The `git for-each-ref` command allows you to iterate over all references in a Git repository and print details about each reference in a customizable format.
git for-each-ref --format='%(refname:short) %(*:short)' refs/heads
Understanding References in Git
What are References? In the world of Git, references (or refs) play a crucial role in managing different branches, tags, and remote connections in a repository. Understanding references helps developers maintain a clearer organizational structure for the project's history and versions.
Types of References
Branches: Branches are pivotal in enabling parallel development. Each branch represents an independent line of development, making it easier for teams to collaborate. They can be accessed via `refs/heads/branch_name`.
Tags: Tags act as markers on specific points in a Git history, typically used to denote release versions. Tags are generally more stable than branches and can be found under `refs/tags/tag_name`.
Remote References: These references connect your local repository to remote ones, allowing multiple users to work together seamlessly. Remote branches are accessed through `refs/remotes/origin/branch_name`.
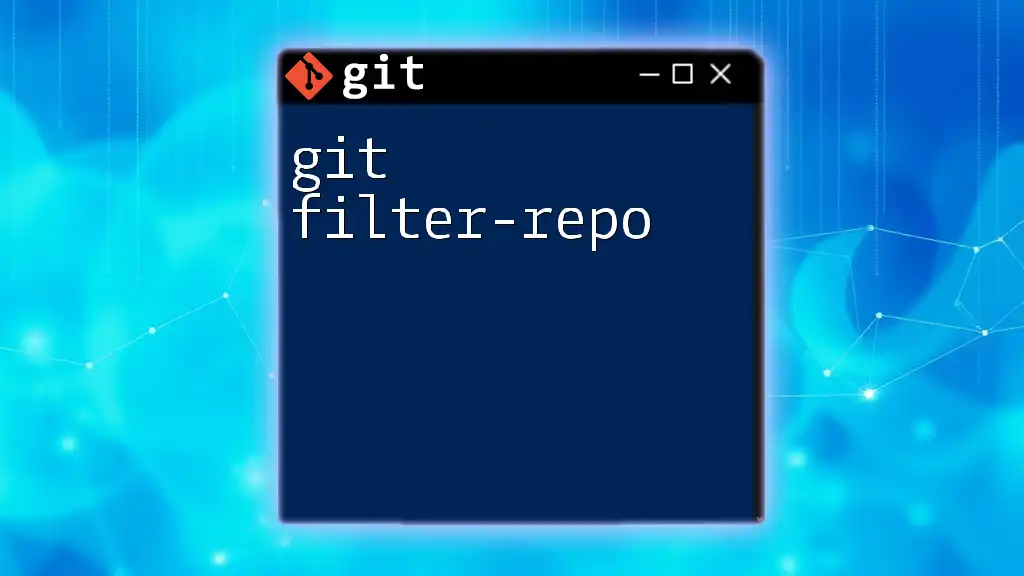
Introduction to `git for-each-ref`
What is `git for-each-ref`? The `git for-each-ref` command is a powerful tool that allows you to iterate over references in a repository. It's specifically designed for retrieving information about various refs and presenting them in a customizable manner, making it a vital command for both scripting and manual inspection.
Basic Syntax of `git for-each-ref`: The basic command structure is straightforward. You can execute it in your terminal as follows:
git for-each-ref
When run, it provides a simple list of all refs in the repository, making it easy to see what’s available.
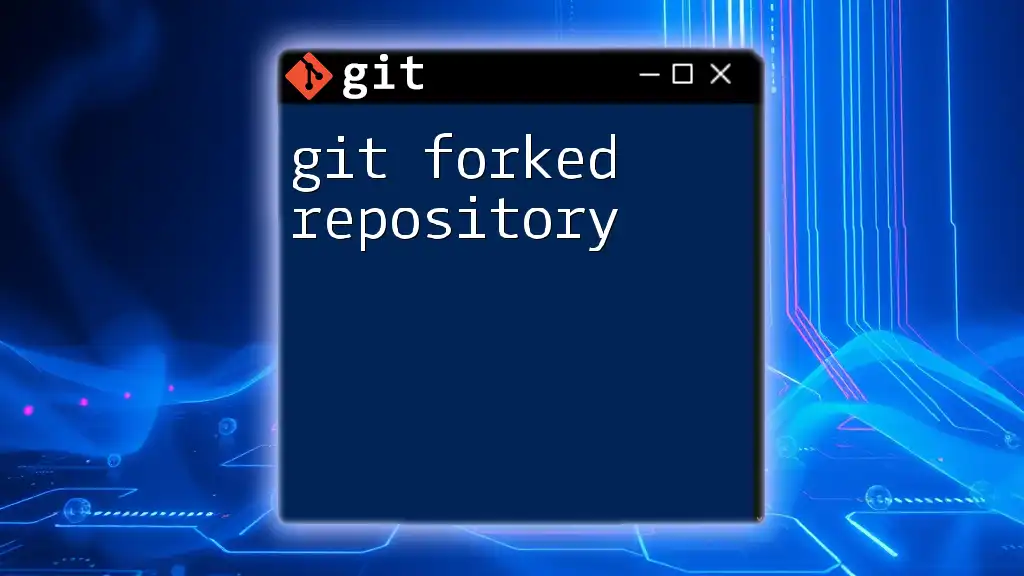
Using `git for-each-ref`
Listing All References: By simply typing:
git for-each-ref
you will receive a comprehensive list of all references in the repository. The output typically looks something like this:
ref/heads/main
ref/tags/v1.0
ref/remotes/origin/feature-a
This command provides a quick overview of your branches, tags, and remote references.
Filtering References: If you want to narrow your focus to specific types of references, you can utilize the `--match` option. For instance, if you’re only interested in listing branches, your command would be:
git for-each-ref --match="refs/heads/*"
This will return only the branches in your repository, providing a more focused view.
Customizing Output with `--format`
Understanding the `--format` option: One of the standout features of `git for-each-ref` is its ability to customize output using the `--format` flag. This allows you to specify exactly what information you want to display per ref.
Common Format Variables: Git provides several format placeholders to help customize the output. For example:
- `%(refname)`: Displays the full reference name.
- `%(refname:short)`: Gives the short version of the reference name.
- `%(committerdate)`: Shows the date of the last commit for the ref.
Example: Customized Output: To list all branches along with their last commit dates, use the command:
git for-each-ref --format="%(refname:short) - %(committerdate)" --sort=-committerdate
This provides output sorted by the latest commit date, clearly identifying the most recently updated branches.
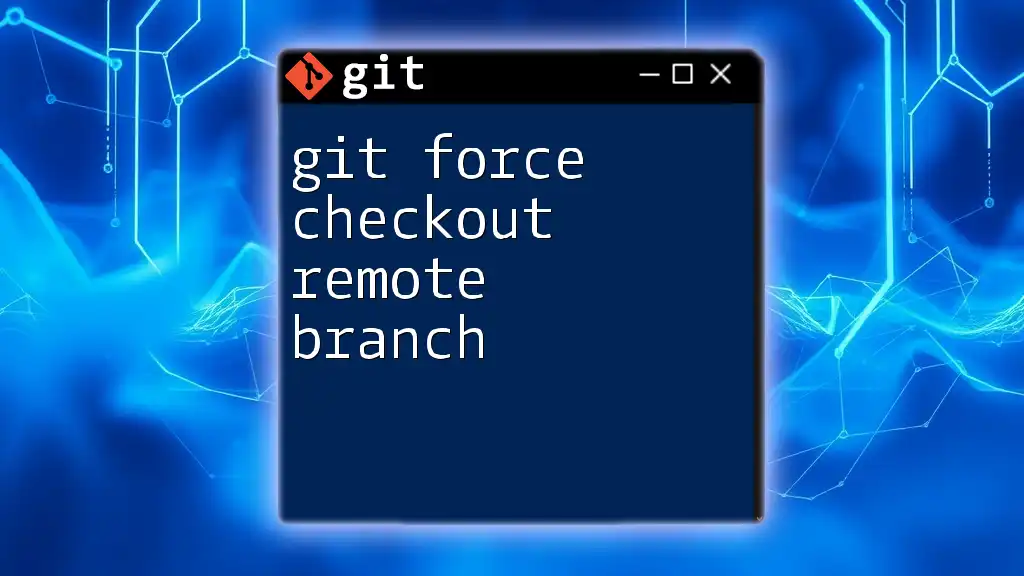
Practical Applications of `git for-each-ref`
Scripting and Automation: The versatility of `git for-each-ref` shines in automation scripts. Imagine needing to check each branch for certain criteria — you could implement this with a loop in a shell script, as follows:
for branch in $(git for-each-ref --format='%(refname:short)'); do
echo "Checking $branch..."
# Additional checks can be added here
done
This script prints a message for each branch, allowing for further automated processes.
Git Hooks: Integrating `git for-each-ref` in Git hooks can automate checks before commits or merges. For example, you could run a pre-commit hook that ensures specific patterns in branch names or tags.
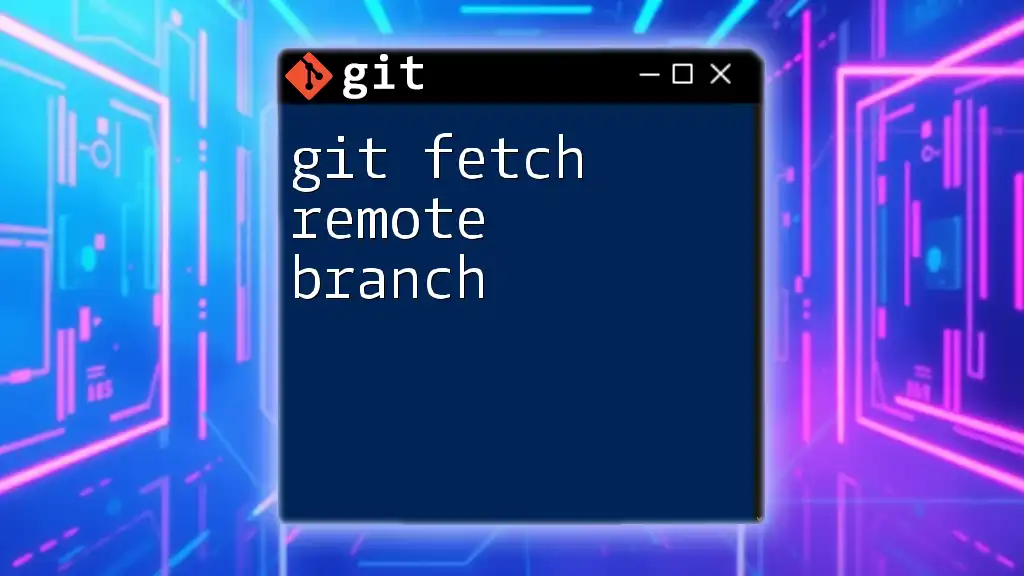
Common Use Cases for `git for-each-ref`
Finding Active Branches: When working on a project, it’s often essential to quickly identify which branches are currently in use. The following command will list active branches along with their latest commits:
git for-each-ref --format="%(refname:short) - %(objectname:short)" refs/heads/
Identifying Tags for Releases: You can easily retrieve all tags to see what releases have taken place. The command would be:
git for-each-ref --format="%(refname:short)" refs/tags/
This is useful for understanding the historical context of your project’s changes.
Sorting References: Git allows sorting of references based on various criteria. For example, to list all references sorted by their creation date, you’d use the command:
git for-each-ref --sort=creatordate
This command helps visualize your project's growth over time.
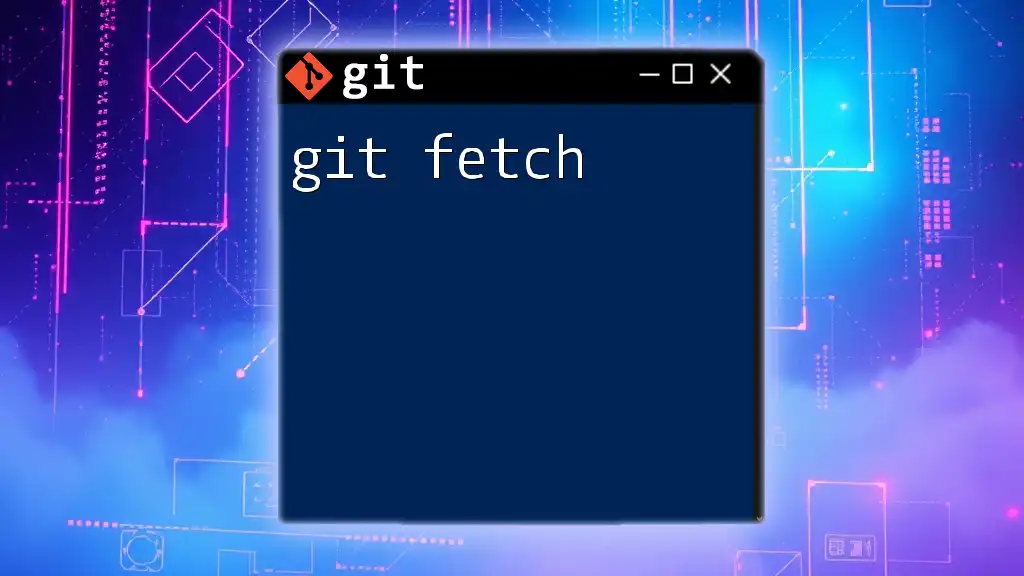
Conclusion
In summary, the `git for-each-ref` command stands out as a versatile tool for developers looking to manage and analyze the references within their Git repositories. By leveraging its capabilities, you can customize the output, automate repetitive tasks, and gain insights into your code management strategies. Exploring `git for-each-ref` enhances your productivity and efficiency in version control.
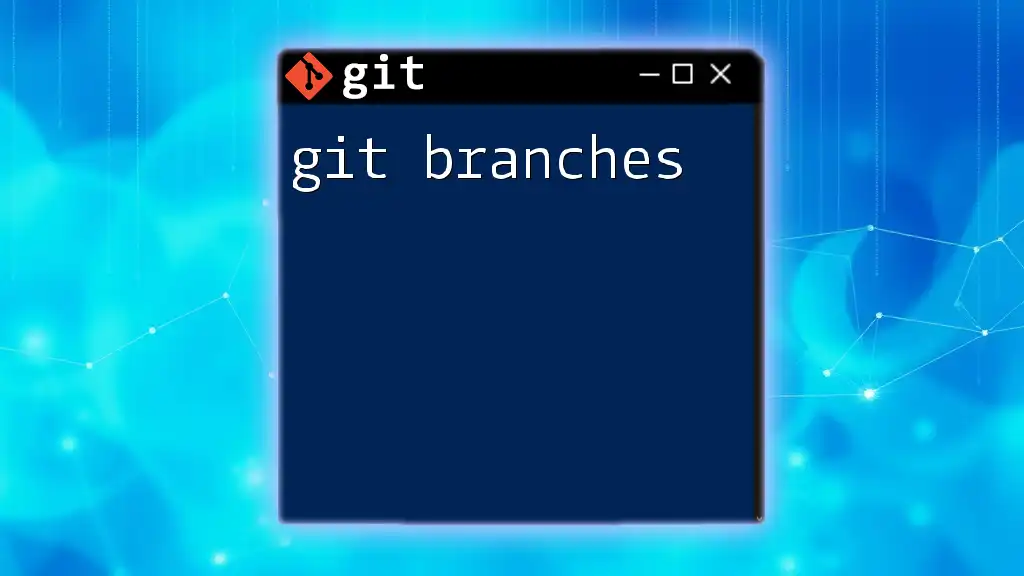
Additional Resources
For those eager to extend their Git knowledge, the official Git documentation serves as an excellent starting point. Numerous tutorials and learning platforms are also available to provide in-depth insights into Git’s capabilities.
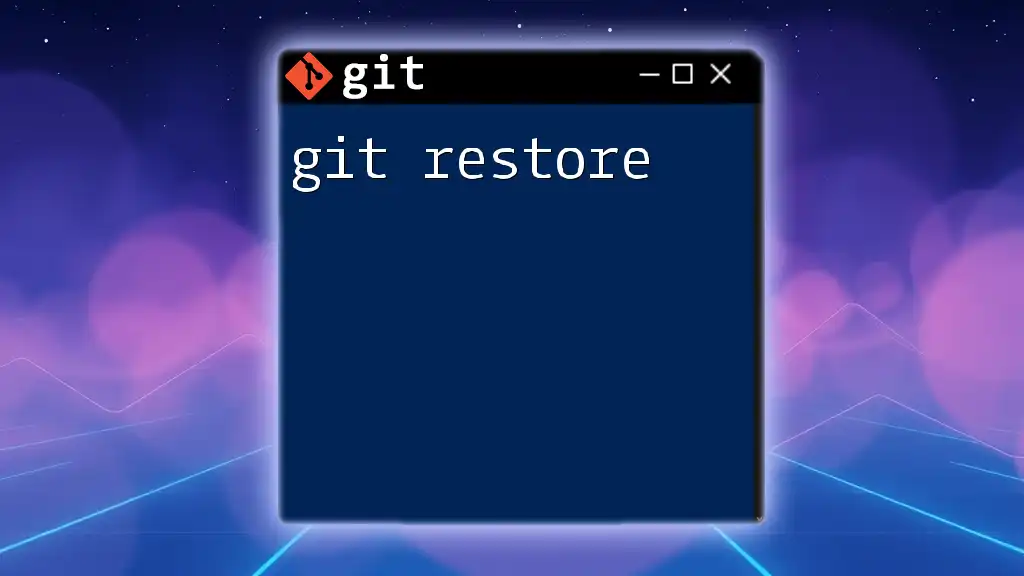
FAQs
What if I don't see any output when running `git for-each-ref`? There are a few potential reasons for this. Most commonly, it could be that you are not in a Git repository or that there are no refs available (e.g., in a newly initialized repository without any commits).
Can I use `git for-each-ref` with aliases? Indeed, using Git aliases allows for streamlining commands and enhancing your workflow efficiency. To create a custom alias for `git for-each-ref`, simply add it to your Git configuration. For instance:
git config --global alias.lord 'for-each-ref --format="%(refname:short)"'
Now, you can simply run `git lord` to execute the command.
By exploring these functionalities, you can maximize your Git experience and effectively manage your project’s references.