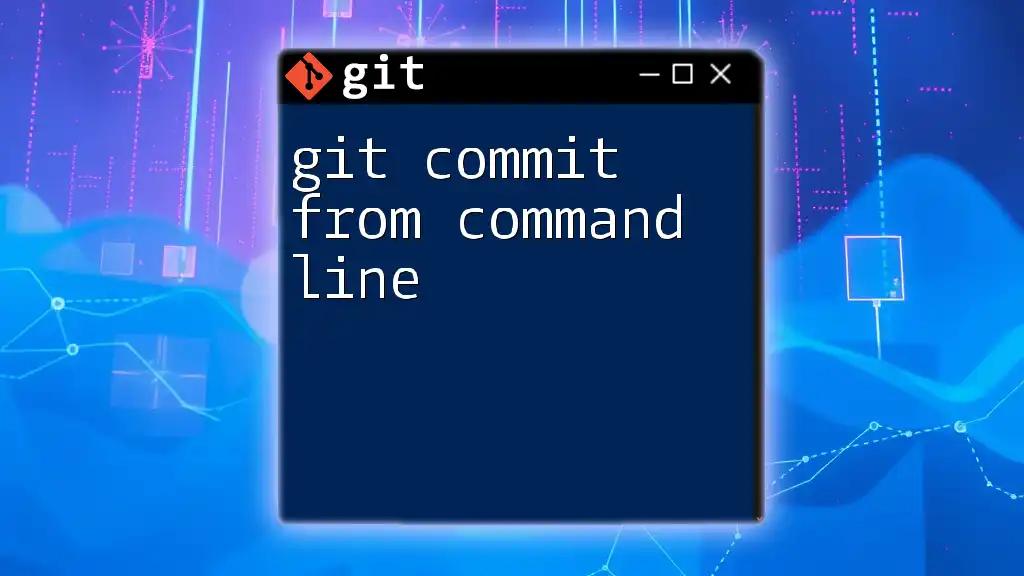
Getting Started with the Command Line
Setting Up the Command Line Environment
To utilize Git via the command line, the first step is to install Git on your system. This can be accomplished on various operating systems.
- On Windows: Download the installer from the official Git website. Follow the prompts to complete the installation.
- On Mac: Use Homebrew with the command `brew install git` or download directly from the Git website.
- On Linux: Use your package manager, for example, `sudo apt-get install git` for Ubuntu-based systems.
Familiarize yourself with popular command line tools, such as Terminal for Mac/Linux and Command Prompt or PowerShell for Windows. These interfaces will serve as your gateway to issuing Git commands.
Basic Command Line Navigation
Knowledge of basic commands for navigation is essential. Here are a few fundamental commands that will help you move around your files and directories:
- `cd [directory]` - Change to the specified directory.
- `ls` - List the files in the current directory (or `dir` on Windows).
These commands empower you to locate your Git repositories and access the files within them.
Customizing the Command Line Experience
You can enhance your command line experience by customizing it in a way that benefits your workflow:
-
Using Aliases for Git Commands: Simplify lengthy commands by creating aliases. For instance, instead of typing `git status`, you can set an alias like so:
git config --global alias.s status
-
Change Prompt Appearance: Adjusting the command line prompt can improve usability. You can modify it to show the current Git branch, making it easier to manage multiple branches.
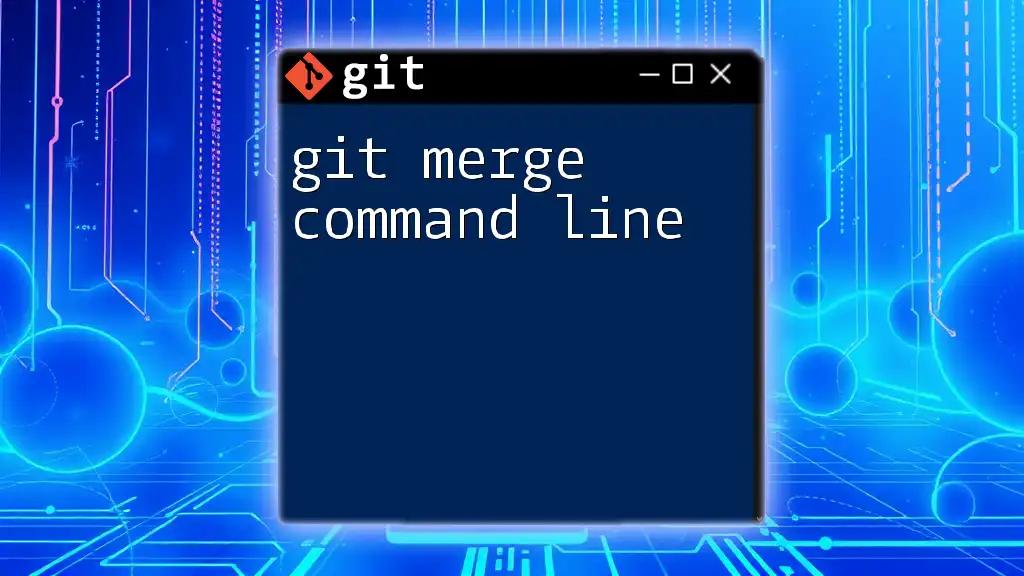
Core Git Commands Using the Command Line
Creating a New Repository
To begin using Git, you need to create a repository. This can be achieved with the command:
git init
This command initializes a new Git repository in the current directory. After running this, you can start tracking files and making commits. Initial commits are vital as they establish the beginning of your project’s history.
Cloning an Existing Repository
To work on an existing project, use the following command to clone it:
git clone [repository URL]
This command creates a copy of a remote repository locally. Cloning is efficient because it retains the entire commit history, allowing you to work on a local version of the project seamlessly.
Basic Workflow with Git Commands
Staging Changes
Staging changes is a critical step before committing your work. Use the command:
git add [file]
or to stage all changes at once:
git add .
Staging indicates which changes are ready to be included in the next commit. This step is essential because it allows you to selectively choose what changes go into your version history.
Committing Changes
Once changes are staged, perform a commit to save those changes in your repository's history:
git commit -m "Your commit message here"
Crafting good commit messages is important. Aim for concise yet descriptive messages that explain the changes made. A well-written commit message helps collaborators (and your future self) understand the project history.
Viewing Repository Status
It’s always useful to check the status of your repository. This can be done with:
git status
This command provides a view of staged changes, unstaged changes, and files not being tracked. Keeping track of your repository’s status is key to avoiding confusion during workflows.
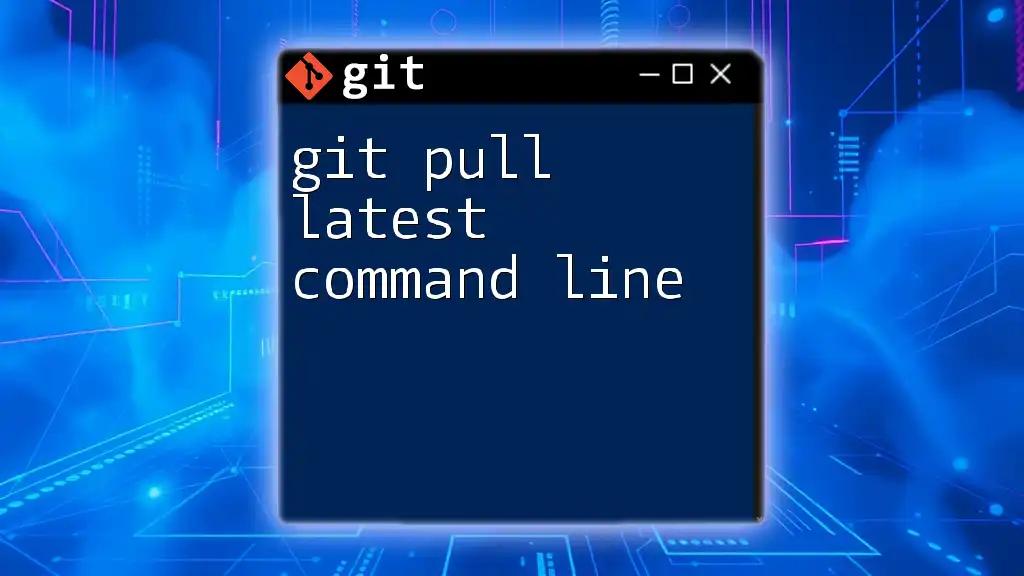
Advanced Command Line Git Commands
Branching and Merging
Branching allows you to work on features or fixes independently. You can create a new branch with:
git branch [branch-name]
Switch to that branch with:
git checkout [branch-name]
Once the new feature is ready, you can merge it back into the main branch:
git merge [branch-name]
Merging integrates changes from one branch into another. Understanding this process is crucial for collaborating effectively within teams.
Dealing with Conflicts
Conflicts may arise when merging branches—particularly if changes were made to the same line in both branches. Git will pause the merging process and prompt you to resolve the conflicts. Commands like:
git status
will help you identify the conflicts, which need to be manually resolved in the code before finalizing the merge.
Undoing Changes
Mistakes happen. When you need to undo changes, you can use:
git reset [file]
to unstage a file, or:
git revert [commit]
to create a new commit that undoes a previous commit. Knowing how to safely undo changes is essential for maintaining a clean project history.
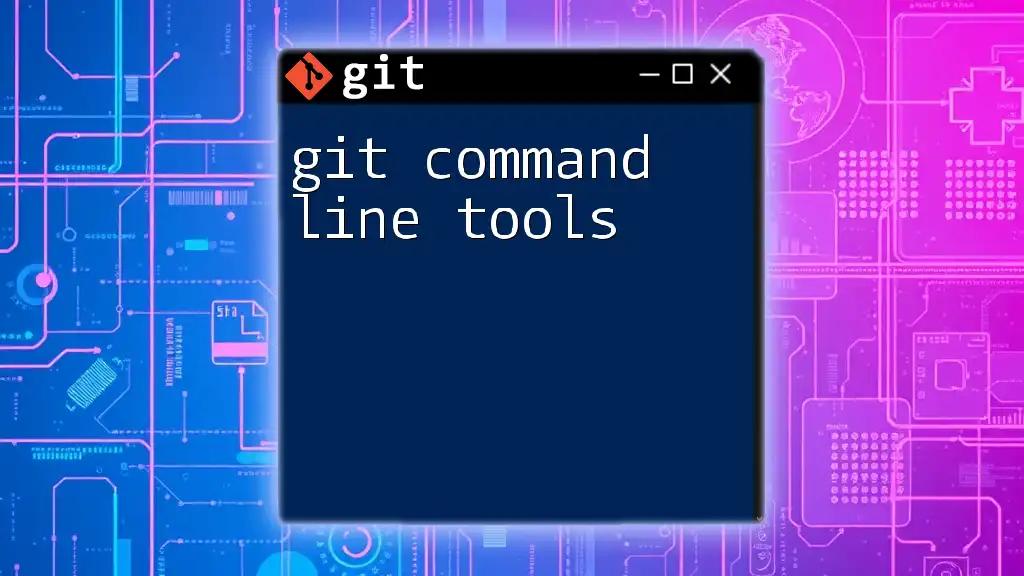
Benefits of Using Command Line for Git
Speed and Efficiency
The command line allows for rapid entry of commands. By memorizing frequently used commands and leveraging shortcuts, you can significantly cut down on time spent navigating through your project.
Full Access to Git Features
Many advanced features in Git are only accessible via the command line. For example, commands such as `git cherry-pick`, `git rebase`, or `git stash` offer functionality that is crucial for complex workflows and are often absent from GUIs.
Better Control Over Version History
Using the command line gives you precise control over your commit history. You can create, modify, and delete commits in ways that are more difficult through a graphical interface. This level of control allows for a more tailored approach to version management.
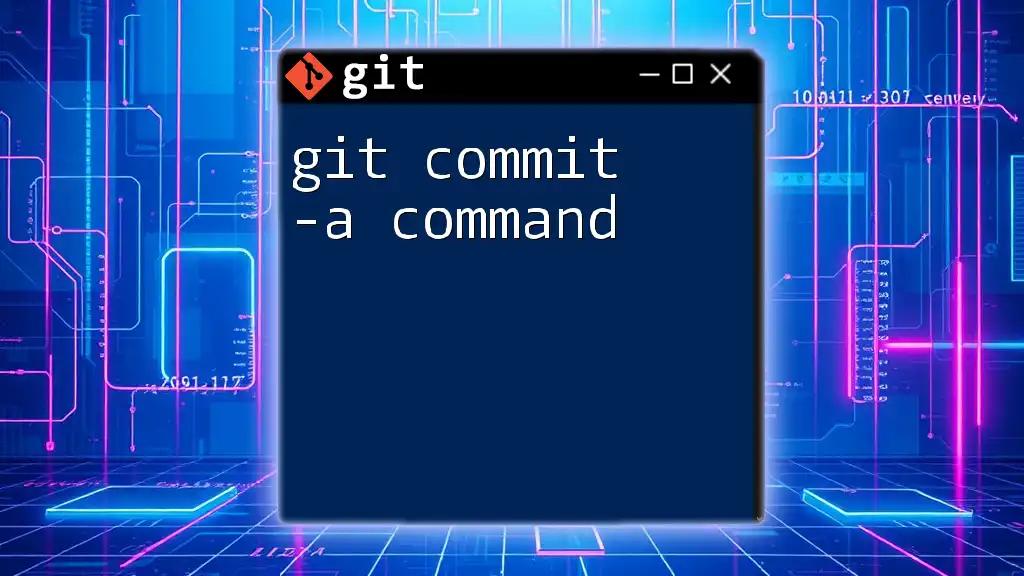
Conclusion
The git command requires the command line to unlock the full power and capabilities of version control within your projects. From setting up your environment to mastering advanced commands, the command line serves as a crucial tool for any developer working with Git. By practicing these commands and workflows, you’ll gain the confidence to utilize Git effectively and streamline your collaborative coding efforts.
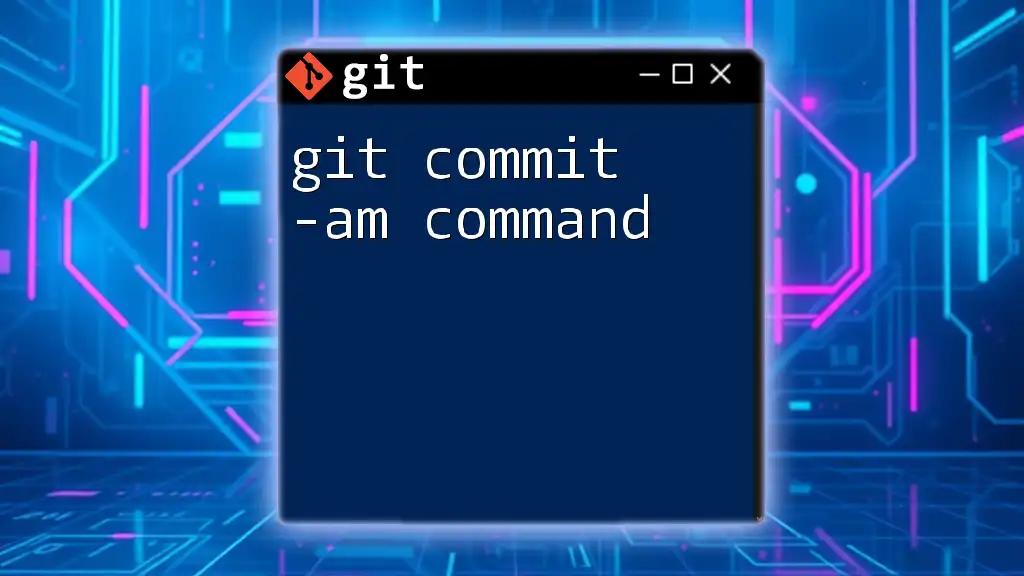
Additional Resources
For further learning, explore Git’s official documentation, online courses, and community forums. Immersing yourself in these resources will enhance your understanding and ability to use Git commands seamlessly in your development projects.