To undo a git merge conflict and return to the state before the merge attempt, use the following command:
git merge --abort
Understanding Git Merge Conflicts
What is a Git Merge Conflict?
A Git merge conflict occurs when two branches being merged into one contain modifications to the same line of a file or when one branch modifies a file while another branch deletes it. The version control system is unable to automatically determine how to combine the changes, leading to a conflict that must be manually resolved.
When Does a Merge Conflict Occur?
One common scenario that leads to merge conflicts is when two developers edit the same line of code in a file. Imagine Developer A and Developer B working on separate branches, both deciding to change a greeting in a file called `hello.py`. Upon attempting to merge their changes, Git will identify the conflicting lines and generate a merge conflict.
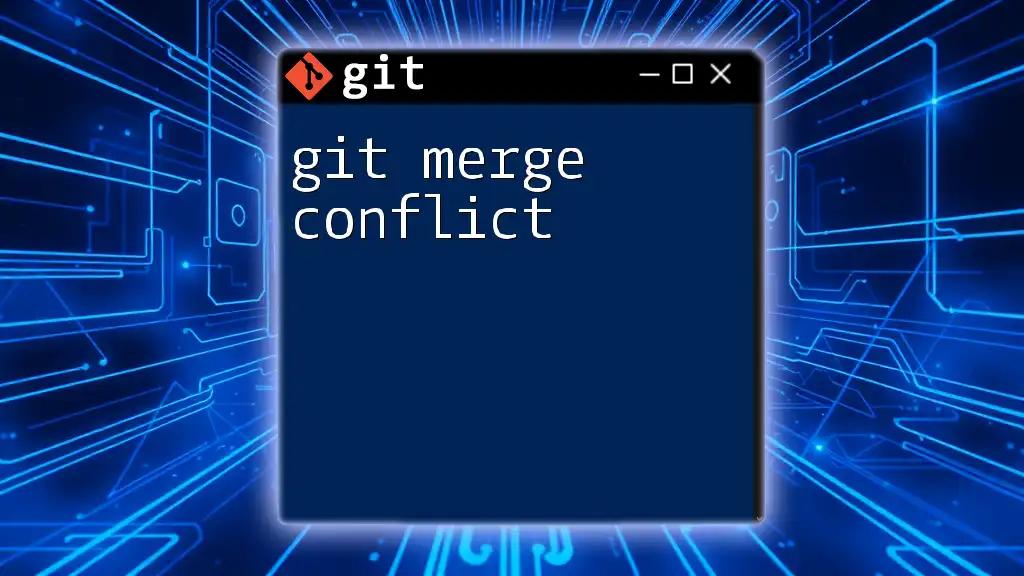
How to Identify a Merge Conflict
Viewing Conflict Markers
When a merge conflict occurs, Git places conflict markers within the affected files. These markers help identify the conflicting sections:
- `<<<<<<< HEAD`: This marks the beginning of the current branch's changes.
- `=======`: This separates the conflicting changes.
- `>>>>>>> [branch-name]`: This marks the end of the incoming branch's changes.
Here is an example of what file content may look like during a conflict:
def hello_world():
<<<<<<< HEAD
print("Hello from the main branch!")
=======
print("Hello from the feature branch!")
>>>>>>> feature-branch
Using Git Commands to Identify Conflicts
To determine which files have merge conflicts, you can use the following commands. First, check the status of your repository with:
git status
If conflicts exist, you will see a message indicating which files are unmerged. To examine the specific changes that led to the conflict, utilize:
git diff
This command will provide a detailed line-by-line comparison of the conflicting changes.
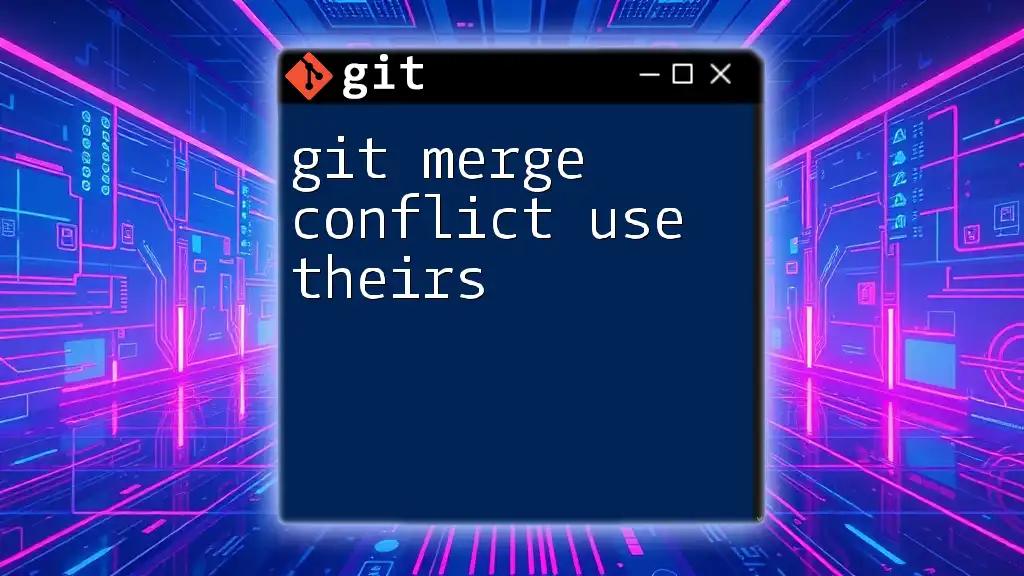
Options for Resolving a Merge Conflict
Manual Resolution
Resolving merge conflicts manually involves several key steps:
- Open the Conflicted File: Locate the files marked by Git and open them in your code editor.
- Edit and Remove Conflict Markers: Carefully resolve the conflict by choosing which changes to keep. Remove the conflict markers (`<<<<<<`, `========`, `>>>>>>`) after making your edits.
- Stage Changes: Once your edits are complete, add the resolved file to the staging area:
git add hello.py
- Complete the Merge: Finally, finalize the merge process by committing your changes:
git commit -m "Resolved merge conflict in hello.py"
Automatic Resolution using Git
Git also offers commands to resolve merge conflicts automatically. The most notable is:
- `git merge --abort`: This command stops the merge process and returns your branch to the state it was in before the merge started. Use this command when you identify a complex merge conflict you aren't ready to resolve.
git merge --abort
- `git reset --hard`: This reverts your working directory to the last commit, discarding all changes made during the merge. Exercise caution with this command, as it may lead to data loss.
git reset --hard HEAD
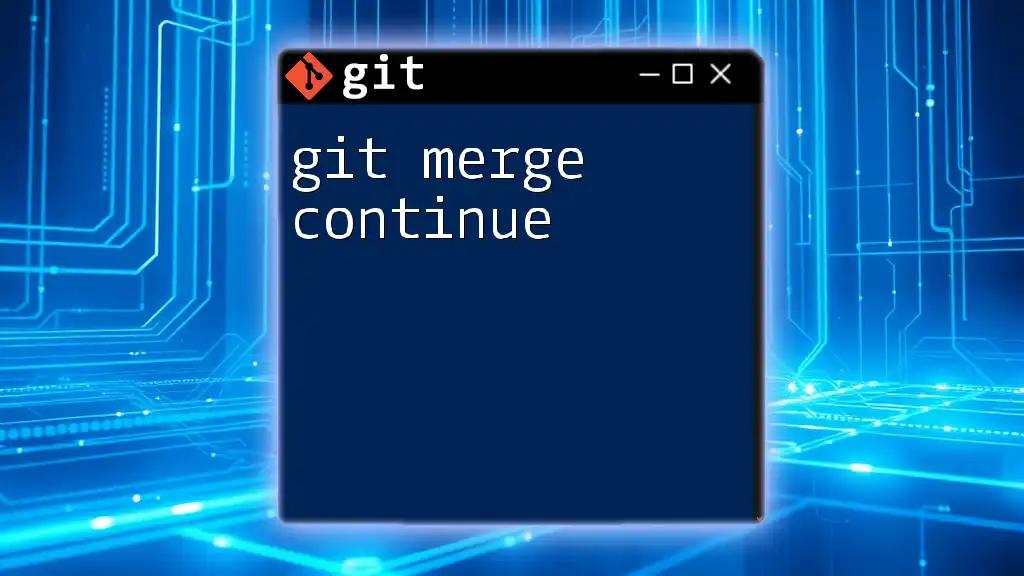
Undoing a Merge Conflict
Using Git Merge --Abort
The `git merge --abort` command is particularly useful when you want to cancel a merge altogether. If you realize that the conflict is more complicated than anticipated or you'd like to reconsider your merging strategy, this command effectively resets your workspace back to its previous state.
To use this command, simply run:
git merge --abort
This command works under the premise that the merge is still in progress and has not been completed yet.
Using Git Reset
Hard vs Soft Reset
When discussing resetting in Git, it is essential to understand the distinction between hard and soft resets. A hard reset (`git reset --hard`) will remove all changes from your working directory and staging area, reverting your repository to the specified commit.
A soft reset (`git reset --soft`) only resets the current branch to the specified commit, keeping your changes untouched in the staging area. Use soft resets when you want to recommit changes after resolving conflicts.
Example of Using Git Reset
To completely discard all the changes made during the merge and return to the last committed state:
git reset --hard HEAD
This command will erase all changes since the last commit. Ensure you are certain before using it to avoid unintended data loss.
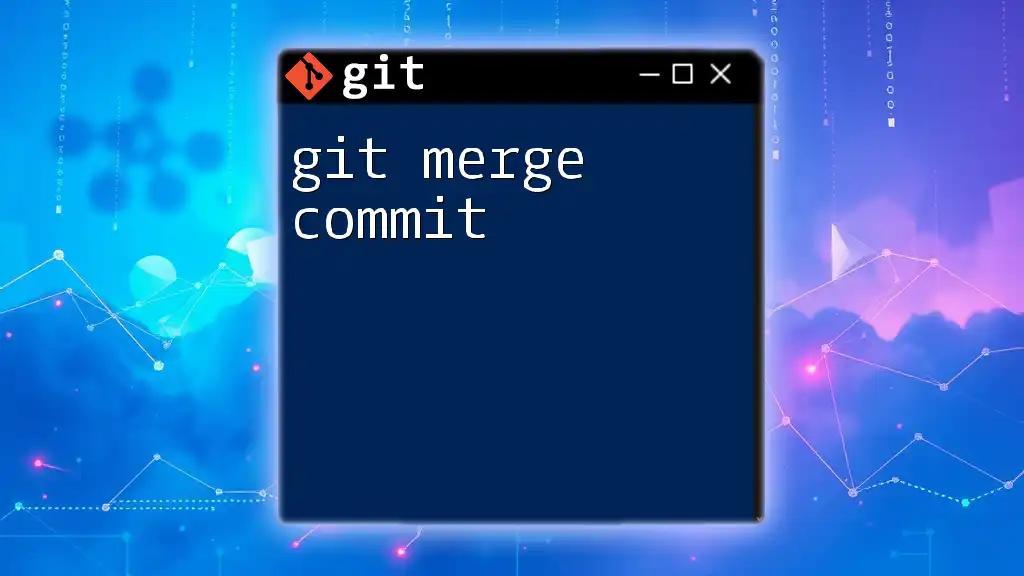
Best Practices for Avoiding Merge Conflicts
Communicate with Your Team
Effective communication within your team can significantly reduce the instances of merge conflicts. Make use of project management tools and regular discussions to align everyone on changes being made simultaneously.
Small, Frequent Commits
Encourage a workflow that includes small, frequent commits. This practice minimizes the chance of conflicts and enhances the integration of code changes, ensuring a smoother merging process.
Feature Branch Workflow
Adopting a feature branch workflow is another effective strategy for minimizing merge conflicts. By keeping each new feature in its own branch and merging them back to the main branch only when complete, you greatly reduce the chances for conflicting changes to arise.
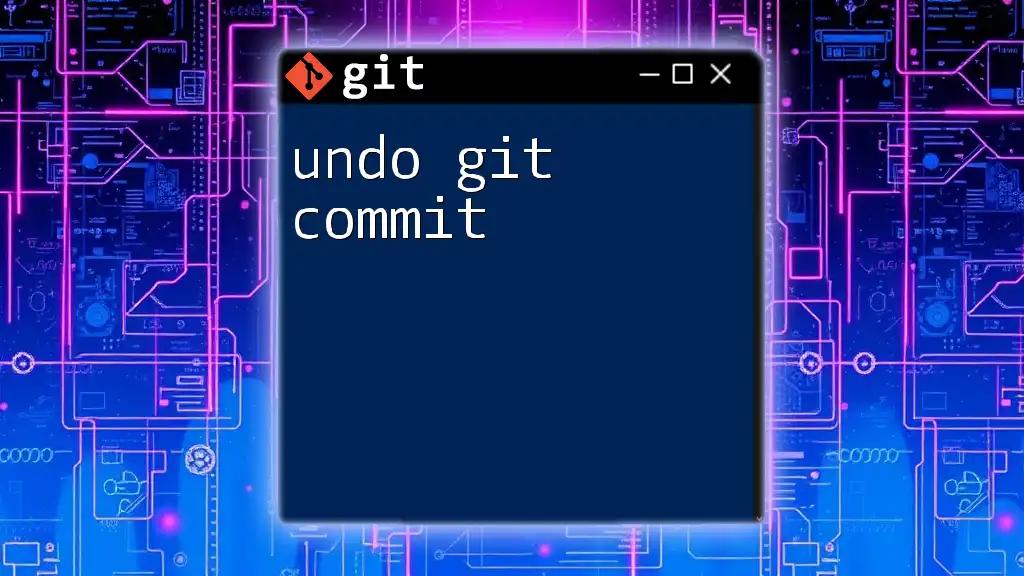
Conclusion
In summary, knowing how to undo git merge conflict situations is crucial for any developer working with Git. Whether you prefer manual resolution, using the automated `git merge --abort`, or leveraging the commands to reset your changes, mastering these techniques can improve your workflow drastically. Remember to communicate with your team, maintain small and frequent commits, and utilize a feature branch workflow to minimize conflicts in the first place. Implementing these practices will help you navigate Git more effectively and bolster your development efficiency.
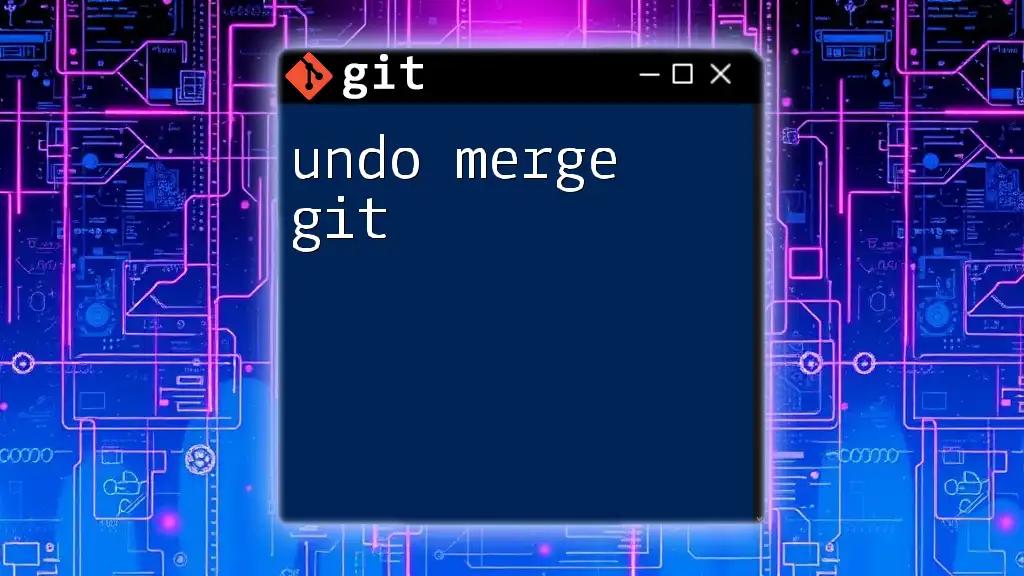
Additional Resources
Helpful Git Commands
Familiarity with essential Git commands can aid in efficient conflict resolution and management:
- `git status` - Check the status of your repository.
- `git diff` - View differences between files.
- `git log` - View commit history.
- `git rebase` - Reapply changes from one branch onto another.
Online Tutorials and Documentation
Explore these trusted resources for further learning and in-depth knowledge on Git:
- [Git Documentation](https://git-scm.com/doc)
- [Atlassian Git Tutorials](https://www.atlassian.com/git/tutorials)
- [GitHub Learning Lab](https://lab.github.com/)
These resources will help you deepen your understanding of Git and enhance your conflict resolution skills.