In Visual Studio Code (VSCode), you can instruct Git to ignore specific files or directories by creating a `.gitignore` file in your repository and listing the patterns of files you want to exclude.
Here’s a code snippet to create a simple `.gitignore` file that ignores all `.log` files and the `node_modules` directory:
echo -e "*.log\nnode_modules/" > .gitignore
Understanding `.gitignore`
What is `.gitignore`?
The `.gitignore` file is a simple, text file created in the root directory of a Git repository. Its primary purpose is to specify files and directories that should be ignored by Git. These are typically files that do not need to be tracked, such as temporary files, build artifacts, and sensitive data (like configuration files containing secrets). The effective use of the `.gitignore` file can significantly reduce clutter in your repository and keeps it clean and organized.
Why Use `.gitignore` Files?
When you ignore certain files or directories, you prevent them from being added to the version control history. Ignoring files is essential for several reasons:
- Maintaining a Clean Repository: By ignoring files that are not necessary for version control (like compiled code or cache files), you keep the repository tidy, which facilitates collaboration.
- Preventing Credential Leaks: Sensitive files, such as API keys or configuration files, should not be included for security reasons.
- Reducing Conflicts: When team members use personalized settings or machine-specific files, ignoring these helps minimize merge conflicts in collaborative projects.
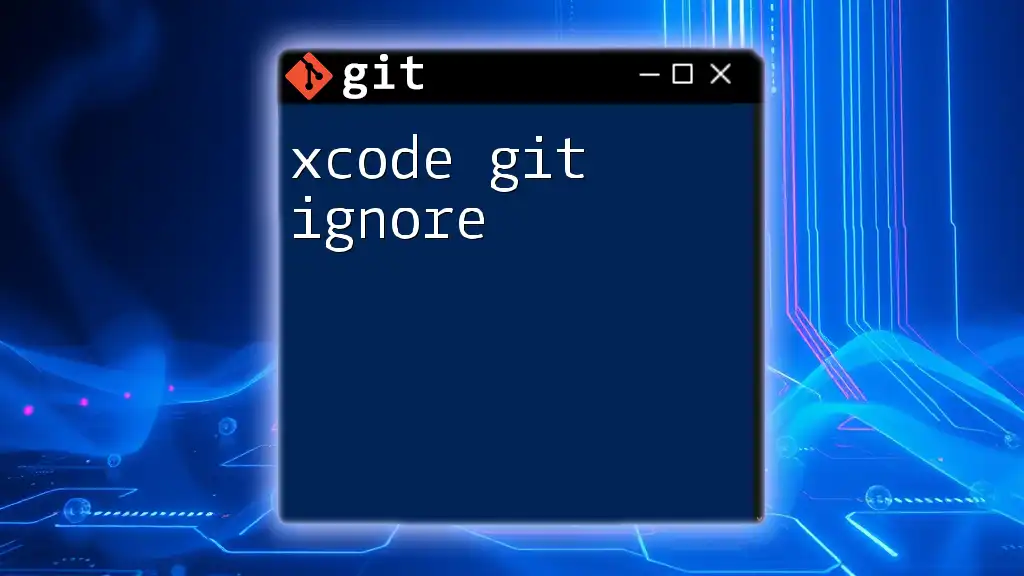
Creating a `.gitignore` File in VSCode
Steps to Create a `.gitignore` File
Creating a `.gitignore` file in Visual Studio Code is straightforward:
- Open your project in VSCode.
- In the Explorer sidebar, right-click on the root directory of your project.
- Select New File and name it `.gitignore`.
You can also create it using the terminal within VSCode:
touch .gitignore
Editing the `.gitignore` File
To edit the `.gitignore` file, simply double-click on the newly created file in VSCode. The editor supports syntax highlighting, making it easier to read and edit. This is particularly helpful when dealing with complex rules.
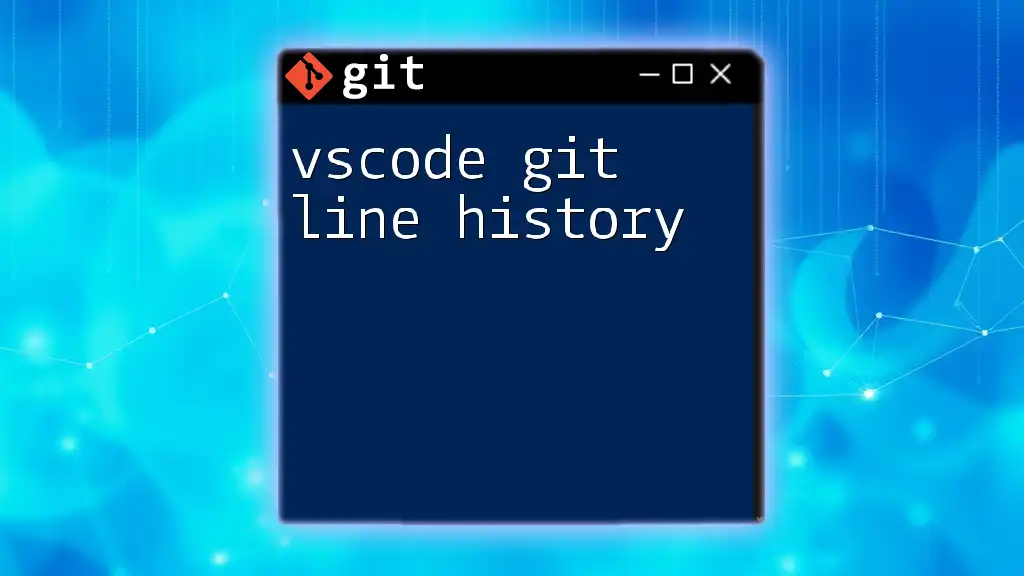
Structure of a `.gitignore` File
Basic Syntax and Patterns
The syntax of a `.gitignore` file is relatively straightforward. Here are the main components:
- Wildcards: Use `` as a wildcard to match multiple files. For example, `.log` ignores all files with the `.log` extension.
- Negation: Prefixing a pattern with `!` inverts its effect, allowing you to track specific files within ignored directories.
- Directory-specific ignores: Use `/` to specify directories clearly. For instance, `/build/` ignores the `build` directory only when it is in the root.
Here are some examples to illustrate these points:
# Ignore all .log files
*.log
# Ignore a specific directory
/build/
# Do not ignore this particular file, even if its folder is ignored
!important.txt
Specific Use Cases
Ignoring OS Files
Operating systems often generate files that are unnecessary for version control. For instance, macOS creates a file called `.DS_Store` in directories to store custom attributes. To ignore such files, include them in your `.gitignore`:
# Ignore macOS files
.DS_Store
Ignoring IDE/Editor Files
Many Integrated Development Environments (IDEs) and editors create settings files which may not be useful to other developers. For example, VSCode creates a `.vscode` folder with various settings. To ignore these settings, add the following to your `.gitignore`:
# Ignore VSCode settings
.vscode/
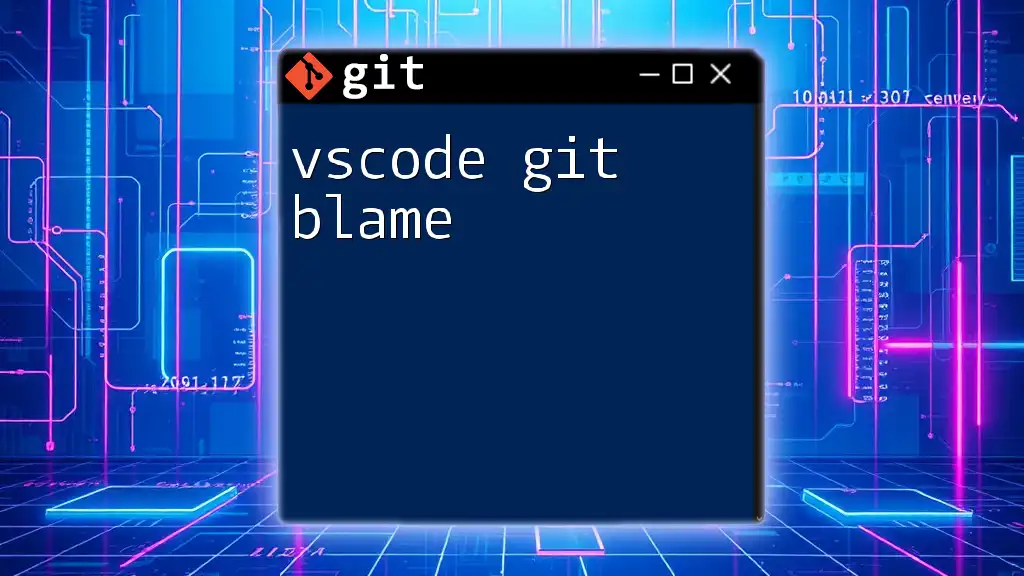
Integrating `.gitignore` with VSCode Extensions
Popular Extensions for Managing `.gitignore`
There are several VSCode extensions designed to simplify the management of `.gitignore` files. Two popular options include:
- GitIgnore: This extension allows for easy creation and editing of `.gitignore` files using templates specific to various programming languages and frameworks.
- GitLens: Not only does this enhance Git capabilities within VSCode, but it also provides some suggestions related to `.gitignore` best practices.
Utilizing these extensions can greatly increase productivity and help you stay organized.
Using Git Template for Common Projects
When starting new projects, it can be beneficial to use a pre-defined `.gitignore` template tailored to your project type (Node.js, Python, etc.). Websites like GitHub maintain repositories with curated `.gitignore` files for various technologies. Accessing these templates can save time and ensure that you cover all necessary files to ignore for your specific stack.
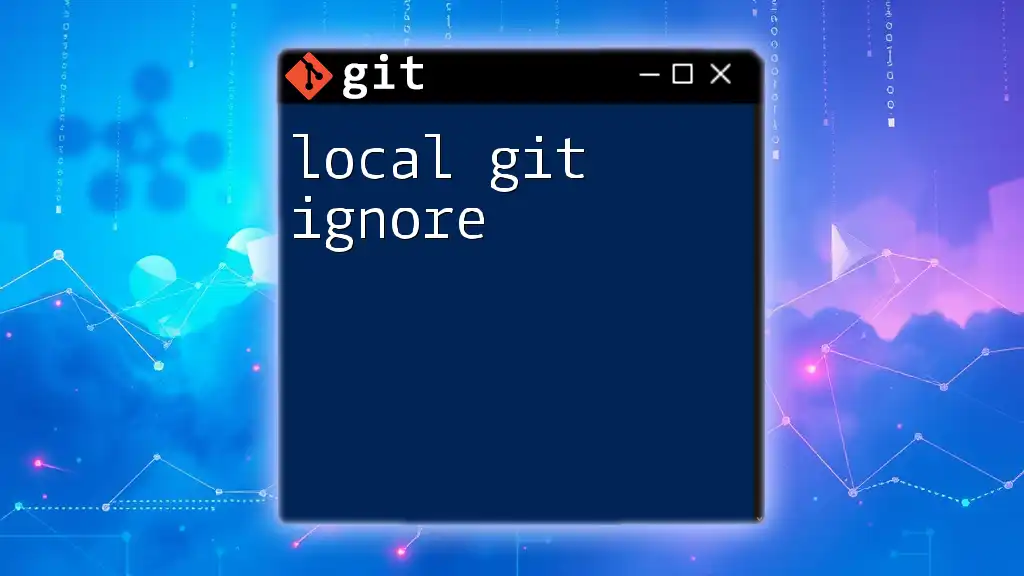
Verifying Your `.gitignore` Effectiveness
Checking Ignored Files
To check if `.gitignore` is functioning correctly, you can use the following command in your terminal:
git check-ignore -v path/to/file
This command will show you whether a particular file is being ignored and, if so, which rule in the `.gitignore` file is causing it.
Troubleshooting Common Issues
One common issue is when a file that should be ignored is still being tracked. If you encounter this, it may be because the file was added before you created your `.gitignore`. To resolve this, you can use the following commands:
git rm --cached path/to/file
This command stops tracking that file in Git while keeping it in your local directory.
Additionally, the order of rules in your `.gitignore` file matters; Git processes them from top to bottom, so ensure that more general rules are placed before specific ones.
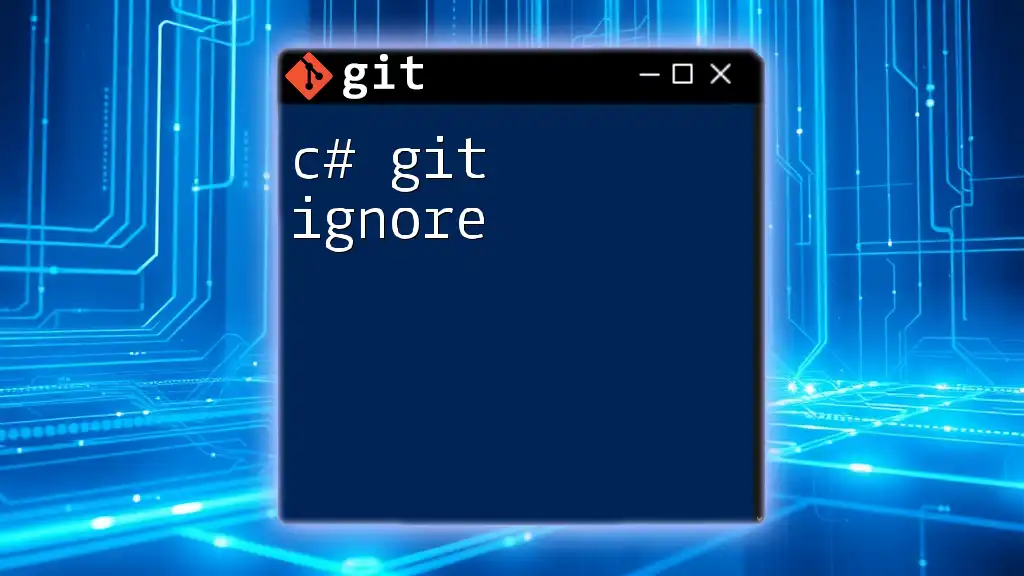
Best Practices for `.gitignore` Files
Keeping Your `.gitignore` File Updated
As your project evolves, it's essential to keep your `.gitignore` file current. Regularly review and modify the file based on new dependencies, generated files, or changes in project structure.
Sharing `.gitignore` with the Team
In collaborative environments, sharing a common `.gitignore` file helps ensure consistency within the team. Include a `.gitignore` in your repository's root directory so that all team members use the same rules, minimizing confusion and potential tracking errors.
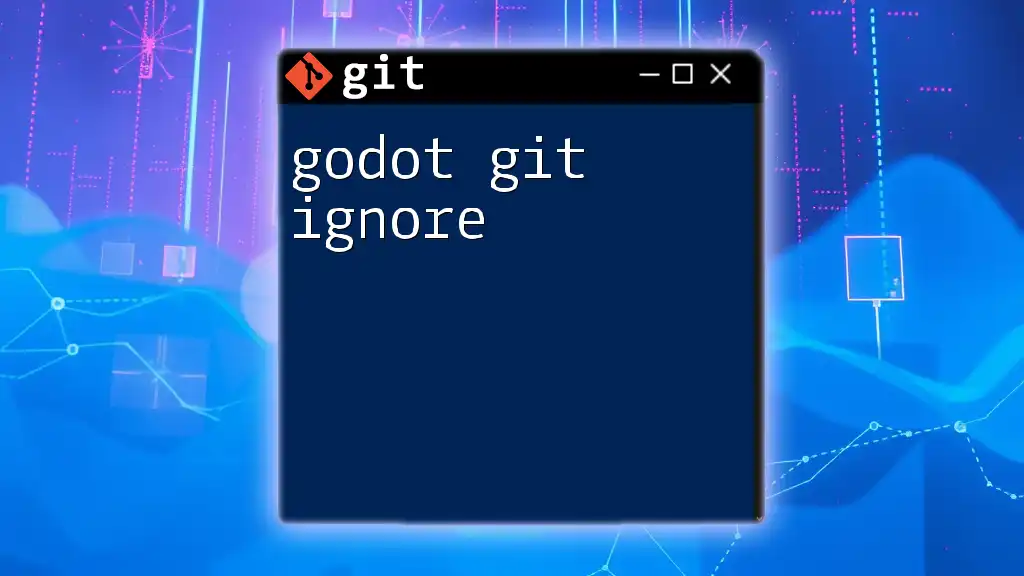
Conclusion
Incorporating a well-structured `.gitignore` file into your VSCode workflow is crucial for maintaining a clean and organized Git repository. By following the practices outlined in this guide, you will help ensure that your projects are easier to manage and free of unnecessary clutter.
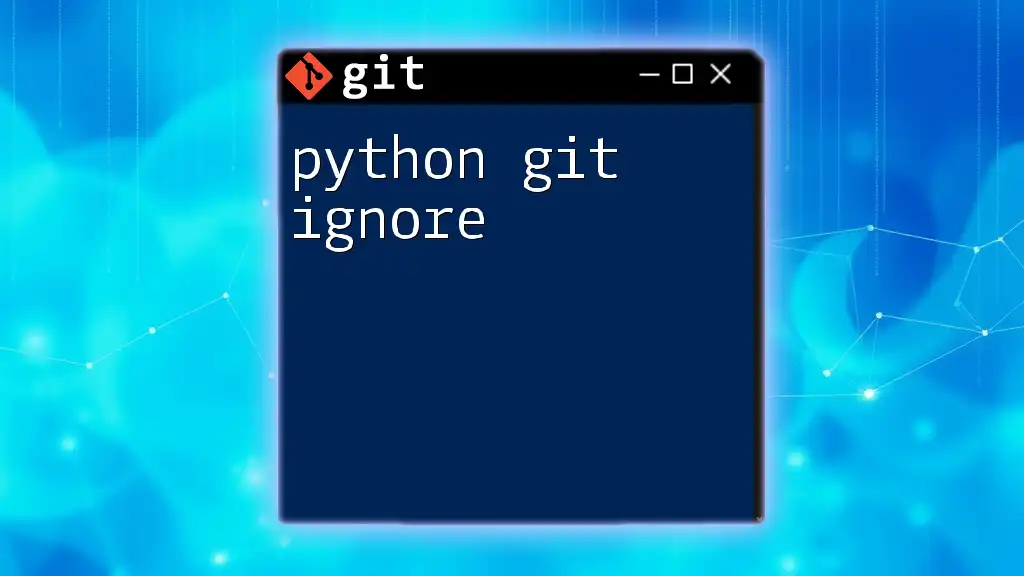
Additional Resources
For further information, check out the official Git documentation on `.gitignore` and explore curated `.gitignore` templates on GitHub. These resources can greatly assist you in tailoring your version control strategies effectively.
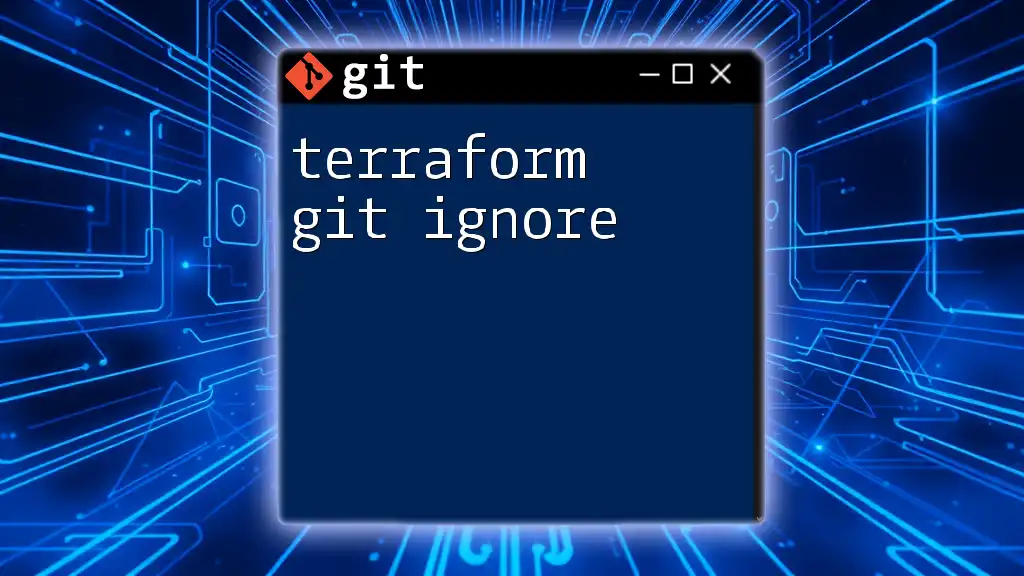
Call to Action
If you found this guide helpful, consider signing up for more resources and guides from our company to enhance your Git knowledge. Share this article with fellow developers who can benefit from a better understanding of managing `.gitignore` files in VSCode!