"Laravel Git" refers to the integration of Git version control in Laravel projects, enabling developers to efficiently manage code changes and collaborate on application development.
Here's a simple Git command to initialize a new Laravel project:
composer create-project --prefer-dist laravel/laravel your-project-name
Setting Up Your Laravel Project with Git
Installing Laravel
To begin your journey with Laravel and Git, you first need to install Laravel. This can be accomplished through Composer, which is a dependency manager for PHP.
composer create-project --prefer-dist laravel/laravel your-project-name
Replace `your-project-name` with your desired project directory. This command will download and install a fresh Laravel application with all necessary dependencies.
Initializing a Git Repository
Once you have your Laravel project set up, the next step is to initialize a Git repository. This allows you to track changes in your code.
Navigate to your project directory:
cd your-project-name
Then, initialize a new Git repository:
git init
Now, you have successfully set up a Git repository linked to your Laravel project; you can start tracking your changes!
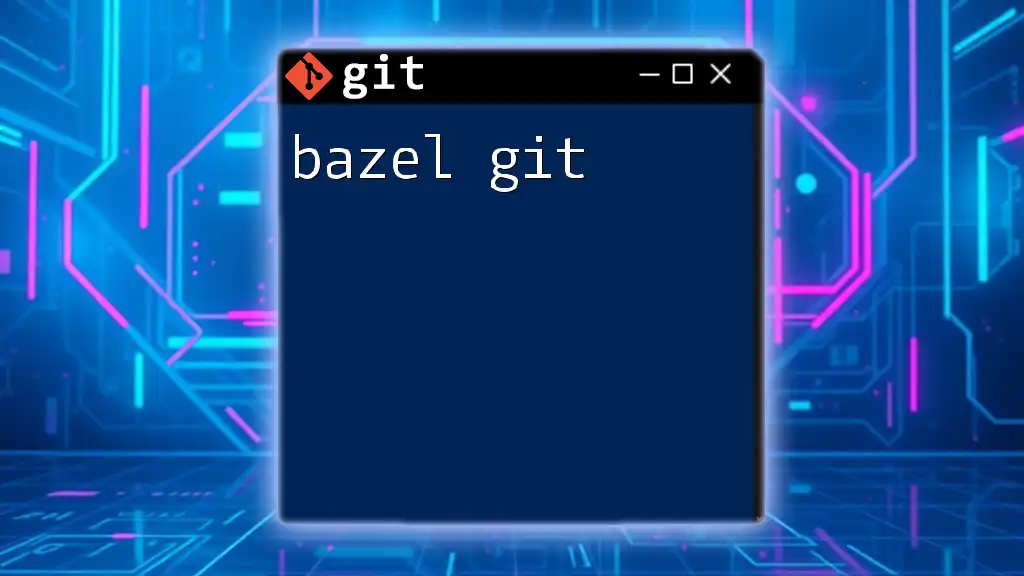
Essential Git Commands for Laravel Development
Staging Changes
The staging area is crucial in version control as it allows you to prepare changes before committing them. To stage all changed files, use:
git add .
This command stages all modified, deleted, and new files. It’s important to review what you are about to stage using `git status` before proceeding.
Committing Changes
After staging your changes, you must commit them to save your work history. A well-crafted message makes it easier to understand the context of changes later on.
To commit your changes, run:
git commit -m "Add user authentication feature"
The message should be concise yet descriptive enough to reflect the changes made. Best practices recommend starting with a noun that summarizes the change.
Creating Branches
Branching is a powerful feature that allows you to create independent lines of development. This is particularly useful when working on new features or bug fixes. To create a new branch, use:
git checkout -b feature/authentication
This command creates and switches you to a new branch called `feature/authentication`. Make sure to name your branches descriptively to understand their purpose easily.
Merging Branches
Once you complete a feature or fix a bug in a branch, it’s time to merge those changes back into your main codebase. To do this, switch to the main branch first:
git checkout main
Then, merge the branch:
git merge feature/authentication
If there are any conflicts, Git will prompt you to resolve them. You will need to edit the files with conflicts and manually choose which changes to keep.
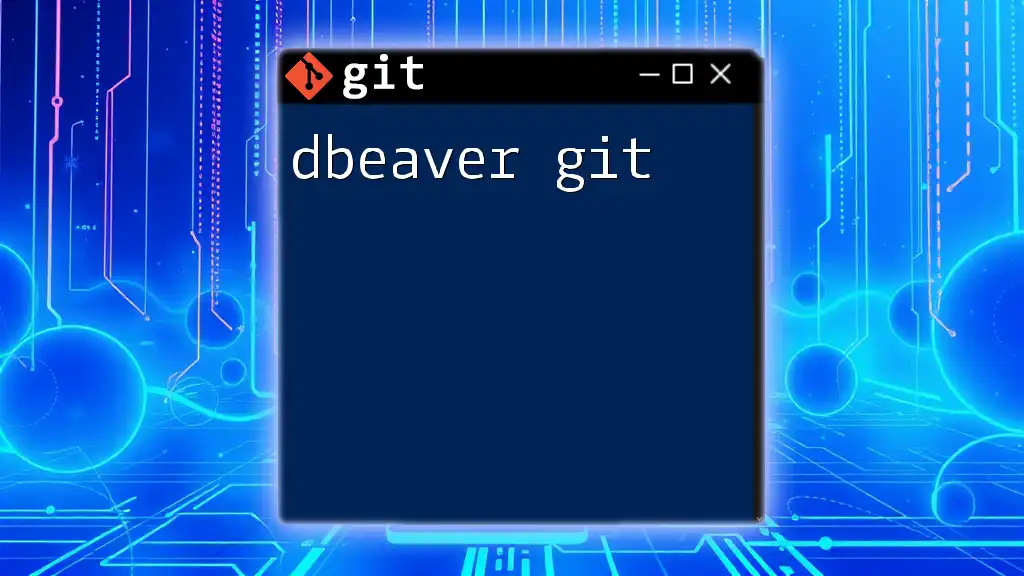
Git Workflow Best Practices in Laravel
Feature Branch Workflow
The feature branch workflow is a popular method for managing changes in a collaborative environment. Each new feature should be developed in its branch. This keeps the main branch clean of experimental code, making it easier to manage releases and hotfixes.
Regular Pulls and Pushes
To keep your local repository in sync with the remote one, it’s essential to regularly pull and push changes. For pulling the latest changes from the remote repository, use:
git pull origin main
Once your local changes are committed, push them to the remote repository with:
git push origin main
Maintaining these practices will help avoid conflicts and ensure that all team members are working with the latest code.
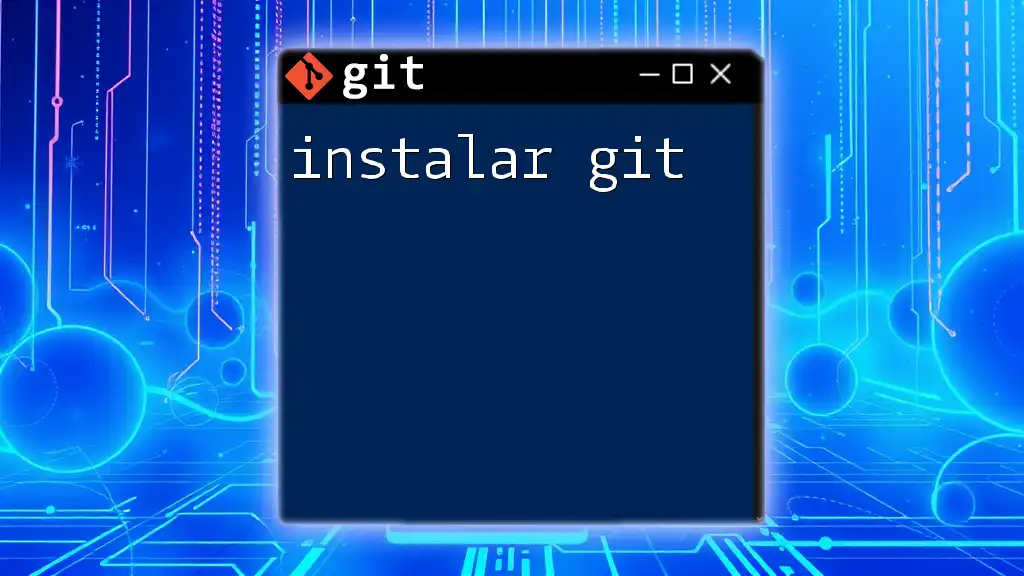
Collaborating with Git in Laravel Projects
Working with Remote Repositories
Setting up a remote repository on platforms like GitHub or GitLab is fundamental when working collaboratively. To link your local repository to a remote one, add a remote URL:
git remote add origin https://github.com/yourusername/your-repo.git
Replace the URL with the one for your repository.
Collaborating with Team Members
When working with a team, good collaboration practices are essential. Use Pull Requests for code reviews. This not only ensures code quality but also fosters communication among team members.
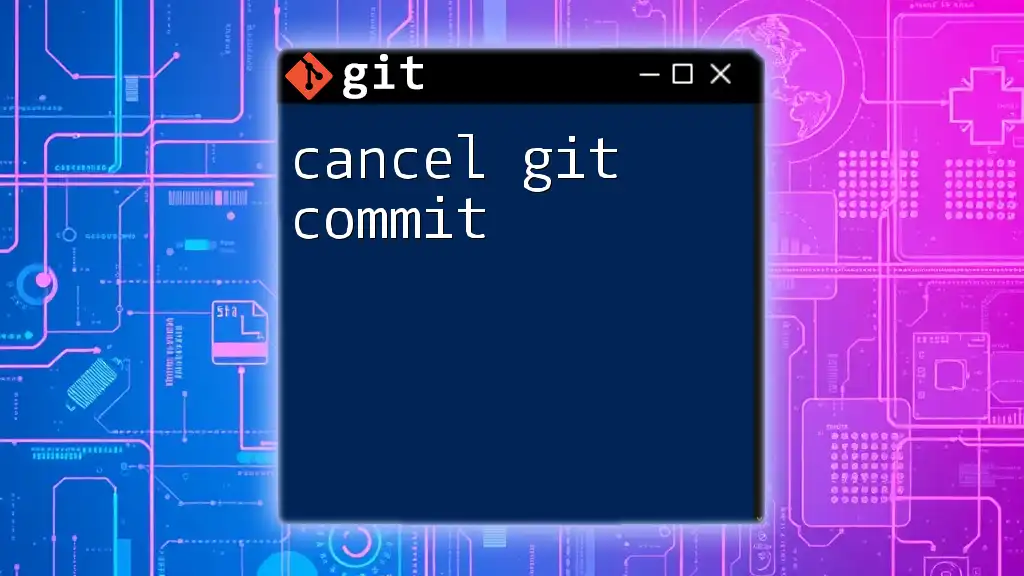
Handling Common Git Issues in Laravel
Resolving Merge Conflicts
Merge conflicts usually occur when multiple developers make changes to the same line of code or to the same file. When this happens, Git will prompt you to resolve the conflicts manually. You can check for conflicts with:
git status
Open the files with conflicts and you will find markers indicating the conflicting sections. Edit these files to resolve the discrepancies, then stage and commit your changes.
Undoing Changes
Sometimes, you may need to revert or undo changes in your Laravel project. You can do this in several ways using Git:
- To completely reset your working directory to the last commit:
git reset --hard HEAD
- If you want to discard changes to a specific file, use:
git checkout -- filename.php
- To create a new commit that undoes the last commit:
git revert HEAD
Understanding these commands is critical for maintaining a clean and functional codebase.
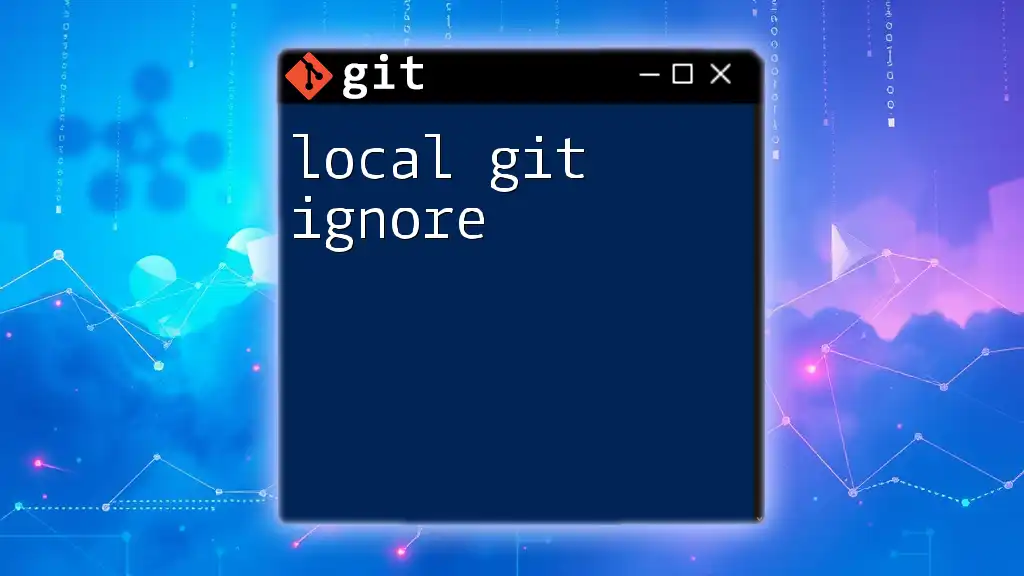
Best Tools for Using Git with Laravel
Git GUI Tools
For those who prefer a graphical interface over the command line, there are several Git GUI tools available. Tools like SourceTree, GitKraken, and GitHub Desktop provide visual representations of your repository, making it easier to manage branches, commits, and merges.
Laravel Specific Packages
Incorporating specific Laravel packages can enhance Git integration. For example, packages that facilitate better versioning of migrations or manage your `.env` file can streamline development workflows for Laravel projects.
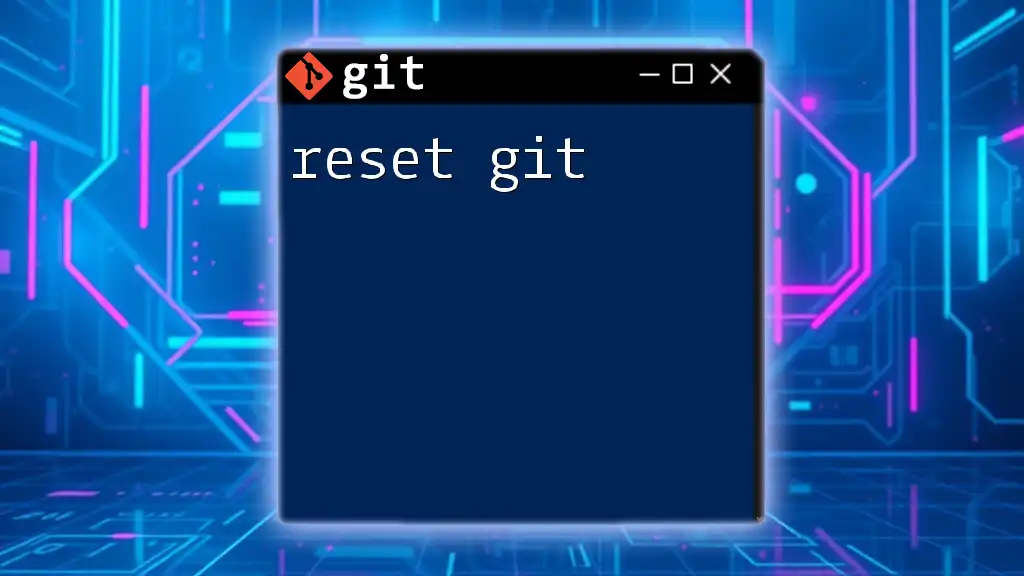
Conclusion
Incorporating Git into your Laravel development process significantly enhances project management and collaboration. Familiarize yourself with these commands and best practices to ensure a smooth development experience. Remember to continuously practice your Git skills and leverage the tools available to you. Join our community for further learning and hands-on workshops focused on mastering Git in Laravel!