The `git replace` command allows you to substitute one object in the Git database with another, often used for replacing old commits or tags with new ones for easier management.
git replace <object> <replacement>
What is `git replace`?
`git replace` is a powerful command used in Git to create temporary replacements for Git objects, such as commits or blobs. This command allows developers to modify their Git history or the content of files without altering the underlying repository. By using `git replace`, you can substitute one object with another, enabling scenarios like fixing mistakes in a commit or updating file contents without a complete rewrite of history.
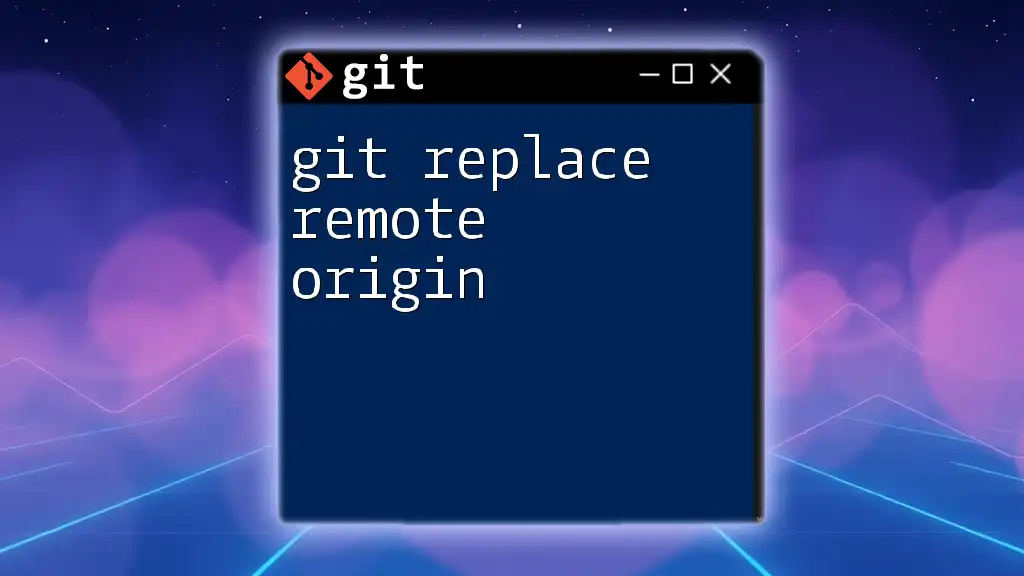
Why Use `git replace`?
The utility of `git replace` lies in its ability to simplify some complex workflows. Unlike commands like `git rebase` or `git cherry-pick`, which modify the commit history and affect the overall structure of the repository, `git replace` maintains a clean history. It achieves this by creating a mapping of an old object to a new one without actually changing the existing object. Therefore, `git replace` is particularly useful in cases where you want to keep track of changes while avoiding the need for extensive rewrites or potential errors associated with those processes.
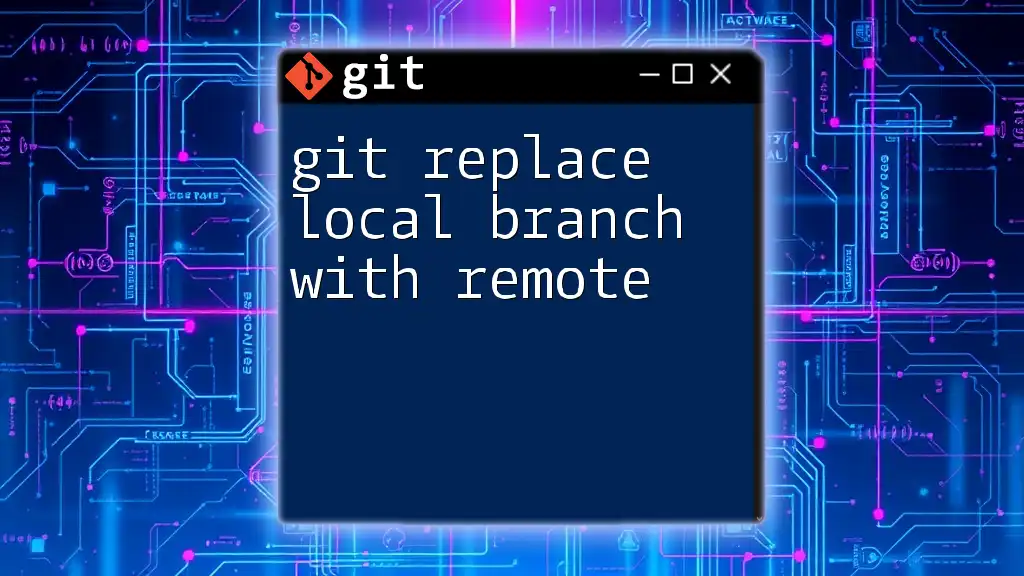
Understanding Git Objects
What are Git Objects?
In Git, everything is represented as an object. There are primarily three types of objects:
- Commits: Represent snapshots of the entire repository at a certain point in time.
- Trees: Directories that contain references to blobs or other trees.
- Blobs: File contents stored as Git objects.
Each of these objects is identified by a unique SHA-1 hash, which plays a crucial role in identifying and referencing them.
How `git replace` Works with Objects
`git replace` operates at the object level. When you issue a replace command, it effectively tells Git to use a different object whenever the original object is referenced. This pointer-based system means old objects remain intact, and you can revert or delete replacements as needed.
For example, if you had a commit that introduced a bug, instead of rewriting the commit history, you could create a simple replacement using `git replace`, redirecting references to a corrected commit.
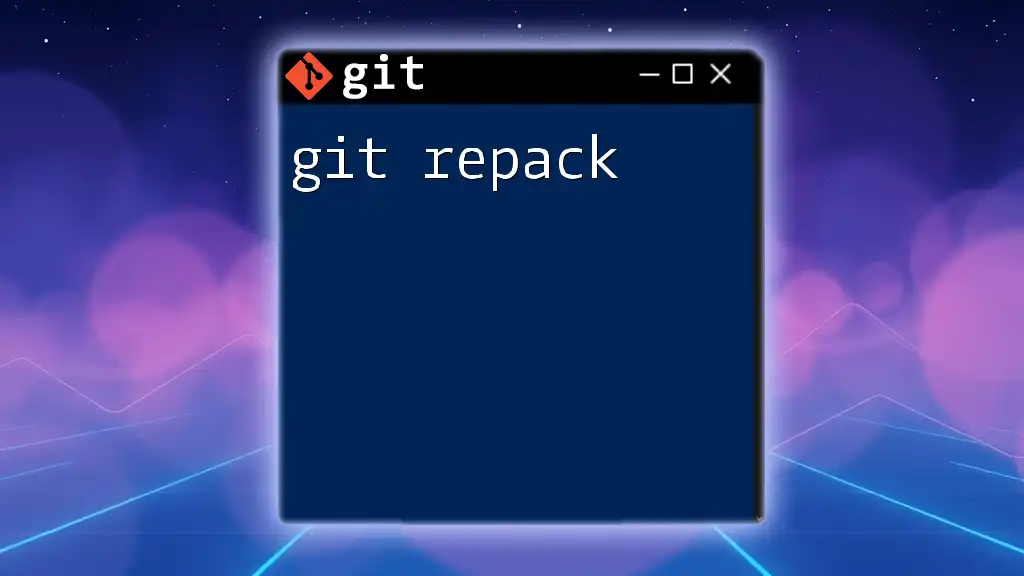
Basic Usage of `git replace`
Creating a Replacement
To create a replacement, you will use the following syntax:
git replace <old-commit> <new-commit>
For instance, if you want to replace an old commit `abc1234` with a new correction found in `def5678`, you’d run:
git replace abc1234 def5678
This command allows any references to the old commit `abc1234` to point to the new commit `def5678`. It’s important to understand that the old commit is still preserved in your repository; the replacement is non-destructive.
Viewing Replacements
To view existing replacements, you can use the following command:
git replace -l
This will list all current replacements in the repository, providing insight into how many and which objects are being temporarily redirected.
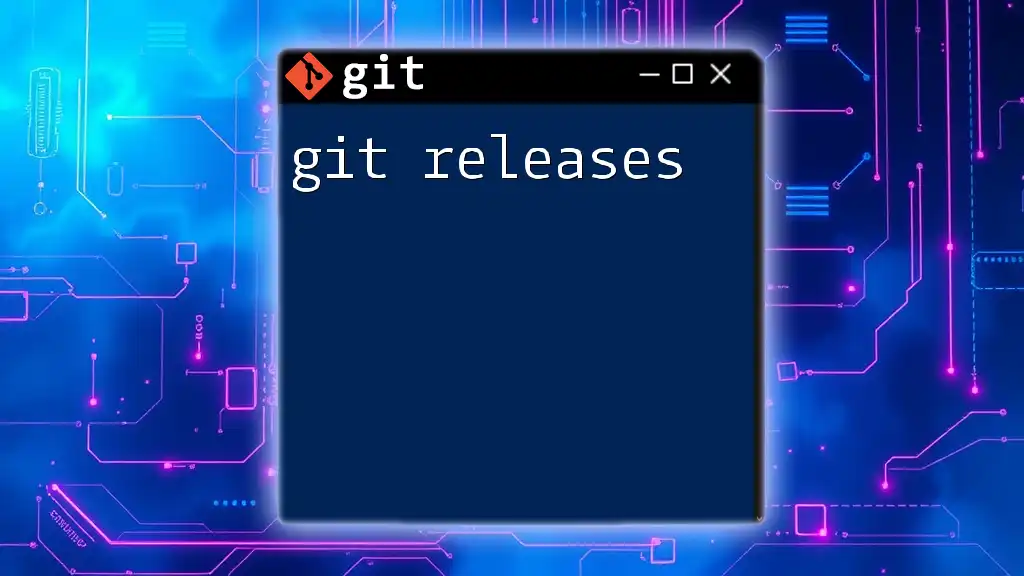
Advanced Options and Features
Deleting a Replacement
If at any point you decide to remove a replacement, the command structure is straightforward:
git replace -d <old-commit>
So for our earlier example, to delete the replacement for `abc1234`, you would execute:
git replace -d abc1234
This command will remove the replacement, reverting any references back to the original object.
Limitations of `git replace`
While `git replace` is a powerful tool, it does come with certain limitations. Replacements are local to your repository and may not be recognized by others unless they also use the same replacements. Additionally, it might not be suitable for complex scenarios or when multiple contributors are making extensive changes, as it could lead to confusion or miscommunications.
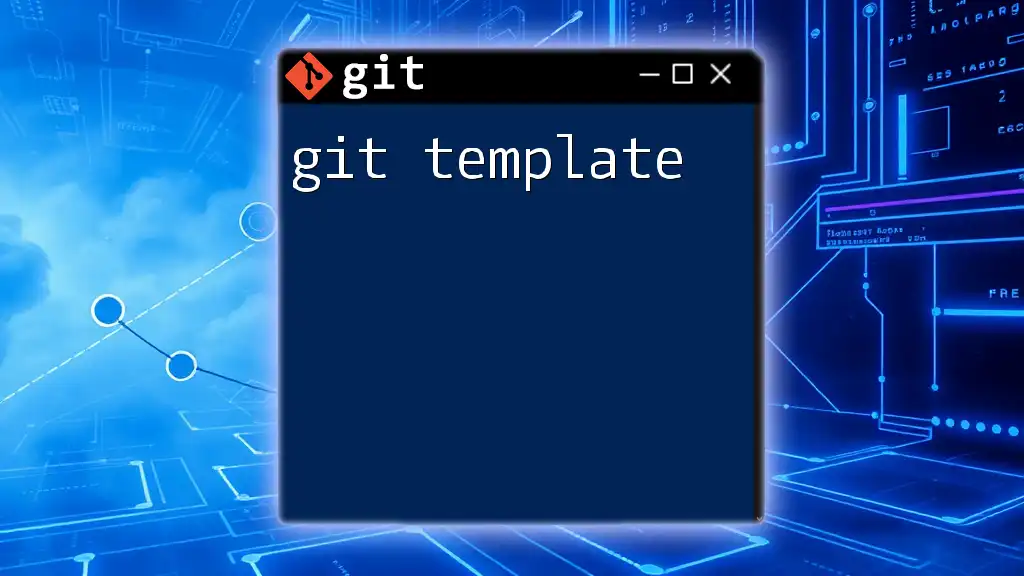
Best Practices for Using `git replace`
When to Use `git replace`
Utilizing `git replace` is most beneficial in scenarios like:
- Temporarily overriding a faulty commit: Redirecting to a corrected version without losing historical context.
- Refreshing file contents: Updating a blob with new content while keeping the commit history intact.
Common Pitfalls to Avoid
When using `git replace`, it is vital to be mindful of:
- Confusing team members: Since replacements are local, make sure your team is aware of any changes you’ve made and that they do not have local replacements pointing to different objects.
- Not overwriting essential objects: Be careful when choosing the objects to replace; overwriting important states could lead to loss of information.
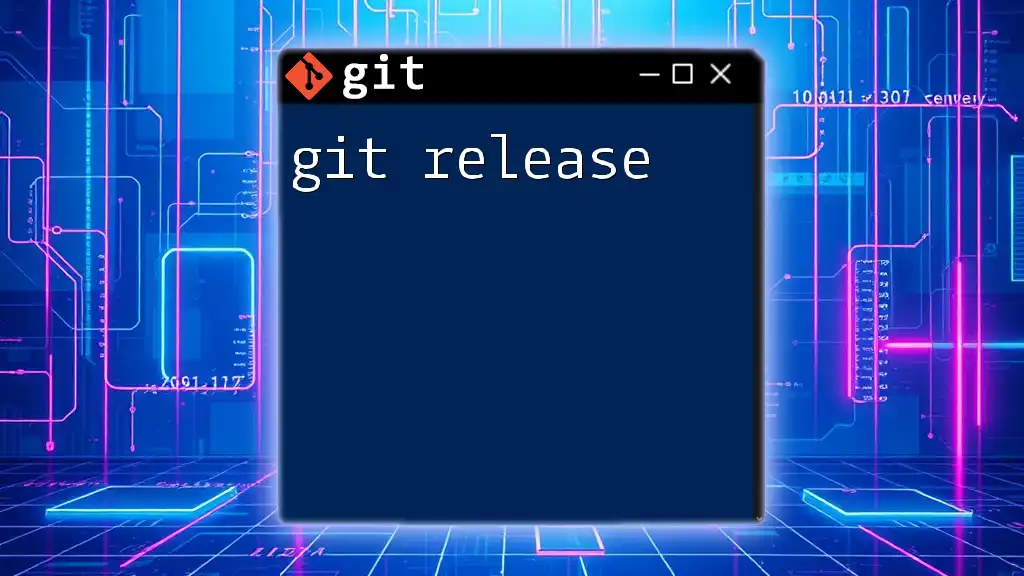
Examples and Real-World Applications
Example 1: Replacing a Commit for Feature Rollback
Let’s say you realized that a feature introduced in commit `abc1234` broke certain functionalities. Instead of performing a rebase, you could create a temporary replacement:
git replace abc1234 def5678
Now, anywhere the repository references `abc1234`, it will use the changes from `def5678` instead. This approach keeps your main commit history clean while allowing you to address the issue quickly.
Example 2: Replacing a Blob to Update a File
Imagine you completed a project and later discovered a typo in one of the files that was committed as a blob. You can replace this blob with an updated version. First, you would commit your corrected file to create a new blob, then run:
git replace <old-blob-hash> <new-blob-hash>
This change will redirect all references from the old blob to the new one, effectively updating the content as needed.
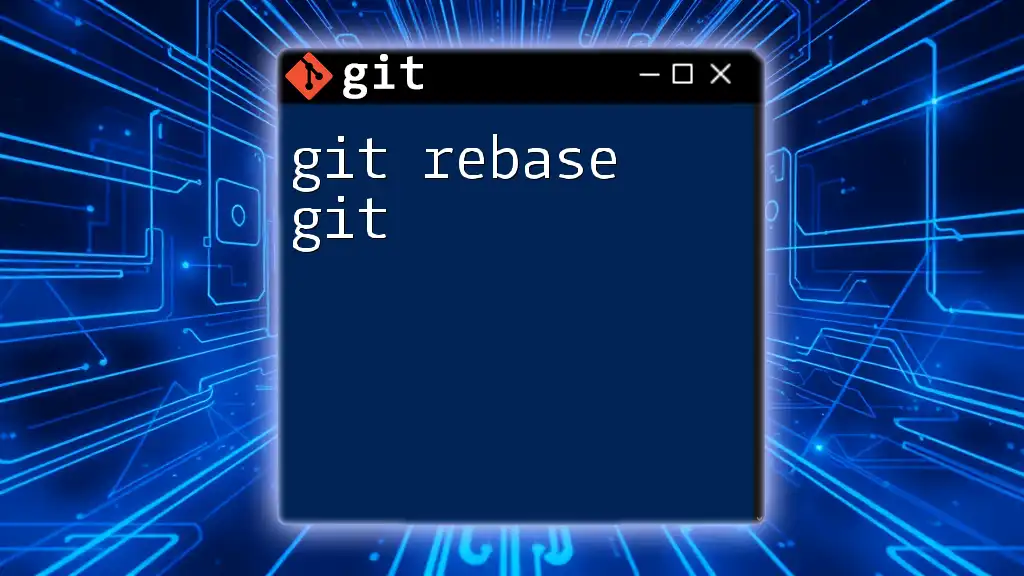
Conclusion
In summary, `git replace` is a powerful command that offers a unique way of handling object references within a Git repository. Its ability to create temporary replacements without altering the core history makes it an effective tool for developers looking to manage errors or update file contents seamlessly.
As you navigate through your Git journey, don’t hesitate to experiment with `git replace` and see how it can simplify your workflows and enhance your version control practices.
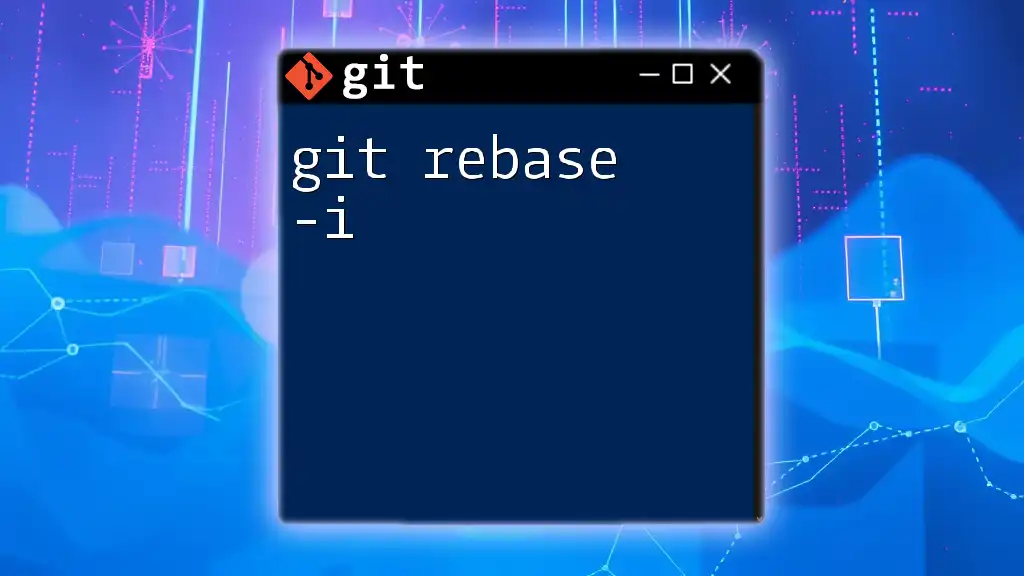
Additional Resources
For further deep diving into `git replace` and similar commands, explore the official Git documentation and seek out community forums for valuable insights and experiences from fellow developers. Engaging with resources and peer discussions will strengthen your understanding and application of this command and others like it in your daily development tasks.