Git Flow is a branching model for Git that helps streamline version control by defining roles for different branches and guiding developers through the workflow of managing releases and features.
# Common Git Flow commands
git checkout -b feature/my-feature # Start a new feature branch
git checkout develop # Switch to the develop branch
git merge feature/my-feature # Merge feature branch into develop
git checkout master # Switch to the master branch
git merge develop # Merge develop into master for a release
git tag -a v1.0 -m "Release v1.0" # Tag the release
What is Git Flow?
Git Flow is a branching model that was introduced by Vincent Driessen. It provides a robust framework for managing a project's versioning and involves using specific branch types for different stages of the development lifecycle. Unlike simpler branching models, Git Flow sets clear guidelines that help teams work collaboratively and maintain a clean history of their project.
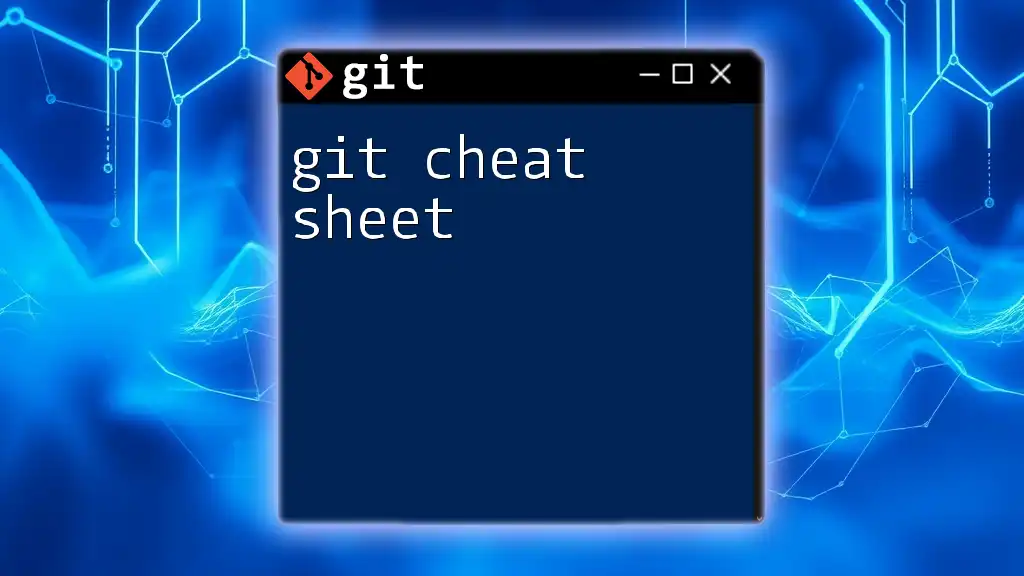
Why Use Git Flow?
Using Git Flow has numerous benefits. It encourages discipline among developers by promoting organized branching and merging practices. This structured workflow is particularly effective in larger teams or continuous integration environments, as it clearly separates feature development, preparation for production, and urgent fixes.
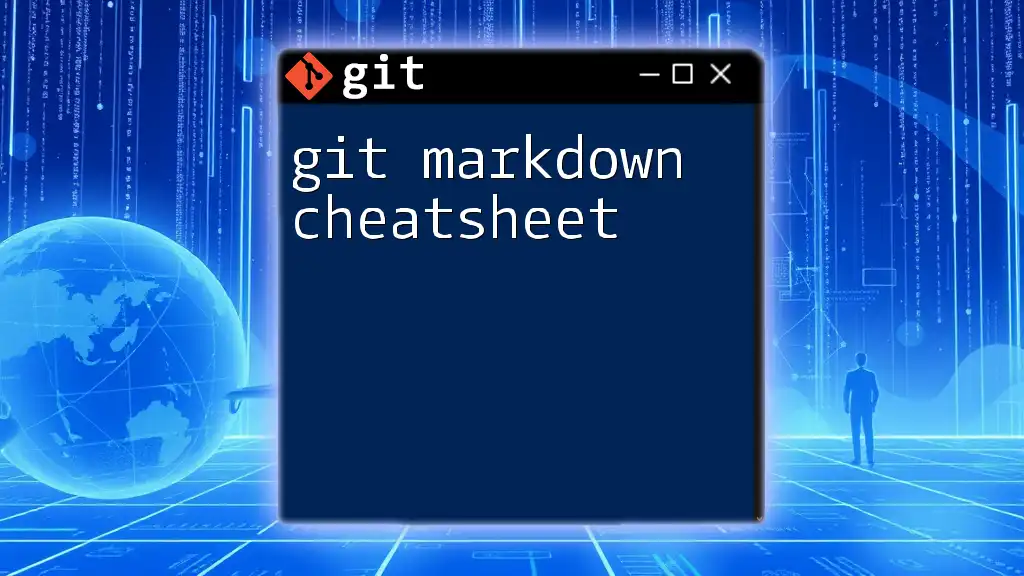
Branches Overview
Understanding the different branch types in Git Flow is fundamental to effectively utilizing it.
Main Branch
The main branch is the cornerstone of any Git Flow strategy. It holds the production-ready code, and each commit represents a recorded state of the project that is suitable for deployment.
Develop Branch
The develop branch serves as an integration branch for features. It is where the ongoing work is merged, and it represents the latest delivered development changes for the next release.
Feature Branch
Feature branches are where new features are developed. Each feature should have its own branch originating from the develop branch. Using descriptive names for feature branches enhances clarity, e.g., `feature/login-form`.
Release Branch
Once the development for a new release is stabilized in the develop branch, a release branch is created. This branch is crucial for preparing for production, allowing time for final testing and bug fixes before merging back to the main branch.
Hotfix Branch
In the event of critical bugs affecting production, hotfix branches come into play. These branches allow developers to address issues promptly by branching off from the main branch, applying the fix, and then merging it back into both the main and develop branches.
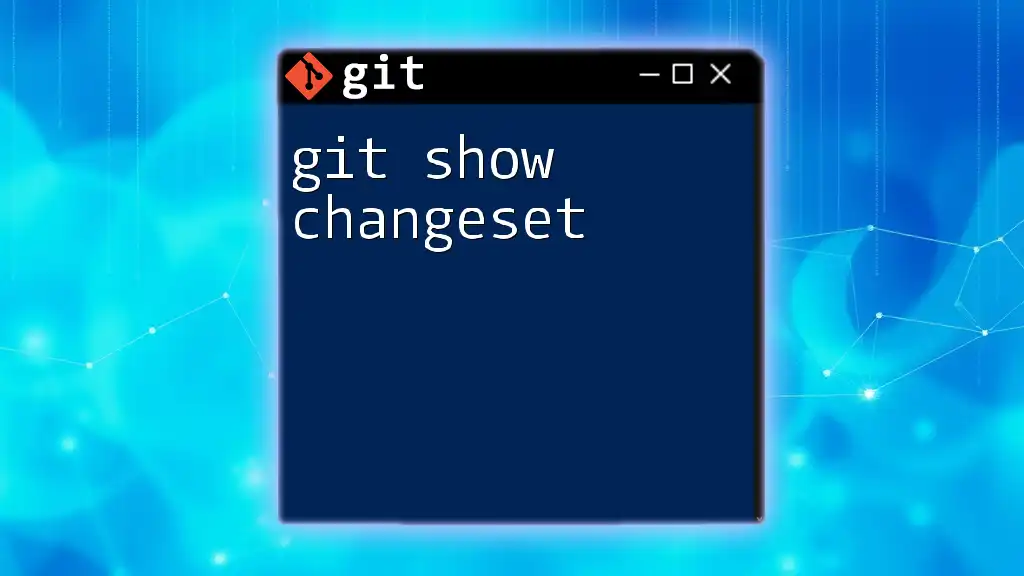
Understanding Merge vs Rebase
When working with branches, developers often face a choice between merging or rebasing.
- Merging creates a new commit that combines changes from different branches, preserving history but potentially leading to a more complex commit history.
- Rebasing rewrites commit history by applying changes from one branch to another directly. While this can result in a cleaner history, it requires careful management to avoid complications.
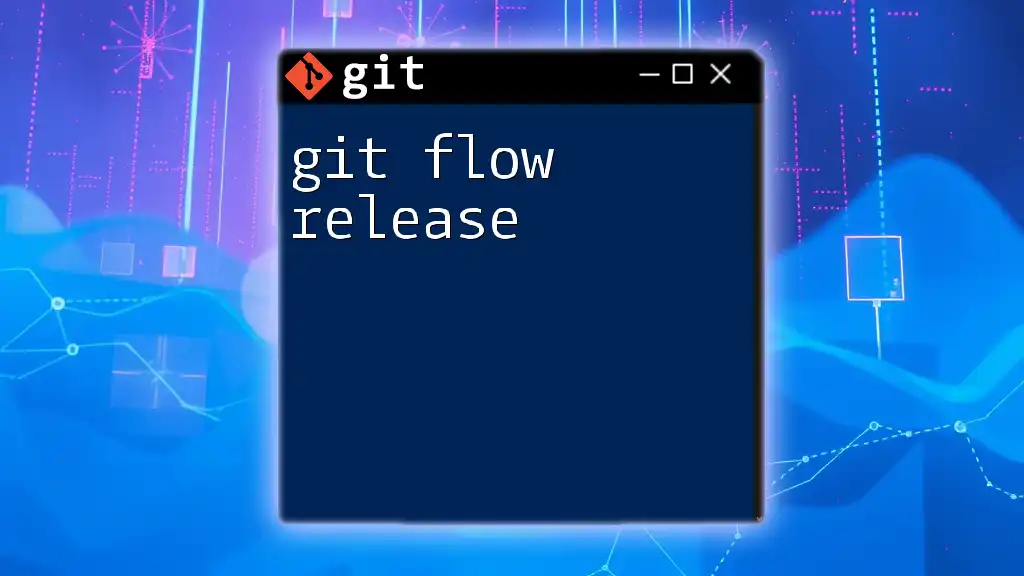
Setting Up Git Flow
Installation
To get started with Git Flow, it must first be installed. Here are the commands for popular platforms:
-
For macOS, use Homebrew:
brew install git-flow
-
For Ubuntu, use APT:
sudo apt-get install git-flow
-
For Windows, use Chocolatey:
choco install git-flow
Initializing Git Flow
Once installed, Git Flow can be initialized in your current repository. Run the following command:
git flow init
During initialization, you will be prompted to specify branch names. Default conventions are usually sufficient, but customization is possible if necessary.
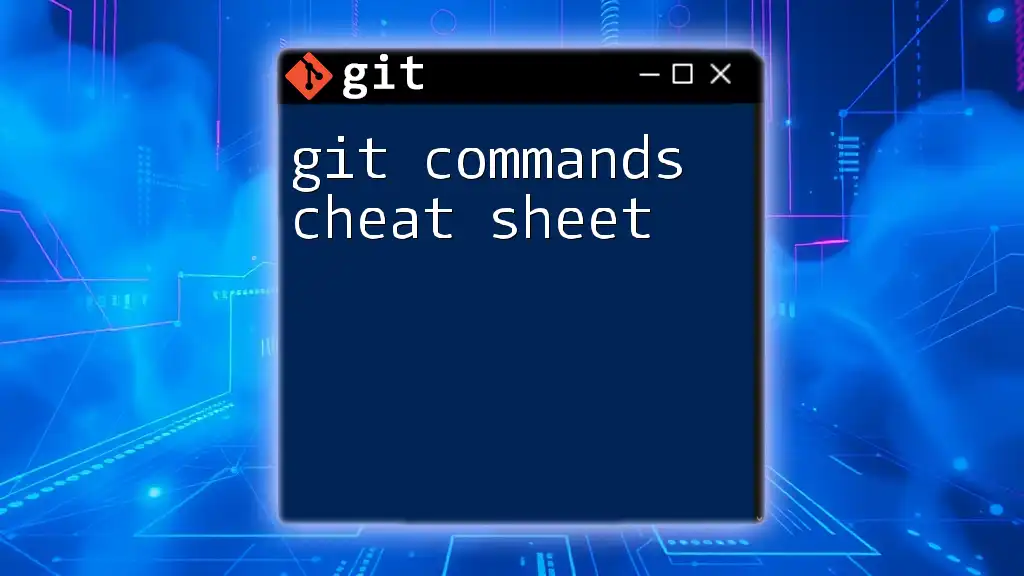
Common Git Flow Commands
Feature Branch Workflow
Creating and managing feature branches is central to Git Flow practice.
Creating a Feature Branch
To start working on a new feature, you can create a branch with the following command:
git flow feature start <feature-name>
Here, `<feature-name>` should be descriptive, such as `add-user-authentication`.
Completing a Feature
Once development on a feature is complete, finish the feature branch with:
git flow feature finish <feature-name>
This command will merge the feature branch into the develop branch and delete the feature branch.
Release Branch Workflow
When preparing for a release, creating and managing a release branch is essential.
Starting a Release Branch
To create a release branch, run:
git flow release start <version>
Here, `<version>` usually follows semantic versioning, such as `1.0.0`.
Completing a Release
Finalize the release with the following command:
git flow release finish <version>
This will merge changes into the main and develop branches and tag the release appropriately.
Hotfix Branch Workflow
Handling urgent fixes through hotfix branches follows a similar pattern.
Creating a Hotfix
To start a hotfix, use:
git flow hotfix start <hotfix-name>
This allows you to address urgent issues while keeping the main branch stable.
Finishing a Hotfix
After applying your fix, complete it by executing:
git flow hotfix finish <hotfix-name>
The changes from the hotfix branch will merge back into both the main and develop branches.
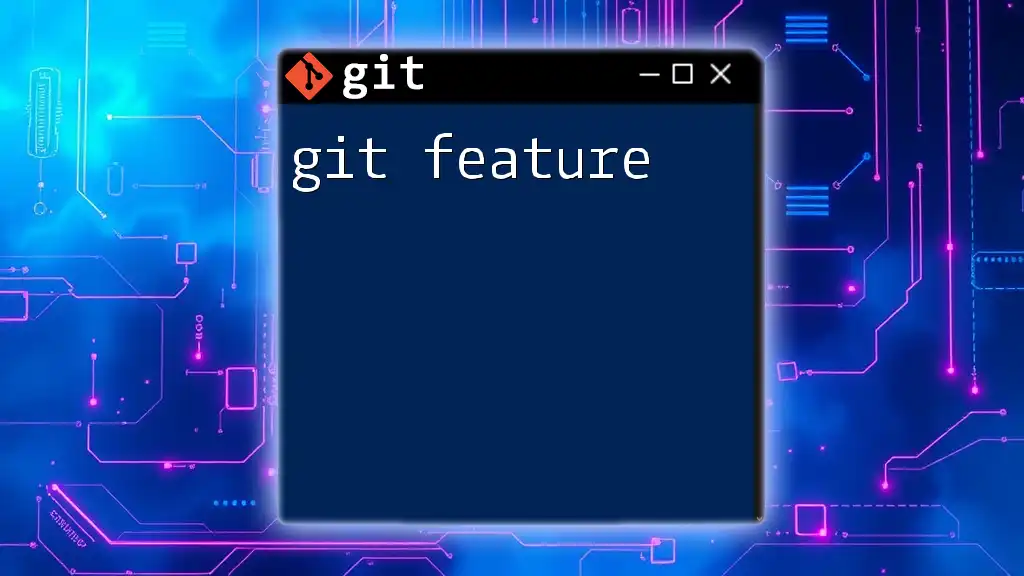
Best Practices for Using Git Flow
Consistent Branch Naming Conventions
Adopting clear and consistent naming conventions for branches enhances team communication. For instance, use prefixes like `feature/`, `release/`, and `hotfix/` to categorize branches easily.
Regularly Syncing with Remote
To avoid integration issues, ensure branches are regularly updated with the latest changes from the remote repository. Commands such as `git fetch` and `git merge` can help keep all branches synchronized with the main branch.
Using Pull Requests
Utilizing pull requests within your Git Flow strategy facilitates code reviews and supports collaborative development. This practice accentuates quality assurance and encourages open communication among team members.
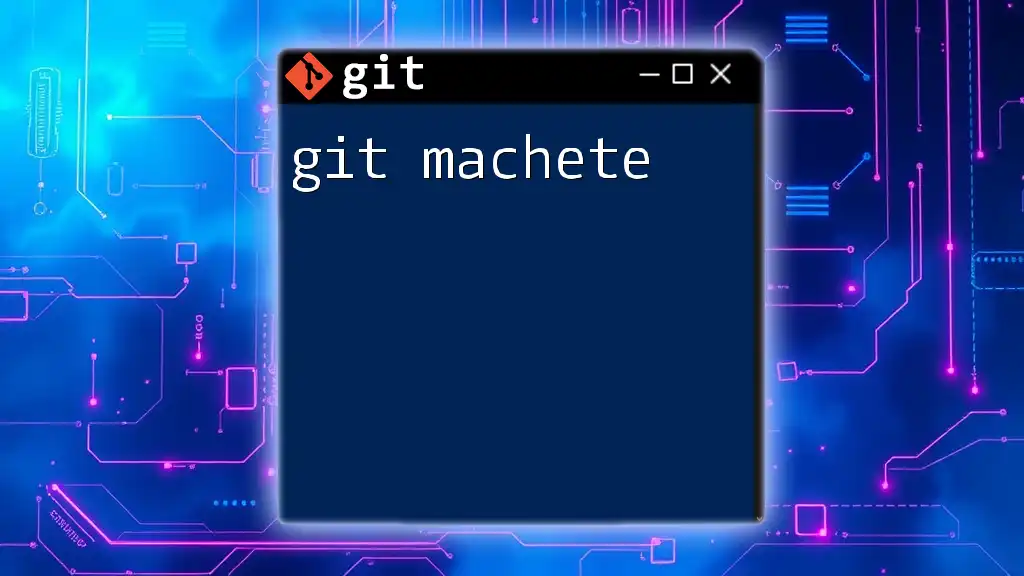
Troubleshooting Common Git Flow Issues
Conflicts While Merging
Merge conflicts are commonplace, especially when multiple developers work on similar areas of the codebase. Git provides tools to help resolve these conflicts, but understanding how to use `git mergetool` can streamline the process.
Accidental Deletions or Loss of Branches
If a branch is accidentally deleted, recovery is often possible. You can recover it using the following command:
git reflog
This command allows you to view all recent actions and can help locate the lost branch commits.
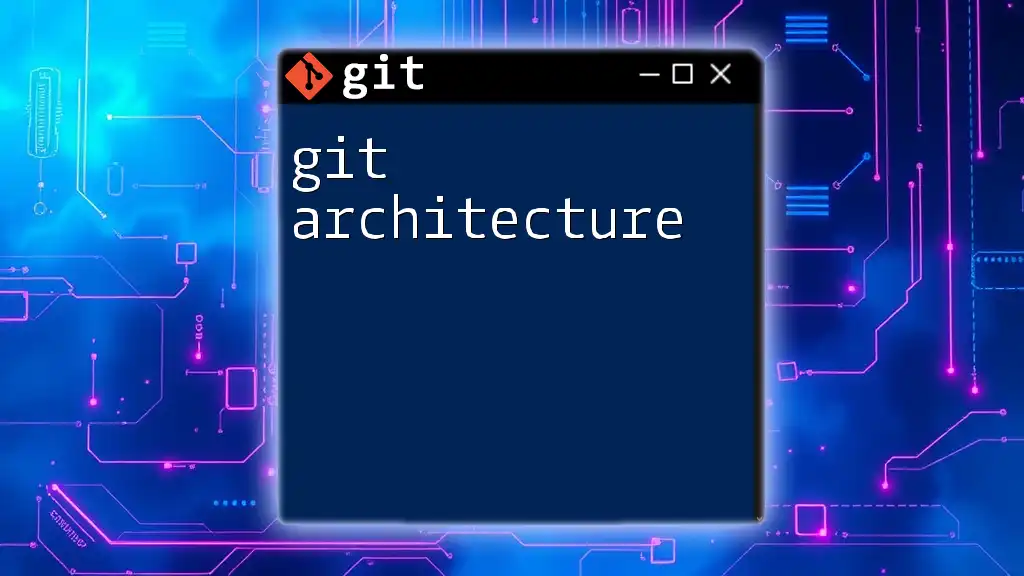
Conclusion
Git Flow provides a structured framework for managing the development process efficiently. By adopting this branching model, developers can improve their workflow, reduce conflicts during integration, and ensure a more disciplined approach to software releases. Practice utilizing the Git Flow cheat sheet to refine your command of Git and enhance your team's collaborative effectiveness.
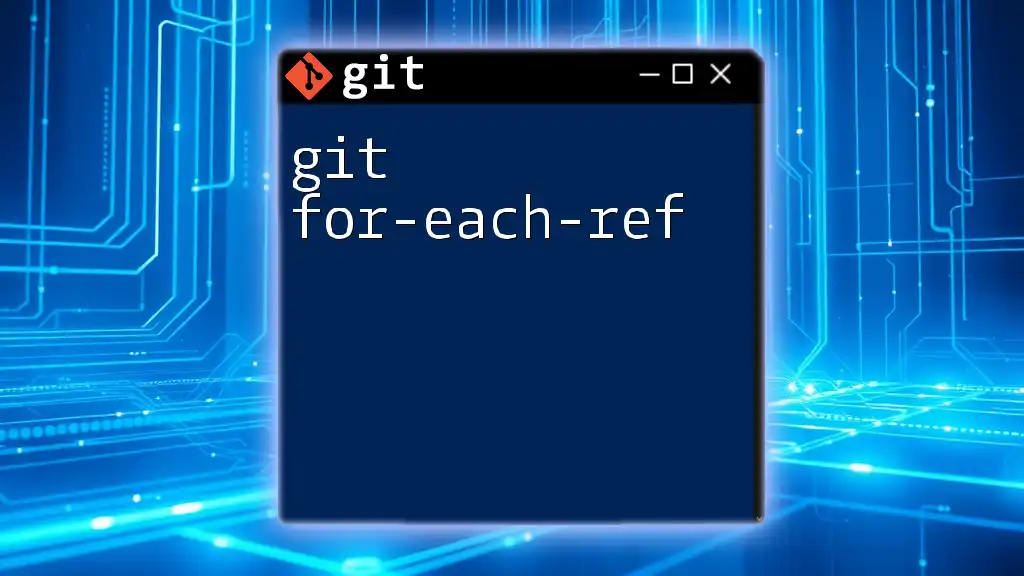
Call to Action
Stay informed with more tips on Git commands by signing up for our newsletter. Share your experiences with Git Flow in the comments, and don't hesitate to ask any questions for further clarification!