A git merge conflict occurs when two branches have made changes to the same line of code or when one branch deletes a file that another branch has modified, preventing Git from automatically merging the branches.
# Attempt to merge branches that may have conflicts
git merge <branch-name>
What are Git Merge Conflicts?
Merge conflicts occur when two branches have changes that are incompatible with each other. This typically happens during the merge operation, when Git tries to combine multiple changes into a single branch and detects that some changes are conflicted. Understanding merge conflicts is essential for effective collaboration, especially in team environments where developers frequently work on shared codebases.
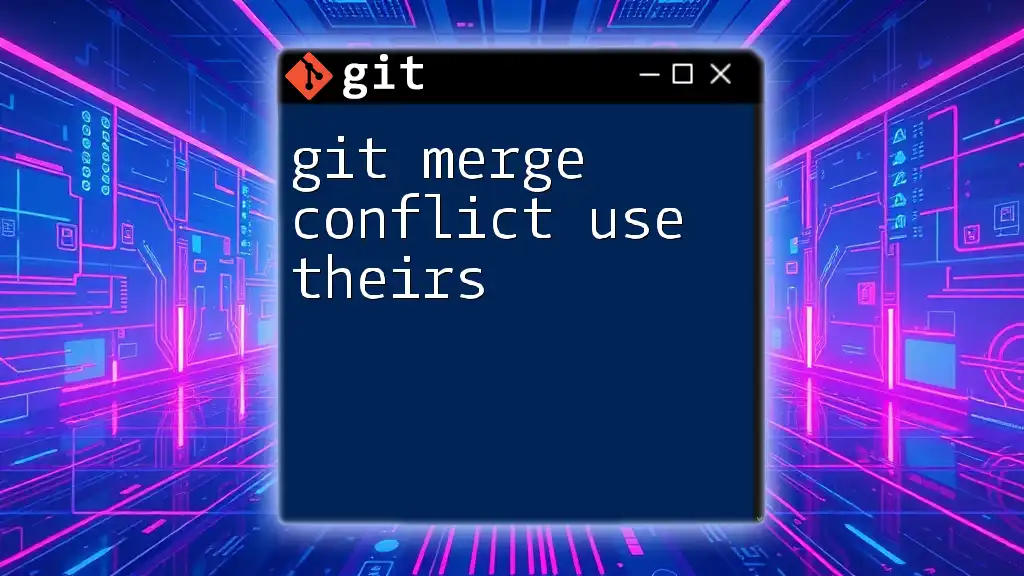
Understanding the Basics of Git Merging
What is Git Merge?
At its core, merging in Git is the process of integrating changes from one branch into another. Git employs two main types of merges:
-
Fast-forward merges occur when the branch being merged into has not diverged from the branch being merged. In this scenario, Git can simply move the branch pointer forward to the latest commit.
-
Three-way merges are used when both branches have diverged. This merging method utilizes the most recent common ancestor of the two branches to combine their changes.
When Do Merge Conflicts Occur?
Merge conflicts typically arise in these situations:
- Simultaneous Edits: When two developers edit the same line of code in separate branches.
- Adjacent Changes: If two developers make changes in lines that are close to each other, resulting in inconsistencies.
For example, if Developer A modifies a function in `feature-branch` while Developer B edits the same function in `main`, merging these branches will likely lead to a conflict.
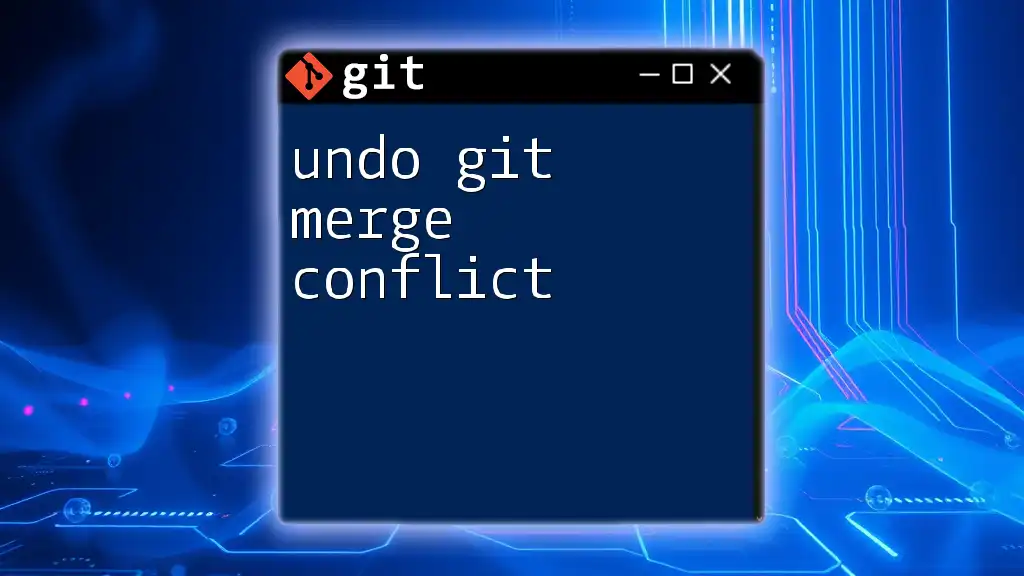
Identifying Merge Conflicts
How to Detect a Merge Conflict?
Merge conflicts manifest themselves during a merge operation. When you run the command to merge branches, like so:
git merge feature-branch
If there are conflicting changes, Git will halt the merge process and inform you of the conflict.
Understanding Conflict Markers
When you open your code editor after a merge conflict, you will see conflict markers that visually indicate the problem areas:
<<<<<<< HEAD
This is my content from the main branch.
=======
This is my content from the feature branch.
>>>>>>> feature-branch
Here’s what each marker indicates:
- `<<<<<<< HEAD`: This marks the beginning of the changes that exist in the current branch (in this case, the `main` branch).
- `=======`: This separates changes from the two branches.
- `>>>>>>> feature-branch`: This indicates the changes coming from the `feature-branch`.
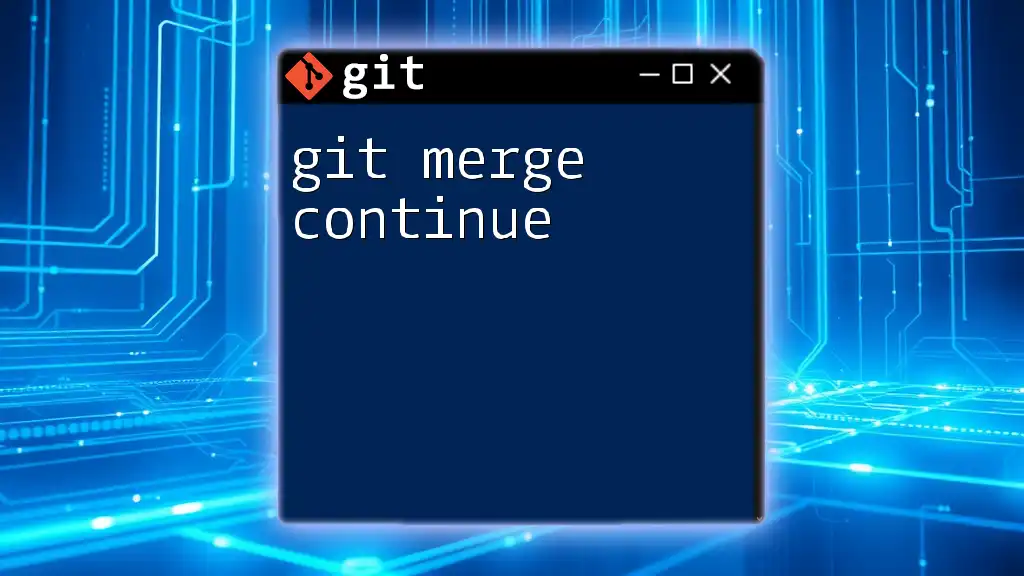
Resolving Merge Conflicts
Manual Conflict Resolution
The manual resolution process typically involves these steps:
- Identify Conflicting Sections: Locate where the conflict markers are in the code.
- Make a Decision: Choose which changes to keep—whether it be retaining one version, combining both, or rewriting the entire section based on context.
- Remove Conflict Markers: Once you've made your edits, ensure you delete all conflict markers (i.e., `<<<<<<<`, `=======`, `>>>>>>>`).
- Add and Commit: After resolving conflicts, stage the changes and commit:
git add .
git commit -m "Resolved merge conflicts"
Using a Merge Tool
Utilizing a merge tool can streamline this process. Popular choices include KDiff3, Meld, and Beyond Compare. Setting up a merge tool in Git is simple; for example, to configure Git to use Meld, run:
git config --global merge.tool meld
Once set up, you can invoke the merge tool using:
git mergetool
This opens the selected merge tool, making it easier to resolve conflicts visually.
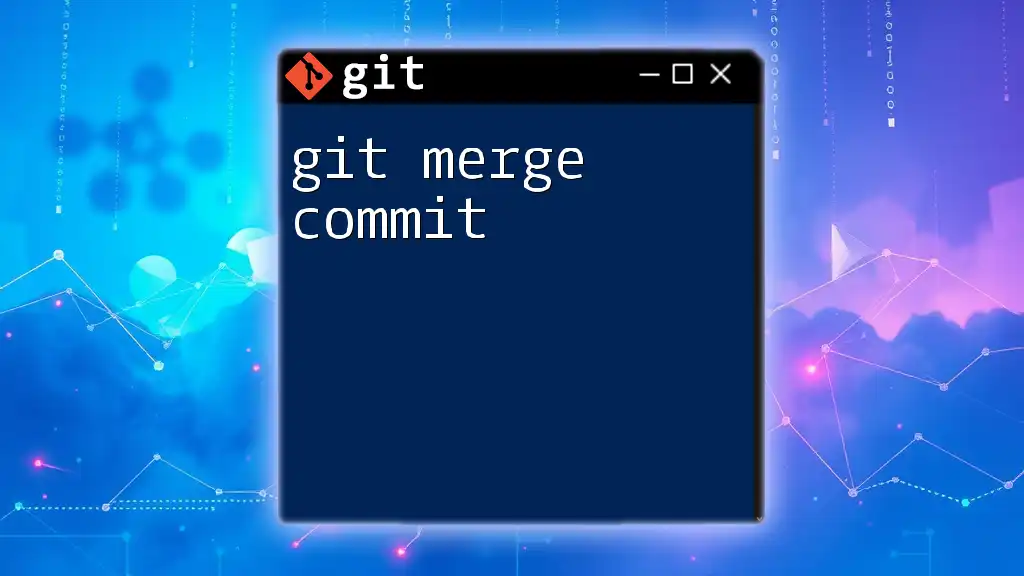
Common Strategies for Conflict Resolution
The Acceptance Strategy
In some cases, you might choose to accept all changes from one branch over the other. This can be appropriate if one branch represents more complete or accurate work.
The Combination Strategy
When both branches contain valuable changes, adopting a combination strategy is effective. For instance, you may merge text from both branches that create a new, comprehensive piece of content.
When to Resolve Conflicts in Favor of the Main Branch
Team collaboration often favors resolving conflicts in favor of the main branch (e.g., the `main` or `master` branch) when maintaining the project's integrity or stability is crucial. This approach is particularly important when critical features or functionalities are involved.
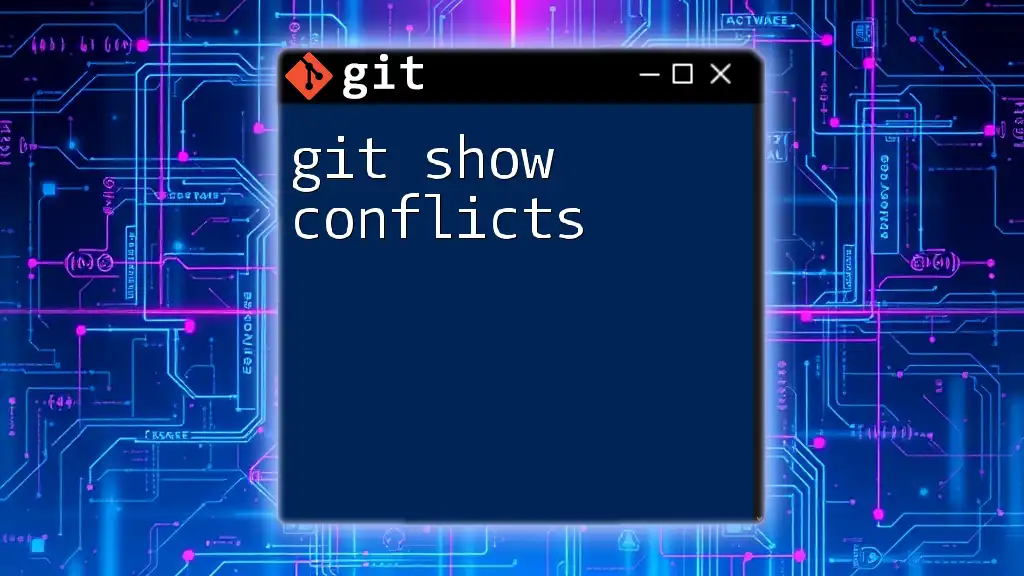
Best Practices to Avoid Merge Conflicts
Frequent Pulling and Merging
Frequent updates with `git pull` and regular merges can significantly reduce the chance of conflicts. By synchronizing your branches regularly, you minimize divergence.
Smaller, More Frequent Commits
Making smaller, more frequent commits is advisable. Less extensive changes are typically easier to understand and resolve, reducing the likelihood of conflicts.
Effective Communication with Team Members
Ensure there’s open communication among team members about who is working on what. Regular updates regarding changes can help coordinate efforts and avoid stepping on each other’s toes.
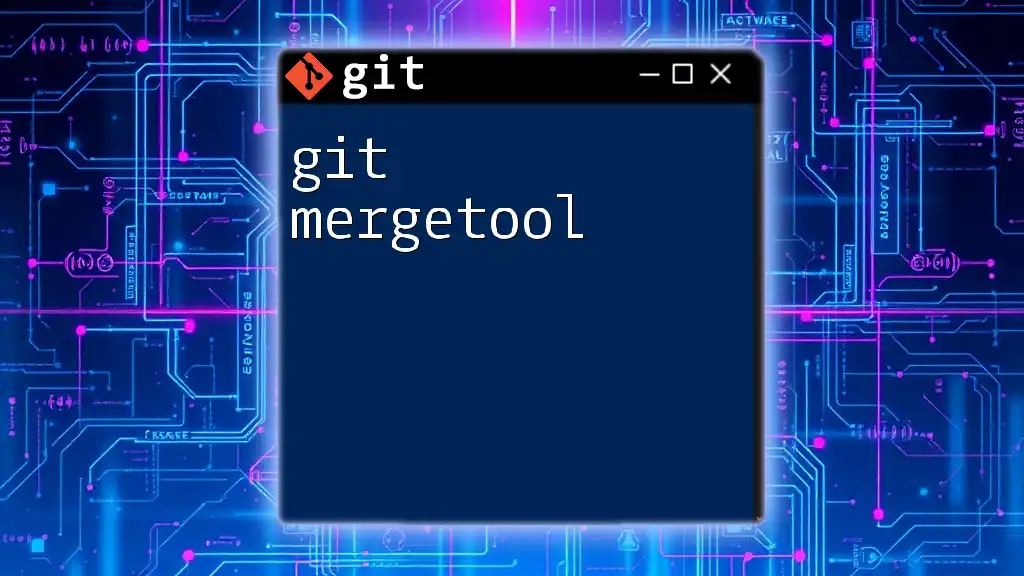
Conclusion
In summary, understanding git merge conflicts is critical for anyone working in collaborative environments using Git. By recognizing how conflicts arise, effectively resolving them, and implementing best practices to avoid them, you can enhance both personal productivity and team collaboration.
Further Resources
To deepen your knowledge, you might explore additional tutorials and documentation on Git conflict resolution. Such resources can provide valuable insights into advanced conflict management techniques.
Call to Action
Feel free to share your experiences with merge conflicts in the comments below! We invite you to sign up for our Git training programs to sharpen your command skills and navigate Git with confidence.