To clone a Git repository without its history, you can use the `--depth` option with the `git clone` command to create a shallow copy.
git clone --depth 1 <repository-url>
Understanding Git Clone
What is Git Clone?
`git clone` is a command used to create a local copy of a specific repository. This command not only copies all the files in the repository but also brings along the full history of all commits, branches, and tags. Cloning is essential in collaborative projects, as it allows developers to work in local environments while maintaining synchronization with remote repositories.
Why Clone Without History?
Cloning a repository with history can lead to significant storage consumption, especially with large projects that have extensive commit histories. When you want to work with just the latest version of the code, cloning without history becomes an effective solution. This method provides a way to:
- Reduce disk space usage: By omitting the commit history, you keep your local repository lightweight.
- Speed up the cloning process: Fewer data means faster retrieval times, ideal if you are working with multiple clones.
- Simplify large repositories: If you’re only interested in the latest code, excluding the history avoids the complexity associated with it.
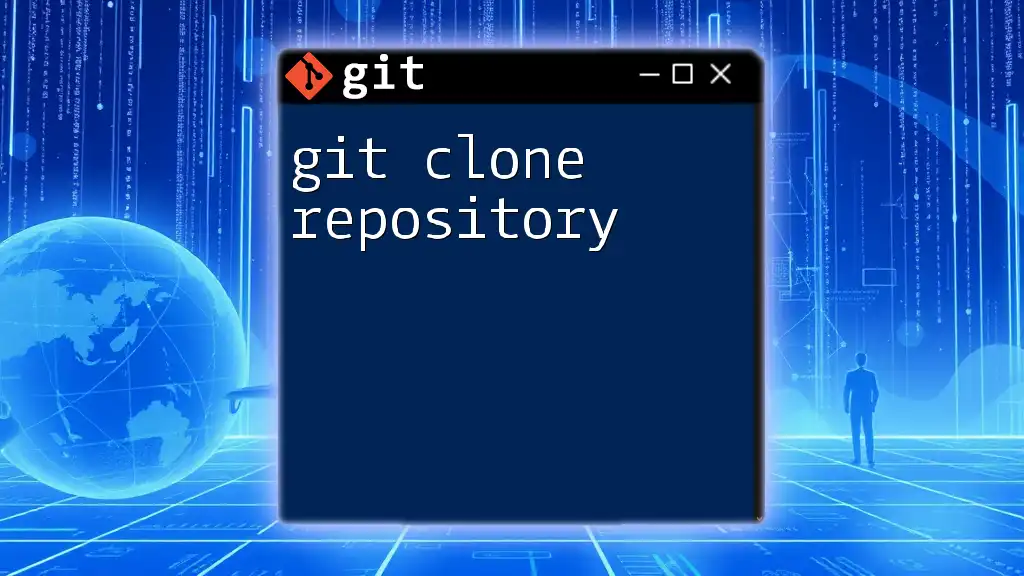
Methods to Clone Without History
Using `git clone --depth`
One of the most straightforward methods to clone without history is utilizing the `--depth` option. This flag allows you to create a shallow clone, meaning only the most recent commits are downloaded.
To perform a shallow clone, run the following command:
git clone --depth 1 <repository_url>
In this command, `--depth 1` specifies that you want only the latest snapshot of the branch without any preceding commits. This means that if you later decide to fetch more history, you will need to run additional commands, as the previous commits are not present in your local repository.
Using Sparse Checkout
Another method for when you want limited data is through Sparse Checkout. This technique allows you to check out only particular files or directories from a repository without requiring the entire history. Here’s how you can set it up:
-
Initialize a new repository: Start by creating a new local Git repository.
-
Enable sparse checkout: Configure the repository to allow sparse checkout.
-
Specify the files or directories you want: Define which parts of the repository to check out.
Here’s a step-by-step process with the necessary commands:
git init <repository_name>
git remote add origin <repository_url>
git config core.sparseCheckout true
echo "path/to/folder/*" >> .git/info/sparse-checkout
git pull origin main
This method grants you the flexibility of selecting only the pieces of the repository you need, while still reducing your overall data intake.
Cloning with No History using Custom Scripts
For those who prefer a scripted approach, creating a custom script can automate the process of cloning without history. This method allows for more customization depending on your specific requirements. Here’s an example of a simple Bash script that accomplishes this:
#!/bin/bash
REPO_URL="$1"
git init
git remote add origin "$REPO_URL"
git fetch --depth=1 origin main
git checkout -b main origin/main
In this script, replace `"$1"` with the actual repository URL, making it easy to reuse for multiple repositories. It’s a clean and efficient way to ensure you only pull in the most critical data.
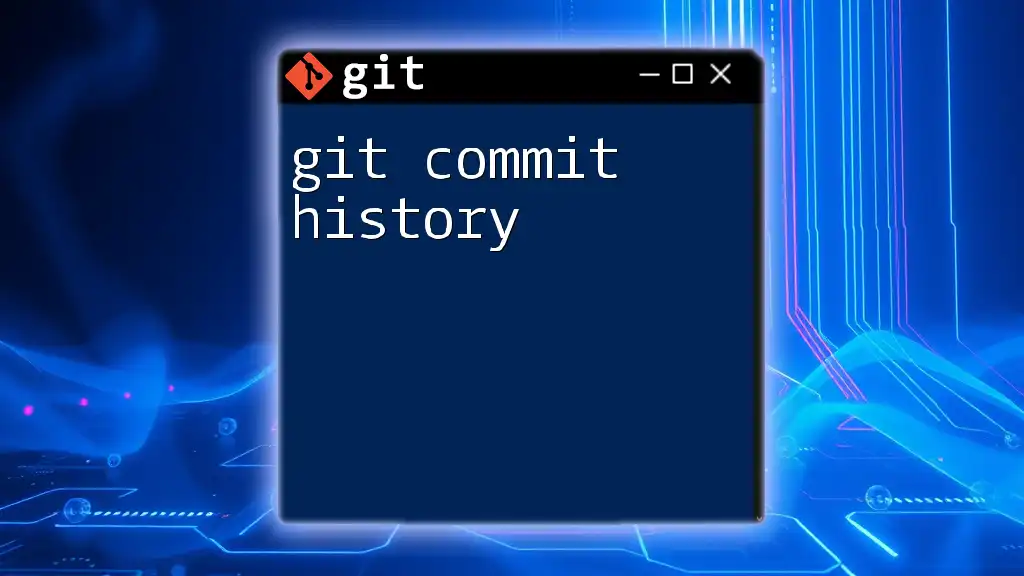
Advantages and Limitations
Advantages of Cloning Without History
- Reduced cloning time: As mentioned earlier, fewer files and data lead to a swifter clone process, saving you time, particularly in environments where speed is essential.
- Lower resource consumption: This method conserves both storage space and system resources, making it suitable for development environments with limited resources.
- Cleaner working environment: By focusing solely on the most recent files, your workspace remains uncluttered and easier to navigate.
Limitations
Despite its advantages, cloning without history does come with limitations that should be noted:
- Lack of commit history for tracking changes: Without access to previous commits, you won't be able to see who made what changes, which can be crucial for debugging or understanding project evolution.
- Potential issues with branch management and merge conflicts: Without historical context, managing branches and understanding how the code has evolved over time may become more challenging.
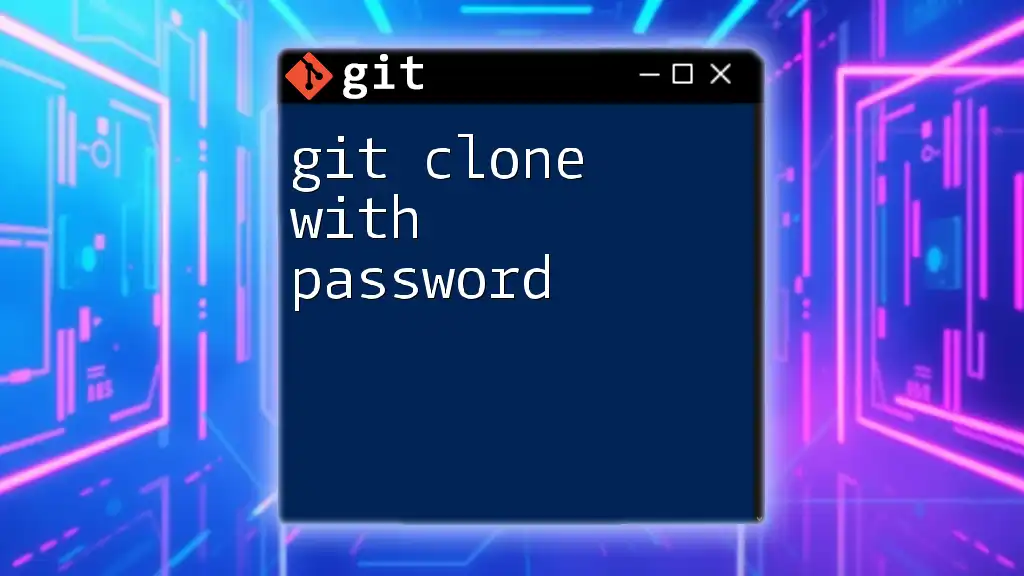
Use Cases for Cloning Without History
There are several real-world scenarios where cloning without history proves useful:
- Using only the latest version of large projects: If you're working with libraries or frameworks where only the latest stable version matters, excluding the history is efficient.
- Development of temporary features: For projects with short-lived features, where full history isn't necessary, cloning without history might make sense.
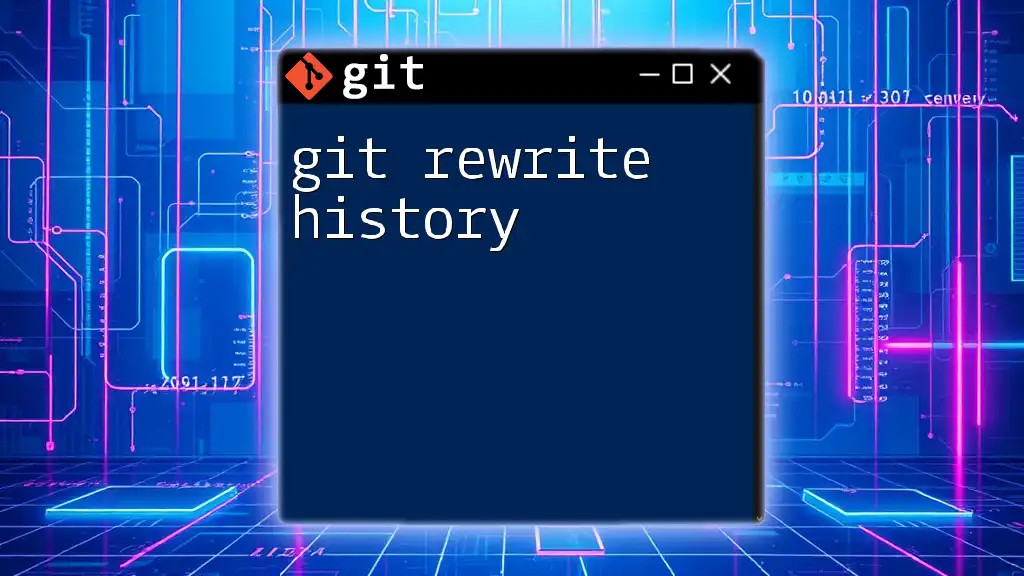
Best Practices
When to Use Cloning Without History
It’s essential to determine when cloning without history is appropriate. This technique is most beneficial when:
- You are working on a large project but only require a small component.
- You need to focus on prototyping or development of temporary features without the complexity of full commit history.
- You are collaborating in a fast-paced environment where quick cloning times are more significant than version tracking.
Recommendations for Project Management
To manage projects effectively while working with shallow or sparse clones, consider the following:
- Regularly assess your need for commit history: Be ready to switch to a complete clone if your dependencies or tasks evolve.
- Maintain clear communication with team members about cloning practices so everyone understands which version of the repository is being used.
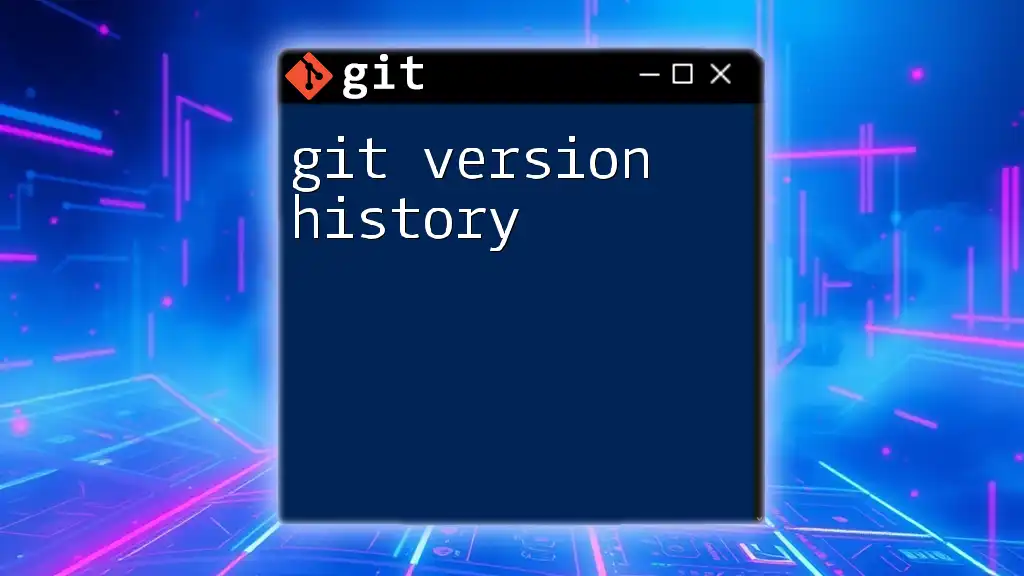
Conclusion
Understanding the various commands and methods for git clone without history is vital for optimizing your workflow and resource management. Whether you choose to leverage shallow clones or utilize sparse checkouts, these techniques can greatly enhance your development experience. Experiment with the approaches outlined, and consider how you can streamline your cloning processes to meet your project needs effectively.
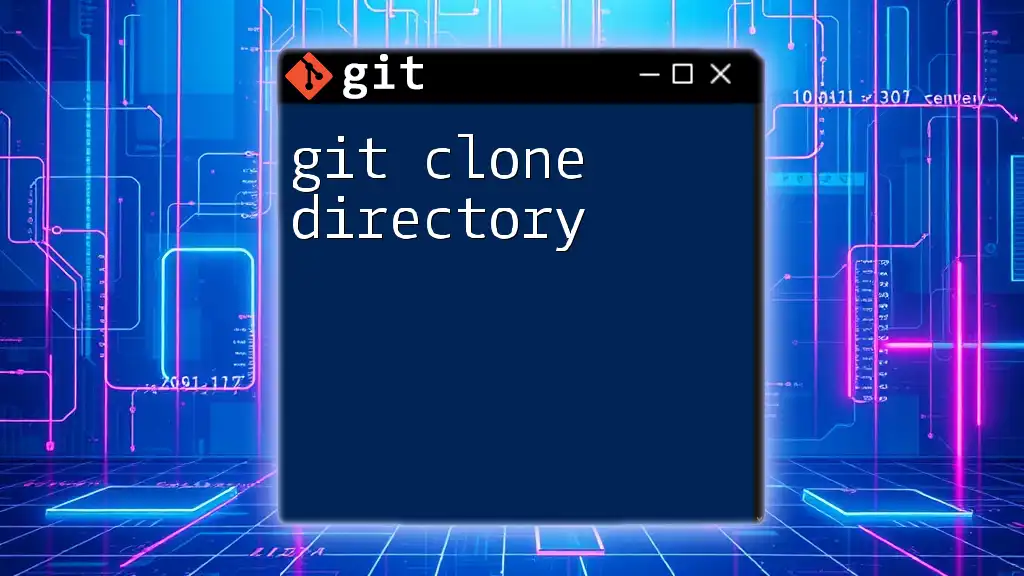
Additional Resources
To further your knowledge, explore the official [Git Documentation](https://git-scm.com/doc), or look into video tutorials that illustrate these concepts in practice. As you become more comfortable with Git, you'll find that mastering cloning techniques will help simplify collaboration and enhance productivity.