A Git template is a pre-defined directory structure that can be used to initialize new repositories with custom files or configurations, making it easier to maintain consistency across multiple projects.
git init --template=/path/to/your/template
What is a Git Template?
Git templates serve as pre-configured setups that streamline and standardize the initialization of new Git repositories. Essentially, a Git template encompasses scripts, configurations, and other elements that automate common tasks, providing a consistent starting point for all new repositories created on your system.
The primary benefit of using Git templates lies in their ability to save time and ensure uniformity across different projects. For teams, this means less variation in configuration and quicker onboarding for new project contributors.
When to Use Git Templates
Think of Git templates as a tool to enhance your workflow. They are particularly useful in situations where:
- New repositories are frequently created.
- Consistency is critical across multiple projects, such as in large teams.
- Specific hooks or configurations are needed in every new repository.
If you find yourself repeating the same setup steps for each new project, incorporating Git templates can significantly ease your development process.
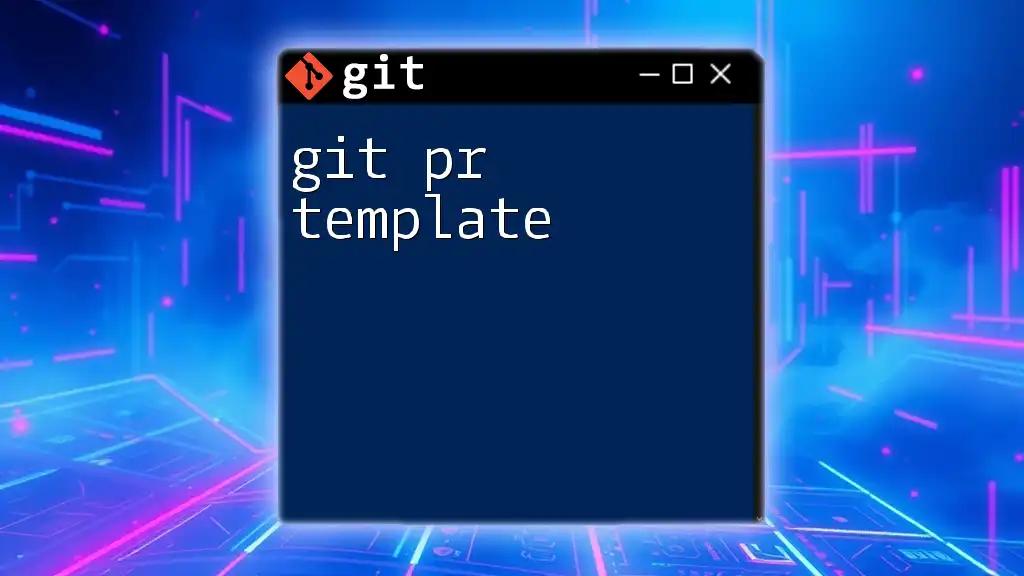
Setting Up Git Templates
Global Git Template Configuration
Configuring a global Git template allows you to set up a standard template that applies to all your new repositories. To create a global Git template, you’ll need to specify a directory containing your template files. You can accomplish this with the following command:
git config --global init.templateDir '/path/to/your/template/directory'
Ensure that the specified directory contains the appropriate structure and files, such as hooks and configuration options that you want to apply globally.
Project-specific Templates
If you desire a more tailored approach, setting up project-specific templates is your answer. You can opt for unique configurations for individual projects. This can be configured by pointing to a specific template directory for your project:
git config init.templateDir '/path/to/project/template'
This flexibility allows you to maintain diverse setups without interfering with your global configurations.
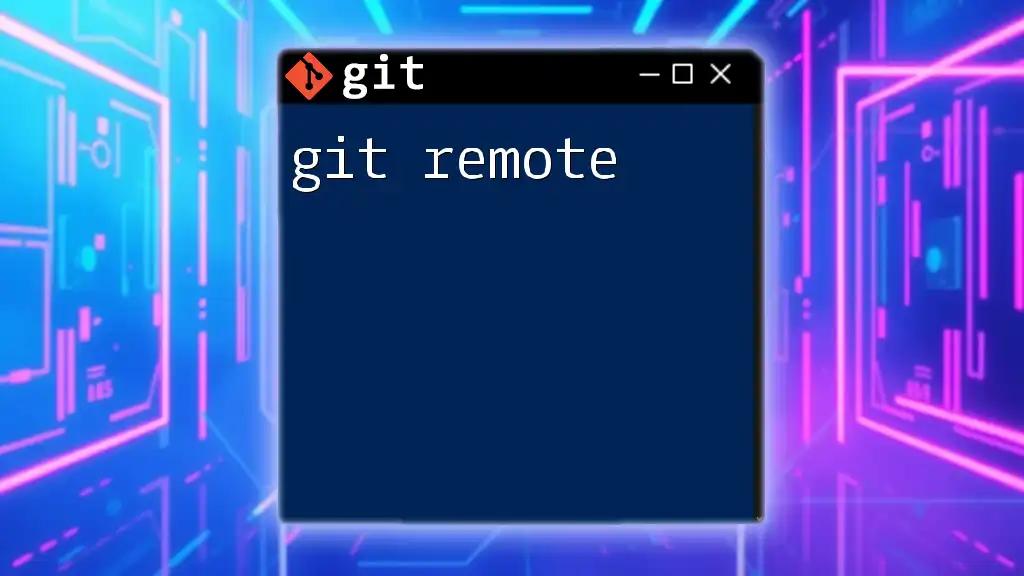
Creating a Git Template
Structure of a Git Template
A Git template consists of a specific file structure that allows Git to recognize and apply your custom setup. Key components to include in your Git template are:
- Hooks: Scripts that run automatically on certain events (e.g., commit, push).
- Branches: Pre-defined branches that can be set up right from the start.
- Configuration files: Custom `.git/config` settings to tailor repository behavior immediately.
Example: Simple Git Template
Let’s walk through how to create a simple Git template. Consider a directory with the following structure:
my_template/
│
├── hooks/
│ └── post-commit
│
└── config
The `post-commit` hook could take the form of the following script, which logs a message after each commit:
#!/bin/sh
echo "This commit was created by a template!" >> template-commit-log.txt
Make sure to give the script execute permissions with `chmod +x hooks/post-commit`.
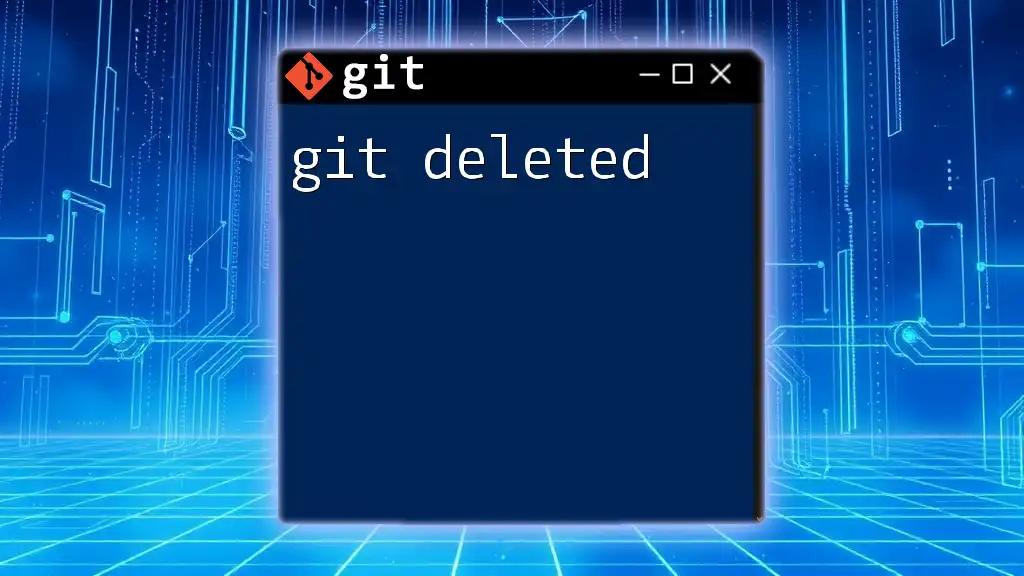
Using Git Templates
Initializing a Repository with a Template
Once you have your template ready, initializing a new repository with it is straightforward. Use the command:
git init --template='/path/to/template'
This command creates a new Git repository by populating it with the contents of your specified template directory. All hooks and configurations defined in the template will automatically become part of the new repository.
Verifying Template Application
After initializing a repository using your Git template, you can verify that the template was applied correctly. This involves checking the presence of the hooks and configurations. For instance, you can navigate to the `.git/hooks/` directory to ensure your custom hooks are there.
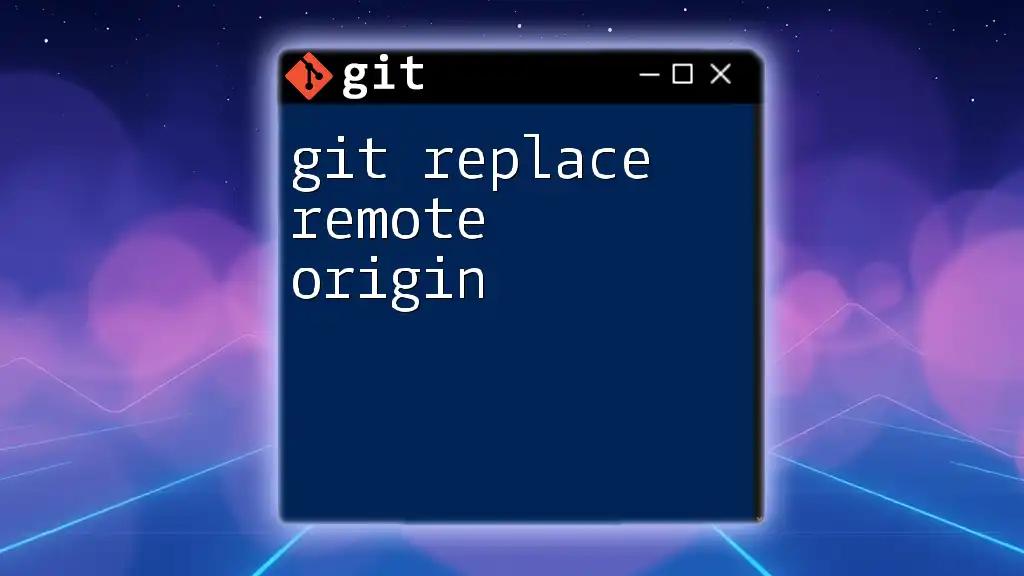
Modifying an Existing Git Template
Updating the Template
It’s possible that over time, your projects may evolve, necessitating a change in your templates. Updating the contents of a Git template is simple, but it's essential to maintain a consistent approach to avoid disruption. When making changes, always consider how existing repositories may be affected.
Example: Adding New Hooks
Suppose you want to enhance your Git template by adding a new `pre-commit` hook. You could create another script in the hooks directory:
#!/bin/sh
echo "New pre-commit hook!" >> template-pre-commit-log.txt
Like the previous example, ensure that you change the permissions to make it executable.
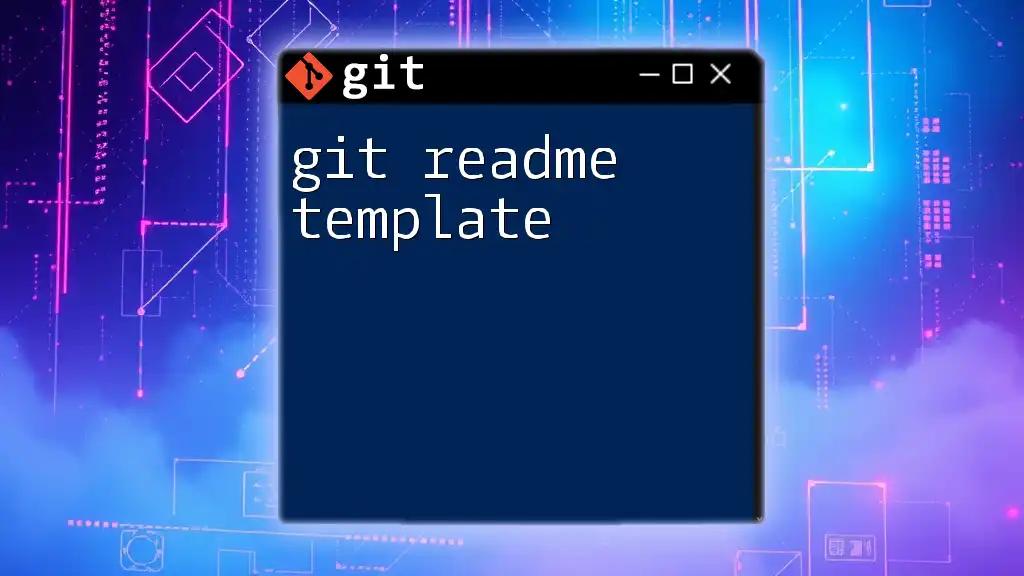
Advanced Git Template Features
Template for Multiple Repositories
A Git template can be leveraged across multiple repositories. By maintaining a single template and applying it to various projects, you ensure consistent standards and practices. This is especially beneficial for organizations managing numerous codebases or teams.
Customizing Configurations
Another advanced feature is the ability to customize configuration files within your Git template. You can edit the `config` file present in your template to include various settings such as:
- User information (name and email).
- Default branch names.
- Aliases for frequently used commands.
These customizations ensure that every new repository inherits your preferred settings from the start.
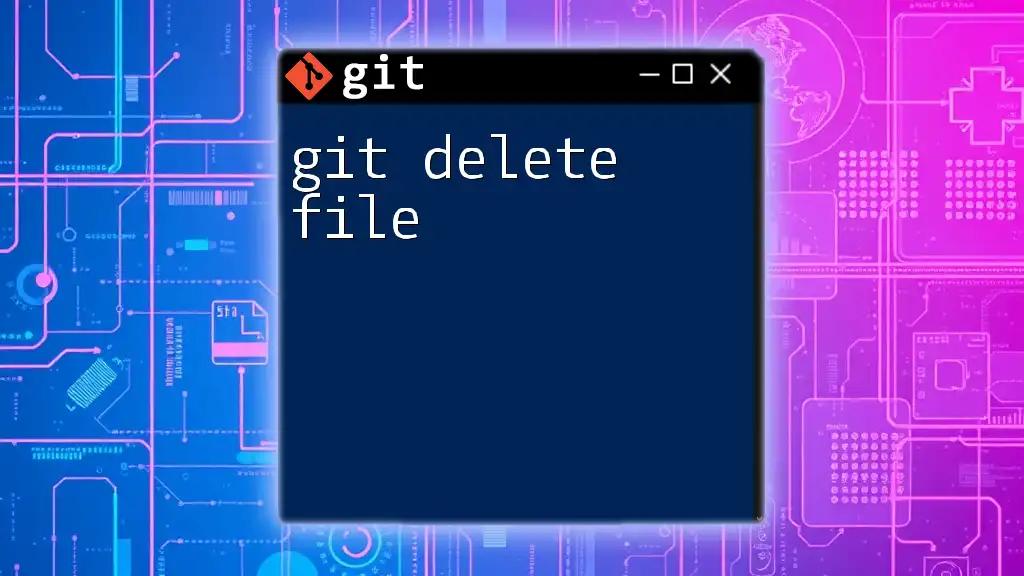
Common Issues and Troubleshooting
FAQ on Git Templates
-
What happens if a template file is missing? If your configured template files are missing, Git will proceed with the default settings, meaning any intended customizations won’t be applied.
-
Can I share my Git template with others? Yes, Git templates can easily be shared by distributing the template directory, making it convenient for teams to adopt the same configurations.
-
Can I remove or edit hooks after initializing a repository? Absolutely! You can modify or remove hooks from the `.git/hooks` directory of any repository at any time.
Troubleshooting Template Issues
If you encounter issues while applying a Git template, consider the following troubleshooting steps:
- Check Directory Paths: Ensure that the specified paths to your template directories are correct.
- Review Hook Scripts: Ensure your scripts have the correct executable permissions.
- Inspect Configurations: Use `git config --list` to check current configurations and verify if they reflect your template settings.
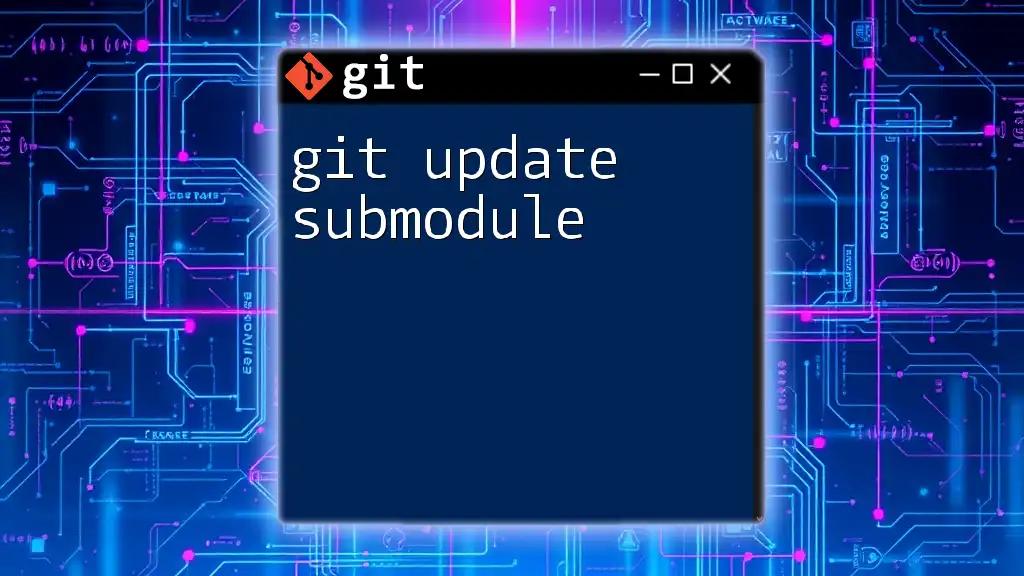
Conclusion
Utilizing a Git template can dramatically improve the efficiency and consistency of your development workflow. Whether you're working solo or in a team, Git templates empower you to set up new repositories quickly and uniformly. Exploring and creating your own templates can further optimize your processes, making it easier to maintain high standards across projects.
Take the initiative to experiment with Git templates and elevate your skills—it's a worthwhile investment for any developer. For deeper learning on Git commands and practices, connect with comprehensive courses that can enhance your command-line expertise.
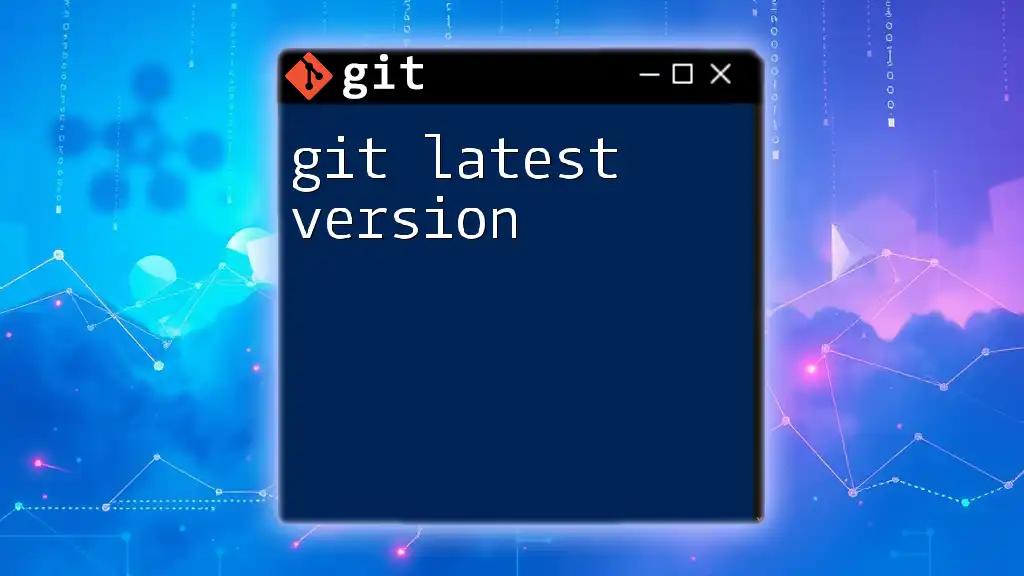
Additional Resources
For further exploration, refer to the official Git documentation, which provides extensive insights into advanced configurations and best practices. Additionally, consider investing in books or online courses dedicated to mastering Git for professional development.