In Git, the `reset` command is used to undo changes by moving the current branch's head to a specified commit, effectively modifying the staging area and working directory as needed.
Here’s a simple example of using the `git reset` command:
git reset --hard HEAD~1
What is Git Reset?
`git reset` is a powerful command that allows you to alter the commit history of your Git repository. Its primary purpose is to remove commits from the current working branch. Understanding how to use `git reset` effectively is crucial for maintaining a clean and logical commit history. This command can be especially helpful in situations where you need to backtrack, whether due to an accidental commit or an incomplete feature.
When to Use Git Reset
Resetting your Git history can be beneficial in a variety of scenarios. Here are some reasons you might consider using `git reset`:
- Correcting Mistakes: If you've accidentally committed changes that you didn't intend to, `git reset` allows you to reverse those commits easily.
- Managing Staging Area: You can manipulate which changes are staged for the next commit.
- Cleaning Up History: If you want to maintain a more coherent history, resetting can help streamline your commits before sharing them with others.
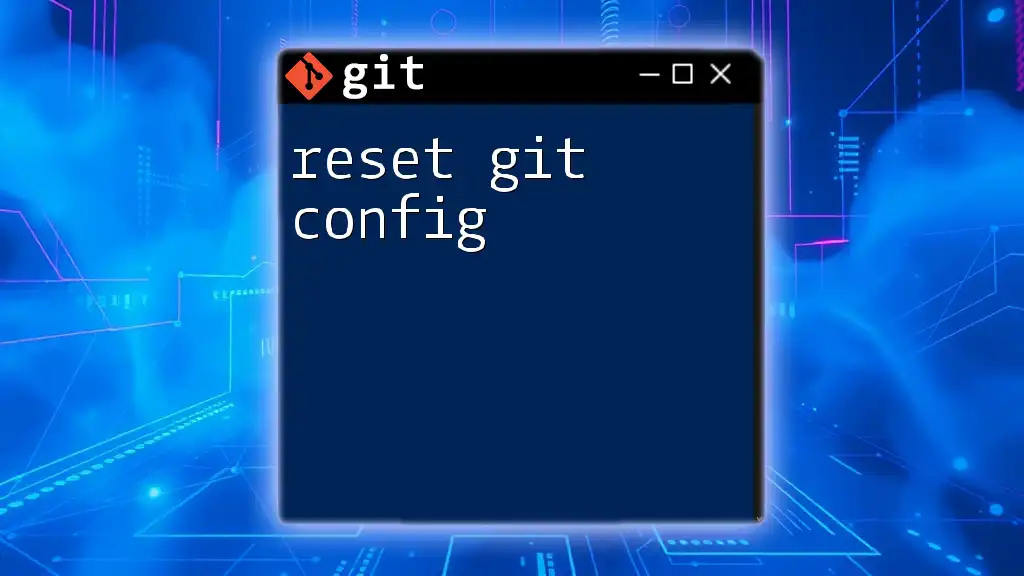
Understanding Git Reset Variants
There are different types of resets you can perform, each serving distinct purposes.
Soft Reset
What is a Soft Reset?
The `--soft` option is designed to reset the current branch to a specified state while leaving your working directory and staging area untouched. This means your changes will still exist as unstaged modifications after the reset.
Example of Soft Reset
To execute a soft reset, you would use:
git reset --soft HEAD^
This command will move the current branch pointer back one commit, but your changes remain staged. This is particularly useful if you realize you need to make additional changes before finalizing a commit.
Mixed Reset
What is a Mixed Reset?
A mixed reset (the default option, without any flags) will reset the current branch to a specific commit but keep your working directory intact. However, it will unstage any changes that were previously staged.
Example of Mixed Reset
To perform a mixed reset, you can use:
git reset HEAD^
In this case, you're effectively undoing your last commit, keeping your changes in the working directory for further modifications or new commits.
Hard Reset
What is a Hard Reset?
The `--hard` option resets the branch pointer and modifies both the staging area and the working directory to match a specific commit. This means all changes after that commit will be lost, so it should be used cautiously.
Example of Hard Reset
The command for a hard reset is:
git reset --hard HEAD^
Be aware that this command erases all subsequent uncommitted changes, making it critical to use it with care to avoid losing valuable work.
Other Types of Resets
Keeping Changes After Reset
If you want to preserve your changes while resetting, you can use either `--mixed` or `--soft`, depending on whether you want to keep those changes staged or unstaged.
For example:
git reset HEAD~1 --soft
This command resets your branch to the previous commit while retaining all changes in the staging area for optional further editing.
Conditional Reset
Resetting to Another Commit
The flexibility of `git reset` allows you to reset to a specific commit using its hash. This is useful for reverting your project state to a known good version.
The command for this action would be:
git reset --mixed abc1234
This command will reset the branch to the commit identified by `abc1234`, modifying the staging area but keeping your local changes intact.
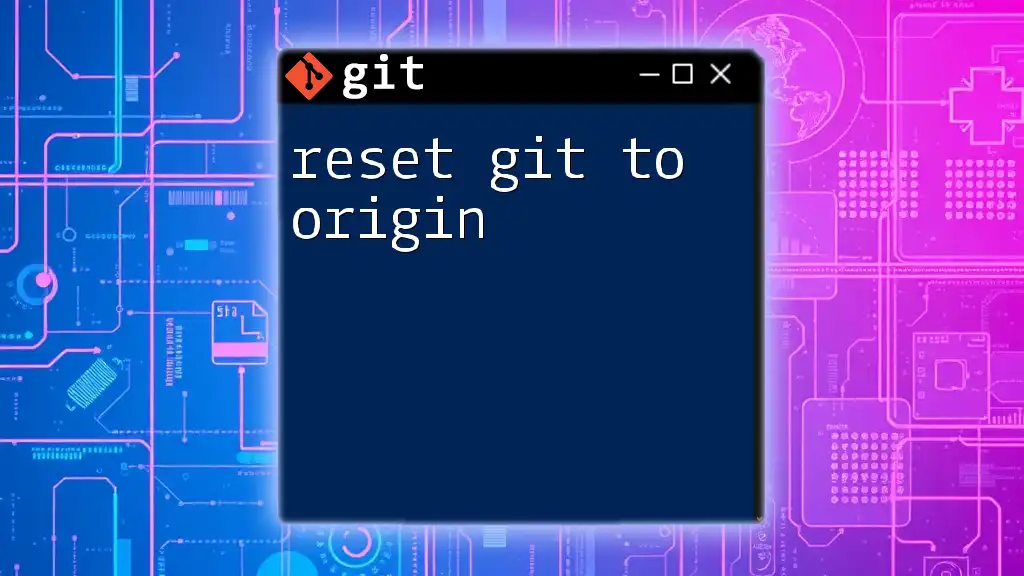
Practical Applications of Git Reset
Undoing Commits
Using `git reset` is one of the simplest ways to revert recent commits. Imagine you’ve committed code that’s not functional and need to undo that commit. By utilizing a mixed reset, you can undo the commit while maintaining your changes for further refinement.
Reverting Changes in Working Directory
In situations where your working directory contains changes you want to backtrack on without losing them entirely, the reset command can be invaluable. For instance:
git reset HEAD -- path/to/file
This command unstages your specified file(s) but keeps them as untracked changes in your working directory, allowing you to alter them further.
Fixing Merge Conflicts
Resetting can help you tackle problematic merges. If a merge results in too many conflicts, you can reset to a previous commit:
git reset --hard HEAD~1
This command allows you to erase the merge attempt and start negotiations again, promoting a cleaner resolution of conflicts.
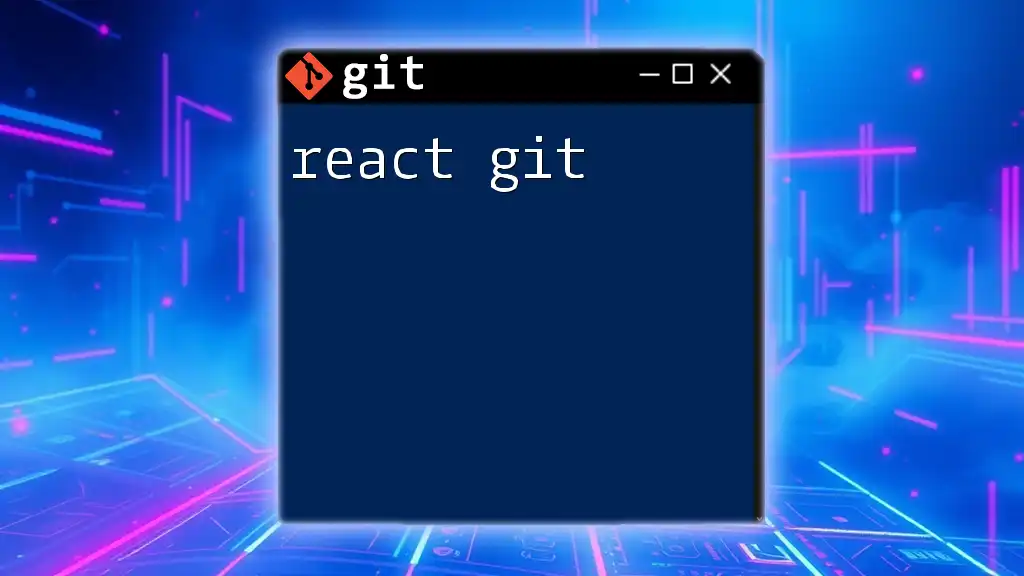
Best Practices with Git Reset
When to Avoid Git Reset
While `git reset` offers significant advantages, it's important to recognize scenarios where other commands might be more appropriate. For example, if a commit has already been shared with others, using `git revert` is safer because it doesn’t alter history in the same way `reset` does.
Using Git Reset in Collaboration
If you're working in a team environment, coordination is key when using `git reset`. Make sure that other team members are aware of changes to shared branches. Using reset can rewrite commit history, which may have unintended consequences for those collaborating on the same codebase.
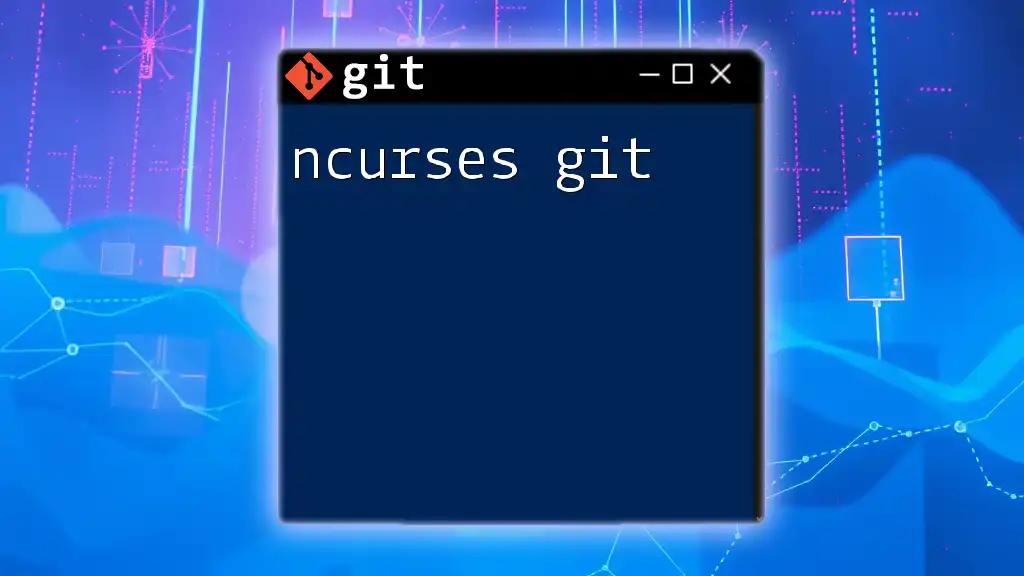
Conclusion
Understanding the various functions of `git reset` is essential for efficient version control. With capabilities to undo commits, manipulate staging areas, and manage your project’s history, `git reset` can be a formidable part of your Git toolbox.
Summary of Key Takeaways
In this guide, we've explored the different types of resets — soft, mixed, and hard — along with specific use cases and examples. Each type of reset serves unique purposes that are valuable in various development scenarios.
Final Tips
Always make sure to back up your work or practice commands in a test environment to prevent unintentional data loss. Familiarizing yourself with `git reset` will enhance your Git skills and streamline your development workflow.
Call to Action
We encourage you to experiment with the `git reset` command in a safe environment, such as a test repository. For more insights and tutorials on Git commands, be sure to subscribe or follow our content!