To overwrite an existing directory while using `git clone`, you can remove the target directory first or use the `--mirror` option, as the command does not inherently support overwriting.
Here’s a code snippet demonstrating how to remove the existing directory and clone anew:
rm -rf existing-directory && git clone https://github.com/username/repository.git existing-directory
What Does "Clone Overwrite" Mean?
Clone overwrite refers to the process of replacing an existing cloned repository with a fresh copy from a remote source. It is particularly useful in scenarios where the local repository might be corrupted, misconfigured, or simply outdated compared to the remote version. Understanding clone overwrite helps users manage their repositories more effectively and avoid potential data loss.
Common Scenarios for Using Clone Overwrite
There are various situations in which a clone overwrite may become necessary:
- Loss of Changes: If you accidentally lose uncommitted changes or the repository becomes unstable, a fresh clone can restore the last-known good state.
- Corrupt Repositories: Sometimes, local repositories may become corrupted due to failed merges or interrupted operations. Overwriting them can be an effective fix.
- Migrating to a New Branch: When moving to a different branch with distinct configurations and commits, it might be easier to clone the new branch afresh.
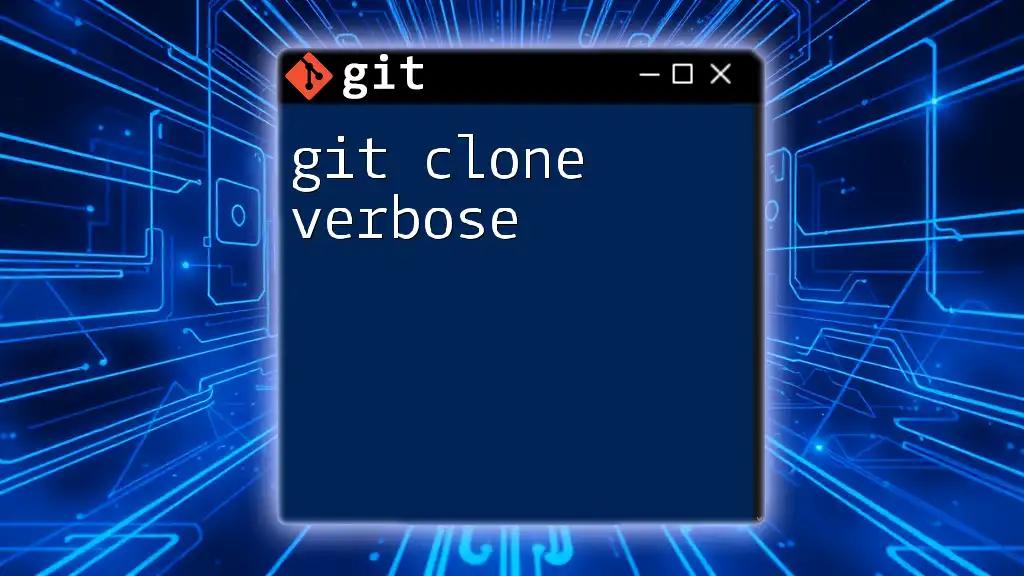
Preparation Before Cloning
Before executing a clone overwrite, it’s essential to prepare adequately for the operation.
Assessing the Current Repository State
Checking the status of your current repository is vital. You can use the following command to see if there are any uncommitted changes:
git status
If you have changes that haven’t been committed yet, you need to decide if you want to keep them or not.
Backup Existing Work
If you have any unsaved work, consider backing it up to preserve your progress. You can use the `git stash` command to temporarily save changes:
git stash
This command will allow you to perform the clone overwrite while keeping your changes safe for later retrieval.
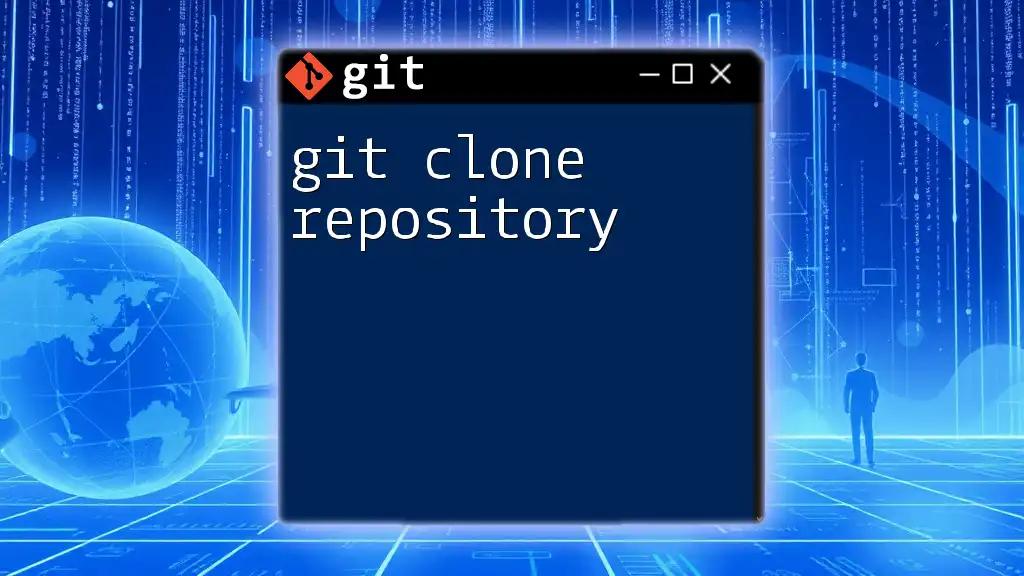
How to Overwrite a Local Repository with Git Clone
Basic Git Clone Command
The fundamental command to clone a repository is:
git clone <repository-url> <target-directory>
This command retrieves a copy of a repository from a remote source and places it in the specified directory.
Forcing an Overwrite on a Local Repository
Although there is no direct `--force` option for `git clone`, we can achieve the same effect through a few alternative steps.
Deleting the Old Directory
To prepare for a clone overwrite, you must first remove the existing repository directory. You can do this using the following command:
rm -rf <target-directory>
Pros and Cons of Directory Deletion
- Pros: Starting with a clean slate can resolve issues associated with corrupted files or improperly merged branches.
- Cons: You may lose any local changes that weren’t committed or stashed, so always be sure to back up your work first.
Executing the Clone Command
Once the existing repository directory is deleted, you can proceed with the clone command:
git clone <repository-url> <target-directory>
This command will download a fresh copy of the repository, ensuring that you have the latest version available from the remote source.
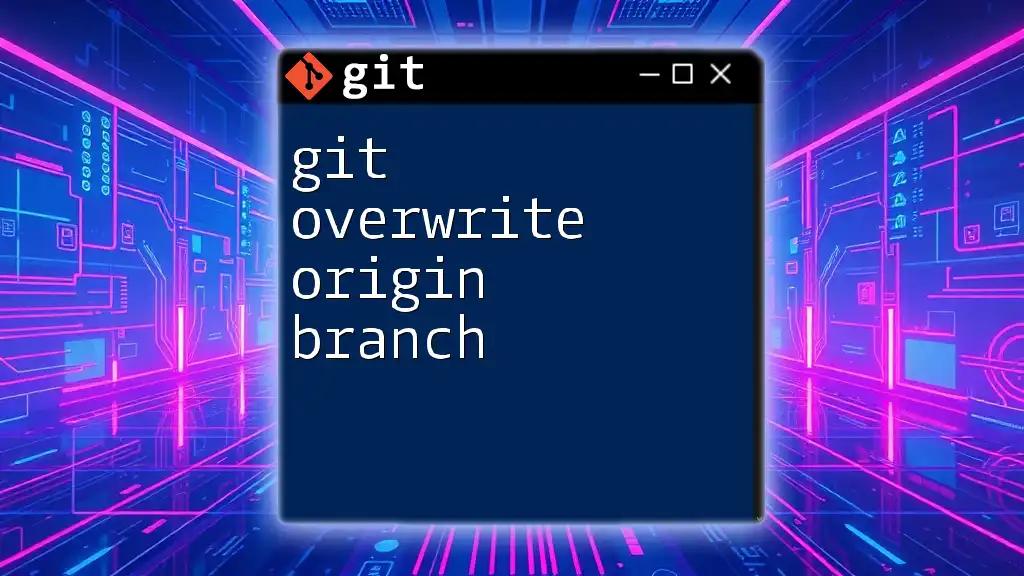
Alternatives to Cloning for Overwriting
Using Git Pull for Updates
If your goal is simply to update your local repo while maintaining any changes, consider using `git pull`. The command integrates changes from the remote branch into your current branch:
git pull origin main
This method is generally safer for those who have made local changes that they do not want to lose.
Using Git Fetch and Reset
Another alternative to cloning is to fetch the changes you need and then reset your local branch. This approach allows you to overwrite your local changes without deleting your entire repository. Use the following commands:
git fetch origin
git reset --hard origin/main
This command sequence will update your local repository with the latest remote changes while discarding local changes.
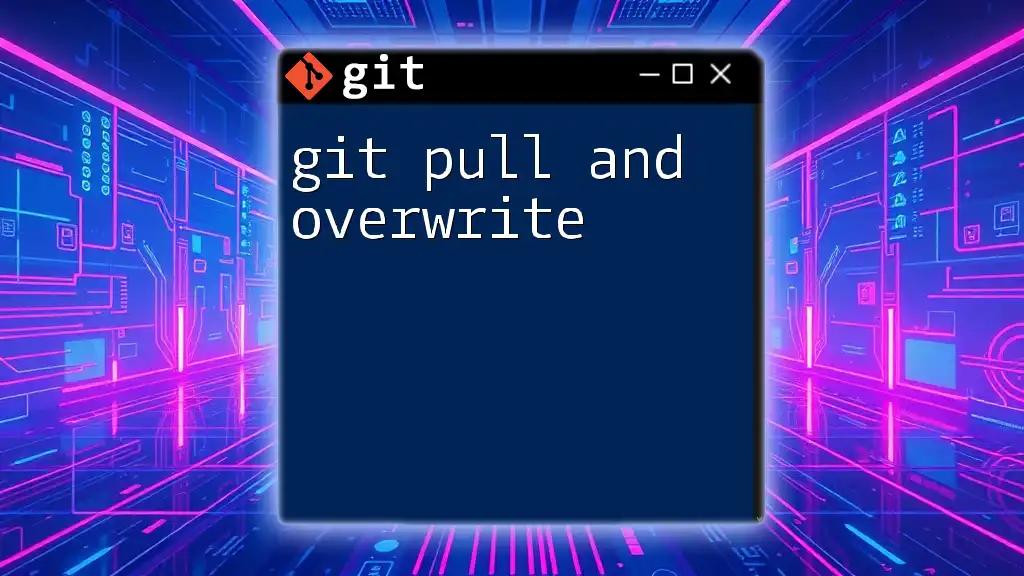
Best Practices for Cloning Repositories
Documentation and Commenting
Always document your processes when working with Git. Clear comments can facilitate easier navigation and understanding of past actions for you and others.
Regular Updates
Encourage making regular updates through `git pull` or `git fetch` to avoid the need for drastic overwrites. Regularly syncing your local repository ensures you are aware of changes and can avoid losing any vital work.
Setting Up Remotes Properly
Properly manage your remotes to avoid confusion later on. Check your remote URLs with:
git remote -v
Ensuring your remotes are set up correctly can prevent potential clone overwrite issues down the line.
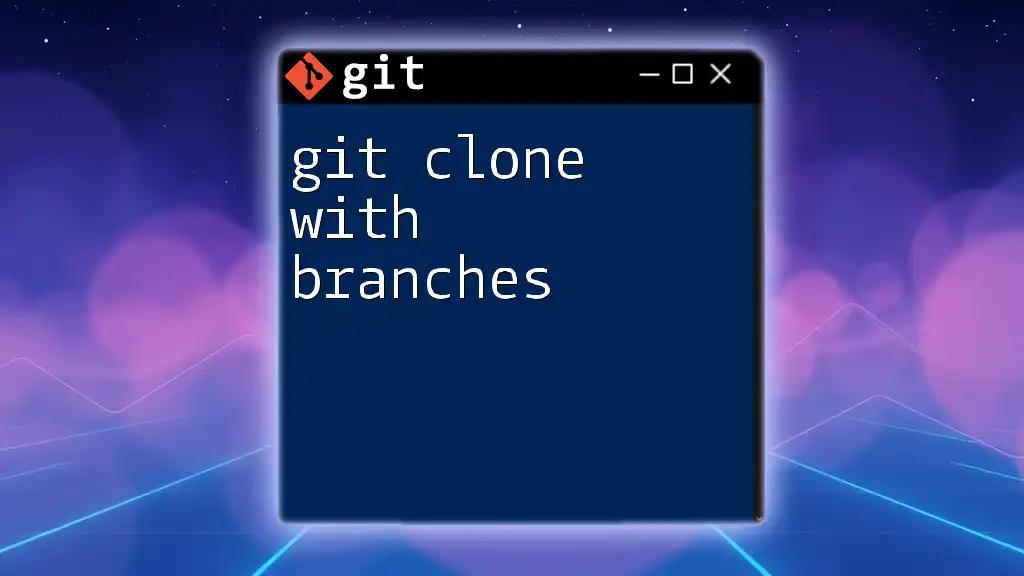
Common Pitfalls and Troubleshooting
Removing a Non-Empty Directory
Attempting to remove a directory that contains files or subdirectories may lead to errors. Always ensure that you are deleting the right directory and are okay with losing its contents.
Handling Permission Errors
When executing delete commands, you might encounter permission errors, especially on operating systems with more restricted permissions settings. You may need to run commands with `sudo` for higher privilege levels.
Understanding Merge Conflicts Post-Clone
If you choose to update rather than clone, be aware of potential merge conflicts. A merge conflict arises when changes in the local and remote repositories conflict and cannot be automatically reconciled by Git. Always review unresolved changes carefully and resolve them promptly.
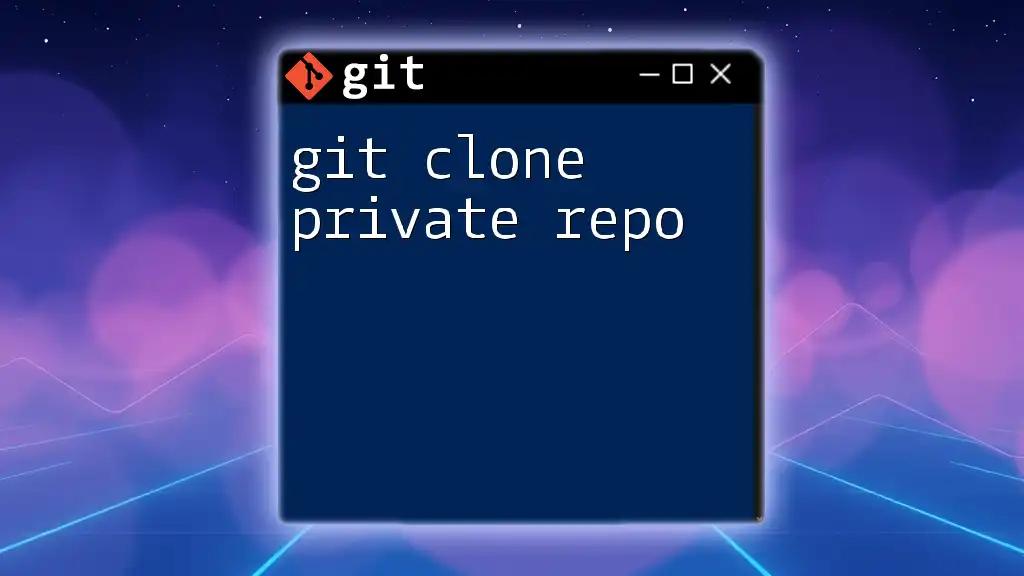
Conclusion
Understanding how to effectively use git clone overwrite is vital for anyone working in version control. By mastering the preparation, execution, and alternatives to the cloning process, you can maintain a clean and efficient workflow. Always remember to document your processes, keep backups, and stay updated to minimize the need for drastic measures in the future.