A Git workflow is a set of rules and processes that teams follow to collaborate effectively using Git commands for version control and project management.
Here's a simple example of a Git workflow involving creating a branch, making changes, and merging those changes back to the main branch:
# Create a new branch for feature development
git checkout -b feature/new-feature
# Make changes to files, then stage and commit them
git add .
git commit -m "Add new feature"
# Switch back to the main branch
git checkout main
# Merge the new feature branch into the main branch
git merge feature/new-feature
# Delete the feature branch after merging
git branch -d feature/new-feature
Understanding Git Workflow
Git Workflow refers to the systematic process of using Git in our projects, especially in collaboration environments. A well-defined Git workflow improves productivity and ensures that developers can work together seamlessly. It provides a structured way to manage code changes, which is crucial in avoiding conflicts and managing feature development effectively.
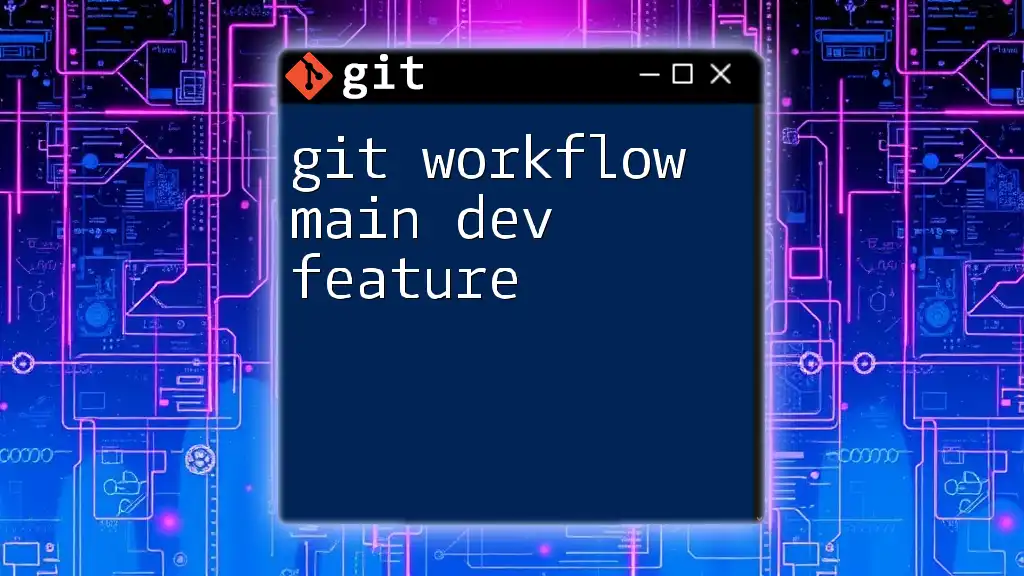
Overview of Common Git Workflows
Centralized Workflow
The Centralized Workflow is one of the simplest approaches. In this setup, all team members work off a single central repository. The primary focus is on the `master` branch, where all changes are pushed after they are tested locally.
Key Commands Used:
- `git clone`: This command retrieves a copy of the repository and is usually the first command used to download the repository to a local machine.
- `git pull`: This command is used to update the local repository with any changes from the central repository.
- `git push`: After making changes, this command is utilized to upload the committed changes to the central repository.
Example Workflow:
- A developer clones the repository with:
git clone https://github.com/username/repo.git
- They create a new feature or fix an issue, regularly pulling changes from the main repository:
git pull origin master
- After testing, they push changes back to the master branch:
git push origin master
Feature Branch Workflow
The Feature Branch Workflow enhances collaboration by creating isolated branches for new features or bug fixes. Each feature or fix is developed in its own branch, making it easier to manage.
Key Commands Used:
- `git checkout -b`: This creates a new branch and checks it out at the same time. It’s a common practice in this workflow.
- `git merge`: This merges the feature branch back into the main branch after completion.
- `git branch`: This command lists all the branches in your repository.
Example Workflow:
- Start by creating a new feature branch:
git checkout -b new-feature
- Make changes and commit them:
git add . git commit -m "Implement new feature"
- Switch back to the master branch:
git checkout master
- Merge the feature branch:
git merge new-feature
Gitflow Workflow
Gitflow Workflow is a more structured approach that includes specific roles for different branches. It is ideal for managing large projects or teams.
Key Branches and Their Purposes:
- `master`: Stable, production-ready code.
- `develop`: Contains the latest delivered development changes for the next release.
- `feature/*`: Used for developing new features.
- `release/*`: Responsible for finalizing a release.
- `hotfix/*`: Used for quick fixes directly to the master branch.
Example Workflow:
- Initialize Gitflow in your repository:
git flow init
- Start a new feature:
git flow feature start new-feature
- Complete the feature:
git flow feature finish new-feature
- Create a release:
git flow release start 1.0.0
Forking Workflow
The Forking Workflow is particularly well-suited for open-source projects. Instead of direct collaborations, contributors create their own fork of the repository, work on features independently, and propose changes through pull requests.
Key Concepts and Commands:
- `git fork`: Usually accomplished through UI on platforms like GitHub; you create a personal copy of a project.
- `git remote add upstream`: This command sets up a connection to the original repository to fetch changes.
Example Workflow:
- Fork a repository on GitHub.
- Clone your fork locally:
git clone https://github.com/your-username/repo.git
- Add the original repository as an upstream remote:
git remote add upstream https://github.com/original-owner/repo.git
- Create a new branch, make changes, and push them to your fork:
git checkout -b fix-issue git add . git commit -m "Fix issue" git push origin fix-issue
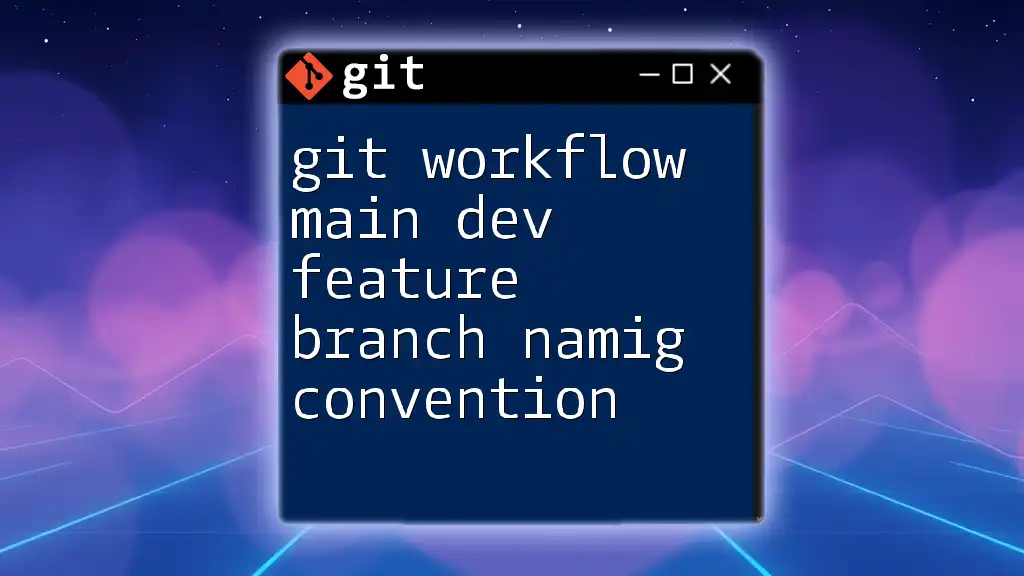
Setting Up Your Git Workflow
Choosing the Right Workflow for Your Team
Choosing a workflow depends on several factors, including team size, project requirements, and collaboration style. For example, smaller teams might benefit from the Centralized or Feature Branch workflows due to their simplicity. In contrast, larger teams with multiple developers working on various features benefit from the structure provided by the Gitflow Workflow.
Tools and Integrations for Effective Workflow
Utilizing Git GUI clients like SourceTree or GitKraken can help visualize branching and merging, making it easier to manage complex workflows. Furthermore, integrating Continuous Integration/Continuous Deployment (CI/CD) tools like Jenkins or CircleCI can streamline the development process, allowing teams to automatically run tests and deploy code.
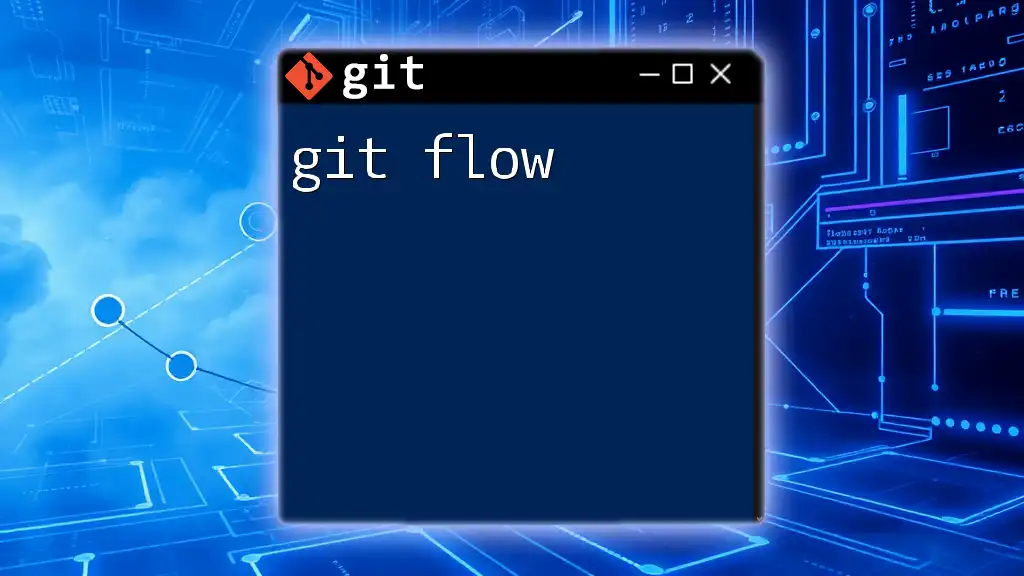
Best Practices for Git Workflows
Consistent Commit Messages
Commit messages are vital for project clarity. They enable team members to understand changes without digging into the code. A recommended format includes:
type(scope): subject
Where type can be `feat`, `fix`, `docs`, etc., and scope briefly indicates the affected component.
Regular Syncing with Remote Repositories
It’s essential to regularly `git fetch` and `git pull` to keep your local repository in sync with the remote, reducing the chances of conflicts and ensuring everyone works with the most recent codebase.
Code Review and Pull Requests
Integrating code reviews through pull requests fosters a collaborative environment. It allows team members to give constructive feedback before merging changes into the main branch and helps maintain code quality.
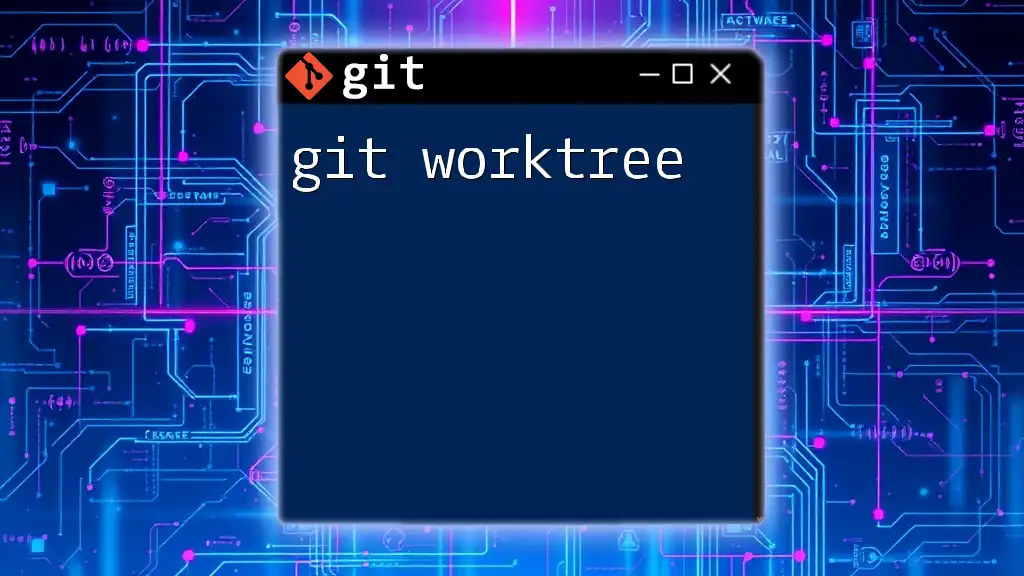
Troubleshooting Common Workflow Issues
Handling Merge Conflicts
Merge conflicts occur when two branches have competing changes. Resolving these conflicts manually is often required. Git notifies you when this happens, and you can use:
git mergetool
To open a diff and manually edit the conflicting files.
Undoing Changes in Your Workflow
Sometimes, you may need to undo changes. Familiarize yourself with:
- `git reset`: Resets your current branch to a specified state.
- `git checkout`: Used to restore files.
- `git revert`: Safely creates a new commit that undoes the changes from a previous commit.
Examples: To reset to the previous commit:
git reset --hard HEAD~1
To undo changes in a specific file:
git checkout -- filename.txt
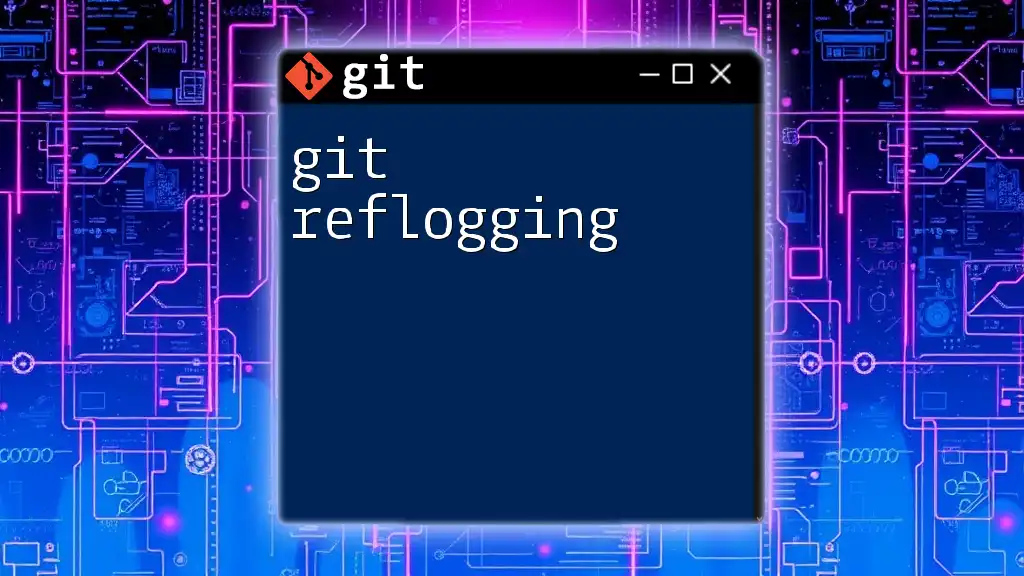
Conclusion
Understanding and implementing a solid Git Workflow is crucial in today’s collaborative development landscape. By choosing the right workflow for your team and adhering to best practices, you can minimize conflicts, maintain code quality, and streamline the development process.
Next Steps for Learning and Implementation
Put your knowledge into practice. Experiment with different workflows, engage in peer reviews, and utilize the resources available, including documentation and online courses, to gain deeper insights into using Git effectively.
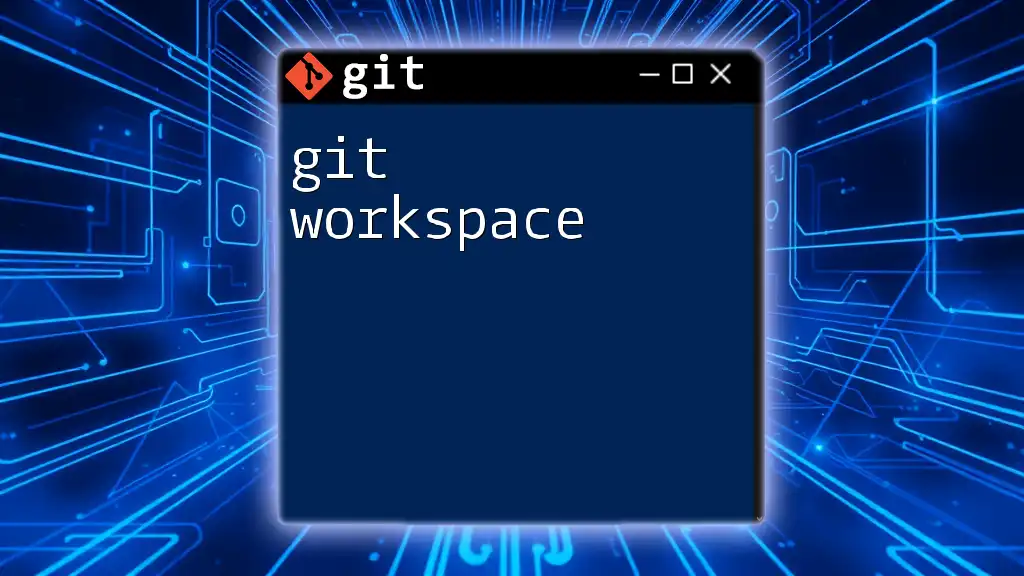
Call to Action
Consider joining our Git training sessions to enhance your skills further and streamline your development processes. Gain confidence in using Git commands with ease, and take your project management to the next level!