In Git, a "topic branch" is a short-lived branch created for a specific feature or bug fix, allowing for tidy isolation of changes before merging them into the main codebase.
# Create a new topic branch for a feature
git checkout -b feature/my-new-feature
# Make your changes, then stage and commit them
git add .
git commit -m "Add my new feature"
What is Git?
Git is a powerful, distributed version control system that enables developers to track and manage changes to their codebase over time. It is designed to handle projects of any scale efficiently, allowing for collaborative workflows. By utilizing Git, teams can work in parallel, maintaining a streamlined process that enhances productivity and minimizes conflicts.
Key Features
- Distributed Version Control: Each user has a full copy of the repository, including its history, enabling offline work and full-fledged backups.
- Staging Area: Git enables users to prepare changes before committing them, offering more control over what goes into a commit.
- Branching and Merging: Allows developers to create branches for new features or experiments without affecting the main codebase. When changes are ready, merging brings them back into the main line of development seamlessly.
Why Use Git?
Using Git is advantageous for multiple reasons:
- Collaboration: Teams can collaborate on projects with ease, facilitating contributions from multiple developers without overwriting one another's changes.
- Backup and Restore: Because the entire history of the project is stored locally, users can easily revert to previous versions or recover lost work.
- Efficient File Management: Git efficiently manages large files and repositories, ensuring swift performance even with substantial data.
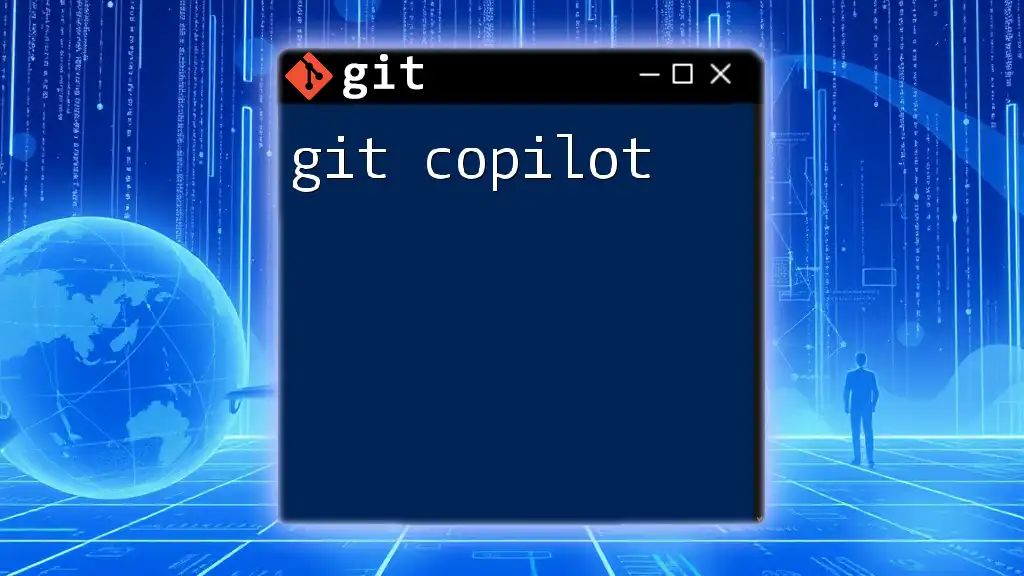
Getting Started with Git
Installing Git
To use Git, it needs to be installed on your machine.
For Windows:
- Download the installer from the [Git official website](https://git-scm.com/downloads).
- Follow the installation prompts and choose the default options unless custom settings are desired.
For macOS:
You can install Git using Homebrew. Open your terminal and run:
brew install git
For Linux:
Git can typically be installed through your package manager.
sudo apt-get install git # For Debian-based systems
sudo yum install git # For Red Hat-based systems
Configuring Git
Once Git is installed, it’s essential to configure it with your user information. Set your name and email, as they will appear in your commit messages.
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
To see the current configuration, use:
git config --list
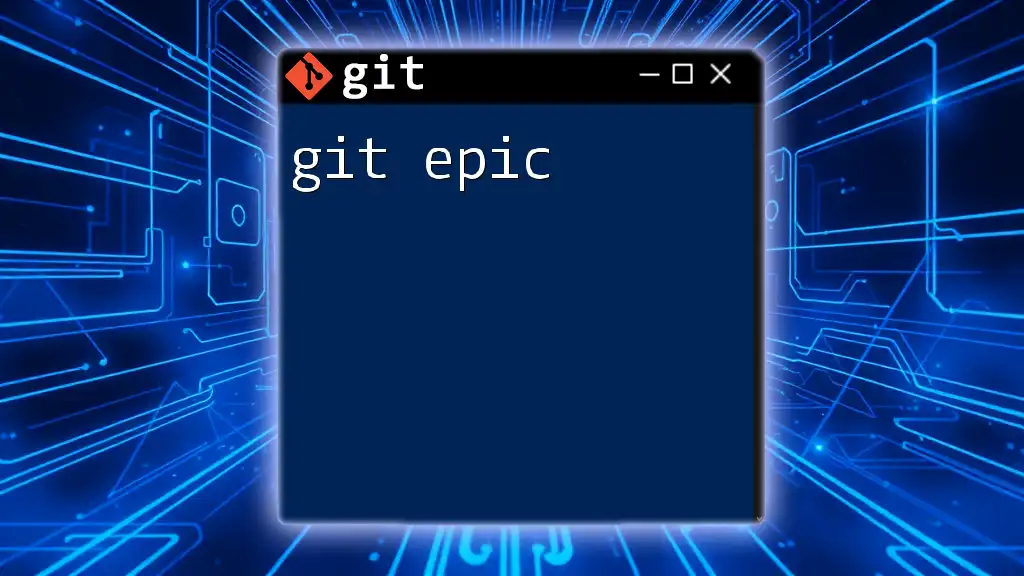
Basic Git Commands
Creating a Repository
To start working with Git, you'll need to create a repository.
Local Repository Creation: You can initialize a new Git repository by running this command:
git init my-repo
Cloning an Existing Repository: If you want to work on an existing project, you can clone a repository with:
git clone https://github.com/username/repo.git
Managing Changes
Adding Changes
To prepare changes for a commit, you need to stage them. You can stage specific files or all changes:
git add filename
git add .
Committing Changes
After staging changes, you should commit them with a descriptive message to document your changes:
git commit -m "Your message here"
Checking Status and Log
You can review the current status of your repository to see which files are staged, unstaged, or untracked with:
git status
To view your commit history and track what has changed, use:
git log
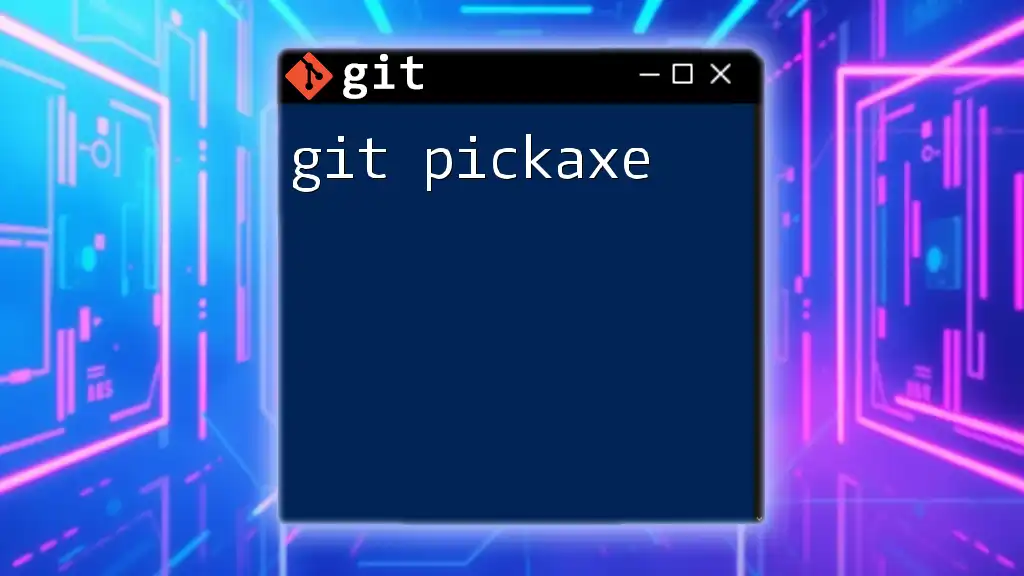
Branching and Merging
What are Branches?
Branches in Git allow you to diverge from the main line of development. This feature is especially helpful for working on new features or bug fixes without disrupting the stable codebase.
Creating and Switching Branches
Creating a new branch can be done with:
git branch new-branch
To switch to this new branch, use:
git checkout new-branch
Merging Branches
Once your work on a branch is complete, you can merge it back into the main branch. To do this, first switch to the branch you want to merge into (typically `main` or `master`), and then run:
git merge another-branch
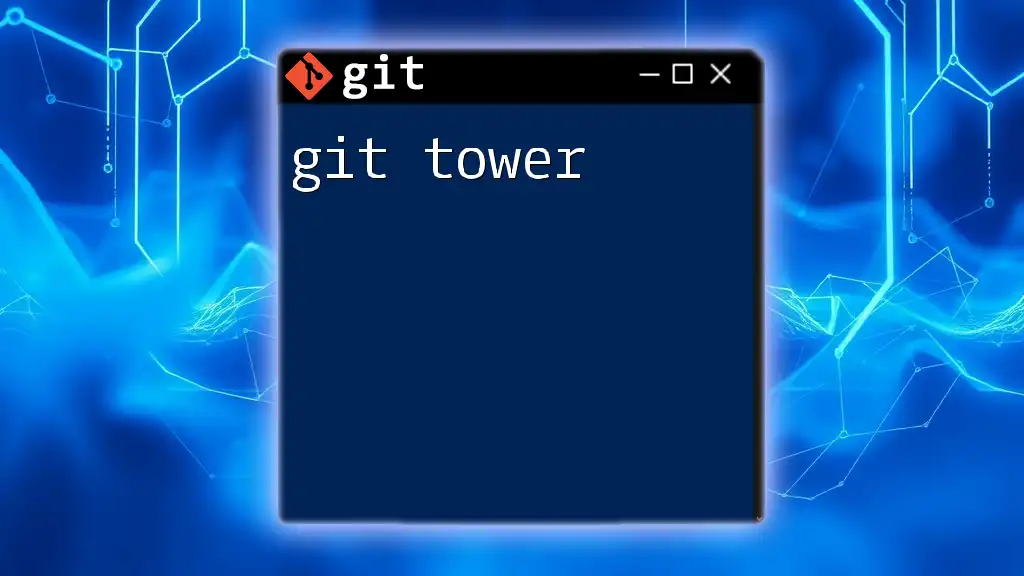
Resolving Merge Conflicts
Understanding Merge Conflicts
Merge conflicts occur when two branches make changes to the same line in a file or when one branch deletes a file that another branch is trying to modify. Resolving these conflicts is crucial for maintaining a clean project history.
Steps to Resolve Conflicts
- Identify the files with conflicts indicated in your terminal.
- Open each conflicted file and look for conflict markers (`<<<<<<<`, `=======`, `>>>>>>>`). Edit the files to resolve discrepancies between the versions.
- After resolving conflicts, mark the files as resolved with:
git add resolved-file
- Finally, complete the merge process by committing:
git commit -m "Resolved merge conflict"
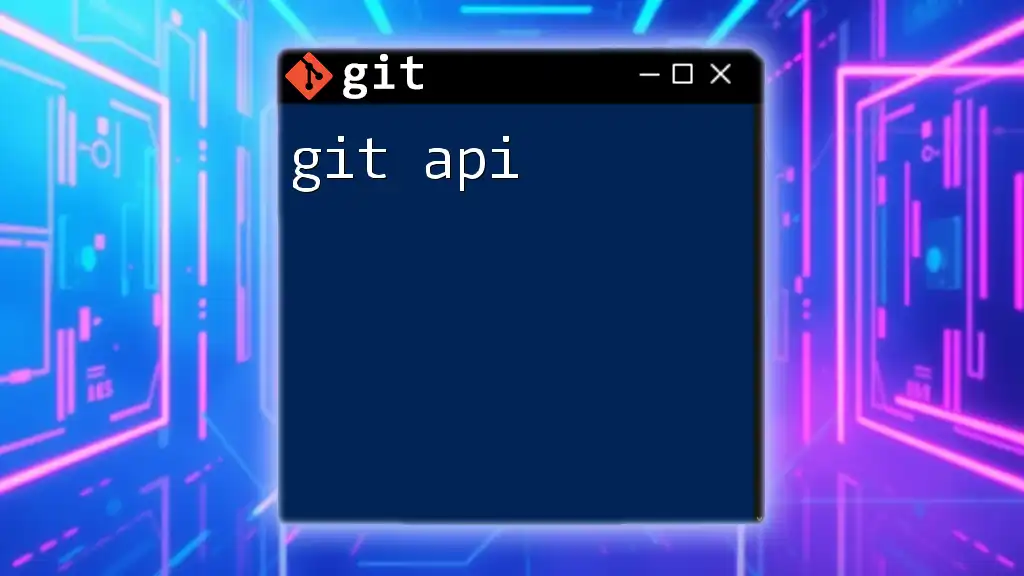
Remote Repositories
Pushing Changes
Remote repositories, like those hosted on GitHub or GitLab, allow for collaboration with others. To share your local changes, you can push them to the remote repository:
git push origin main
Pulling Changes
To update your local repository with the latest changes from a remote, you can pull those updates:
git pull origin main
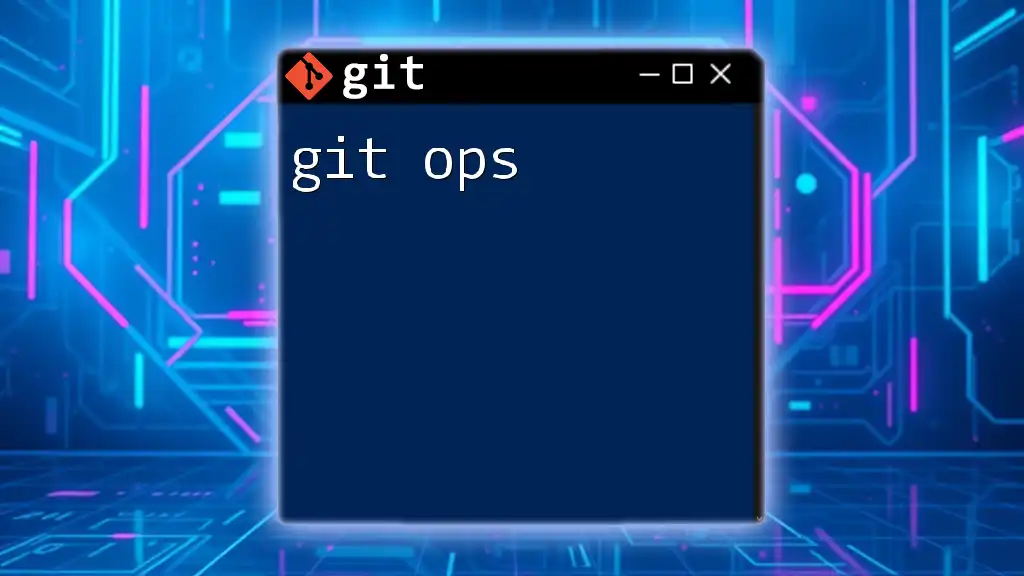
Advanced Git Commands
Stashing Changes
When you want to save your work temporarily without committing it, you can stash your changes. This is particularly useful when you need to switch branches but aren't ready to commit yet:
git stash
To apply stashed changes back to your working directory, use:
git stash apply
Rebasing
Rebasing allows you to move or combine a sequence of commits to a new base commit. This is primarily used in feature branches to maintain a linear project history. To rebase a feature branch onto the main branch, you can run:
git rebase main
Undoing Changes
Git enables you to undo changes effectively. Here are some key commands:
- To discard changes in the working directory:
git checkout -- filename
- To unstage files:
git reset filename
- To revert a specific commit:
git revert commit-hash
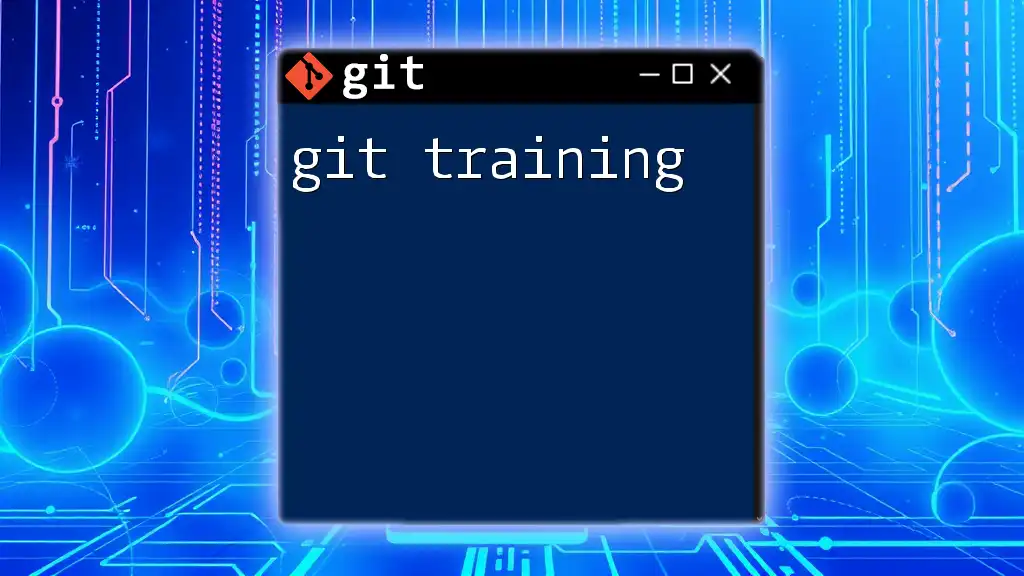
Conclusion
Mastering Git is an invaluable skill for any developer or team. By understanding the fundamental commands and operations, you can efficiently manage your code, collaborate with others, and maintain a clear project history. As you continue to practice using Git, make sure to explore its advanced features, and don't hesitate to seek out additional resources and communities to further your understanding. Through continual learning, you can leverage the full power of Git to enhance your development workflow and productivity.
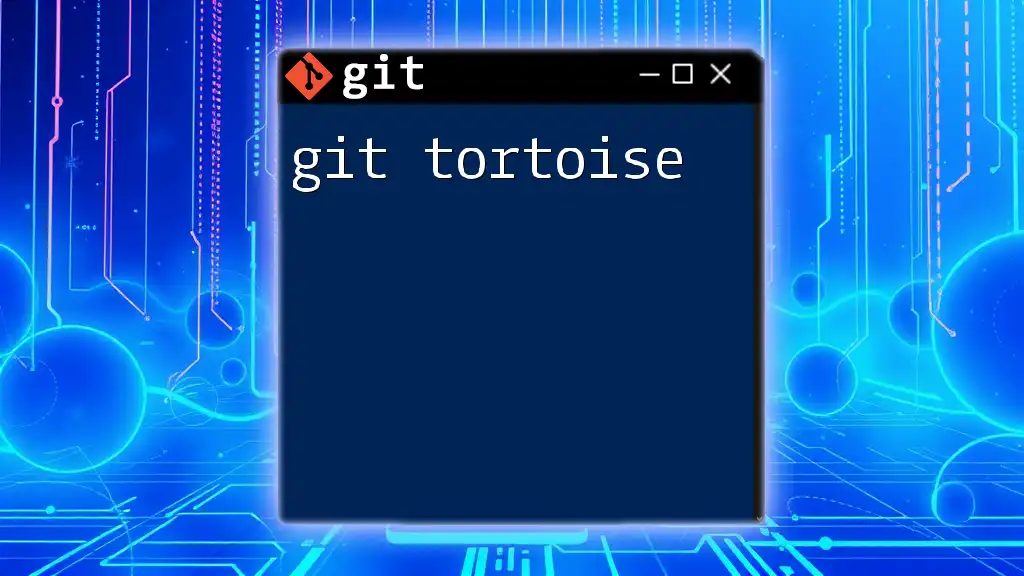
Additional Resources
For those looking to dive deeper into Git, refer to the [official Git documentation](https://git-scm.com/doc) for comprehensive guides. Furthermore, platforms like GitHub and GitLab often provide tutorials, while community forums offer assistance and networking opportunities for developers at any level.