This beginner-friendly Git tutorial provides a quick introduction to essential Git commands to help you manage your code efficiently.
Here's a basic example of initializing a new Git repository and making your first commit:
# Initialize a new Git repository
git init
# Stage changes
git add .
# Commit changes with a message
git commit -m "Initial commit"
What is Git?
Git is a distributed version control system that allows multiple users to collaborate on projects efficiently. Unlike traditional version control systems, which typically have a centralized repository, Git enables each user to have a full copy of the project’s history on their local machine. This decentralized approach enhances speed, flexibility, and collaboration.
Key Features of Git
-
Branching and Merging: One of Git's standout features is branching. Developers can create separate branches for new features, bug fixes, or experiments without affecting the main codebase. Once changes are validated, branches can be merged back into the main repository, simplifying collaboration.
-
Lightweight and Fast Operations: Most Git operations are performed locally, making them significantly faster than systems that rely on a remote server. Whether you're committing changes or switching branches, you'll see quick responses.
-
Strong Integrity of Your Data: Git has built-in mechanisms that ensure the integrity of your codebase. Each commit is stamped with a unique hash, allowing users to track and verify changes reliably.
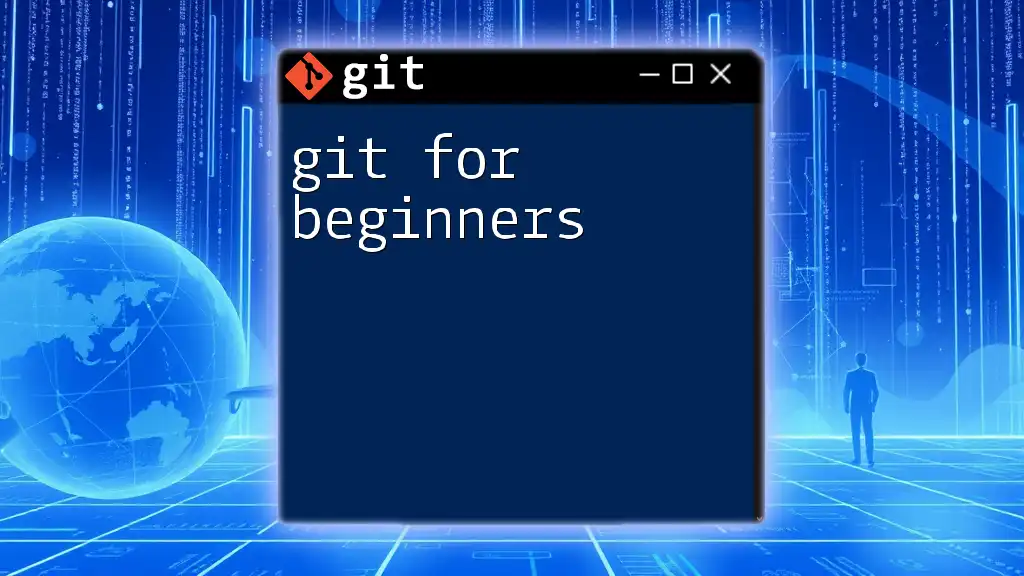
Setting Up Git
Installing Git
To begin using Git, you first need to install it. The process varies by operating system:
-
Windows: Download the Git installer from the [official Git website](https://git-scm.com/download/win) and follow the installation prompts.
-
macOS: The easiest way to install Git is through Homebrew. Simply run:
brew install git
-
Linux: For most distributions, you can install Git via the package manager. For example, on Ubuntu:
sudo apt-get install git
Configuring Git
After installation, configuring Git will personalize your experience. The following commands set up your identity:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
You can verify your configuration by running:
git config --list
This command lists all your current settings, ensuring you’re set up correctly.
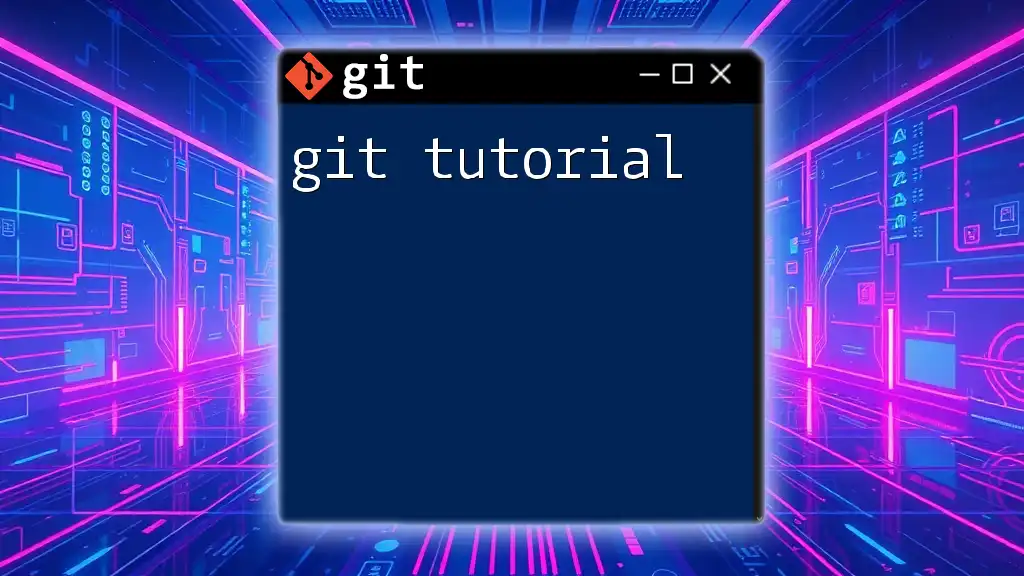
Basic Git Commands
Creating a Repository
To get started with Git, you can either initiate a new repository or clone an existing one.
-
Initiating a New Repository:
git init my-project
This command creates a new directory called `my-project` with a Git repo initialized.
-
Cloning an Existing Repository:
git clone https://github.com/username/repo.git
This command will create a local copy of the specified repository, allowing you to work on it offline.
Checking the Status
To check the state of your repository and see which files have been modified, added, or staged, use:
git status
This command gives you an overview of your current working directory and staging area.
Adding Changes
Before committing your changes, you need to stage them. Staging allows you to include only specific changes in a commit:
git add <file_name>
git add .
Using `git add .` stages all modified files in your working directory, preparing them all for the next commit.
Committing Changes
Once you've staged changes, you can commit them. A commit saves your current changes with a descriptive message about what was done:
git commit -m "Your commit message"
It's essential to write clear and descriptive commit messages, as they serve as the history of your project.
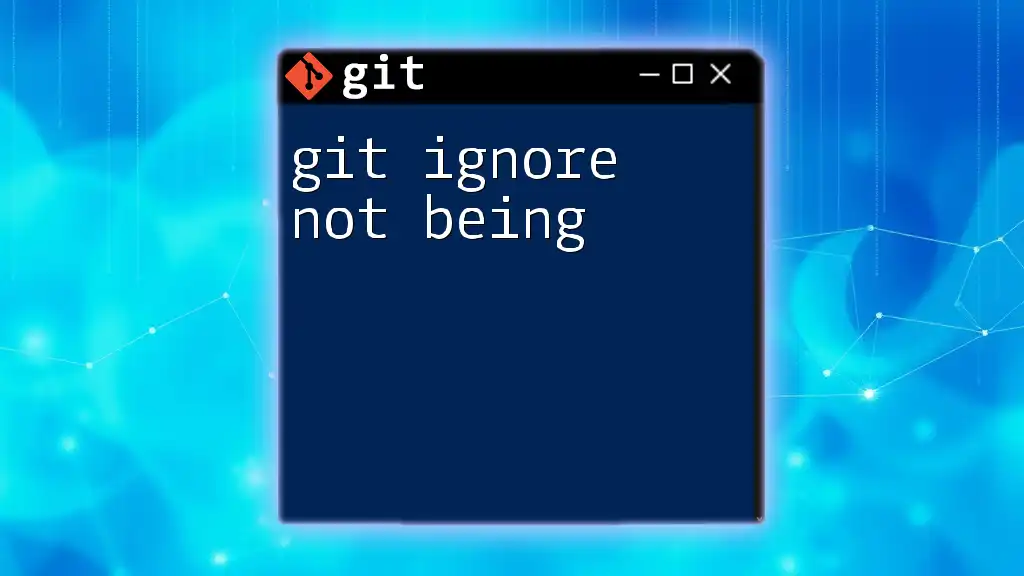
Working with Branches
Understanding Branching
Branches allow you to work on different features simultaneously without disrupting the main codebase. Each branch is essentially a pointer to one of these commits.
Creating a Branch
To create a new branch for developing a feature:
git branch new-feature
Switching Branches
To start working on your new branch, you need to switch to it:
git checkout new-feature
Merging Branches
Once your feature is complete and tested, you can merge it back into the main branch:
git checkout main
git merge new-feature
This command integrates changes from `new-feature` into `main`. If conflicts occur, Git will alert you, allowing you to resolve them manually before finalizing the merge.
Resolving Merge Conflicts
Merge conflicts arise when changes in different branches collide. To resolve conflicts, Git marks the conflicting areas in the files, allowing you to decide which changes to keep. After resolving conflicts, make sure to stage the modified files and commit the merge.
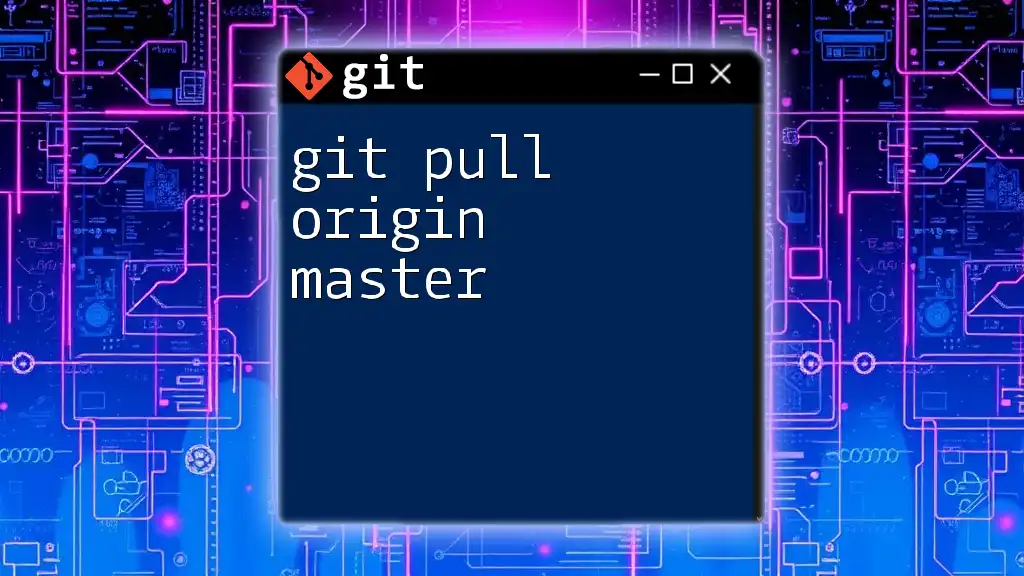
Remote Repositories
Adding a Remote Repository
To link your local repository to a remote one, use:
git remote add origin https://github.com/username/repo.git
This command sets the remote repository URL, allowing you to interact with it.
Pushing Changes
To upload your local changes to the remote repository, use:
git push origin main
This command sends your commits from the local `main` branch to the remote `main` branch.
Pulling Changes
To fetch and integrate changes from the remote repository into your local working directory, use:
git pull origin main
This command ensures your local copy is up to date.
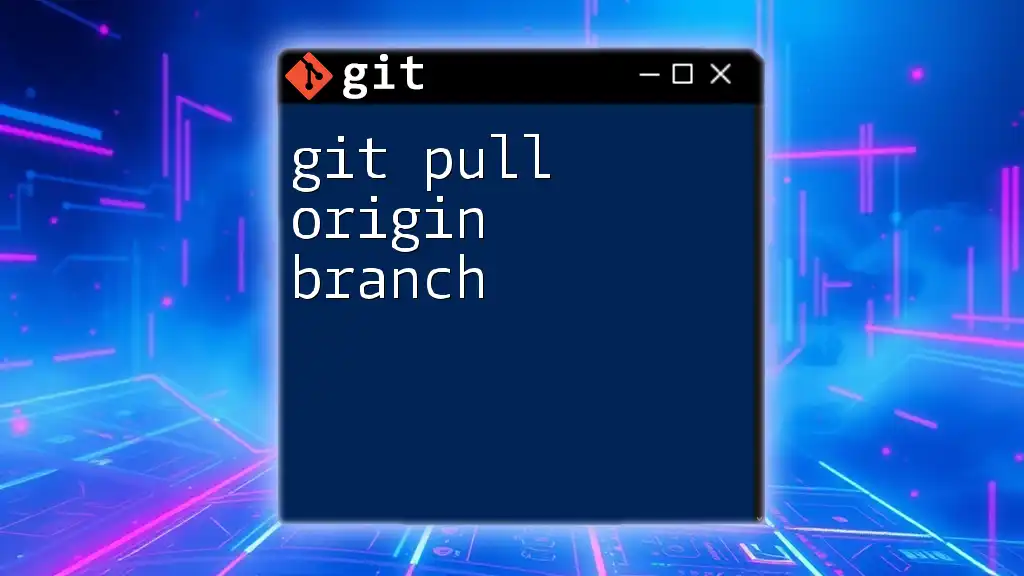
Common Git Workflows
Feature Branch Workflow
In this workflow, each new feature or bug fix is developed in its branch. This separation allows for independent work and easy integration. Once the feature is completed, the branch can be merged back into the main branch.
GitFlow Workflow
GitFlow is a structured workflow that employs specific branch types (main, develop, feature, and release) to manage project lifecycles effectively. Understanding GitFlow can help streamline collaboration in larger projects.
Forking Workflow
The forking workflow is common in open-source projects. Developers copy (fork) a repository to their account, make changes, and then submit a pull request to the original repository. This method allows for robust collaboration and contribution while maintaining oversight.
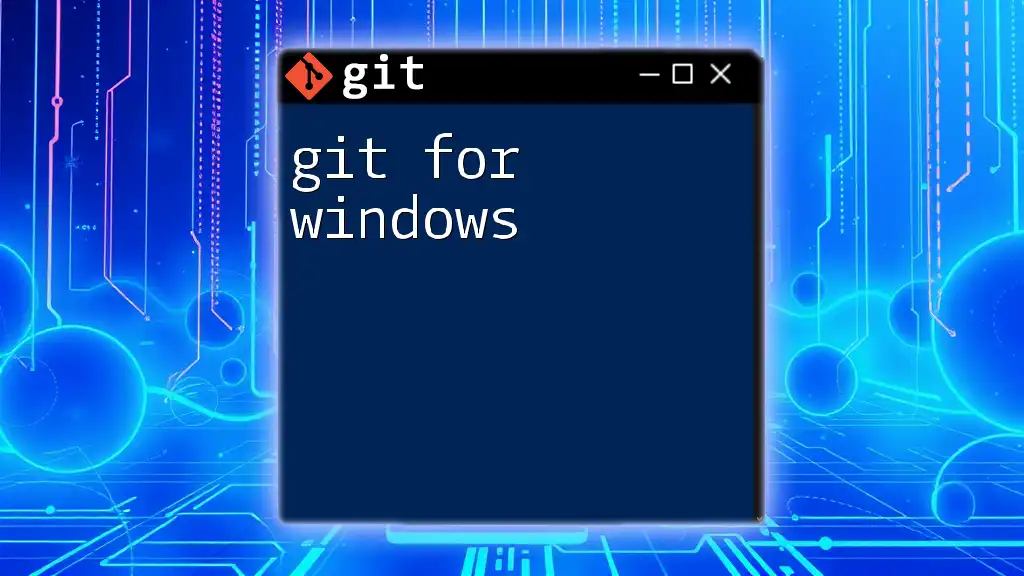
Best Practices for Using Git
Writing Descriptive Commit Messages
Good commit messages enhance collaboration. They provide context and clarity, helping team members understand why changes were made. A useful structure is to start with a short summary followed by a more in-depth explanation.
Committing Often
Frequent commits capture your progress and make it easier to track changes. It’s often easier to debug smaller increments of changes rather than large, sweeping updates.
Using Branches Effectively
Create branches for features, fixes, or experiments rather than working directly on the main branch. This separation prevents accidental changes to stable code and promotes smoother collaboration.
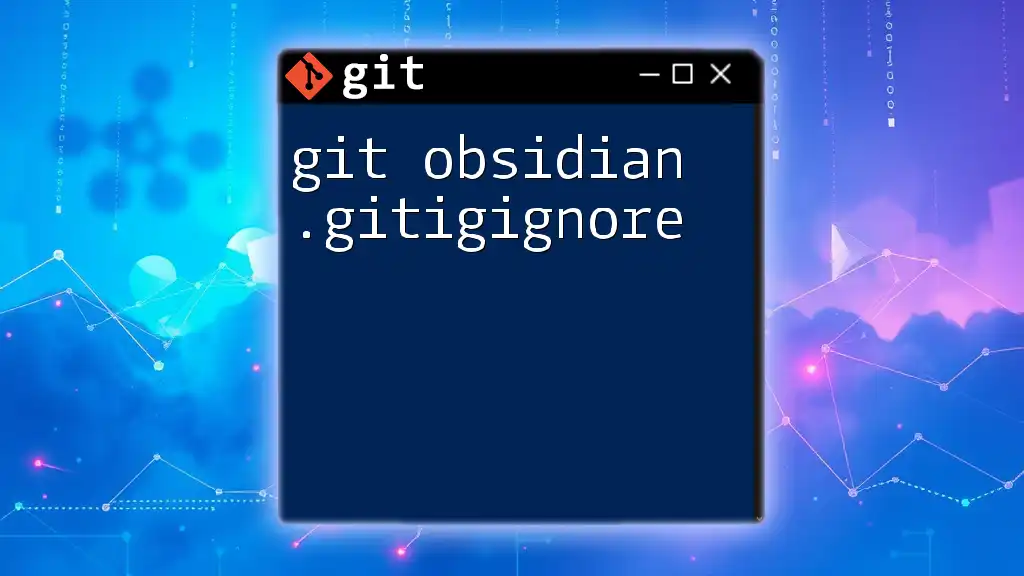
Troubleshooting Common Issues
Undoing Changes
If you need to revert a commit, you can use:
git revert <commit_hash>
This command creates a new commit that undoes the specified commit's changes.
For more drastic measures, you can reset your changes:
git reset --hard HEAD^
This command will discard your last commit and reset your working directory to match the previous state.
Recovering from Mistakes
Mistakes happen, and Git provides tools to recover lost commits. If you find yourself in a tricky situation, commands like `git reflog` can show you a log of all the references in your repository, making it easier to find previous states.
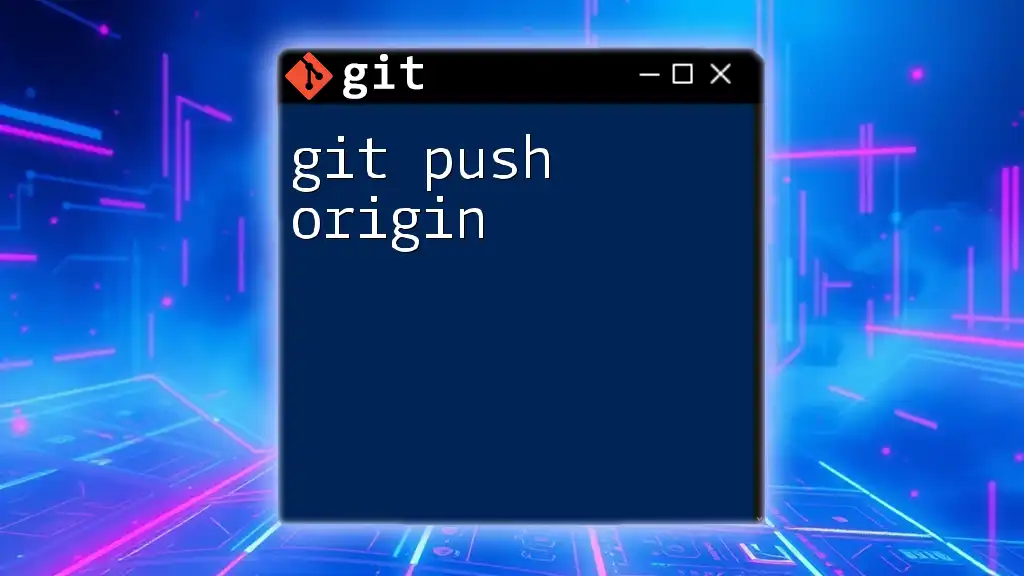
Conclusion
Understanding Git is an essential skill for any developer. This tutorial serves as a foundation, introducing you to the core commands, workflows, and best practices for using Git effectively. The more you practice, the more comfortable you will become with these commands, enabling you to manage your projects with confidence. Continue to explore further tutorials and resources to deepen your knowledge and proficiency in using Git.
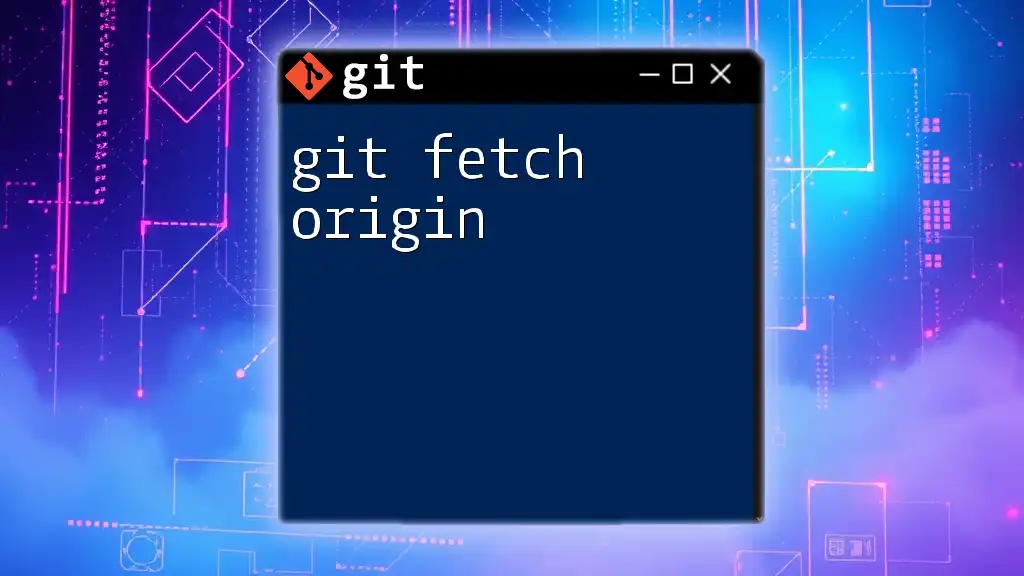
Additional Resources
- Recommended Books and Courses: Look for resources that delve deeper into advanced Git topics.
- Useful Online Tutorials: Many platforms offer interactive exercises to practice Git commands.
- Git Documentation Links: Always refer to the [official Git documentation](https://git-scm.com/doc) for comprehensive guidance.
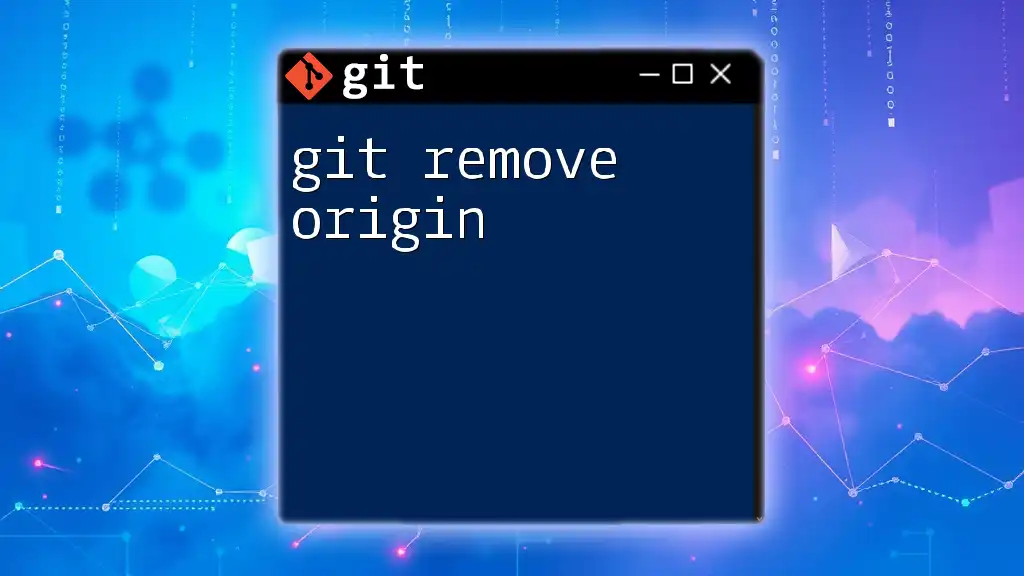
FAQs about Git
-
What is the difference between `git pull` and `git fetch`?
`git fetch` downloads changes from the remote repository but does not integrate them into your local branch. In contrast, `git pull` fetches changes and immediately tries to merge them. -
Can I delete a branch in Git?
Yes, you can delete a local branch using:git branch -d branch-name
To delete a remote branch, use:
git push origin --delete branch-name
The mastery of Git significantly enhances your ability to manage code and collaborate effectively within a team environment. Remember, practice makes perfect!