A Git workspace is the directory where you manage your files and track changes using Git, allowing you to edit, add, and commit your project efficiently.
git status
Understanding the Git Workspace
What is a Git Workspace?
A Git workspace is essentially your working environment for version control using Git. It comprises everything you need to manage your files, track changes, and maintain your project's history. The workspace can be thought of as the place where you make modifications to your files. It is crucial to understand that your workspace is not the same as your Git repository; while the workspace is where your current work happens, the repository is where Git stores all the history and metadata.
Components of a Git Workspace
To fully grasp the functionality of a Git workspace, it's important to be aware of its three primary components: the Working Directory, the Staging Area (Index), and the .git Directory.
The Working Directory
The Working Directory is the directory where you keep your project files. It contains all the files you are currently working on and allows you to edit and create new files. This directory is the focal point for making changes.
The relationship between the working directory and the staging area is fundamental. When you modify a file in your working directory, you need to stage it before it's committed to the repository.
The Staging Area (Index)
The Staging Area, also known as the index, acts as a middle ground between the working directory and the repository. It is where you prepare files before committing them. You can think of the staging area as a buffer zone where you can review and finalize your changes before they become part of the Git history.
For instance, if you want to include only certain changes in your next commit, you can stage specific files or lines. This gives you control over exactly what you want to commit.
The .git Directory
The .git Directory is where Git stores all of its objects and references. It contains critical data such as the history of commits, branches, and tags. Essentially, it is the brain of Git. Inside this directory, you will find various files and subdirectories that Git uses to manage your repository, including commit history and configuration settings.
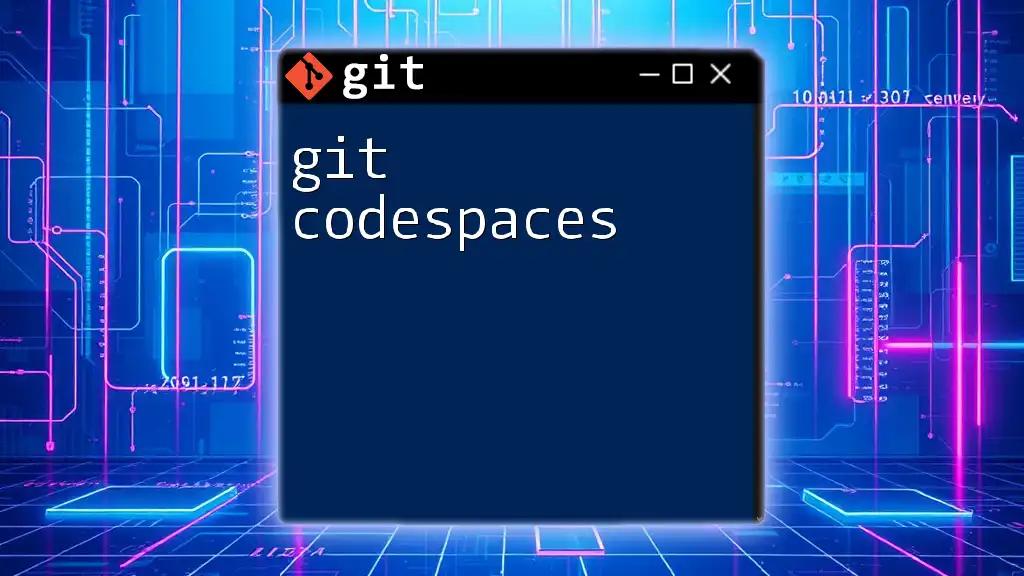
Setting Up a Git Workspace
Prerequisites
Before getting started, ensure you have a basic understanding of command-line usage and Git installed on your machine. If you haven't installed Git yet, you can follow an installation guide relevant to your operating system.
Creating a New Git Workspace
To create a new Git workspace, you can either initialize a new repository or clone an existing one.
To initialize a new repository:
git init my-repo
cd my-repo
This command creates a new directory named `my-repo` and initializes an empty Git repository in it.
To clone an existing repository, which brings an entire project and its history to your local machine, use:
git clone https://github.com/user/repo.git
This command will create a copy of the specified repository, including all files, branches, and commit history.
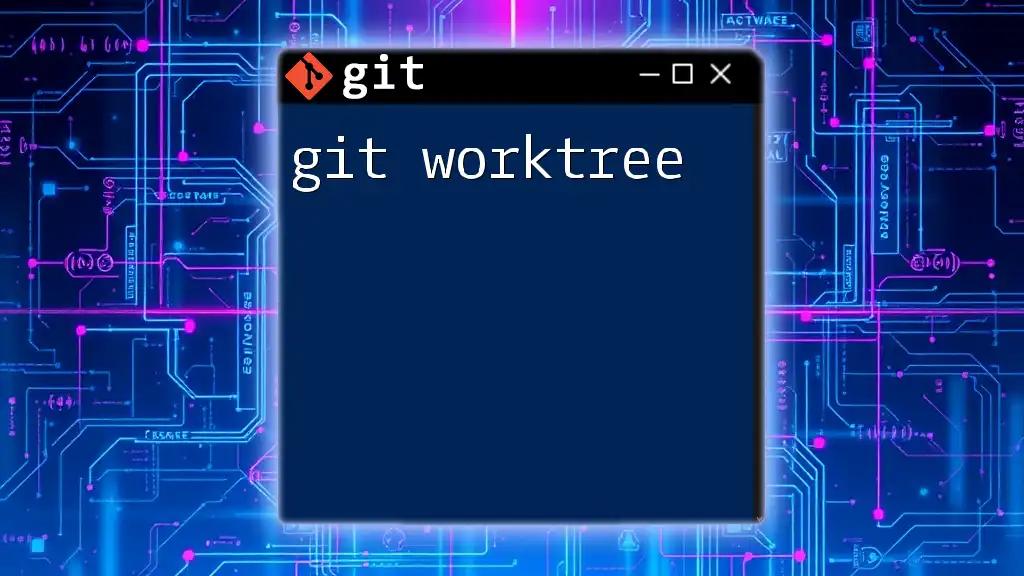
Navigating Your Git Workspace
Key Git Commands for Workspace Management
Managing your Git workspace effectively requires familiarity with several key commands.
Checking the Current Status
One of the first commands you should use to understand your workspace is `git status`. This command provides information about the current state of your workspace, including which files are staged for commit and which are untracked or modified.
git status
Viewing Changes
To see what changes you’ve made relative to the last commit, use the `git diff` command:
git diff
This command will show you line-by-line differences between your working directory and the last commit, helping you to review your changes before staging or committing them.
Staging Changes
When you're ready to prepare your files for a commit, you can stage them using `git add`. For instance, if you want to stage a specific file:
git add filename
You can also stage all changes in your workspace using:
git add .
Selective staging is important for keeping your commits coherent and manageable.
Understanding File States in a Workspace
In a Git workspace, files can exist in three states:
- Untracked Files: New files that are not yet part of version control.
- Modified Files: Files that have changed but haven’t been staged.
- Staged Files: Files that have been added to the staging area and are ready for the next commit.
Understanding these states is essential for effectively managing your changes.
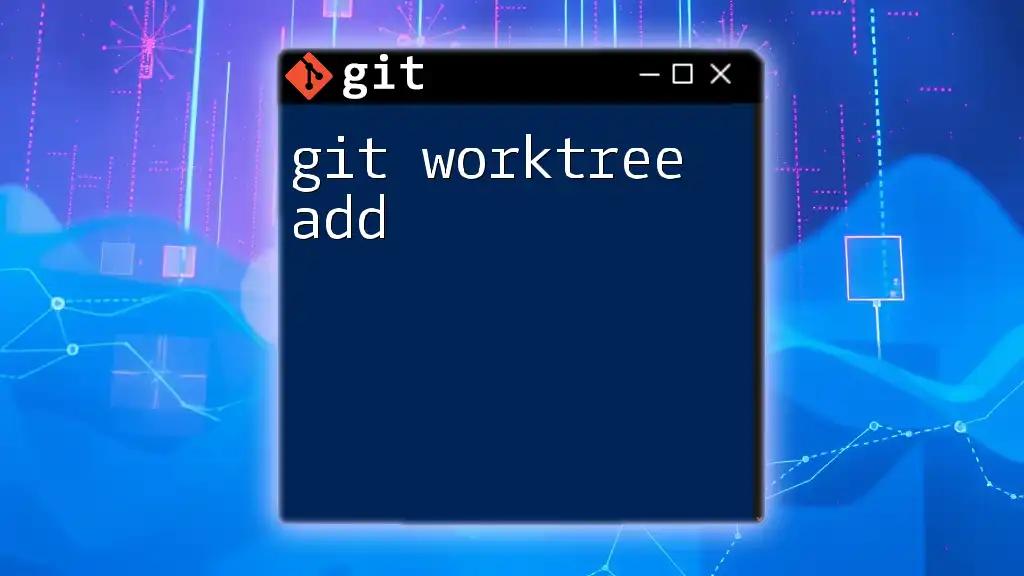
Working with Branches in Your Git Workspace
What is a Branch?
A branch in Git allows you to diverge from the main line of development, enabling parallel development. When you create a new branch, you're essentially creating an isolated environment for new features, bug fixes, or experiments without affecting the main codebase.
Creating and Switching Branches
Creating a new branch is straightforward. Use the following command to create and switch to a new branch:
git checkout -b new-branch
To switch back to an existing branch, you can use:
git checkout existing-branch
This flexibility makes it easier to manage different features or revisions without disrupting the main code.
Merging Changes
After you’ve made changes on a branch and are ready to integrate them into your main branch, you can merge them. Here is how you merge changes from one branch into another:
git merge feature-branch
This command will take the changes from `feature-branch` and apply them to your current branch, making it a powerful collaboration tool.
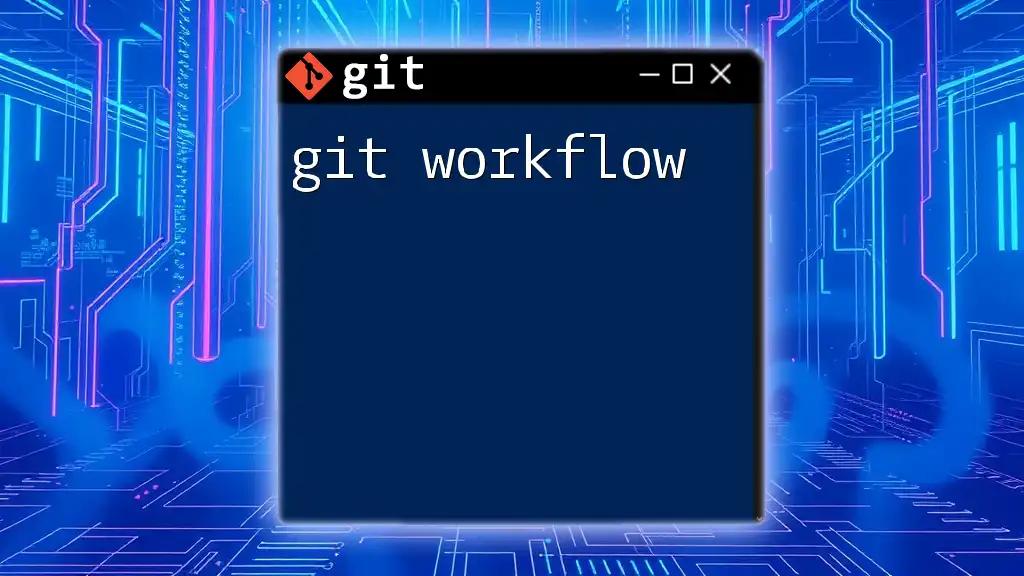
Best Practices for Managing Your Git Workspace
Organizing Your Files
Keep your workspace organized. A clean workspace can prevent errors and confusion. It's a good practice to group related files logically and adhere to naming conventions.
Regularly Committing Changes
Commit your changes regularly with clear, concise commit messages. Good commit messages make it easier to understand the history and purpose of changes:
git commit -m "Clear, concise commit message"
Adopting frequent commits fosters a more manageable history, making it easier to identify and revert changes if necessary.
Keeping Your Workspace Up-to-Date
To avoid falling behind on your team’s developments, regularly sync your local repository with the remote one. Use the following command to pull the latest changes:
git pull origin main
This ensures you are always working on the latest updates from your team.
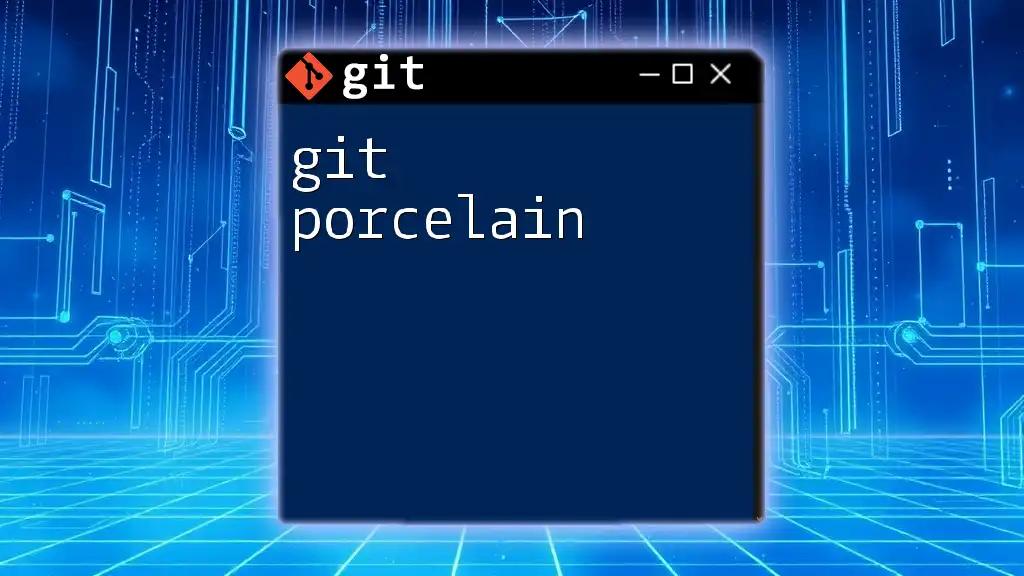
Troubleshooting Common Workspace Issues
Resolving Conflicts
Sometimes, merging changes from different branches can lead to conflicts, where Git cannot automatically reconcile differences. When this happens, you will need to manually resolve the conflicts. Git will mark the conflicting areas in the files:
<<<<<<< HEAD
// Your code
=======
– Incoming code
>>>>>>> feature-branch
Edit the file to keep the changes you want and remove the conflict markers, then save the file and mark it as resolved with `git add <filename>`.
Undoing Changes
If you make a mistake or want to discard changes, you can revert unstaged changes with:
git checkout -- filename
This command will restore the specified file to the last committed state, removing any modifications.
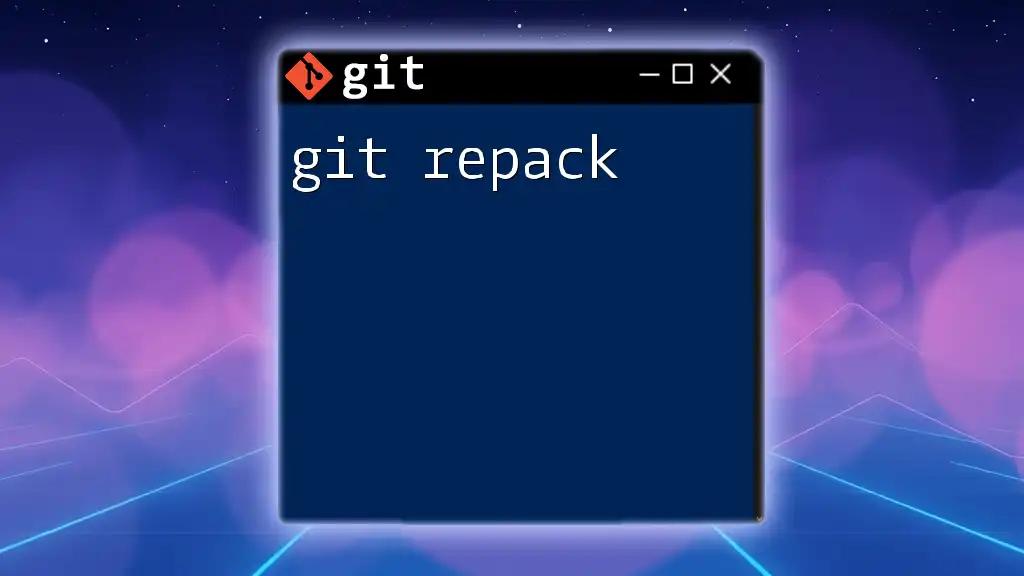
Conclusion
Understanding your Git workspace is fundamental to leveraging Git for version control effectively. By mastering the components of a workspace, setting it up correctly, and managing it with best practices, you'll streamline your development process and enhance your collaboration with team members.
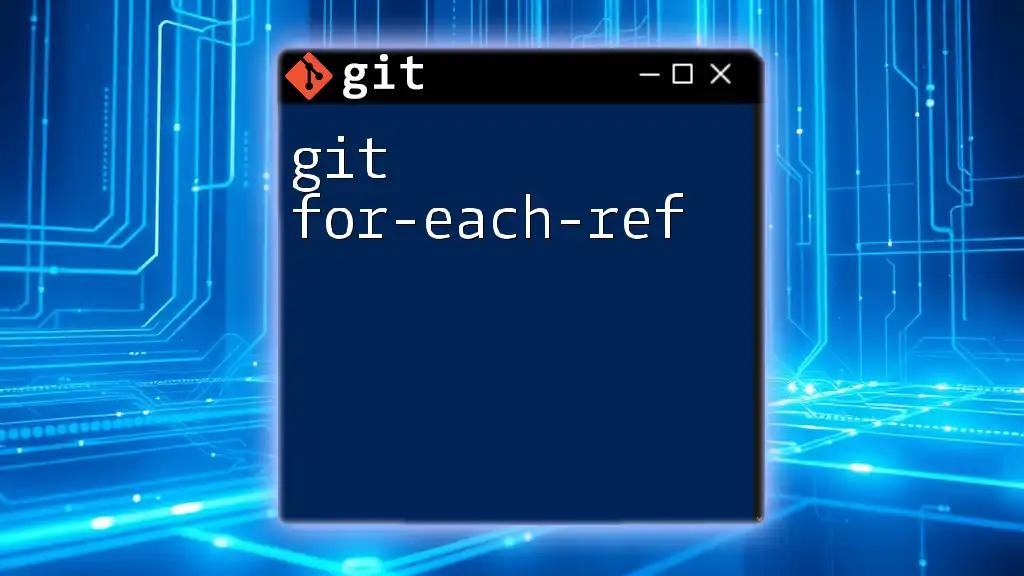
Additional Resources
For further learning, consult the official Git documentation or consider enrolling in targeted Git tutorials and courses to strengthen your skills and deepen your understanding of version control with Git.