"Bazel is a build tool that can efficiently manage and execute projects that use Git for version control, allowing developers to streamline their workflows."
Here's a simple example of how to clone a Git repository using Bazel:
git clone https://github.com/your-username/your-repo.git
Understanding Bazel and Git
What is Bazel?
Bazel is a powerful open-source build tool developed by Google. It is designed to handle large-scale builds in complex software projects. The key advantages of Bazel include its ability to support multi-language builds, efficient caching mechanisms, and robust dependency management. Its declarative build language allows developers to specify how to build their projects while Bazel handles the detailed execution, making it a preferred choice for projects that require speed and reliability.
What is Git?
Git is a widely-used version control system that enables teams to track changes, collaborate on code, and maintain version history. Its distributed nature allows developers to work offline, branch for features or fixes, and merge contributions from different team members efficiently. Key features of Git include easy branching and merging, commit histories, and effective collaboration.
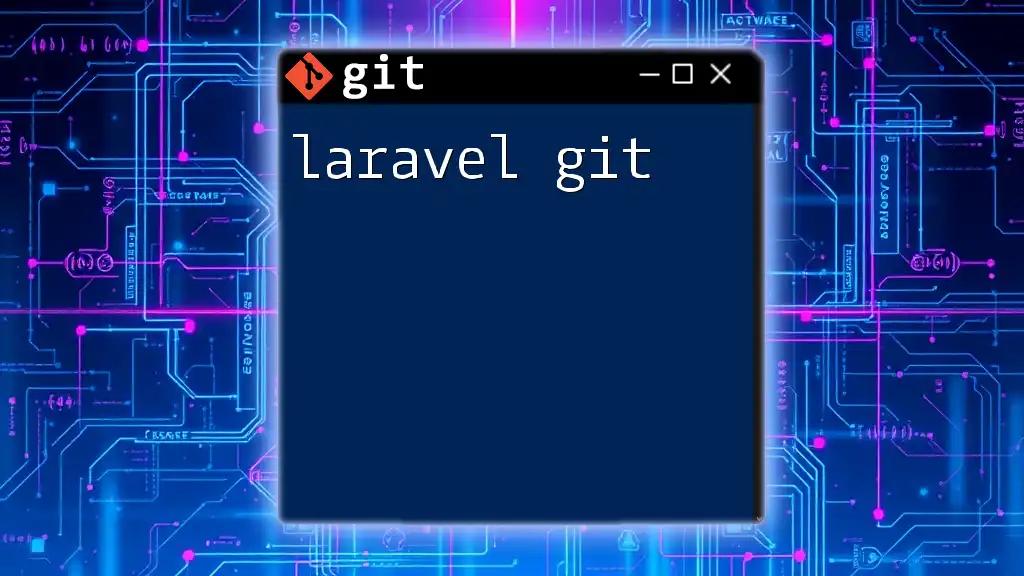
The Intersection of Bazel and Git
How Bazel and Git Work Together
Integrating Git into a Bazel workflow can significantly improve your build processes. Often, a project will have multiple dependencies that evolve over time, and managing these dependencies alongside your code becomes critical. Bazel's efficient dependency management and Git's version control capabilities allow developers to keep their builds consistent, track configuration changes, and revert to previous states if needed. This integration is particularly beneficial for teams that follow agile methodologies.
Why Use Git in a Bazel Workflow?
Using Git in conjunction with Bazel allows developers not only to manage their source code effectively but also to keep track of changes in build configurations. This becomes essential during team collaborations, simplifying code merges and reducing conflicts. Furthermore, it enables repeatable builds through the use of commit identifiers to lock down project dependencies at specific points in time.
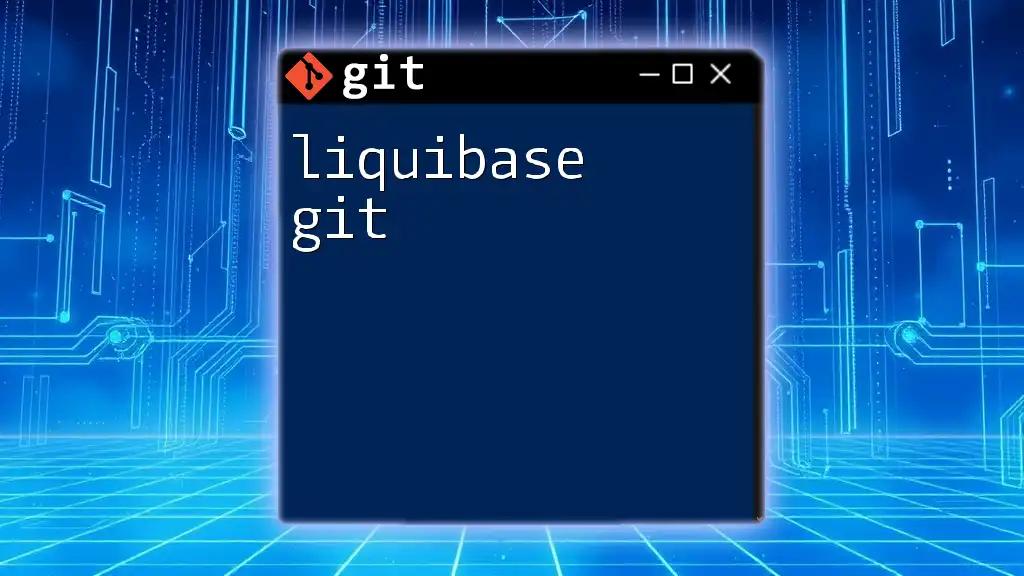
Setting Up Your Environment
Prerequisites
Before diving into using Bazel and Git together, ensure you have the following:
- System Requirements: Ensure that your system meets the installation requirements for both Git and Bazel. This typically includes:
- Stable internet for downloading software.
- Basic knowledge of command-line operations.
Basic Commands for Git and Bazel
Familiarize yourself with the essential commands for both tools. Here’s a quick reference:
For Git:
- `git init`: Initializes a new Git repository.
- `git clone <repo-url>`: Clones a repository from a remote source.
- `git commit -m "message"`: Commits your changes with a descriptive message.
For Bazel:
- `bazel build //path/to:target`: Builds the target specified.
- `bazel test //path/to:test`: Runs tests on the specified target.
Installation Guidelines
Installing Git
Installing Git varies by operating system:
- Windows: Use the Git for Windows installer from the official Git website. Follow the prompts to complete the installation.
- macOS: Use Homebrew with the command:
brew install git
- Linux: Use the package manager for your distribution. For example:
sudo apt-get install git # Ubuntu
Verify your installation with:
git --version
Installing Bazel
To install Bazel, follow these steps, which may differ slightly depending on your operating system:
- Windows: Download the Bazel installer from the official website and follow the instructions.
- macOS: Use Homebrew as follows:
brew install bazel
- Linux: Use the package manager, such as:
wget https://github.com/bazelbuild/bazel/releases/download/x.y.z/bazel-x.y.z-linux-x86_64 chmod +x bazel-x.y.z-linux-x86_64 sudo mv bazel-x.y.z-linux-x86_64 /usr/local/bin/bazel
Check if it’s installed properly with:
bazel version
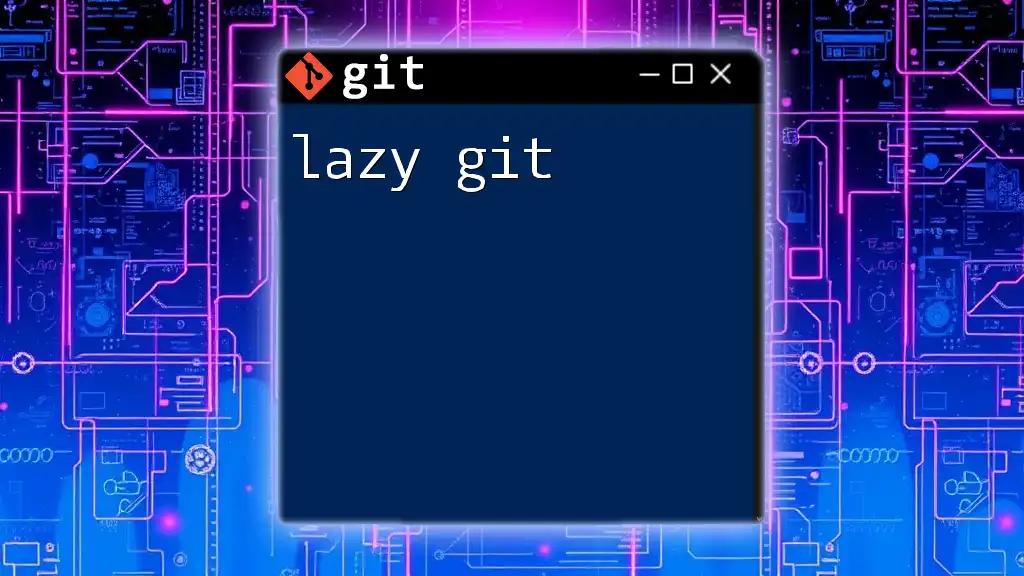
Best Practices for Using Bazel with Git
Structuring Repositories
When setting up your Git repository for a Bazel project, maintaining an organized structure is crucial. A well-structured repository typically includes:
- WORKSPACE file: This is critical for your Bazel project because it indicates the root of your Bazel build and helps define external dependencies.
- Directory layout for your `BUILD` files corresponding to different modules, libraries, or components of your project.
Version Control Practices
Using Branches
Branches are a powerful feature in Git that help you isolate changes. For instance, when working on a new feature, it is recommended to create a separate branch. This keeps your main branch stable while allowing you to experiment and make changes without affecting others. Use commands like:
git branch new-feature
git checkout new-feature
Commit Messages in a Bazel Context
Writing clear and impactful commit messages enhances the tracking of changes in Bazel projects. Commit messages should reflect the essence of the changes made, especially when they pertain to build configurations. For example:
git commit -m "Updated BUILD file for new dependency management"
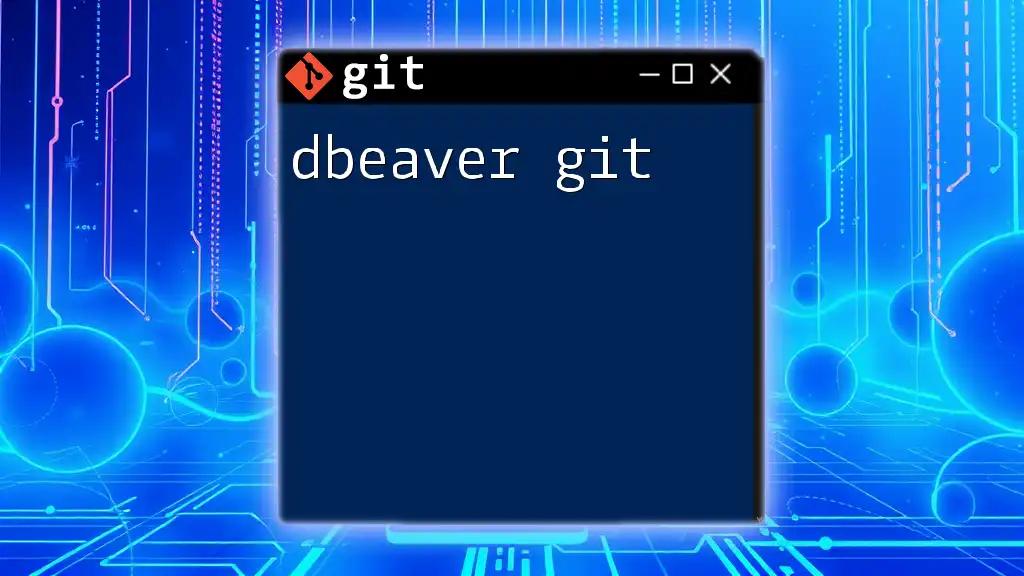
Working with Bazel and Git: A Step-by-Step Example
Creating a Bazel Project with Git
To start a new project:
Initialize Git Repository
Begin by creating a new directory for your project and initializing a Git repository:
mkdir my-bazel-project
cd my-bazel-project
git init
Creating a Basic Bazel BUILD File
Next, create a simple `BUILD` file to specify how the project is built. For instance:
cc_binary(
name = "hello",
srcs = ["hello.cc"],
)
This example defines a C++ binary target.
Committing Your Changes
After creating your `BUILD` file, you will want to commit your changes:
git add BUILD
git commit -m "Initial Bazel setup with hello binary"
Updating and Managing Dependencies
Using Git Submodules with Bazel
When your project relies on external repositories, consider using Git submodules. For example:
git submodule add <repo-url>
git submodule update --init --recursive
This approach ensures that your dependencies are tied to specific commits, improving stability.
Bazel’s Dependency Rules
Within your Bazel `BUILD` file, declare dependencies like so:
cc_library(
name = "my_lib",
srcs = ["lib.cc"],
deps = ["//path/to:dependency"],
)
This syntax specifies that `my_lib` depends on another target, allowing Bazel to manage these changes efficiently.
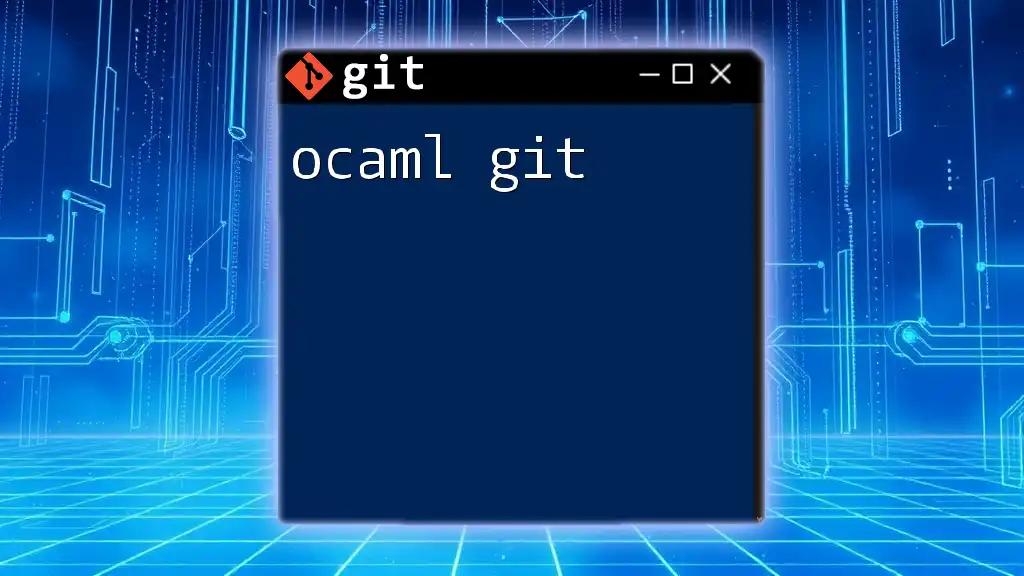
Advanced Integration Techniques
Custom Scripts for Automation
Automating your build process can be achieved by using Git hooks. For example, a post-commit hook can trigger Bazel to build project changes. Create a file in your `.git/hooks` directory named `post-commit`:
#!/bin/sh
bazel build //path/to:target
Make it executable:
chmod +x .git/hooks/post-commit
Now, every time you commit changes, Bazel will automatically build the updated targets.
Continuous Integration (CI) with Bazel and Git
Setting up Continuous Integration is essential for modern development practices. Use tools like GitHub Actions or Jenkins to automate your builds and tests upon Git events.
Here’s a basic configuration example for GitHub Actions:
name: CI
on:
push:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Bazel
run: |
curl -LO "https://github.com/bazelbuild/bazel/releases/download/x.y.z/bazel-x.y.z-linux-x86_64"
chmod +x bazel-x.y.z-linux-x86_64
sudo mv bazel-x.y.z-linux-x86_64 /usr/local/bin/bazel
- name: Run Bazel build
run: bazel build //path/to:target
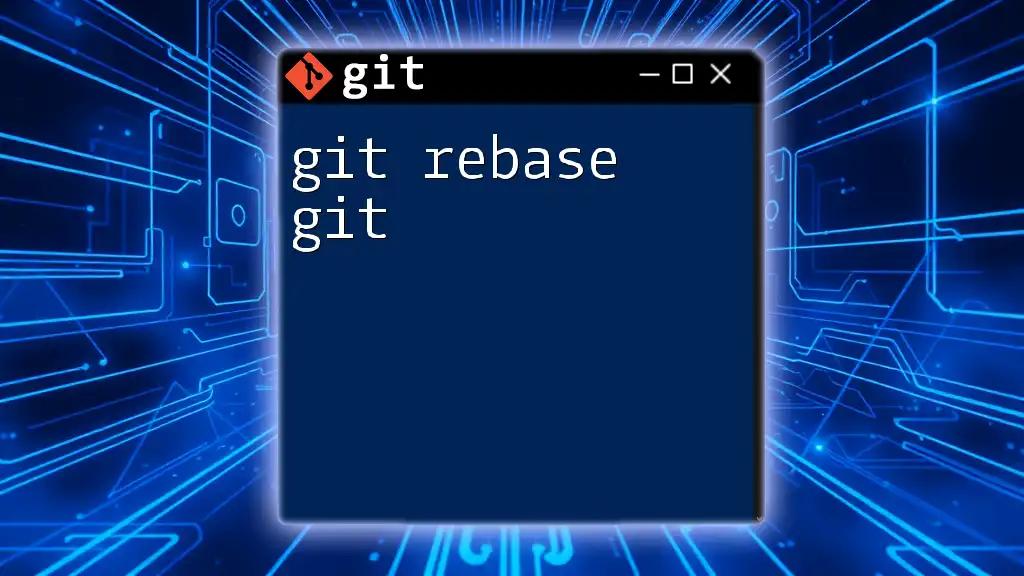
Common Issues and Troubleshooting
Troubleshooting Git Issues in Bazel Projects
While working on projects, you may encounter various issues, such as merge conflicts or broken builds after a pull. Familiarity with resolving merge conflicts in Git ensures a smoother workflow. Use the command:
git mergetool
This allows you to manually resolve conflicts.
Debugging Bazel Builds with Git Changes
If a build fails, it is essential to understand which changes caused the issue. The command `git blame <file>` can help you identify the last modification for each line, aiding in pinpointing recent changes that might have introduced errors.
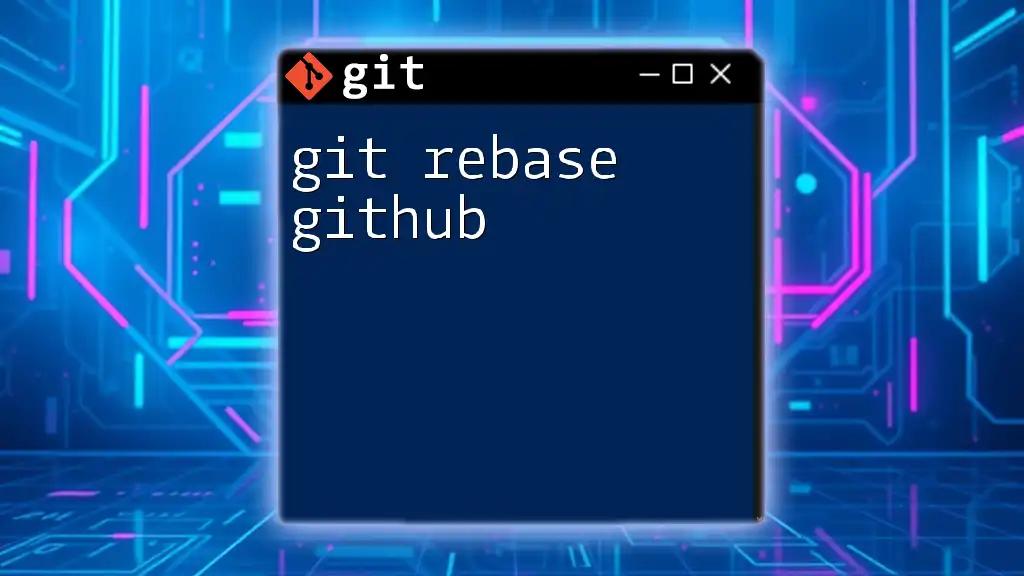
Conclusion
Integrating Bazel and Git offers powerful benefits for managing complex projects. With the right setup and adherence to best practices, teams can enjoy a streamlined workflow that enhances collaboration and productivity. Start experimenting with these tools today to experience the advantages firsthand!
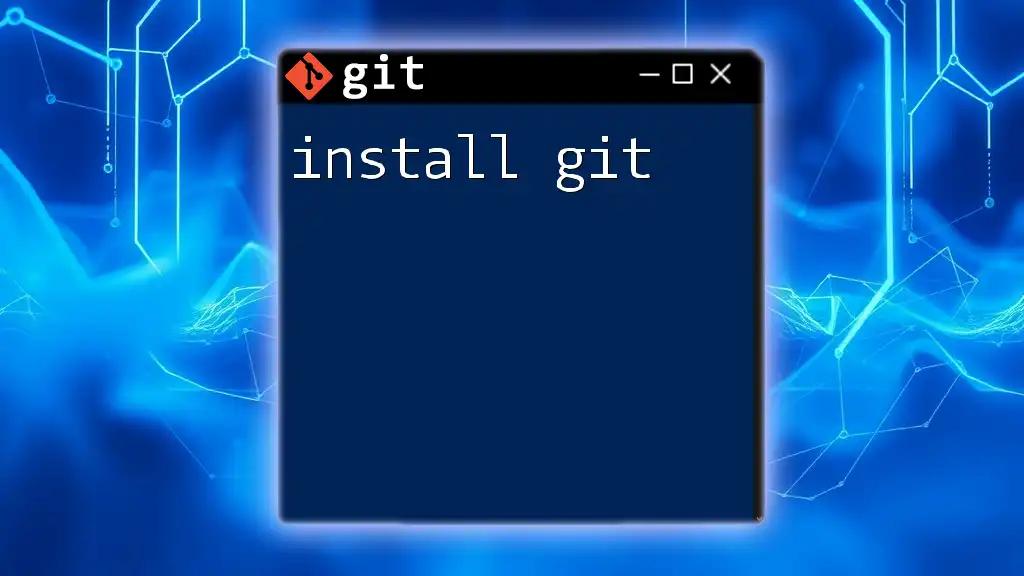
Additional Resources
For further learning, refer to the official documentation for both Bazel and Git. There are several excellent books and online courses available that provide in-depth insights into version control and build tools.