Git porcelain refers to the higher-level commands in Git that are user-friendly and designed for everyday tasks, as opposed to the lower-level plumbing commands that provide more granular control.
Here’s a basic example of a Git porcelain command:
git commit -m "Your commit message here"
What is Git Porcelain?
Git porcelain refers to the set of high-level commands in Git that are designed for managing version control tasks without delving into the underlying complexities of the system. These commands abstract away the intricate details of Git’s plumbing commands, which handle the low-level mechanics that make version control operate. Understanding git porcelain is essential for users ranging from beginners to seasoned developers looking to streamline their workflow without getting lost in the technicalities.
Porcelain commands provide a more user-friendly interface to achieve common tasks. While the underlying plumbing commands manipulate objects in repositories directly, porcelain commands help users focus on the tasks at hand without needing to understand the internal architecture of Git.
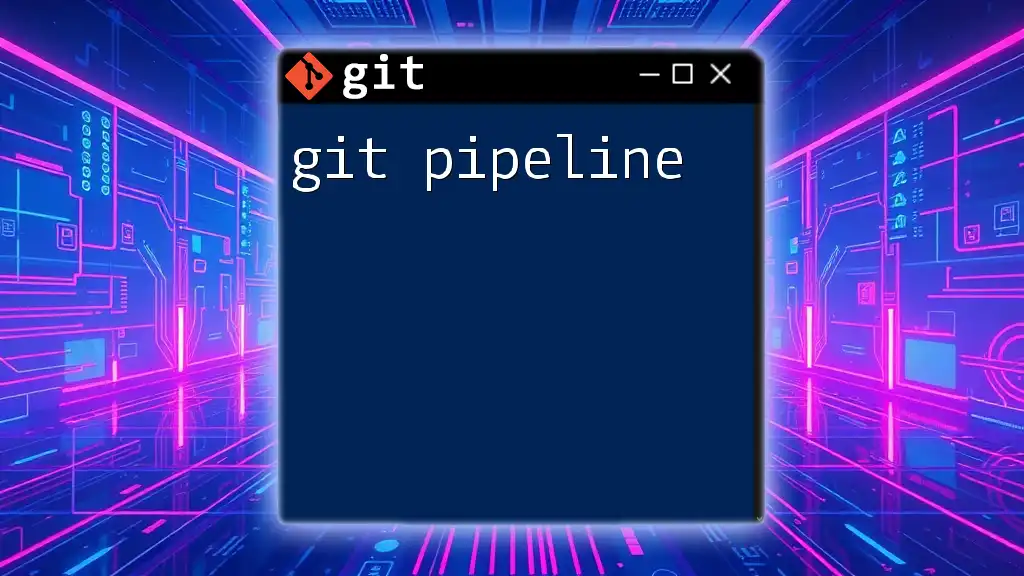
The Purpose of Git Porcelain
The purpose of git porcelain is to make version control accessible. Many users may not require the granularity provided by plumbing commands, and thus, porcelain commands cater to their needs by simplifying operations such as staging changes, committing, and syncing with remote repositories. This user-focused approach makes it easier for beginners to adopt Git without feeling overwhelmed by its capabilities.
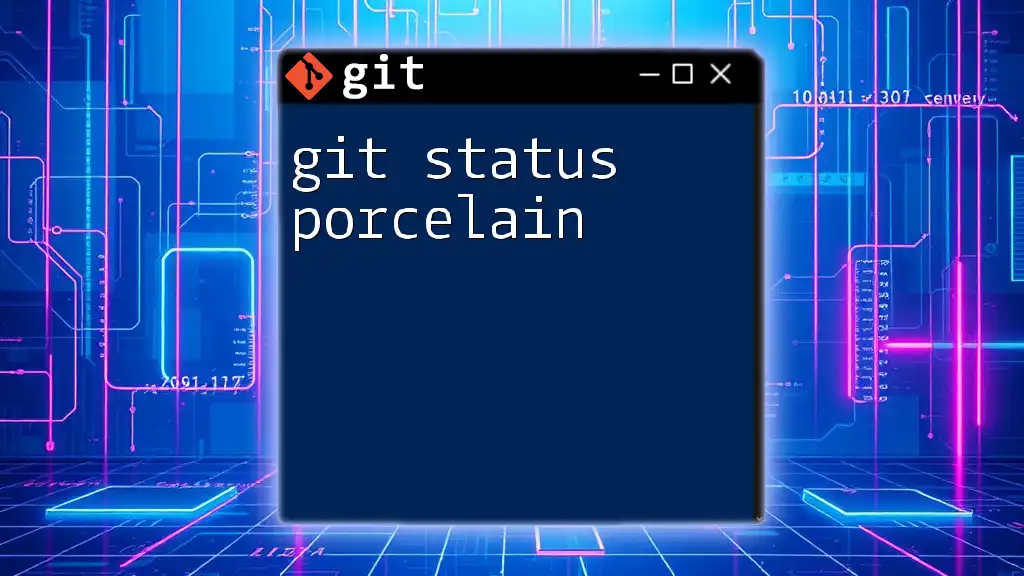
Common Git Porcelain Commands
Git Clone
The `git clone` command is used to create a copy of an existing Git repository. This command is essential when you want to contribute to a project hosted on platforms like GitHub or GitLab.
git clone https://github.com/username/repository.git
When you run this command, Git creates a complete copy of all the repository’s data, including branches and commit history, making it easy for collaborators to start working immediately on the project.
Git Add
Once you've made changes to your files, you need to stage them for a commit using the `git add` command. Staging allows you to select which modifications to include in the next commit.
git add filename.txt
Understanding the staging area is crucial; it acts as a buffer between changes in your working directory and the commit history, allowing you to incrementally build your commit.
Git Commit
The `git commit` command is used to create a snapshot of the staged changes in your repository. It's essential to provide meaningful commit messages to facilitate project tracking and collaboration.
git commit -m "Your commit message"
Committing regularly helps maintain a clean project history, allowing team members and future collaborators to understand the evolution of the project simply by viewing commit logs.
Git Push
After committing your changes, the `git push` command sends those changes to a remote repository. This command is vital for collaborating with others and maintaining an up-to-date project in the cloud.
git push origin main
Using `git push` ensures that your changes are reflected in the remote repository, making them available to team members and contributing to a cohesive codebase.
Git Pull
If you're working with others, you'll want to frequently integrate their changes into your local repository. The `git pull` command is used to fetch and merge changes from a remote repository.
git pull origin main
This command simplifies collaboration, but it's crucial to be aware that conflicts may arise if local changes clash with those fetched from the remote.
Git Status
To keep track of your current changes, the `git status` command is essential. This command provides information about the status of your files in the working directory and staging area.
git status
Using `git status` often can help prevent issues by ensuring you know which files are staged, unstaged, or untracked before you proceed with further Git operations.
Git Branch
Branching is fundamental to Git's version control capabilities, and the `git branch` command allows you to create, list, or delete branches in your repository.
git branch new-feature
Creating branches enables you to work on features or fixes in isolation, contributing to a clean and organized project structure.
Git Checkout
The `git checkout` command is used for switching branches or restoring files in your project. This command is particularly useful for navigating between different lines of development.
git checkout new-feature
By checking out different branches, you can easily switch contexts and test new features without disrupting the main codebase.
Git Merge
When your feature is ready to be integrated into the main project, the `git merge` command allows you to combine two branches. It’s crucial to ensure that the changes from both branches are aligned without conflicts.
git merge new-feature
Merging is an important aspect of collaboration and ensures that contributions from team members are consolidated into a unified codebase.
Git Remote
Managing remote repositories is vital for collaboration in Git. The `git remote` command allows you to view, add, or remove remote connections to repositories.
git remote -v
Understanding how to manage remotes ensures that you can connect your local repository to various online platforms, facilitating seamless collaboration.
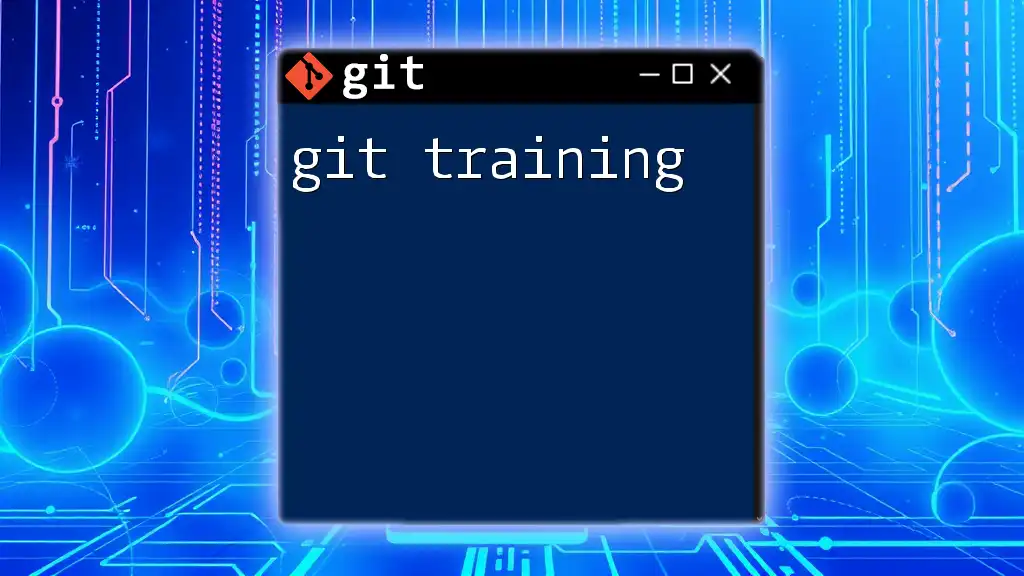
Advanced Porcelain Commands
Git Fetch
The `git fetch` command downloads objects and references from another repository without automatically merging changes. This command is useful for reviewing changes without directly affecting your working branch.
git fetch origin
The key difference between `git fetch` and `git pull` is that fetch only retrieves updates without applying them, allowing you to assess changes before integrating them.
Git Rebase
The `git rebase` command is an advanced feature that allows you to reapply commits on top of another base commit. This helps in maintaining a linear project history.
git rebase main
Rebasing can help streamline your commit history by eliminating unnecessary merge commits, but it’s important to use it carefully, especially in shared branches, to avoid complicating collaboration.
Git Reset
The `git reset` command is powerful and allows you to change the current branch’s HEAD, potentially unstaging or discarding changes.
- Soft Reset:
git reset --soft HEAD~1
This command keeps your staged changes, allowing you to edit your last commit without losing your work.
- Hard Reset:
git reset --hard HEAD~1
A hard reset will remove all changes in your working directory as well as the staging area, effectively reverting to the state of the previous commit. This should be used with caution as it permanently deletes changes.
Git Stash
When you need to pause your current work without committing it, `git stash` saves your changes temporarily. This is useful when you need to switch branches or pull updates but wish to keep your current changes intact.
git stash
Stashing allows you to save your current work, switch to another task, and then return to your stashed changes later, helping to maintain focus and avoid clutter in your timeline.
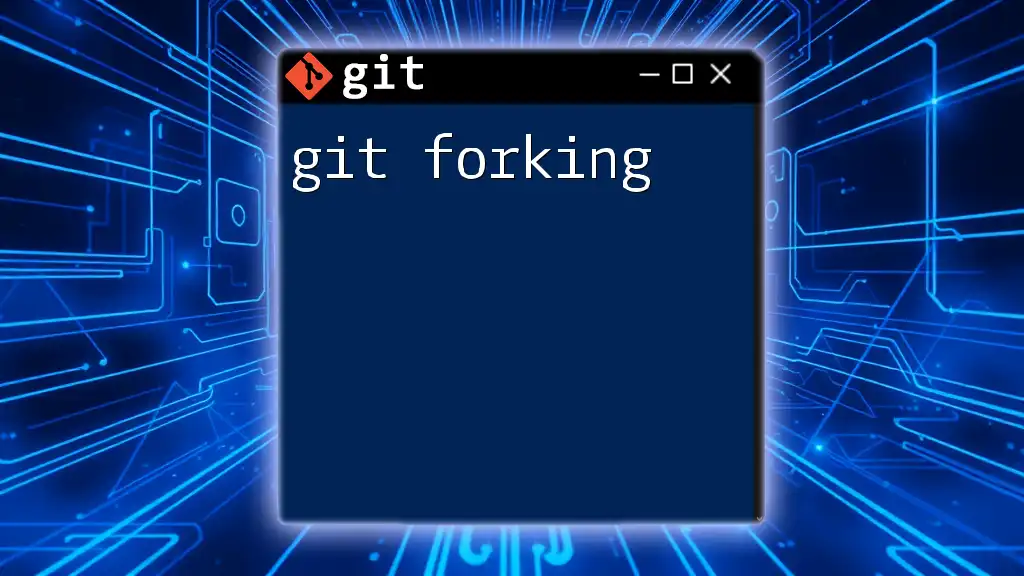
Conclusion
Recap of Git Porcelain Commands
Git porcelain commands provide a streamlined approach to version control, ensuring ease of use for both beginners and experienced developers. Understanding and utilizing these commands enhances productivity, enabling users to manage their projects effectively.
Final Thoughts
Encourage users to practice these commands regularly to gain fluency and confidence in using Git. Mastery of git porcelain will greatly enhance a team’s ability to collaborate and maintain a cohesive project.
Call to Action
If you're eager to learn more about leveraging Git in your workflow, explore our courses or tutorials that delve deeper into the realm of version control with Git!