To undo a `git restore`, you can use `git checkout` to retrieve the last committed version of the file before the restore was executed. Here's the command you can use:
git checkout -- <filename>
Understanding `git restore`
What is `git restore`?
The `git restore` command is a powerful tool introduced in Git 2.23 that simplifies the process of restoring files. Its primary purpose is to discard changes made in the working directory or to move changes from the staging area back to the working directory. Unlike `git checkout`, which serves multiple purposes, `git restore` is specifically focused on the restoration of files, making it easier to understand and use.
Use Cases of `git restore`
The `git restore` command is used in various scenarios, such as:
- Discarding Local Changes: If you've made changes to a file and decide you want to discard those changes, `git restore` allows you to revert it to its last committed state.
- Restoring Staged Changes: If you've staged some files but realize you need to make additional changes before committing, you can unstage them using this command.
- Recovering from Mistakes: Emotions can run high in coding, and sometimes you might hastily change a file. `git restore` can help you revert those changes easily without affecting the commit history.
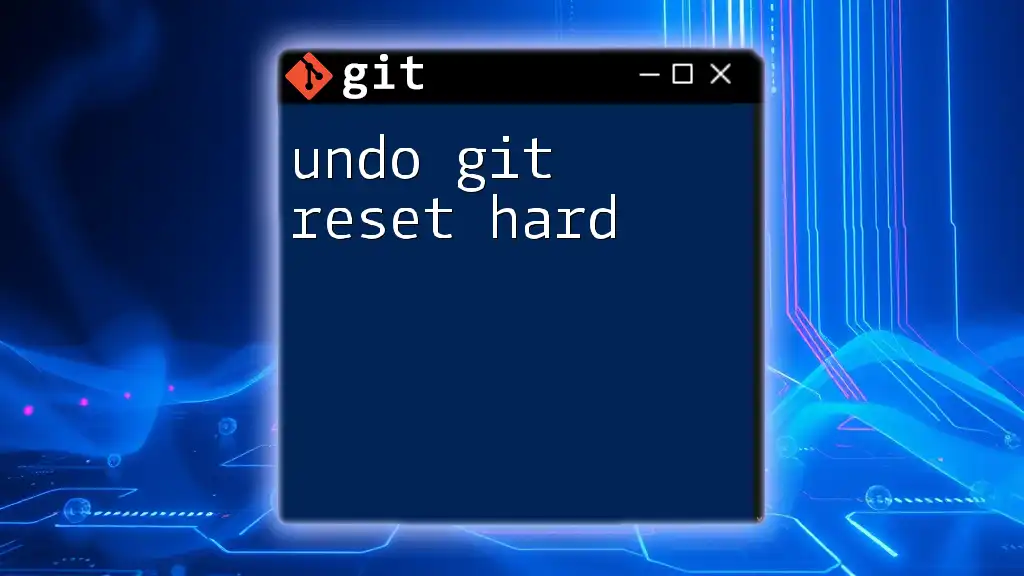
How `git restore` Works
Syntax and Parameters
The syntax of the `git restore` command is straightforward:
git restore [options] [--source <commit>] [--staged] [pathspec...]
- [options]: Various flags that influence how the command executes (for example, `--source`).
- [--source <commit>]: Specifies the commit from which to restore the file.
- [--staged]: Refers to the staging area, allowing you to unstage files.
- [pathspec...]: Specifies the files you want to restore.
Typical Scenarios of Restoration
When you're using `git restore`, some common operations include:
- Restoring files from the working directory to its last committed state.
- Moving files from the staging area back into the working directory.
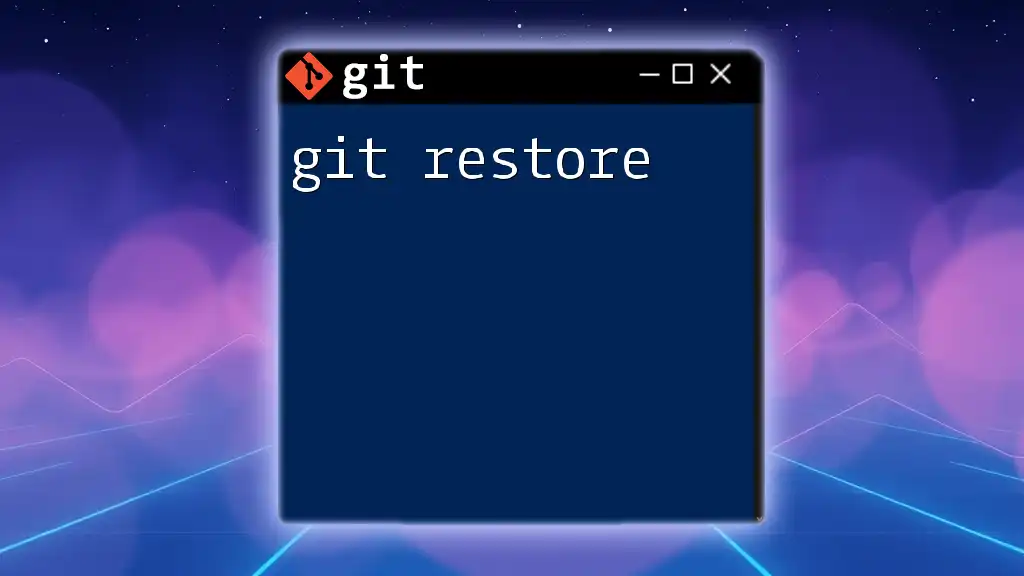
Understanding the Consequences of Using `git restore`
What Happens When You Run `git restore`?
Running `git restore` can modify your working directory. Specifically, it resets the changes of the specified file(s) to match the last commit. If you’ve made changes to a file that you no longer want, using this command will overwrite those changes. It is essential to recognize that any unsaved alterations will be lost if not backed up.
Misconceptions About `git restore`
It's important to clarify that many believe `git restore` permanently deletes changes without the possibility of recovery. While executing this command does modify files, Git offers mechanisms like `reflog` and access to past commits to recover lost work. Therefore, understanding the command's function and implications is crucial before using it.
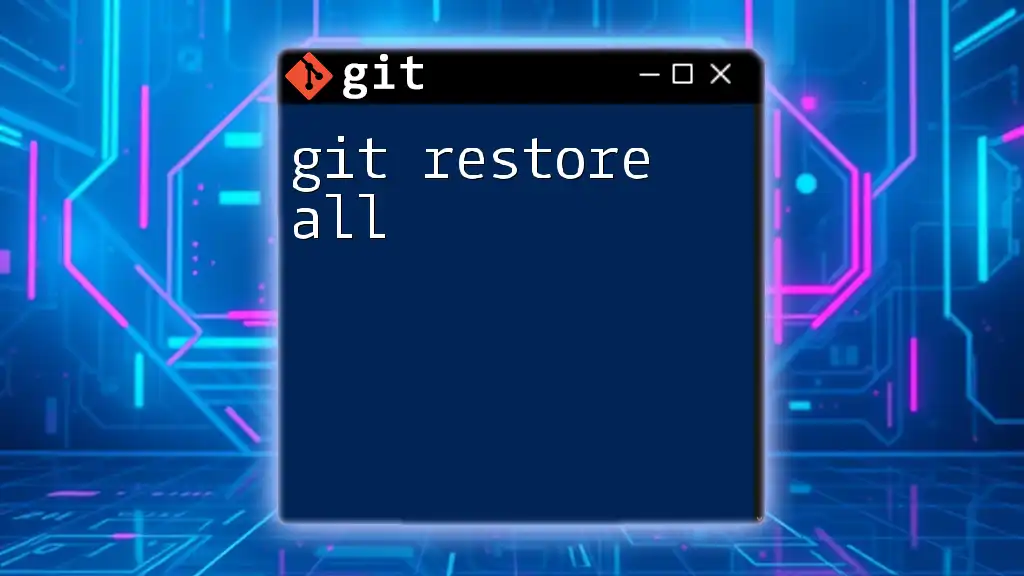
What to Do After Running `git restore`
How to Identify the Impact
After running `git restore`, you might want to verify what changes have occurred. Use the following command to check the status:
git status
This command will display which files have been modified, staged, or restored. Thus, you can confirm if the execution of `git restore` was successful or if further action is necessary.
Using Git's reflog to Recover Changes
What is Git reflog?
The `git reflog` is a mechanism that logs all changes made to the repository, keeping track of where your branches and HEAD have pointed over time. This feature is immensely valuable as it enables you to revert to previous states even after the use of commands like `git restore`.
How to Use Git reflog
You can view your repository's history saved in `reflog` using:
git reflog
This command provides a chronological list of the recent actions you've performed, demonstrating commits, checkouts, and restores. By reviewing these logs, you can identify the desired state to which you can revert.
To recover a lost version, you can check out a specific commit referenced in the reflog:
git checkout HEAD@{n}
`{n}` represents the position in the reflog, allowing you to navigate back to the state you want to restore.
Alternative Recovery Methods
In addition to `reflog`, two notable commands can aid in recovery:
-
Using `git fsck`: This command verifies the integrity of your repository and can help identify dangling commits that may represent lost data.
-
Resorting to `git checkout`: Similar to `git restore`, this command can switch branches and restore files, albeit with broader functionality. For example:
git checkout -- <file>
This command effectively discards changes in the specified file, similar to what `git restore` accomplishes.
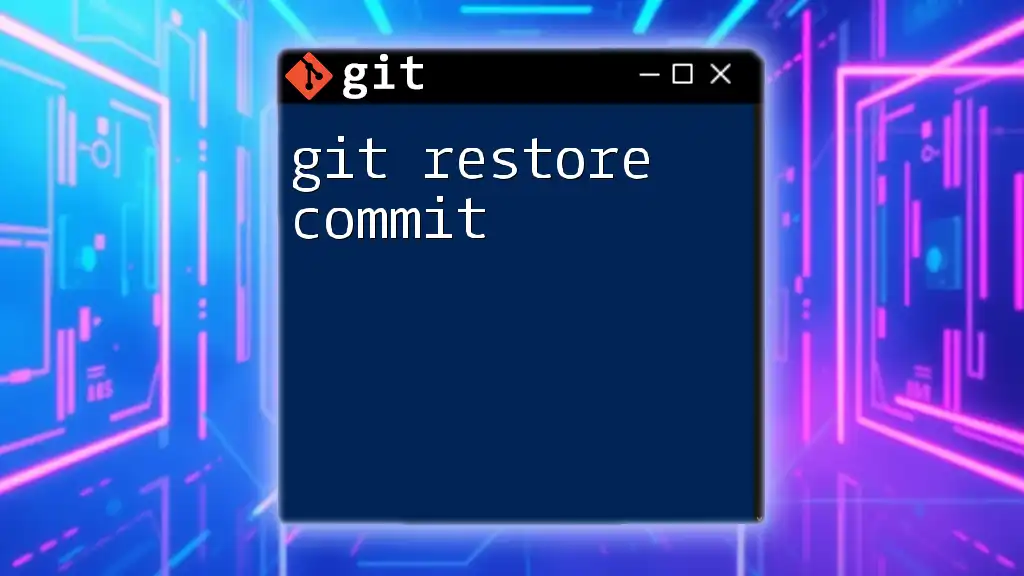
Practical Examples of Undoing `git restore`
Example 1: Undoing a Restore from Working Directory
Suppose you edited a file called `myfile.txt`, but you used `git restore myfile.txt` because the changes were misleading. Here’s how to recover your work:
-
Run `git reflog` to check the previous states.
-
Identify the entry before the restoration and note its reference.
-
Use the following command to check out that state:
git checkout HEAD@{n}
This will restore your previous state of `myfile.txt` prior to the unwanted restore.
Example 2: Undoing a Restore from Staging Area
If you staged changes in `myfile.txt` using:
git add myfile.txt
git restore --staged myfile.txt
and then regretted un-staging, follow these steps:
-
Run `git reflog` as mentioned.
-
Recognize the correct commit point.
-
Execute:
git checkout HEAD@{n}
This allows you to revert to the previously staged state of the file, ensuring those changes are reinstated.
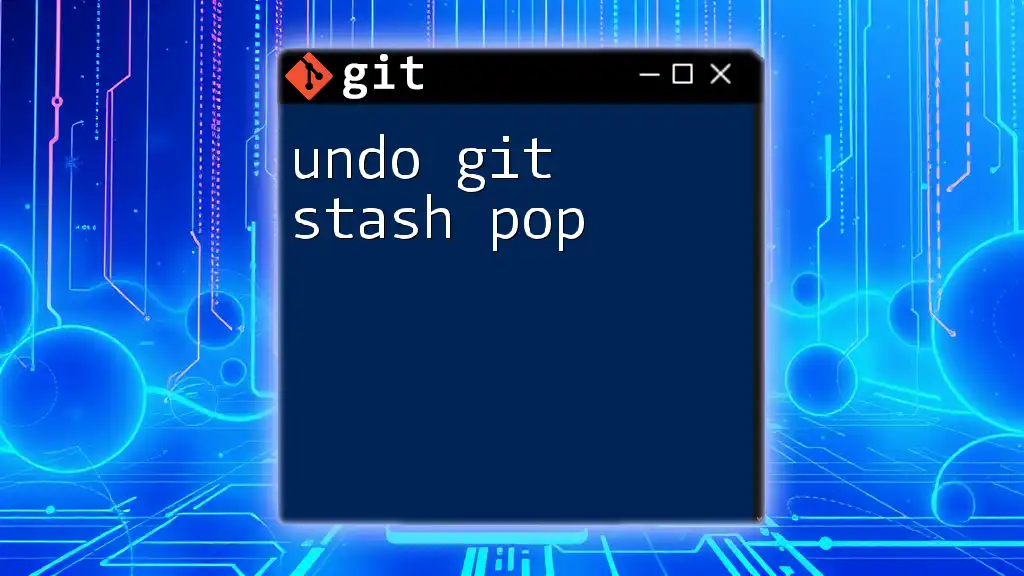
Best Practices for `git restore` and Data Recovery
Tips to Prevent Unintended Data Loss
To reduce the risk of accidental alterations and data loss, consider implementing these practices:
- Frequent Commits: Commit your work often to create check-points.
- Feature Branches: Use branches to isolate changes, reducing the likelihood of errors in your main branch.
Importance of Backup Strategies
Maintaining a robust backup strategy is necessary for effective source control management. Regularly push your changes to a remote repository or utilize services like GitHub or GitLab, ensuring you can recover your work even after drastic command executions.
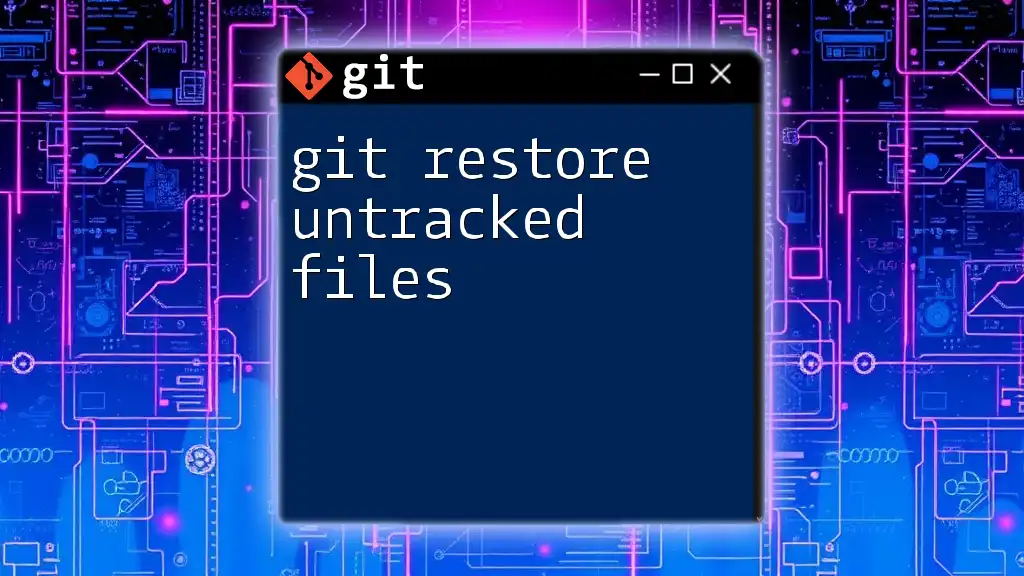
Conclusion
In summary, understanding how to undo `git restore` involves not just knowledge of the command itself but also awareness of Git's broader recovery mechanisms. By mastering this command and implementing best practices, you can navigate your projects more confidently, ensuring minimal disruption from unintentional changes. The journey to becoming proficient in Git commands can be simplified with just a bit of deliberate practice and understanding of the underlying processes.