Visual Studio Code (VSCode) seamlessly integrates Git, allowing you to manage version control efficiently with simple commands directly from the editor.
Here's a quick command to initialize a new Git repository:
git init my-new-repo
Setting Up Visual Studio Code for Git
To begin your journey with vscode git, the first step is to ensure that both Visual Studio Code and Git are installed properly.
Installing VSCode
You can download Visual Studio Code from its [official website](https://code.visualstudio.com/Download), which provides builds for different operating systems. Make sure to follow the installation instructions specific to your operating system to get everything set up correctly.
Installing Git
Next, it's essential to have Git installed. Here are the general steps:
- Windows: Download the Git installer from the [Git Downloads page](https://git-scm.com/download/win) and run it. Follow the on-screen prompts.
- macOS: You can install Git using Homebrew with the command:
brew install git
- Linux: Depending on your distribution, you might use:
sudo apt-get install git # For Debian-based systems sudo yum install git # For Red Hat-based systems
After installation, verify it by running the command:
git --version
Configuring Git in VSCode
With Git installed, you should now configure it:
Set your global username and email to ensure proper commit attribution:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
You can check your configuration by running:
git config --list
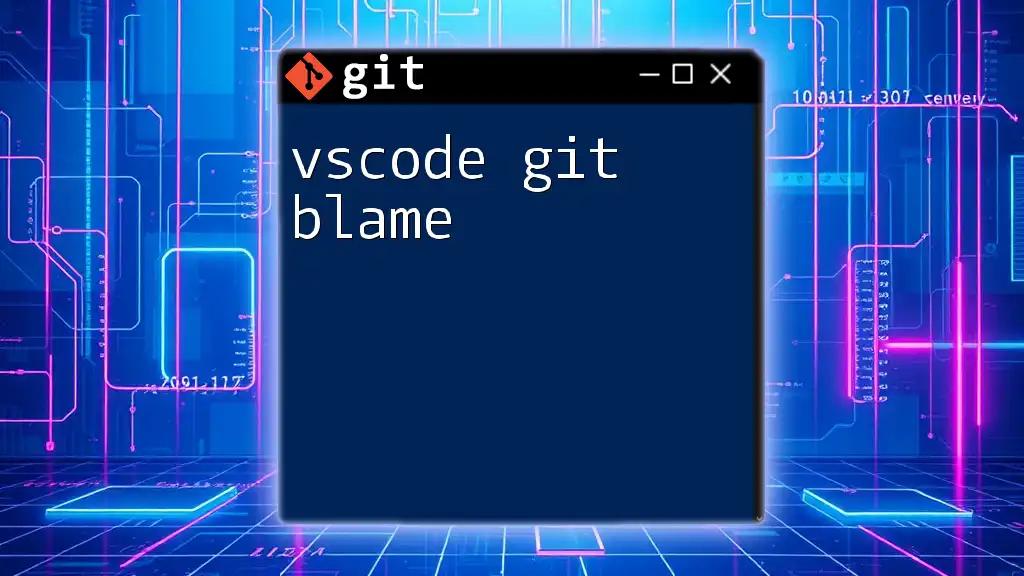
Navigating the Git Interface in VSCode
Visual Studio Code provides a user-friendly interface for managing Git repositories.
Git View Overview
Navigate to the Source Control icon in the Activity Bar on the side. This area displays your changes, staged changes, and the overall status of your repository, making it easy to see what needs attention.
Cloning a Repository
Cloning a repository is straightforward. Use the Command Palette (Ctrl + Shift + P or Cmd + Shift + P on Mac) and type Git: Clone. Enter the repository URL, and select a local directory where you want to clone your code. The repository will appear in your workspace.
git clone https://github.com/username/repo.git
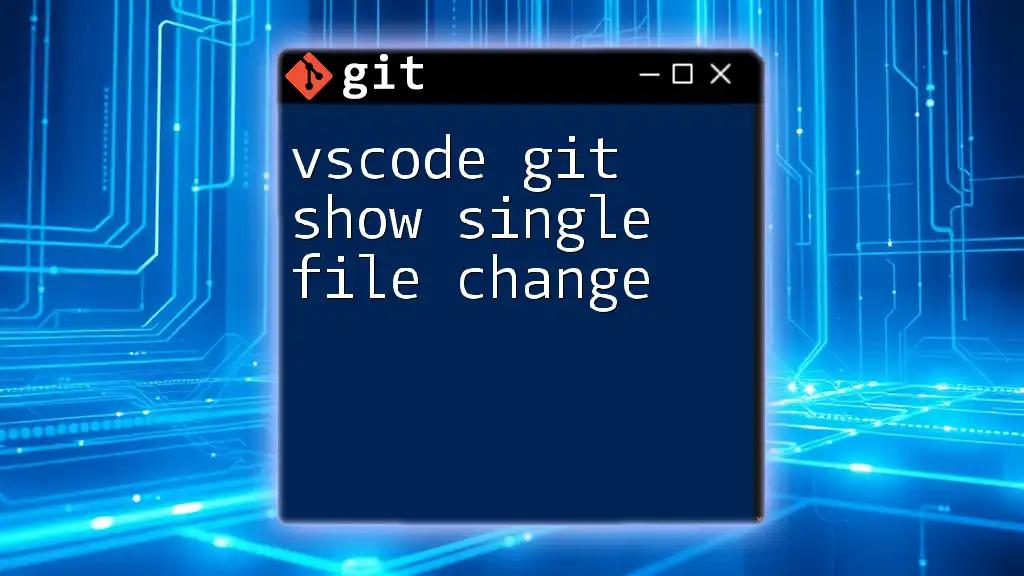
Basic Git Commands in VSCode
Creating a New Repository
To create a new Git repository within VSCode, navigate to your project folder in the terminal and run:
git init
This command initializes a new Git repository in your project directory, enabling version control.
Making Changes and Tracking Them
Modifying Files
You can edit files in your project directly within VSCode. Simply open the file, make your changes, and save it.
Staging Changes
After editing, you will need to stage your changes. This can be done via the Source Control view, where you can click the + (plus) icon next to the files to stage them. Alternatively, use the following command in the terminal to stage all changes:
git add .
Committing Changes
With your changes staged, it’s time to commit. You can either do this through the Source Control view by entering a commit message and clicking the checkmark icon, or you can use the terminal:
git commit -m "Your commit message here"
Important Tip: Write clear and concise commit messages. They help in understanding the history of changes made to the project.
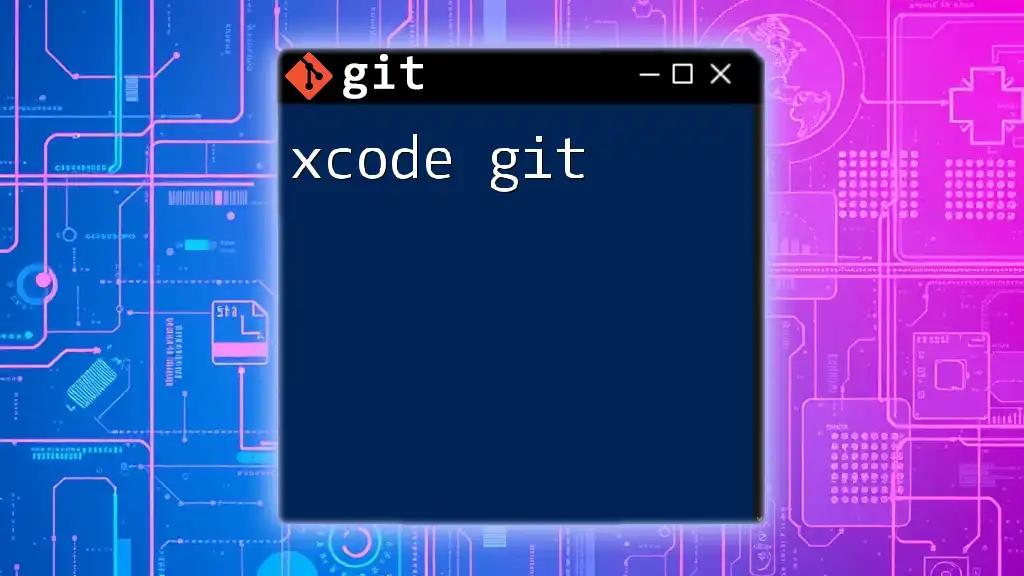
Branching and Merging
Understanding Branches
Branches in Git allow you to work on different versions of your project simultaneously, making it crucial to understand how to create and manage them.
Creating and Switching Branches
To create a new branch, use the terminal or the Source Control view:
git branch your-branch-name
git checkout your-branch-name
You can also combine these steps with:
git checkout -b your-branch-name
This command creates and switches to the new branch in one go.
Merging Branches
When you're ready to merge changes from another branch back into your main branch, ensure you are on the main branch first:
git checkout main
Then execute:
git merge your-branch-name
This merges the specified branch into your current branch. If there are conflicts, VSCode will highlight them in your editor, making it easier to resolve.
Deleting and Renaming Branches
Should you want to delete or rename branches, you can do so with these commands:
git branch -d branch-name # To delete
git branch -m old-name new-name # To rename
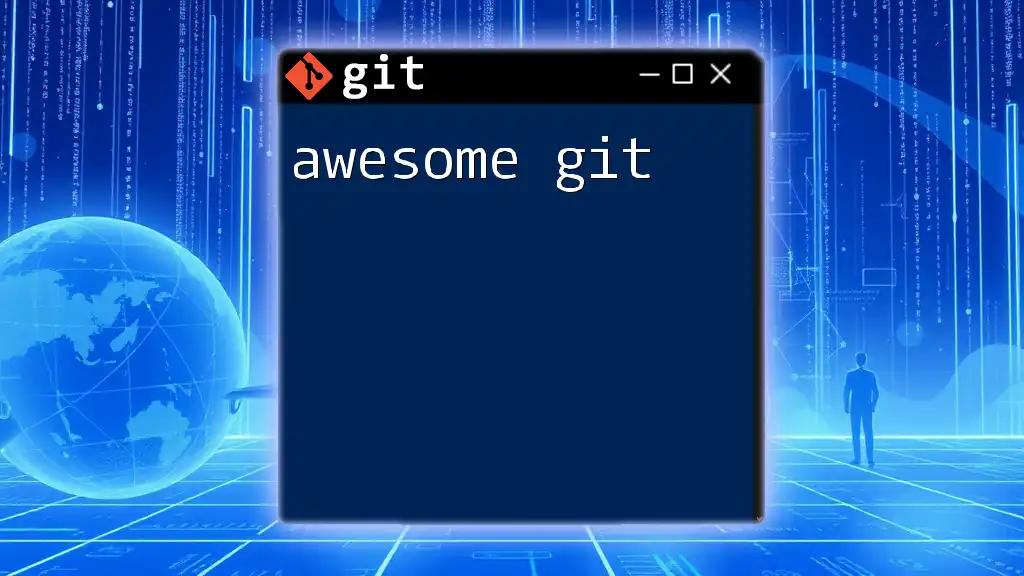
Viewing Repository History
Accessing Git History
VSCode allows you to view your commit history easily. In the Source Control view, you can see past commits and their associated messages. Click a commit to view its changes, or use the command:
git log
Comparing Changes
To compare differences between commits or branches, you can use the built-in diff viewer in VSCode. By right-clicking on a commit, you can select Compare with Previous Commit to see what changes were made.
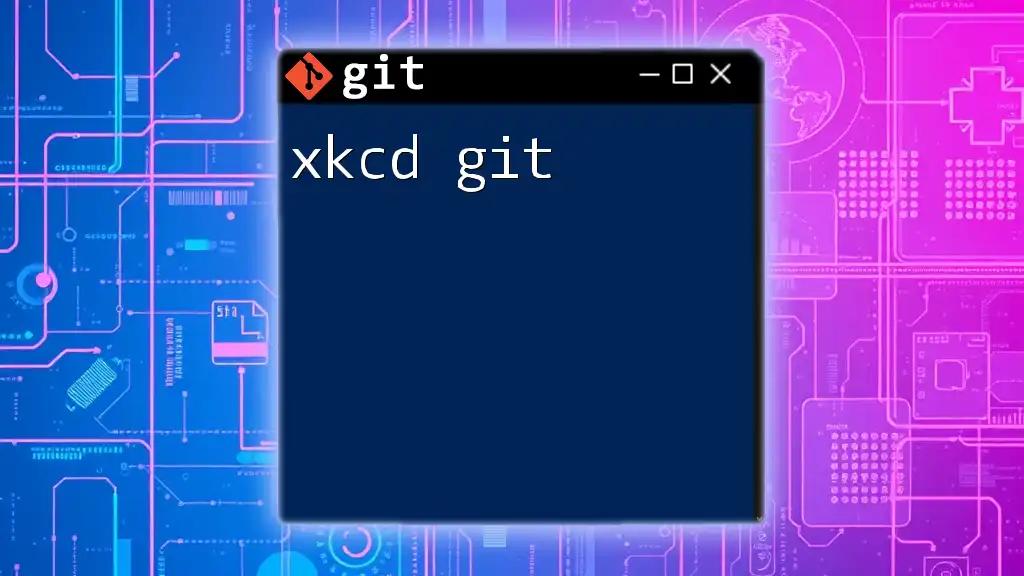
Remote Repositories
Adding a Remote Repository
To collaborate with others, you often work with remote repositories. To add a remote repository, use:
git remote add origin https://github.com/username/repo.git
This command creates a pointer to the remote repository.
Pushing Changes to Remote
Once you've committed your changes locally, you need to push them to the remote repository:
git push origin main
This command updates the remote repository with your committed changes.
Pulling Changes from Remote
To ensure your local repository is up-to-date with the remote, you would pull any changes with:
git pull origin main
This command fetches and merges changes from the remote repository to your local branch.
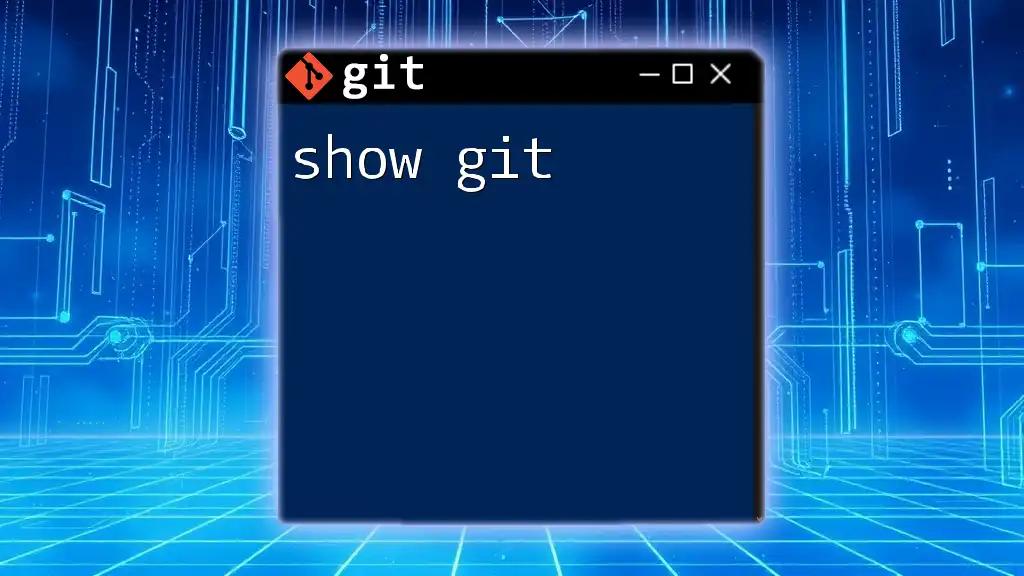
Troubleshooting Common Issues
Common Git Errors and Solutions
While working with Git in VSCode, you might encounter some common errors:
- Merge Conflicts: If two branches have conflicting changes, VSCode will highlight the conflicts, allowing you to resolve them directly in the editor.
- Authentication Issues: If you experience problems pushing to the remote, check your credentials and ensure you're authorized to access the repository.
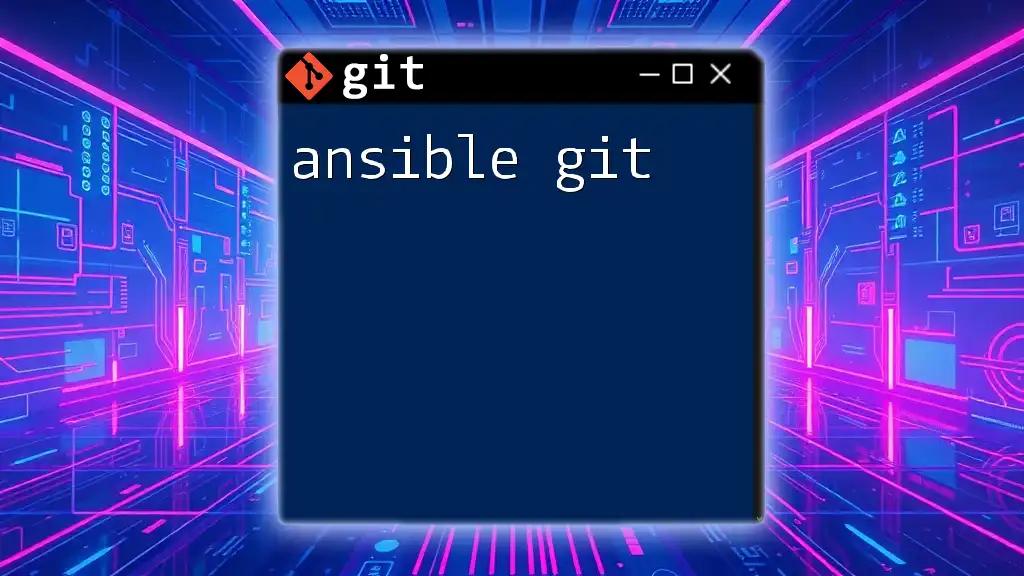
Best Practices for Using Git in VSCode
To make the most of vscode git, adhere to these best practices:
- Clear Commit Messages: Always communicate what changes were made in your commit messages. This aids future developers and collaborators.
- Regularly Push Changes: Pushing your changes frequently keeps your remote repository updated and mitigates the risk of losing work.
- Organize Your Repository: Keep your branches tidy and delete those that are no longer needed. This helps in maintaining a clean project structure.
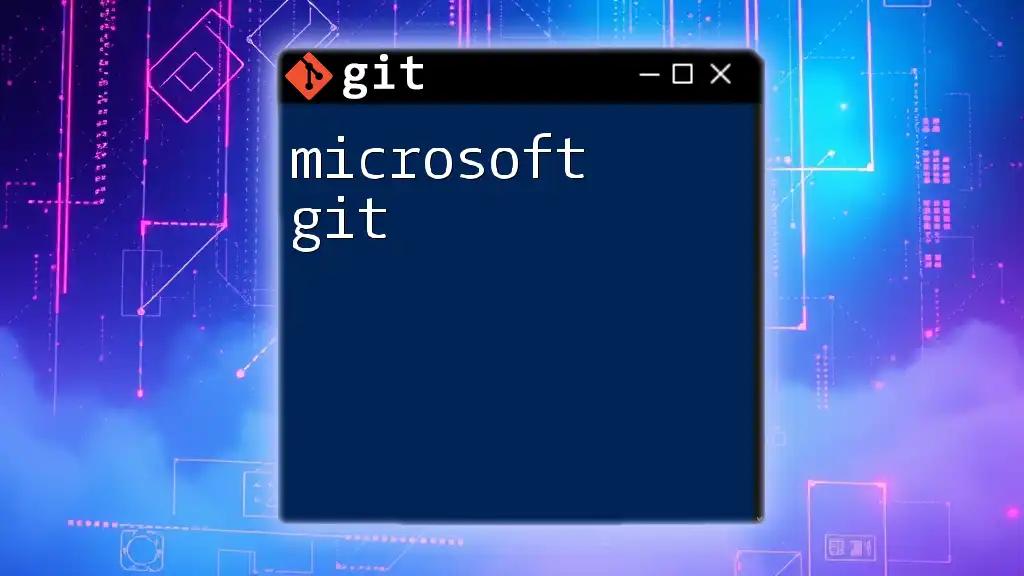
Conclusion
Using vscode git effectively can enhance your development workflow significantly. By mastering these commands and processes, you can manage your codebase with confidence and efficiency. Practice regularly, and explore more features in Git and VSCode to become proficient in version control and collaboration.
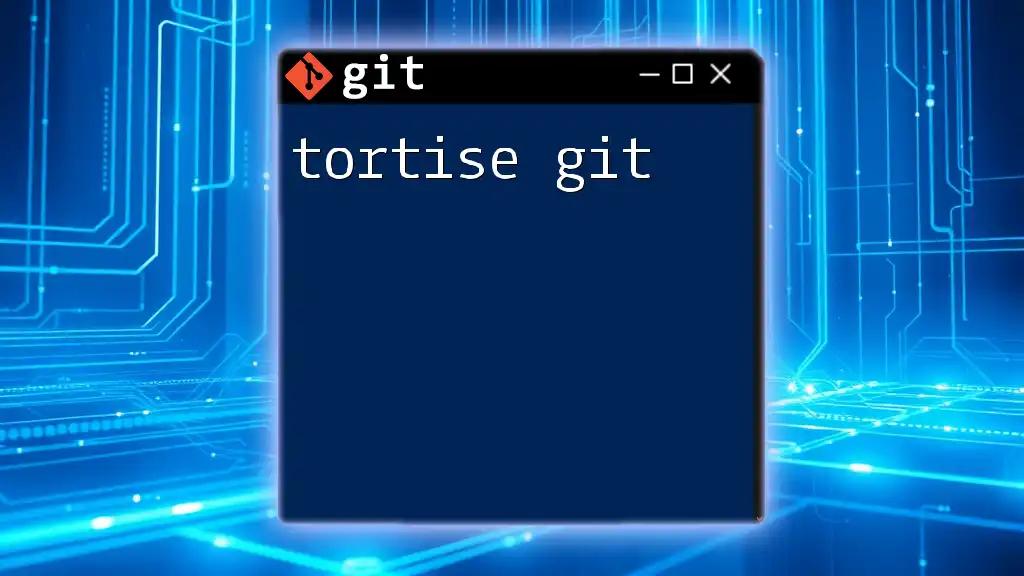
Additional Resources
For further learning, refer to the official Git documentation and explore extensions in VSCode that enhance Git management. The internet is full of tutorials, courses, and resources where you can deepen your knowledge of both Git and Visual Studio Code.