The `git clone` command is used to create a copy of a remote Git repository on your local machine, enabling you to work on the project offline and track changes independently.
git clone https://github.com/username/repository.git
What is Git Clone?
`git clone` is a fundamental command used in Git that creates a local copy of a remote repository. This command is essential in collaborative environments, where multiple developers may work on the same project. Cloning a repository allows you to download all of the project's history, branches, and data, giving you a complete version of the codebase on your own machine, ready for you to explore and contribute to.
Importance of Cloning
By cloning a repository, you're not just creating a snapshot of the code at a single moment in time; you're also obtaining the entire commit history. This is critical for understanding the evolution of the project and for debugging purposes. It enables you to create new features, collaborate with others, and manage your code effectively.
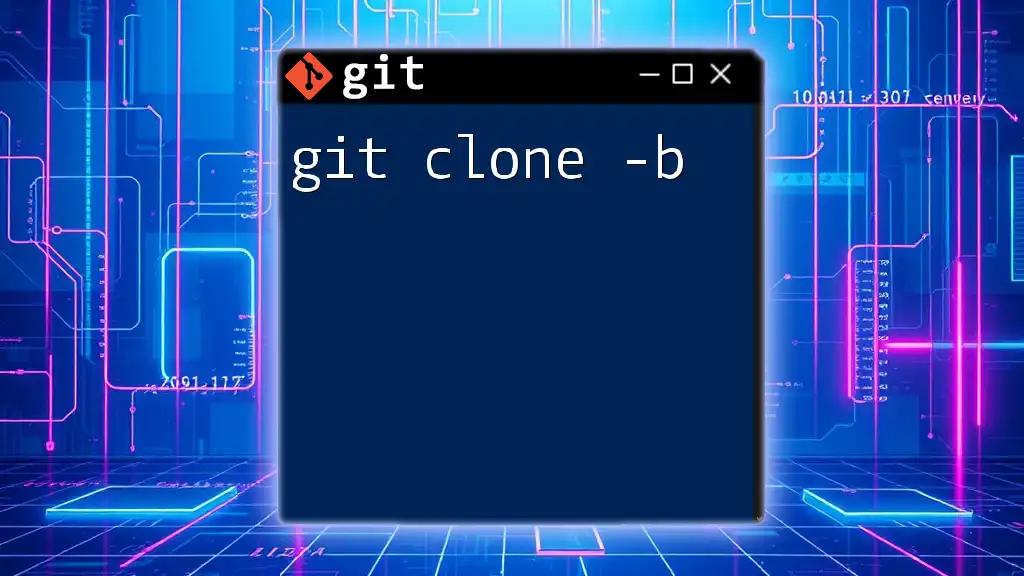
How to Use Git Clone
Syntax
The basic syntax of the `git clone` command is straightforward. It requires the URL of the repository you want to clone:
git clone <repository-url>
This command will create a new directory named after the repository (or the directory name you specify) and populate it with all the files and history from the remote repository.
Examples
- Cloning a Public Repository
To clone a public repository from GitHub, you would use the following command:
git clone https://github.com/username/repo.git
This command will copy the repository located at the specified URL to your machine.
- Cloning a Private Repository
When dealing with private repositories, you have two primary options for authentication: HTTPS and SSH. Here’s an example using SSH:
git clone git@github.com:username/repo.git
Using SSH is advisable for repetitive access, as it allows for easy authentication without the need for username and password prompts.
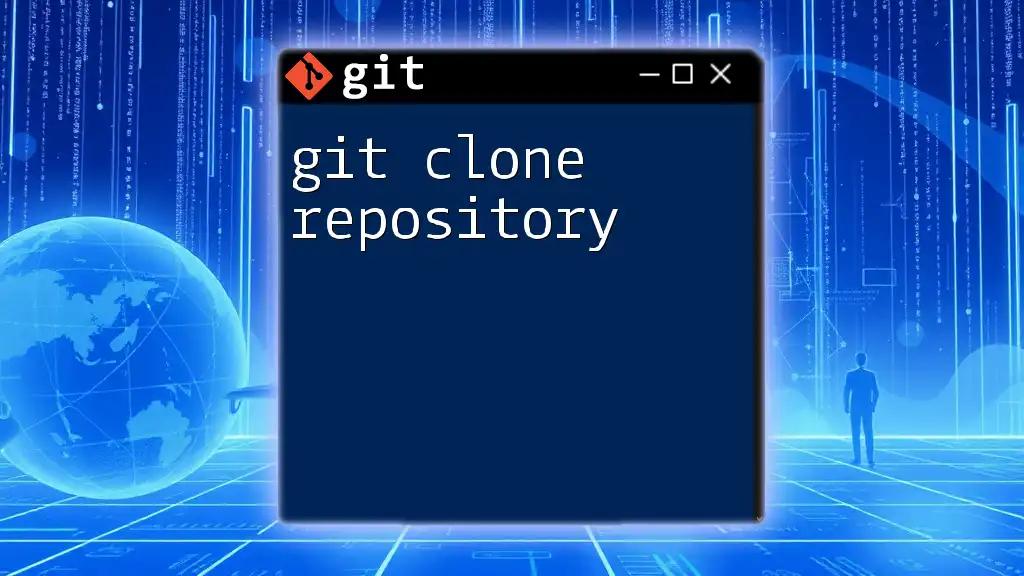
Understanding the Options of Git Clone
Cloning into a Specific Directory
You can customize the destination directory by directly specifying it in the command. This is useful when you want to organize your projects in a specific folder structure. Here’s how to do it:
git clone <repository-url> <directory-name>
This command will clone the repository into a directory named `<directory-name>`, rather than the default name derived from the repository.
Cloning with Depth
Shallow cloning is an option with `git clone` that helps you save time and bandwidth by limiting the history you retrieve. By using the `--depth` flag, you can get only the latest state of the project, without the complete commit history. Here’s an example:
git clone --depth 1 <repository-url>
This command fetches just the most recent snapshot of the repository, which is especially useful for large projects.
Other Useful Flags
- --branch: If you want to clone a specific branch instead of the entire repository, you can do so using the `--branch` flag:
git clone --branch <branch-name> <repository-url>
This will limit the clone to the designated branch, which can save time when you only need to work on a particular feature or fix.
- --recursive: In projects that contain submodules, you will need this option to ensure that those submodules are also cloned:
git clone --recurse-submodules <repository-url>
Without this flag, submodules won't be pulled in, which could lead to missing code dependencies.
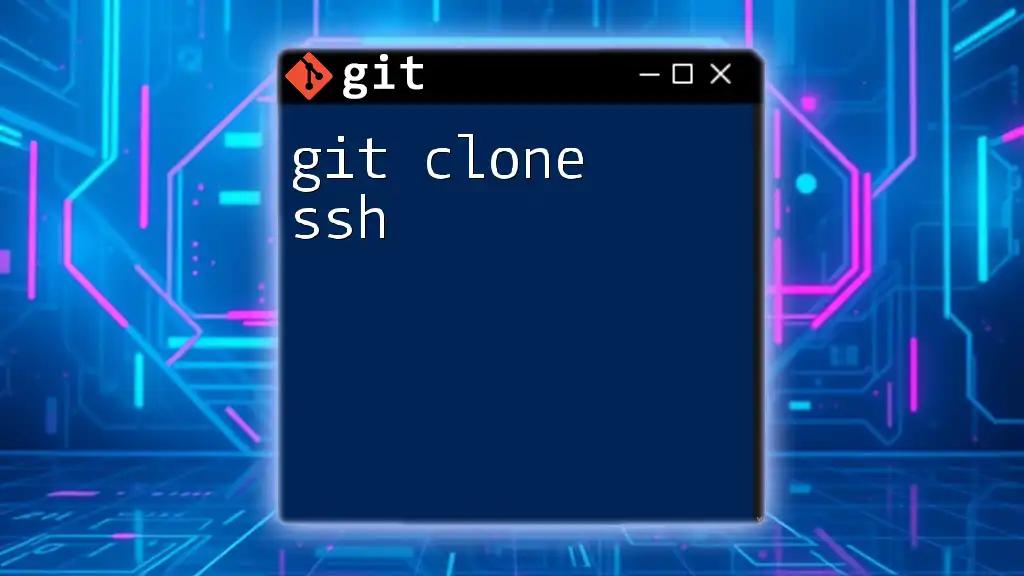
What Happens Under the Hood?
When you execute `git clone`, Git does more than just copy files to your local system. Here's a deeper look at the process:
- Creating a New Directory: Git initializes a new directory that holds your local repository.
- Fetching Data: It downloads all the objects and references (like branches and tags) from the remote repository.
- Creating a Local .git Directory: The `.git` directory is created, where Git stores the metadata and object database. This directory contains all the history of the project, including the complete commit log.
Understanding this process is important, as it highlights how cloning not only provides a snapshot but also the entire development history, enabling you to navigate and manage the project effectively.
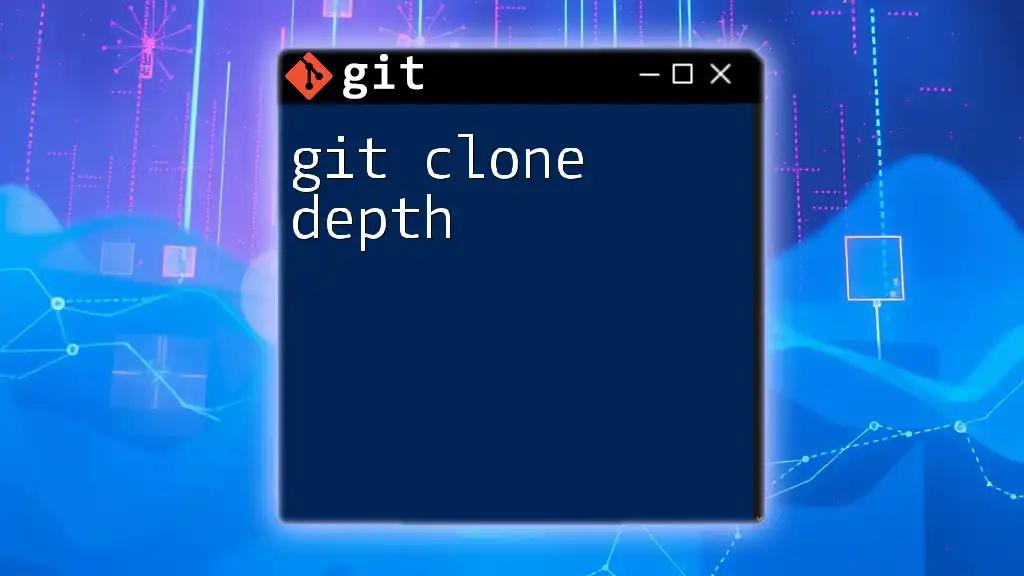
Troubleshooting Git Clone Issues
Common Errors
- Permission Denied: This typically occurs with SSH access. Ensure your SSH key is set up correctly and that your user has permission to access the repository.
- Repository Not Found: A common issue that can arise from typos in the repository URL or due to access restrictions.
Solutions and Workarounds
If you encounter permission issues, consider switching to HTTPS for cloning:
git clone https://github.com/username/repo.git
This method uses typical username and password authentication, which can often simplify access troubles.
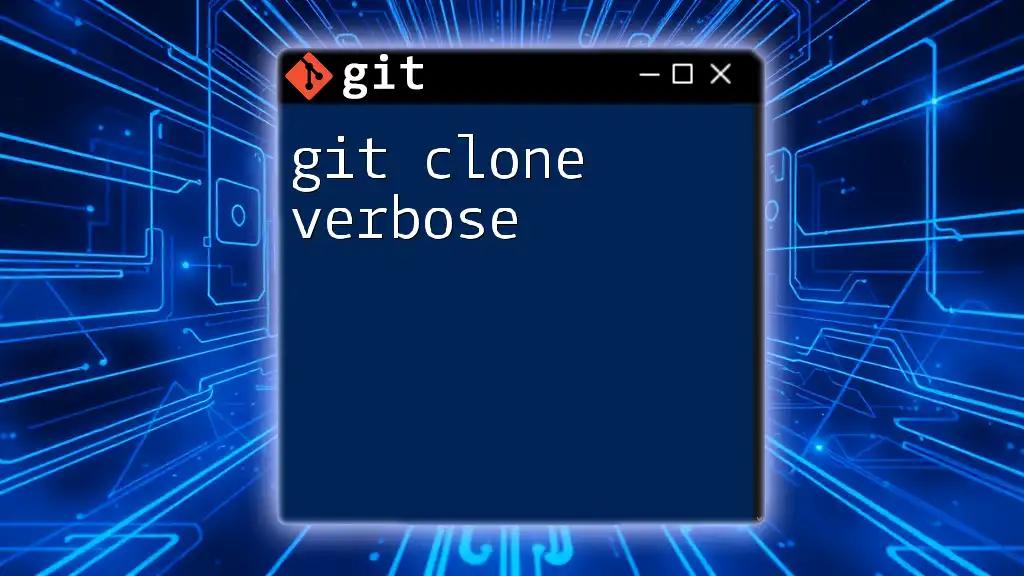
Best Practices for Using Git Clone
Keeping Your Clones Updated
After cloning a repository, it's essential to keep it updated with the latest changes made by collaborators. This can easily be done with the `git pull` command:
git pull origin main
Substituting `main` with the appropriate branch name will integrate the new changes into your local copy, ensuring you're always up to date.
Cloning Efficiently
In projects with large or complex repositories, consider using sparse-checkout. This process involves selectively cloning parts of the repository, allowing you to work with only the files you need. This can significantly reduce the overhead and improve performance when working with massive repositories.
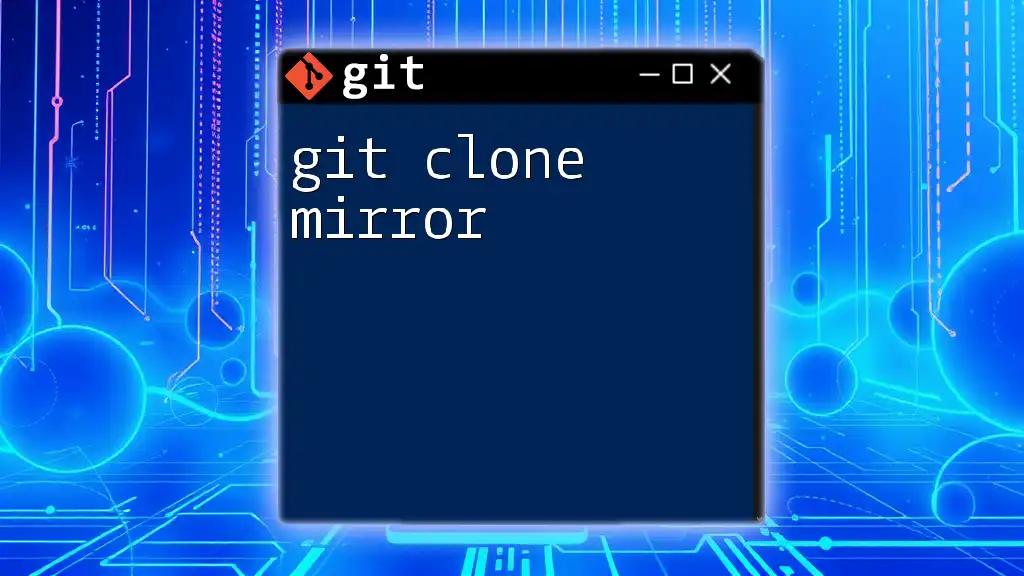
Conclusion
Understanding the `git clone` command is a critical skill for anyone working in collaborative software development. It is the gateway to retrieving and contributing to projects, while also accommodating various workflows and preferences. By leveraging its options and features effectively, you can manage your code more efficiently and maintain alignment with your collaborators.
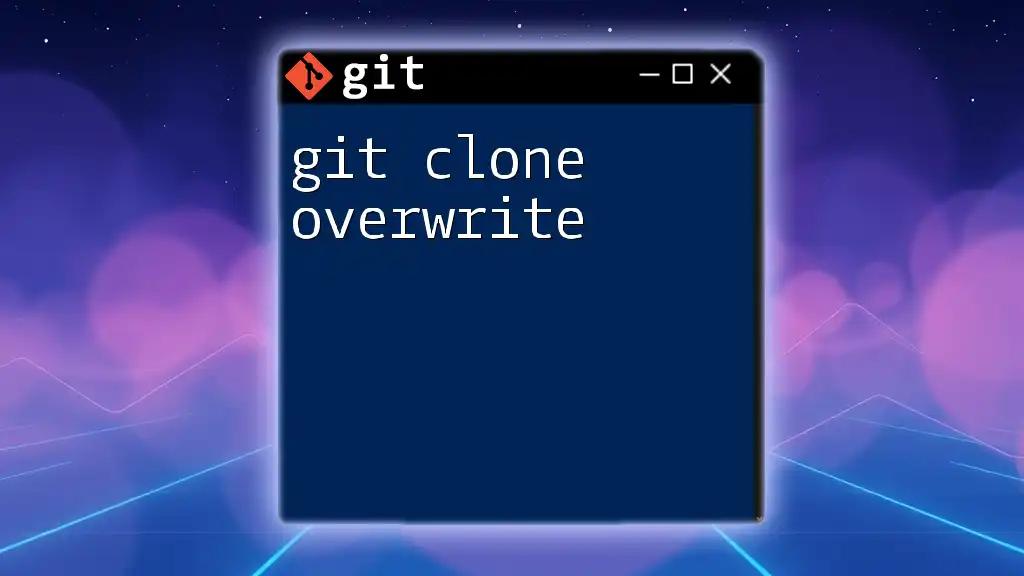
FAQs About Git Clone
What is the difference between `git clone` and `git pull`?
While both commands interact with remote repositories, `git clone` creates a new local copy of a repository, whereas `git pull` updates an existing local repository with changes from its remote counterpart.
Can I `git clone` to a local repository?
Yes, you can clone from a local file path by providing the path instead of a URL. For example:
git clone /path/to/local/repo
How do I remove a cloned repository?
If you need to delete a cloned repository, you can simply remove the directory with the following command, which will delete it entirely:
rm -rf <directory-name>
This command is a powerful tool, so be very careful to specify the correct directory name to avoid accidentally removing important files.
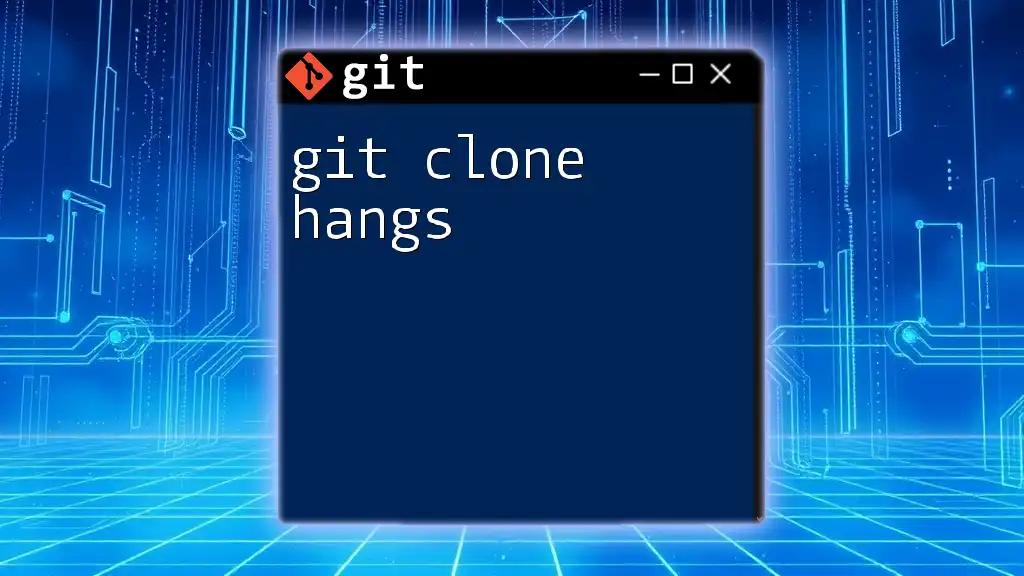
Additional Resources
For further learning and to refine your Git skills, consider exploring the official Git documentation, as well as recommended books and online tutorials that can deepen your understanding and proficiency with version control practices.