To clone a private Git repository, you need to provide the repository's URL along with your authentication credentials; here’s an example command using SSH.
git clone git@github.com:username/private-repo.git
Understanding Git Authentication
What is Git Authentication?
In the context of Git, authentication ensures that you have the right to access a repository. This becomes especially critical for private repositories, where user credentials determine who can view or manipulate the content. Without proper authentication, any attempt to clone a private repo will result in an error.
Types of Authentication Methods
When cloning a private repository, you typically have two main methods of authentication: HTTPS and SSH.
- HTTPS is straightforward and uses your Git username and password (or a personal access token) to authenticate. While it’s easier to set up, it's a bit less secure if your credentials are exposed.
- SSH uses key pairs for authentication. This method provides greater security since it doesn’t require you to enter your username and password frequently. However, it requires an additional setup step of generating SSH keys.
Personal Access Tokens
Due to recent security measures, many platforms have transitioned from password-based authentication to personal access tokens for Git operations. A personal access token allows you to authenticate without using a password and is often required when using the HTTPS method.
To create a personal access token:
- Navigate to your Git service (like GitHub or GitLab).
- Go to your account settings and find the Developer settings or Access tokens option.
- Generate a new token with the necessary scopes (permissions), often `repo` for accessing repositories.
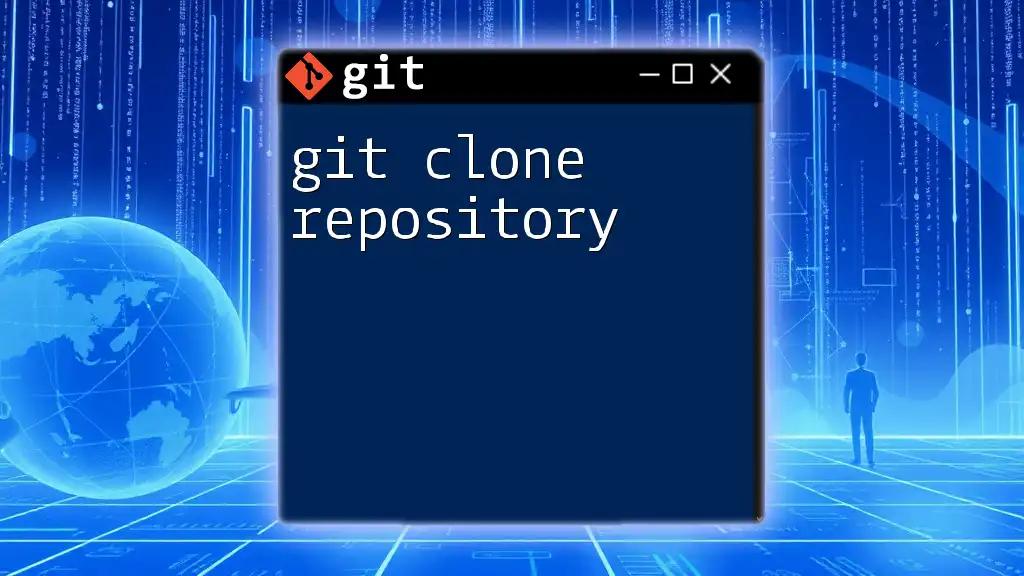
Prerequisites for Cloning a Private Repository
Setting Up Your Git Environment
Before cloning a private repository, ensure you have Git installed on your machine. Check your installation by running:
git --version
If Git is not installed, follow the instructions specific to your operating system to download and install it.
Ensure You Have Access
Ensure you have the right access privileges to the private repository you want to clone. If you’re not sure, reach out to the repository owner for verification. Issues with access can usually be resolved quickly by adjusting permission settings.
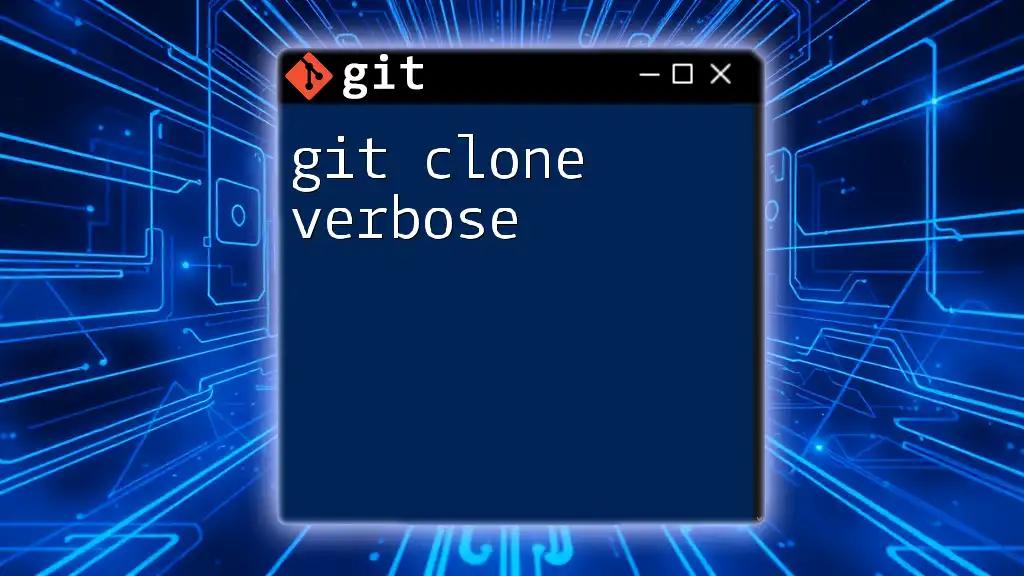
Step-by-Step Guide to Clone a Private Repository
Cloning via HTTPS
Step 1: Getting the Repository URL
The first step is to obtain the clone URL for the private repository:
- On platforms like GitHub, GitLab, or Bitbucket, navigate to the main page of the repository.
- Find the Clone or Download option, and copy the provided HTTPS link.
Step 2: Executing the Clone Command
Now, with the repository URL in hand, you can execute the clone command in your terminal or command prompt:
git clone https://github.com/username/repo.git
Replace `https://github.com/username/repo.git` with the URL you copied.
Step 3: Entering Credentials
Upon running the clone command, you will be prompted to enter your credentials. This usually includes your Git username and personal access token for authentication:
Username: your_username
Password: your_personal_access_token
Simply input your details, and Git will clone the repository to your local machine.
Cloning via SSH
Step 1: Ensure SSH Keys are Set Up
If you prefer using SSH for cloning, ensure that you have SSH keys set up. If you don't have a key yet, you can generate one using this command:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
Follow the prompts to save the key to the default location and add a passphrase for added security.
Step 2: Adding SSH Key to Your Git Account
After generating the SSH key, the next step is to add it to your Git account:
- Copy the contents of your SSH public key:
cat ~/.ssh/id_rsa.pub
- Go to your Git platform’s settings and find the SSH and GPG keys section.
- Click on New SSH key, paste your key into the provided field, and save it.
Step 3: Executing the Clone Command
With your SSH key correctly configured, you can now clone the repository using the SSH URL. You can find this URL alongside the HTTPS URL on your Git platform:
git clone git@github.com:username/repo.git
Just remember to replace `git@github.com:username/repo.git` with the actual SSH link of your repository.
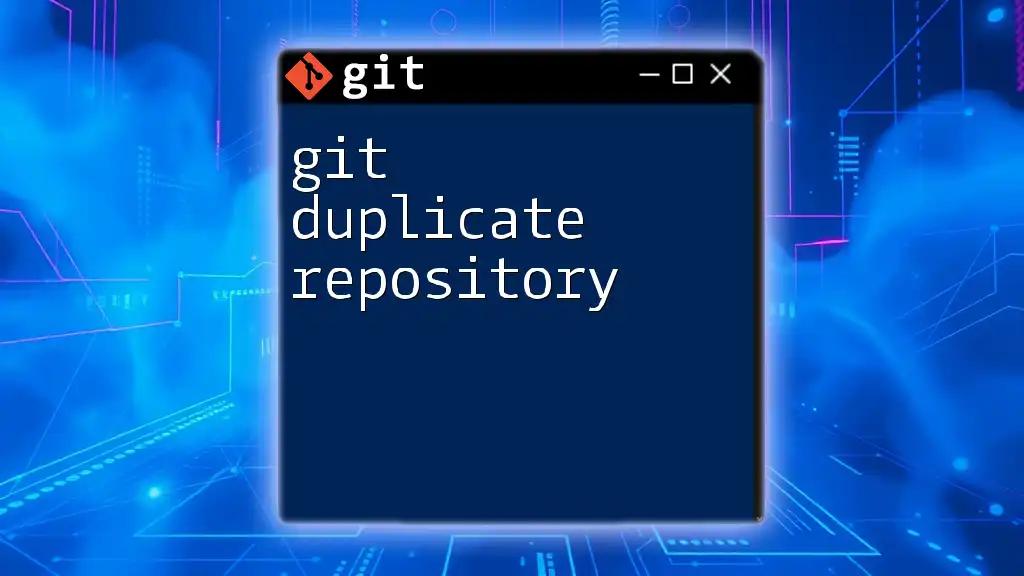
Troubleshooting Common Issues
Authentication Errors
If you encounter authentication errors, review the following tips:
- Ensure that you are using the correct username and personal access token.
- If using HTTPS, check if your personal access token has the appropriate scopes enabled.
- For SSH, make sure your SSH public key is added to your account and that the SSH agent is running.
Repository Not Found
Receiving a "Repository not found" error can be frustrating. Here’s what to check:
- Double-check the clone URL for typos or incorrect repository names.
- Ensure your access rights to the repository are intact and that it is indeed private.
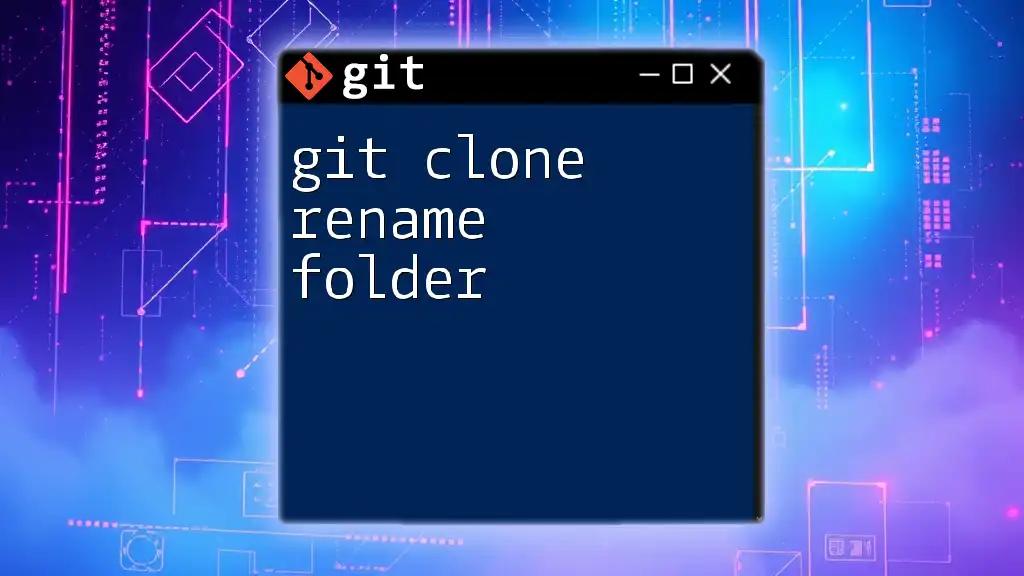
Best Practices for Working with Private Repositories
Keep Your Credentials Secure
It’s crucial to maintain the security of your credentials, especially when working with private repositories. Avoid hardcoding your credentials into scripts. Instead, use environment variables or Git credential helpers that store your credentials securely.
Regularly Update Your Local Copy
After cloning your repository, remember to keep your local copy up to date by regularly pulling in changes from the remote repository. You can do this with:
git pull origin main
Replacing `main` with the relevant branch name if necessary.
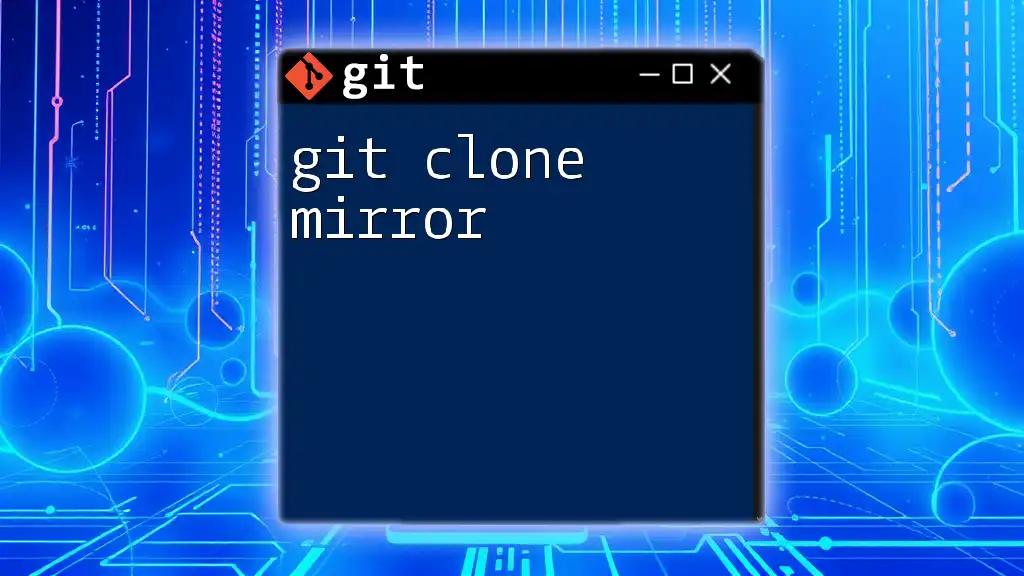
Conclusion
In this guide, we covered the essentials of using the `git clone` command for private repositories, including how to authenticate using both HTTPS and SSH methods. By following these steps, you’ll be well-equipped to clone private repositories securely and effectively.
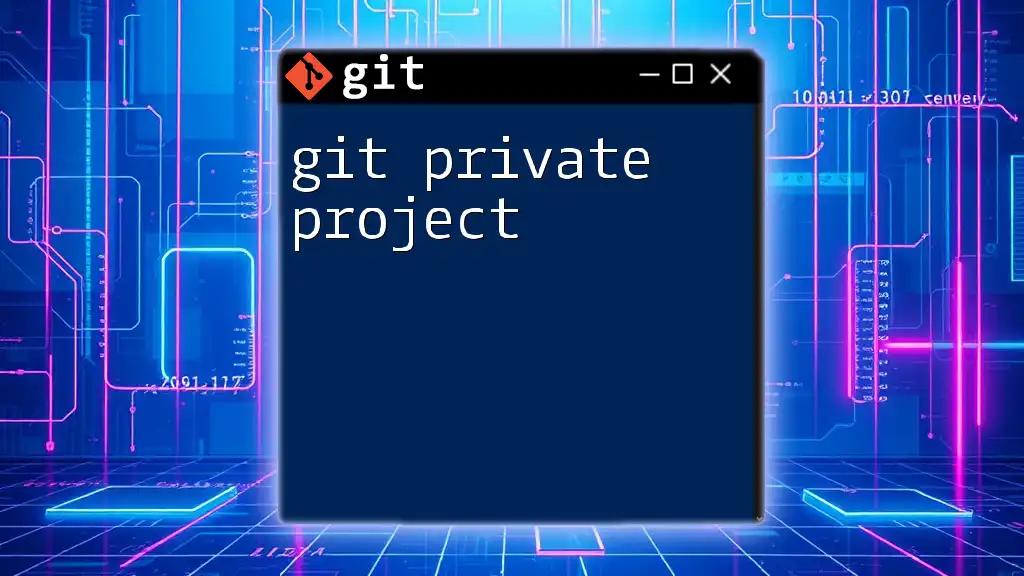
Additional Resources
For further reading and in-depth exploration of Git, consider reviewing the official Git documentation, as well as reputable Git tutorials and online courses. Consistently improving your Git knowledge will benefit your development process immensely.
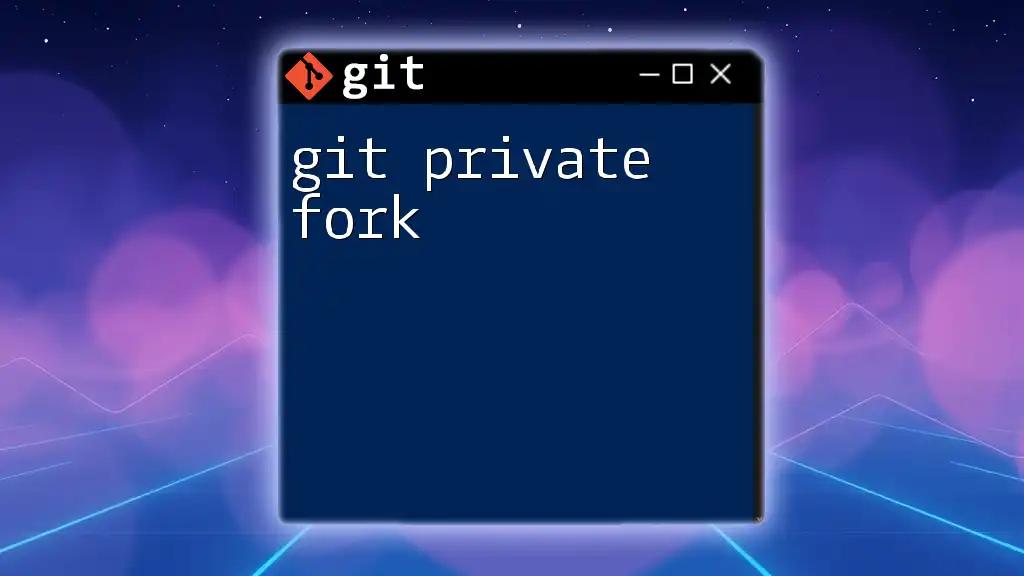
Frequently Asked Questions (FAQs)
Can I clone a private repository without access?
No, you cannot clone a private repository without the appropriate access rights. Always ensure that you are granted permission from the repository owner.
What if I forget my personal access token?
If you forget your personal access token, you will need to create a new one. Navigate to your Git service account settings to generate a new token.
How do I switch from HTTPS to SSH or vice versa?
To switch from HTTPS to SSH or vice versa, you can use the `git remote set-url` command. For example, to switch to SSH, run:
git remote set-url origin git@github.com:username/repo.git
Ensure that you have the necessary authentication method set up before executing the change.