Atomic Git refers to the practice of making small, self-contained commits that encapsulate a specific change or feature, ensuring a clear and manageable project history.
Here’s a code snippet demonstrating how to make an atomic commit:
git add .
git commit -m "Add feature X: Implement user authentication"
Definition of Atomic Git
Atomic Git refers to the practice of making commits that are small, self-contained, and focused on a single change or purpose. The term "atomic" implies that each commit represents a complete unit of work that can stand alone, making it easy to understand and manage in the context of version control. This practice helps maintain a clear project history and allows for easier collaboration among team members.
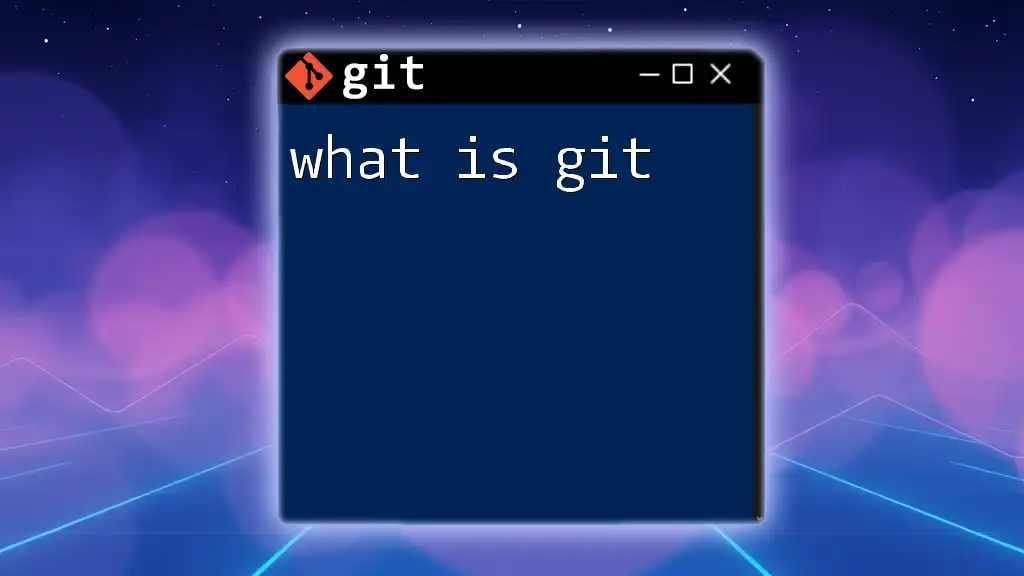
Why Use Atomic Commits?
Choosing to adopt atomic commits comes with significant benefits:
- Clarity: Each commit is easy to understand at a glance, allowing others to grasp the project's progression without sifting through large swathes of changes.
- Reversibility: Smaller, isolated changes mean that if a mistake occurs, it's easier to find and revert just that specific commit. This leads to increased confidence during development.
- Collaboration: When multiple team members contribute, atomic commits help avoid conflicts and confusion, ensuring that each developer can understand the contributions of others.
- History: An atomic commit history is cleaner and easier to follow, enhancing the maintainability of the codebase.
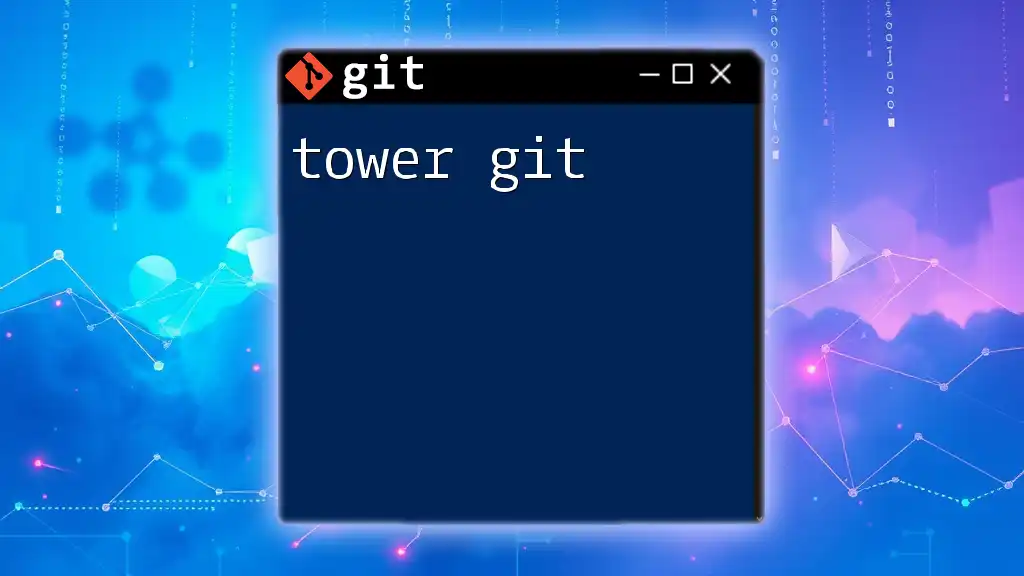
Understanding Atomic Commits
What Makes a Commit Atomic?
An atomic commit embodies the principle of single-responsibility, where each commit addresses one distinct purpose.
Example: Consider a scenario where you make changes related to a new feature and also fix a bug in the same commit. This should be split into two atomic commits:
git commit -m "Add user authentication feature"
git commit -m "Fix typo in login error message"
In this case, each commit clearly conveys a specific intention.
Characteristics of Atomic Commits
Small, Self-Contained Changes
Smaller commits facilitate understanding and review. When changes are segregated into atomic units, they make it easier for others to follow along and manage the codebase.
Descriptive Commit Messages
It's crucial to accompany each atomic commit with a descriptive message that conveys the essence of the change. A poor commit message might say, "Fixed stuff," while a good one would be, "Add input validation for user registration."
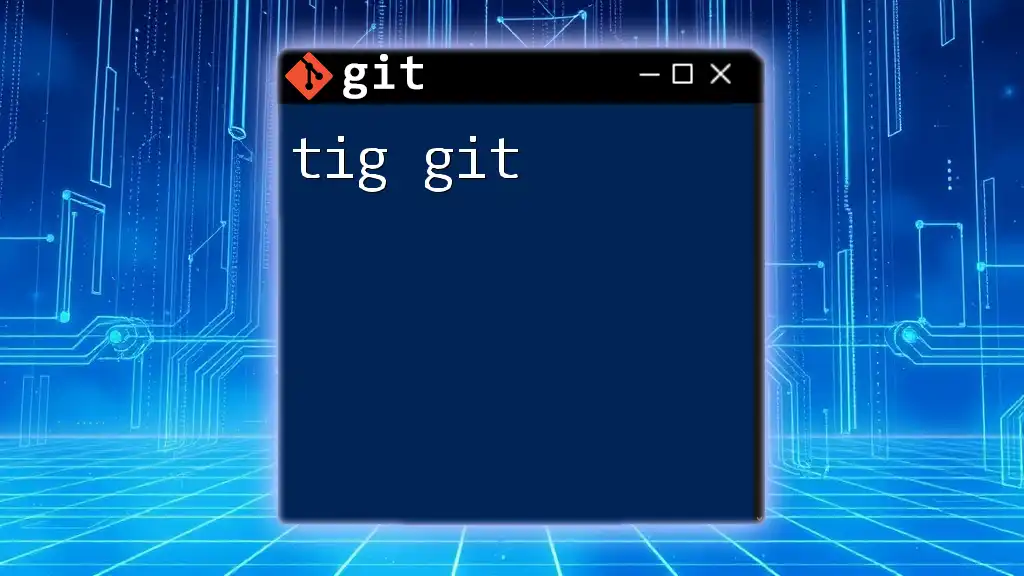
Guidelines for Making Atomic Commits
Structuring Commits
Feature vs Bug Fix Commits
Recognizing the difference between various types of commits is essential. A feature commit introduces new capabilities, whereas a bug fix commit addresses issues or deficiencies. Correctly categorizing these changes enhances project traceability.
Granularity in Changes
When to Split Commits: If a commit has several unrelated changes, it's prudent to split them. For example, if you're updating several styles, adding a new API endpoint, and fixing a bug, you should isolate each change into its own commit.
Example:
git add styles.css
git commit -m "Update button styles to enhance UI"
git add api.js
git commit -m "Add new user profile API endpoint"
git add bugfix.js
git commit -m "Fix null pointer exception in bugfix"
Best Practices for Commit Messages
Format of a Commit Message
Following a structured format for commit messages can tremendously improve project maintainability. An efficient commit message should have:
- Subject line: A brief description of the change (under 50 characters).
- Body: An optional, more in-depth explanation (wrapped at 72 characters).
- Footer: If applicable, references to issues or other related commits.
Using Git Templates
To maintain consistency, consider creating a template for your commit messages. This can be setup in your Git configuration:
git config --global commit.template ~/.git-commit-template.txt
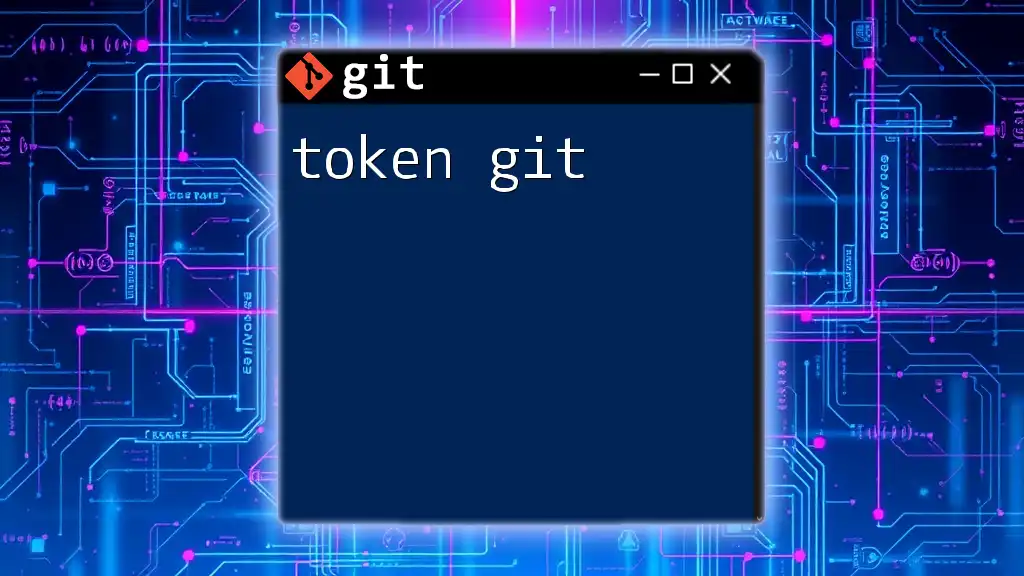
Tips for Leveraging Atomic Commits in Git Workflow
Commit Frequently
Frequent commits lead to safer development practices by serving as incremental backups of your work. The more often you commit, the less loss you experience in case of failure, whether from bugs or external issues.
Staging Changes Effectively
The `git add` command plays a critical role in the atomic commit paradigm. Using `git add -p` allows you to stage changes interactively, offering fine control over what changes to include in your commit:
git add -p
This command breaks down your changes into hunks, allowing you to selectively stage parts of your work.
Interactive Rebase for Cleanup
If your commit history becomes unwieldy, interactive rebase can help you refine it to achieve atomic commits:
git rebase -i HEAD~n
Here, replace `n` with the number of commits you wish to edit. You can then choose to squash or reword commits, which is useful for cleaning up a cluster of related commits into atomic units that represent distinct changes.
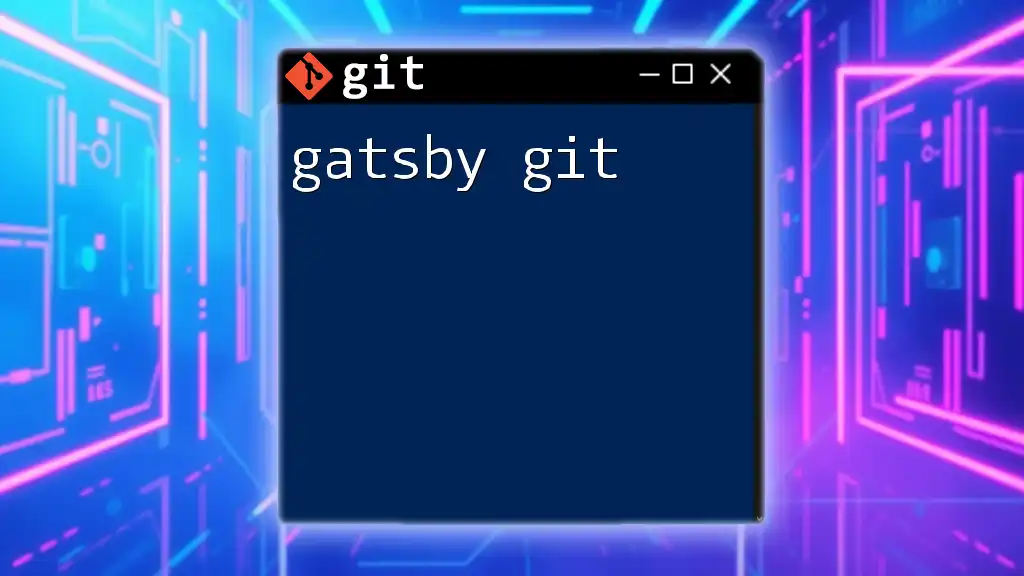
Common Challenges with Atomic Commits
Overcoming Resistance to Change
Introducing atomic commits can face resistance from team members used to a different workflow. Educating your peers about the benefits of atomic commits can help foster buy-in. Consider preparing a presentation or a code review session to demonstrate best practices.
Dealing with Missed Atomicity
If you find yourself with non-atomic commits, you can revise them using `git rebase`. This process involves splitting large commits into smaller, more focused pieces, thereby achieving the atomicity you missed initially.
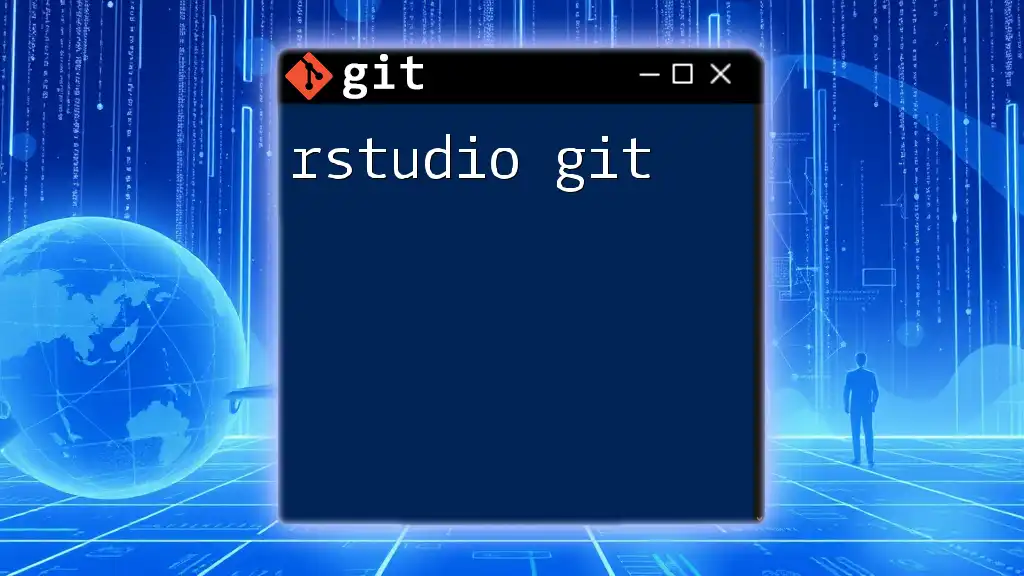
Conclusion
Embracing atomic commits in your Git workflow is a powerful practice that enhances both the clarity and functionality of your version control strategy. By ensuring larger changes are broken down into manageable units, you create a cleaner project history and a more collaborative environment. As you adopt atomic commits, focus on crafting descriptive commit messages, practicing frequent commits, and leveraging tools available in Git. This commitment to atomic Git will undoubtedly lead to a more refined and efficient development process.
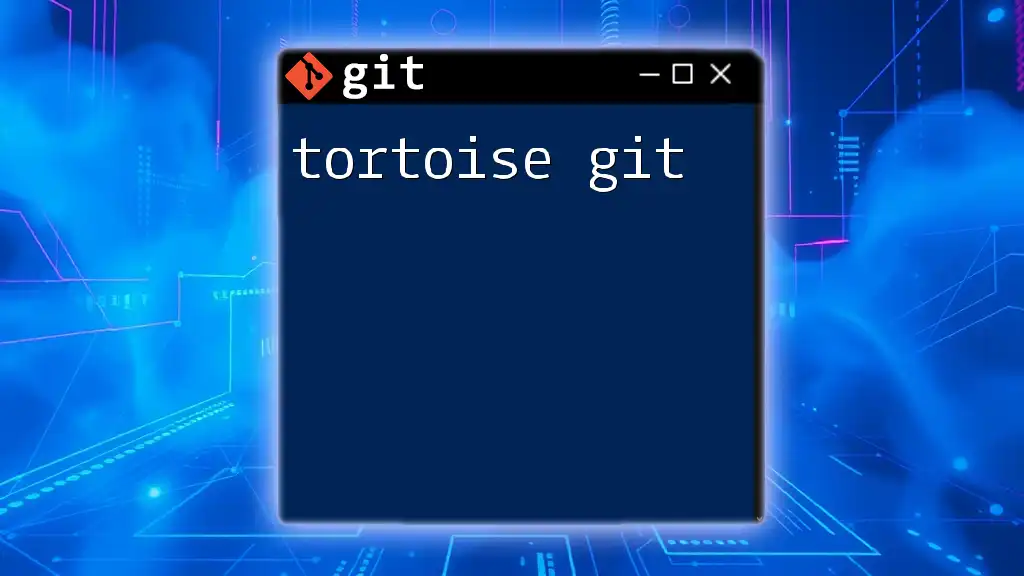
Additional Resources
Links to Git Documentation: Git's official documentation offers comprehensive guidance on all commands, best practices, and usage examples. Consult this resource for deep dives into specific areas of Git.
Recommended Tools and Plugins: Consider exploring tools and plugins designed to facilitate atomic commits, such as Git GUI clients or IDE extensions that help manage commit messages and changes.
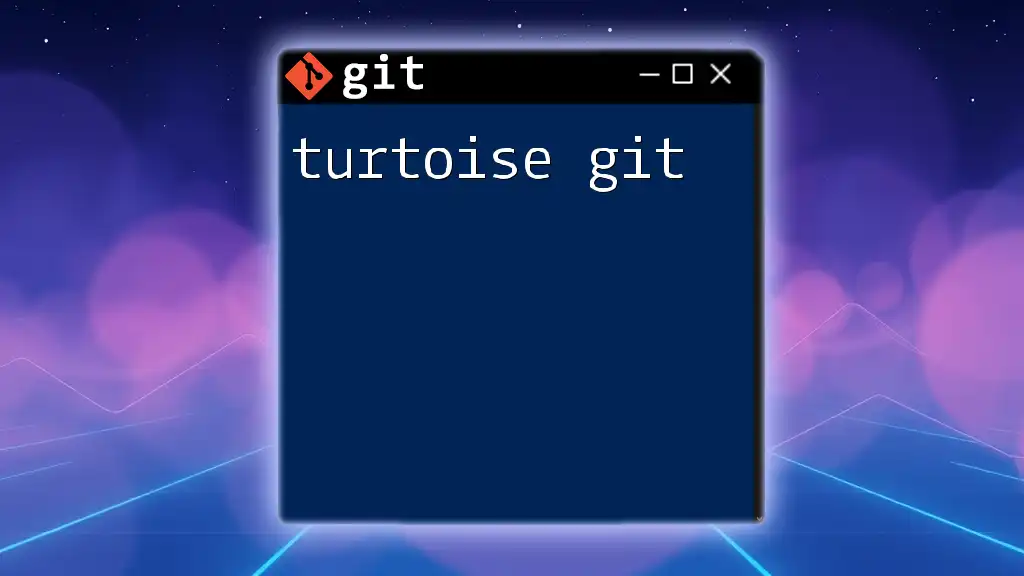
Frequently Asked Questions
What to do if I can't split a commit?
If a commit is too intertwined to split easily, consider committing the entire change and planning to refactor it in the future. Document any intent to separate functionality in your commit message for future reference.
How can I help my team adopt atomic commits?
Creating a conducive environment for learning by offering workshops or integrating code review sessions that focus on atomic commits can help others see the benefits firsthand. Encourage discussions about commit strategies during regular team meetings, which can foster a culture of continuous improvement around atomic Git practices.