The command `git test` is not a standard Git command, but if you're referring to testing changes in a Git repository, you can use `git diff` to see the differences between your working directory and the last committed state.
Here's the command:
git diff
Understanding Git Testing
What is Testing in Git?
Testing in the context of version control encompasses a variety of practices aimed at ensuring code quality and functionality before deployment. In Git, testing plays a crucial role in confirming that every change made does not introduce errors or conflicts. Testing allows developers to verify their code's correctness and maintain the integrity of the main branch, especially in collaborative environments.
Types of Tests in Git
Unit Testing
Unit tests are designed to test the smallest pieces of code, typically individual functions or methods, in isolation. The primary goal is to validate that each unit of the software performs as expected. For instance, consider a simple function in JavaScript that adds two numbers:
function add(a, b) {
return a + b;
}
test('adds 1 + 2 to equal 3', () => {
expect(add(1, 2)).toBe(3);
});
In this example, the test checks that the `add` function returns the correct output when passed specific inputs.
Integration Testing
Integration tests assess the interactions between multiple components or systems to check their combined functionality. Unlike unit tests, which test components in isolation, integration tests focus on how these components work together. For example, if you were building a web application, an integration test might verify that a user can log in and retrieve their profile data from a database.
End-to-End Testing
End-to-end (E2E) testing evaluates the entire application flow, simulating user behaviors to ensure everything functions as intended. These tests cover various components from the frontend to backend services. One popular framework for E2E testing is Cypress, which allows developers to write tests that interact directly with the application as users would.
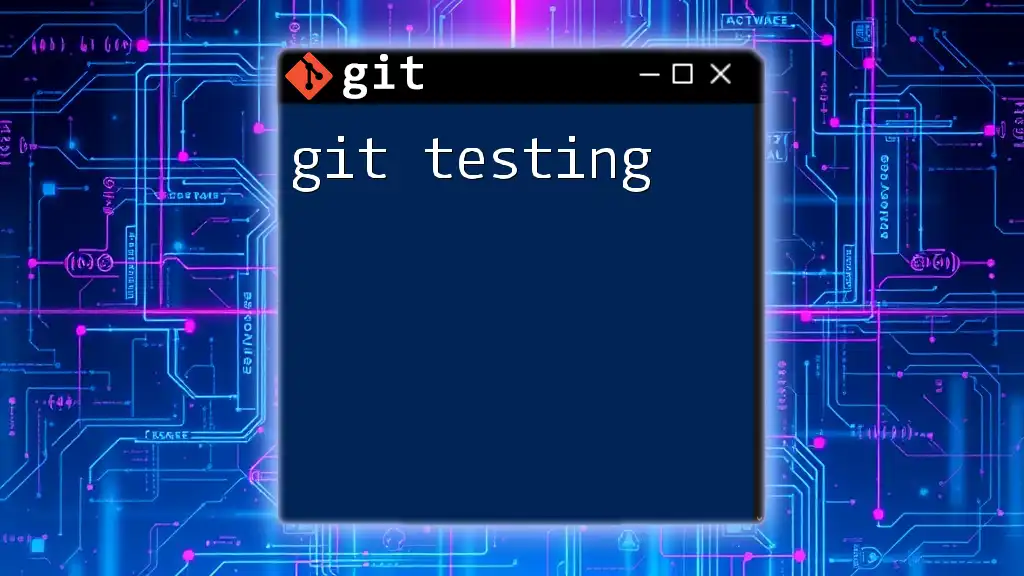
Setting Up a Testing Environment in Git
Prerequisites
Before you can begin writing tests, you'll need several tools and dependencies. Commonly used libraries include Jest for JavaScript testing and Mocha for more general applications. Ensure that Node.js and npm are installed on your system as they provide the foundation for these libraries.
Configuring Your Repository
Creating a new repository and setting up the testing frameworks involves the following steps. Open your terminal and run:
git init my-test-repo
cd my-test-repo
npm init -y
npm install --save-dev jest
This sequence initializes your Git repository and installs Jest as a development dependency.
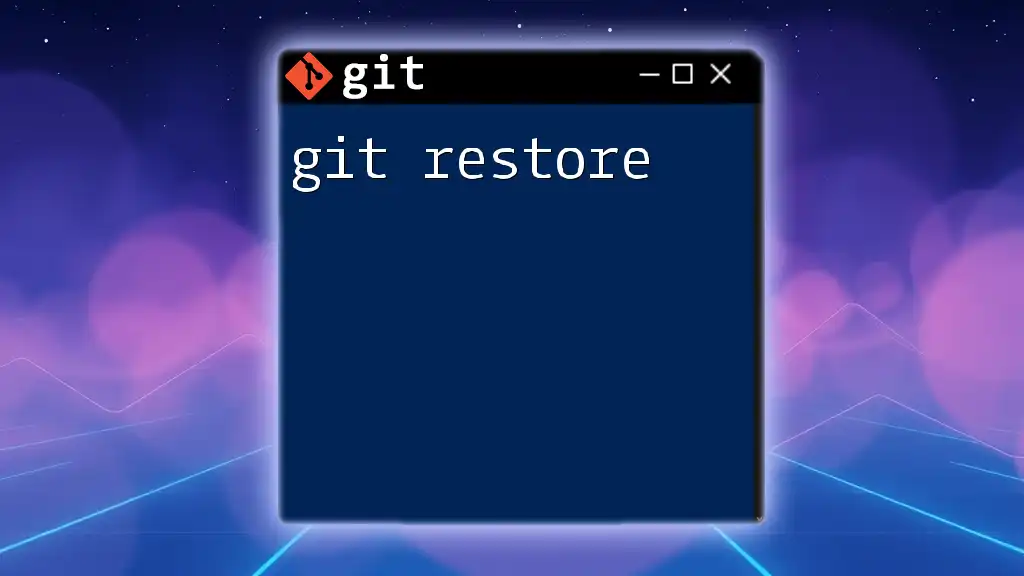
Writing and Running Tests with Git
Writing Tests
Effective tests are foundational for maintaining code quality. When writing tests, adhere to the following best practices:
- Descriptive Names: Ensure your test names clearly communicate their purpose.
- Organized Structure: Place tests in dedicated directories (often a `tests` or `tests` folder) to avoid clutter and enhance navigation.
By maintaining a clean and structured test codebase, you facilitate easier collaboration among team members.
Running Tests
Executing tests is straightforward with Git and your chosen testing framework. To run Jest tests, simply use the command:
npx jest
This command will detect all test files and execute them, giving you immediate feedback on their success or failure.
Interpreting Test Results
When you run your tests, you'll receive output that indicates whether they passed or failed. Green indicates success, while red signals failure. Troubleshooting these failed tests is essential; common strategies include reviewing error messages, checking the test setup, and ensuring all dependencies are correctly defined.
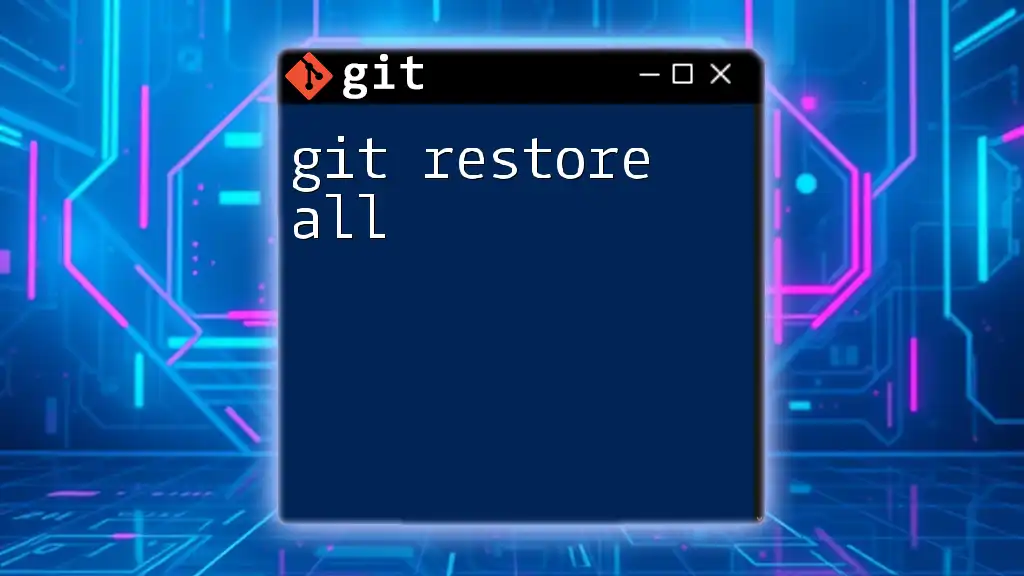
Version Control and Testing with Git
Committing Tests
When you write tests, best practices dictate that you should commit them consistently along with the code changes. This approach—not only demonstrates the development process but also ensures the tests are directly tied to the code they validate. Aim to make atomic commits, focusing on one change at a time.
Creating and Managing Test Branches
Branching is a significant feature of Git that allows you to develop features or fix bugs in isolation. For testing, you can create a dedicated branch. Use the following commands to create and switch to a new branch:
git checkout -b testing
This command creates a new branch named `testing` and switches your working directory to it. From here, you can implement your changes, write tests, and experiment without affecting the main branch.
Merging Test Branches
Once you've confirmed that your tests succeed in the testing branch, it's time to merge them back into the main branch. Merging ensures your tests and the corresponding code changes become a part of the main codebase. Always remember to create a pull request for code reviews, making collaboration smoother.
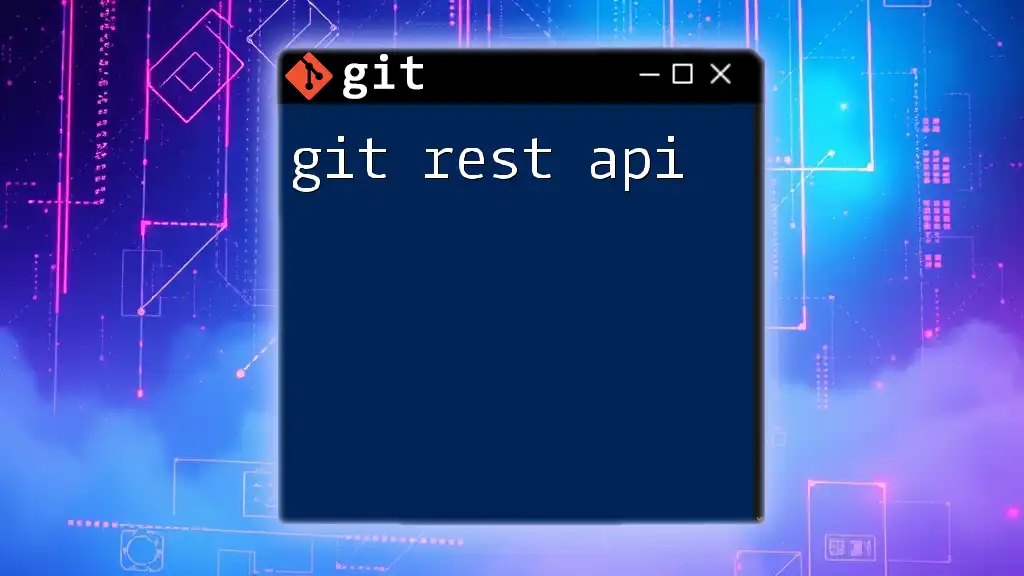
Advanced Testing Techniques in Git
Continuous Integration/Continuous Deployment (CI/CD)
CI/CD processes leverage Git testing by automating the testing workflow. By integrating tools such as GitHub Actions or Travis CI, you can set up automation that runs tests on every push, ensuring that your code remains reliable and deployable. Implementing CI/CD allows your team to save time and improve workflow efficiency.
Automated Testing with Git Hooks
Git hooks are scripts that run automatically at specific points in your Git workflow. One of the most useful hooks is the pre-commit hook, which allows you to run tests before any code is committed. For instance, you can add a script to `.git/hooks/pre-commit`:
#!/bin/sh
npm test
This script ensures that any tests must pass before a commit can be made, increasing code quality and minimizing the chances of errors being introduced into the codebase.
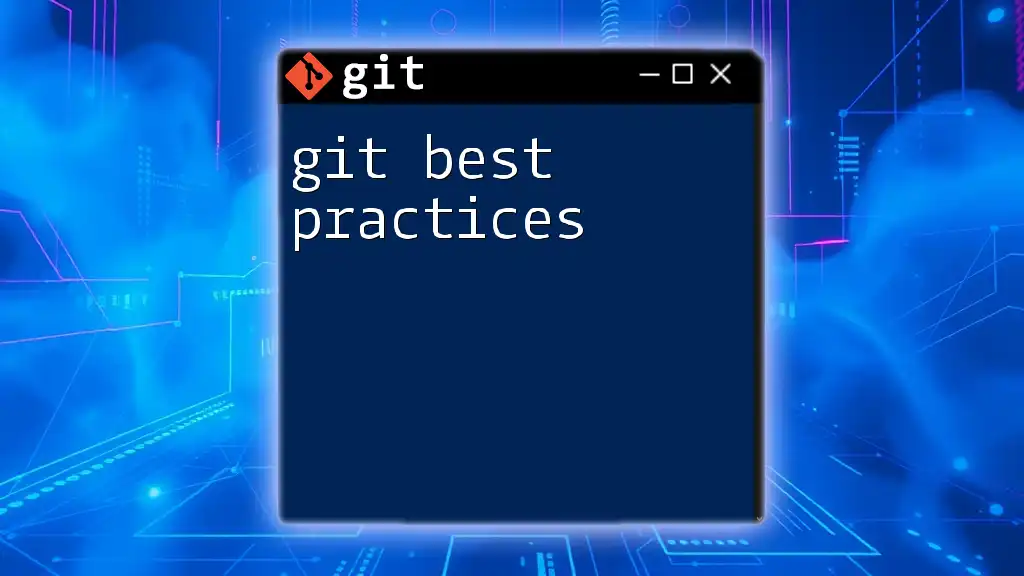
Conclusion
In the increasingly collaborative world of software development, testing your code within Git is not just a best practice but a necessity. By incorporating a robust testing framework and leveraging the full capabilities of Git, you can maintain high code quality, ensure smooth collaboration, and enhance productivity. Embrace these practices and drive your development processes forward—there's always more to learn, and I encourage you to seek out further resources and community support to sharpen your skills.
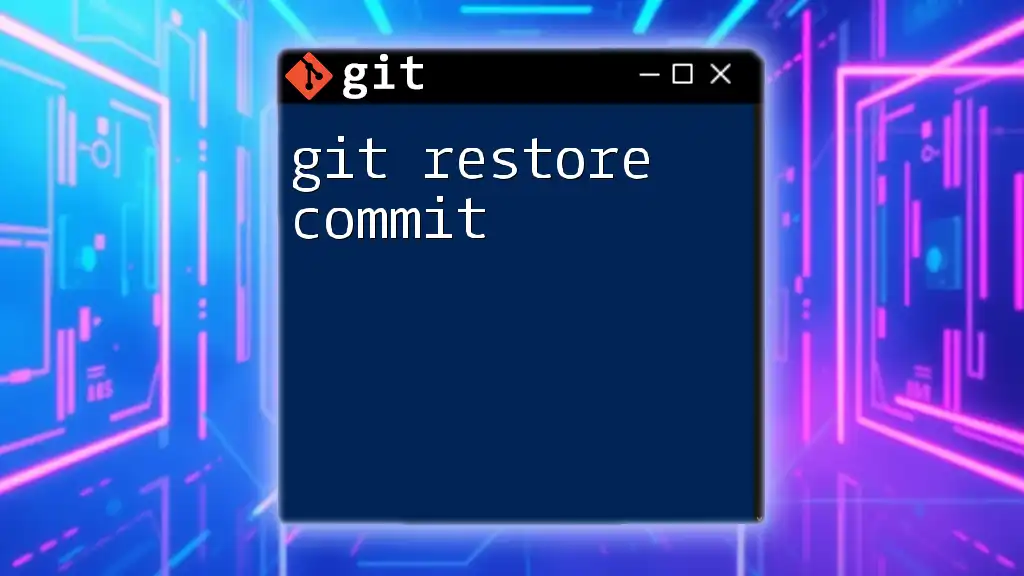
Additional Resources
For those looking to deepen their understanding of Git testing and best practices, consider exploring Git’s official documentation, online tutorials, and educational courses. Connecting with communities and joining forums can also prove immensely valuable as you grow your skills in this vital aspect of software development.