"React Git" refers to using Git version control to manage the code and collaborative development of React applications effectively.
Here’s a common Git command for initializing a new React app:
npx create-react-app my-app
cd my-app
git init
git add .
git commit -m "Initial commit of React app"
Setting Up Your Git Environment
Installing Git
To begin using Git, it is essential to have it installed on your system. Here are the steps:
- For Windows: Download the installer from [git-scm.com](https://git-scm.com/download/win) and follow the setup instructions.
- For Mac: If you have Homebrew installed, you can run the following command in your terminal:
brew install git
- For Linux: Use the package manager with this command:
sudo apt-get install git
After installation, you can confirm that Git is correctly installed by running:
git --version
Configuring Git
Configuring Git is the next step, and it's crucial because it provides identification for your commits. To set up your user information, use the following commands in your terminal:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
Make sure to replace `"Your Name"` and `"you@example.com"` with your actual details. This information will be used in the commit history, making it easier to identify contributions.
Choosing a Git Workflow
Understanding Git workflows is crucial for maintaining a clean and efficient process in your development projects. The most popular workflows include Feature Branch, which allows for isolating development activities, and Gitflow, which organizes larger projects into clear stages. For novice React developers, starting with a simple Feature Branch approach is often the best way to ensure clarity and ease of collaboration.
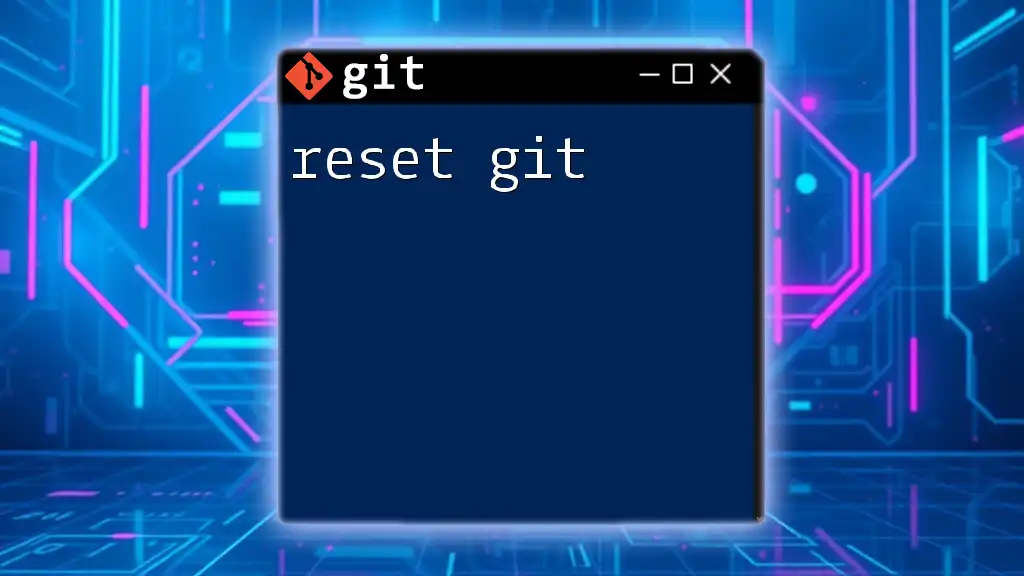
Basic Git Commands for React Projects
Initializing a Git Repository
Once Git is installed and configured, the first step in your React project is to initialize a repository. Navigate to your project directory in the terminal and run:
git init
This command creates a new Git repository and prepares it for version control.
Cloning a Repository
If you need to work on an existing React project, you can clone a repository. Just load the terminal and execute:
git clone https://github.com/username/repo.git
Replace `https://github.com/username/repo.git` with the URL of the repository you want to clone. This command creates a local copy of the remote repository on your machine.
Making Your First Commit
After working on your project, you will likely need to save your changes. First, stage the changes:
git add .
Then, commit those changes with a message describing what you've accomplished:
git commit -m "Initial commit with React setup"
Your commit message should be clear and concise, allowing others (and your future self) to understand the purpose of that commit.
Branching in Git
Branches in Git allow you to work on new features without affecting the main codebase. To create a new branch, use:
git branch new-feature
To switch to this branch, run:
git checkout new-feature
This creates an isolated environment for your feature, enabling you to develop independently from the main branch.
Merging Branches
Once your feature is complete, you’ll want to merge your changes back into the main branch. First, switch to the main branch:
git checkout main
Then, merge your feature branch into the main branch:
git merge new-feature
If everything goes smoothly, your changes are now integrated into the main codebase.
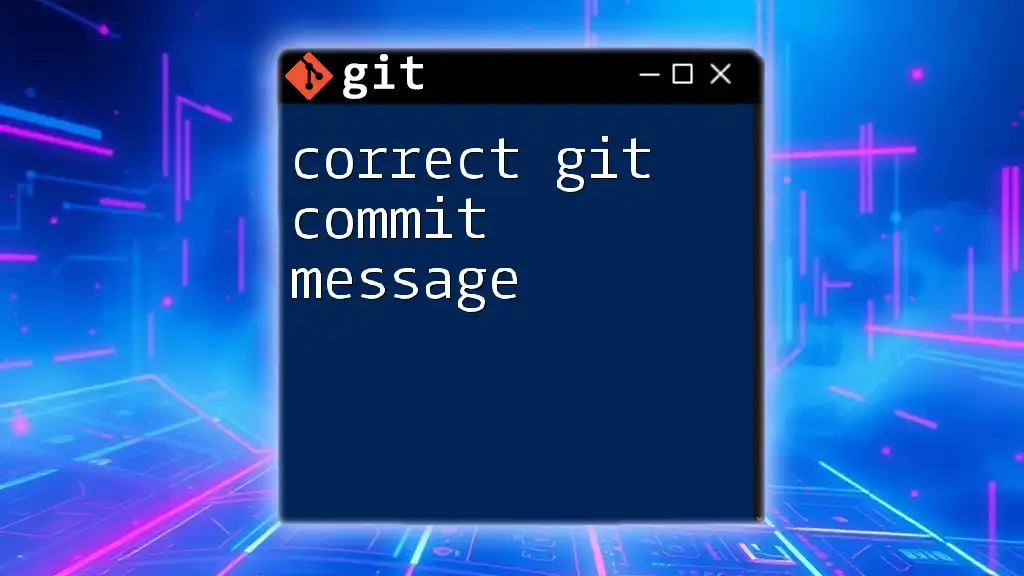
Collaborating with Git in React Development
Setting Up a Remote Repository
To collaborate with others, set up a remote repository on platforms such as GitHub or GitLab. Once you create this remote, link your local repository:
git remote add origin https://github.com/username/repo.git
git push -u origin main
This command establishes a connection to your remote repository, allowing you to share your code.
Pull Requests and Code Reviews
In collaborative environments, pull requests are vital. They serve as a request for code review before merging changes into the main branch. Ensure your pull requests are descriptive, detailing what changes you've made and any specific points that require feedback.
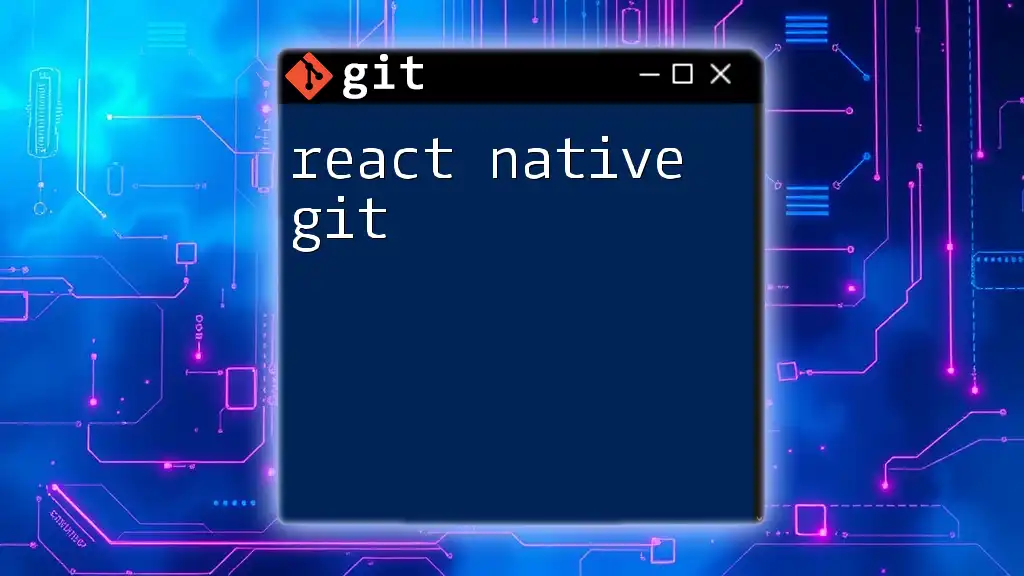
Advanced Git Commands for React Developers
Rebasing
One advanced feature of Git is rebasing, which allows you to integrate the changes from one branch into another, keeping a cleaner project history. This can be accomplished with:
git rebase main
Rebasing can streamline the commit history, making it easier for others to follow changes.
Stashing Changes
Sometimes, you need to switch branches but aren't ready to commit your current changes. The stash command allows you to save your work temporarily:
git stash
Later, you can retrieve your stashed changes with:
git stash pop
This command restores your stashed changes back to your working directory.
Resolving Merge Conflicts
Merge conflicts often occur when two branches make different changes to the same line of code. When Git can't resolve these automatically, you need to manually fix the conflicts in your code editor. After resolving the conflicts, stage your changes and commit them:
git add .
git commit -m "Resolved merge conflicts"
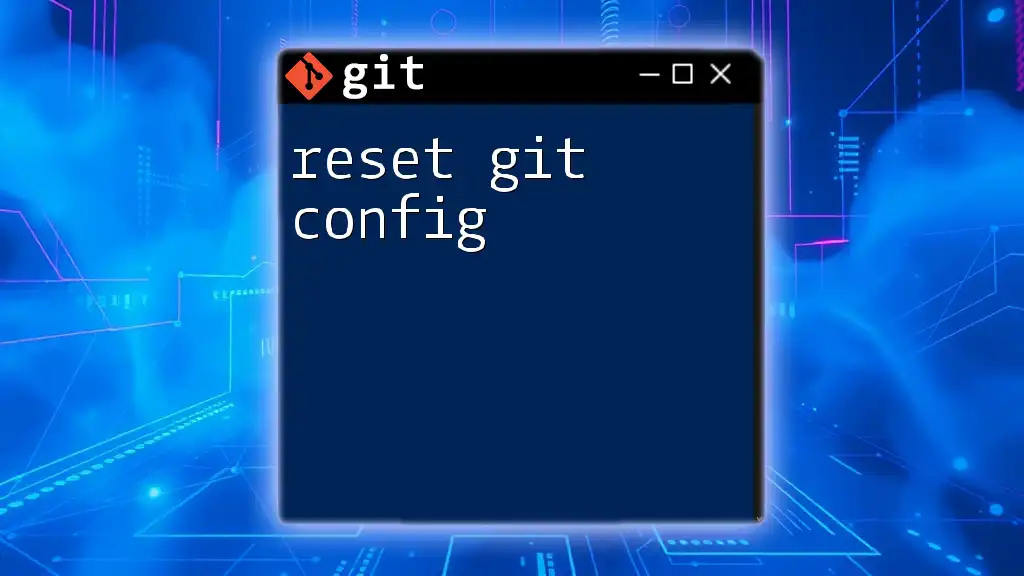
Best Practices for Using Git with React
Commit Messages
Effective commit messages are indispensable in Git. A good convention is to start with a concise subject line followed by a more detailed explanation, if necessary. For example:
Fix: Correct typo in the login component
- Changed "submist" to "submit"
Structuring Your Repository
Organizing your React project adequately can greatly assist both in development and collaboration. Common practices include maintaining a well-defined `src` folder for source files, a `public` folder for static assets, and a `test` folder for testing scripts.
Using `.gitignore`
A `.gitignore` file is essential for specifying which files or directories Git should ignore. Common entries for a React project include:
node_modules/
build/
This keeps unnecessary items out of your repository, which helps keep it clean and focused.
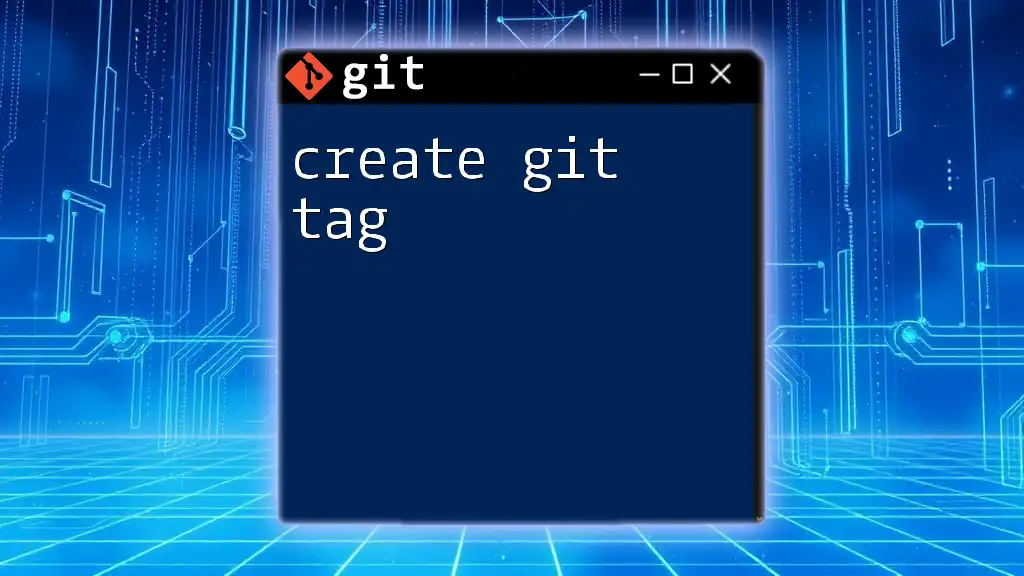
Integrating Git with Development Tools
Setting Up Your IDE
Many popular Integrated Development Environments (IDEs), including Visual Studio Code and WebStorm, come with built-in Git support. This allows developers to commit, push, and pull changes right from the IDE, streamlining the development process.
Continuous Integration/Continuous Deployment (CI/CD)
Using CI/CD tools such as GitHub Actions or Travis CI can automate testing and deployment, enhancing the effectiveness and reliability of your React projects. Setting up these systems effectively ensures that new code changes won't break existing functionality.
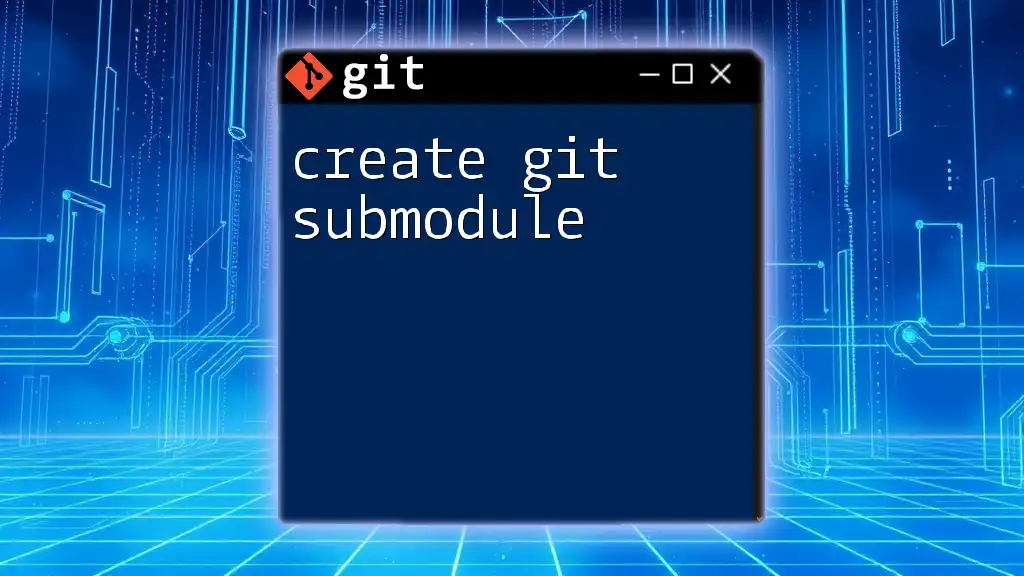
Conclusion
Mastering Git is crucial for React developers, as it enhances collaboration, enables effective version control, and fosters better coding practices. By becoming proficient with basic and advanced Git commands, as well as integrating best practices into your workflow, you'll significantly improve your software development process and project management skills.
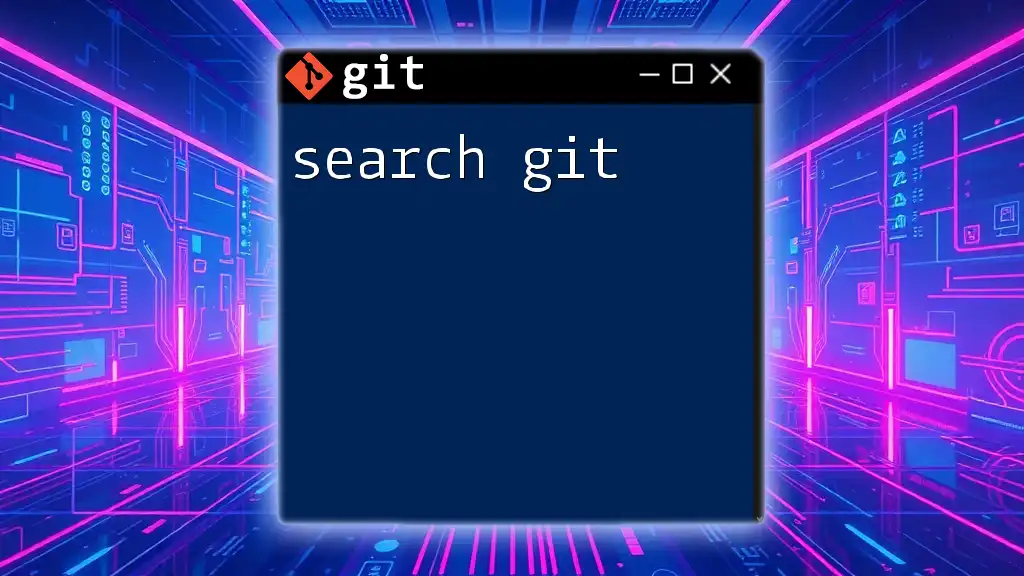
Additional Resources
For further reading, consider exploring Git’s official documentation and popular Git tutorials that delve deeper into specific areas of interest. Engaging with community resources can also provide valuable insights tailored to your specific needs as you navigate the world of react git.