"OCaml Git" refers to using Git for version control in OCaml projects, ensuring efficient management of code changes and collaboration among developers.
Here’s an example command to initialize a new Git repository for an OCaml project:
git init my_ocaml_project
Setting Up Your OCaml Environment
Installing OCaml
OCaml, known for its powerful type system and functional programming features, is often used in applications ranging from systems programming to web development. To start working on OCaml projects, you first need to install OCaml itself.
One of the best ways to do this is by using OPAM, the OCaml Package Manager. OPAM handles OCaml installations and packages, making it easy to manage different OCaml versions and libraries.
Installation Instructions:
-
Using OPAM: To install OPAM, you can follow the installation instructions from the official [OPAM website](https://opam.ocaml.org/doc/Install.html).
-
Native Installation Methods: Alternatively, you can install OCaml through native package managers like `apt` for Debian-based systems or `brew` for macOS:
sudo apt install ocaml
Once OCaml is installed, you can confirm your installation by checking the version.
ocaml -version
Setting Up Git
Git is a vital tool for managing version control in software projects, including OCaml. With features like branching, merging, and collaboration through remote repositories, it is an indispensable part of software development.
Installation Guide:
-
Installing Git: Depending on your platform, installation methods vary:
- For Windows, download the Git installer from the [Git website](https://git-scm.com/download/win).
- For macOS, you can use Homebrew:
brew install git
- For Linux, use the package manager:
sudo apt install git
-
Verification of Installation: Once installed, ensure Git is working by checking the version:
git --version
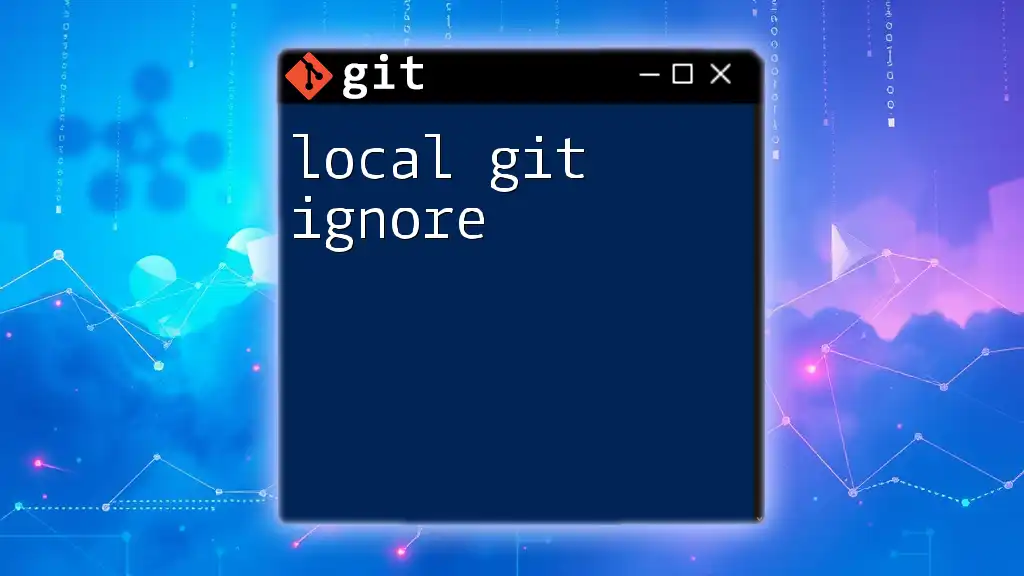
Creating an OCaml Project
Initializing a Git Repository
After setting up your environment, the next step is to create an OCaml project and initialize a Git repository to track its evolution. This process is essential in keeping a comprehensive history of changes and collaborates smoothly with others.
Example Commands to Create a Repository:
mkdir my_ocaml_project
cd my_ocaml_project
git init
This command sets up a new directory named `my_ocaml_project` and initializes it as a Git repository.
Organizing Your OCaml Project
The structure of your OCaml project plays a crucial role in its maintainability and scalability. A well-organized project can help you and others understand the code better and enhance collaboration.
A common structure for an OCaml project might include:
my_ocaml_project/
├── src/
│ └── main.ml
├── lib/
└── test/
- `src/`: Contains the main source code of your application, including implementation files.
- `lib/`: May store reusable modules and libraries that can be shared across different projects.
- `test/`: This directory can hold test cases and related files.
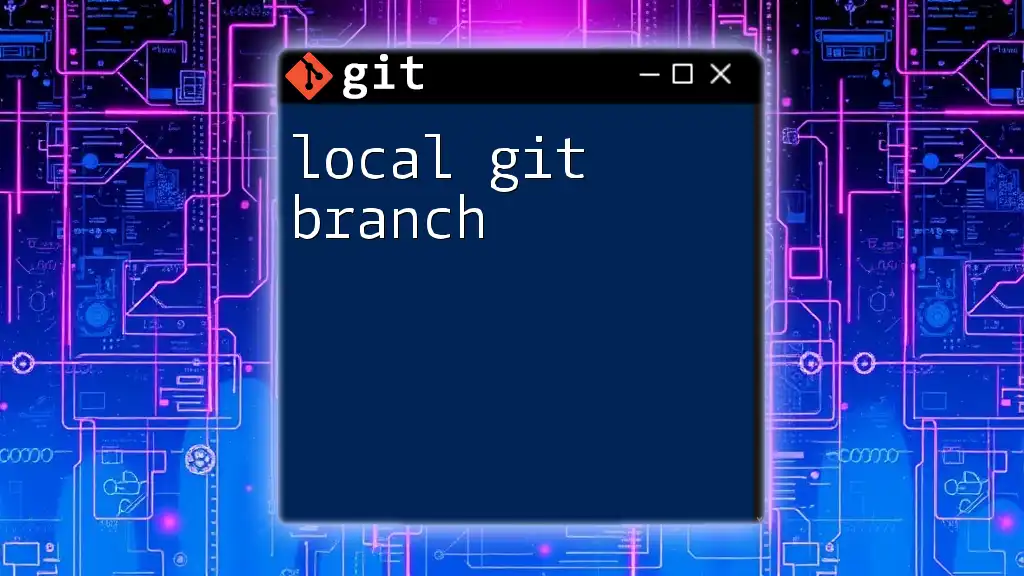
Making Your First Commit
Staging Changes
Once you have organized your files and made some changes, the next step is to stage your files. Staging is a way of marking changes that you want to include in your next commit.
Example Command to Stage Files:
git add src/main.ml
This command tells Git to track changes made to `main.ml`. You can continue to add other files in a similar manner.
Committing Changes
Committing your staged changes creates a snapshot of your current work. This is where a clear and concise commit message becomes crucial. Good messages help you and others understand the purpose of the changes at a glance.
Example Command for a Commit:
git commit -m "Initial commit: add main.ml"
Here, `-m` specifies the message that describes what this commit includes. This practice ensures proper documentation of your project’s evolution.
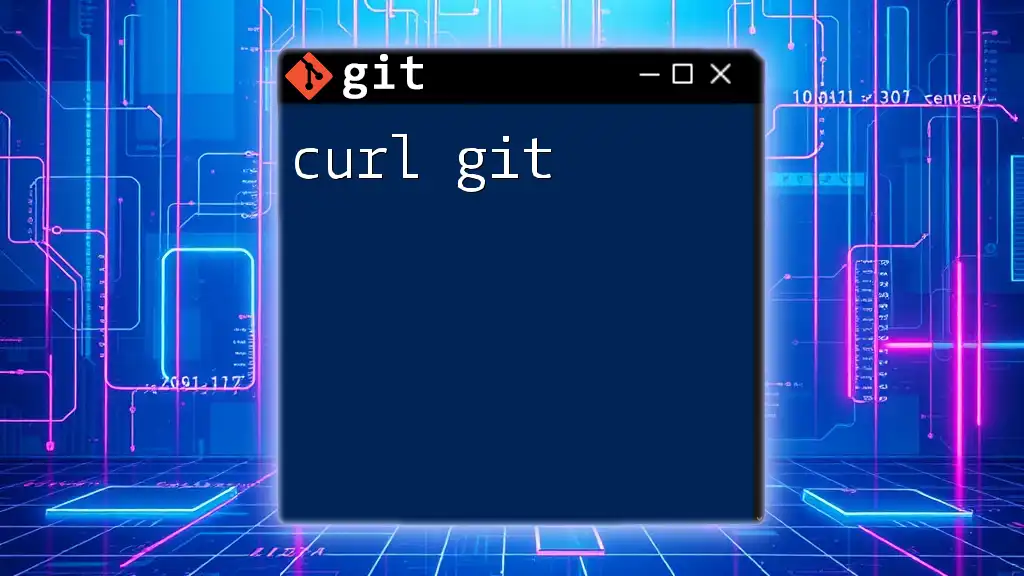
Branching in OCaml Projects
Why Use Branches?
Branches allow developers to work on new features or bug fixes without affecting the main codebase. This isolation prevents unfinished work from impacting the main application.
Example Commands to Create and Switch to a New Branch:
git branch new_feature
git checkout new_feature
Coming up with meaningful names for your branches can help you maintain clarity in your workflow.
Merging Branches
Once you finish working on a feature in a separate branch, you'll want to integrate those changes back into the main branch.
Example Commands for Merging:
git checkout main
git merge new_feature
On executing these commands, Git will attempt to merge the changes from the `new_feature` branch into the `main` branch.
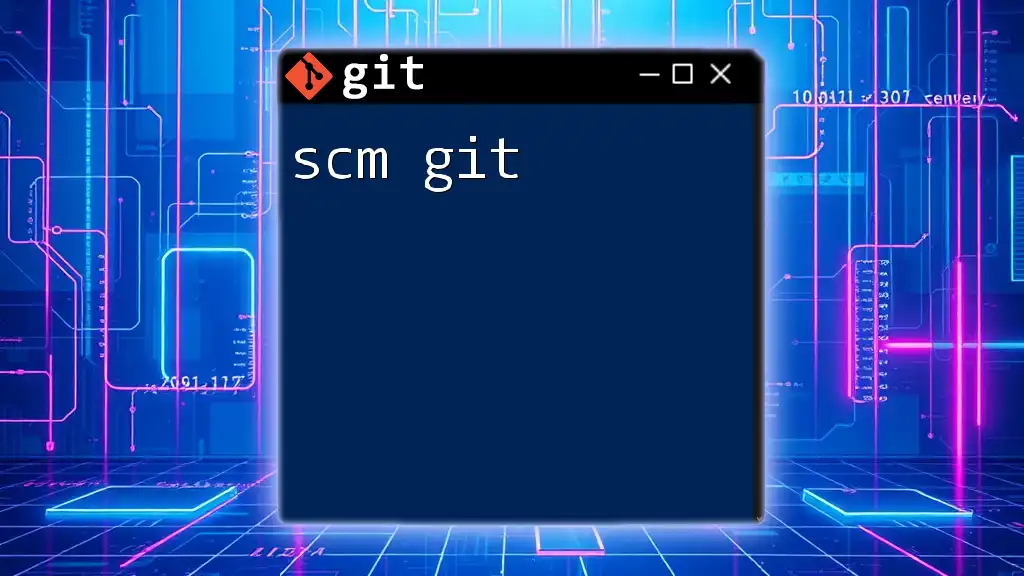
Working with Remote Repositories
Setting Up a Remote
Integrating Git with remote repositories allows you to collaborate effectively with others. Services like GitHub, GitLab, and Bitbucket provide platforms to host your repositories.
Example Command to Add a Remote:
git remote add origin https://github.com/username/my_ocaml_project.git
This command associates your local repository with the remote repository hosted on GitHub.
Pushing and Pulling Changes
Once your remote is set up, you'll frequently push your changes to the remote repository to keep your collaborators updated.
Pushing to a Remote Repository:
git push -u origin main
The `-u` option sets the upstream for your local branch to track the remote branch, making future pushes easier.
Pulling Changes from Remote Repository:
git pull origin main
This command fetches changes from the `main` branch on your remote repository and merges them into your local branch.
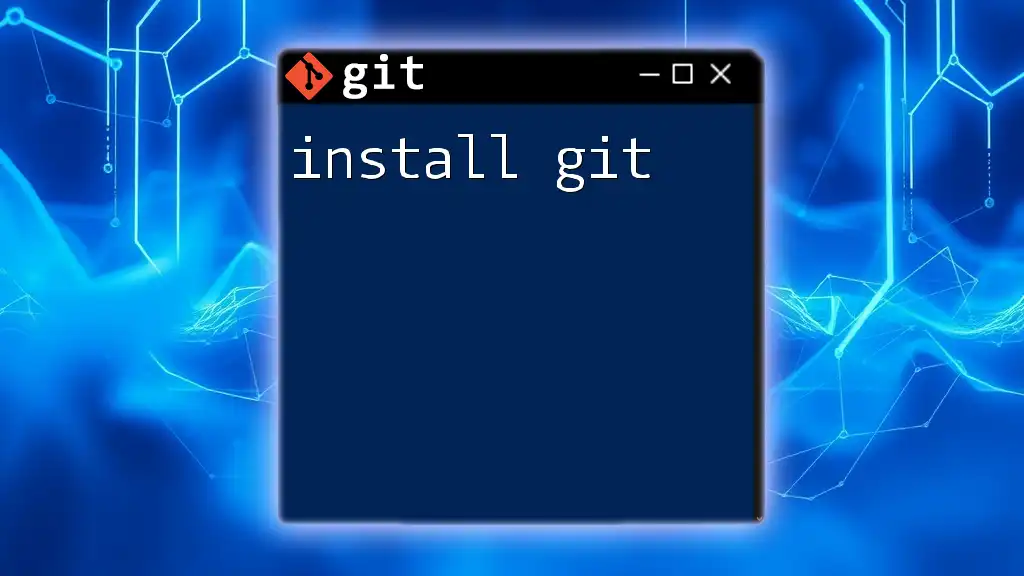
Handling Merge Conflicts
Understanding Merge Conflicts
A merge conflict occurs when multiple developers modify the same part of a file in different branches. Understanding how to resolve these conflicts is essential for smooth collaboration.
Resolving Merge Conflicts
When conflicts arise, Git will notify you, allowing you to address them:
-
Identifying Conflicting Files: Run:
git status
This command will list files that need resolution.
-
Manual Conflict Resolution: Open the conflicting files in an editor, look for `<<<<<<< HEAD` indicators, and edit the code to resolve the conflict.
-
Staging and Committing Resolved Changes:
git add <resolved_file>
git commit -m "Resolved merge conflict"
This process finalizes the resolution of your conflicts and allows you to continue working seamlessly.
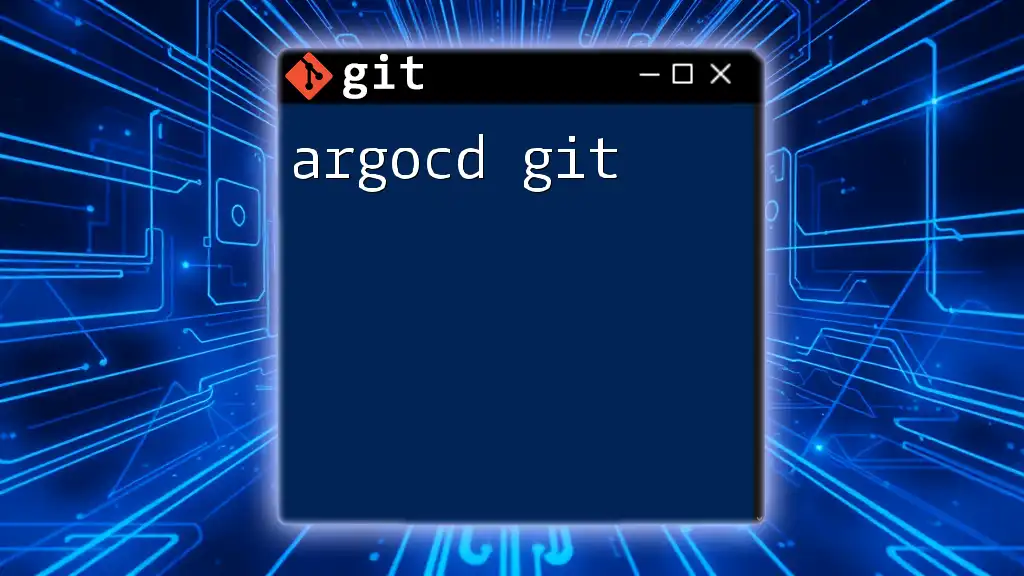
Best Practices for Using Git with OCaml
Writing Good Commit Messages
Crafting precise and descriptive commit messages helps others understand your intentions easily. Commit messages should answer the questions: What? Why? and How?
Regularly Syncing with the Remote
To ensure your work is consistent and backed up, regularly sync your local repository with the remote. Using commands like `git fetch` allows you to review changes without merging them, while `git status` helps check if your branch is up-to-date.
Effective Branching Strategies
Consider adopting models like Feature Branching or Git Flow. Feature branching isolates each new feature, while Git Flow incorporates strict rules for branch management, promoting a cleaner development process.
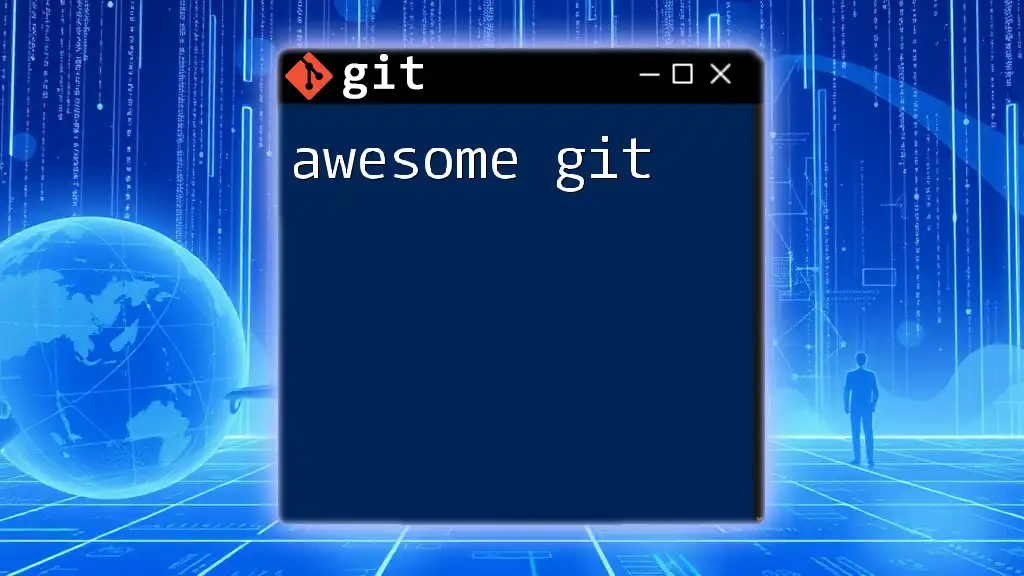
Conclusion
Using Git effectively with OCaml can greatly enhance your development experience, enabling you to manage changes more efficiently while collaborating seamlessly with others. Adopting the best practices outlined in this guide will not only aid your personal projects but also prepare you for working on larger OCaml codebases.
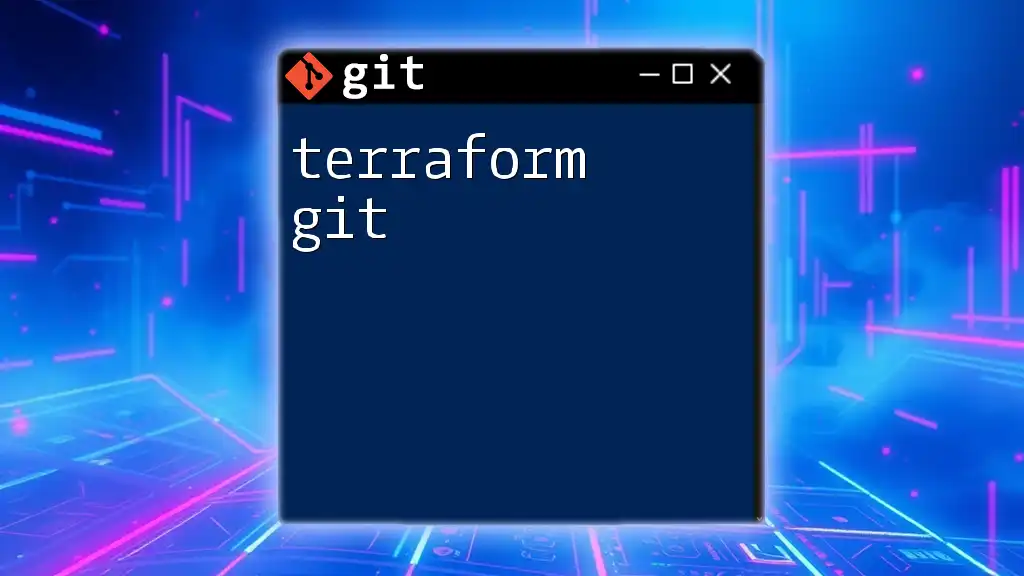
Additional Resources
For further reading and resources, be sure to check:
- The official OCaml documentation for advanced language features.
- Comprehensive Git tutorials to solidify your version control skills.
- Community forums where you can seek assistance and share experiences.
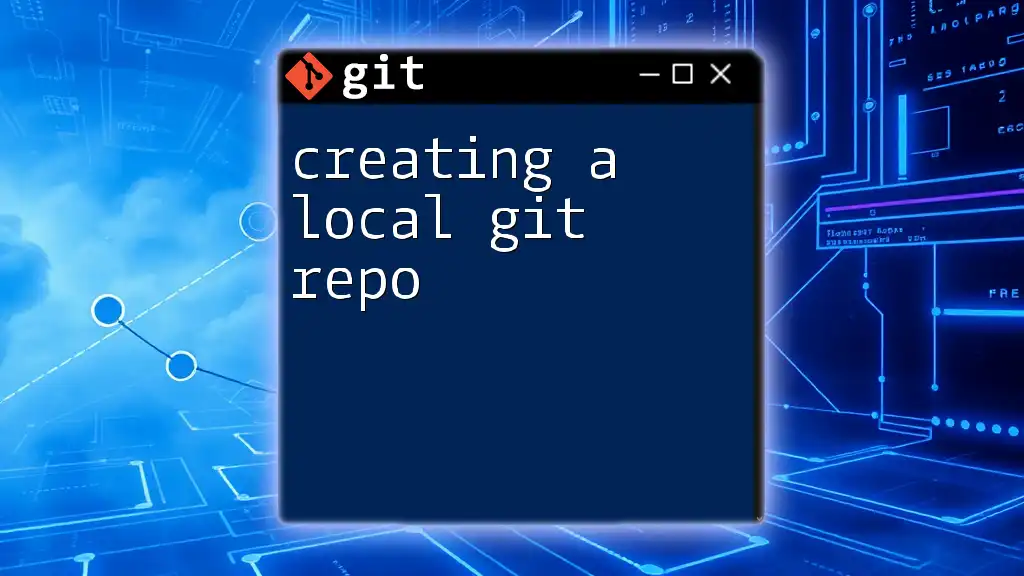
Code Examples Repository
For practical reference, check out this GitHub repository containing all the command examples discussed in this guide. This repository serves as a codebase that you can refer back to as you navigate your OCaml and Git journey.