"Git TypeScript" refers to using Git in a TypeScript project to manage version control efficiently; for example, to clone a repository containing TypeScript code, you can use the following command:
git clone https://github.com/username/repository-name.git
What is Git?
Git is a distributed version control system that helps developers manage changes to source code over time. Its primary purpose is to enable teams to collaborate on projects effectively, allowing multiple developers to work on the same codebase without stepping on each other's toes. Through features such as branching, merging, and version history, Git provides a robust framework for keeping track of code changes and facilitating collaboration.
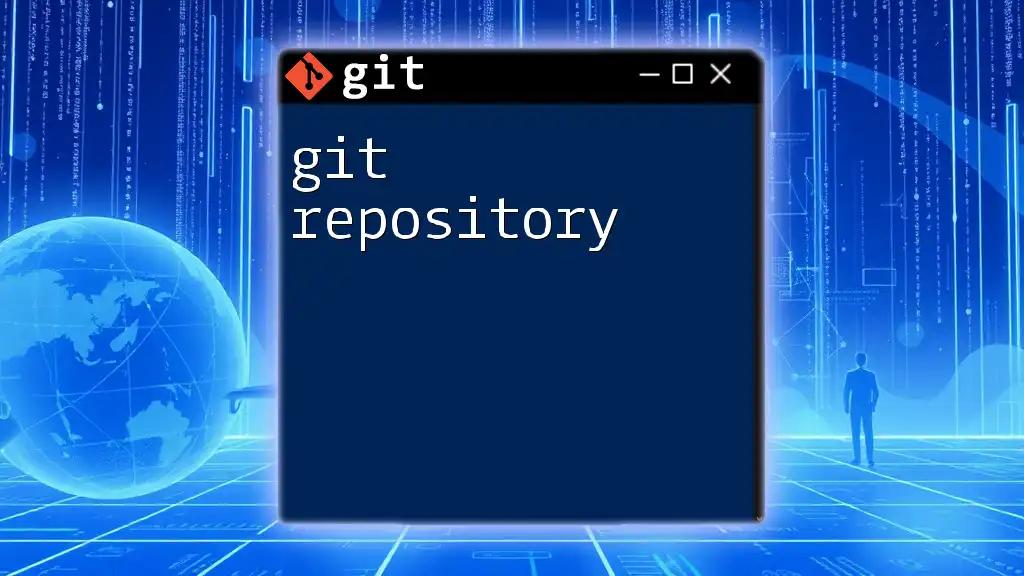
What is TypeScript?
TypeScript is a superset of JavaScript that adds type annotations to the language, allowing for static type checking. Developed by Microsoft, TypeScript aims to improve the development process by offering better Tooling and code quality. Its features, such as optional static typing, interfaces, and classes, provide stronger tooling support when compared to JavaScript. This leads to a more predictable and manageable codebase, ultimately resulting in fewer runtime errors and improved maintainability.
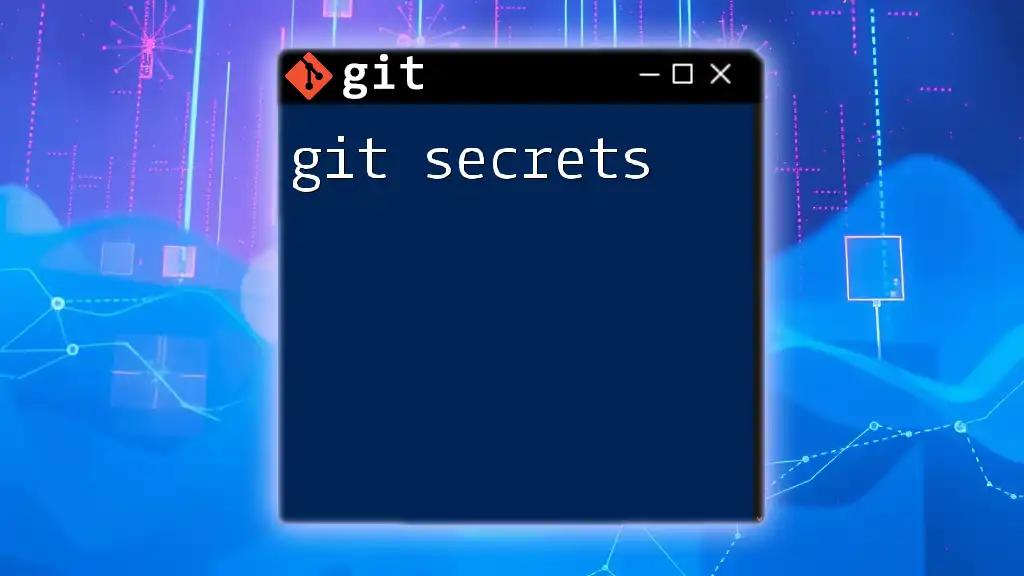
Why Use Git with TypeScript?
Integrating git with typescript projects brings numerous advantages:
- Version Control: Manage different versions of TypeScript files seamlessly.
- Collaboration: Multiple developers can work on the same project without conflicts.
- Simplified Rollbacks: Easily revert to previous versions or changes.
- Branch Flexibility: Experiment with new features without affecting the main codebase.
Using Git with TypeScript enhances the overall development workflow, making it easier to manage complex applications.
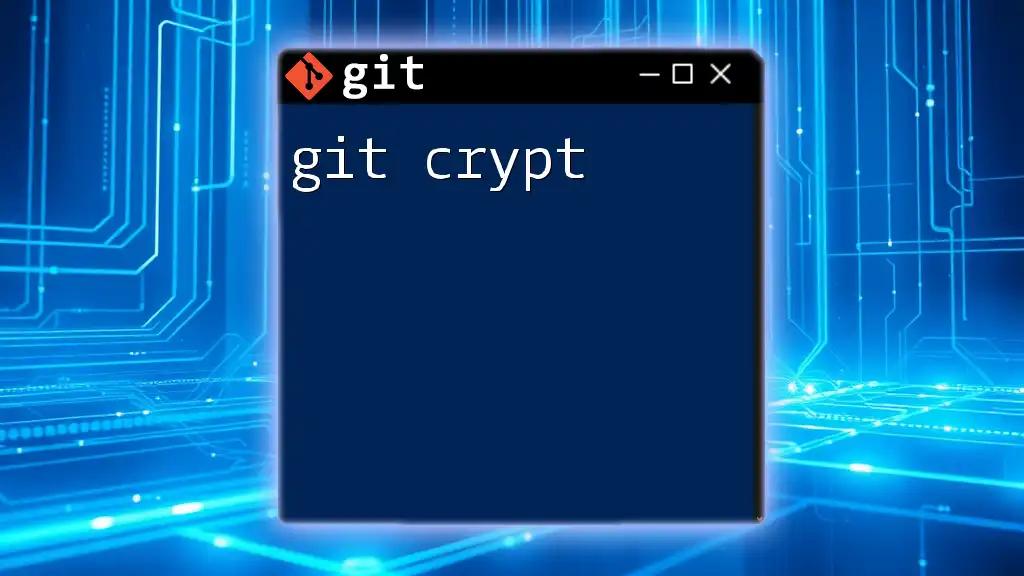
Setting Up Your Environment
Installing Git
Before diving into your TypeScript project, you need to install Git on your system. Here’s how to do it across different platforms:
-
Windows: Download the installer from the [official Git website](https://git-scm.com/download/win) and follow the installation instructions.
-
macOS: You can install Git via Homebrew with the command:
brew install git
-
Linux: Use your distribution's package manager. For example, on Ubuntu, run:
sudo apt-get install git
Installing TypeScript
To get started with TypeScript, you’ll need Node.js and npm (Node Package Manager) installed. Once you have them, install TypeScript globally by running:
npm install -g typescript
This command will make the TypeScript compiler available across your system.
Configuring Your Git Repository for TypeScript
Now that you have Git and TypeScript set up, you can initialize your Git repository for your new TypeScript project. Here’s how:
-
Create a new directory for your project:
mkdir my-typescript-project
-
Navigate into the directory:
cd my-typescript-project
-
Initialize a new Git repository:
git init
At this point, you have a blank Git repository ready to manage your TypeScript files.
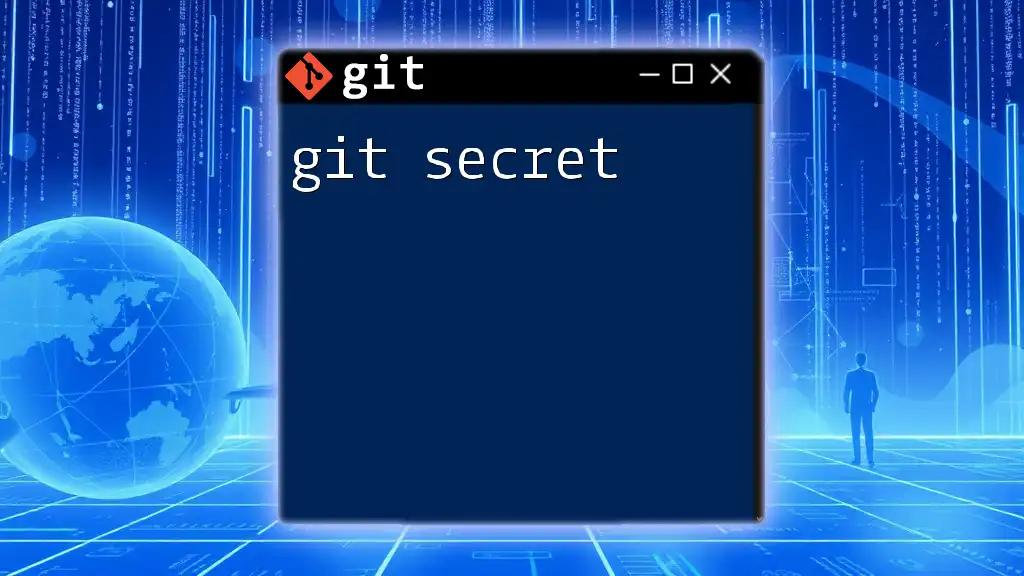
Structuring a TypeScript Project
Setting Up TypeScript Configuration
A well-structured TypeScript project should include a `tsconfig.json` file, which contains compiler options and file paths. This file is vital for configuring how TypeScript compiles your code. You can create a basic `tsconfig.json` as follows:
{
"compilerOptions": {
"target": "es5",
"module": "commonjs",
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true,
"forceConsistentCasingInFileNames": true
},
"include": ["src/**/*"],
"exclude": ["node_modules", "**/*.spec.ts"]
}
Organizing Your Project Files
A clean directory structure is essential for maintainability. Here’s a recommended structure for your TypeScript project:
my-typescript-project
├── src
│ ├── index.ts
│ └── utils.ts
├── tests
├── node_modules
└── package.json
- src/: Contains your TypeScript source files.
- tests/: Holds your test files.
- node_modules/: Where your packages reside.
- package.json: Manages project dependencies and scripts.
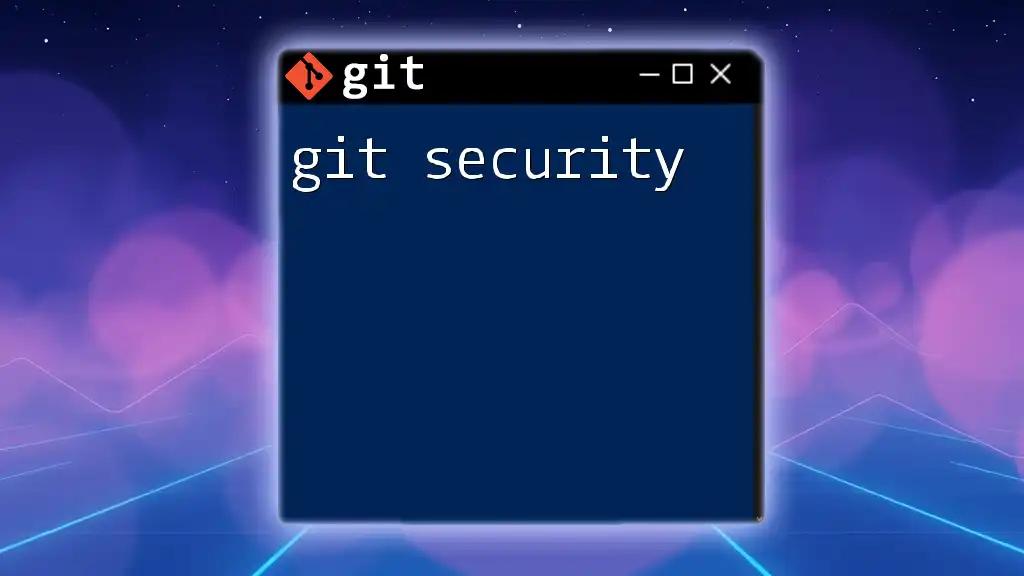
Common Git Commands for TypeScript Projects
Basic Commands
Understanding the fundamental Git commands is crucial for managing your code:
-
git init: Initializes a new repository.
-
git add: Stages files for commit. For a TypeScript project, you can stage your files like this:
git add src/index.ts
-
git commit: Commits staged changes to your repository. Always write meaningful commit messages. For instance:
git commit -m "Add initial TypeScript setup"
Branching and Merging
Branches allow you to develop features in isolation. Here’s how to create and merge branches:
-
Creating a new branch:
git checkout -b feature/new-feature
-
Merging branches involves switching to your main branch and merging the changes:
git checkout main git merge feature/new-feature
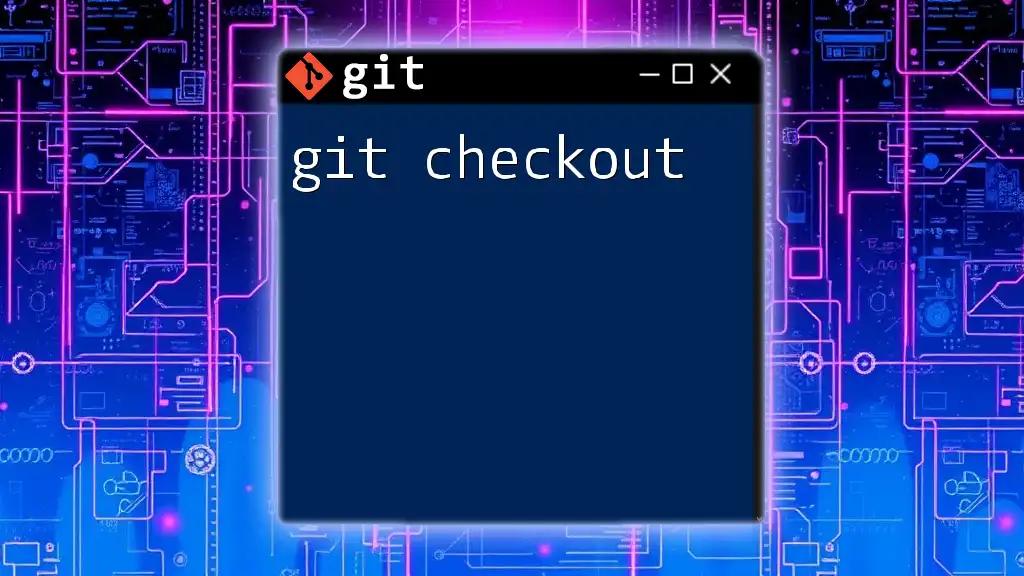
Advanced Git Features for TypeScript Development
Stashing Changes
When you're in the middle of work and need to switch contexts, you can stash your changes. This command helps you save your work without committing it:
git stash push -m "WIP on type conversion"
Later, you can retrieve your stashed changes using:
git stash apply
Resolving Merge Conflicts
Merge conflicts might occur when changes from different branches overlap. Here’s how to handle them effectively:
-
After a failed merge, run:
git status
This will show you which files have conflicts.
-
Open the conflicted files and look for markers indicating the conflicting code.
-
Decide how to resolve the conflict, then stage the resolved files:
git add src/index.ts
-
Commit your resolution:
git commit -m "Resolved merge conflict in index.ts"
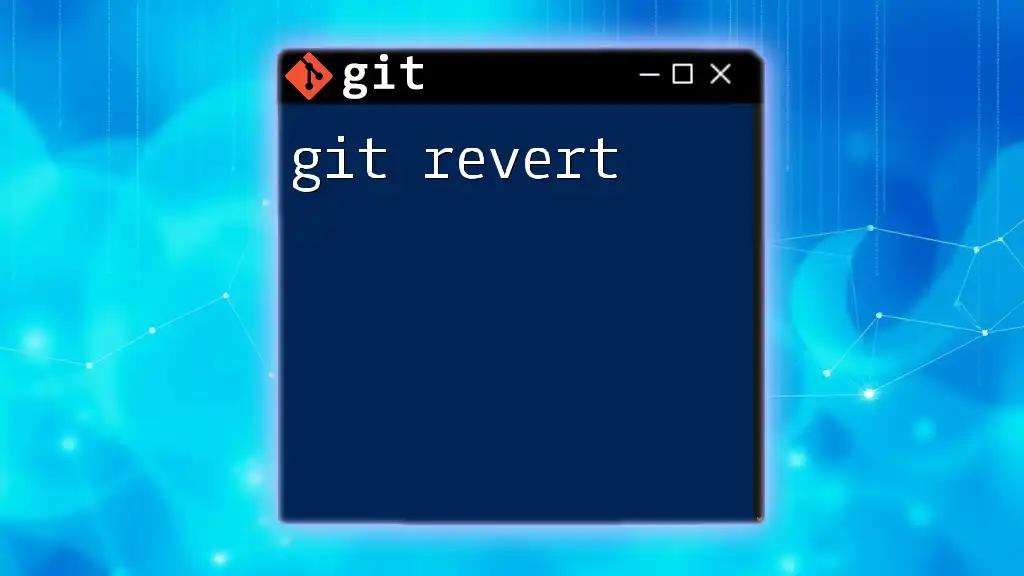
Managing TypeScript Dependencies with Git
Keeping Your Package.json File Up-to-Date
As your TypeScript project grows, managing dependencies becomes crucial. To add TypeScript-related packages, you can use npm. For example, to install TypeScript and the Node.js type definitions, run:
npm install --save-dev typescript @types/node
Version Control for Node_modules
It’s common practice to ignore the `node_modules` directory when using Git because it can be regenerated from the `package.json` file. Create a `.gitignore` file in your project root and add the following lines:
node_modules
dist
This ensures that unnecessary files are not tracked in your repository.
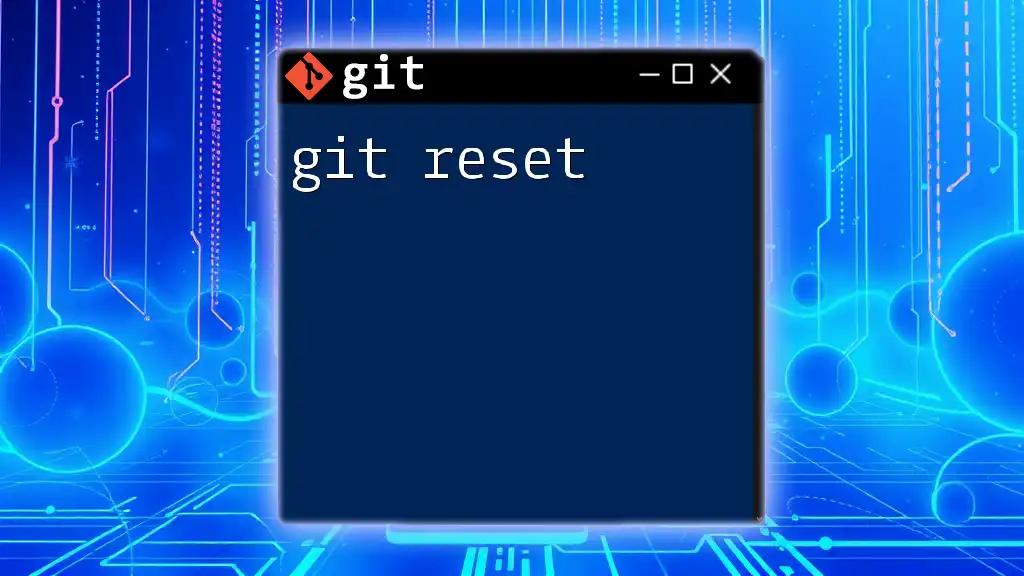
Conclusion
Integrating git with typescript enhances your development process, offering an organized way to manage code changes, collaborate with team members, and maintain a clean codebase. By understanding the fundamental commands and best practices outlined in this guide, you'll be well-equipped to leverage both Git and TypeScript efficiently in your projects.
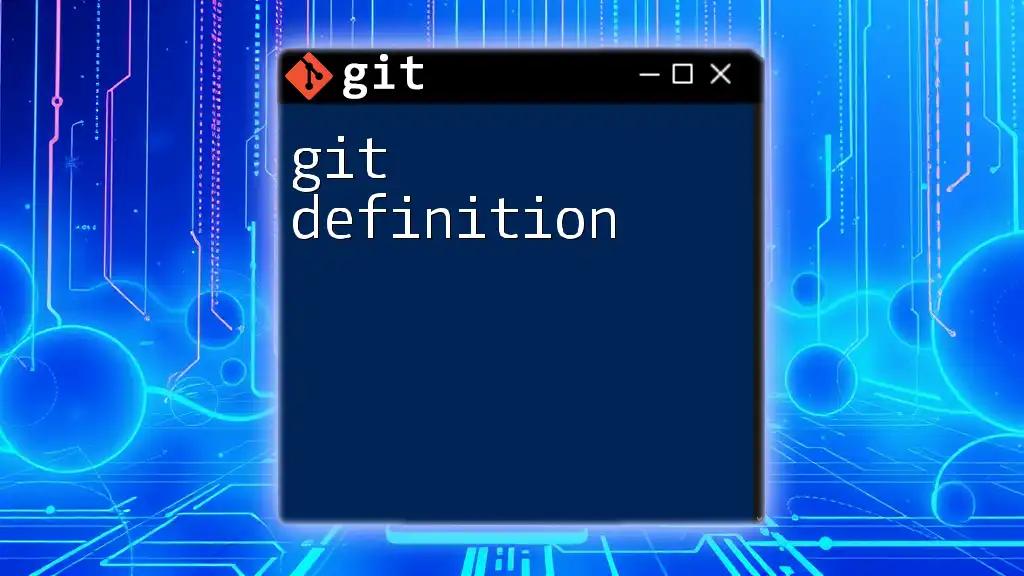
Additional Resources
- For in-depth guidance, refer to the [official Git documentation](https://git-scm.com/doc) and [TypeScript documentation](https://www.typescriptlang.org/docs/).
- Consider exploring GitHub repositories that showcase TypeScript projects for practical examples.
- Engage with online communities dedicated to Git and TypeScript development for continued learning and networking.