Git is a powerful version control system that allows beginners to track changes in their code with simple commands, such as creating a new repository.
Here’s a basic command to initiate a new Git repository:
git init my-repo
What is Git?
Git is a powerful version control system that allows developers to track changes in their code and collaborate with others seamlessly. Originally created by Linus Torvalds in 2005 to support the development of the Linux kernel, Git has since become the de facto standard in version control. Unlike traditional version control systems such as Subversion (SVN) or Mercurial, Git operates using a distributed model. This means every developer has a full copy of the repository, making it easier to work offline, branch, merge, and manage versions efficiently.
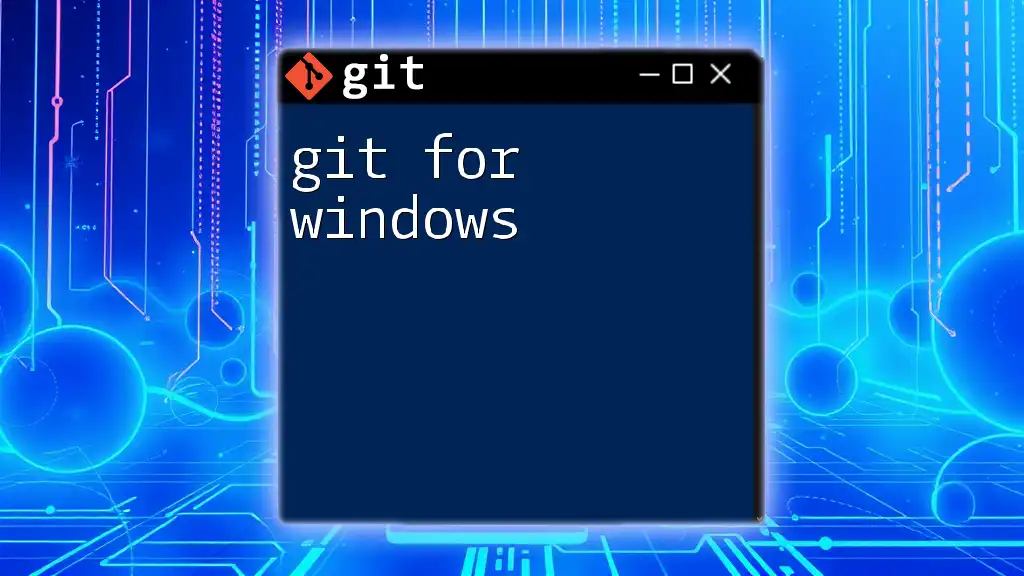
Key Concepts in Git
Repositories
A repository is a storage space where your project resides. It keeps all the project files and the complete history of changes. Local repositories are stored on your computer, while remote repositories are hosted on platforms like GitHub or GitLab.
To set up a new local Git repository, you can use the following command:
git init my-repo
This command creates a new directory called `my-repo`, initializing it as a Git repository where you can start versioning your files.
Commits
A commit in Git is a snapshot of your files at a particular point in time. Commits are fundamental as they allow you to save your progress and document changes. It is essential to write meaningful commit messages that explain the purpose of the changes. A typical command for making a commit looks like this:
git add <file>
git commit -m "Initial commit"
In this example, `git add <file>` stages the changes, and `git commit -m` captures a snapshot along with a message.
Branches
Branching is a feature that lets you diverge from the main line of development and continue to work independently without disturbing the main codebase. This is particularly useful for developing new features or fixing bugs.
You can create a new branch and switch to it with these commands:
git branch new-feature
git checkout new-feature
Now you are on the `new-feature` branch, free to make changes without affecting the `main` branch.
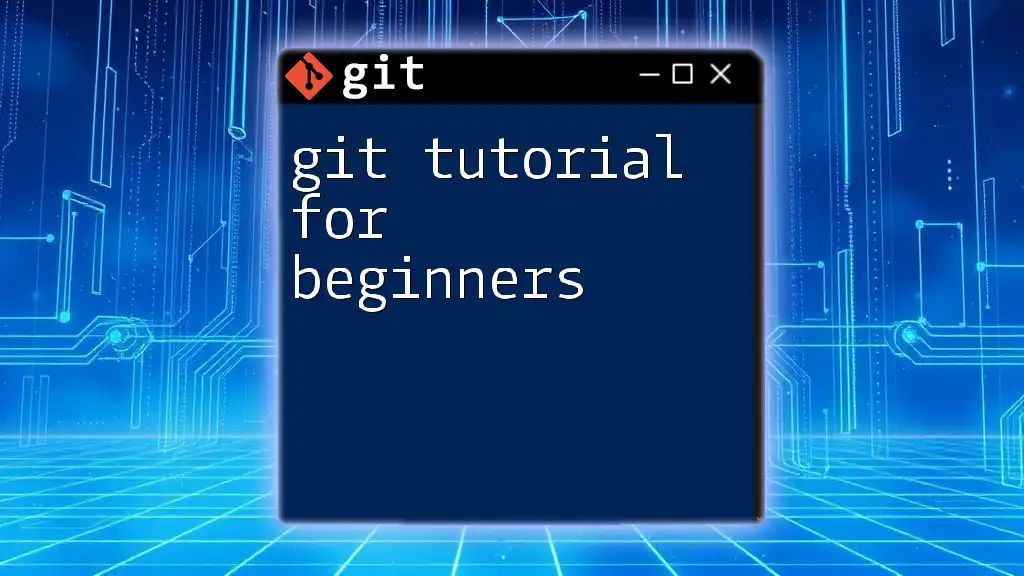
Getting Started with Git
Installation
To begin using Git, you need to install it on your system. Here are the steps for popular operating systems:
- Windows: Download the Git installer from the official website and follow the installation wizard.
- macOS: You can install Git using Homebrew with the following command:
brew install git
- Linux: Most distributions come with Git. You can install it using:
sudo apt-get install git # For Debian/Ubuntu
Configuring Git
Once Git is installed, set up some global configurations to personalize your Git experience. You can configure your username and email with the following commands:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
These configurations are important as they will be associated with your committed code.
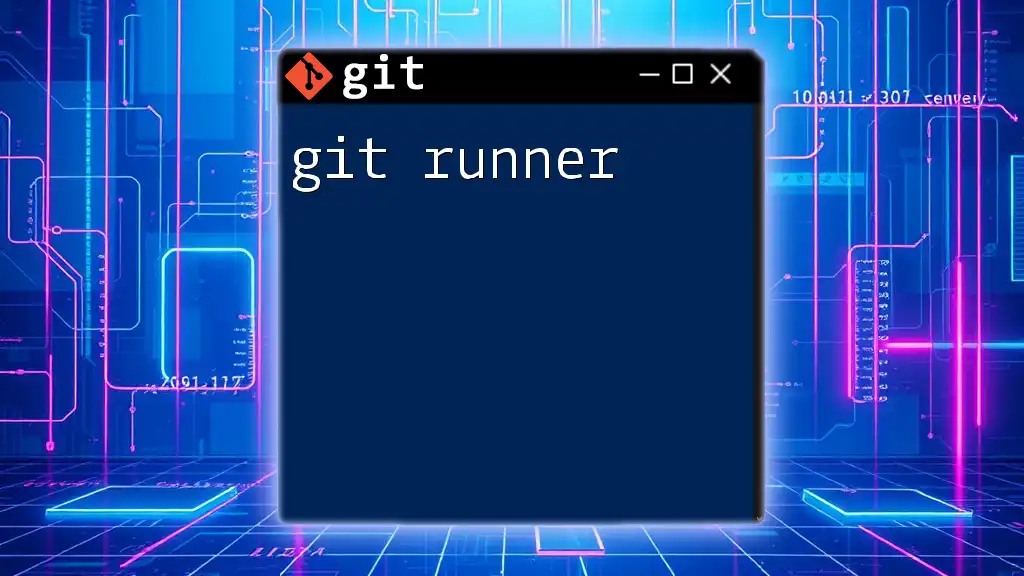
Basic Git Commands
Git Workflow Steps
A typical Git workflow consists of three main stages: Modify → Stage → Commit. You modify files in your working directory, stage those changes in the staging area, and finally create a commit to record the updates in your repository.
Commonly Used Commands
git init
This command initializes a new Git repository in your current directory. After running `git init`, you can begin tracking changes in your files.
cd my-repo
git init
git status
The `git status` command shows the current state of the working directory and staging area. It helps you see which changes have been staged, which files are untracked, and which files are modified.
git status
git add
Use the `git add` command to stage changes you want to commit. This is an essential step in the workflow, as it prepares your changes for the next commit.
git add myfile.txt
git commit
With `git commit`, you create an official snapshot of your staged changes. Adding a descriptive message helps make your commit history understandable.
git commit -m "Added new feature"
git log
To view the history of your commits, you can use the `git log` command. This helps you understand the evolution of your project over time.
git log --oneline
Viewing Differences
git diff
The `git diff` command displays the differences between your working directory and the last commit, helping you visualize what has changed.
git diff myfile.txt
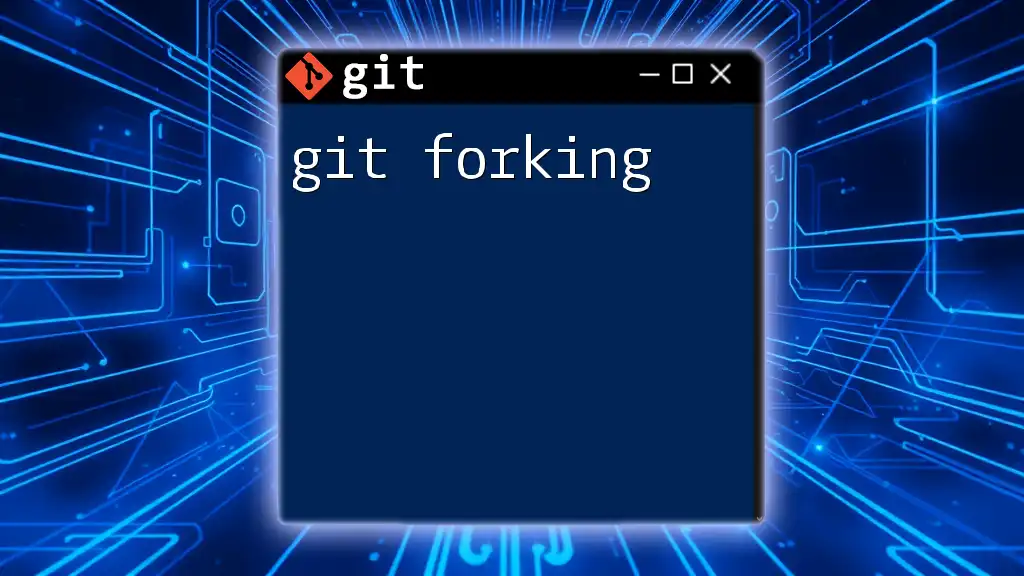
Working with Remote Repositories
Cloning a Repository
If you're working on an existing project, you'll want to use the `git clone` command to copy a remote repository to your local machine. This creates a complete local version of the repository you can work with.
git clone https://github.com/username/repository.git
Pushing Changes
After committing your local changes, push them to the remote repository using the `git push` command. This updates the remote repository with your latest commits.
git push origin main
Pulling Changes
To synchronize your local repository with the latest changes from the remote repository, use the `git pull` command. This command fetches and integrates changes from the remote branch into your local branch.
git pull origin main
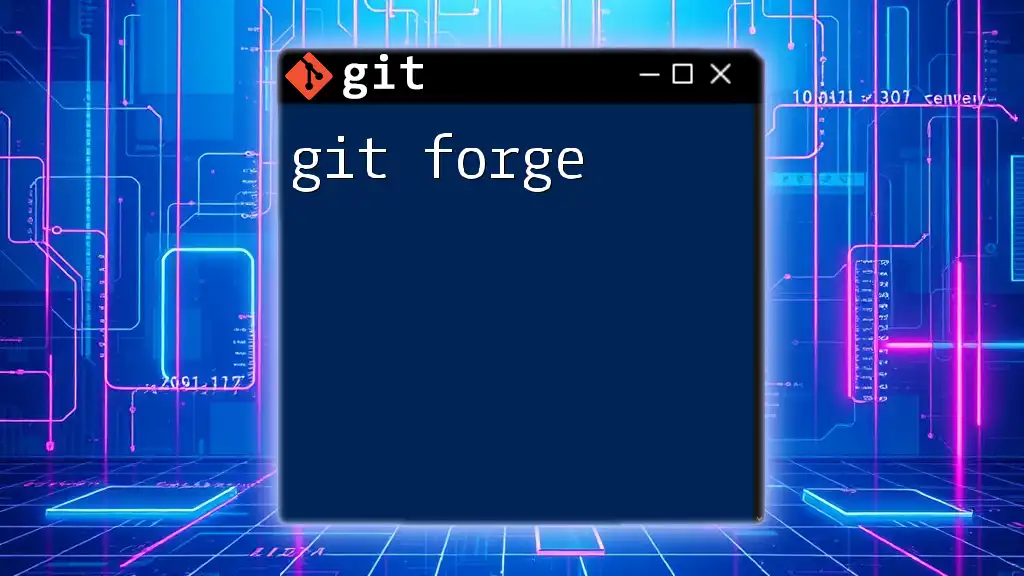
Branching and Merging
Creating Branches
Creating branches allows you to experiment and develop features without affecting the main project. You can create a new branch with:
git branch feature
git checkout feature
Merging Branches
Once your feature is complete, you’ll want to merge it back into the main branch. This can be done by checking out the main branch and then merging:
git checkout main
git merge feature
Handling Merge Conflicts
What are Merge Conflicts?
Merge conflicts occur when changes from different branches cannot be merged automatically. This typically happens when two changes on the same line in a file conflict with each other.
Steps to Resolve Conflicts
To resolve conflicts, identify the files with conflicts using `git status`. Edit the conflicted files to decide which changes to keep and then stage the resolution:
git add resolved-file.txt
git commit -m "Resolved merge conflicts"
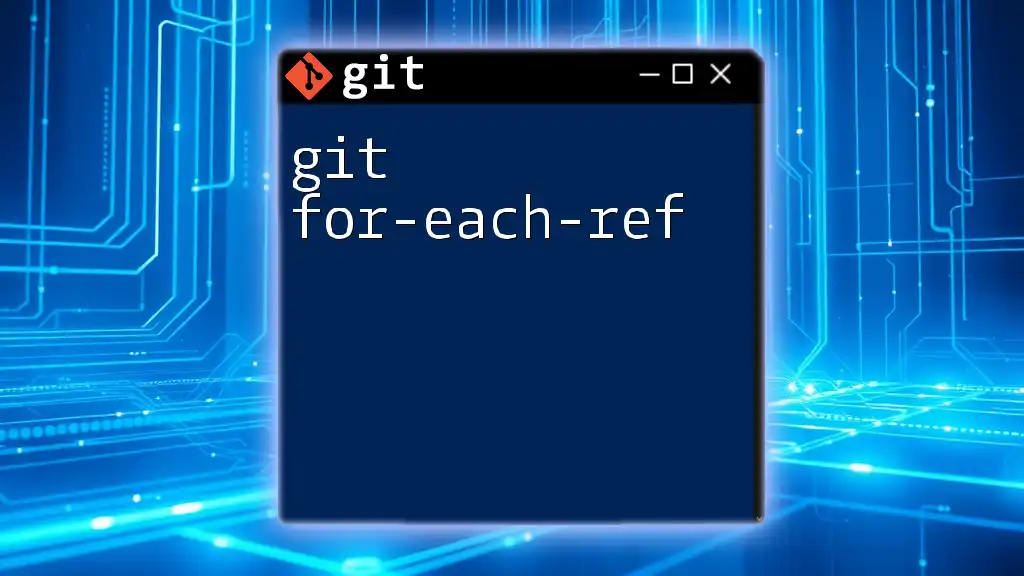
Best Practices for Using Git
Writing Good Commit Messages
To maintain a clear project history, write informative commit messages. Each message should summarize what changes were made and why. A clear structure can improve collaboration:
- Use the present tense (e.g., "Add feature" instead of "Added feature").
- Be concise but descriptive.
Regular Commits and Branching Strategy
Make it a habit to commit changes regularly. This gives you a safety net to roll back to earlier states if necessary. Using a branching strategy like feature branches keeps your code organized and minimizes disruptive changes in the main branch.
Backing Up and Collaborating
Always push your changes to a remote repository after committing. This helps in creating backups and enables collaboration. Remember to pull regularly to keep your local copy in sync with the remote repository.
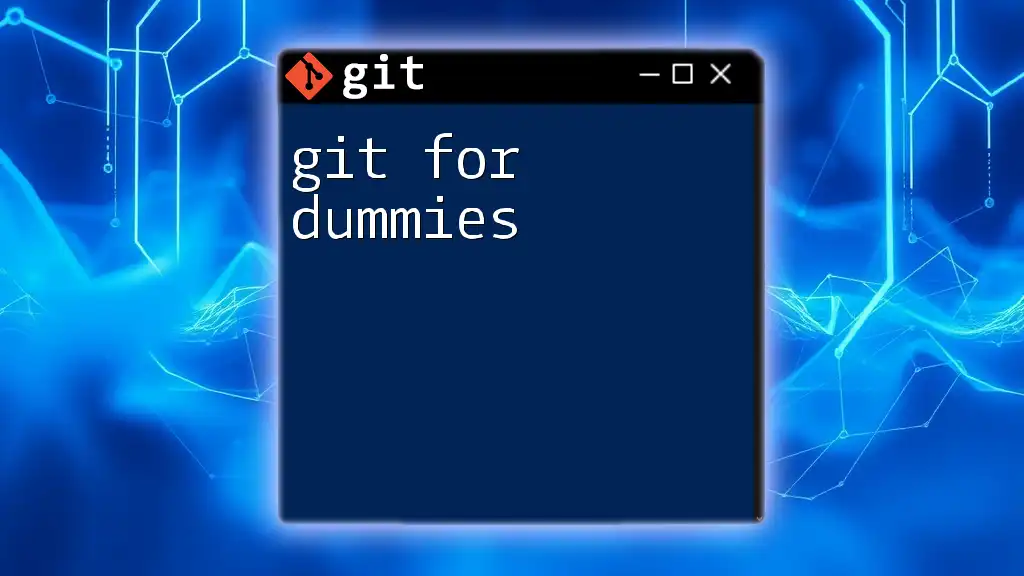
Conclusion
In this guide, we've discussed the essentials of Git for beginners, covering its core concepts, commands, and best practices. Mastery of Git can significantly improve your development workflow, enabling you to manage code versions effectively and collaborate seamlessly with others. As you practice with real projects, you’ll gain confidence and skill, allowing you to harness the full power of Git. For continuous learning, refer to the official documentation and available online resources.