CI/CD, or Continuous Integration/Continuous Deployment, in the context of Git involves automating the process of integrating code changes and deploying applications to ensure faster development cycles while maintaining code quality.
Here’s a simple Git command snippet often used in CI/CD pipelines:
git commit -m "Deploying changes to production" && git push origin main
What is CI/CD?
Continuous Integration (CI) refers to the practice of automatically integrating code changes from multiple contributors into a shared repository multiple times a day. The goal is to ensure that integration happens frequently, allowing teams to detect and fix integration problems early in the development process.
Continuous Delivery (CD) builds upon CI by ensuring that the code is always in a deployable state and can be released to production at any time. Continuous Deployment, on the other hand, goes a step further by automatically deploying every code change that passes the automated tests, without manual intervention.
The importance of CI/CD in modern software development cannot be overstated. It enables faster delivery of features, more efficient collaboration among teams, and, ultimately, a better experience for users.
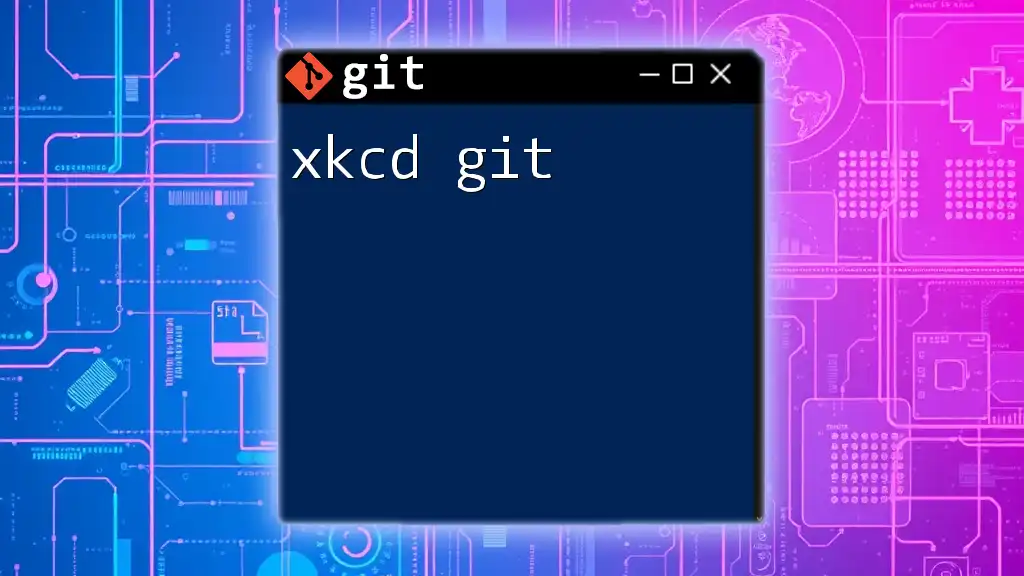
Why Use CI/CD with Git?
Integrating Git into your CI/CD pipeline brings several significant benefits. First, Git provides a robust version control system that tracks changes in your code. This makes collaboration smoother as multiple developers can work on different features simultaneously without conflicts.
Second, CI/CD enhances automation, which minimizes human errors during the build, test, and deployment processes. This automation fosters a culture of quality, where code changes are continuously validated through automated tests.
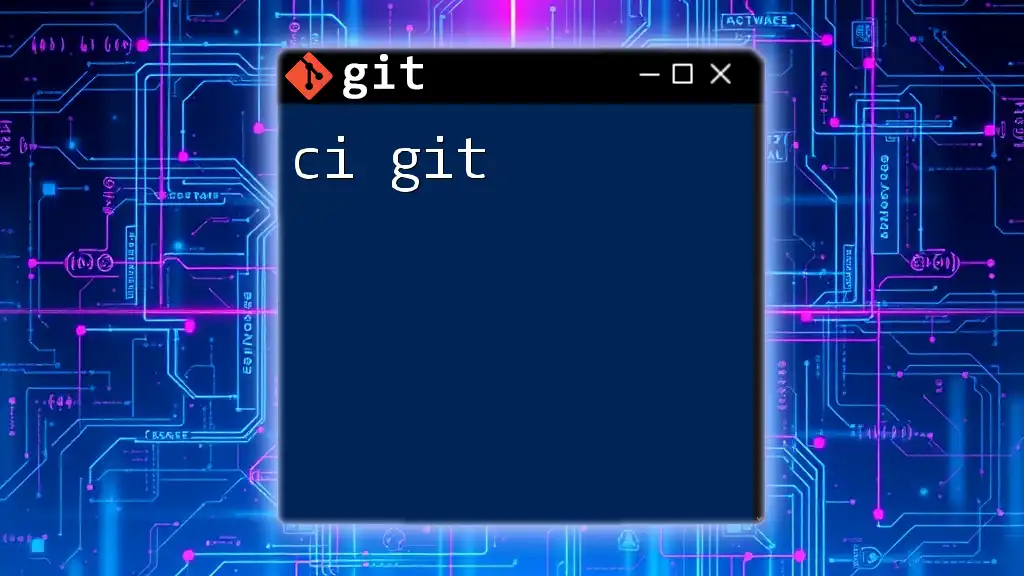
Setting Up Your Git Repository for CI/CD
Choosing the Right Git Hosting Platform
There are several popular Git hosting platforms to choose from, including GitHub, GitLab, and Bitbucket. Each has its own set of features, so it's important to evaluate which aligns best with your team's needs. Look for features such as built-in CI/CD capabilities, issue tracking, and integration with various software development tools.
Initializing Your Repository
Creating a new repository is the foundational step in setting up your CI/CD process. Here’s a quick guide to creating a repository on GitHub:
- Log in to your GitHub account.
- Click on the "New" button in the repositories section.
- Fill in the repository name and description.
- Choose to make it public or private.
- Click on "Create repository."
With your repository set up, you’ll want to start adding your code.
Best Practices for Structuring Your Repository
A well-structured repository eases the path to a smooth CI/CD implementation. Keep these best practices in mind:
- Organize your codebase logically, placing related files in appropriate directories.
- Implement a clear branching strategy. Use feature branches for new developments and hotfix branches for urgent updates.
- Example: Adopt a naming convention like `feature/awesome-feature` or `hotfix/fix-bug-123`.
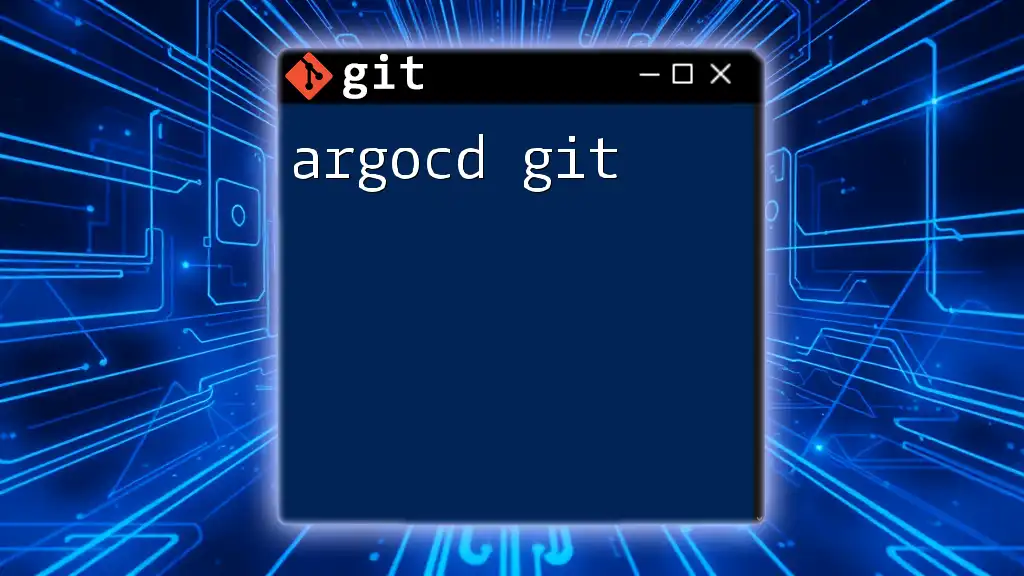
Integrating CI/CD Tools with Git
Popular CI/CD Tools for Git
Several CI/CD tools integrate seamlessly with Git. Some of the most popular ones include Travis CI, CircleCI, Jenkins, and GitHub Actions. When choosing a tool, consider essential factors like ease of integration, community support, and the density of documentation provided.
Setting Up a CI/CD Pipeline
Step 1: Configuring Your CI/CD Tool
Once you've chosen a CI/CD tool, you need to configure it for your repository. Here's how to set up Travis CI with a sample Python repository:
Create a `.travis.yml` file in the root of your repository with the following configuration:
language: python
python:
- "3.8"
script:
- python -m unittest discover
This configuration sets the language to Python and defines the testing command to run unit tests whenever changes are pushed to the repository.
Step 2: Writing Build Scripts
Writing effective build scripts is crucial for maximizing your CI/CD efficiency. Ensure the scripts are clear, concise, and documented. For a Node.js application, you might write a build script like this:
#!/bin/bash
# Install dependencies
npm install
# Run the build process
npm run build
Executing this script ensures that all dependencies are installed and the application builds correctly before deployment.
Automating Tests in Your CI/CD Pipeline
Automated tests are essential for a successful CI/CD pipeline. They help catch issues early, reducing costs and improving product quality. For instance, if you're using Jest for testing, your `package.json` might include a test script like this:
{
"scripts": {
"test": "jest"
}
}
When your CI tool runs this command during the pipeline, it executes your tests and reports any failures.
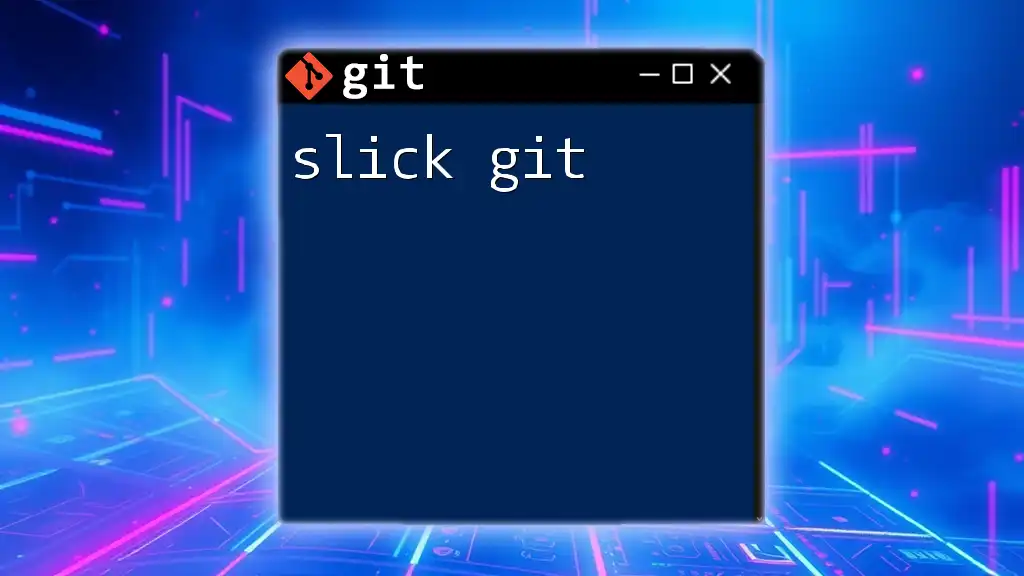
Continuous Delivery vs. Continuous Deployment
Understanding the Difference
Although the terms Continuous Delivery and Continuous Deployment are often used interchangeably, they have distinct meanings:
- Continuous Delivery means that your code is always in a deployable state, but requires manual approval before it goes live.
- Continuous Deployment automates the release process, so every change that passes the tests is automatically deployed to production.
Knowing when to use each approach depends on factors like team size, project complexity, and risk tolerance.
Implementing Continuous Delivery
Setting up a Continuous Delivery pipeline involves key components such as:
- Automated testing frameworks that ensure code quality.
- Deployment scripts that handle the complexities of deploying the application.
A practical example would involve configuring your CI/CD tool to deploy to a staging environment upon successful builds and tests, allowing team members to verify changes before they are released to production.
Implementing Continuous Deployment
To implement Continuous Deployment successfully, you must establish a robust suite of automated tests. The pipeline will automatically deploy every successful build directly to production, which requires a strong emphasis on quality control.
Establishing a rollback strategy is also a good practice. In the event of a failed deployment, having a mechanism to revert to a previous version ensures that your production environment remains stable.
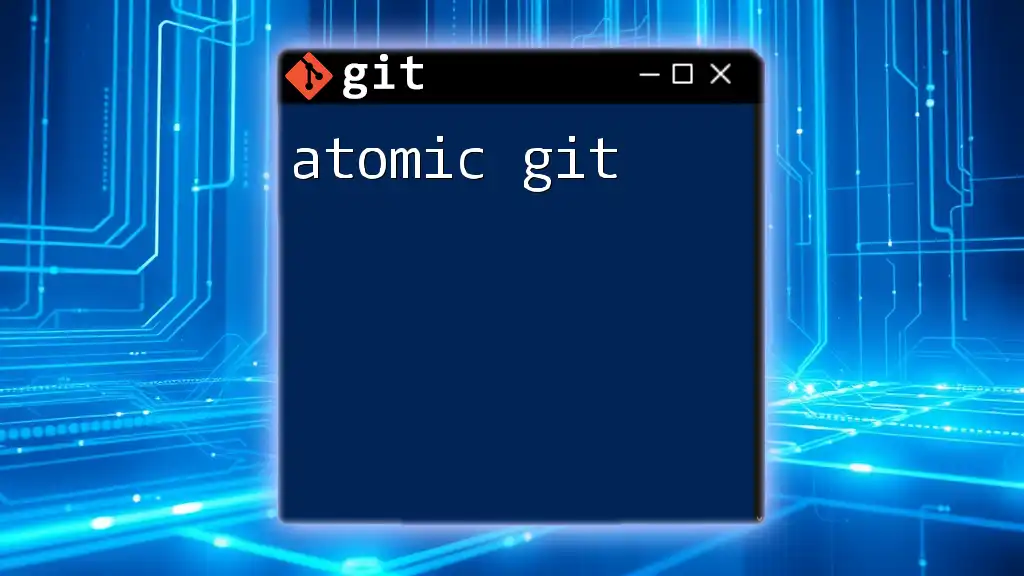
Best Practices for CI/CD with Git
Branching Strategies
A clear branching strategy is essential for successful collaboration and CI/CD implementation. Git Flow is a popular strategy that involves distinct branches for features, releases, and hotfixes, helping teams manage their tasks clearly.
Managing Secrets and Environment Variables
It’s crucial to manage sensitive data such as API keys securely within your CI/CD pipelines. Use tools like GitHub Secrets to store sensitive information safely, ensuring it’s only accessible by the CI/CD environment.
Monitoring and Logging
Monitoring your CI/CD pipeline is vital for quickly identifying and resolving issues. Implement tools that provide insights into build performance, test results, and deployment statuses. This visibility helps ensure confidence in the automated processes you’ve put in place.
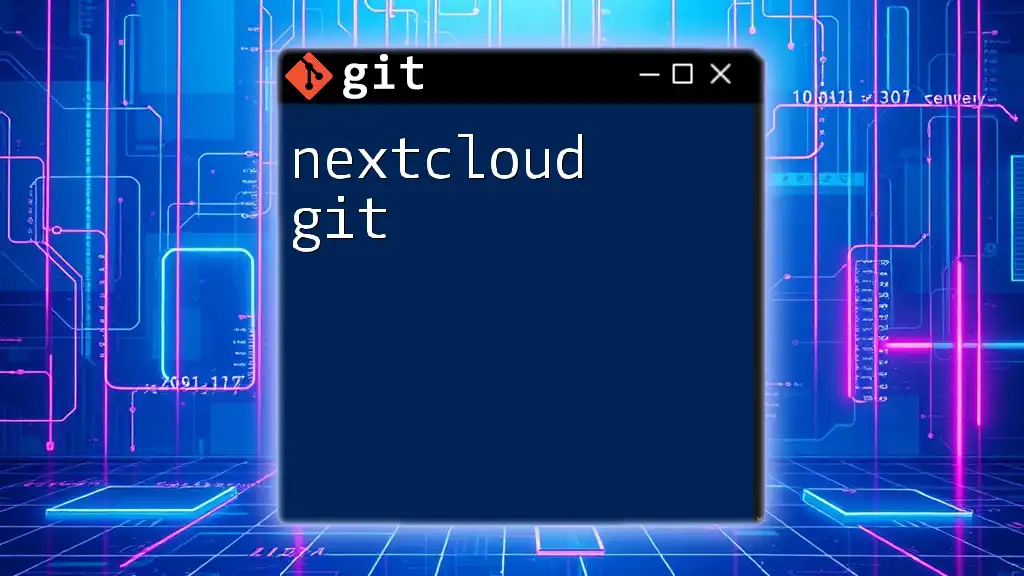
Troubleshooting CI/CD Issues
Common CI/CD Challenges
While implementing CI/CD with Git offers many advantages, it can also introduce challenges such as flaky tests, environment discrepancies, and integration failures. Understanding these common problems is the first step to effective resolution.
Debugging Your Pipeline
When a CI/CD process fails, debugging can be daunting. Start by examining the logs generated during the build and deployment processes. Most CI/CD tools provide logs that detail each step taken, making it easier to identify what went wrong.
# Example of a command to view logs
travis logs --job <job_id>
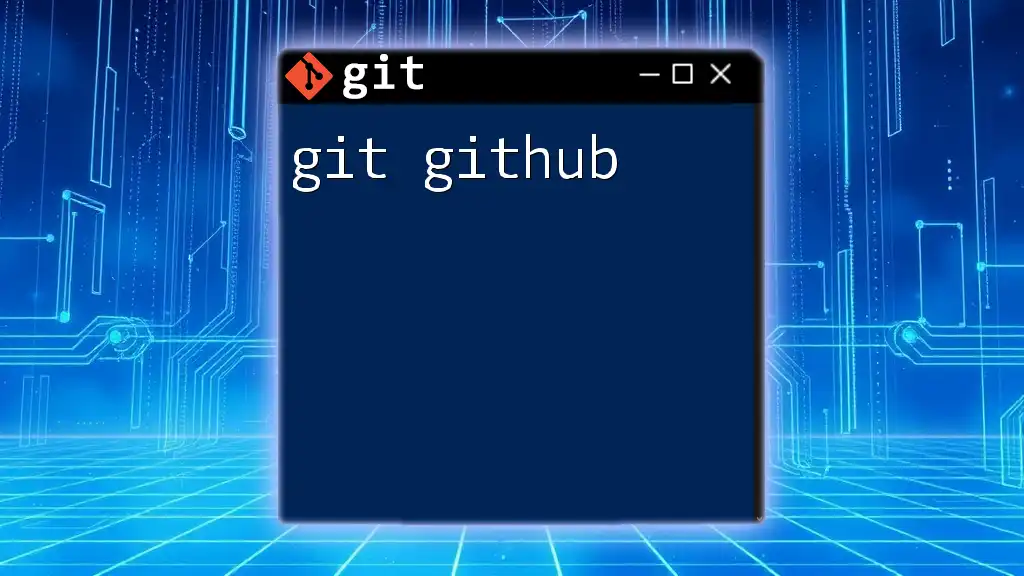
The Future of CI/CD with Git
The landscape of CI/CD is continually evolving. Staying current with trends, such as the adoption of Infrastructure as Code (IaC) and enhanced cloud capabilities, will be key to maintaining a competitive edge.
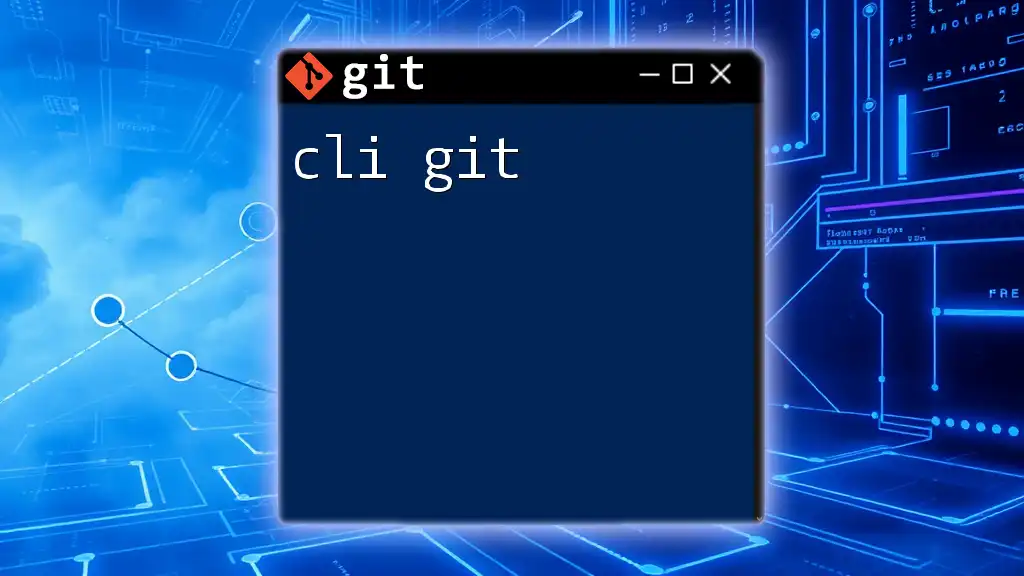
Final Thoughts
Incorporating CI/CD into your Git workflow dramatically enhances your software development cycle. It encourages better collaboration, increases code quality, and ensures faster delivery of features. As you explore the world of CI/CD with Git, remember that adaptability and continuous learning are vital in staying ahead in software development.