In this Git tutorial, you'll learn how to efficiently use essential Git commands to manage your version control with ease.
Here's a quick example of how to initialize a new Git repository:
git init
What is Git?
Git is a distributed version control system designed to handle everything from small to very large projects with speed and efficiency. It allows multiple developers to work on the same project simultaneously without interfering with each other's changes. Originally created by Linus Torvalds in 2005 for developing the Linux kernel, Git has since become the most widely used version control system in the world.
Key features of Git include:
- Branching: Git makes it easy to create, merge, and delete branches, allowing developers to experiment with new features or bug fixes without affecting the main codebase.
- Staging Area: Git has an intermediate stage called the staging area where changes can be reviewed before committing, helping maintain a clean commit history.
- Distributed: Every contributor has a full copy of the repository on their local machine, making it possible to work offline and then sync changes later.
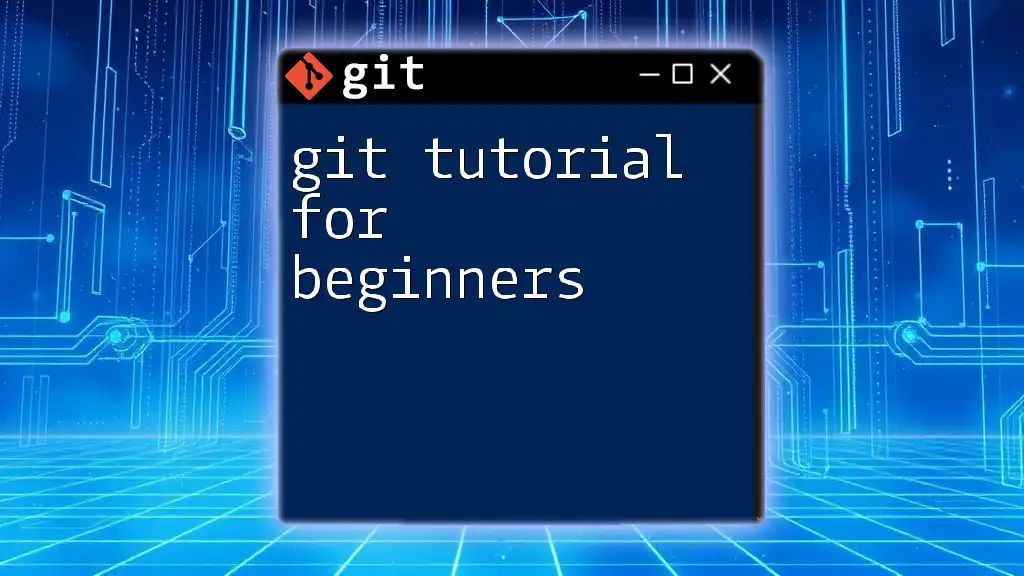
Benefits of Using Git
Using Git offers a myriad of advantages:
-
Collaboration and Teamwork: With Git, multiple team members can work on different parts of a project simultaneously. Changes can be merged easily, fostering collaboration without fear of overwriting each other’s work.
-
Tracking Changes: Every change made to files is logged along with metadata, making it easy to track who made what changes and why. This is essential for accountability and understanding the evolution of a project.
-
Branching and Merging Capabilities: Git’s ability to create branches allows developers to work on features independently. When a feature is complete, it can be merged back into the main branch seamlessly.
-
Open Source and Community Support: As an open-source project, Git benefits from a large community of users who contribute to its ongoing development, provide support, and create educational resources.
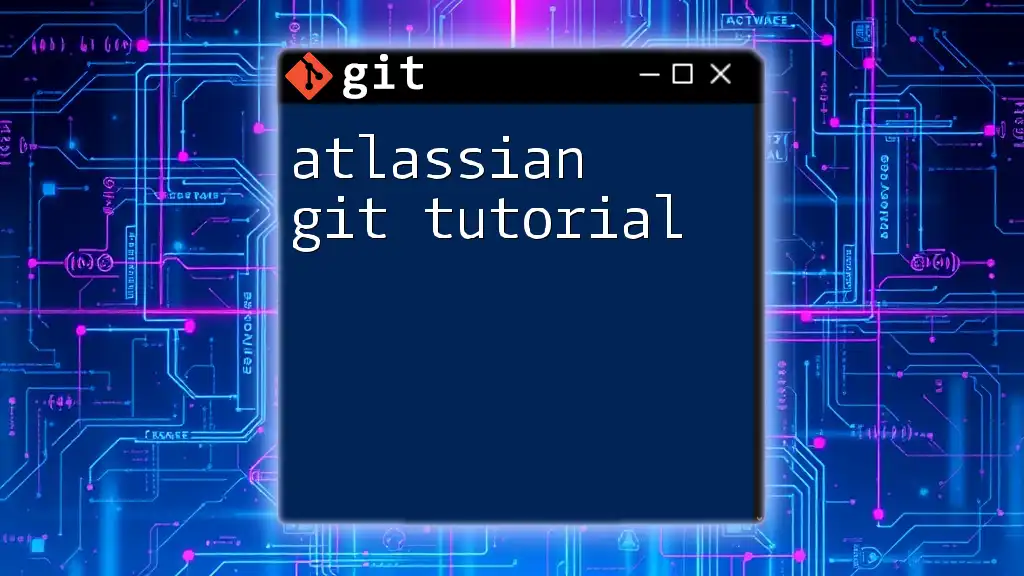
Getting Started with Git
Installation of Git
It's important to have Git installed on your computer before you can start using it. Here’s how to install Git on various operating systems:
-
Windows Installation: You can download the Git installer from the official Git website. Follow the installation wizard, accepting the default options.
-
Mac Installation: For most Mac users, Git is pre-installed. You can check by running `git --version` in the Terminal. If it’s not installed, you can install it via Homebrew with the command:
brew install git
-
Linux Installation: Depending on your distribution, you can use the following commands:
sudo apt-get install git # For Debian/Ubuntu sudo yum install git # For CentOS/RHEL
Configuring Git
Once Git is installed, it's crucial to configure your username and email address, as these details will be linked to your commits.
To set up your username, run:
git config --global user.name "Your Name"
To set your email address, use:
git config --global user.email "youremail@example.com"
To verify your settings, execute:
git config --list
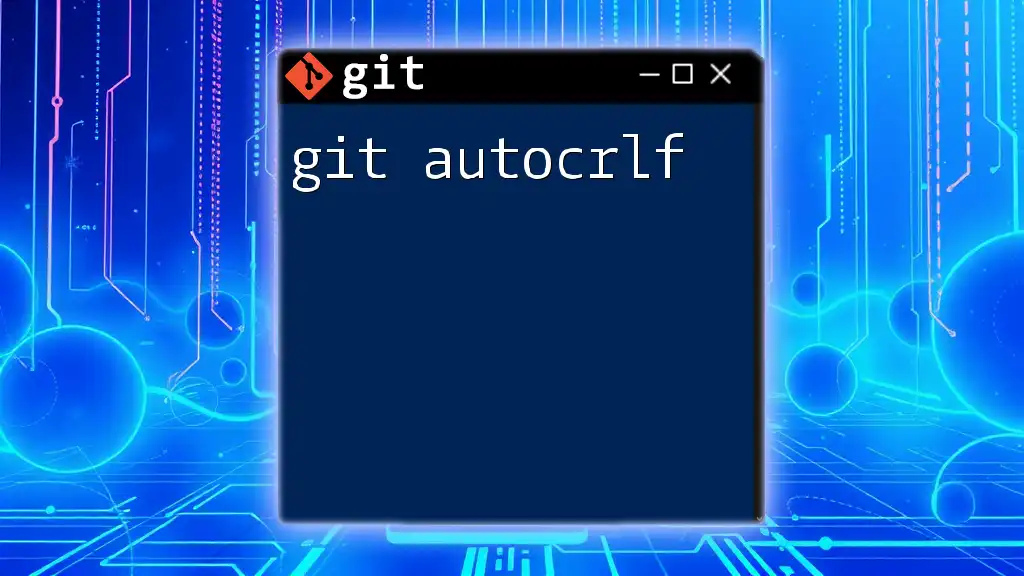
Basic Git Commands
Initializing a Repository
Before you can start using Git, you need to create or clone a repository.
A repository is a container that holds all the project files and their history. You can create a new repository using:
git init my-project
This command initializes a Git repository in the folder named `my-project`.
If you want to work on an existing project, you can clone a repository:
git clone https://github.com/username/repository.git
This will create a local copy of the repository on your machine, including all its history.
Making Changes to Your Code
Adding Files
To begin tracking changes made to files, you need to add them to the staging area using:
git add filename.txt
To add all changes in the current directory, you can use:
git add .
Committing Changes
Committing changes saves the changes you've staged in your repository's history. It’s important to write a descriptive message that clearly explains what changes have been made:
git commit -m "Your commit message"
Viewing Changes
Checking the Status
You can see which changes are staged, unstaged, or untracked by using:
git status
This command is helpful to confirm if you have staged your changes properly before a commit.
Viewing Commit History
To view your commit history, use:
git log
This command shows a list of all commits made, including their commit hashes, authors, dates, and messages.
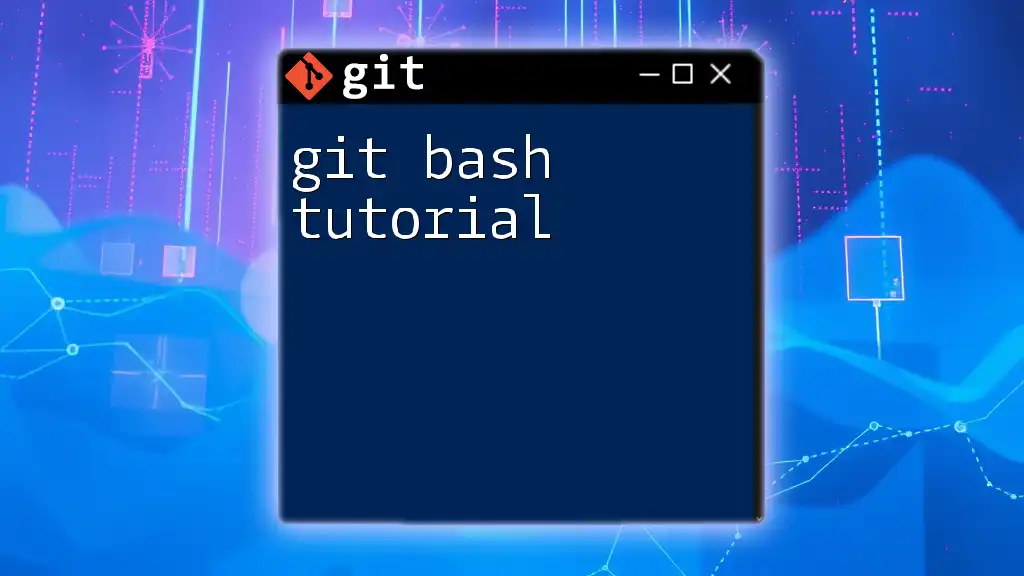
Branching and Merging in Git
Understanding Branches
Branches are pivotal in allowing you to work concurrently on various features or bug fixes. You can create a new branch with the command:
git branch new-branch
Switching Branches
To switch to the newly created branch, execute:
git checkout new-branch
This command will change your current working branch to `new-branch`.
Merging Branches
Once the work on your branch is complete, you can merge it back into the main branch (often called `main` or `master`):
git merge new-branch
This command integrates changes from `new-branch` into the current branch, allowing you to combine work seamlessly.
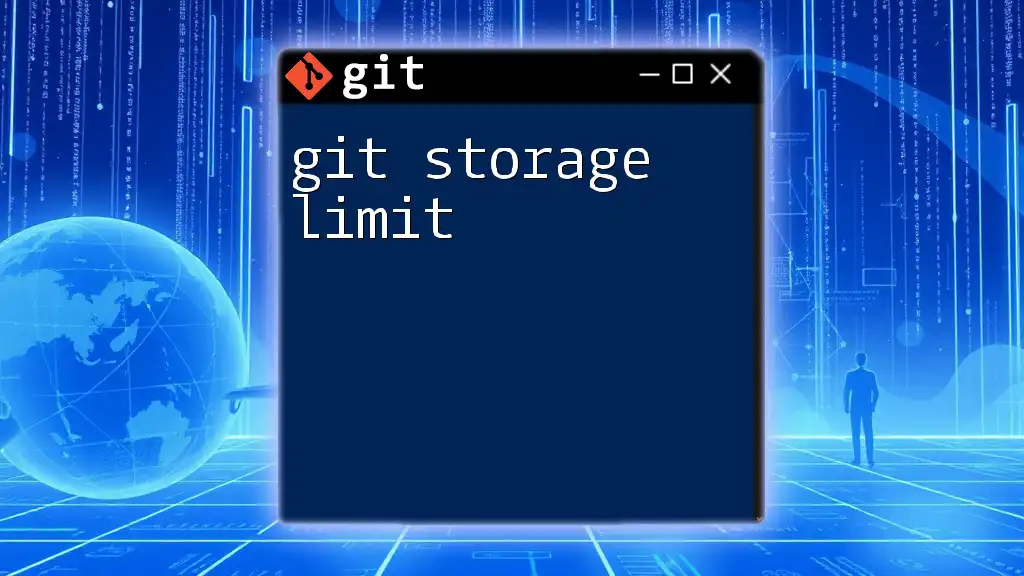
Remote Repositories
Connecting to a Remote Repository
Remote repositories store your code in the cloud, making collaboration easier. To link your local repository to a remote one, use:
git remote add origin https://github.com/username/repository.git
Pushing and Pulling Changes
Pushing Changes
When you want to upload your commits to the remote repository, use the push command:
git push origin main
This command sends your changes to the remote repository, making them accessible to others.
Pulling Changes
To get the latest changes from the remote repository, you can use:
git pull origin main
This command merges changes from the remote `main` branch into your current branch.
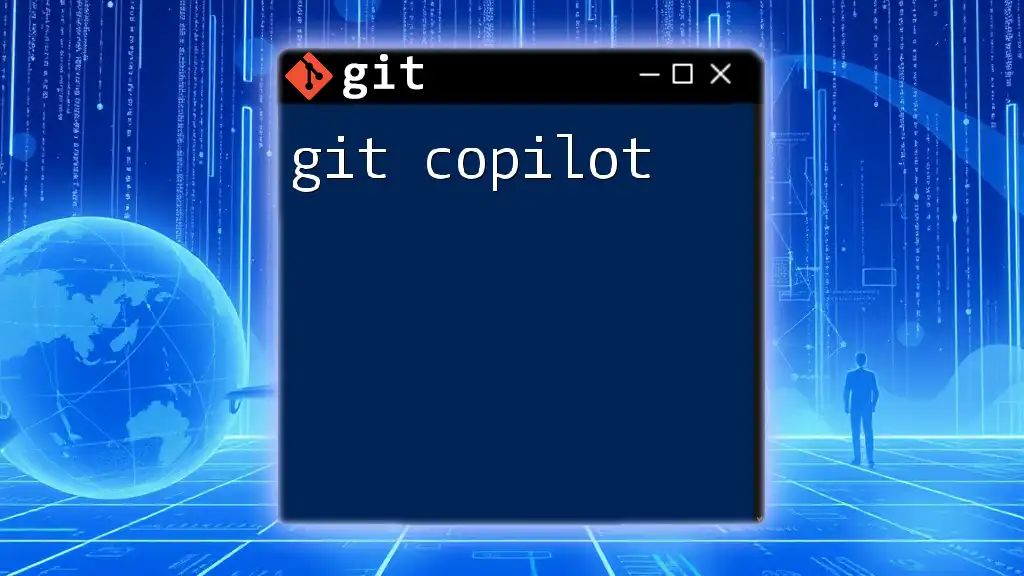
Common Git Workflows
Feature Branch Workflow
In this workflow, each new feature or fix is developed in its branch separate from the main branch. Once it's ready, it will be merged back into the main branch after review. This method promotes clean and organized code management.
Git Flow Workflow
Git Flow is a structured branching model that defines specific processes for managing features, releases, and hotfixes. Adopting Git Flow enhances collaboration and reduces the chaos of handling simultaneous changes.
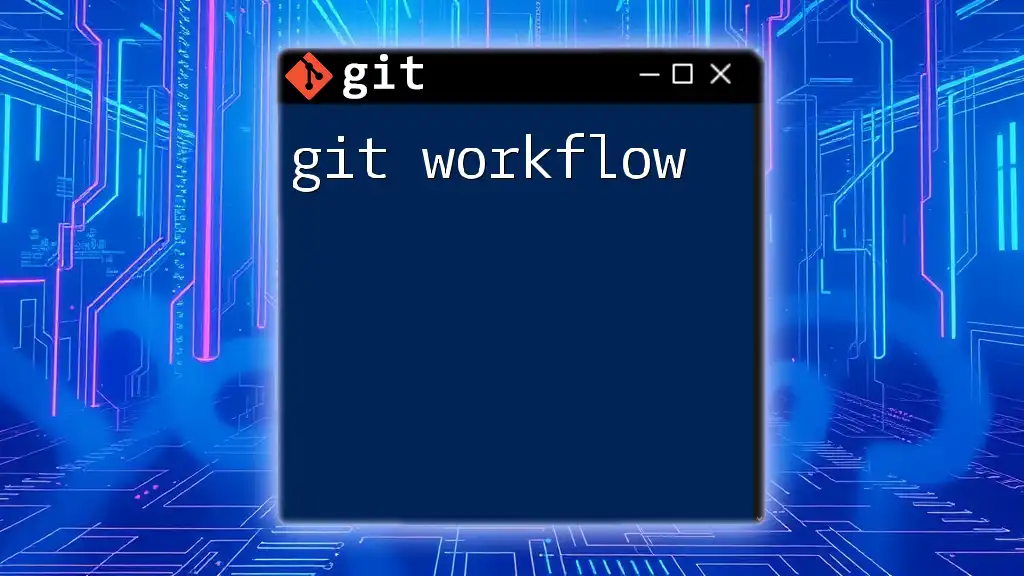
Best Practices for Using Git
Writing Good Commit Messages
A well-written commit message is essential for understanding the history of a project. A good message starts with a short summary (50 characters) followed by further explanations if needed. Think of your message as a way to communicate what you've accomplished.
Regularly Pulling from Remote
To minimize merge conflicts when working collaboratively, it's crucial that you regularly pull changes from the remote repository. This practice ensures that your local branch is always up-to-date with the latest changes made by others.
Using `.gitignore`
Not all files should be tracked by Git. You can specify files and directories that Git should ignore by creating a `.gitignore` file in your repository. For example:
# Ignore node_modules
node_modules/
This file is essential for ignoring temporary files generated by your IDE or build system.
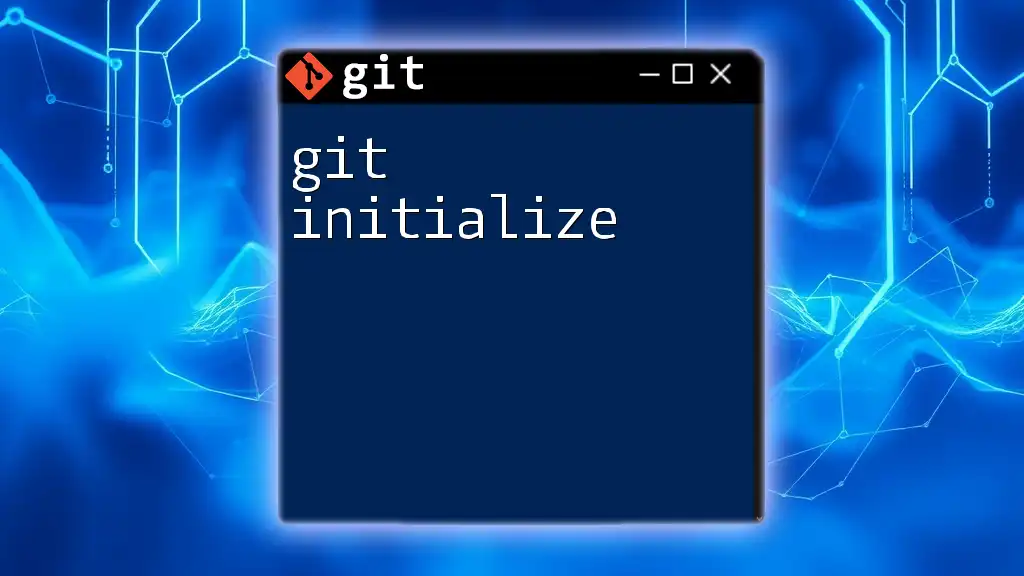
Troubleshooting Common Git Issues
Resolving Merge Conflicts
Merge conflicts occur when two branches have changes in the same part of a file. When this happens, Git will pause the merge process and allow you to manually resolve the conflicts. You can do this by opening the affected files, reviewing the changes, and deciding how to merge them.
Undoing Changes
Undoing a Commit
If you need to undo a commit, you can reset your branch to a previous state:
git reset HEAD~1
This command will remove the last commit but keep your changes in the staging area.
Discarding Changes
To discard uncommitted changes in a specific file, you can use:
git checkout -- filename.txt
This command restores the file to the last committed state, losing any changes made since then.
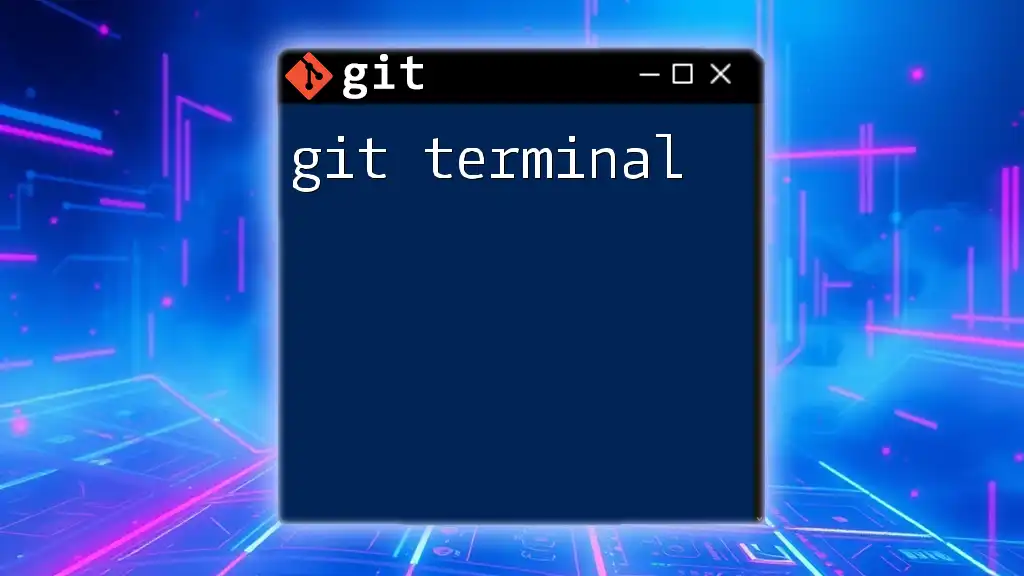
Conclusion
In summary, Git is an invaluable tool for version control, encouraging collaboration and code management efficiency. From initializing a repository to resolving conflicts, understanding Git commands and workflows can greatly enhance your programming experience.
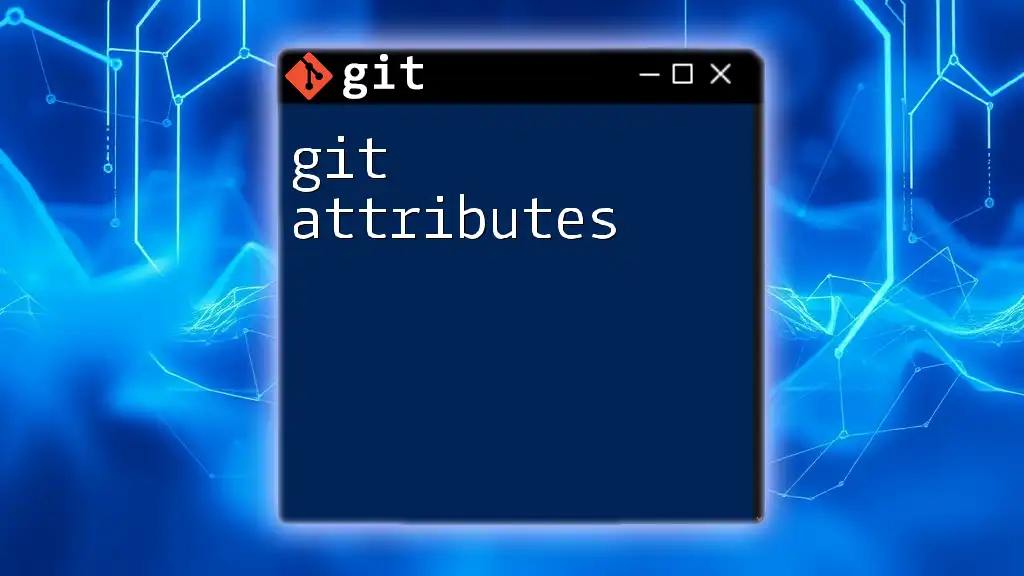
Further Resources
To deepen your understanding of Git, consider exploring recommended books like "Pro Git" by Scott Chacon and Ben Straub, or online courses available on platforms like Codecademy or Udemy. The official Git documentation is also an excellent resource for comprehensive learning.
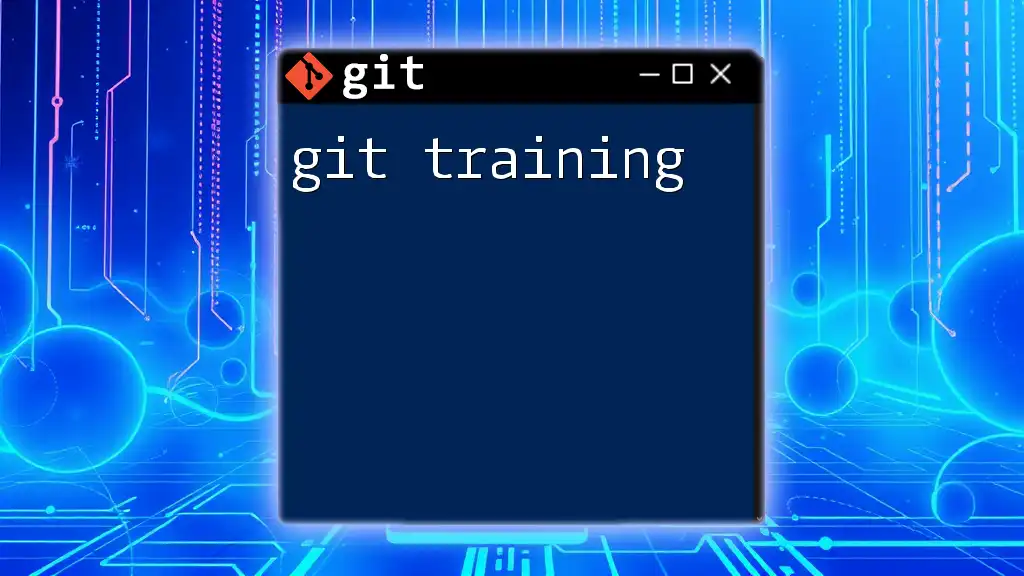
Call to Action
Now that you've learned the essentials of Git, try implementing these commands and practices in your projects. Experiment with features, branches, and merge to get hands-on experience. Don’t forget to follow us for more tutorials and resources on mastering Git!