Git is a distributed version control system that helps developers track changes in their code and collaborate efficiently, using commands like `git clone` to copy a repository.
git clone https://github.com/username/repository.git
What is Git?
Git is a distributed version control system designed to handle projects of any size with speed and efficiency. It allows multiple developers to work collaboratively without interfering with each other’s changes. The core aim of Git is to preserve the history of all modifications made to files in a project, enabling developers to revert to previous versions if needed and facilitating collaboration through branching and merging.
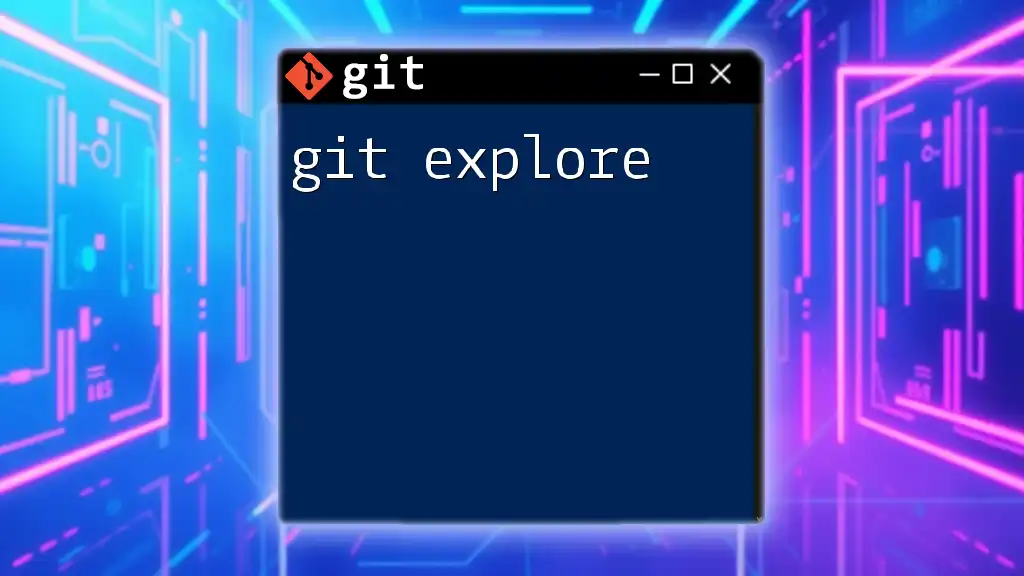
Why Use Git?
Using Git provides numerous advantages for both teams and individual developers. Some of the key benefits include:
- Collaboration: Enables multiple developers to contribute to the same project concurrently without conflicts.
- Version History: Maintains a comprehensive log of every change made, making it easy to track the evolution of a project.
- Branching and Merging: Facilitates experimentation in isolated branches before integrating changes back into the main project.
- Backup and Recovery: Offers a safety net by preserving older versions of files, which can be restored if necessary.
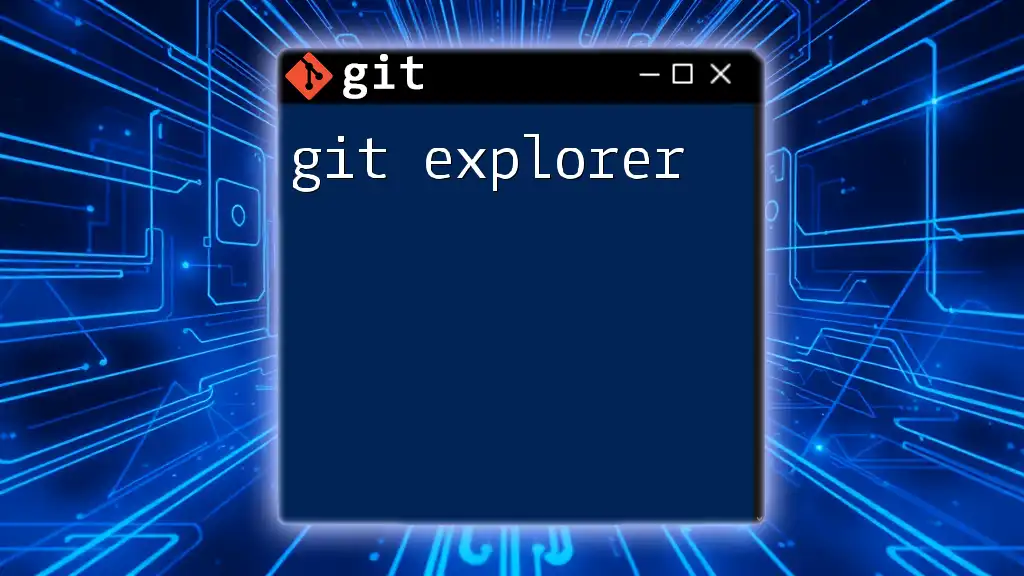
Understanding Version Control
What is Version Control?
Version control is a system that records changes to files over time so that you can recall specific versions later. This is essential in software development, where many changes can be made simultaneously.
Types of Version Control Systems
Version control systems fall into two main categories:
-
Centralized Version Control Systems (CVCS): These systems have a single central repository that developers commit their changes to. Examples include Subversion (SVN) and CVS.
-
Distributed Version Control Systems (DVCS): Unlike CVCS, DVCS allows each developer to have their own local copy of the entire repository, enabling them to work offline and commit their changes whenever they like. Git is one such system, making it highly flexible for collaborative work.
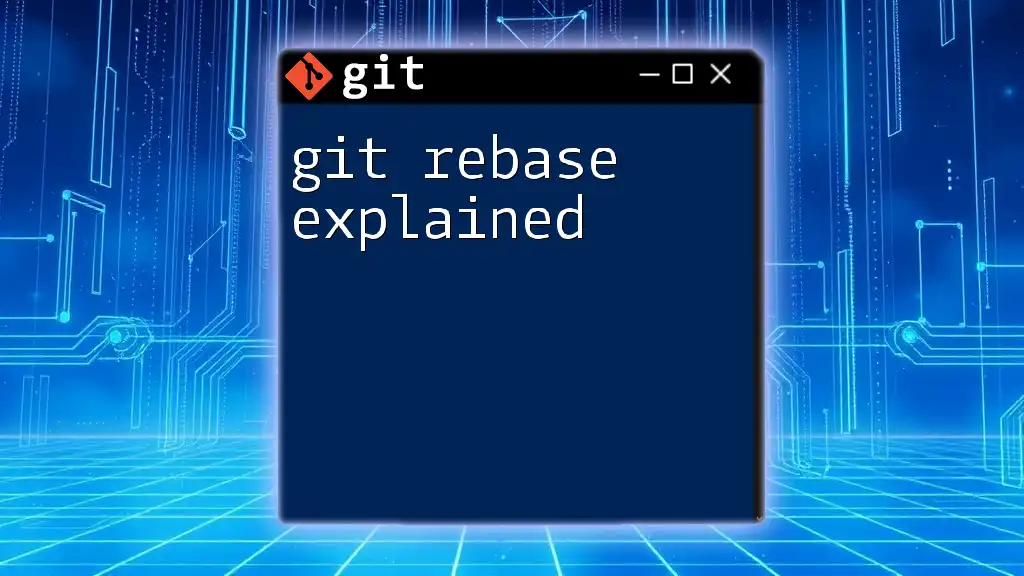
Getting Started with Git
Installing Git
To start using Git, you first need to install it on your machine. Here’s how to install Git on various operating systems:
- Windows: Download the installer from the official Git website and follow the on-screen instructions.
- macOS: Use Homebrew by running `brew install git` in the terminal.
- Linux: Install Git using your package manager. For example, for Ubuntu, run `sudo apt-get install git`.
Configuring Git
After installation, you need to configure Git for your personal use. This includes setting your username and email, which will appear in your commit messages:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
These configurations ensure that your contributions are correctly attributed in the project history.
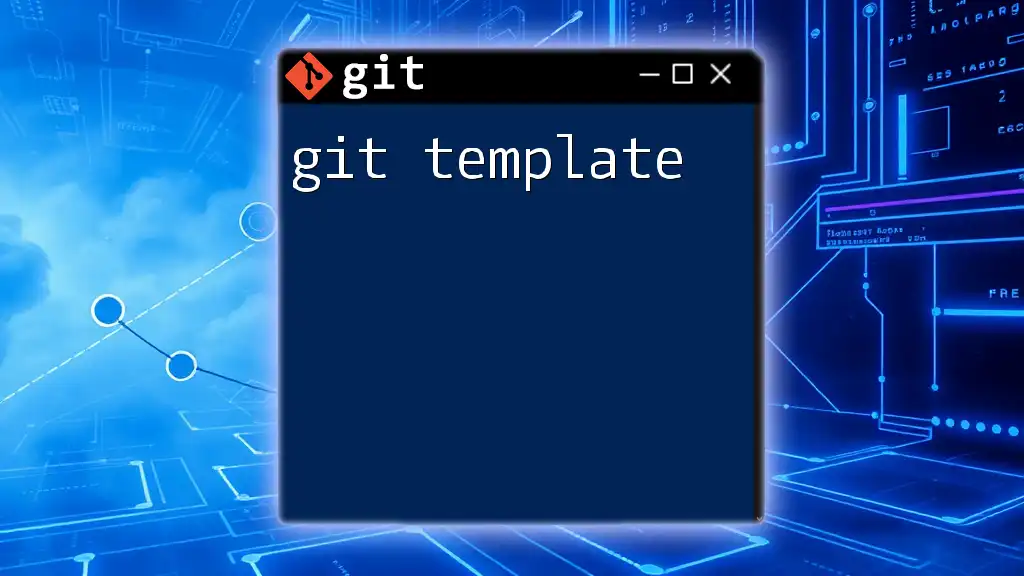
Core Git Concepts
Repositories
A repository (often referred to as a "repo") is a storage space for your project. There are two types of repositories:
- Local Repository: The copy of the repository that resides on your machine.
- Remote Repository: The central repository hosted on platforms such as GitHub, GitLab, or Bitbucket.
Commits
A commit is a snapshot of your project at a specific point. Each commit has a unique ID and contains metadata like the author, date, and a commit message describing the changes made. A well-written commit message helps others understand the purpose of changes. Here's an example of how to make a commit:
git commit -m "Fix bug in user login"
Branches
Branches are powerful tools in Git that allow you to work on different features or fixes independently from the main codebase. By creating a branch, you can isolate development efforts without affecting the stable version of your project. To create and switch to a new branch, you can use:
git branch feature-branch
git checkout feature-branch
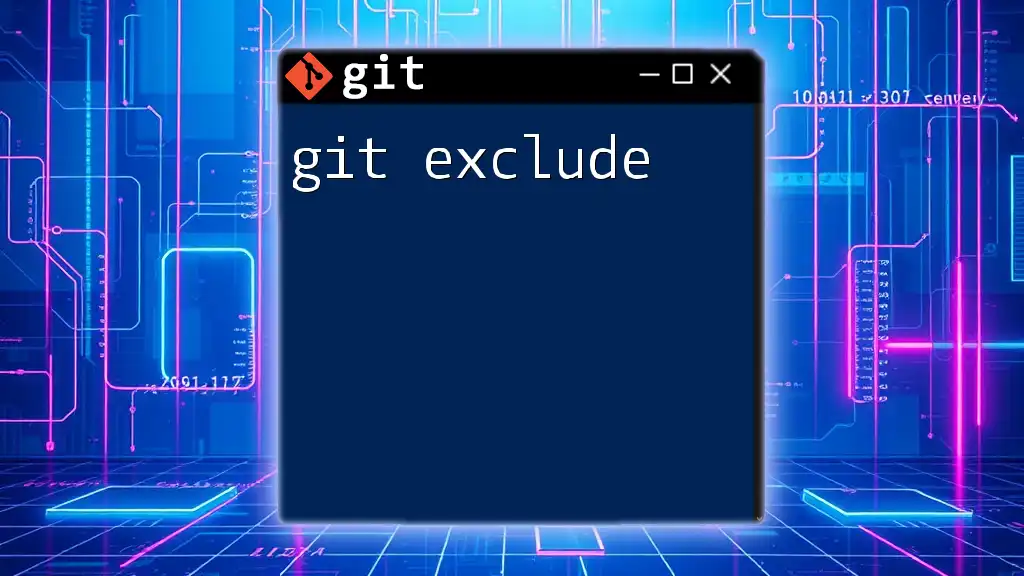
Essential Git Commands
Basic Commands Overview
To effectively manage your projects with Git, getting familiar with its basic commands is crucial:
- Initialize a new repository: `git init`
- Clone an existing repository: `git clone <repository-url>`
- Stage changes: `git add <filename>`
- Commit those changes: `git commit -m "your message"`
Viewing Changes
To monitor the status of your repository and understand what has changed, use:
- `git status`: Displays staged and unstaged changes.
- `git diff`: Shows changes between the working directory and the staging area.
Working with Remote Repositories
To collaborate effectively, it’s essential to interact with remote repositories. You can add a remote repository and push your changes with:
git remote add origin <repository-url>
git push origin feature-branch
If you need to fetch changes made by others, use:
git pull
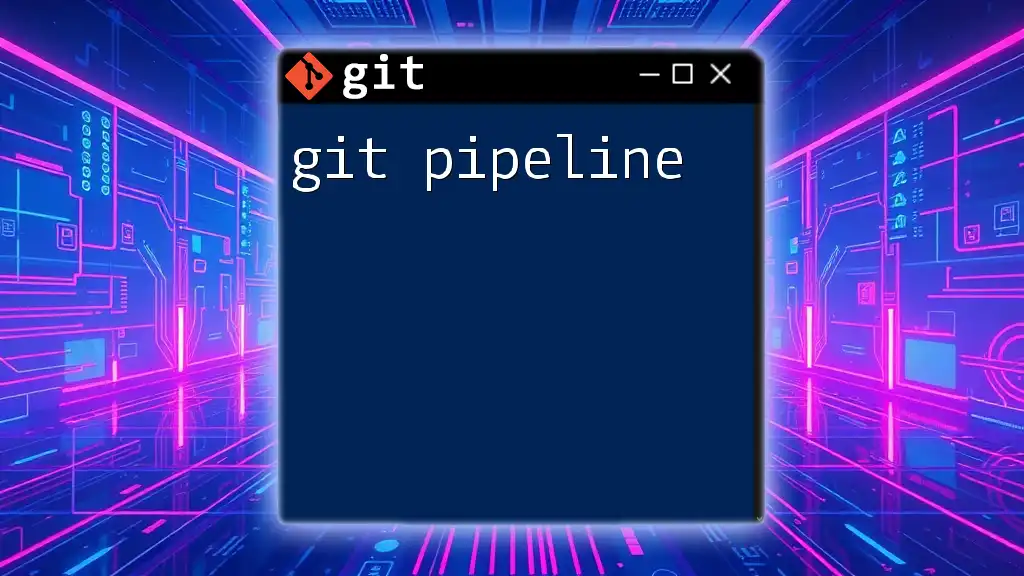
Collaboration with Git
Merging Branches
When your feature is complete and tested, you can integrate the changes back into the main branch through merging. This combines the histories of both branches. Consider the following example for merging:
git merge feature-branch
If there are conflicting changes, Git will notify you, and you'll have to resolve these conflicts before completing the merge.
Pull Requests
A pull request is a way to propose changes to a project and request that someone reviews and pulls your changes into their branch. This practice is fundamental in open-source and collaborative projects, promoting code quality and shared knowledge. When you submit a pull request, often integrated into platforms like GitHub, this initiates discussions around your proposed changes.
Git Workflows
Various Git workflows help teams standardize their development practices. Popular options include:
- Feature Branch Workflow: Each new feature is developed in its own branch, which is then merged into the main branch.
- Git Flow: A more structured approach to branching, allowing for planned releases and hotfixes.
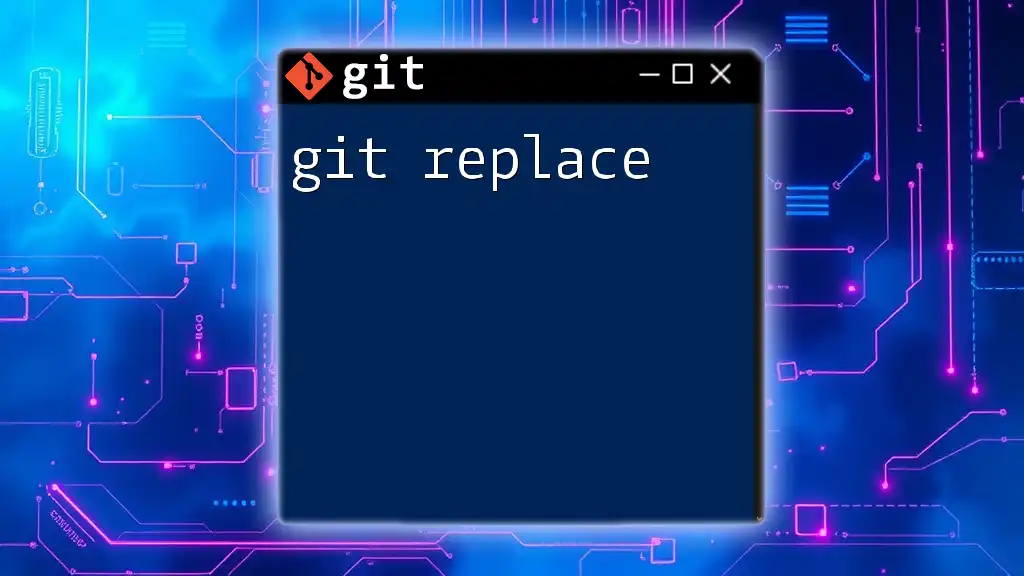
Advanced Git Features
Stashing Changes
While working on a project, you may need to switch branches but want to save your uncommitted changes temporarily. Stashing allows you to do this smoothly:
git stash
git stash apply
By stashing, you can clean your working directory without committing unready changes.
Rebasing
Rebasing is an alternative to merging that allows you to incorporate changes from one branch into another. It rewrites the commit history but can help create a cleaner project history. For example, to rebase your feature branch onto the main branch, you could use:
git rebase main
Undoing Changes
Mistakes are part of any workflow. Git offers several commands for undoing changes, using the reset and checkout commands effectively. For instance, if you want to undo the last commit, use:
git reset --hard HEAD~1
However, this command should be used with caution, as it will remove any changes made in that commit.
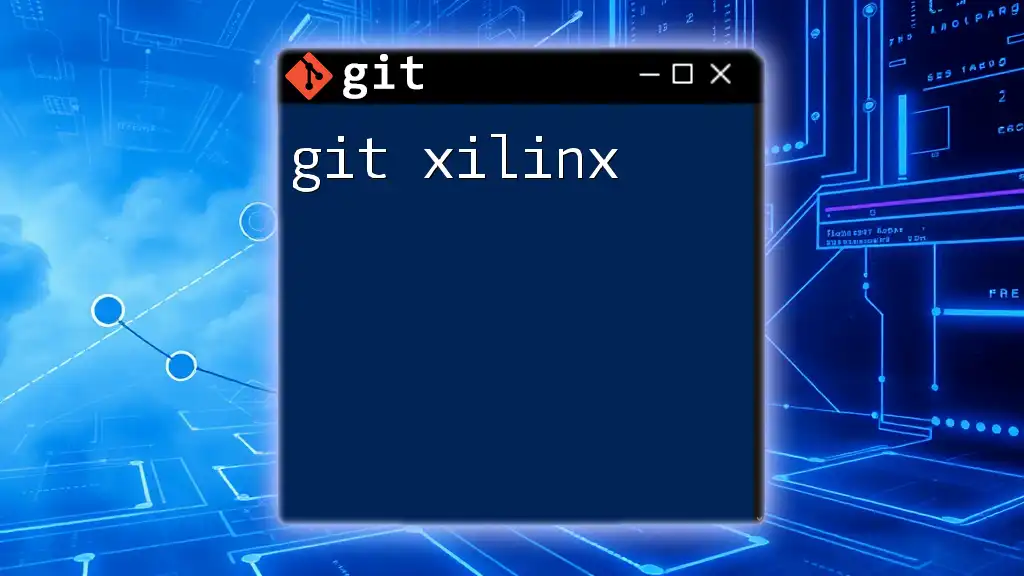
Best Practices for Using Git
Writing Good Commit Messages
Good commit messages have a clear and concise description of the changes made. Use the imperative tense, such as "Fix bug" rather than "Fixed bug," and keep the message under 72 characters if possible.
Keeping a Clean Repository
Regularly review your branches and commits to avoid accumulating obsolete features or fixes. Clean repositories are easier to manage and collaborate on.
Collaborative Best Practices
Encourage frequent pushes and pull requests with team members to keep everyone up-to-date. Communication, especially regarding changes and new features, fosters a productive working environment.
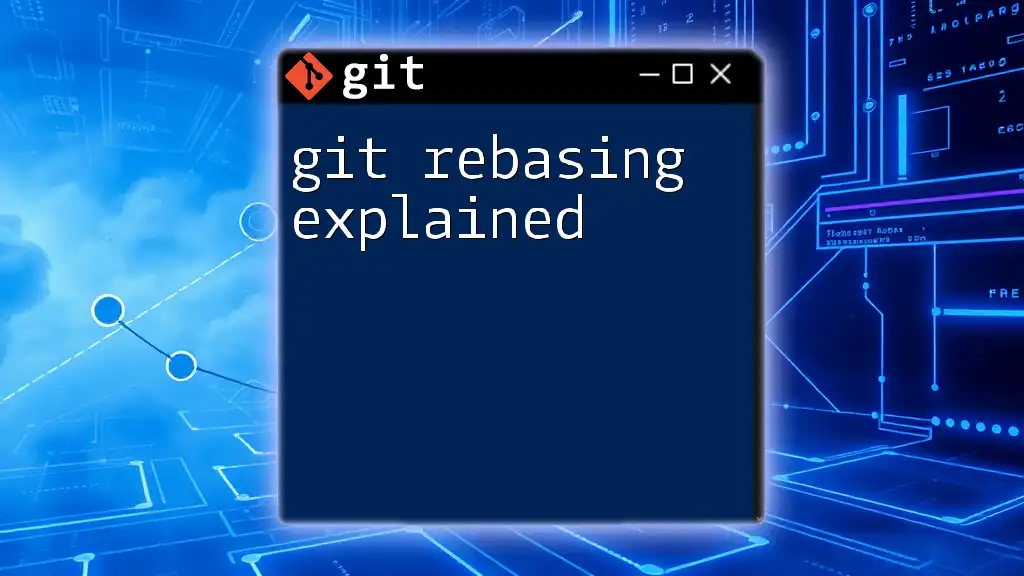
Conclusion
Navigating Git and understanding its features will significantly enhance your development workflow, whether working individually or collaboratively. By mastering the essential commands and concepts, you can leverage Git to its fullest potential, ensuring your projects remain organized and efficient.
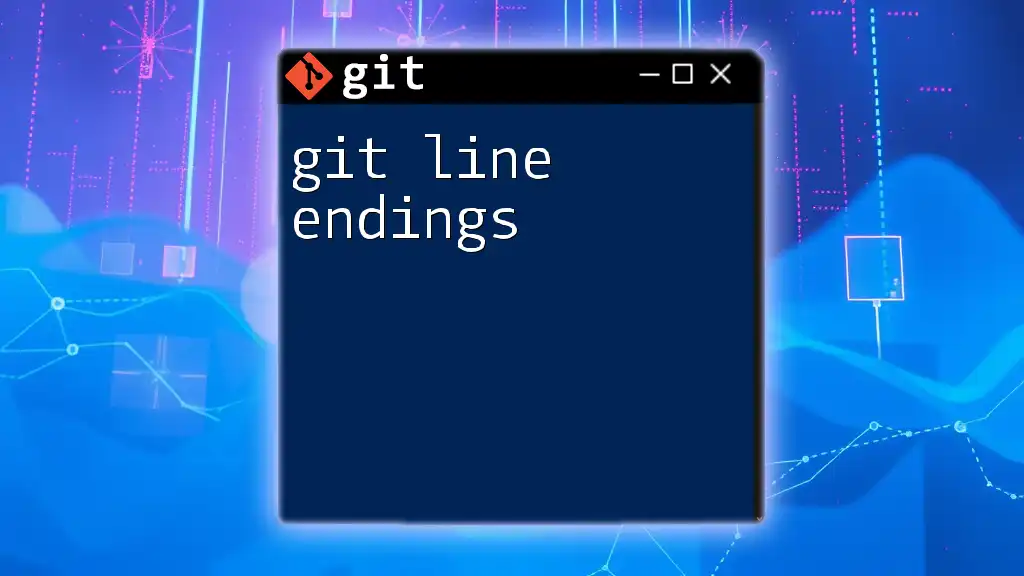
Call to Action
Join our workshop to explore Git in-depth and master these commands and techniques that can transform your coding experience. If you have any feedback or suggestions, please share your thoughts or questions in the comments section. Your insights matter to us!