Git forking is the process of creating a personal copy of someone else's repository on GitHub, allowing you to freely experiment with changes without affecting the original project.
Here’s an example command to fork a repository:
git clone https://github.com/username/repository.git
What is a Fork?
A fork in the context of Git is a personal copy of someone else's repository. It allows you to experiment freely with changes without affecting the original project. Forks are crucial in collaborative development, especially in open-source projects, enabling multiple contributors to work on a project simultaneously without interfering with the upstream project.
The primary difference between a fork and a clone lies in their purpose and how they operate. While cloning creates a direct copy of a repository to your local machine, forking creates a new repository under your account on platforms like GitHub, where you can make changes and easily propose those changes back to the original repository.
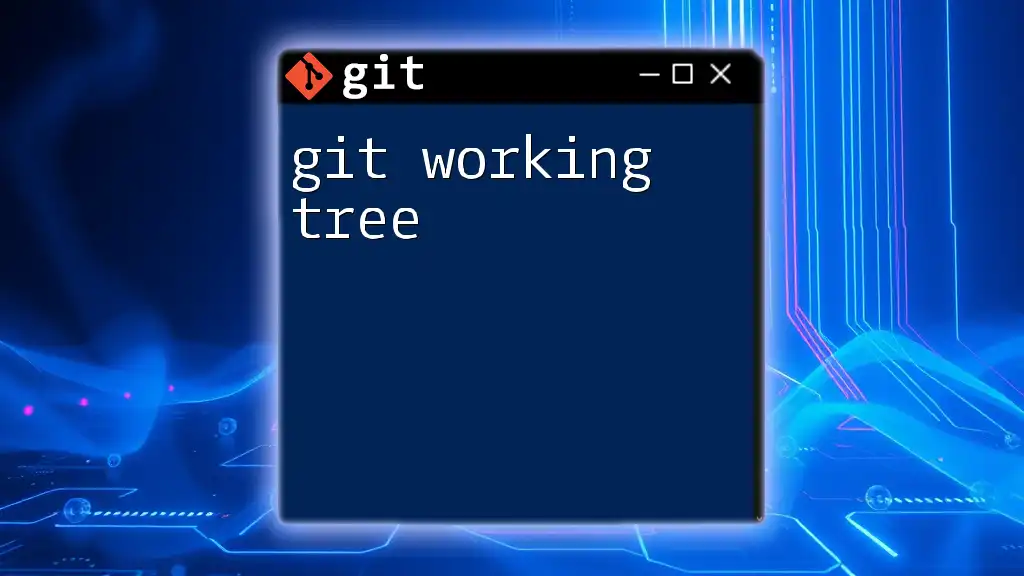
Why Fork a Repository?
Forking a repository serves several vital purposes:
-
Facilitating Contributions: When you fork a project, you can contribute back to the original repository by submitting your changes through a pull request. This is especially useful in open-source projects, where many contributors might be working on different features simultaneously.
-
Allowing Experimentation: Forking lets you try out new ideas or features without risking disruption to the main project. This is beneficial for testing changes or developing features in a sandbox environment.
Real-world scenarios demonstrate the power of forking. For example, projects like React and TensorFlow have hundreds of contributors; many of these contributions originated from forks. This structure fosters innovation while protecting the stability of the main codebase.
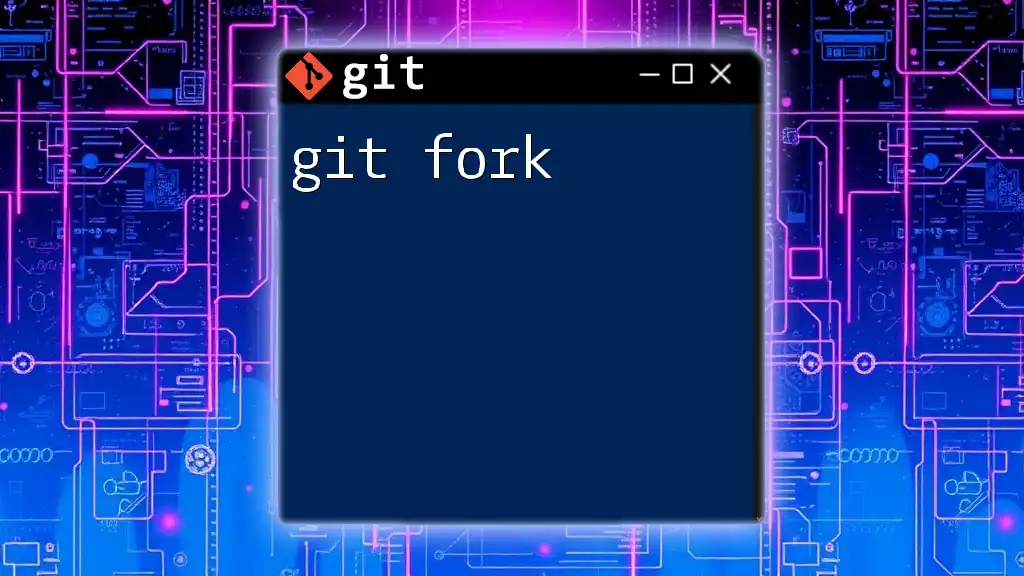
How to Fork a Repository on GitHub
Step-by-step Process
-
Navigate to the Repository: Go to the GitHub page of the repository you wish to fork.
-
Click the “Fork” Button: In the upper right corner, you will see a “Fork” button. Click it.
-
Choose Your Account: If you belong to multiple organizations or have several accounts, select where you want the forked repository to reside.
-
Access Your New Fork: After forking, GitHub will redirect you to your copy of the repository, which now belongs to your account.
Example: Forking a Repository
# No command needed, just navigate and click "Fork"
This simple action creates a copy of the original repository in your GitHub account, allowing you to make changes without impacting the original project.
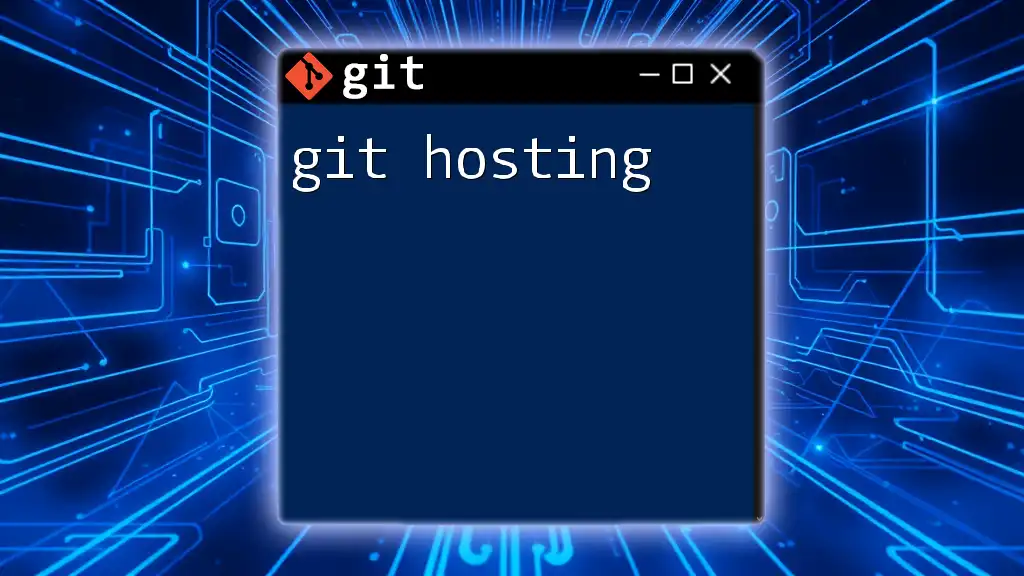
Working with Your Fork
Cloning Your Fork Locally
Once you’ve forked a repository, the next step is to clone it to your local machine. Cloning ensures you can work on your code without needing to be online or modifying anything on GitHub.
git clone https://github.com/YOUR_USERNAME/REPOSITORY_NAME.git
After cloning, navigate into your cloned directory and verify remote settings using:
cd REPOSITORY_NAME
git remote -v
Making Changes to Your Fork
When modifying your fork, it's best practice to create separate branches for each new feature or bug fix. This approach keeps your work organized and makes collaboration easier.
To create a new branch for a feature, use:
git checkout -b new-feature
Now you can begin making changes, commit them, and keep your features compartmentalized until they’re ready for integration.
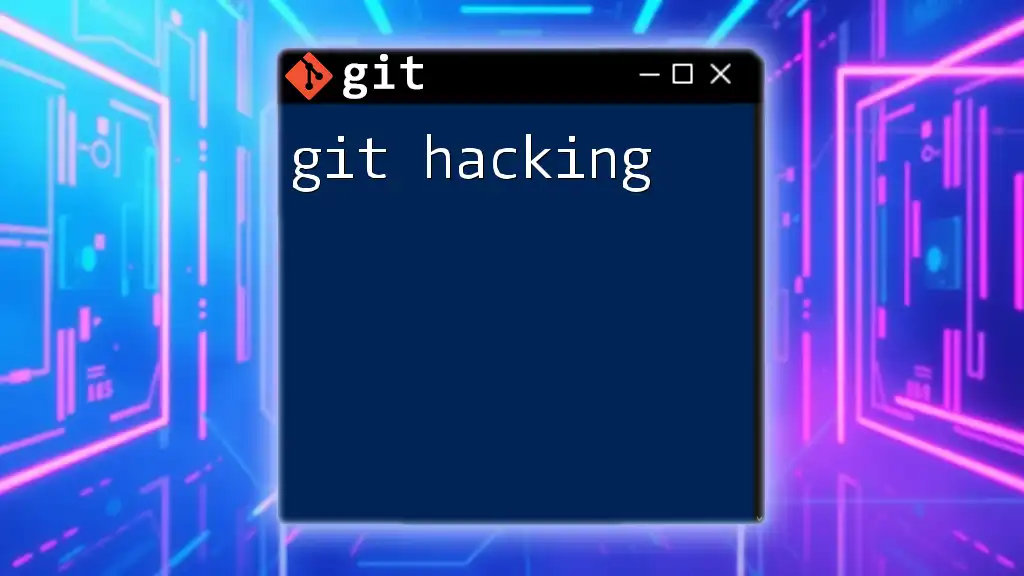
Syncing Your Fork with the Original Repository
Keeping your fork updated with the original repository is crucial. As the original repo evolves, you need to ensure your fork can still merge changes smoothly.
Adding the Original Repository as a Remote
To facilitate this synchronization, add the original repository as a remote named `upstream`:
git remote add upstream https://github.com/ORIGINAL_OWNER/REPOSITORY_NAME.git
Fetching Updates from the Original Repository
To check for updates in the upstream repository, fetch the changes:
git fetch upstream
This command retrieves the new commits from the upstream repository without merging them into your local branches immediately.
Merging Changes into Your Fork
To incorporate changes from the upstream repository into your fork, follow these steps:
-
Switch to your main branch:
git checkout main
-
Merge the changes:
git merge upstream/main
Resolving merge conflicts can be a common occurrence when working in collaborative environments. Be sure to address these conflicts by following Git’s guidance for conflict resolution.
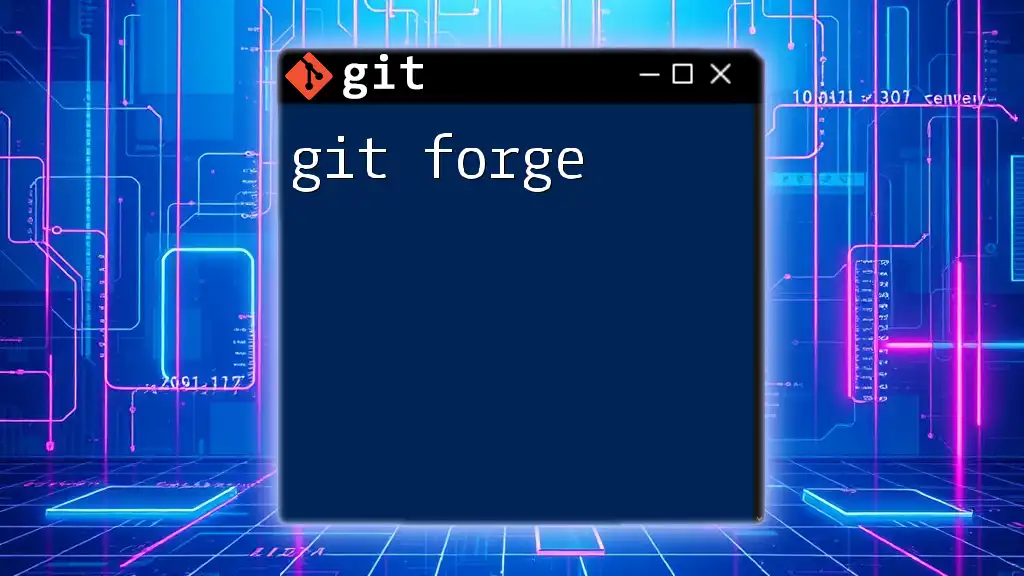
Submitting Changes Using Pull Requests
Once you've made changes and are ready to contribute them back to the original project, you need to create a pull request.
Preparing Your Changes for a Pull Request
Before submitting a pull request, rebase your feature branch to ensure it’s up to date with the upstream:
git rebase main
This command integrates the latest changes from the main branch into your feature branch, helping avoid potential conflicts when your changes are merged.
Creating a Pull Request on GitHub
After you’ve rebased and confirmed your changes are ready, go to your forked repository on GitHub and follow these steps:
- Navigate to the “Pull Requests” tab.
- Click on “New Pull Request.”
- Select the branch you’re merging into the original repository’s base (usually `main`).
- Write a succinct title and provide a detailed description of the changes you’ve made, focusing on what your modifications accomplish and why they are essential.
Reviewing and Addressing Feedback
After submission, project maintainers may review your changes and provide feedback. Responding to this feedback may require making additional changes in your branch:
git commit -m "Address feedback comments"
git push origin new-feature
This process ensures that any improvements can be easily integrated before final acceptance.
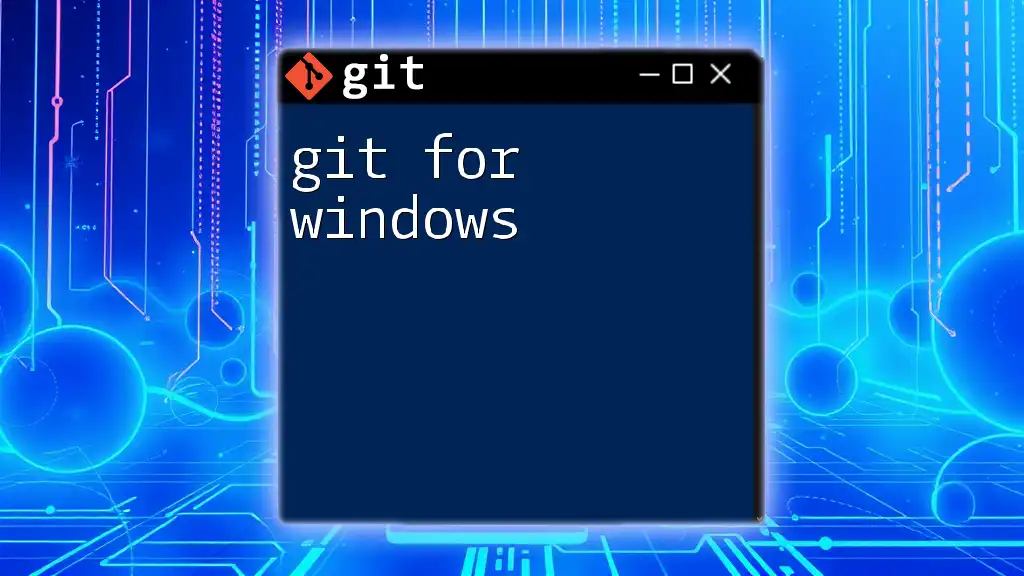
Best Practices for Forking
When working with forks, implementing best practices is crucial for maintaining clarity and efficiency:
-
Keep Your Fork Organized: Regularly clean up old branches and ensure that every feature branch is clearly named according to the feature it develops or the issue it addresses.
-
Use Descriptive Commit Messages: Each commit should narrate what has changed and why. This documentation can be invaluable in collaborative scenarios.
-
Regularly Sync: Frequent synchronization with the upstream repository helps minimize complicated merge conflicts and ensures your code remains compatible with any project changes.
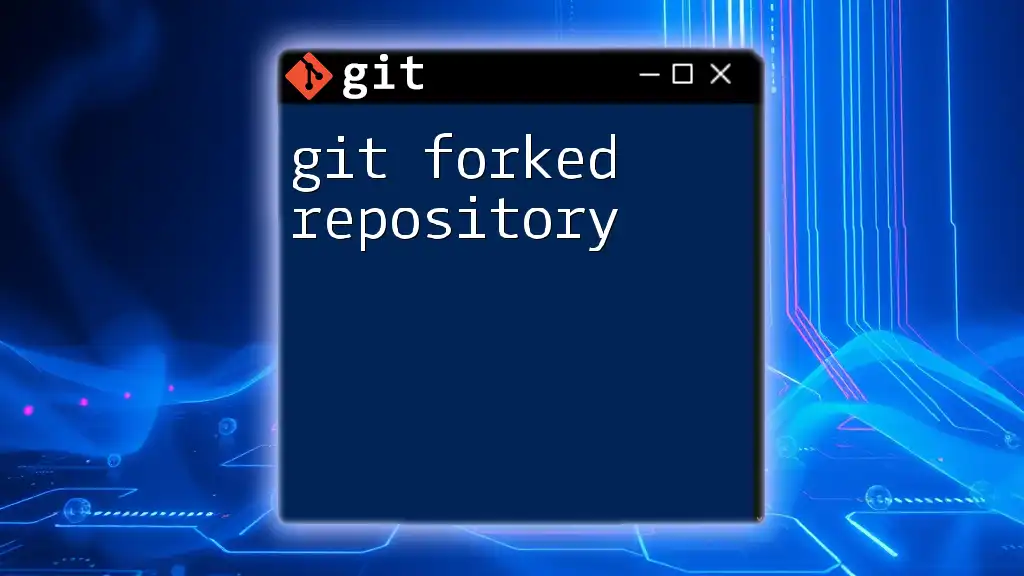
Conclusion
In conclusion, understanding git forking is essential for anyone involved in collaborative software development. Forking provides the flexibility to develop independently while still allowing the opportunity to integrate contributions into the original project. By practicing and applying the concepts outlined in this guide, you can effectively manage your Git repositories and contribute meaningfully to the open-source community.
Feel free to engage with various repositories and leverage the power of forking in your projects!