A Git project is an overall initiative that can encompass multiple repositories, while a repository (or repo) is a specific collection of files and version history managed by Git.
Here's a code snippet to illustrate how to clone a repository from a Git project:
git clone https://github.com/username/repository.git
Understanding Git Repositories
What is a Git Repository?
A Git repository (often abbreviated as "repo") is a data structure used by Git to store metadata for a project. It serves as a central hub where all the project files, their revisions, and the whole history of changes are tracked. The concept of repositories is one of the critical components that defines how Git organizes projects and manages versions.
Types of Repositories
-
Local Repository: This is a repository stored on your local machine. When you create a local repository, Git initializes it within a directory that contains all your project files along with the entire history of changes you have made. To create a local repository, you can run the following command:
git init
-
Remote Repository: In contrast, a remote repository is stored on a server and can be accessed by multiple collaborators. This can include platforms such as GitHub, GitLab, or Bitbucket. You can clone a remote repository to your local machine using the command:
git clone <repo-url>
Structure of a Git Repository
A typical Git repository includes a hidden directory named `.git`, which contains:
- Commits: Snapshots of your project at certain points in time.
- Branches: Different lines of development in your project.
- Tags: Markers for important points, often used for releases.
- HEAD: A reference to the current branch or commit you are working on.
Understanding this structure helps you navigate your repository effectively.
How to Create a Git Repository
Creating a Git repository can vary slightly depending on whether you are starting locally or working with a remote source.
-
For a Local Repository:
mkdir project-name cd project-name git init
-
For a Remote Repository:
After creating a repository on a platform like GitHub, you can run:
git clone <your-repo-url>
Best Practices for Managing Repositories
Managing your repositories effectively is essential for maintaining a robust development workflow. Here are some best practices:
-
Version Control Strategies: Commit regularly with meaningful messages. Avoid vague messages like "fixed stuff," as it can cause confusion later. A good commit message should explain what changes were made and why.
-
Branching Strategies: Use branches to enable collaborative workstreams without disrupting the main codebase. The GitFlow strategy is popular among teams; it includes feature branches, release branches, and hotfix branches to organize work efficiently.
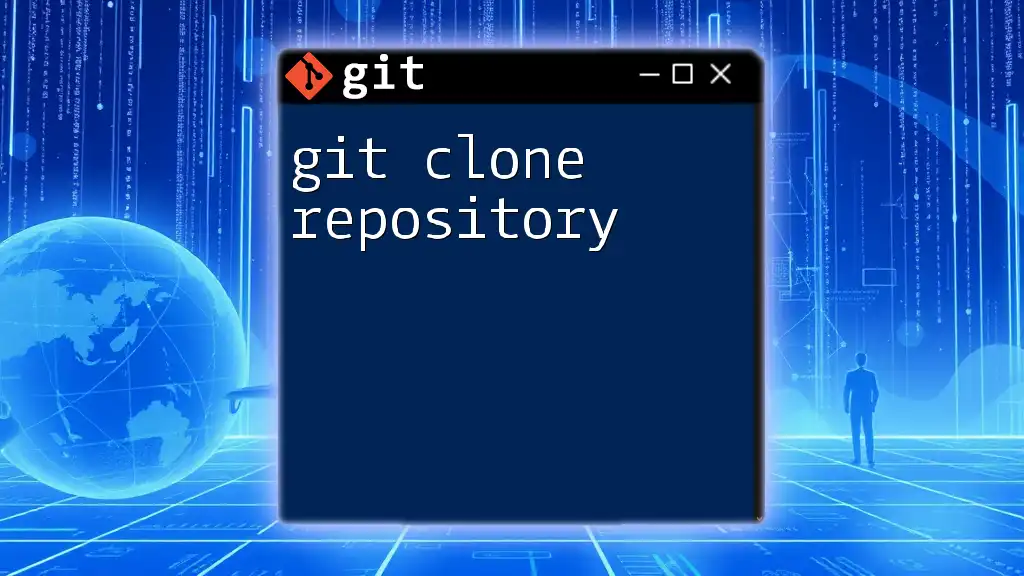
Understanding Git Projects
What is a Git Project?
A Git project is more than just the files; it encompasses the entire development effort, including organization, documentation, and collaborative practices. Essentially, a Git project refers to a collection of files and their version history managed within a repository. Understanding this distinction helps in utilizing Git to its full potential.
Components of a Git Project
-
Files and Directories: Each Git project contains various files needed for functionality, such as source code, configuration files, and test scripts. Organizing these files in a logical manner is crucial for the ease of navigation and understanding.
-
Project Documentation: Essential components of any Git project include:
- README.md: Explains the project, how to install it, and how to use it. It is often the first point of contact for new developers.
- LICENSE: Specifies the licensing terms for the project.
- CONTRIBUTING.md: Guidelines for contributing to the project.
Examples of Git Projects
Consider a simple Node.js project structure:
project-name/
│
├── src/ # Source files
│ ├── index.js # Main JavaScript file
│ └── ... # Other module files
│
├── tests/ # Test files
│ └── test.js # Example test file
│
├── README.md # Project description
├── LICENSE # Licensing information
└── package.json # Project metadata
This layout makes it easy for collaborators to find what they need without digging through files.
Best Practices for Git Projects
To ensure your Git project remains manageable and understandable, consider these practices:
-
Organizing Project Files: Adopt a consistent naming convention for files and directories. This practice helps in finding files quickly and avoiding confusion.
-
Documentation and Maintenance: Invest time in writing and updating documentation throughout the development process. This not only aids current collaborators but also smoothens the onboarding process for future team members.
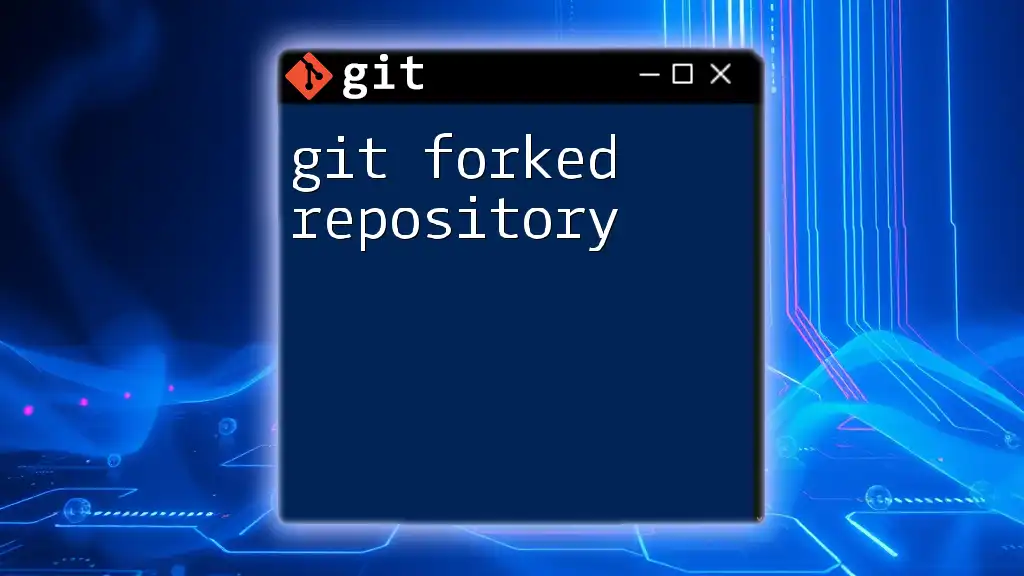
Key Differences Between Git Projects and Repositories
Definition and Purpose
While both terms are often used interchangeably, distinguishing between a Git project and a repository is crucial. A project refers to the functional workspace that encompasses your development efforts, whereas a repository serves as the version control mechanism that tracks changes and stores the project files.
Scope and Usage
Consider scenarios for differentiation:
- Project: When discussing functionality, features, or collaboration, you are usually referring to the entire project.
- Repository: When discussing version history, commits, or branching, you're focused on the technical storage aspects within Git.
Visualization
To visualize the relationship, think of the repository as the container that holds all the files and historical data associated with a project, which can be a single application, library, or even an entire system.
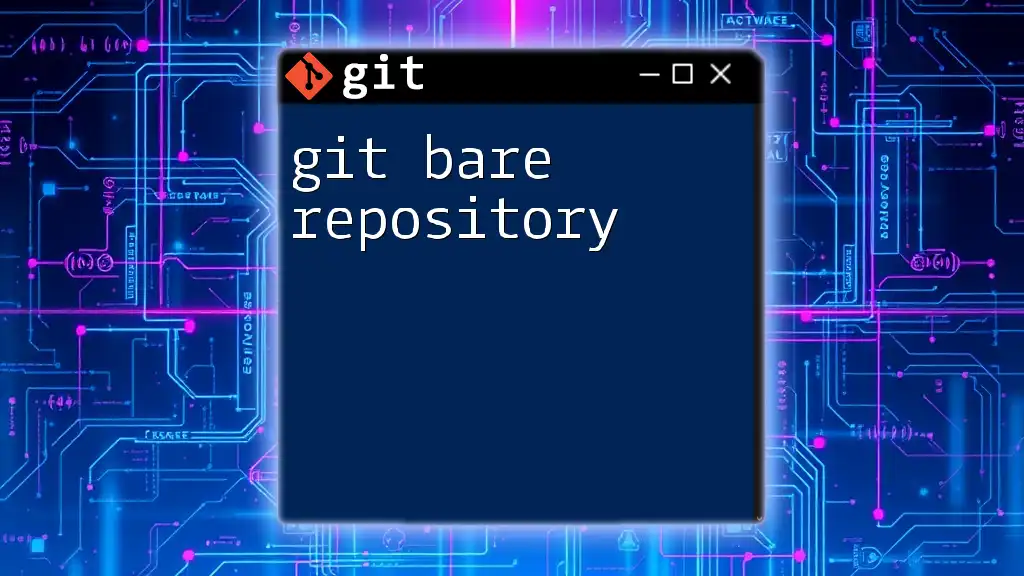
Conclusion
Understanding the differences between a Git project vs repository is foundational for effectively using Git in development workflows. Knowing how to create and manage both allows developers to optimize their version control processes and ensure clear communication in collaborative environments. Continued education and practice will enhance your skills in using Git, leading to better project management and smoother teamwork.
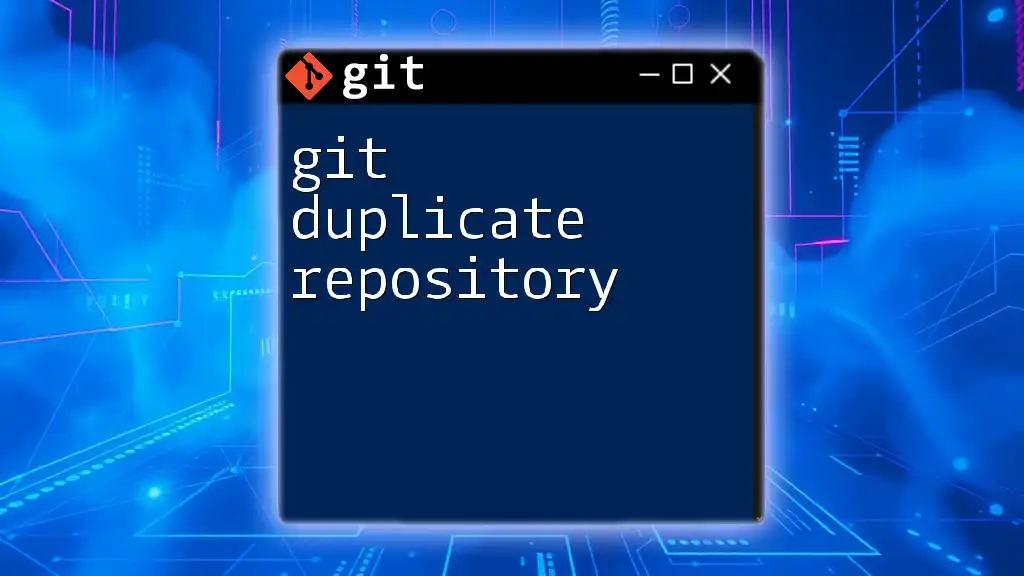
References and Further Reading
For those looking to deepen their understanding, consider exploring the following:
- Pro Git by Scott Chacon and Ben Straub (available online).
- Git documentation on the official Git website.
- Online courses that specialize in Git and version control.
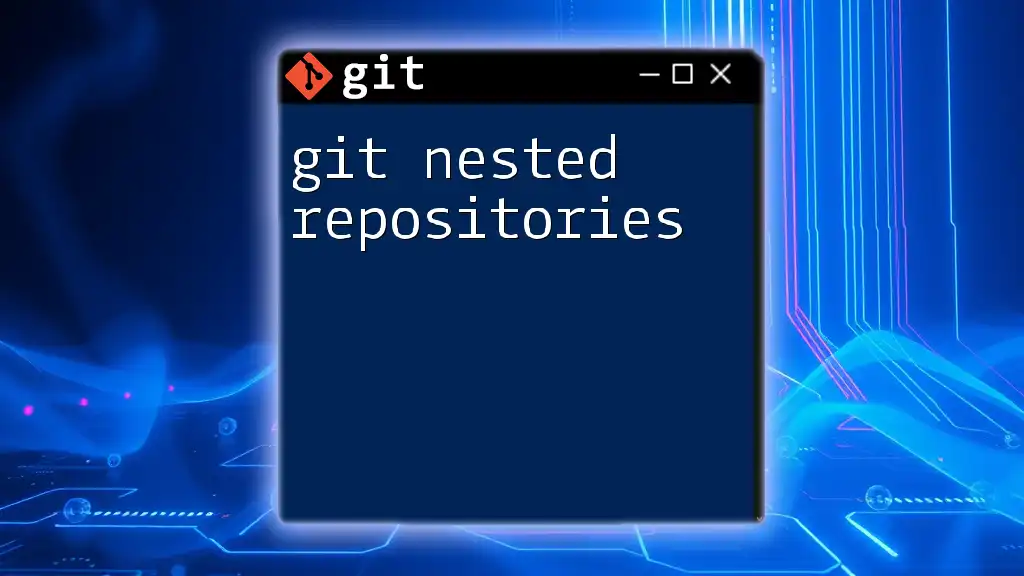
FAQ Section
Common Questions about Git Projects and Repositories
What is a branching strategy?
A branching strategy defines how developers will manage branches in a Git repository, ensuring organized workflows and smoother collaboration.
How can I collaborate on Git projects?
You can collaborate by cloning repositories, creating branches for features or fixes, and using pull requests to merge changes.
Why is documentation important in a project?
Documentation clarifies the purpose, setup, and use of a project, making it easier for new contributors and ensuring that future maintenance is straightforward.