A Git commands cheat sheet is a concise reference guide that lists essential Git commands, enabling users to quickly recall how to manage their version control.
# Initialize a new Git repository
git init
# Clone an existing repository
git clone <repository-url>
# Check the status of your repository
git status
# Add changes to the staging area
git add <filename>
# Commit your changes
git commit -m "Your commit message"
# Push changes to remote repository
git push origin <branch-name>
# Pull updates from the remote repository
git pull origin <branch-name>
# Create a new branch
git branch <new-branch-name>
# Switch to a different branch
git checkout <branch-name>
# Merge a branch into the current branch
git merge <branch-name>
Getting Started with Git
What is Git?
Git is a powerful version control system that helps individuals and teams manage changes to their codebase over time. It allows for tracking modifications, collaborating with others, and rolling back to previous versions effortlessly. Understanding Git is essential for developers and anyone involved in the software development process.
Installing Git
To get started, you’ll need to install Git on your system. Different operating systems have different installation processes:
- Windows: Download and run the installer from the [official Git website](https://git-scm.com/).
- macOS: Install via Homebrew using the command:
brew install git
- Linux: Use your distribution's package manager, for example:
sudo apt-get install git # For Debian/Ubuntu
Once installed, you can verify the installation by checking the version:
git --version
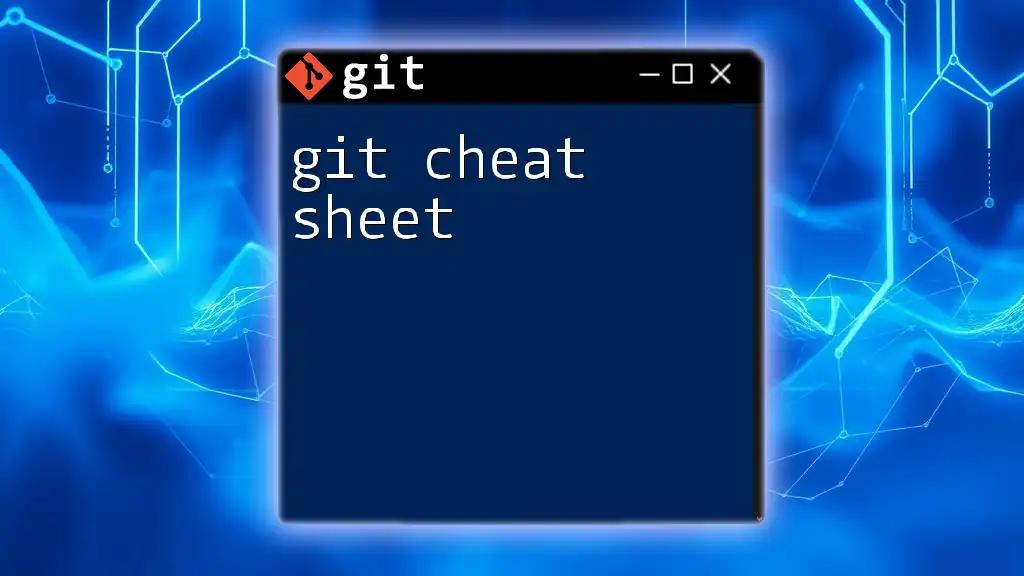
Basic Git Commands
Creating a New Repository
To start a new project or repository, use the command:
git init my-repo
This creates a new directory called `my-repo` and initializes it as a Git repository, setting everything up for you to start tracking your files.
Cloning an Existing Repository
If you want to work on an existing project, you can clone it using:
git clone https://github.com/username/repo.git
This command duplicates the specified repository into a new directory on your local machine.
Checking Repository Status
To see the current status of your repository, including modified files and what’s staged for the next commit, use:
git status
This will give you a clear picture of how your working directory compares to the committed states.
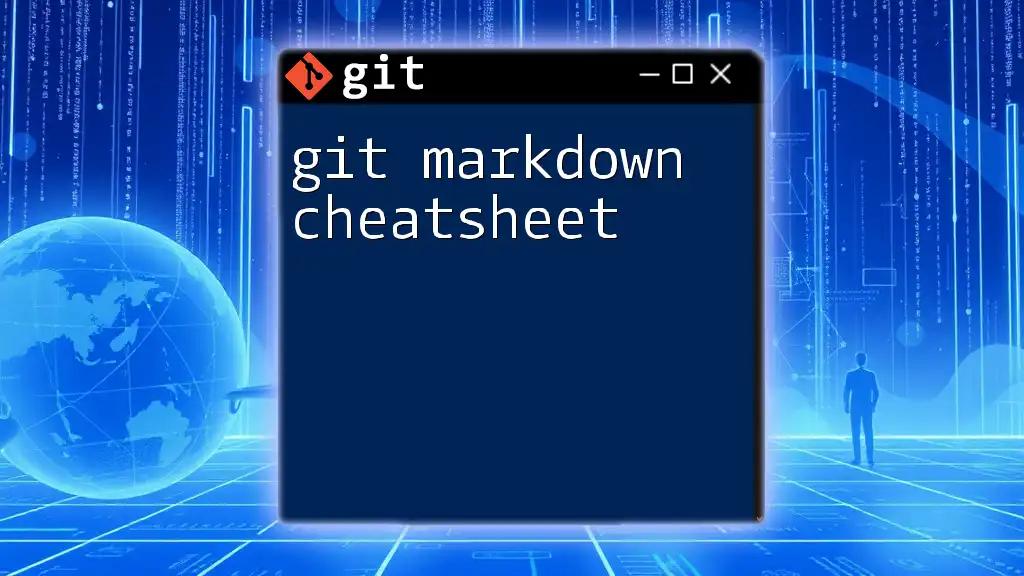
Working with Branches
Understanding Branches
Branches are a fundamental feature of Git. They allow you to work on different versions of your code simultaneously without affecting the main project. The `main` or `master` branch is usually the primary stable version.
Creating a New Branch
To create a new branch, use:
git branch feature-branch
This command allows you to add new features or make changes without impacting the main codebase.
Switching Branches
To start working on your new branch, switch to it with:
git checkout feature-branch
This enables you to work on the new feature in isolation.
Merging Branches
Once your feature is complete, merge changes back into the main branch with:
git merge feature-branch
This command takes the changes made in `feature-branch` and integrates them into your current branch.
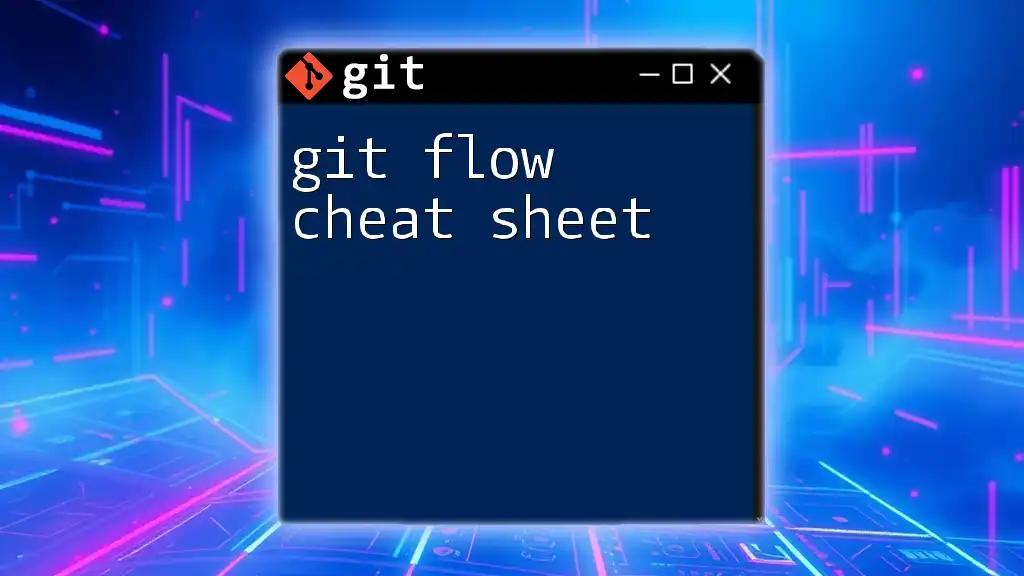
Committing Changes
Staging Changes
Before committing changes, you need to stage the files you want to include:
git add .
This command stages all modifications in your directory. It’s essential to stage only the changes you want to be included in your next commit.
Committing Changes
To save your changes to the repository, use:
git commit -m "Added new feature"
Including a descriptive message is crucial as it provides context for future reference.
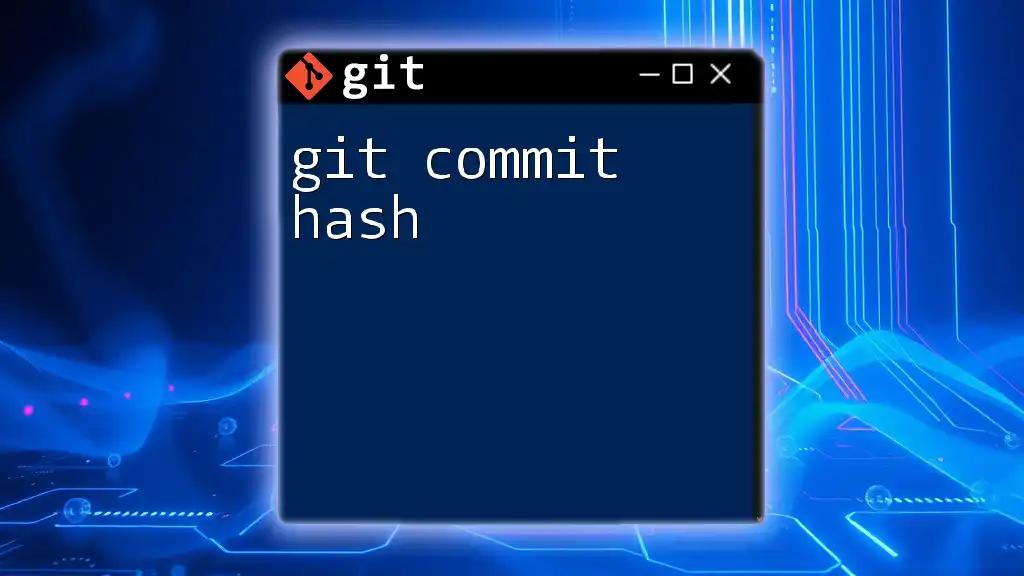
Viewing Changes and History
Viewing the Status
Reiterate the current status of your files with:
git status
This command helps in understanding which files are staged, modified, or untracked.
Viewing Commit History
To review past commits and their messages, use:
git log --oneline
This provides a concise history of commits, making it easy to track changes over time.
Viewing Changes in Files
To see differences that haven’t been staged yet, use:
git diff
This command displays line-by-line changes, allowing you to verify what you’ve modified before committing.
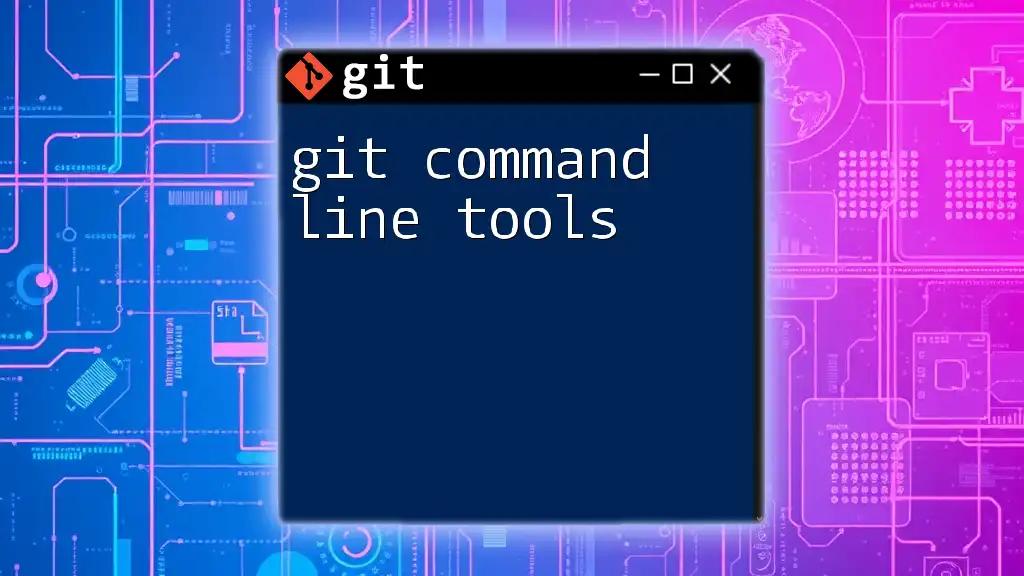
Remote Repositories
Adding a Remote Repository
To connect your local repository to a remote one, use:
git remote add origin https://github.com/username/repo.git
This command associates your local repository with the remote URL.
Pushing Changes
To send your committed changes to the remote repository, execute:
git push origin main
This updates the remote branch with your local changes.
Pulling Changes
Conversely, to fetch and merge changes from the remote repository, use:
git pull origin main
This command ensures your local copy is updated with the latest changes made by others.
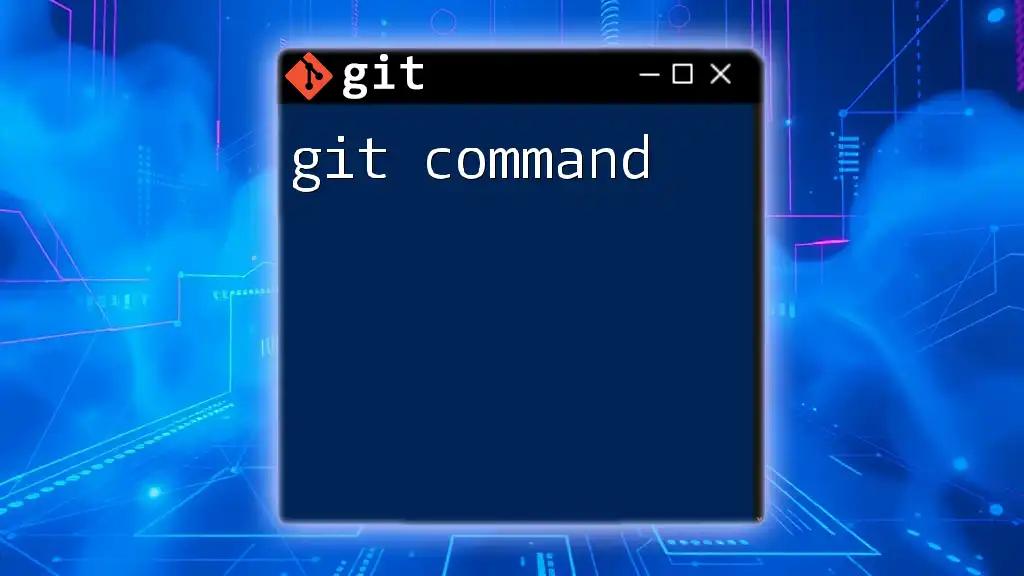
Working with Tags
Creating a Tag
Tags are useful for marking specific points in your repository’s history, like releases. Create a tag with:
git tag v1.0
This can help you keep track of versions and significant milestones.
Listing Tags
To see all tags in your repository, simply run:
git tag -l
This will give you a list of all the tagged versions.
Deleting a Tag
If you need to remove a tag, use:
git tag -d v1.0
This command deletes the specified tag locally.
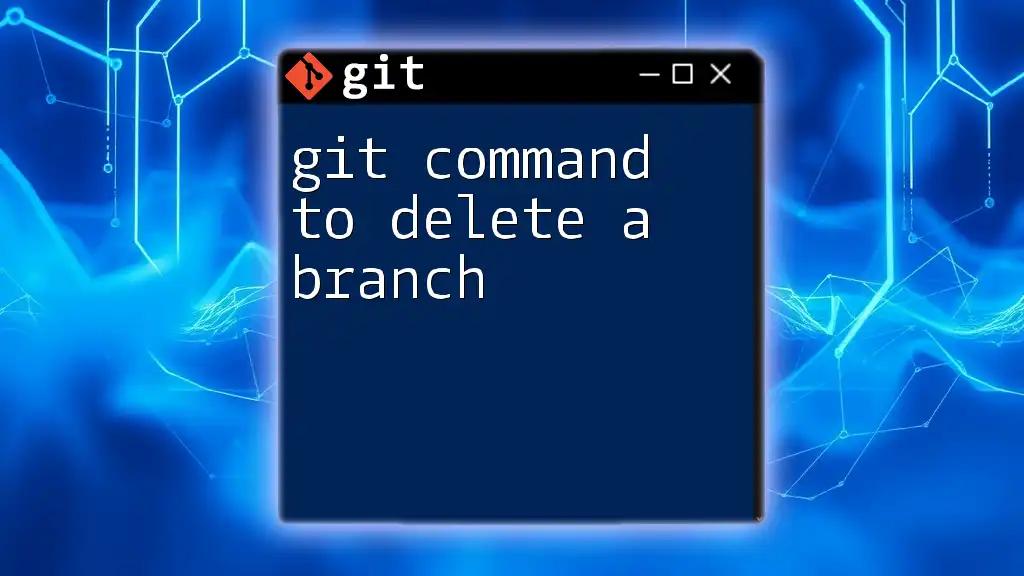
Safe Practices and Troubleshooting
Undoing Changes
It’s easy to make mistakes, and Git provides several ways to undo actions:
- To discard changes in a file, use:
git checkout -- <filename>
- To unstage a file, use:
git reset HEAD <filename>
- To remove the last commit but keep your changes, use:
git reset HEAD~1
If you need to undo a commit with a public commit history, use:
git revert <commit-hash>
This commands create a new commit that reverses changes in the specified commit.
Fixing Common Issues
Common issues include merge conflicts and a detached HEAD state. If you encounter a merge conflict, you’ll need to manually resolve it by editing conflicting files. After resolving, stage the changes and complete the merge with `git commit`.
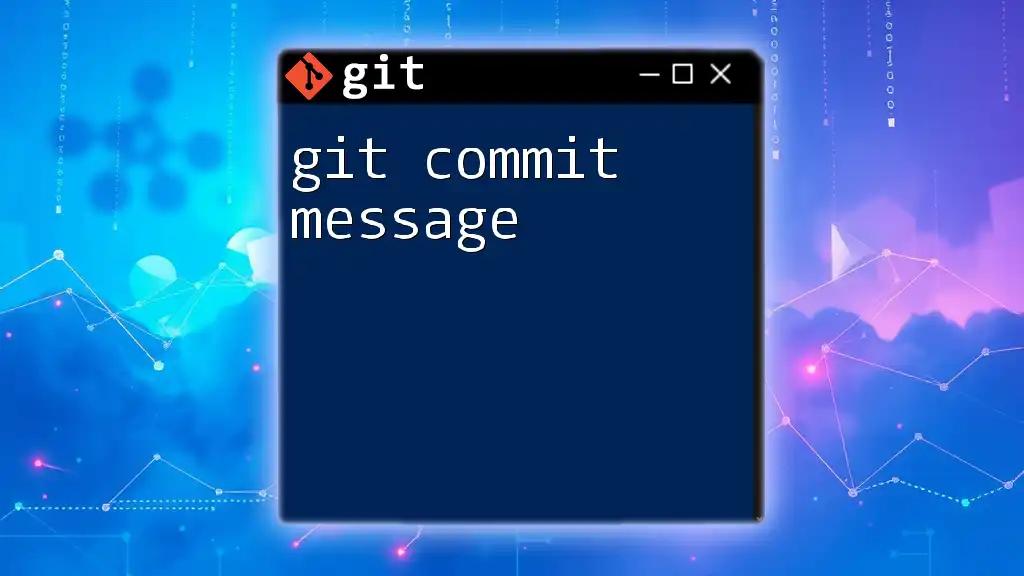
Conclusion
Mastering Git commands is invaluable for developers, enabling efficient code management and collaboration. By using this Git commands cheat sheet, you can enhance your productivity and streamline your workflow. Always remember that practice is key to becoming proficient in Git.
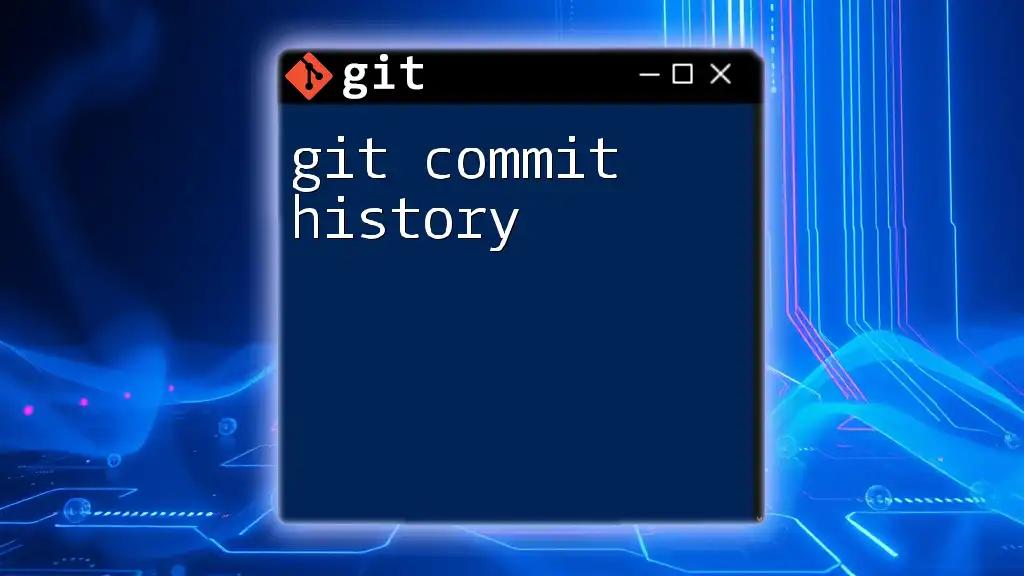
FAQs
What is the difference between `git pull` and `git fetch`?
`git fetch` just downloads the changes from the remote repository without merging them. In contrast, `git pull` fetches and then merges those changes into your local branch.
How can I see what branch I am currently on?
You can check your current branch by running:
git branch
The current branch will be highlighted with an asterisk (*).
What do I do if I encounter a merge conflict?
In case of a merge conflict, Git will pause the merge and allow you to resolve the conflicting changes. Edit the files to resolve conflicts, stage them with `git add`, and then commit the resolution.