Git workspaces are isolated environments where you can manage and edit files independently, allowing you to efficiently work on multiple features or fixes without interfering with each other's changes.
Here's a code snippet to create a new branch in a workspace:
git checkout -b feature-branch
Understanding Git Workspaces
What are Git Workspaces?
Git workspaces are crucial components of the Git version control system. A workspace refers to the collection of files and directories—essentially your project—that you are currently working on, along with the underlying version control information stored in the `.git` directory. This directory holds all the metadata and object database for your repository, allowing you to keep track of changes, branches, and other various features.
If you think of your workspace as your "local project environment," it plays a pivotal role when collaborating with team members. A well-organized workspace not only helps in maintaining a clear structure but also enhances communication among developers.
Why Use Git Workspaces?
Using Git workspaces provides numerous benefits, such as:
- Streamlined Collaboration: When working with multiple developers, a well-managed workspace helps eliminate confusion and reduces the chances of conflicting changes.
- Enhanced Productivity: Workspaces allow developers to isolate features and tasks, enabling them to focus without distractions and facilitating easier code reviews.
Workspaces are particularly beneficial in scenarios such as feature development, bug fixes, and experimental coding, where you might need to juggle several tasks at once.
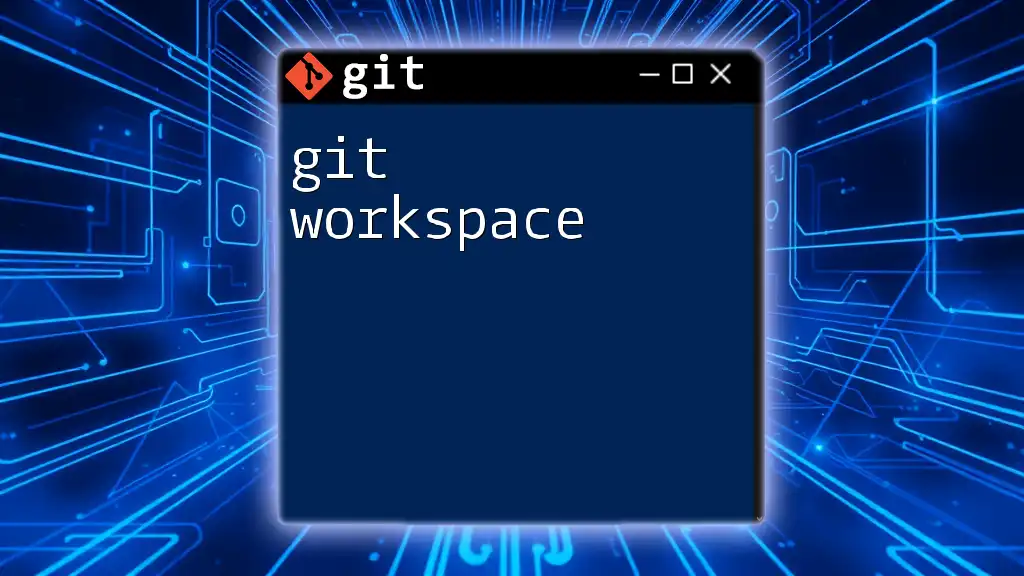
Setting Up Your Git Workspace
Prerequisites for Using Git Workspaces
Before diving into the world of Git workspaces, ensure that you have Git installed on your system. Once installed, familiarize yourself with basic command-line navigation and operations, as Git relies heavily on commands. Understanding version control workflows—such as committing changes, branching, and merging—will also help you navigate Git workspaces effectively.
Creating Your First Git Workspace
To create a new Git workspace, follow these simple steps:
- Open your terminal or command prompt.
- Navigate to the directory where you want your project to reside.
- Run the following command to initialize a new Git repository:
git init my-project
This command will create a new directory named `my-project` and a hidden `.git` folder inside it, marking it as a Git repository. You can then navigate into your new project folder:
cd my-project
Cloning an Existing Repository
If you're joining an existing project, you might need to clone a repository rather than starting fresh. Cloning copies the entire repository, including history and branches. To clone a repository, run:
git clone https://github.com/username/repo.git
This command creates a local copy of the repository in your current directory, allowing you to start working on the project right away.
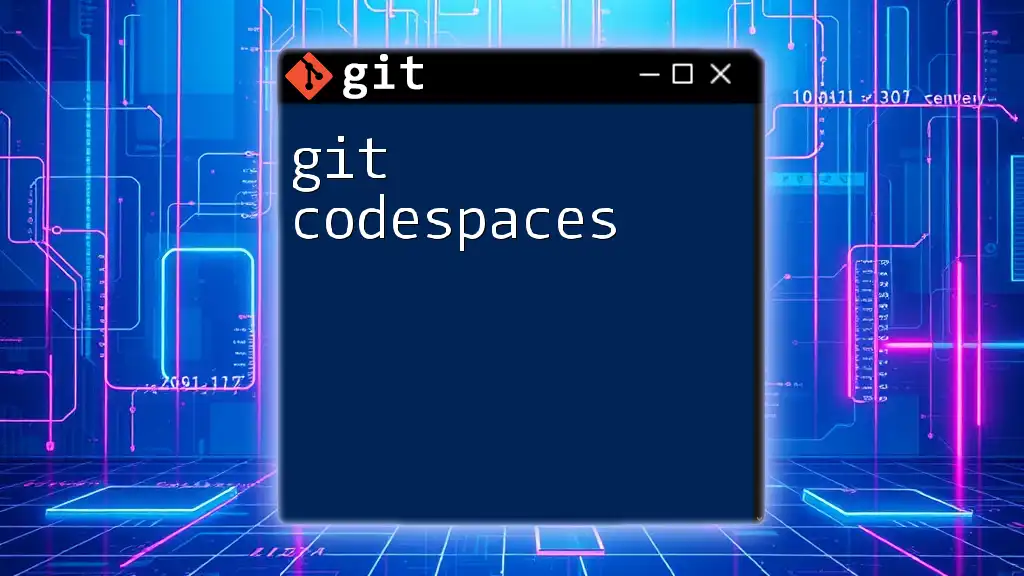
Managing Workspaces
The Structure of a Git Workspace
Understanding the structure of your Git workspace is essential. In your workspace, you’ll typically encounter:
- Project Files: These are your source code files and directories where your actual work occurs.
- The .git Directory: This hidden directory contains all the necessary files Git uses to manage version control, including your commit history, branches, and configuration.
This relationship between your workspace and the `.git` directory is vital for effective version control.
Switching Between Branches
Branches are a cornerstone of Git, enabling developers to work on different features or fixes simultaneously. To switch between branches, use the `git checkout` command:
git checkout branch-name
This command allows you to navigate to the specified branch, making it ready for you to work on. Ensure you commit any changes in your current branch before switching to avoid losing work.
Creating New Branches
When you want to work on a new feature or a fix, creating a new branch is advisable. Use this command to create and immediately switch to a new branch:
git checkout -b new-branch-name
This command creates a new branch based on your current branch, allowing you to work in isolation. Naming conventions for branches can enhance clarity, so consider consistent patterns, like `feature/login-button` or `bugfix/crash-on-start`.
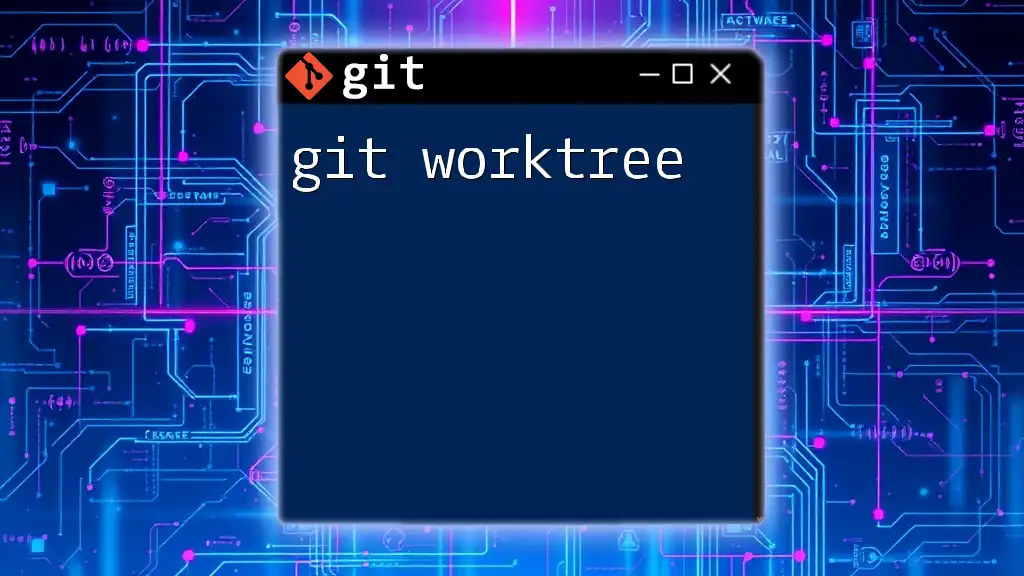
Advanced Workspace Management
Staging Changes
Before committing changes to your repository, you need to stage them. The staging area acts as a preparatory zone where you can review your changes. To stage a specific file, use:
git add filename
If you want to stage all modified files, you can simplify the command as follows:
git add .
This command adds all changes in your current directory to the staging area, ready for the next commit.
Committing Your Changes
Once your changes are staged, the next step in Git workspaces is committing them to the repository. Each commit serves as a snapshot of your project at a given time. A well-crafted commit message is crucial for clarity and maintainability. To commit staged changes, run:
git commit -m "Descriptive commit message"
Aim for concise yet descriptive messages that explain the changes made. This practice can significantly aid in tracking the evolution of the codebase over time.
Discarding Changes
If you decide you want to discard local changes, Git provides several methods to do so. You can revert changes to a specific file using:
git checkout -- filename
If you’ve staged changes but want to reset the staging area, the command you need is:
git reset HEAD filename
Use these wisely, as discarding changes can permanently lose work.
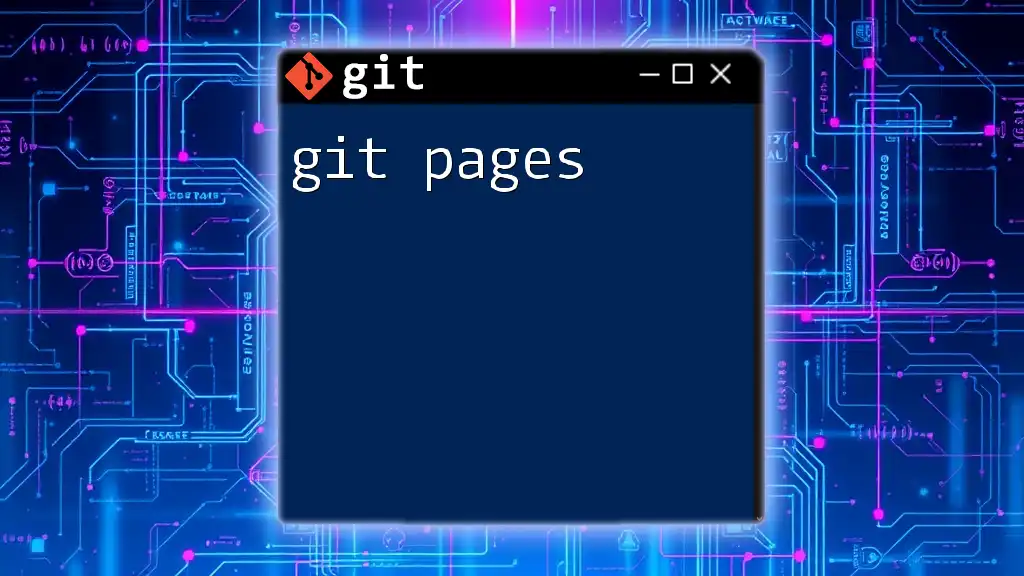
Workspace Best Practices
Keeping Your Workspace Organized
An organized workspace leads to efficient development. Use directories to segregate various aspects of your project—like source files, tests, and documentation. Naming your files clearly will also ease the navigation process and improve team collaboration.
Regularly Update Your Workspace
Don’t forget to keep your workspace updated with changes from your team or the original repository. Pulling the latest changes is essential to avoid conflicts. You can do this easily with:
git pull origin main
This command retrieves updates from the main branch of the remote repository, allowing your local workspace to remain in sync.
Utilizing Remote Repositories
Managing remote repositories is equally vital when working with Git workspaces. Setting up remotes allows you to collaborate effectively with others while maintaining the integrity of your own workspace. You can add a remote connection with:
git remote add origin https://github.com/username/repo.git
This command links your local repository to a remote one, letting you push and pull changes as needed.
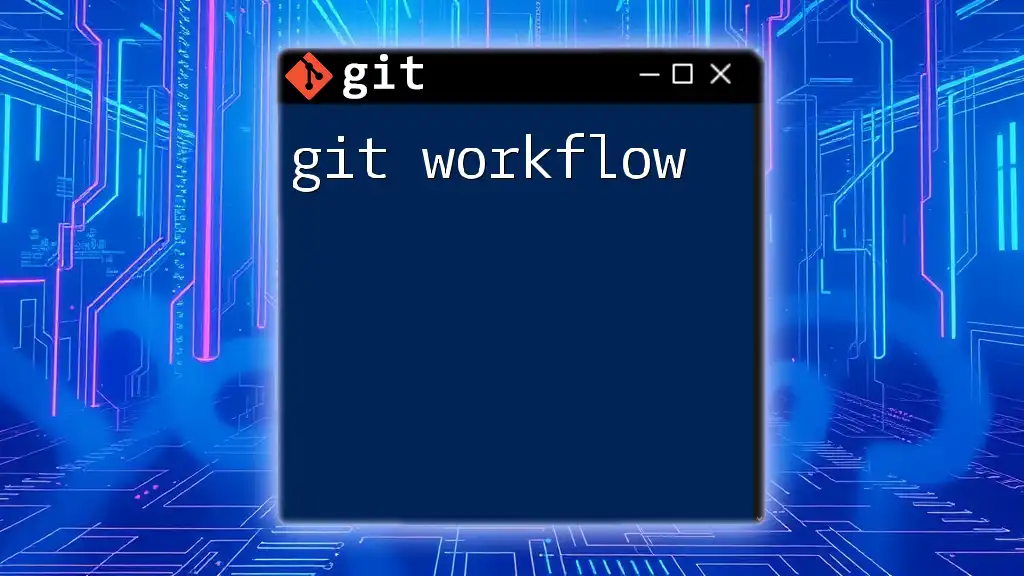
Conclusion
Recap of Git Workspaces
Throughout this guide, we explored the concept of git workspaces—from their definition and importance to how to set up, manage, and maintain them effectively. Understanding the ins and outs of your workspace is crucial for maximizing productivity and collaboration in any development environment.
Next Steps
As you continue to develop your skills, practice managing your git workspaces effectively. Apply the tips you've learned to enhance your workflow, and don't hesitate to explore other resources and documentation to deepen your understanding. Happy coding!