To undo the last commit in Git without losing your changes, you can use the following command:
git reset --soft HEAD~1
Understanding Git Commits
What is a Git Commit?
A Git commit serves as a snapshot of your project's current state, encapsulating changes made at a specific time. Each commit is associated with a unique identifier, usually a hash, that allows you to track changes over time. This identifier, along with a commit message, helps you understand the context of changes made.
How Commits are Structured
In Git, a commit is often viewed as a collection of files, their statuses, and an accompanying message reflecting what was accomplished. When you create a commit, Git records:
- The hash ensuring the integrity of your commit.
- The commit message explaining the changes.
- A reference to the parent commit, forming a chain of history.
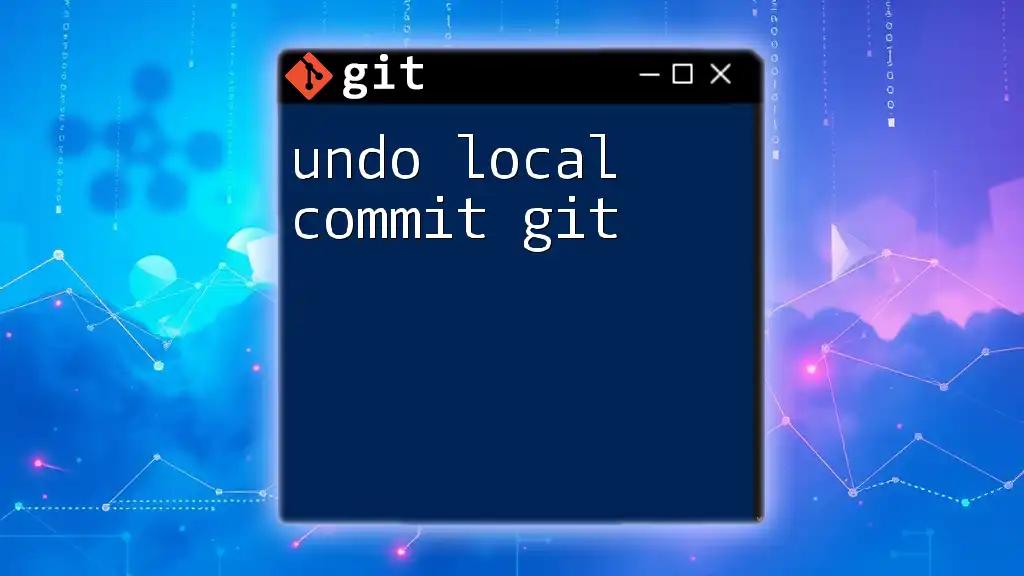
Methods to Undo the Last Commit
Using `git reset`
What is `git reset`?
The command `git reset` allows you to move the current `HEAD` to a specified state, which can be crucial for undoing changes. However, it affects your project history and can alter your staging area.
Different Options for `git reset`
-
Soft Reset
When you need to keep your changes in the staging area but want to clear the last commit, a soft reset is the way to go. The command to execute is:git reset --soft HEAD~1
Explanation: This command reverts the last commit while keeping your files staged, allowing you to make any modifications before recommitting.
Example and Scenario: If you've committed changes but realized you need to add more functionality or correct mistakes, this option provides the flexibility you need to update your commit.
-
Mixed Reset
If you prefer your changes to remain in the working directory yet unstage them, you can use:git reset HEAD~1
Explanation: This resets your last commit while maintaining your changes in the working directory but removes them from the staging area.
Example and Scenario: Use this method when you want to change files before recommitting without losing your recent work.
-
Hard Reset
A hard reset is for when you want to discard the last commit entirely—both its changes and the files. Use this with caution as it cannot be undone easily.git reset --hard HEAD~1
Explanation: This completely resets your commit history to the previous state, removing all changes made in the last commit.
Example and Scenario: This option is suitable when you’ve made significant errors and want to revert to a known good state without keeping any changes.
Using `git revert`
What is `git revert`?
Unlike `git reset`, which changes your commit history, `git revert` offers an alternative that is both safer and more collaborative. Instead of deleting a commit, it creates a new one that effectively reverses the changes of the last commit.
Reverting the Last Commit
To revert your last commit, use the command:
git revert HEAD
Explanation: This command creates a new commit that undoes the changes made in the last commit while keeping the history intact, an essential aspect when working on shared projects.
Example and Scenario: If you're collaborating with others and cannot risk altering the commit history, reverting is ideal. It enables you to correct mistakes without disrupting the project flow for your team.
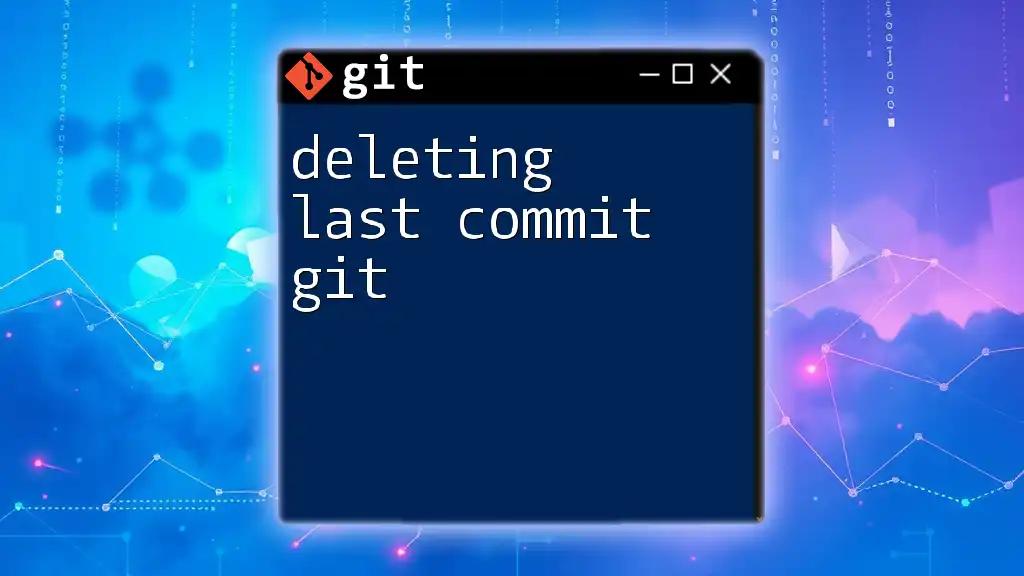
Comparing `git reset` and `git revert`
When to Use Each Method
Choosing between `git reset` and `git revert` largely depends on the context of your work:
- Use `git reset` when you have made local commits that you control. It’s especially useful for cleaning up your history before pushing to a shared repository.
- Use `git revert` when working in a collaborative environment. It’s the ideal route when you need to negate a change without affecting your teammates or the project history.
Pros and Cons
-
Pros of `git reset`:
- Allows you to rewrite commit history.
- More control over local changes.
-
Cons of `git reset`:
- Risk of losing changes permanently.
- Can cause conflicts in a shared environment.
-
Pros of `git revert`:
- Safe for collaborative work; preserves history.
- Clear understanding of the changes made.
-
Cons of `git revert`:
- Results in a more cluttered history.
- Requires additional commits to reverse changes.
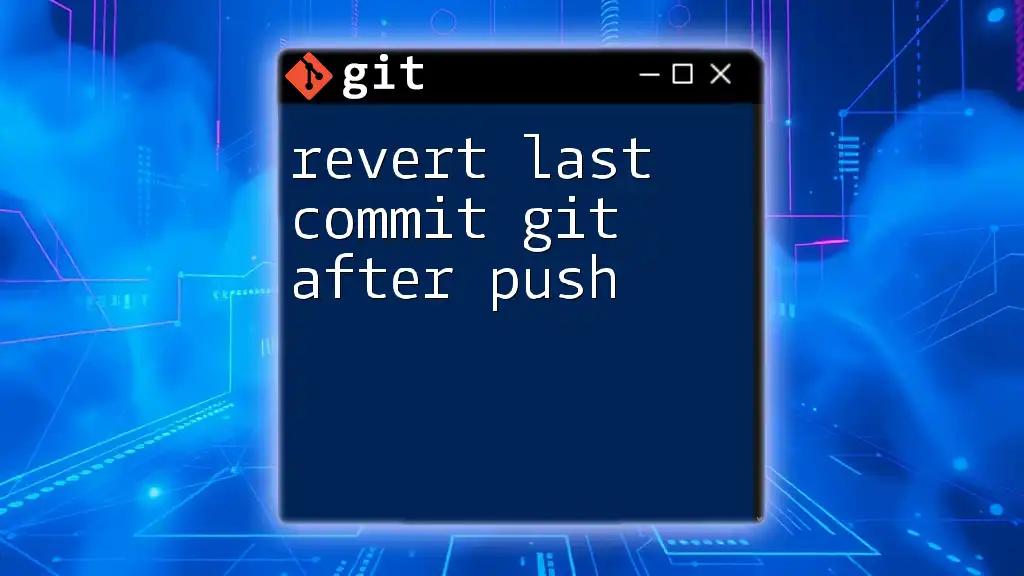
Real-World Scenarios and Examples
Scenario 1: Accidentally Committed the Wrong File
Imagine you've accidentally committed a file that should not be part of your project. To undo this commit and keep your changes, you can use the command:
git reset --soft HEAD~1
This keeps your changes staged, enabling you to remove the unintended file before committing again.
Scenario 2: Committed with Typos in the Message
If you realize that your last commit message contains a typo, proceed as follows:
-
Reset your last commit:
git reset --soft HEAD~1
-
Make necessary adjustments and recommit with the correct message using:
git commit -m "Corrected commit message"
Scenario 3: Undoing the Last Commit on a Shared Branch
While working on a team, you accidentally commit changes that should not be part of the shared repository. You can swiftly handle this with:
git revert HEAD
This not only undoes the changes but also logs the action, allowing your team to see that a mistake was corrected.
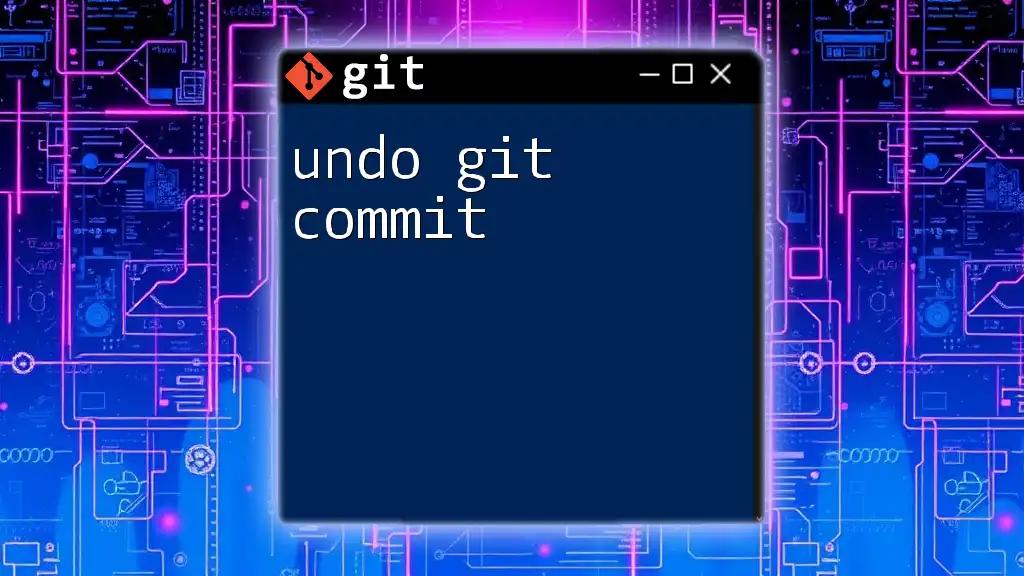
Best Practices for Managing Commits
Creating Clear Commit Messages
Always focus on crafting concise and meaningful commit messages. A good practice is to write messages in the imperative tense (e.g., "Fix bug" rather than "Fixed bug"). This clarity can reduce the need to undo commits.
Using Branches for Feature Development
Adopt a policy of developing features on separate branches. This keeps your main branch clean and minimizes the impact of accidental commits on the main codebase.
Regularly Review Commits
Engage in a routine practice of reviewing your commits before pushing. This can help identify issues early and prevent the need for undoing commits later.
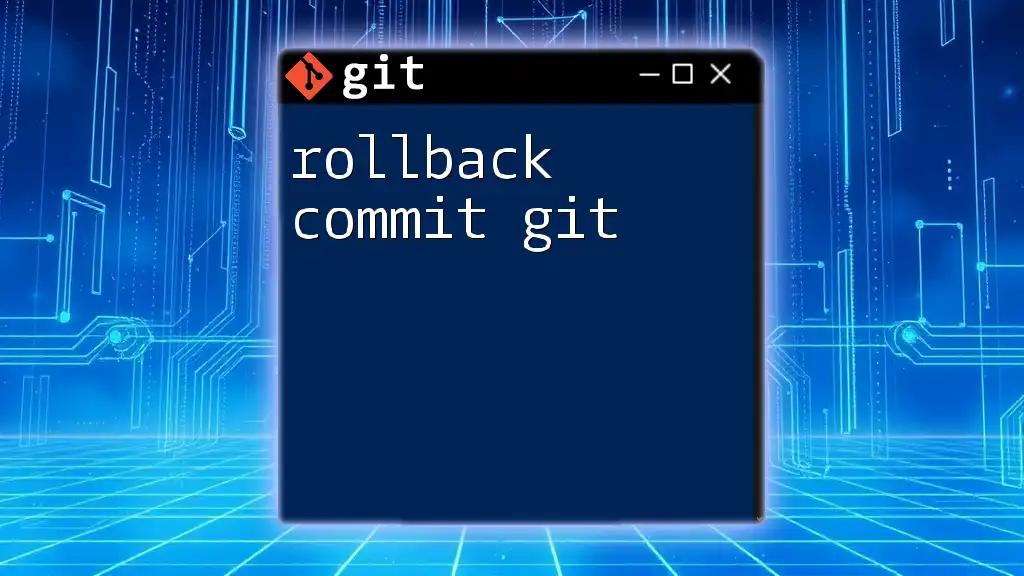
Conclusion
Understanding how to undo the last commit in Git is an essential skill for any developer. Whether you choose to use `git reset` or `git revert`, knowing the differences, capabilities, and appropriate contexts is key to effective version control. Utilize these commands thoughtfully, and always practice good commit hygiene for a more manageable workflow.
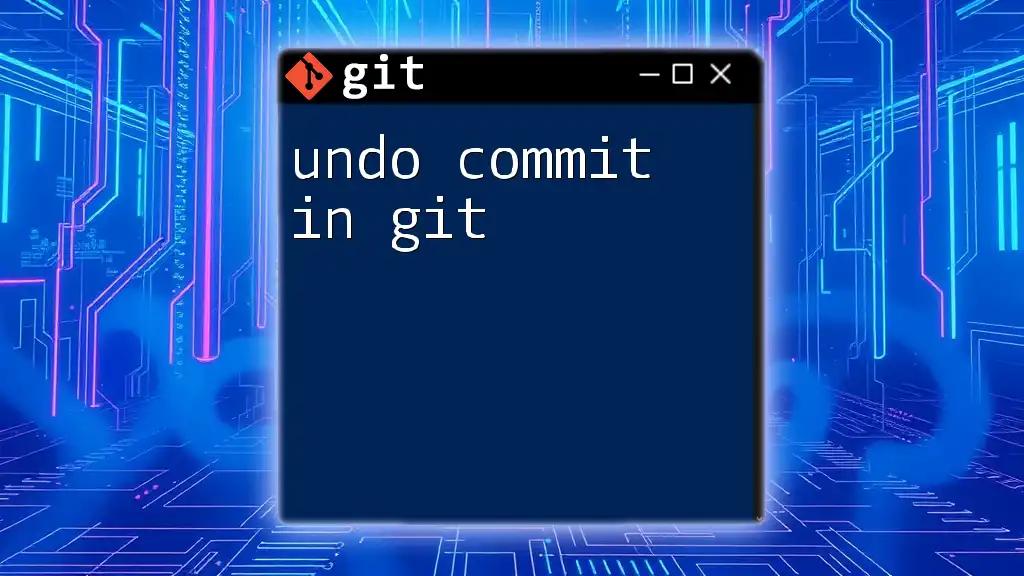
Call to Action
Want to dive deeper into mastering Git commands? Sign up for our comprehensive courses and hands-on workshops designed to boost your Git proficiency. You'll learn not just to undo commits but also to harness the full power of version control in your projects.
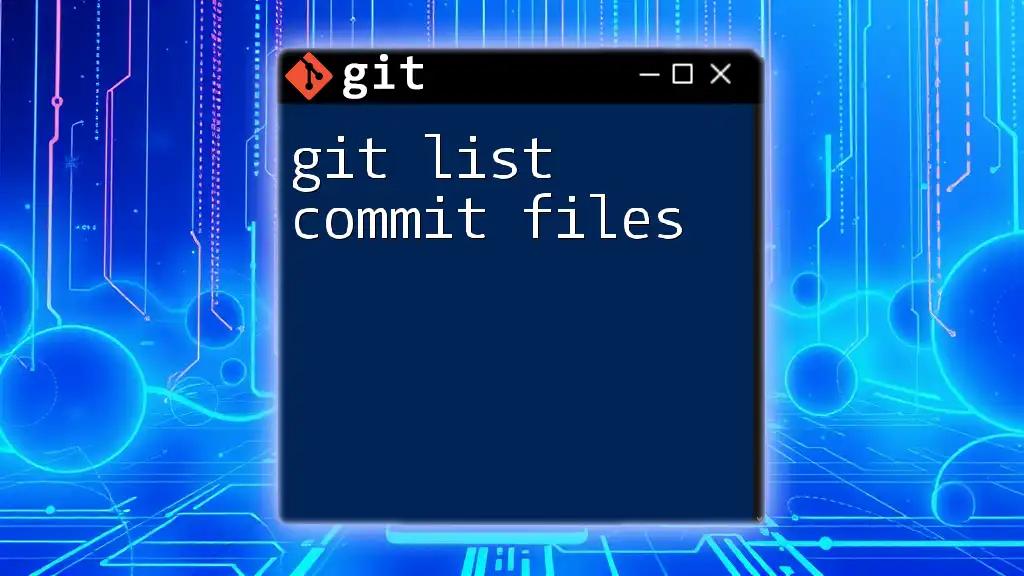
References and Further Reading
For those keen on advancing their Git skills, we recommend checking the official Git documentation and relevant community articles for more insights on advanced Git usage and best practices.