To undo a Git commit while keeping the changes in your working directory, use the following command:
git reset --soft HEAD~1
Understanding Git Commits
What is a Git Commit?
A Git commit is a fundamental command in version control that serves to save changes to a repository. Each commit acts as a snapshot of the project at a specific point in time, recording what changes were made and under what circumstances. You can think of it as a timestamped save point, allowing you to track the evolution of your project over time.
The importance of commits cannot be overstated. They allow developers to revert to previous states, investigate the history of changes, and collaborate with others effectively. Once a commit is made, it captures the state of files in the staging area and creates an immutable entry in the project history.
Common Scenarios That Require Undoing a Commit
There are several scenarios where you might find yourself needing to undo a Git commit. Here are a few common examples:
-
Mistakes Made in the Commit Message: Sometimes, you may accidentally use the wrong wording or forget to include important details in the message.
-
Unintended Changes Included in the Commit: You might realize that you included changes that should not have been committed, such as debug code or unnecessary file adjustments.
-
Committing the Wrong Files: It’s easy to mix up files, and you may find that your last commit includes changes to a file you did not intend to touch.
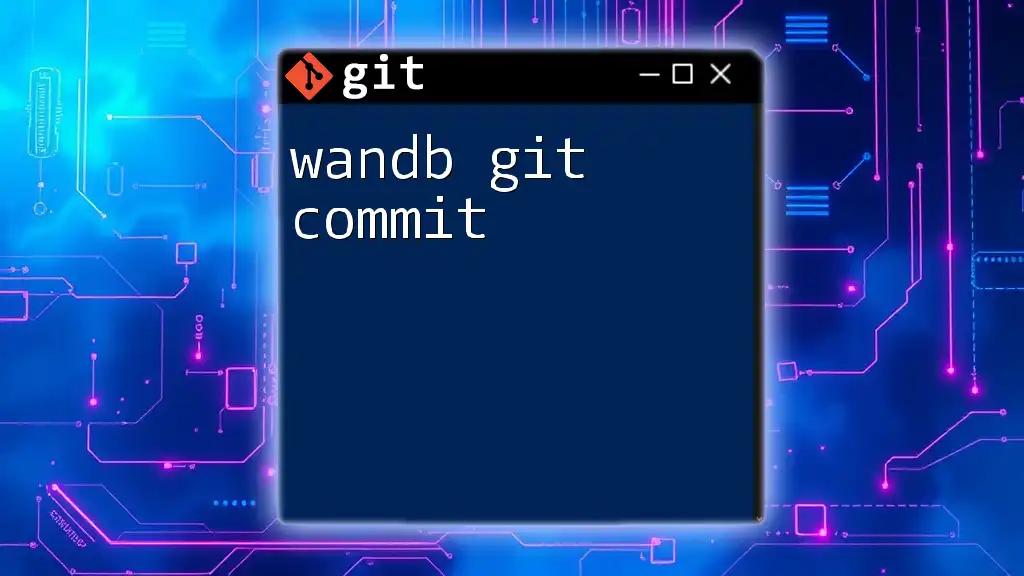
Methods to Undo a Git Commit
Using `git reset`
Understanding `git reset`
The `git reset` command is a powerful way to undo changes in your Git repository by moving the current branch pointer to a specified commit. The command can take different options that determine how changes are handled:
-
`--soft`: Moves the branch pointer without affecting the working directory or the staging area. Changes are preserved as staged.
-
`--mixed`: Moves the pointer and resets the staging area, making changes untracked but keeping the working directory unchanged.
-
`--hard`: Resets the pointer and changes the staging area and the working directory to match the commit, resulting in data loss for uncommitted modifications.
Examples of `git reset`
-
Undoing Last Commit but Keeping Changes
git reset --soft HEAD~1
This command will leave your changes staged and allow you to make further modifications or adjust your commit message before committing again.
-
Undoing Last Commit and Unstaging Changes
git reset HEAD~1
With this command, your last commit is undone, but the changes remain in your working directory as unstaged. This gives you the freedom to re-evaluate what should be committed next.
-
Completely Undoing Last Commit
git reset --hard HEAD~1
Caution: This action will delete all changes in your previous commit and any untracked changes in your working directory as well. Use this command only when you are certain you no longer need the last commit and none of the changes.
Using `git revert`
What is `git revert`?
The `git revert` command is an alternative to `git reset` that is used when you want to undo a commit while keeping a complete project history. Unlike `git reset`, which moves the branch to a previous state, `git revert` creates a new commit that effectively negates changes made in a specific commit, avoiding any history disruption.
Examples of `git revert`
-
Reverting the Last Commit
git revert HEAD
This command will create a new commit that undoes the changes introduced by the last commit. This is particularly useful in shared repositories where maintaining commit history is crucial.
-
Reverting a Specific Commit by Hash
git revert <commit_hash>
Replace `<commit_hash>` with the actual hash of the commit you wish to revert. This allows selective retraction of changes in larger histories.
Using `git commit --amend`
What is `git commit --amend`?
The `git commit --amend` command allows you to modify the most recent commit. This command is particularly powerful for fixing mistakes immediately after a commit has been made, such as incorrect messages or missed files.
Example of Commit Amendment
-
Fixing the Last Commit’s Message
git commit --amend -m "New commit message"
With this command, you can change the commit message of the last commit. It creates a new commit that replaces the last one without altering its content.
-
Adding Changes to the Last Commit
git add <file> git commit --amend
After staging the intended file (or files), you can amend the last commit to include additional modifications. This merges the new changes with the previous commit.
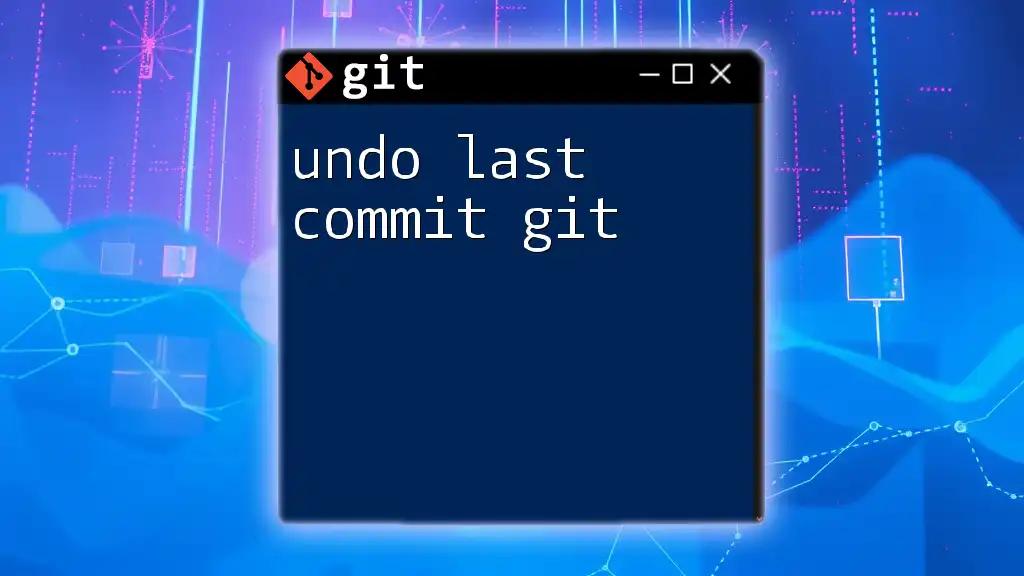
Best Practices for Undoing Commits
It’s essential to understand when to use each method for undoing Git commits. Here are some best practices:
-
Knowing When to Use Each Method: Choose `git reset` for local changes that haven’t been shared with others, and opt for `git revert` for altering changes that are already part of shared history.
-
Importance of Clear Commit Messages: Maintain clarity in your commit messages from the start, as this minimizes the need for amendments or reverts later on.
-
Maintaining a Clean Commit History: Keeping a clean and thoughtful commit history aids in collaboration and debugging in the future. Use amendments judiciously and avoid unnecessary clutter.
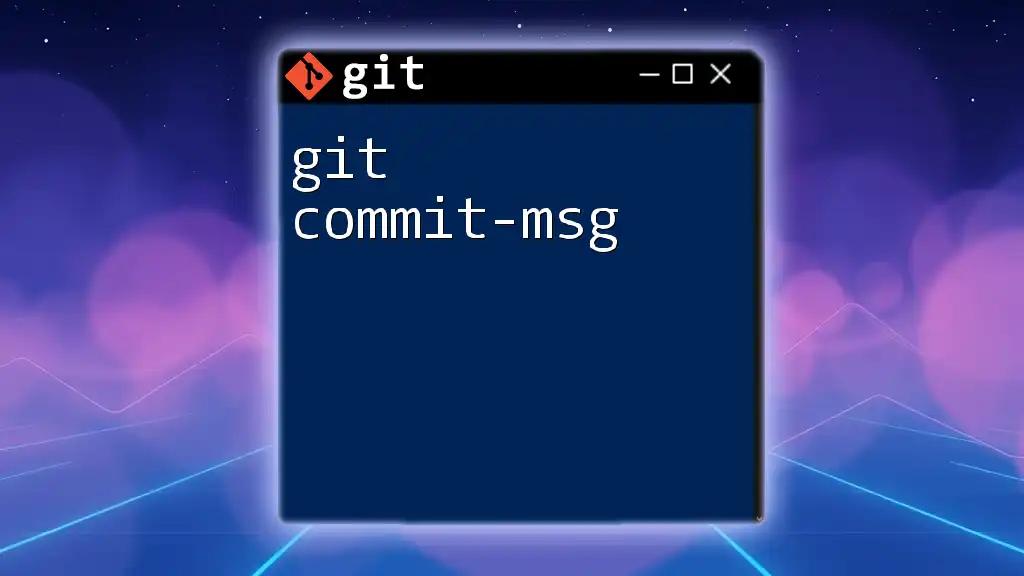
Conclusion
Understanding how to undo Git commits is a vital skill for anyone working with Git. By mastering the techniques of `git reset`, `git revert`, and `git commit --amend`, you’ll enhance your ability to manage your projects effectively while preserving a clean and comprehensible project history.
Incorporating best practices in your Git workflow will ensure a smoother development process, reduced errors, and improved collaboration. As you continue to explore Git, consider practicing these commands in your local repositories to gain confidence and fluency.
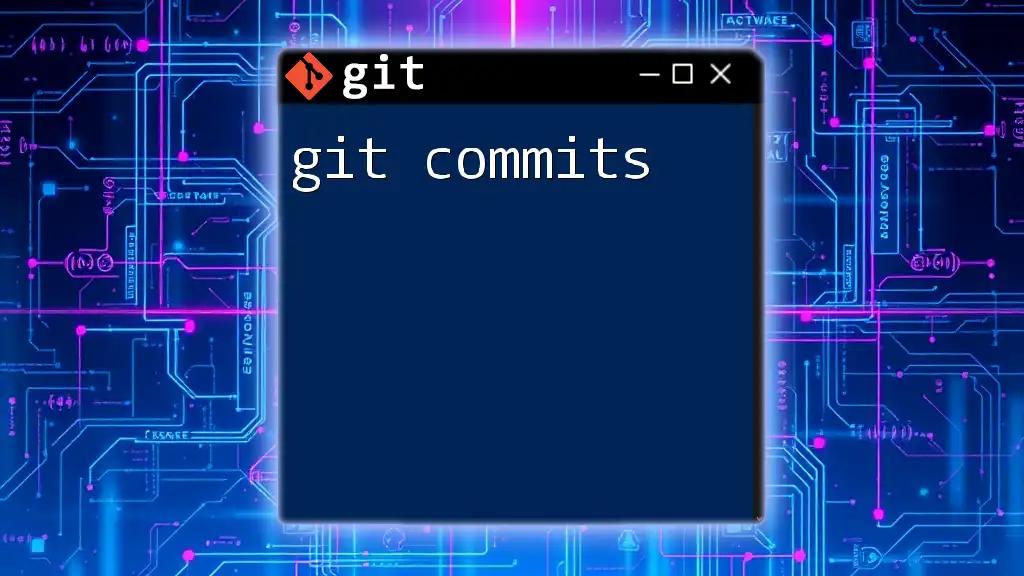
Additional Resources
Further Reading
- Explore the official Git documentation for an in-depth understanding of each command.
- Search for video tutorials that illustrate real-world scenarios for visual demonstrations.
Tools for Git Users
- Consider using recommended Git GUI tools that simplify version control tasks.
- Familiarize yourself with command-line tips and tricks to improve productivity and efficiency.