When working with a Java project, you can easily manage version control using Git commands to track changes and collaborate with others effectively.
Here's a sample code snippet to initialize a Git repository for your Java project:
git init my-java-project
cd my-java-project
echo "# My Java Project" > README.md
git add README.md
git commit -m "Initial commit"
Understanding Git Basics
What is Git?
Git is a distributed version control system that allows developers to track changes in source code during software development. It enables multiple developers to work on a project simultaneously without interfering with each other's work. The importance of Git in Java projects cannot be overstated, as it provides a reliable structure for collaboration, making it easier to manage updates, roll back changes, and maintain a clear history of all modifications.
Important Git Terminology
Before diving deeper into the practical applications of Git within Java projects, it's crucial to understand some key terms:
- Repository (Repo): A directory that contains your project's files and the entire history of all changes made to those files.
- Commit: A snapshot of your changes in the repository, representing a point in the overall history of your project.
- Branch: A separate line of development created to avoid impacting the main codebase until the work is ready to be merged.
- Merge: Combining different branches together. This might involve resolving conflicts if changes don't align properly.
- Pull Request: A request to merge changes in one branch into another, allowing for discussion and review before integration.
- Remote vs. Local Repositories: A local repository is on your machine, while a remote repository, such as on GitHub, is stored on the internet and accessible to others.
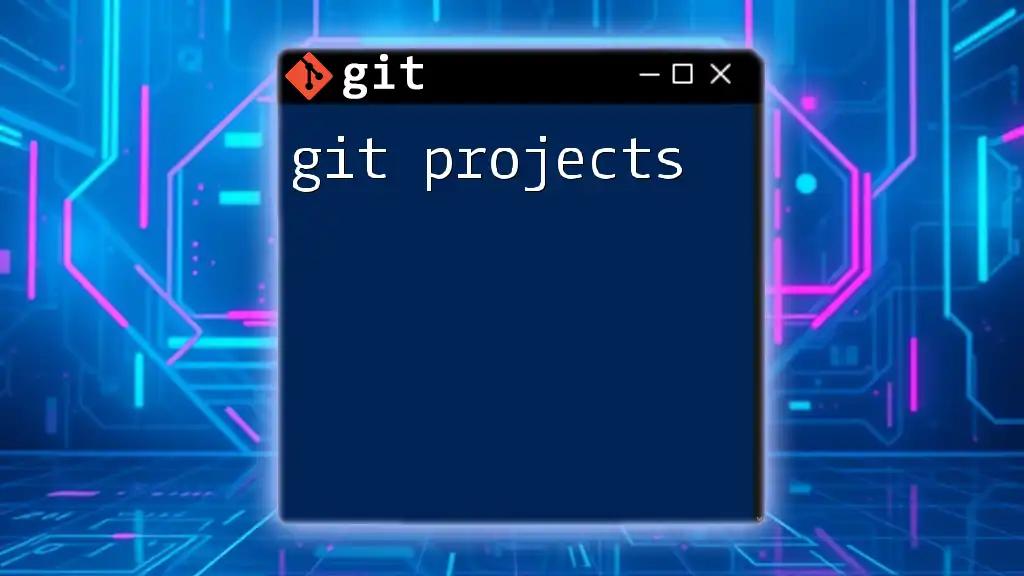
Setting Up Git for Your Java Project
Installing Git
To start using Git, you first need to install it on your machine. Here’s how you can do that based on your operating system:
- Windows: Download the installer from the [official Git website](https://git-scm.com/) and follow the instructions.
- macOS: You may use Homebrew with the command:
brew install git
- Linux: Use your distribution’s package manager, for example:
sudo apt-get install git # For Ubuntu/Debian
Initializing a Git Repository
Once Git is installed, you can create a new repository for your Java project. Navigate to your project directory in the terminal and run:
git init
This initializes a new Git repository, creating a hidden `.git` folder that stores all versioning information.
Cloning an Existing Repository
If you want to start with an existing project, you can clone a repository. Use the following command:
git clone <repository-url>
This command will create a local copy of the repository on your machine, linking it to the remote origin.
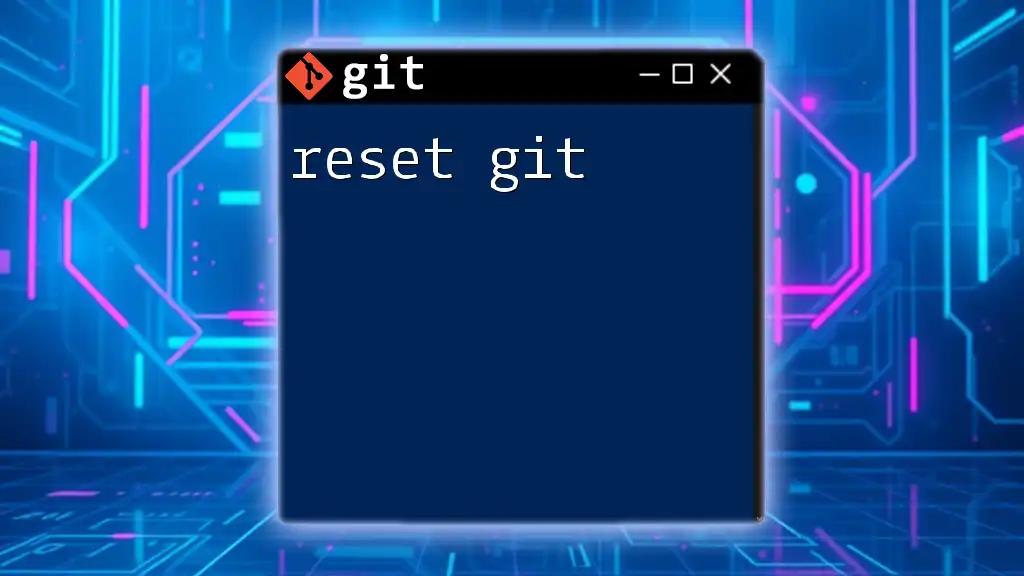
Structuring Your Java Project with Git
Recommended Project Structure
A well-organized Java project typically follows a standard layout:
my-java-project/
├── src/ # Source files
├── lib/ # Libraries
├── tests/ # Unit tests
└── README.md # Project documentation
Following this structure helps in maintaining clarity and organization as your project scales.
.gitignore for Java Projects
A .gitignore file is crucial to ensure unnecessary files do not clutter your repository. It specifies which files or directories Git should ignore. A sample `.gitignore` for a Java project might look like this:
# Compiled class files
*.class
# IDE-specific files
.idea/
*.iml
Including a .gitignore file will help streamline your project and keep it clean.
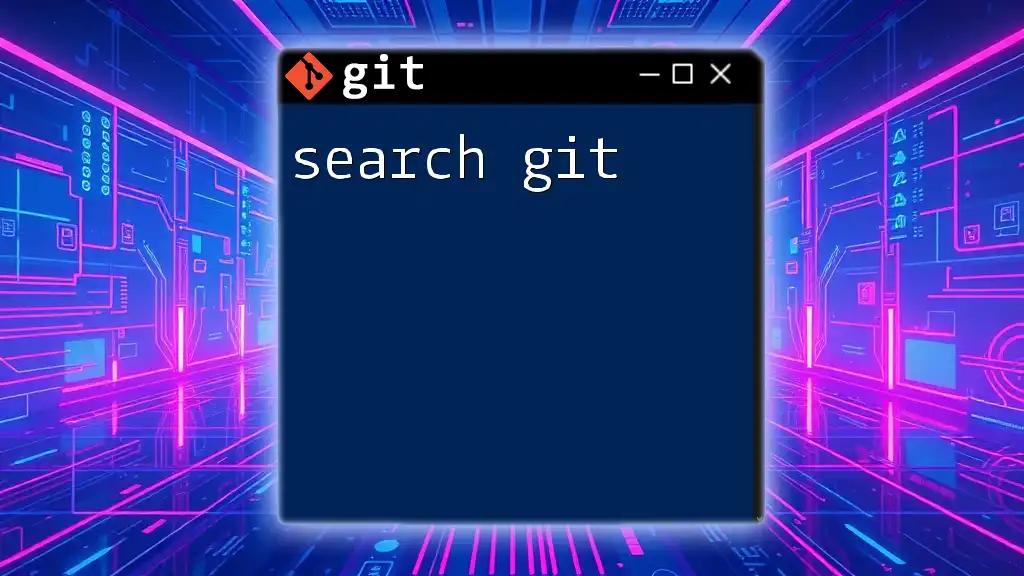
Common Git Commands for Java Projects
Staging and Committing Changes
To save your changes in Git, you must stage them first. Use the following commands:
git add <file> # Stages a specific file
git add . # Stages all changes
Once staged, you can commit the changes:
git commit -m "Descriptive message about what was changed"
Commit messages should be clear, concise, and encapsulate the essence of the change.
Branching Strategies
Branching is essential for collaborative development. You'll commonly use feature branches for new developments and bugfix branches for solving issues.
Creating and Switching Branches
To create and switch to a new branch, use:
git checkout -b <branch-name>
This command creates the branch and immediately switches to it, allowing you to start working on new features without altering the main codebase.
Merging Branches
When your feature is complete, you can merge it back into the main branch:
git checkout main
git merge <branch-name>
It's imperative to resolve any conflicts that arise during this process, ensuring that your code functions as expected.
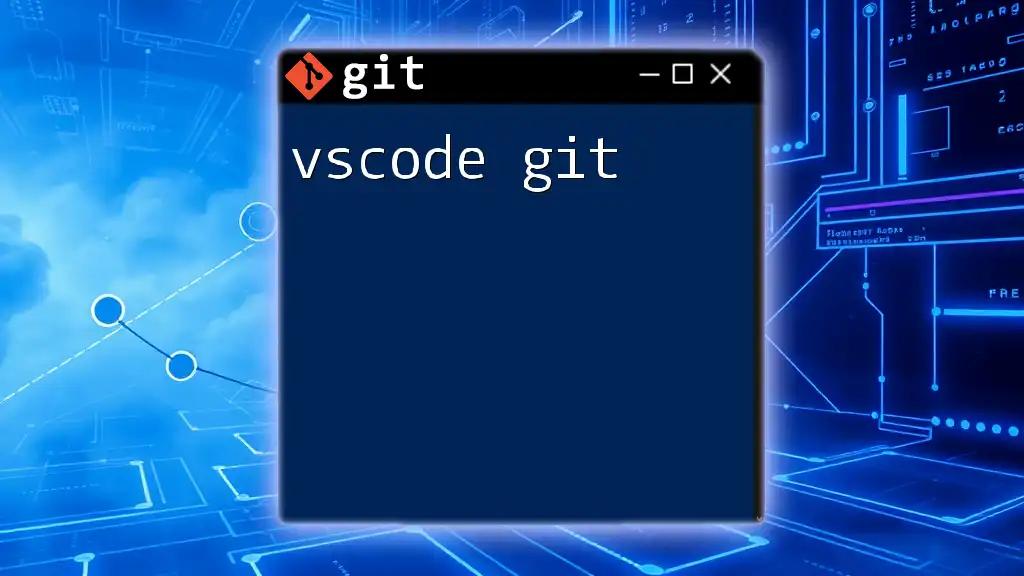
Collaborative Development with Git
Working with Remote Repositories
To collaborate effectively, you'll typically use a remote repository hosted on platforms like GitHub or GitLab. After creating an account and setting up a repository, you can link it with your local project using:
git remote add origin <repository-url>
Pulling Changes
When working in a team, it's essential to stay updated with the latest changes. Pulling changes from the remote repository will help do this:
git pull origin main
This command fetches and integrates changes from the main branch of the remote repository to your local copy.
Pushing Changes
Once you've committed your changes locally, push them to the remote repository to share them with your team:
git push origin <branch-name>
Consistently pushing your changes helps maintain synchronization among team members.
Collaborating through Pull Requests
A pull request serves as a request to merge changes from one branch to another. It facilitates code reviews and discussions around the proposed changes. To create a pull request, navigate to your repository on GitHub, select the branch you’ve worked on, and click “Create Pull Request.” Always contribute to code reviews, ensuring best practices and quality are adhered to.
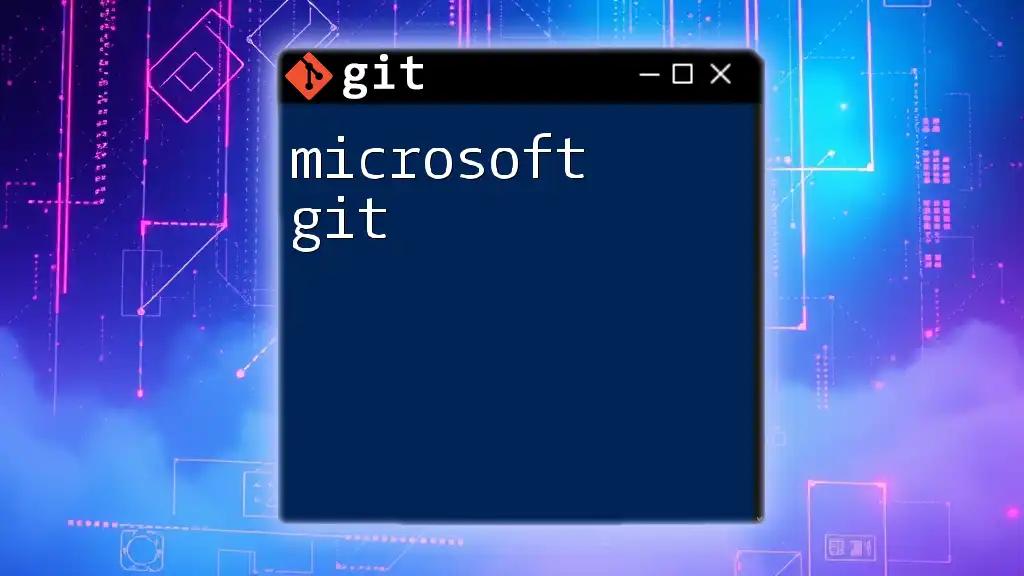
Best Practices for Using Git in Java Projects
Commit Often, Commit Early
Regular commits help keep track of your progress and avoid the dread of losing hours of hard work. Aim to commit after completing focused tasks or reaching a logical juncture in development.
Keeping Your Branch Up to Date
To avoid conflicts and merging issues, ensure your branch is regularly synchronized with the main branch. You can do this by merging or rebasing:
git fetch origin
git rebase origin/main
Using rebase helps maintain a cleaner project history, making it easier to understand project evolution.
Regularly Back Up Your Work
Remember to push your changes to the remote repository frequently. Regular backups minimize the risk of losing your progress and enhance collaboration with teammates.
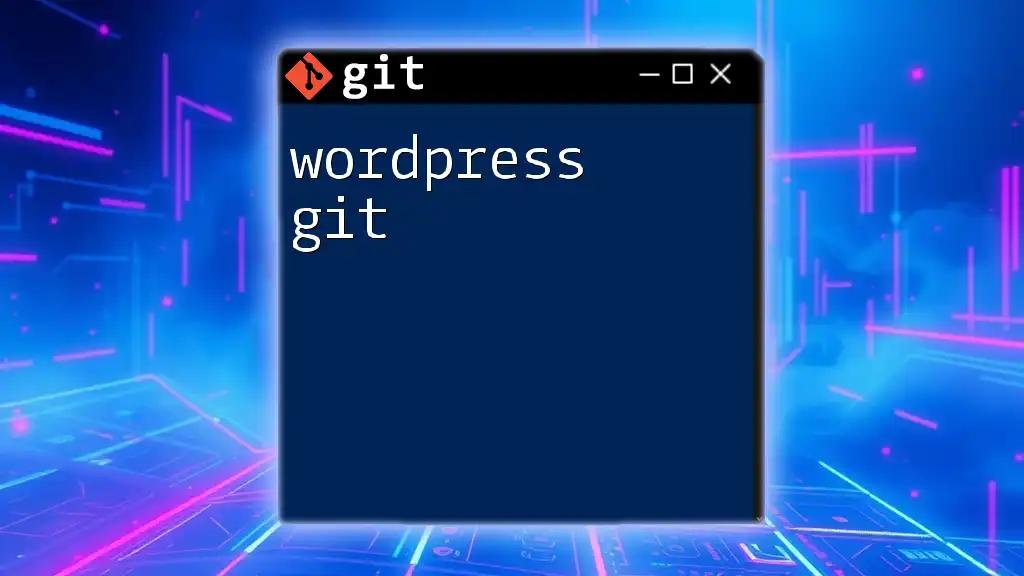
Dealing with Common Issues
Troubleshooting Merge Conflicts
Merge conflicts occur when two branches have competing changes. This is what it looks like when merging:
Auto-merging file.txt
CONFLICT (content): Merge conflict in file.txt
Automatic merge failed; fix conflicts and then commit the result.
To resolve conflicts, locate the affected file, edit the conflicting portions, and then stage and commit your changes.
Undoing Changes
Sometimes, you might need to revert to a previous state. Use the following commands to undo commits or changes:
git reset --soft HEAD~1 # Undo the last commit but keep changes staged
git checkout -- <file> # Discard changes in a file
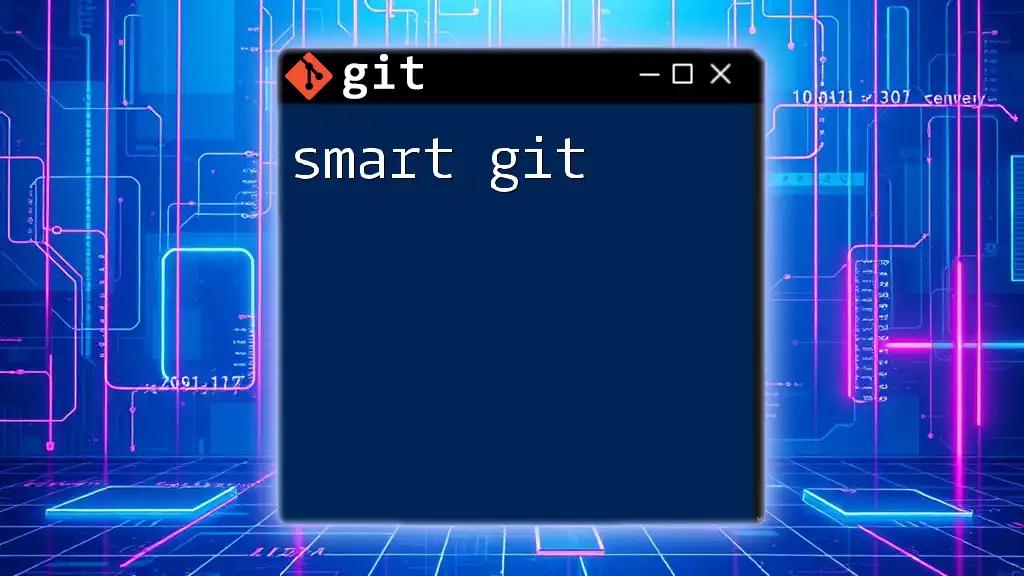
Conclusion
Using Git effectively in your Java projects can immensely streamline your development process, enhance collaboration, and mitigate risks associated with version management. By incorporating best practices and understanding essential commands, you'll establish a robust workflow that will serve you well throughout your coding journey.
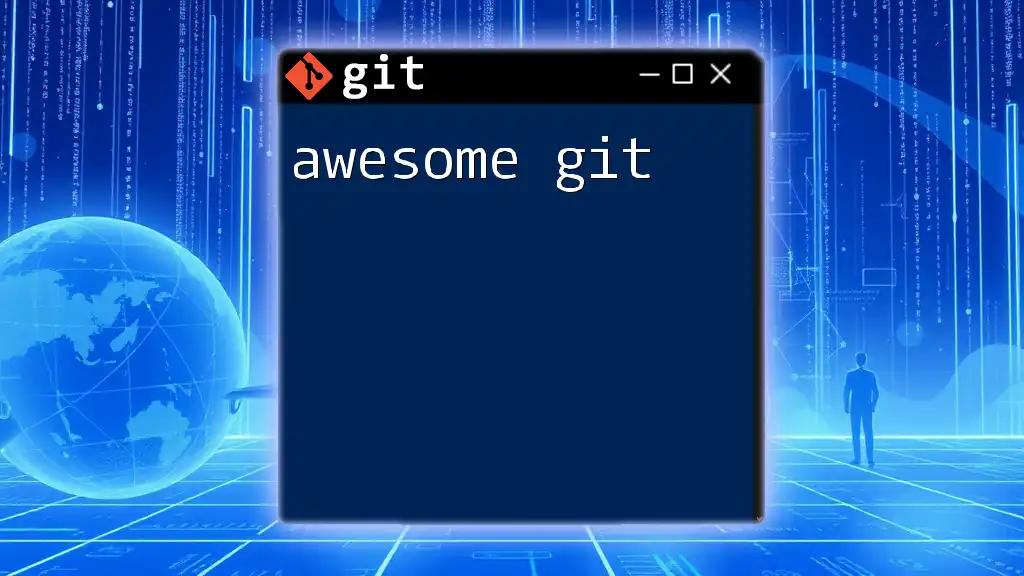
Additional Resources
As you continue to improve your Git skills, consider reading further materials, exploring tutorials, and checking out official documentation. Look into tools such as IDE plugins that integrate Git functionality to elevate your Java development experience even more.