A local `.gitignore` file allows you to specify files and directories that Git should ignore for tracking, ensuring that unnecessary items do not clutter your repository.
# Create a .gitignore file and add files to ignore
echo "node_modules/" >> .gitignore
echo "*.log" >> .gitignore
Understanding the Purpose of `.gitignore`
What is `.gitignore`?
The `.gitignore` file is a crucial element in any Git repository. It is a text file that specifies intentionally untracked files which Git should ignore. This functionality is essential to maintain a clean version control system by preventing unwanted files from cluttering the repository. Typically, this includes files created by your development environment or OS that aren't needed in your project's versioning.
Why Use a Local `.gitignore`?
Utilizing a local `.gitignore` has several advantages. It helps prevent the accidental inclusion of personal or sensitive data, like API keys or temporary files, in your commits. Moreover, it keeps the repository lightweight by ignoring files that are irrelevant to the project. Common use cases include ignoring local development environments' settings (like IDE configurations) or build artifacts that are automatically generated and should not be shared.
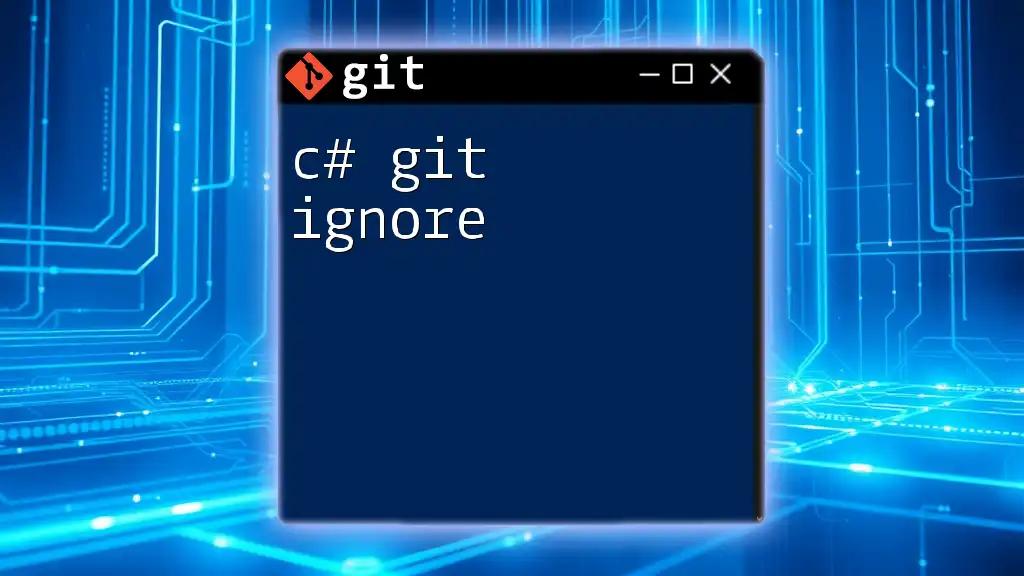
Creating a Local `.gitignore` File
Step-by-Step Guide to Create a Local `.gitignore`
Creating a local `.gitignore` file is simple. You can do this directly from your terminal or through your favorite text editor.
To create it via the terminal, navigate to your project directory and run:
touch .gitignore
If you prefer to manually create it, just open your text editor, create a new file, and save it as `.gitignore`.
Where to Place the `.gitignore` File
The `.gitignore` file should be located in the root directory of your repository. If your project has multiple folders with different purposes, consider placing additional `.gitignore` files in subfolders. However, keep in mind that global rules apply to the entire repository, while local rules can be specified per folder.
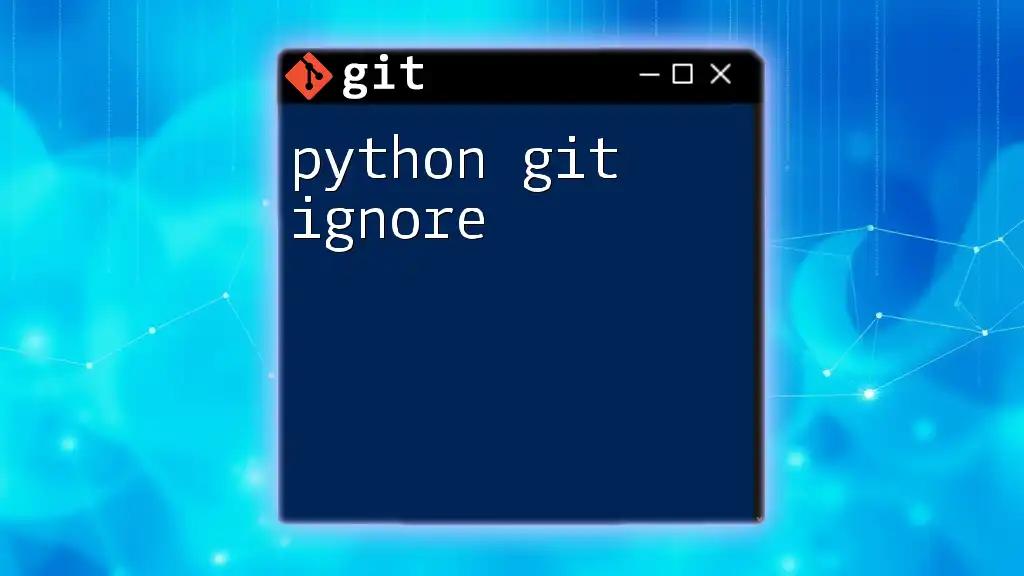
Syntax and Patterns in `.gitignore`
Basic Syntax of `.gitignore`
The syntax for a `.gitignore` file is straightforward. Each line in this file represents a rule and can include patterns for file and directory names. Lines that begin with `#` are comments and are ignored.
Here’s a basic structure:
# Ignore all .log files
*.log
# Ignore the directory folder
temp/
Using Wildcards and Patterns
Wildcards are powerful tools in `.gitignore`, allowing you to specify groups of files with similar naming conventions.
- `*` matches any string of characters
- `?` matches any single character
- `[]` matches any single character within the brackets
For example, to ignore all files with a `.tmp` extension:
*.tmp
If you want to ignore any file that starts with `temp` and has any other characters following it:
temp*
Negation Patterns
Sometimes you may want to include a file that would otherwise be ignored. This is where negation patterns come into play. You can prefix a pattern with an exclamation mark `!` to exclude it from being ignored. For instance:
# Ignore everything in logs/
logs/*
!logs/keep.log
In this case, all files in the logs directory will be ignored except for `keep.log`, which will still be tracked.
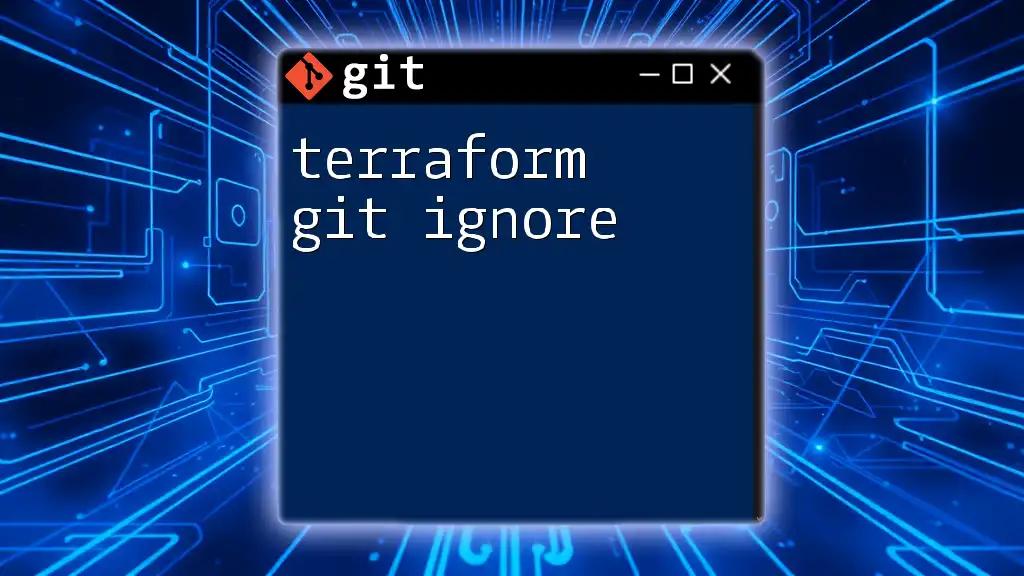
Examples of Common Local `.gitignore` Configurations
Ignoring OS Generated Files
Operating Systems often create hidden files that aren’t beneficial for your repository. Common examples include `.DS_Store` (macOS) and `Thumbs.db` (Windows). You can easily ignore these with the following entries in your `.gitignore`:
# Ignore macOS specific files
.DS_Store
# Ignore Windows specific files
Thumbs.db
Ignoring IDE and Editor Specific Files
Various Integrated Development Environments (IDEs) generate configuration files that are personal and unnecessary for other developers. For instance, if you're using Visual Studio Code, consider adding:
# Ignore VS Code settings
.vscode/
If you're using IntelliJ IDEA, you might want to include:
# Ignore IntelliJ IDEA project files
.idea/
Ignoring Build and Dependency Files
When working on a project, many files and folders should not be included in your commits, particularly built files and dependencies. For example, in JavaScript projects, you would likely want to ignore the `node_modules` directory:
# Ignore node modules
node_modules/
Similarly, in other environments, the build artifacts could include folders such as `dist` or `build`:
# Ignore build artifacts
dist/
build/
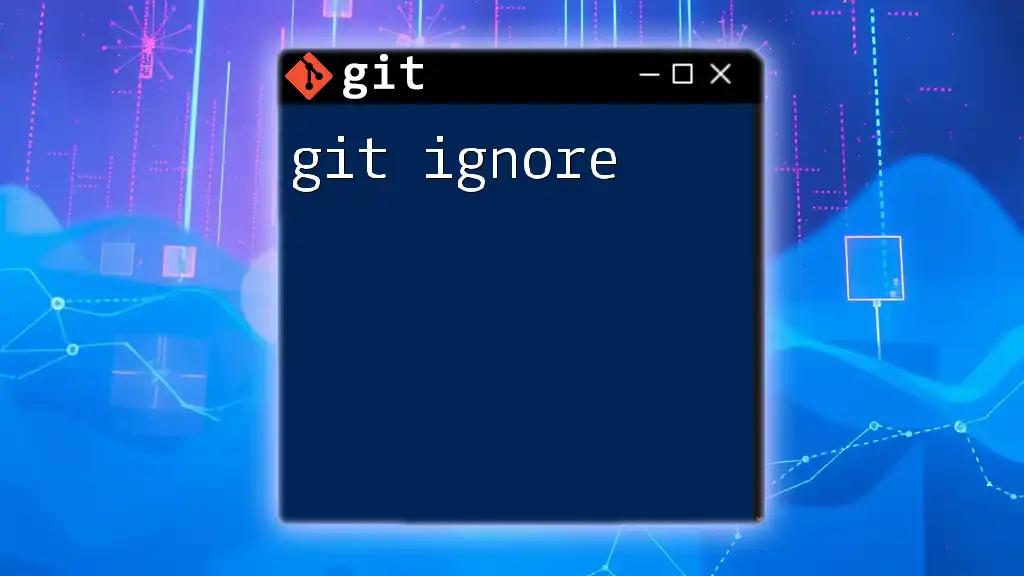
Managing Your Local `.gitignore`
Tips for Maintaining Your `.gitignore`
To ensure your `.gitignore` remains effective, regularly review and update your ignore rules, especially as your project develops and changes. This practice prevents unnecessary files from being inadvertently tracked.
Troubleshooting Ignored Files
If you find that files you expect to be ignored are still appearing in your commits, you can use the `git check-ignore` command to troubleshoot this issue. For example:
git check-ignore -v filename
This command will show the specific rule from your `.gitignore` that is affecting the `filename`. If you need to track a file again, you must remove it from being ignored in your `.gitignore` and stage it again.
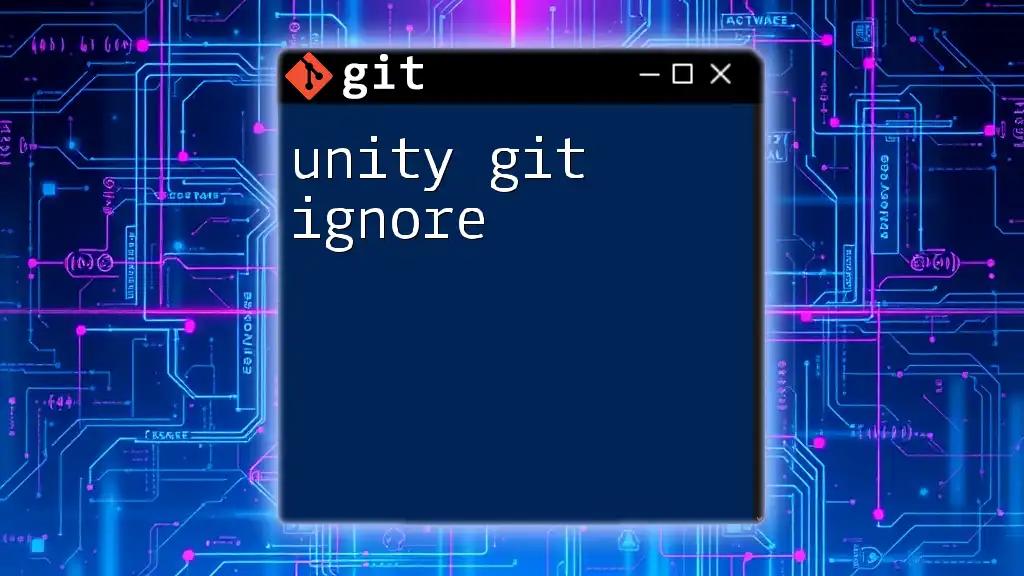
Advanced Usage of `.gitignore`
Global `.gitignore` for User-Specific Settings
A global `.gitignore` is an effective way to maintain personal ignore rules across all your repositories. You can create a global ignore file and configure Git to use it by running:
git config --global core.excludesfile '~/.gitignore_global'
In this file, you can add rules for files and directories that you always want to ignore, such as OS files, IDE configurations, etc.
Using `.gitignore` in Submodules
When working with Git submodules, you can enforce a similar `.gitignore` file within each submodule. It is essential because a global `.gitignore` does not apply to them. Just create a `.gitignore` file in each submodule as you would in a regular repository, ensuring that each submodule maintains its clean state.
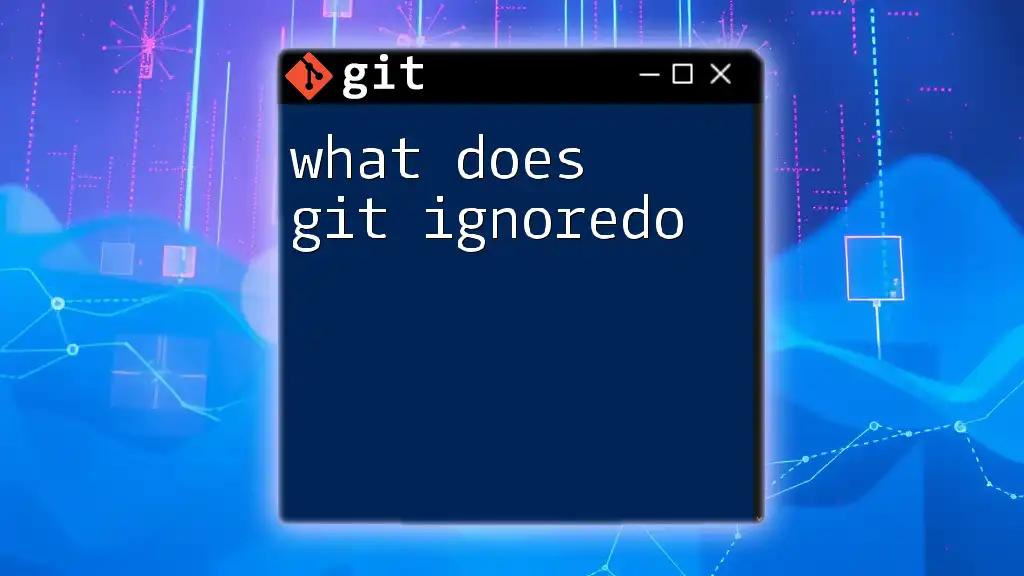
Conclusion
Recap of Key Points
The local `.gitignore` file plays an essential role in managing your project's cleanliness by preventing unwanted files from being tracked in your repository. Employing effective strategies for `.gitignore` management allows you to maintain control over what is committed, ultimately leading to a more efficient and organized development process.
Final Tips for Git Users
To maximize the effectiveness of your local `.gitignore`, take the time to establish clear rules tailored to your project needs. Regular reviews of your `.gitignore` can also help prevent clutter. Finally, consider exploring additional resources and guides to deepen your understanding of Git and its vast capabilities.