To clone a Git repository using an SSH key for authentication, you can execute the following command in your terminal:
git clone git@github.com:username/repository.git
Make sure to replace `username` and `repository` with the appropriate GitHub username and repository name.
Understanding Git Clone
What is Git Clone?
The `git clone` command is an essential feature in Git that allows users to create a local copy of a remote repository. When you clone a repository, you get not only the files but also the entire history of the project, including all branches and commits. This provides a complete working copy that you can modify independently from the original.
Typical use cases for cloning a repository include:
- Contributing to open-source projects by obtaining their source code.
- Creating a backup of an existing repository.
- Setting up a new development environment or onboarding new team members.
The Role of SSH in Git
SSH, or Secure Shell, is a protocol that allows secure communication between systems. When using Git, SSH provides a secure method for authenticating your identity without needing to repeatedly enter a username and password.
Benefits of using SSH for Git:
- Security: SSH encrypts the data transmitted between your local machine and the remote repository, protecting your credentials.
- Convenience: Once set up, SSH key authentication allows you to clone and pull repositories without entering your credentials every time.
- Compatibility: Most major Git hosting platforms, such as GitHub, GitLab, and Bitbucket, support SSH.
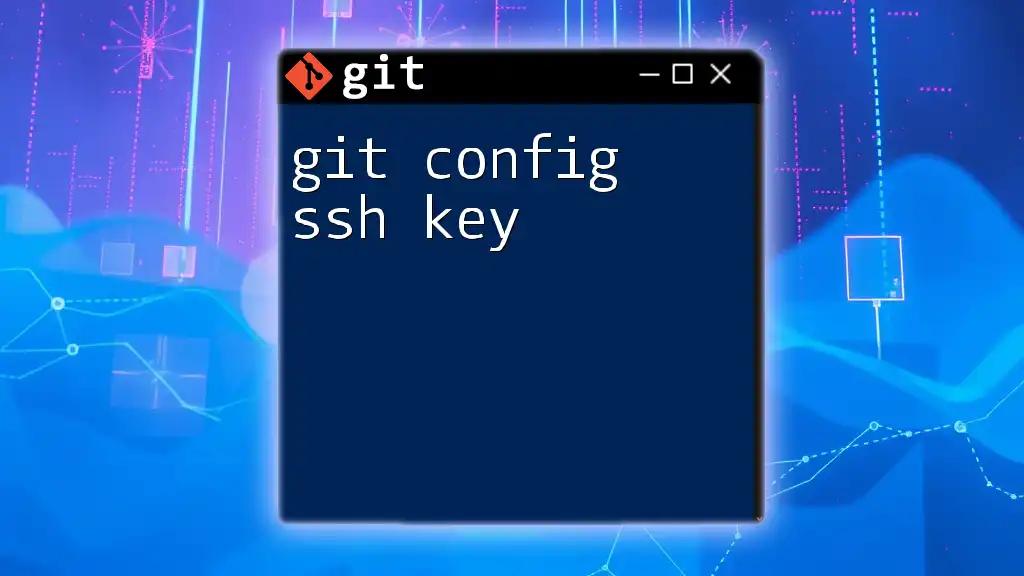
Setting Up SSH Keys
Generating SSH Keys
To use `git clone with ssh key`, the first step is to generate an SSH key pair on your local machine. This consists of a public key and a private key.
Run the following command in your terminal:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
Understanding the command:
- `ssh-keygen`: The command used to create a new SSH key pair.
- `-t rsa`: Specifies the type of key. RSA is a widely used algorithm.
- `-b 4096`: Sets the length of the key. A longer key is generally more secure.
- `-C "your_email@example.com"`: Adds a comment to the key, making it easier to identify.
Follow the prompts to specify where to save the key and add a passphrase if desired.
Adding SSH Key to the SSH Agent
After generating the keys, you need to ensure that they are added to the SSH agent, which manages your SSH keys for authentication.
Start the SSH agent with:
eval "$(ssh-agent -s)"
Then, add your SSH private key to the agent:
ssh-add ~/.ssh/id_rsa
Note: The `~/.ssh/id_rsa` path assumes you’ve accepted the default location during key generation. If you chose a different name or location, adjust accordingly.
Adding SSH Key to Your Git Hosting Service
For your SSH keys to be recognized by a Git hosting service, you need to add the public key to your account.
GitHub
- Navigate to Settings.
- Select SSH and GPG keys.
- Click on New SSH key.
- Paste your public key (found in `~/.ssh/id_rsa.pub`) into the key field and provide a title.
- Click Add SSH key.
GitLab
- Go to User Settings.
- Click on SSH Keys.
- Paste your SSH public key and a title.
- Click Add key.
Bitbucket
- Select your avatar and choose Personal settings.
- Click on SSH keys.
- Click Add key and paste your public key.
- Click Add SSH key.
Ensure that the key is correctly added; otherwise, you will face authentication issues when cloning repositories.
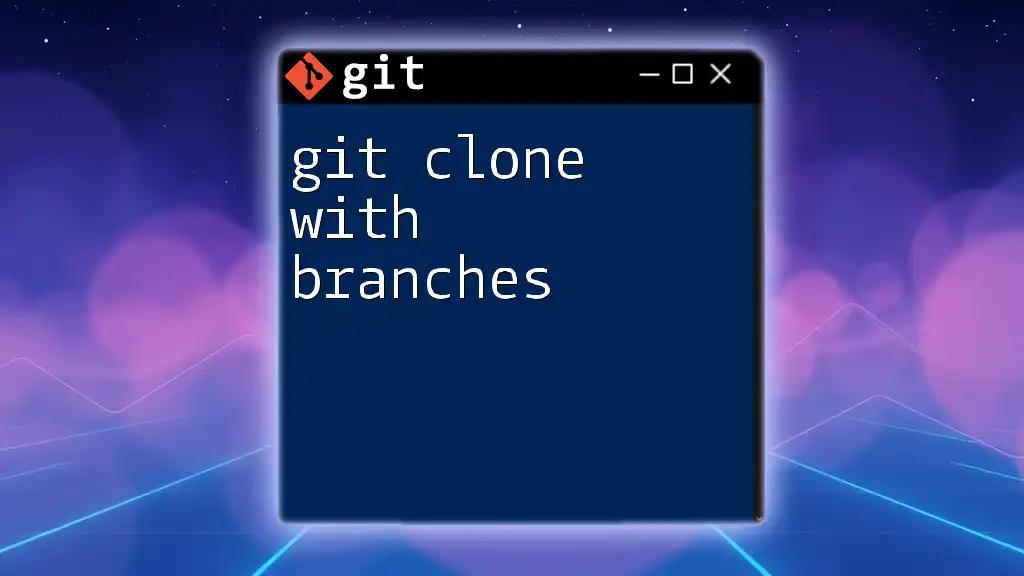
Cloning a Repository Using SSH
Finding the SSH URL
To clone a repository, you need to obtain the SSH URL. This can usually be found on the repository’s main page.
Example of an SSH URL:
git@github.com:username/repository.git
Make sure to copy the SSH URL rather than the HTTPS one to ensure you're using SSH authentication.
Executing the Git Clone Command
Once you have the SSH URL, you can clone the repository. The general syntax for the `git clone` command is as follows:
git clone git@github.com:username/repository.git
Explanation of the command:
- `git clone`: This command is used to create a copy of the target repository.
- The SSH URL (`git@github.com:username/repository.git`): This specifies the remote repository from which you are cloning.
Common Issues and Troubleshooting
While using `git clone with ssh key`, some common issues may arise. Here are the typical problems along with troubleshooting steps:
Permission Denied (publickey)
- This error occurs when Git cannot authenticate your identity using the SSH key.
- Possible solutions include:
- Checking if your public key is correctly added to your Git hosting service.
- Ensuring that your SSH agent is running and the SSH key is added.
- Verifying that the SSH key permissions are correct (use `chmod 600 ~/.ssh/id_rsa`).
Connection Timeout
- This can happen due to network issues or incorrect SSH configuration.
- Check your internet connection, and ensure that the SSH service is available for your Git hosting service.
SSH Key Not Recognized
- If your key isn’t recognized, ensure that you are using the correct version of Git and that your SSH configuration file is set up accurately (`~/.ssh/config`).
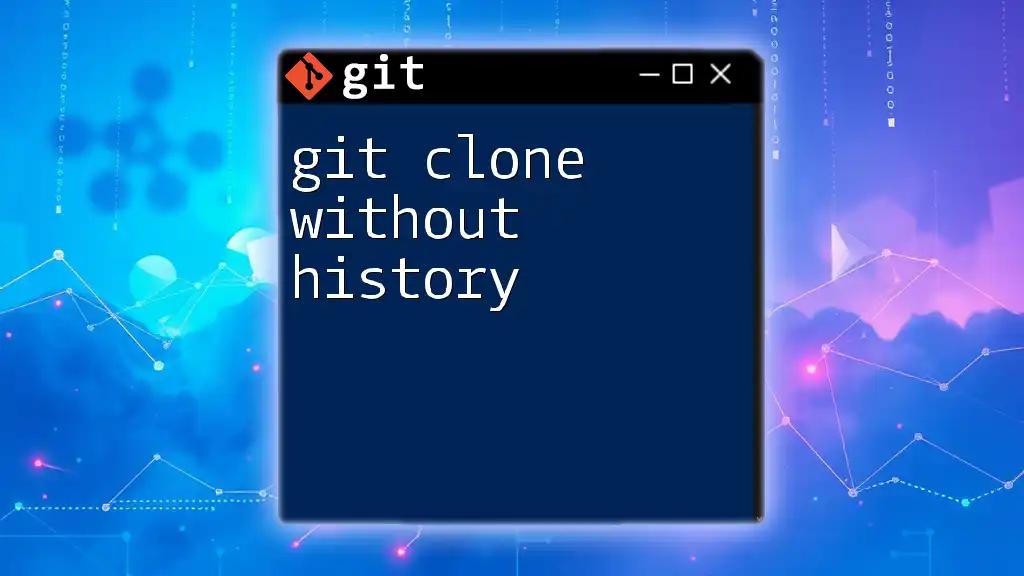
Best Practices for Using SSH with Git
Regularly Rotate Your SSH Keys
To maintain strong security, it’s wise to regularly rotate your SSH keys. This means generating new key pairs and removing old ones from your Git hosting accounts. Regular rotations help limit exposure if a key is compromised.
Use a Passphrase for Your SSH Key
Adding a passphrase to your SSH key provides an additional layer of security. Even if someone obtains your private key, they cannot use it without the passphrase. It’s a simple yet effective measure to safeguard your credentials.
Monitor SSH Key Usage
Regularly review the SSH keys added to your Git service accounts. If you see inactive keys that are no longer in use, consider revoking their access. This can help prevent unauthorized access and enhance your overall security posture.
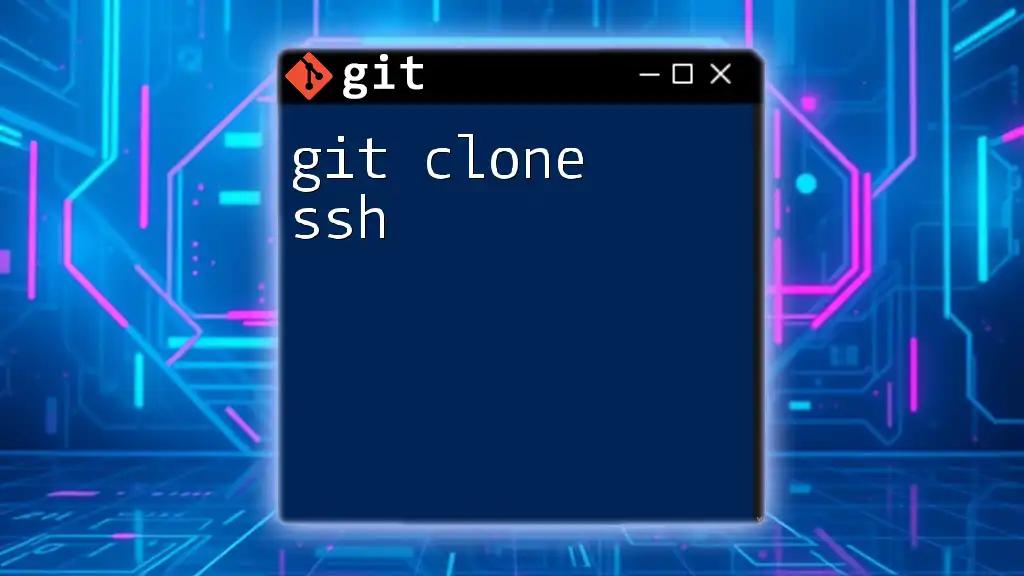
Conclusion
Using `git clone with ssh key` significantly enhances the security and convenience of managing your Git repositories. This guide has provided you with the necessary steps to set up SSH keys, clone repositories, and troubleshoot common issues. By following these best practices, you can ensure a more secure and efficient workflow in your Git activities.